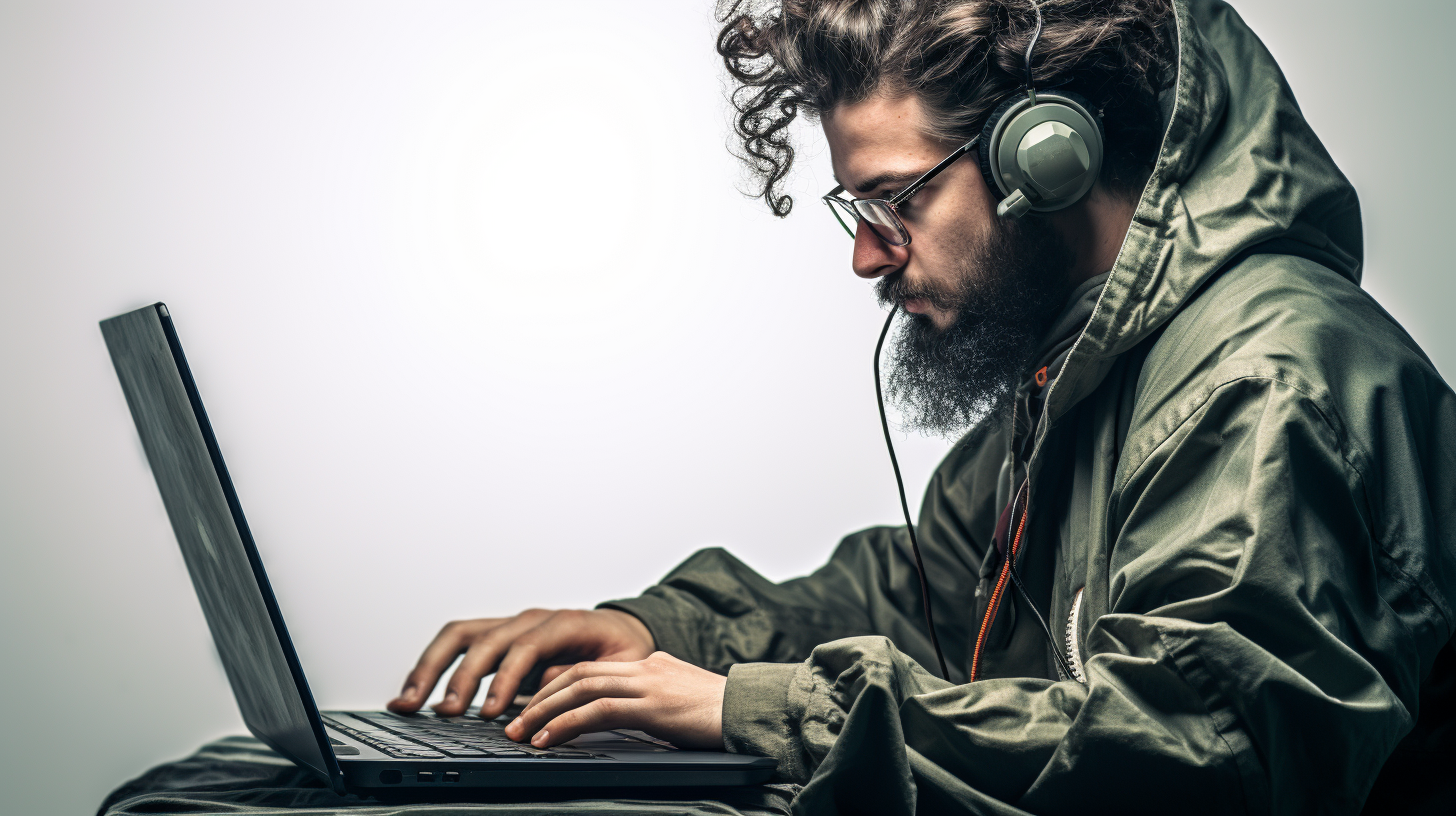
Python for Data Analysis: An Introduction
Python, famed for its simplicity and readability, serves as an excellent starting point for anyone venturing into the realm of programming, particularly in data analysis. The essence of Python lies in its syntax, which allows developers to express concepts in fewer lines of code than might be used in languages such as C++ or Java. This resultant conciseness not only enhances productivity but also fosters a clearer understanding of the code.
At its core, Python employs a simpler structure for defining variables, making it easy for newcomers to get up and running. For example, to define a variable and assign it a value, you would write:
x = 42
This simplicity extends to data types as well. Python supports various built-in data types, such as integers, floats, strings, and booleans. To check the type of a variable, the type()
function can be utilized:
print(type(x)) # Output:
Python’s control flow structures, including if statements, for loops, and while loops, further enhance its capacity for handling logic in a clear manner. Ponder the following example that demonstrates a simple loop:
for i in range(5): print(i)
The output of this snippet will be a sequence of numbers from 0 to 4, showcasing a fundamental use of iteration in Python.
Functions in Python allow for the encapsulation of reusable code. They can take arguments and return values, which very important for maintaining clean and organized code. Here’s how you can define a simple function:
def greet(name): return f"Hello, {name}!" print(greet("World")) # Output: Hello, World!
This function takes a single argument, name
, and returns a greeting string. Functions enable the modularization of code and are key in developing larger applications.
Another essential aspect of Python programming is its capability to handle collections of data, primarily through lists, tuples, and dictionaries. Lists are mutable sequences, allowing for dynamic data manipulation:
fruits = ["apple", "banana", "cherry"] fruits.append("date") print(fruits) # Output: ['apple', 'banana', 'cherry', 'date']
Tuples serve a similar purpose but are immutable, providing a way to store a collection of items that should not change. Dictionaries, on the other hand, are key-value pairs, offering a highly efficient way to store and access data:
person = {"name": "Alice", "age": 30} print(person["name"]) # Output: Alice
Understanding these basic constructs is fundamental in using Python for data analysis. They set the stage for employing Python’s extensive libraries tailored for data manipulation, visualization, and statistical analysis.
Essential Libraries for Data Analysis
To truly harness the power of Python for data analysis, one must become acquainted with a suite of essential libraries designed to streamline various tasks and enhance productivity. These libraries are the backbone of the Python data ecosystem, and each serves a unique purpose that simplifies common operations in data manipulation, statistical analysis, and visualization.
One of the most prominent libraries is Pandas, which provides data structures and functions specifically geared towards data analysis. With its intuitive DataFrame structure, handling structured data becomes effortless. A DataFrame is essentially a two-dimensional table where data is stored in rows and columns, reminiscent of a spreadsheet. Here’s a quick demonstration of how to create a DataFrame and perform basic operations:
import pandas as pd # Creating a DataFrame data = { 'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35], 'City': ['New York', 'Los Angeles', 'Chicago'] } df = pd.DataFrame(data) # Displaying the DataFrame print(df) # Accessing a specific column print(df['Age'])
In the above example, we construct a DataFrame from a dictionary, making it easy to visualize data and access specific columns. Pandas also provides numerous functions for data cleaning, merging, and aggregating, making it an indispensable tool in any data analyst’s toolkit.
Another powerful library is NumPy, which is primarily utilized for numerical computations. NumPy introduces the ndarray, a fast and flexible data structure that can handle large datasets. Operations on ndarrays are optimized for performance, allowing for efficient computation. Below is an example showcasing some basic operations with NumPy:
import numpy as np # Creating a NumPy array arr = np.array([1, 2, 3, 4, 5]) # Basic operations print(arr + 10) # Adding 10 to each element print(arr * 2) # Multiplying each element by 2 print(np.mean(arr)) # Calculating the mean
NumPy not only allows for basic arithmetic operations but also provides a wealth of linear algebra functions, random number generation, and tools for integrating with C/C++ code, which can be crucial for high-performance computing tasks.
For those venturing into data visualization, the Matplotlib library is a must. It offers a robust framework for creating static, animated, and interactive visualizations in Python. With Matplotlib, one can generate plots, histograms, and scatter plots with relative ease. Here’s an example that demonstrates how to create a simple line plot:
import matplotlib.pyplot as plt # Sample data x = [1, 2, 3, 4, 5] y = [2, 3, 5, 7, 11] # Creating a line plot plt.plot(x, y, marker='o') plt.title('Sample Line Plot') plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.grid() plt.show()
The aforementioned libraries—Pandas, NumPy, and Matplotlib—form the foundation of Python’s data analysis capabilities. They empower analysts to tackle complex datasets with ease, enabling them to extract insights and visualize data effectively. Beyond these, there are numerous other libraries such as Seaborn for statistical graphics and Scikit-learn for machine learning, which further expand Python’s prowess in the data space.
Understanding and effectively using these libraries is essential for anyone looking to make a significant impact in the field of data analysis with Python. As you delve deeper into these tools, you’ll uncover their myriad functionalities that can elevate your analytical capabilities to new heights.
Data Manipulation and Cleaning Techniques
Pandas is perhaps the most crucial library for data manipulation and cleaning. It provides an array of functions to handle missing data, which is a common issue when working with real-world datasets. Missing values can skew analysis and lead to incorrect conclusions. Pandas makes it easy to identify and handle these gaps. You can drop rows or columns with missing values using the dropna() method or fill them in using fillna(). Here’s an example:
import pandas as pd # Creating a DataFrame with missing values data = { 'Name': ['Alice', 'Bob', None], 'Age': [25, None, 35], 'City': ['New York', 'Los Angeles', None] } df = pd.DataFrame(data) # Displaying the DataFrame print("Original DataFrame:") print(df) # Dropping rows with missing values df_dropped = df.dropna() print("nDataFrame after dropping missing values:") print(df_dropped) # Filling missing values with a default value df_filled = df.fillna({'Name': 'Unknown', 'Age': 0, 'City': 'Unknown'}) print("nDataFrame after filling missing values:") print(df_filled)
In this example, we first create a DataFrame containing missing values (None). We then demonstrate two strategies for managing these gaps: dropping rows with missing values and filling them with specified defaults. Both approaches are instrumental in maintaining data integrity.
Moreover, data types can often be inconsistent, particularly when merging datasets from various sources. Pandas allows you to convert data types conveniently. The astype() method is invaluable in this context. For instance, you might need to convert a column of numbers stored as strings into integers:
# Creating a DataFrame with string numbers data = { 'Name': ['Alice', 'Bob', 'Charlie'], 'Age': ['25', '30', '35'] } df = pd.DataFrame(data) # Converting the Age column to integers df['Age'] = df['Age'].astype(int) print("nDataFrame after converting Age to integers:") print(df)
With this conversion, we ensure that operations performed on the Age column are mathematical and not string concatenations, which would lead to errors.
Data aggregation is another powerful feature of Pandas that allows you to summarize data based on certain criteria. The groupby() method is particularly useful for this task. For example, if you want to calculate the average age of users grouped by city, you can do so with a simple command:
# Sample DataFrame data = { 'Name': ['Alice', 'Bob', 'Charlie', 'David'], 'Age': [25, 30, 35, 30], 'City': ['New York', 'Los Angeles', 'New York', 'Los Angeles'] } df = pd.DataFrame(data) # Grouping by City and calculating the average age average_age = df.groupby('City')['Age'].mean() print("nAverage age by city:") print(average_age)
This code groups the DataFrame by the ‘City’ column and computes the mean age for each group, yielding insights into demographic distributions.
Lastly, data visualization often plays a pivotal role in data analysis, as it helps in understanding trends and patterns. While Matplotlib serves the purpose, Seaborn builds on it by providing a high-level interface for drawing attractive statistical graphics. For instance, creating a box plot to visualize the distribution of ages across different cities can be done succinctly:
import seaborn as sns import matplotlib.pyplot as plt # Sample DataFrame data = { 'Name': ['Alice', 'Bob', 'Charlie', 'David'], 'Age': [25, 30, 35, 30], 'City': ['New York', 'Los Angeles', 'New York', 'Los Angeles'] } df = pd.DataFrame(data) # Creating a box plot sns.boxplot(x='City', y='Age', data=df) plt.title('Age Distribution by City') plt.show()
This snippet illustrates how easily one can visualize data distributions using Seaborn, providing clear insights into variations within the dataset.
Through the combination of these powerful data manipulation techniques and libraries, Python becomes an indispensable tool for any data analyst. As you engage with data, mastering these tools will facilitate not only efficient data cleaning and manipulation but also enhance your analytical capabilities.
Visualizing Data with Python
Visualizing data is an important aspect of data analysis that empowers analysts to uncover insights, identify trends, and communicate findings effectively. Python, with its rich ecosystem of libraries, offers powerful tools for creating a wide range of visualizations, enabling users to represent data graphically. Among the most popular libraries for this purpose are Matplotlib and Seaborn, each providing unique functionalities and aesthetic enhancements.
Matplotlib serves as the foundational library for plotting in Python. It offers a versatile platform for generating a variety of static, animated, and interactive plots. The library’s flexibility allows for the customization of a high number of plot features, from markers to colors, making it a go-to choice for many data analysts. To illustrate its use, think the following example that demonstrates how to create a scatter plot with Matplotlib:
import matplotlib.pyplot as plt # Sample data x = [1, 2, 3, 4, 5] y = [2, 3, 5, 7, 11] # Creating a scatter plot plt.scatter(x, y, color='blue', marker='o') plt.title('Sample Scatter Plot') plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.grid() plt.show()
In this example, we first define two lists, x and y, representing the coordinates of the points. The plt.scatter() function is used to generate the scatter plot, with additional parameters for customizing the appearance. The result is a visual representation that helps to quickly assess the relationship between the two variables.
While Matplotlib provides the basic plotting capabilities, Seaborn enhances these with a higher-level interface that simplifies the creation of complex visualizations. Built on top of Matplotlib, Seaborn offers built-in themes and color palettes, making it easier to produce aesthetically pleasing graphics. For instance, if we want to create a violin plot to visualize the distribution of data points across different categories, Seaborn simplifies the process significantly:
import seaborn as sns import matplotlib.pyplot as plt # Sample DataFrame data = { 'Category': ['A', 'A', 'B', 'B', 'C', 'C', 'C'], 'Values': [1, 2, 3, 4, 5, 1, 2] } df = pd.DataFrame(data) # Creating a violin plot sns.violinplot(x='Category', y='Values', data=df) plt.title('Violin Plot of Values by Category') plt.show()
This snippet demonstrates how easy it is to create a violin plot with Seaborn, providing a clear view of the distribution of ‘Values’ for each ‘Category’. The plot not only shows the data’s distribution but also its probability density, offering deeper insights beyond traditional box plots.
Moreover, when analyzing time-series data, Python’s visual capabilities shine through. Matplotlib excels in plotting time series, allowing analysts to visualize trends over time effectively. Ponder the following example:
import pandas as pd import matplotlib.pyplot as plt # Sample time series data dates = pd.date_range(start='2023-01-01', periods=10) values = [1, 3, 2, 5, 4, 6, 5, 8, 7, 9] df = pd.DataFrame({'Date': dates, 'Values': values}) # Plotting the time series plt.plot(df['Date'], df['Values'], marker='o') plt.title('Time Series Data') plt.xlabel('Date') plt.ylabel('Values') plt.xticks(rotation=45) plt.grid() plt.show()
In this example, we generate a series of dates and corresponding values, and then visualize them with a line plot. The rotation of the x-axis labels enhances readability, especially when dealing with dense time-series data.
As you delve further into Python’s visualization capabilities, you will discover that combining different plots can lead to richer storytelling. Overlaying multiple plots, adding annotations, and using various color schemes can enhance the interpretability of the data. For instance, combining scatter and line plots can illustrate trends while highlighting individual data points:
plt.plot(x, y, label='Trend Line', color='red') plt.scatter(x, y, label='Data Points', color='blue') plt.title('Combined Scatter and Line Plot') plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.legend() plt.grid() plt.show()
By employing these visualization techniques, analysts can not only interpret complex data but also communicate their findings more effectively to stakeholders. Data visualization in Python is not just about plotting; it is about crafting narratives that engage the audience and foster understanding. As you become familiar with these libraries and techniques, you’ll find that the ability to visualize data is one of the most powerful skills in the data analyst’s toolkit.