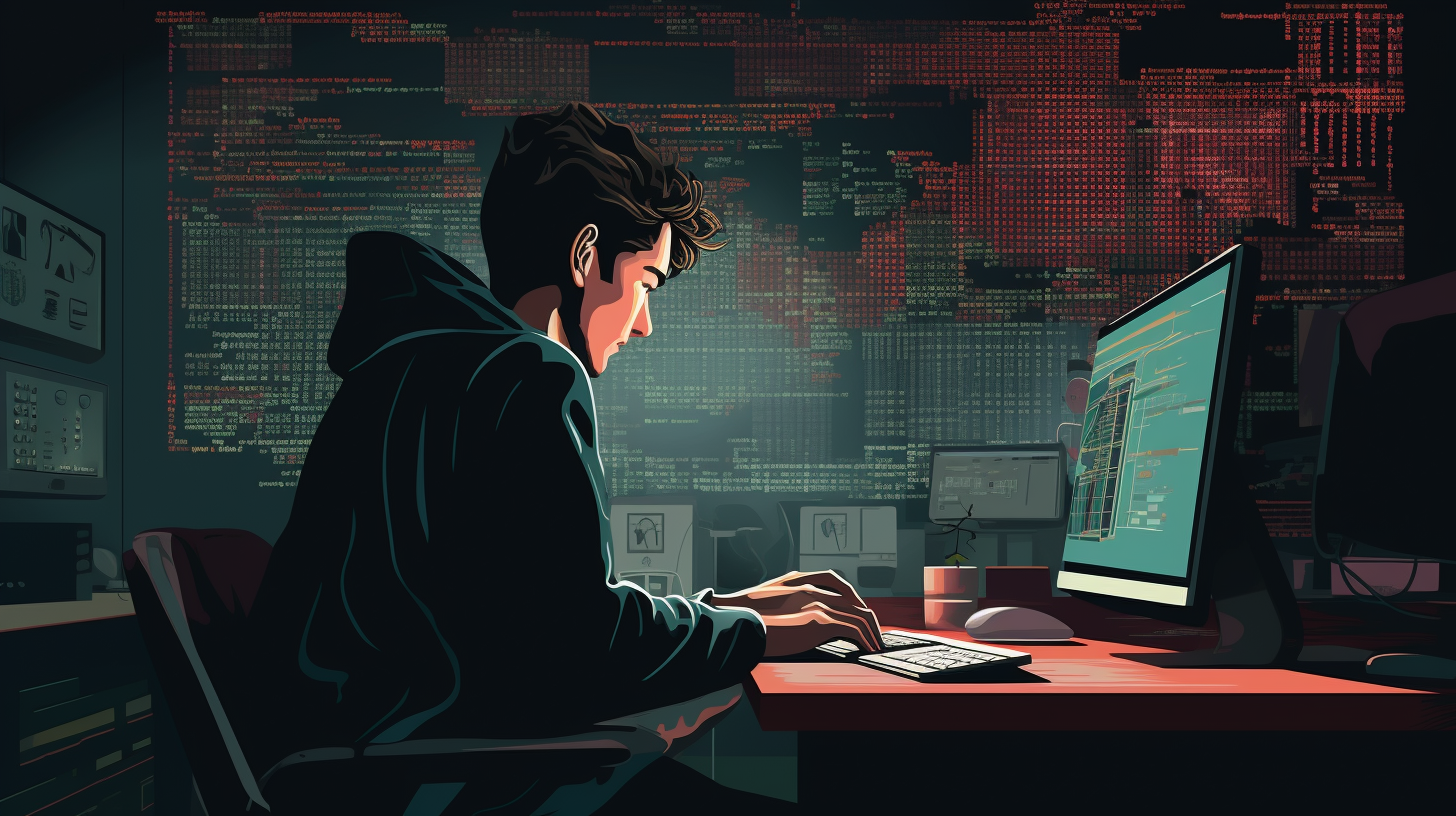
Python for Art and Design: Creative Coding
Within the scope of creativity, Python stands out not merely as a programming language but as a versatile canvas for artistic expression. Its simplicity and readability allow artists and designers to explore new dimensions of their work without the steep learning curve typically associated with programming. Through the lens of Python, the boundaries between art and code blur, inviting experimentation and innovation.
Creative coding represents a form of programming where the end goal is not to create efficient algorithms or data structures, but rather to produce something visually appealing or conceptually engaging. Python’s extensive libraries and frameworks serve as tools that transform abstract ideas into tangible creations. Artists can draw, animate, and even compose music—all through the power of code.
The intersection of Python and creativity is particularly evident in generative art, where algorithms generate complex patterns and visuals. Here, the artist acts more as a collaborator with the machine, setting parameters and letting the program explore possibilities. This collaborative spirit is encapsulated in the following Python example, which uses matplotlib
to create a simple generative pattern:
import numpy as np import matplotlib.pyplot as plt # Generate data x = np.linspace(0, 2 * np.pi, 100) y = np.sin(x) * np.cos(x) # Create plot plt.figure(figsize=(8, 4)) plt.plot(x, y, color='blue') plt.fill_between(x, y, color='lightblue', alpha=0.5) plt.title('Generative Pattern') plt.axis('off') # Turn off the axis plt.show()
This code snippet illustrates how a simple mathematical function can be leveraged to create visually striking graphics. Artists can modify the parameters to produce different variations, showcasing how code can lead to myriad outcomes.
Moreover, the use of Python in creative projects encourages a mindset of exploration. The artist’s role transforms into that of an experimenter, where failure is not an ending, but a stepping stone toward discovery. By pushing the limits of what is possible with Python, creators can uncover innovative techniques and methodologies that may not only enhance their artwork but also inspire others.
As we navigate this vibrant intersection of art and technology, it’s crucial to recognize that Python provides a gateway for artists. This gateway leads not just to new tools and techniques, but also to a deeper understanding of the creative process itself, where code becomes an integral part of the artist’s toolkit, enriching the experience of both creation and interaction.
Fundamentals of Creative Coding with Python
At the core of creative coding with Python lies an understanding of fundamental programming concepts that empower artists to manipulate digital elements freely. These concepts might initially appear daunting, but they are the building blocks that facilitate artistic expression through code. Each line of code represents a brushstroke, a step toward a finished piece, allowing for a deep connection between the artist and the medium.
Variables, loops, and functions form the foundation of any programming endeavor. In creative coding, these components allow for dynamic interactions with visual elements. Variables can represent colors, dimensions, or even the state of an animation, while loops can create repetitive patterns or animations, adding rhythm and movement to the artwork. Functions encapsulate reusable pieces of code, enabling artists to create complex structures without repeating themselves endlessly.
Think the following Python script that employs these fundamentals to generate a colorful spiral pattern using the turtle graphics library:
import turtle def draw_spiral(radius_increment, angle_increment, num_turns): turtle.speed(0) # Fastest drawing speed for i in range(num_turns * 360 // angle_increment): turtle.color(i % 256, (i * 2) % 256, (i * 3) % 256) # Changing colors turtle.circle(i * radius_increment) # Draw a circle with increasing radius turtle.right(angle_increment) turtle.colormode(255) # Enable RGB color mode draw_spiral(0.5, 10, 36) # Parameters for drawing turtle.done() # Finish drawing
This example demonstrates not just the use of loops and functions but also showcases the potential of color manipulation in art. As the spiral expands, the colors change dynamically, giving life to the piece—showing how code can translate into aesthetic experiences. By adjusting parameters such as radius increment and angle increment, artists can explore an infinite variety of spirals and patterns, each unique to the choices made in the code.
Moreover, the integration of randomness can introduce an element of surprise, echoing the spontaneity found in traditional forms of art. Python’s random library provides the tools to inject variability, leading to unexpected outcomes that can enhance the creative process. Here’s a brief example of how random elements can be incorporated into visual art:
import random import matplotlib.pyplot as plt # Create a scatter plot with random colors num_points = 100 x = [random.uniform(-10, 10) for _ in range(num_points)] y = [random.uniform(-10, 10) for _ in range(num_points)] colors = [random.random() for _ in range(num_points)] # Random colors plt.scatter(x, y, c=colors, alpha=0.5) plt.title('Random Scatter Plot') plt.axis('equal') # Equal scaling plt.show()
In this script, random coordinates and colors are generated for each point in the scatter plot, leading to a visually captivating arrangement. The unpredictable nature of randomness oftentimes mirrors the inherent unpredictability of creation, imbuing the artwork with a sense of playfulness.
Ultimately, mastering these fundamental concepts opens the door to a realm of creative possibilities. As artists familiarize themselves with the syntax and structure of Python, they develop an intuitive understanding of how to manipulate digital elements to achieve their artistic vision. With practice, the code becomes second nature, enabling artists to focus on the creative process rather than the intricacies of programming.
Visual Art and Design Libraries in Python
In the exciting world of visual arts and design, Python offers a plethora of libraries that empower artists and designers to translate their visions into reality. These libraries provide tools that enable manipulation of graphics and images, creation of animations, and even interaction with multimedia, all while maintaining the elegance of Python’s syntax. By using these resources, artists can craft engaging works that resonate with their audiences in ways that traditional mediums may not achieve.
One of the most notable libraries for visual art in Python is PIL (Python Imaging Library), which is now maintained under the name Pillow. This library allows for the opening, manipulating, and saving of many different image file formats. For instance, artists can create new images from scratch, apply effects, or modify existing images with ease. The following example demonstrates how to create a simple gradient image using Pillow:
from PIL import Image def create_gradient(width, height): # Create a new image with RGB mode img = Image.new('RGB', (width, height)) for x in range(width): for y in range(height): # Calculate color based on position r = int((x / width) * 255) # Red channel g = int((y / height) * 255) # Green channel img.putpixel((x, y), (r, g, 128)) # Blue channel fixed return img gradient_image = create_gradient(256, 256) gradient_image.show() # Display the image
This code snippet creates a gradient image that transitions from red to green, with a constant blue value. The artist can modify the logic in the loop to explore different color compositions, showcasing how the manipulation of pixel data opens up new avenues for creative expression.
In addition to Pillow, matplotlib continues to be an indispensable tool for artists, especially those interested in data visualization and generative design. Although primarily used for plotting data, its capabilities extend into the realm of artistic creation. Ponder generating a mesmerizing art piece by plotting a parametric equation:
import numpy as np import matplotlib.pyplot as plt # Define the parametric equations t = np.linspace(0, 2 * np.pi, 1000) x = np.sin(2 * t) * np.cos(3 * t) y = np.sin(3 * t) * np.cos(2 * t) # Create the plot plt.figure(figsize=(8, 8)) plt.plot(x, y, color='purple') # Use a vibrant color plt.fill(x, y, color='violet', alpha=0.3) # Fill with transparency plt.axis('off') # Hide axes plt.title('Parametric Art') plt.show()
This example highlights how mathematical functions can yield beautiful and intricate designs. By varying the parameters of the sine and cosine functions, artists can create a vast array of patterns, each unique to the choices made within the code. The ability to visualize complex mathematical relationships through art forms a bridge between science and creative expression.
Moreover, when it comes to interactive and 3D graphics, the Pygame library emerges as a fantastic resource. Although typically associated with game development, Pygame’s powerful capabilities allow artists to build interactive installations and visuals that respond to user input. Below is a simple example demonstrating how to create a colorful bouncing ball using Pygame:
import pygame import random # Initialize Pygame pygame.init() # Set up display width, height = 800, 600 screen = pygame.display.set_mode((width, height)) pygame.display.set_caption('Bouncing Ball') # Ball properties radius = 20 x, y = width // 2, height // 2 dx, dy = random.choice([-5, 5]), random.choice([-5, 5]) color = (255, 0, 0) # Red color # Main loop running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False # Move the ball x += dx y += dy # Bounce off the walls if x = width - radius: dx *= -1 color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255)) # Random color if y = height - radius: dy *= -1 color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255)) # Random color # Draw everything screen.fill((255, 255, 255)) # White background pygame.draw.circle(screen, color, (x, y), radius) pygame.display.flip() pygame.time.delay(30) pygame.quit()
This code illustrates a simple yet captivating interaction where the ball bounces around the window, changing colors upon collision with the edges. The dynamic nature of Pygame allows artists to create installations that are not only visually striking but also invite viewer interaction, thus fostering a participatory experience that engages the audience.
Through these libraries—Pillow, matplotlib, and Pygame—Python opens a world of possibilities for artists and designers alike. Each library provides unique functionalities that can be harnessed to create stunning visual works, engage audiences, and explore the uncharted territories of digital art. As the creative journey continues, the intersection of Python and visual arts becomes increasingly rich, offering endless opportunities for innovative expression.
Interactive Projects: Combining Art and Code
As we delve into the realm of interactive projects, we uncover the magic of combining art and code in ways that engage audiences and invite them to participate in the artistic experience. Interactivity breathes life into static artworks, transforming them into dynamic and responsive creations. Python, with its robust libraries and frameworks, serves as an ideal platform for developing these interactive experiences, allowing artists to create pieces that respond to user inputs, environmental changes, or even data streams.
One of the most compelling aspects of interactive art is its ability to foster a dialogue between the artwork and its audience. By allowing viewers to influence the piece through their actions, artists can create a more immersive experience that deepens emotional engagement. For instance, using the Pygame library, artists can develop projects that integrate user inputs in real-time. Below is an example of how to create a simple interactive drawing application using Pygame, where users can draw on the screen with their mouse:
import pygame # Initialize Pygame pygame.init() # Set up display width, height = 800, 600 screen = pygame.display.set_mode((width, height)) pygame.display.set_caption('Interactive Drawing') # Color definitions white = (255, 255, 255) black = (0, 0, 0) # Main loop running = True drawing = False while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if event.type == pygame.MOUSEBUTTONDOWN: drawing = True if event.type == pygame.MOUSEBUTTONUP: drawing = False if drawing: mouse_x, mouse_y = pygame.mouse.get_pos() pygame.draw.circle(screen, black, (mouse_x, mouse_y), 5) # Draw a circle at mouse position # Refresh the screen screen.fill(white) # Clear the screen with white pygame.display.flip() pygame.quit()
This example showcases a simple drawing application where users can click and drag their mouse to create art on the screen. The interactivity lies in the user’s ability to influence the artwork directly, making each creation unique to the individual artist. The user experience is enriched by the immediacy and spontaneity of the drawing process, which mirrors traditional artistic practices.
Further exploring interactivity, artists can also utilize libraries such as Processing.py, which allows for deeper engagement through interactive installations. By using visual and auditory stimuli that respond to movement—be it physical movement of viewers or environmental changes—artists can create a multisensory experience. The following example uses Processing.py to create a simple interactive sketch that changes colors based on mouse movement:
def setup(): size(800, 600) # Set window size def draw(): # Get mouse position mouseX = pmouseX mouseY = pmouseY # Map mouse position to color fill(mouseX % 255, mouseY % 255, (mouseX + mouseY) % 255) noStroke() # No outline ellipse(mouseX, mouseY, 50, 50) # Draw a circle at mouse position
In this sketch, as the user moves the mouse, the color of the circle changes dynamically, creating a playful interaction that invites exploration. This type of engagement not only captivates viewers but also encourages them to experiment with their own actions, leading to a deeper connection with the artwork.
Another intriguing approach to interactivity can be found in web-based applications using Flask or Django. By creating interactive web art installations, artists can reach broader audiences, allowing people from various geographic locations to participate. Below is a basic Flask application that captures mouse clicks on a webpage and displays them as points on a canvas:
from flask import Flask, render_template import random app = Flask(__name__) @app.route('/') def index(): return render_template('index.html') if __name__ == '__main__': app.run(debug=True)
In the accompanying HTML file, users can click to add points on a canvas, which can be visualized using JavaScript. This method opens vast avenues for collaborative art-making, where multiple users can contribute to a single piece in real-time. The combination of Flask for backend operations and JavaScript for frontend interactions allows for a powerful synergy between art and technology.
Ultimately, interactive projects represent a frontier where the realms of programming and creativity blend seamlessly. The choice of tools, from Pygame to Processing.py and Flask, empowers artists to push their boundaries and explore novel ways to communicate their vision. As we embrace this transformative power, the potential for artistic expression through interactivity becomes not just a possibility but a vibrant reality, inviting all to engage, create, and experience art like never before.
Case Studies: Artists Who Use Python in Their Work
In the contemporary landscape of digital art, a high number of artists have embraced Python as a tool for their creative endeavors, crafting remarkable works that illustrate the language’s versatility and power. These innovators span a variety of disciplines, each using the capabilities of Python to improve their artistic vision, create immersive experiences, and challenge conventional boundaries. By examining the practices of a few notable artists, we can gain insights into how Python can elevate artistic expression in compelling ways.
One prominent figure is Manfred Mohr, a pioneer in the field of generative art. Mohr’s work often reflects a deep understanding of algorithms and their role in the creative process. Using Python, he creates intricate visual compositions that evolve over time, driven by complex mathematical rules. For instance, his piece “P-511/D” employs generative algorithms to produce unique visual patterns that are never the same twice. By programming the parameters of the algorithms, Mohr allows chance and randomness to play an important role in the outcome, mirroring the unpredictability of nature itself.
Consider the following simplified example of a generative art piece inspired by Mohr’s techniques:
import numpy as np import matplotlib.pyplot as plt def generate_pattern(iterations): points = [] for i in range(iterations): angle = np.random.uniform(0, 2 * np.pi) # Random angle radius = np.random.uniform(0, 1) # Random radius x = radius * np.cos(angle) y = radius * np.sin(angle) points.append((x, y)) return points # Generate and plot the pattern pattern = generate_pattern(1000) x_vals, y_vals = zip(*pattern) plt.figure(figsize=(8, 8)) plt.scatter(x_vals, y_vals, s=1, color='purple', alpha=0.5) plt.title('Generative Pattern Inspired by Manfred Mohr') plt.axis('equal') plt.axis('off') plt.show()
This simple script generates random points in a polar coordinate system, showcasing the potential for generating complex and abstract visual forms through mathematical principles.
Another artist making waves in the Python art scene is Rafael Lozano-Hemmer, known for his interactive installations that engage the audience directly. His work often involves real-time data manipulation and visual feedback, creating an immersive environment that transforms viewers into active participants. One of his notable projects, “33 Questions per Minute,” uses natural language processing algorithms to explore the relationship between language and machine learning. By using Python’s extensive libraries for data analysis and visualization, Lozano-Hemmer blurs the lines between art, technology, and communication.
To illustrate the potential of Python in creating interactive experiences, consider the following example that captures user input to create a dynamic visual display:
import pygame import random # Initialize Pygame pygame.init() # Set up display width, height = 800, 600 screen = pygame.display.set_mode((width, height)) pygame.display.set_caption('Interactive Art - Click to Create') # Main loop running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if event.type == pygame.MOUSEBUTTONDOWN: mouse_x, mouse_y = event.pos color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255)) pygame.draw.circle(screen, color, (mouse_x, mouse_y), 20) pygame.display.flip() # Clean up pygame.quit()
This code enables users to click anywhere on the screen to create colorful circles, engaging them in the artistic process and allowing for unique, spontaneous creations. The randomness of color selection adds a layer of unpredictability, reflecting the essence of interactive art.
Additionally, the work of Casey Reas, co-founder of Processing and an influential figure in generative art, illustrates the potential of Python in combining programming with visual aesthetics. Reas’s projects often involve the exploration of systems and their outputs, using code to investigate the relationship between order and chaos. By incorporating Python into his practice, he expands the toolbox available to artists, encouraging experimentation and innovation.
For example, a simple simulation of particle behavior could reflect the principles Reas explores:
import matplotlib.pyplot as plt import numpy as np # Parameters num_particles = 100 positions = np.random.rand(num_particles, 2) # Random initial positions velocities = np.random.rand(num_particles, 2) * 0.01 # Small random velocities # Simulation loop for _ in range(100): positions += velocities # Update positions plt.scatter(positions[:, 0], positions[:, 1], s=10) plt.xlim(0, 1) plt.ylim(0, 1) plt.pause(0.05) plt.clf() # Clear the plot for the next frame plt.show()
This simulation visually captures the notion of emergence, where simple rules lead to complex behaviors—an idea central to Reas’s artistic philosophy. By employing Python, he demonstrates how code can serve as a medium for artistic exploration and expression.
Through the lens of these artists, we observe how Python becomes more than a programming language; it transforms into a medium of creativity that provides artists with the tools to explore new ideas, engage audiences, and redefine the boundaries of artistic expression. Each artist’s unique approach highlights the possibilities that arise when one combines code with creativity, revealing a rich tapestry of potential waiting to be discovered within the scope of digital art.