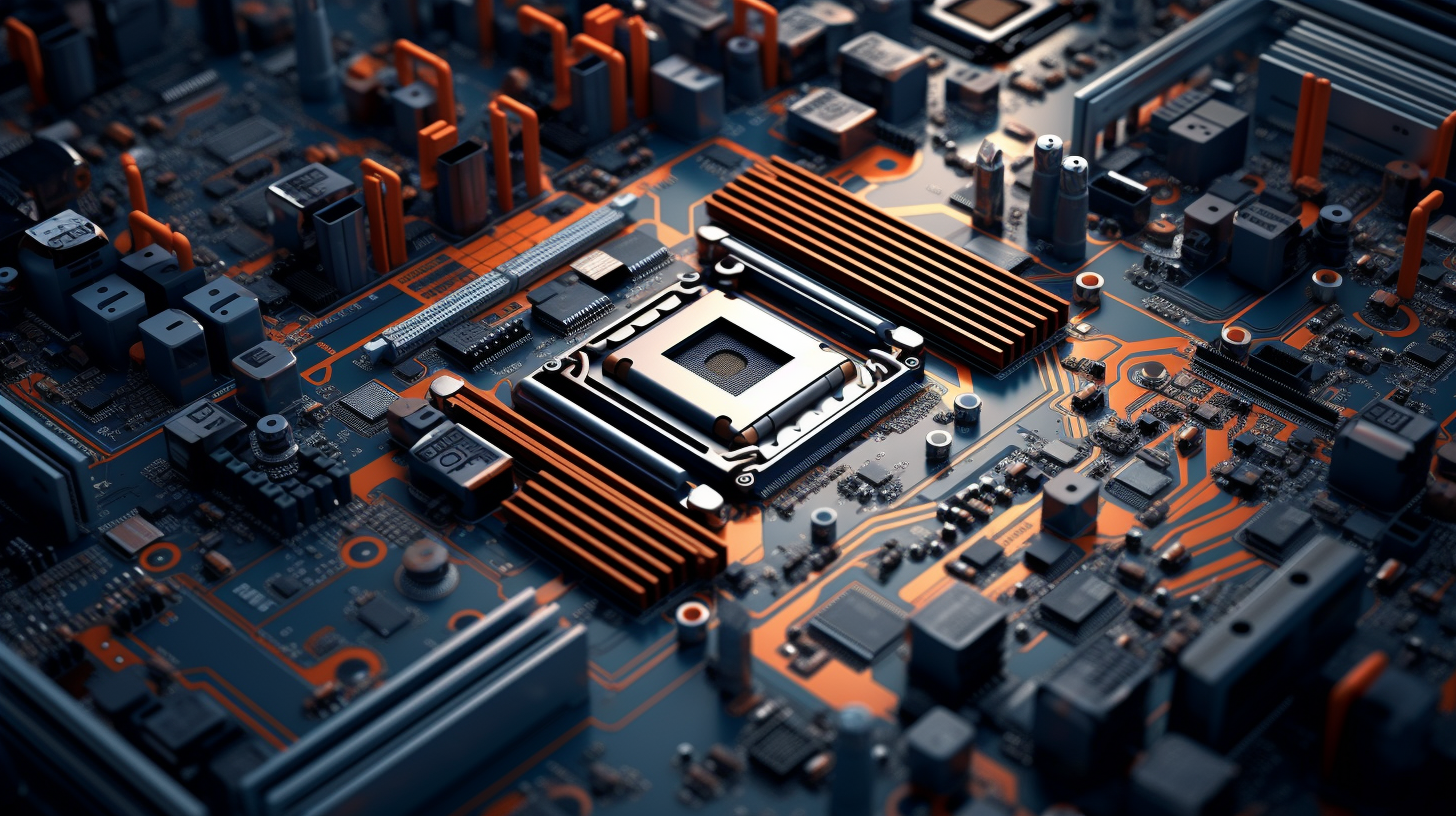
Python for Environmental Science: Analysis and Modeling
The integration of Python into environmental data analysis has transformed the way researchers approach complex datasets. Its versatility and ease of use allow scientists to manipulate and analyze large volumes of data efficiently. With a rich ecosystem of libraries designed specifically for data analysis and manipulation, Python equips environmental scientists with the tools they need to derive meaningful insights from their data.
Pandas is one of the most prominent libraries in this domain. It provides data structures like DataFrame
that are ideal for handling structured data. For instance, environmental scientists can easily read, manipulate, and analyze CSV files containing climate data. Ponder the example below where we read a CSV file containing temperature data and calculate some basic statistics:
import pandas as pd # Load temperature data data = pd.read_csv('temperature_data.csv') # Display the first few rows of the DataFrame print(data.head()) # Calculate basic statistics mean_temp = data['temperature'].mean() std_temp = data['temperature'].std() print(f'Mean Temperature: {mean_temp:.2f}') print(f'Standard Deviation: {std_temp:.2f}')
Another powerful library is NumPy, which allows for efficient numerical calculations. It is particularly useful in environmental modeling where large arrays or matrices of data are common. For example, if we want to compute the yearly average of monthly precipitation data stored in a NumPy array, we could do the following:
import numpy as np # Monthly precipitation data for a year monthly_precipitation = np.array([70, 85, 90, 100, 120, 150, 200, 180, 130, 90, 60, 50]) # Calculate the yearly average precipitation yearly_average = np.mean(monthly_precipitation) print(f'Yearly Average Precipitation: {yearly_average:.2f} mm')
Moreover, Python’s Matplotlib and Seaborn libraries offer robust data visualization capabilities. Visualizations are crucial in environmental science as they help communicate findings effectively. Here’s a simple example of how to plot the monthly precipitation using Matplotlib:
import matplotlib.pyplot as plt # Months and corresponding precipitation months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'] precipitation = monthly_precipitation # Create a bar plot plt.bar(months, precipitation, color='blue') plt.title('Monthly Precipitation') plt.xlabel('Months') plt.ylabel('Precipitation (mm)') plt.show()
In addition to these libraries, Python’s scikit-learn library can be leveraged for statistical modeling and machine learning, allowing scientists to apply various predictive models to their environmental datasets. For example, a linear regression model can be used to predict future temperature trends based on historical data:
from sklearn.linear_model import LinearRegression # Sample historical temperature data (years and corresponding average temperature) years = np.array([[2000], [2001], [2002], [2003], [2004]]) temperatures = np.array([15.1, 15.6, 16.0, 16.5, 17.0]) # Create a linear regression model model = LinearRegression() model.fit(years, temperatures) # Predict future temperatures future_years = np.array([[2005], [2006], [2007]]) predictions = model.predict(future_years) print(f'Predicted Temperatures for 2005, 2006, 2007: {predictions}')
Ultimately, the power of Python in environmental data analysis lies in its ability to combine various libraries seamlessly, providing a comprehensive toolkit for researchers. The efficiency and clarity that Python brings to data handling, statistical modeling, and visualization make it an indispensable resource in the field of environmental science.
Modeling Ecosystems and Biodiversity with Python
Modeling ecosystems and biodiversity is a complex task that requires not only a firm understanding of ecological principles but also the ability to analyze and interpret large datasets. Python offers a rich suite of libraries that empower researchers to create detailed models of ecosystems, enabling them to simulate interactions within biodiversity and predict changes over time.
One of the key libraries used for ecological modeling in Python is PyEcoLib, which provides tools for simulating ecological processes. This library helps researchers model populations, species interactions, and ecosystem dynamics. For example, think a simple Lotka-Volterra model which describes the dynamics of predator-prey relationships. Below is an implementation using the NumPy library:
import numpy as np import matplotlib.pyplot as plt # Lotka-Volterra Parameters alpha = 0.1 # Growth rate of prey beta = 0.02 # Rate of predation delta = 0.01 # Growth rate of predators gamma = 0.1 # Mortality rate of predators # Time parameters time = np.linspace(0, 200, num=1000) # Initial populations prey = 40 predators = 9 # Lists to hold population values prey_pop = [] predator_pop = [] # Lotka-Volterra equations for t in time: prey = prey + (alpha * prey - beta * prey * predators) predators = predators + (delta * prey * predators - gamma * predators) prey_pop.append(prey) predator_pop.append(predators) # Plotting results plt.figure(figsize=(10, 5)) plt.plot(time, prey_pop, label='Prey Population', color='blue') plt.plot(time, predator_pop, label='Predator Population', color='red') plt.title('Lotka-Volterra Model') plt.xlabel('Time') plt.ylabel('Population') plt.legend() plt.grid() plt.show()
This model demonstrates the cyclical nature of prey and predator populations over time. By adjusting parameters such as growth rates and mortality, researchers can explore various scenarios and their impacts on biodiversity.
Another important library for modeling ecosystems is BioPython, which is specifically designed for biological computation. It provides tools for analyzing biological data, including sequence analysis and structural biology. For example, researchers can analyze genetic diversity within populations using BioPython’s capabilities. Below is an example of how to calculate nucleotide diversity from a sequence alignment:
from Bio import AlignIO from Bio.Seq import Seq # Load sequence alignment alignment = AlignIO.read("sequence_alignment.fasta", "fasta") # Calculate nucleotide diversity def nucleotide_diversity(alignment): num_sequences = len(alignment) seq_length = alignment.get_alignment_length() diversity_count = 0 for i in range(seq_length): column = alignment[:, i] if len(set(column)) > 1: # More than one type of nucleotide diversity_count += 1 return diversity_count / seq_length diversity = nucleotide_diversity(alignment) print(f'Nucleotide Diversity: {diversity:.4f}')
In this example, the function calculates the proportion of variable sites in the sequence alignment, giving a measure of genetic diversity within a population.
Combining these modeling capabilities with data processing libraries, researchers can create comprehensive models that incorporate various ecological factors. For instance, if we aim to model species distribution based on environmental variables, we could utilize the scikit-learn library to perform a logistic regression analysis. Below is a basic example of how environmental predictors can influence species presence:
from sklearn.linear_model import LogisticRegression # Environmental variables (e.g., temperature, precipitation) X = np.array([[22, 150], [25, 200], [30, 180], [35, 100], [20, 60]]) # Species presence (1 for presence, 0 for absence) y = np.array([1, 1, 0, 0, 1]) # Create and fit the model model = LogisticRegression() model.fit(X, y) # Predict species presence for new environmental conditions new_conditions = np.array([[28, 170], [32, 80]]) predictions = model.predict(new_conditions) print(f'Predictions for new conditions: {predictions}')
This logistic regression model allows for the assessment of how changes in environmental conditions can affect species presence, which is critical for conservation efforts and biodiversity management.
In essence, the power of Python in modeling ecosystems and biodiversity lies in its flexibility and the integration of various libraries that cater to specific needs. By enabling researchers to simulate complex ecological interactions and analyze biological data effectively, Python stands as a cornerstone in ecological research and biodiversity conservation.
Data Visualization Techniques for Environmental Studies
Data visualization is a fundamental aspect of environmental science that allows researchers to interpret and communicate complex data more effectively. Python, with its rich set of libraries, provides powerful tools for creating informative and visually appealing plots. Effective data visualization can reveal patterns, trends, and outliers in data that may go unnoticed in raw datasets, thus facilitating better decision-making in environmental management and policy.
Among the most popular libraries for data visualization in Python are Matplotlib and Seaborn. While Matplotlib serves as the foundation for creating static, animated, and interactive visualizations, Seaborn builds on Matplotlib’s foundation to simplify the creation of more aesthetically pleasing statistical graphics.
Let’s start with Matplotlib. This library is highly customizable and allows for the creation of a wide range of plots. Below is an example of how to visualize air quality data, showcasing the relationship between air pollutants over time.
import matplotlib.pyplot as plt import pandas as pd # Load air quality data data = pd.read_csv('air_quality_data.csv') # Convert date column to datetime format data['date'] = pd.to_datetime(data['date']) # Plotting the concentrations of different pollutants plt.figure(figsize=(12, 6)) plt.plot(data['date'], data['CO'], label='Carbon Monoxide (CO)', color='blue') plt.plot(data['date'], data['NO2'], label='Nitrogen Dioxide (NO2)', color='red') plt.plot(data['date'], data['PM10'], label='Particulate Matter (PM10)', color='green') plt.title('Air Quality Over Time') plt.xlabel('Date') plt.ylabel('Concentration (µg/m³)') plt.legend() plt.grid() plt.xticks(rotation=45) plt.tight_layout() plt.show()
This code reads a CSV file containing air quality data, then plots the concentrations of different pollutants over time. The resulting graph allows researchers to quickly identify trends and fluctuations in air quality.
Seaborn, on the other hand, simplifies the process of creating attractive visualizations and supports more complex statistical plots. For instance, it integrates well with Pandas DataFrames and can produce heatmaps, violin plots, and pair plots with minimal code. Here’s an example of how to visualize the correlation between various environmental variables using Seaborn:
import seaborn as sns # Load a dataset containing environmental variables data = pd.read_csv('environmental_variables.csv') # Create a heatmap to visualize the correlation matrix plt.figure(figsize=(10, 8)) correlation_matrix = data.corr() sns.heatmap(correlation_matrix, annot=True, cmap='coolwarm', fmt='.2f', square=True) plt.title('Correlation Heatmap of Environmental Variables') plt.show()
This code generates a heatmap that visualizes the correlation coefficients between various environmental variables, allowing researchers to spot relationships and potentially significant predictors for further analysis.
Another essential visualization library is Plotly, which enables the creation of interactive graphs. Interactivity can be particularly useful in environmental science, where users may want to explore the data dynamically. Here’s an example of how to create an interactive scatter plot using Plotly:
import plotly.express as px # Load a dataset containing species observations data = pd.read_csv('species_observations.csv') # Create an interactive scatter plot for species distribution fig = px.scatter(data, x='longitude', y='latitude', color='species', title='Species Distribution Across Locations', labels={'longitude': 'Longitude', 'latitude': 'Latitude'}) fig.show()
In this example, the scatter plot illustrates the distribution of different species based on their geographical coordinates. The inclusion of interactive features allows users to hover over points to see more information, enhancing data exploration.
Effective data visualization not only aids in analysis but also plays a critical role in communicating findings to stakeholders, policymakers, and the general public. By using Python’s visualization libraries, researchers can create compelling visual narratives that foster understanding and awareness of environmental issues.
Machine Learning Approaches for Environmental Predictions
The application of machine learning in environmental predictions harnesses the power of data-driven algorithms to uncover patterns and trends within complex datasets. Python’s wide array of machine learning libraries facilitates this analysis, enabling environmental scientists to make informed predictions about phenomena such as climate change, pollution impacts, and biodiversity shifts.
One of the foundational libraries for machine learning in Python is scikit-learn, which provides a simple and efficient toolkit for data mining and data analysis. By employing various supervised and unsupervised learning algorithms, researchers can build predictive models that aid in environmental forecasting.
For instance, let’s think a scenario where we want to predict air quality based on various environmental factors such as temperature, humidity, and wind speed. A regression model could be applied to forecast pollutant levels. Here’s how one might implement a linear regression model using scikit-learn:
from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression import pandas as pd # Load air quality data data = pd.read_csv('air_quality_data.csv') # Features and target variable X = data[['temperature', 'humidity', 'wind_speed']] y = data['pollutant_level'] # Split the data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create and train the model model = LinearRegression() model.fit(X_train, y_train) # Make predictions predictions = model.predict(X_test) # Display the predictions print(predictions)
This example illustrates how to prepare data, train a model, and make predictions. The model is trained on a subset of data and then evaluated on unseen data to ensure its reliability. By adjusting the features or experimenting with different models, researchers can improve prediction accuracy.
Another powerful approach in environmental predictions is employing decision trees or ensemble methods such as Random Forests. These methods excel in managing non-linear relationships and interactions between variables, making them suitable for complex environmental datasets. Below is an example using the Random Forest algorithm to predict species distribution based on environmental conditions:
from sklearn.ensemble import RandomForestClassifier # Features and target variable X = data[['temperature', 'precipitation', 'elevation']] y = data['species_presence'] # Split the data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42) # Create and fit the Random Forest model rf_model = RandomForestClassifier(n_estimators=100) rf_model.fit(X_train, y_train) # Make predictions species_predictions = rf_model.predict(X_test) # Display the predictions print(species_predictions)
Random Forests aggregate the results of multiple decision trees, improving the robustness of predictions. This method is particularly beneficial in ecological studies where interactions among variables can be pivotal.
In addition to regression and classification techniques, clustering algorithms can be utilized to identify patterns within environmental data. For instance, k-means clustering can help categorize regions based on similar environmental characteristics, enabling targeted conservation efforts:
from sklearn.cluster import KMeans # Load environmental data data = pd.read_csv('environmental_data.csv') # Select relevant features for clustering X = data[['temperature', 'precipitation', 'humidity']] # Apply k-means clustering kmeans = KMeans(n_clusters=3) data['cluster'] = kmeans.fit_predict(X) # Display the cluster assignments print(data[['temperature', 'precipitation', 'humidity', 'cluster']])
This example demonstrates how to classify data points into distinct groups based on environmental features, which could yield insights into ecological zones that require different management strategies.
Ultimately, machine learning approaches in Python not only enhance the predictive capabilities of environmental science but also deepen our understanding of complex ecological systems. By using these advanced techniques, researchers can generate actionable insights that inform policy decisions and conservation strategies, contributing to the sustainability of our planet.