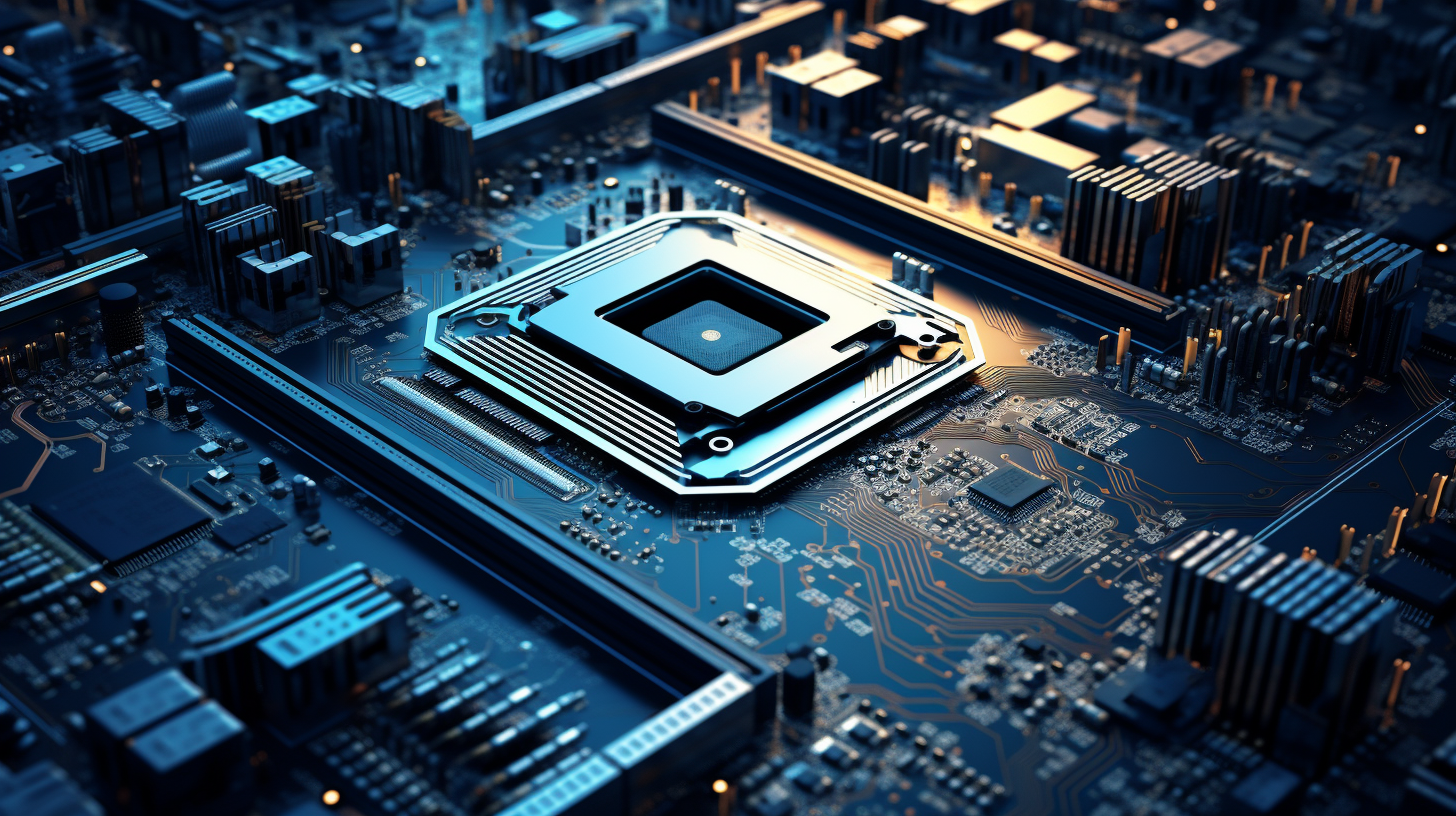
Python for Ethical Hacking: An Introduction
Ethical hacking is the practice of intentionally probing systems and networks for vulnerabilities from an adversarial perspective, yet with the permission and knowledge of the organization that owns the system. This discipline very important because it allows organizations to identify and rectify security flaws before they can be exploited by malicious hackers. The importance of ethical hacking cannot be overstated, especially in today’s digital landscape where cyber threats are pervasive and increasingly sophisticated.
At its core, ethical hacking serves as a proactive defense mechanism. By simulating real-world attacks, ethical hackers help organizations bolster their security postures. In doing so, they not only protect sensitive data but also preserve an organization’s reputation and trustworthiness. The insights gained from such simulations can be instrumental in shaping security policies, training programs, and incident response strategies.
Reflecting the renewed demand for where data breaches can lead to significant financial losses and legal repercussions, ethical hackers play an essential role in safeguarding assets. They utilize a variety of tools and techniques to assess vulnerabilities, and Python, with its simplicity and versatility, has emerged as a favored language in this field. With a rich ecosystem of libraries and frameworks, Python empowers ethical hackers to automate tasks, analyze data, and develop scripts to test security measures efficiently.
Ponder a scenario where a company wants to perform a vulnerability assessment on its web application. An ethical hacker might write a Python script that utilizes the requests library to automate sending various payloads to the server, checking for responses that indicate potential vulnerabilities. Here’s a simplified example:
import requests url = "http://targetwebsite.com/login" payloads = ["' OR '1'='1", "' OR 'x'='x", "' OR '1'='2"] for payload in payloads: data = { "username": "admin", "password": payload } response = requests.post(url, data=data) if "Welcome" in response.text: print(f"Potential vulnerability found with payload: {payload}")
This example illustrates how Python can be employed to automate the tedious process of testing multiple input payloads against a web application, thereby enhancing the speed and efficiency of the testing process.
Moreover, the importance of ethical hacking extends into compliance and regulatory domains. Many industries are subject to regulations that require regular security assessments. Ethical hackers ensure that organizations meet these requirements, reducing the risk of non-compliance fines and legal issues.
As the cyber threat landscape evolves, ethical hacking remains a vital component of a robust security strategy. Those who engage in this practice not only contribute to the safety of their organizations but also to the broader digital ecosystem. The marriage of ethical hacking and Python is a powerful one, providing the tools necessary to tackle challenges head-on.
Essential Python Libraries for Ethical Hacking
When it comes to ethical hacking, the importance of having the right tools at your disposal cannot be overstated. Python’s extensive library ecosystem provides ethical hackers with a plethora of options that streamline various tasks, enhancing both efficiency and effectiveness. Here are some essential Python libraries that every ethical hacker should ponder integrating into their toolkit:
1. Scapy: Scapy is a powerful Python library designed for network packet manipulation and analysis. It allows users to send, sniff, dissect, and forge network packets, making it invaluable for tasks such as network scanning and vulnerability discovery. With Scapy, you can craft custom packets and analyze traffic on your network, which is critical for penetration testing.
from scapy.all import * # Sniffing packets on a specific interface def packet_sniffer(interface): sniff(iface=interface, prn=process_packet, count=10) def process_packet(packet): print(packet.summary()) # Call the sniffer on the desired interface packet_sniffer('eth0')
2. Requests: As mentioned previously, the Requests library is essential for making HTTP requests. It simplifies the process of sending requests and handling responses, making it easier to test web applications for vulnerabilities such as SQL injection or cross-site scripting (XSS).
import requests url = "http://example.com" response = requests.get(url) print(f"Response code: {response.status_code}nResponse body:n{response.text}")
3. Beautiful Soup: For web scraping and extracting data from HTML and XML documents, Beautiful Soup is a must-have. Ethical hackers can use it to gather information from web pages, assess the structure of a target site, and automate data collection for reconnaissance purposes.
from bs4 import BeautifulSoup import requests url = "http://example.com" response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') # Extracting all links from the page links = soup.find_all('a') for link in links: print(link.get('href'))
4. Nmap: While Nmap is primarily a network scanning tool, its Python interface, python-nmap, allows ethical hackers to automate network scans and gather information about live hosts, open ports, and services running on those ports.
import nmap # Initialize the Nmap PortScanner nm = nmap.PortScanner() # Scan a target nm.scan('127.0.0.1', '22-80') print(nm.all_hosts())
5. Socket: The socket library is a fundamental part of Python’s standard library that provides low-level networking interfaces. Ethical hackers can utilize it to create custom clients and servers, enabling them to understand network protocols more deeply and test the robustness of applications against various network-related attacks.
import socket # Create a socket and connect to a server s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect(('localhost', 8080)) s.sendall(b'GET / HTTP/1.1rnHost: localhostrnrn') data = s.recv(1024) print(f'Received: {data.decode()}') s.close()
6. Paramiko: For ethical hackers working with SSH, Paramiko is an excellent library that allows for SSH protocol connection, enabling file transfers and command execution on remote servers securely. That is particularly useful in penetration tests where remote access is required.
import paramiko # Establish an SSH connection ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect('hostname', username='user', password='pass') # Execute a command stdin, stdout, stderr = ssh.exec_command('ls') print(stdout.read().decode()) ssh.close()
These libraries serve as the backbone of many Python scripts used in ethical hacking. By using these tools, ethical hackers can automate tasks, gather intelligence, and conduct thorough assessments with greater speed and accuracy. As the field evolves, staying updated with these libraries and exploring new ones will empower ethical hackers to tackle increasingly complex challenges in cybersecurity.
Setting Up Your Python Environment for Hacking
Setting up your Python environment for ethical hacking is a critical step that can significantly influence your efficiency and effectiveness as a penetration tester. The right environment ensures that you have access to essential tools, libraries, and configurations needed to perform your tasks seamlessly. Here’s how you can create a robust Python setup tailored for ethical hacking.
First and foremost, you need to ensure you have Python installed on your system. Currently, Python 3.x versions are recommended due to their support for state-of-the-art libraries and syntax improvements. You can download the latest version from the official Python website. After installation, validate it by running the following command in your terminal:
python --version
Once Python is installed, it’s wise to set up a virtual environment. This provides an isolated space for your projects, preventing dependency conflicts. You can create a virtual environment using the venv module:
python -m venv myenv
To activate the virtual environment on Windows, use:
myenvScriptsactivate
On macOS or Linux, use:
source myenv/bin/activate
With your virtual environment activated, it’s time to install some essential libraries. The libraries mentioned earlier—like requests, nmap, scapy, and paramiko—can be easily installed using pip, Python’s package manager. For instance, to install the Requests library, execute:
pip install requests
Repeat this for each library that will aid your ethical hacking efforts:
pip install scapy beautifulsoup4 python-nmap paramiko
Next, ponder using an integrated development environment (IDE) or a text editor that supports Python. Popular options include PyCharm, Visual Studio Code, and Sublime Text. These tools offer features like syntax highlighting, debugging, and code completion, which can significantly enhance your coding experience.
For collaborative work and version control, integrating Git into your environment is beneficial. You can install Git from its official site and configure it by setting your username and email:
git config --global user.name "Your Name" git config --global user.email "[email protected]"
Finally, don’t forget to keep your libraries updated. Regularly check for updates using:
pip list --outdated
This command will show you which packages have newer versions available. To update them, simply run:
pip install --upgrade package_name
By setting up a well-structured Python environment, you equip yourself with the necessary tools to conduct effective ethical hacking. This environment not only accelerates your workflow but also aids in maintaining organization and clarity in your projects. With this foundation laid, you can dive deeper into Python scripting and build the custom tools you need for successful penetration testing.
Common Python Scripts for Penetration Testing
In the context of penetration testing, Python scripts are invaluable tools that enable ethical hackers to automate their tasks, gather data, and identify vulnerabilities quickly. Here, we delve into some common Python scripts that can be used effectively during penetration tests, showcasing their structure and functionality.
One of the simplest yet powerful scripts involves using the requests library to perform a basic web application vulnerability scan. This script checks for SQL injection vulnerabilities by sending modified queries to a login form:
import requests url = "http://targetwebsite.com/login" payloads = ["' OR '1'='1';--", "' OR 'x'='x';--", "' OR '1'='2';--"] for payload in payloads: data = { "username": "admin", "password": payload } response = requests.post(url, data=data) if "Welcome" in response.text: print(f"Potential SQL injection vulnerability detected with payload: {payload}")
Another essential script utilizes Scapy for network scanning and packet manipulation. Below is an example of a simple script that scans for active hosts on a network:
from scapy.all import ARP, Ether, srp def scan_network(ip_range): # Create an ARP request to find active hosts arp_request = ARP(pdst=ip_range) ether_frame = Ether(dst="ff:ff:ff:ff:ff:ff") packet = ether_frame / arp_request result = srp(packet, timeout=2, verbose=False)[0] # Parse and display the results active_hosts = [] for sent, received in result: active_hosts.append({'ip': received.psrc, 'mac': received.hwsrc}) return active_hosts # Example usage devices = scan_network("192.168.1.1/24") for device in devices: print(f"IP: {device['ip']}, MAC: {device['mac']}")
For more advanced network scanning, integrating nmap into your Python scripts can yield comprehensive results. The following script demonstrates how to use the python-nmap library to scan for open ports on a target:
import nmap def scan_ports(target): nm = nmap.PortScanner() nm.scan(target, '1-1000') # Scan ports 1 to 1000 print(nm.all_hosts()) for host in nm.all_hosts(): print(f'Host : {host} ({nm[host].hostname()})') print(f'State : {nm[host].state()}') for proto in nm[host].all_protocols(): print(f'Protocol : {proto}') lport = nm[host][proto].keys() for port in sorted(lport): print(f'Port : {port}tState : {nm[host][proto][port]["state"]}') # Example usage scan_ports('192.168.1.1')
Lastly, a common ethical hacking task is to establish a secure SSH connection to a remote server for further testing. The following script illustrates how to use Paramiko to log into a server and execute a command:
import paramiko def ssh_connect(hostname, username, password): ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect(hostname, username=username, password=password) stdin, stdout, stderr = ssh.exec_command('uname -a') print(stdout.read().decode()) ssh.close() # Example usage ssh_connect('192.168.1.100', 'user', 'password')
These scripts represent just a fraction of the capabilities Python offers to ethical hackers. By mastering these tools and techniques, you can significantly enhance your penetration testing efforts, allowing for more thorough assessments and better security measures to be implemented in the systems you are evaluating.
Best Practices for Ethical Hackers Using Python
When engaging in ethical hacking with Python, adhering to best practices is paramount to ensure the effectiveness of your tests while maintaining professionalism and integrity. Here are several best practices that ethical hackers should embrace to elevate their work and contribute positively to the cybersecurity landscape.
1. Always Obtain Permission
The cornerstone of ethical hacking is consent. Before conducting any sort of penetration testing, it’s crucial to have explicit permission from the organization whose systems you’re testing. This not only protects you legally but also helps in building trust with the organization. Document the scope of the testing, including what systems are in scope, what tests will be performed, and any limitations.
2. Document Everything
Documentation plays a vital role in ethical hacking. Keep detailed records of all your tests, findings, methodologies, and scripts used during the assessment. This documentation will be invaluable when reporting your findings to stakeholders and can serve as a reference for future tests. It also enhances the reproducibility of your tests, allowing others to verify and understand your methods.
3. Use Version Control
Using version control systems like Git can help you manage your scripts and documentation effectively. This practice allows for tracking changes, collaborating with team members, and maintaining a history of your work. Create a repository for your projects, and commit changes regularly to ensure that your work is organized and recoverable.
4. Adhere to Coding Standards
Writing clean, readable, and efficient code is essential. Follow Python’s PEP 8 style guide to maintain consistency in your scripts. Well-structured code not only enhances readability for others but also reduces the likelihood of introducing bugs that could compromise your tests. Comments and docstrings should be used liberally to explain the intent of complex logic or functions.
5. Test in Controlled Environments
Whenever possible, conduct your tests in a controlled environment rather than directly against production systems. Setting up a virtual lab where you can simulate attacks and test your scripts allows for safer experimentation without risking real data or systems. Tools like Docker can help you create isolated environments for testing without needing extensive hardware resources.
6. Keep Security in Mind
As an ethical hacker, you should always prioritize security in your scripts and methodologies. Be cautious with sensitive data, such as passwords or API keys, and ensure these are not hard-coded in your scripts. Use environment variables or configuration files to manage sensitive information securely. Additionally, be mindful of the potential impact of your tests on the target systems; avoid actions that could cause downtime or data loss.
7. Stay Updated
The cybersecurity landscape is ever-evolving, and keeping your skills and knowledge current is important. Regularly update your Python libraries and tools to leverage the latest features and security patches. Engage with the cybersecurity community through forums, blogs, and conferences to learn about new vulnerabilities and techniques. Continuous learning strengthens your ability to identify and mitigate emerging threats.
8. Respect Privacy and Confidentiality
Ethical hackers must handle any data they encounter with the utmost care. Whether it’s during a security assessment or in the process of gathering intelligence, ensure that you respect the confidentiality and privacy of sensitive information. Report findings responsibly and avoid disclosing vulnerabilities publicly without the organization’s prior consent.
By adhering to these best practices, ethical hackers using Python can conduct their assessments in a way that’s not only effective but also ethical and responsible. Emphasizing professionalism and integrity in your work will ultimately contribute to a stronger cybersecurity framework and help organizations safeguard their critical assets.
Legal and Ethical Considerations in Hacking with Python
Understanding the legal and ethical considerations in ethical hacking is paramount. Ethical hackers operate in a complex landscape where the line between legitimate testing and illegal intrusion can sometimes blur. To navigate this terrain successfully, it is important to be well-versed in relevant laws and ethical standards.
First and foremost, the legality of ethical hacking hinges on obtaining explicit permission from the organization whose systems are being tested. This permission is typically formalized through a contract or engagement letter that outlines the scope of the testing and delineates what is permitted. Without such permission, any probing of systems could be considered unauthorized access, which is a violation of laws such as the Computer Fraud and Abuse Act in the United States or similar legislation in other jurisdictions.
Moreover, ethical hackers must be mindful of data protection laws. Regulations such as the General Data Protection Regulation (GDPR) in Europe impose strict guidelines on how personal data must be handled. This means that ethical hackers should take care to avoid exposing, storing, or mishandling sensitive data during their assessments. Always anonymize or encrypt data when necessary and ensure that any identifiable information is managed according to the applicable legal requirements.
In addition to legal considerations, ethical hackers are bound by a code of ethics. The primary tenet is to avoid causing harm. This principle emphasizes that while testing may involve exploiting vulnerabilities, the intent is to enhance security rather than disrupt services or compromise data integrity. An ethical hacker must also report all findings transparently to the organization, allowing them to address vulnerabilities without undue panic or public exposure.
To further illustrate these ethical obligations, consider a scenario in which an ethical hacker uncovers a significant vulnerability that could lead to a data breach. The ethical course of action would be to inform the organization immediately, providing them with enough information to understand the severity of the issue without disclosing it publicly until they have had the opportunity to address the vulnerability.
Another critical aspect is to maintain confidentiality. Ethical hackers often have access to sensitive information that should never be shared with unauthorized parties. This confidentiality extends beyond the duration of the engagement; ethical hackers should avoid discussing any findings with third parties unless consent has been granted by the organization.
As an ethical hacker using Python for your assessments, it is vital to incorporate these legal and ethical considerations into your workflow. This not only safeguards you against potential legal repercussions but also reinforces your role as a trusted security advisor. Embracing these principles will lead to more responsible and respectful practices in the field of ethical hacking, ultimately fostering a safer digital environment for everyone.