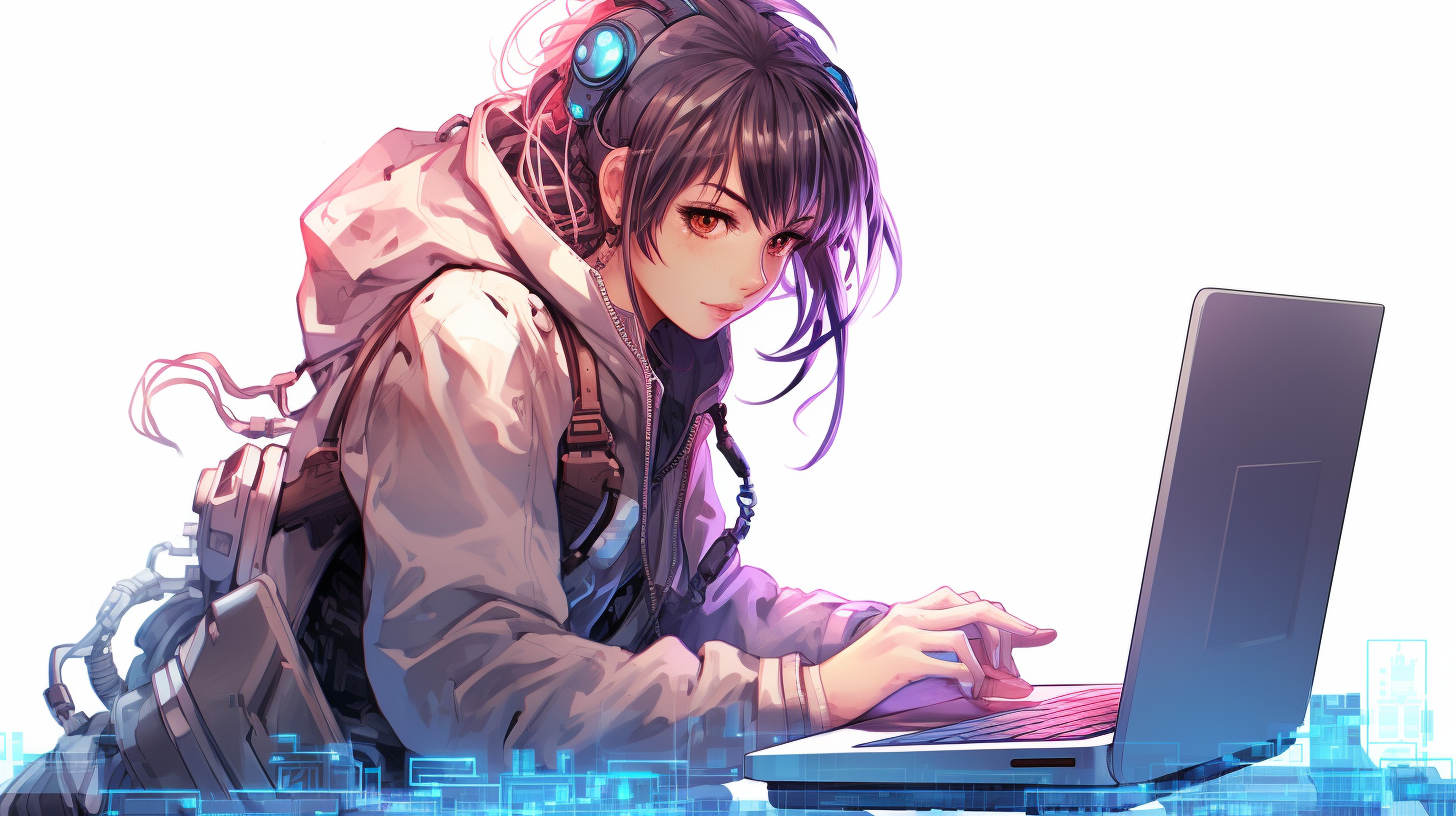
Python for Automotive Industry: Data Analysis and Automation
The automotive industry generates a colossal amount of data, from vehicle performance metrics to consumer behavior analytics. Here, Python plays an essential role in transforming this raw data into actionable insights. With its extensive libraries and frameworks, Python is perfectly suited for data analysis tasks, enabling engineers and analysts to harness the power of data in ways that were previously unimaginable.
One of the standout libraries in Python for data analysis is Pandas. This powerful tool provides data structures and functions to manipulate numerical tables and time series effectively. For automotive data analysis, where datasets can be large and complex, Pandas allows for efficient handling and processing of data.
import pandas as pd # Load a dataset containing vehicle performance data data = pd.read_csv('vehicle_performance.csv') # Display the first few rows of the dataset print(data.head()) # Calculate average fuel efficiency average_fuel_efficiency = data['fuel_efficiency'].mean() print(f'Average Fuel Efficiency: {average_fuel_efficiency} MPG')
In addition to Pandas, NumPy is another cornerstone of data analysis in Python. It provides a powerful array object that can perform complex mathematical operations efficiently. NumPy arrays facilitate the storage and manipulation of large datasets, which is invaluable when analyzing performance metrics across various vehicle models.
import numpy as np # Example array of fuel efficiency data for different models fuel_efficiencies = np.array([25.0, 30.5, 22.0, 28.0, 31.5]) # Calculate standard deviation of fuel efficiency std_dev = np.std(fuel_efficiencies) print(f'Standard Deviation of Fuel Efficiency: {std_dev} MPG')
For visualizing data, Matplotlib and Seaborn are invaluable. They allow for the creation of insightful graphs and plots that can help identify trends and patterns in the data. In the automotive sector, visualizations can be crucial for communicating complex data findings to stakeholders.
import matplotlib.pyplot as plt import seaborn as sns # Sample data for plotting models = ['Model A', 'Model B', 'Model C', 'Model D'] efficiency = [25, 30, 22, 28] # Create a bar plot for fuel efficiency plt.figure(figsize=(10, 6)) sns.barplot(x=models, y=efficiency) plt.title('Fuel Efficiency Comparison') plt.xlabel('Vehicle Models') plt.ylabel('Fuel Efficiency (MPG)') plt.show()
Furthermore, the use of Scikit-learn for machine learning applications within the automotive industry allows for predictive analytics. This can lead to improved vehicle designs, optimized manufacturing processes, and enhanced customer satisfaction through personalized offerings. By using algorithms for regression, classification, and clustering, automotive companies can derive insights that drive innovation.
from sklearn.linear_model import LinearRegression # Simple linear regression for predicting fuel efficiency based on weight X = data[['weight']] # Feature y = data['fuel_efficiency'] # Target model = LinearRegression() model.fit(X, y) predicted_efficiency = model.predict([[3000]]) # Predicting for a vehicle of 3000 lbs print(f'Predicted Fuel Efficiency for 3000 lbs: {predicted_efficiency[0]} MPG')
The applications of Python in automotive data analysis are extensive and growing. From data manipulation and statistical analysis to machine learning and visualization, Python stands out as a versatile tool that empowers automotive professionals to make data-driven decisions and foster innovation.
Using Python for Process Automation in Manufacturing
Within the scope of process automation within the automotive manufacturing sector, Python emerges as a critical enabler. The ability to automate repetitive tasks not only enhances productivity but also minimizes the risks of human error. Python’s simplicity and readability make it an excellent choice for automating various processes, from data entry to complex workflows involving multiple systems.
One of the key libraries that facilitate process automation in Python is the schedule library. This library allows users to easily schedule tasks and execute them at specific intervals, making it perfect for automating routine data collection or reporting tasks.
import schedule import time def job(): print("Collecting vehicle performance data...") # Schedule the job every hour schedule.every().hour.do(job) while True: schedule.run_pending() time.sleep(1)
In addition to scheduling tasks, Python can interact with various software applications through libraries such as pyautogui and selenium. These libraries allow Python to automate GUI interactions or web browser activities, which can be particularly useful in testing automotive applications or gathering data from web platforms.
import pyautogui # Example of automating data entry in an application pyautogui.click(x=100, y=200) # Click on the specified coordinates pyautogui.typewrite('Vehicle Model A', interval=0.1) # Type the vehicle model pyautogui.press('enter') # Press 'Enter'
Moreover, Python’s ability to interact with databases enhances its role in automating data management tasks. By using libraries such as SQLAlchemy or pandas, one can retrieve, manipulate, and store data seamlessly. This capability is essential for automotive manufacturers that need to maintain large volumes of data across different systems.
from sqlalchemy import create_engine import pandas as pd # Create a database connection engine = create_engine('sqlite:///automotive_data.db') # Sample query to retrieve vehicle data query = "SELECT * FROM vehicles WHERE year >= 2020" vehicles_data = pd.read_sql(query, engine) # Display the retrieved data print(vehicles_data.head())
Integrating Python with APIs enables manufacturers to automate data exchange between systems. That’s important for real-time data processing and synchronization across the supply chain. Using the requests library, developers can interact with RESTful APIs to pull in data or push updates efficiently.
import requests # Example of getting data from an API response = requests.get('https://api.automotive.com/v1/vehicles') vehicles = response.json() # Display vehicle data for vehicle in vehicles: print(vehicle['model'], vehicle['make'], vehicle['year'])
Lastly, the power of Python in process automation is magnified when combined with other tools like Jupyter Notebooks or Dash. These tools provide interactive environments where automotive engineers can visualize the results of automated processes in real-time, enhancing their ability to make informed decisions quickly.
import dash from dash import dcc, html app = dash.Dash(__name__) app.layout = html.Div([ dcc.Graph(id='fuel-efficiency-graph'), ]) if __name__ == '__main__': app.run_server(debug=True)
Using Python for process automation in the automotive manufacturing sector not only streamlines operations but also enhances data integrity and accessibility. By capitalizing on Python’s myriad libraries and frameworks, automotive professionals can ensure that their processes are efficient, reliable, and ready for the future of automotive innovation.
Case Studies: Successful Python Implementations in the Automotive Sector
In the automotive sector, numerous organizations have successfully implemented Python to tackle real-world challenges, showcasing its versatility and power. For instance, Tesla has utilized Python for various applications, including data analysis for vehicle performance and operational efficiency. By using libraries such as Pandas and NumPy, Tesla engineers can analyze large datasets from vehicle sensors, enabling them to fine-tune algorithms that enhance driving performance, predictive maintenance, and even battery management.
Think a situation where Tesla engineers need to analyze the battery performance across different driving conditions. They can easily process and visualize this data using Python, which helps in making informed decisions regarding battery technology improvements. The following code snippet demonstrates how they might load and analyze battery performance data:
import pandas as pd # Load battery performance data battery_data = pd.read_csv('battery_performance.csv') # Calculate average discharge rate average_discharge = battery_data['discharge_rate'].mean() print(f'Average Discharge Rate: {average_discharge} A
‘)
Another prominent case is Ford, which employs Python for predictive maintenance. By analyzing historical vehicle data, Ford anticipates potential failures, enabling proactive repairs. This isn’t merely an operational enhancement; it improves customer satisfaction by reducing unexpected breakdowns. Ford’s use of Scikit-learn for machine learning to predict vehicle maintenance needs is a testament to the integration of Python in real-world applications:
from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier # Features and labels for training the model X = battery_data[['mileage', 'age', 'previous_repairs']] # Features y = battery_data['needs_service'] # Labels # Split the dataset into training and test sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create and train the model model = RandomForestClassifier() model.fit(X_train, y_train) # Evaluate the model's accuracy accuracy = model.score(X_test, y_test) print(f'Model Accuracy: {accuracy * 100:.2f}%
‘)
Furthermore, General Motors (GM) has harnessed Python for data visualization and analysis in their supply chain management. By using libraries like Matplotlib and Seaborn, GM is able to visualize data trends that highlight inefficiencies or bottlenecks. This visual analysis aids in streamlining logistics and maximizing resource allocation. A simple example of how GM might analyze supply chain data is illustrated below:
import seaborn as sns import matplotlib.pyplot as plt # Sample data for supply chain deliveries delivery_data = pd.read_csv('supply_chain_deliveries.csv') # Create a line plot for deliveries over time plt.figure(figsize=(12, 6)) sns.lineplot(x='date', y='deliveries', data=delivery_data) plt.title('Deliveries Over Time') plt.xlabel('Date') plt.ylabel('Number of Deliveries') plt.xticks(rotation=45) plt.tight_layout() plt.show()
These case studies illustrate how Python is not just a tool but a cornerstone of innovation in the automotive industry. By analyzing data, automating processes, and enabling predictive maintenance, organizations like Tesla, Ford, and GM are using Python to enhance their operational capabilities and improve customer experiences. Each implementation further solidifies Python’s reputation as an indispensable asset in the drive toward automotive excellence.
Future Trends: The Role of Python in Automotive Innovation
The future of Python in the automotive industry is poised for transformation, driven by advancements in technology and data analytics. As the automotive landscape evolves with the integration of electric vehicles, autonomous driving, and smart connectivity, Python’s role will become increasingly central to innovation. Automotive manufacturers are already recognizing the need for tools that can efficiently process vast amounts of data and enable real-time decision-making, making Python an ideal candidate.
One key area where Python is anticipated to thrive is in the development of connected vehicle technologies. As vehicles become more integrated with the internet and other smart devices, the data generated will exponentially increase. Python’s extensive libraries for data handling, such as Pandas and Dask, make it highly suitable for processing and analyzing this data. For example, ponder a scenario where a fleet of vehicles collects telemetry data on driver behavior, vehicle performance, and environmental conditions. The analysis of this data can lead to improvements in safety features and fuel efficiency.
import pandas as pd # Load telemetry data from connected vehicles telemetry_data = pd.read_csv('telemetry_data.csv') # Perform analysis on driving behavior average_speed = telemetry_data['speed'].mean() print(f'Average Speed: {average_speed} mph') # Identify instances of aggressive driving aggressive_driving = telemetry_data[telemetry_data['acceleration'] > 5] print(f'Instances of Aggressive Driving: {len(aggressive_driving)}')
Additionally, the rise of electric vehicles (EVs) presents new challenges and opportunities for data analysis. Python’s machine learning libraries, such as TensorFlow and PyTorch, can be utilized to optimize battery management systems, improve range prediction algorithms, and enhance overall vehicle performance. By using predictive modeling, manufacturers can better forecast battery life and charging needs, ultimately leading to improved consumer satisfaction.
import tensorflow as tf from sklearn.model_selection import train_test_split # Sample data for battery performance battery_data = pd.read_csv('battery_data.csv') X = battery_data[['temperature', 'usage_pattern']] # Features y = battery_data['battery_life'] # Target # Splitting the data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Build a simple neural network model model = tf.keras.Sequential([ tf.keras.layers.Dense(64, activation='relu', input_shape=(X_train.shape[1],)), tf.keras.layers.Dense(1) ]) model.compile(optimizer='adam', loss='mean_squared_error') model.fit(X_train, y_train, epochs=50) # Evaluate the model loss = model.evaluate(X_test, y_test) print(f'Model Loss: {loss}
‘)
Moreover, the advancement of autonomous vehicles will heavily rely on machine learning and deep learning frameworks, with Python at the forefront. By using computer vision libraries like OpenCV, automotive engineers can develop systems that allow vehicles to interpret their surroundings, identify obstacles, and make real-time navigation decisions. This capability especially important for ensuring the safety and efficiency of self-driving cars.
import cv2 # Load an image from a vehicle's camera image = cv2.imread('road_image.jpg') # Convert the image to grayscale for easier processing gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # Use edge detection to identify obstacles edges = cv2.Canny(gray_image, 100, 200) # Display the results cv2.imshow('Edges', edges) cv2.waitKey(0) cv2.destroyAllWindows()
As the automotive industry embraces the Internet of Things (IoT), Python’s capabilities will extend to managing and analyzing data from a multitude of sensors embedded in vehicles. The use of Python with frameworks like Flask or FastAPI can facilitate the development of APIs that allow for seamless data communication between vehicles and cloud platforms, enabling smart data analysis and real-time processing.
from fastapi import FastAPI app = FastAPI() @app.get("/vehicle/data") async def read_vehicle_data(vehicle_id: int): return {"vehicle_id": vehicle_id, "status": "operational"} if __name__ == "__main__": import uvicorn uvicorn.run(app, host="0.0.0.0", port=8000)
The future of Python in the automotive industry is bright, characterized by its adaptability and the growing demand for data-driven solutions. As manufacturers strive to improve vehicle performance, safety, and customer satisfaction, Python will remain a vital tool in the development of innovative technologies that define the automotive landscape of tomorrow.