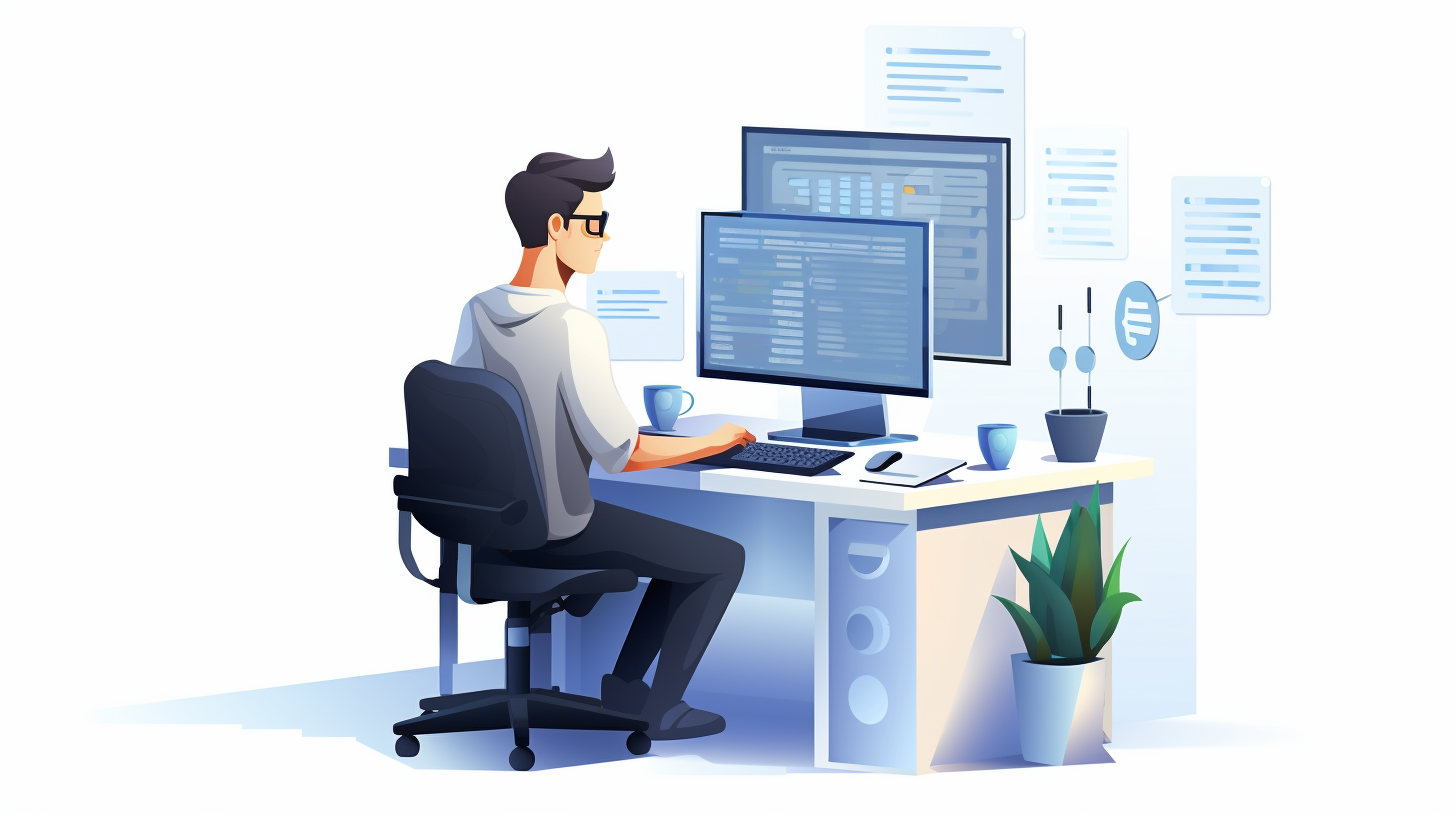
Python for Event Planning: Automation and Analysis
Within the scope of event planning, the complexity of managing logistics, communication, and data analysis can become overwhelming. Python, a versatile and powerful programming language, emerges as a critical ally in simplifying these tasks. By using Python’s capabilities, event planners can automate repetitive processes, thereby allowing them to focus on critical aspects of event execution.
One of the primary advantages of using Python is its readability and simplicity, making it accessible even for those with minimal programming experience. With Python, planners can streamline tasks such as sending invitations, tracking RSVPs, and managing schedules, all while minimizing errors that often occur in manual processes.
Furthermore, Python’s extensive ecosystem of libraries allows for seamless integration with various tools and platforms. For instance, libraries such as pandas and NumPy can be utilized for data analysis, enabling planners to derive insights from attendee information and feedback. This capability transforms raw data into actionable strategies that can significantly enhance the planning of future events.
Think the process of sending invitations. Instead of manually composing and sending emails, Python can automate this task using the smtplib library, which simplifies sending emails directly through a script. Here is a concise example of how to automate email invitations:
import smtplib from email.mime.text import MIMEText def send_invitation(email_recipient, event_details): msg = MIMEText(event_details) msg['Subject'] = 'You're Invited!' msg['From'] = '[email protected]' msg['To'] = email_recipient with smtplib.SMTP('smtp.example.com') as server: server.login('[email protected]', 'your_password') server.send_message(msg) # Example usage send_invitation('[email protected]', 'Join us for an evening of fun and networking!')
In addition to email automation, Python’s capabilities extend to web scraping and data collection, which are essential for gathering information about potential venues, catering options, and attendee demographics. The Beautiful Soup library, for example, allows planners to extract data from web pages easily, enabling them to make informed decisions based on current trends and available options.
The integration of Python into event planning not only enhances efficiency but also fosters a data-driven approach to decision-making. By automating mundane tasks, planners can devote more time to crafting memorable experiences for attendees while using insights obtained through detailed analysis of collected data. In this way, Python becomes an indispensable tool for any event planner aiming to elevate their events to the next level.
Key Libraries and Tools for Event Automation
When delving into the specifics of tools and libraries that enhance event automation in Python, it’s crucial to highlight the breadth of options available to planners. Each library serves a distinct purpose, allowing for a modular approach to building solutions tailored to specific needs.
One key library that stands out in the sphere of automation is pandas. Known for its data manipulation capabilities, pandas can facilitate the management of attendee lists, RSVPs, and feedback forms. Its DataFrame structure allows planners to handle large datasets with ease, performing operations like filtering, sorting, and aggregating data to extract meaningful insights.
import pandas as pd # Example data: Attendee information data = { 'Name': ['Alice', 'Bob', 'Charlie'], 'RSVP': ['Yes', 'No', 'Yes'], 'Email': ['[email protected]', '[email protected]', '[email protected]'] } # Creating a DataFrame df = pd.DataFrame(data) # Filtering to find attendees who have RSVP'd attendees = df[df['RSVP'] == 'Yes'] print(attendees)
Another powerful tool is schedule, a library that enables the automation of repetitive tasks such as sending reminders or follow-up emails. With its intuitive syntax, planners can easily specify when tasks should be executed, thereby keeping all stakeholders informed without needing constant manual intervention.
import schedule import time def send_reminder(): print("Sending reminder email...") # Schedule reminder for every day at 10 AM schedule.every().day.at("10:00").do(send_reminder) while True: schedule.run_pending() time.sleep(1)
Integrating Python with web applications is made seamless with the Flask framework. Planners can create simple web apps to manage event details, display registration forms, and collect feedback. Flask allows for rapid development and deployment of web applications, making it an excellent choice for event planners looking to engage with attendees online.
from flask import Flask, request, render_template app = Flask(__name__) @app.route('/register', methods=['GET', 'POST']) def register(): if request.method == 'POST': # Process registration form data return "Registration Successful" return render_template('register.html') if __name__ == '__main__': app.run(debug=True)
For communication purposes, planners can also integrate the Twilio API for SMS notifications and updates. This capability ensures that last-minute changes or reminders reach attendees quickly and efficiently, enhancing the overall event experience.
from twilio.rest import Client def send_sms(to_number, message): account_sid = 'your_account_sid' auth_token = 'your_auth_token' client = Client(account_sid, auth_token) client.messages.create( body=message, from_='+1234567890', # Your Twilio number to=to_number ) # Example usage send_sms('+1987654321', 'Reminder: Your event starts in 1 hour!')
Moreover, for data visualization, the matplotlib and seaborn libraries can be employed to create charts and graphs that visually represent attendee demographics, feedback, and engagement metrics. These visual tools can aid in presenting data to stakeholders or refining strategies for future events.
import matplotlib.pyplot as plt # Sample data feedback_scores = [5, 4, 3, 4, 5] plt.hist(feedback_scores, bins=5) plt.title('Event Feedback Scores') plt.xlabel('Scores') plt.ylabel('Number of Responses') plt.show()
Using these libraries not only enhances the efficiency of event planning but also provides a framework for continuous improvement through data-driven decisions. By using Python’s extensive tools and libraries, planners can automate, analyze, and adapt their strategies, ultimately leading to more successful and engaging events.
Data Collection and Management Techniques
When it comes to data collection and management in event planning, having a robust system in place is essential. Python’s rich ecosystem provides a variety of tools that simplify the process of gathering, storing, and analyzing data, making it a seamless experience for planners. By employing these techniques, planners can ensure they have the most relevant data at their fingertips, facilitating informed decision-making.
One of the primary methods for data collection is through online forms. Tools like Google Forms or Typeform can be integrated with Python to automatically capture responses. The responses can be fetched using APIs, enabling planners to store them directly into a database or a CSV file for analysis. Using Python’s requests library, planners can make API calls to retrieve this data in real-time.
import requests import pandas as pd # Function to fetch form responses from a hypothetical API def fetch_form_responses(api_url): response = requests.get(api_url) return response.json() # Sample usage api_url = 'https://api.example.com/form_responses' data = fetch_form_responses(api_url) # Convert data to DataFrame for analysis df = pd.DataFrame(data) print(df.head())
Once the data is collected, managing it efficiently becomes a priority. Data can be organized using Python’s pandas library, allowing planners to manipulate data frames freely. For instance, filtering attendee responses based on specific criteria—such as dietary preferences or attendance confirmation—can help in planning logistics more effectively.
# Example of filtering attendees based on dietary preference attendees_data = { 'Name': ['Alice', 'Bob', 'Charlie', 'David'], 'Dietary Preference': ['Vegan', 'Vegetarian', 'None', 'Vegan'] } df_attendees = pd.DataFrame(attendees_data) # Filtering vegan attendees vegan_attendees = df_attendees[df_attendees['Dietary Preference'] == 'Vegan'] print(vegan_attendees)
Data storage is another crucial component. Event planners can utilize databases like SQLite or PostgreSQL to store large amounts of data securely. Python’s SQLAlchemy library provides an ORM (Object-Relational Mapping) system, enabling planners to interact with databases using Python objects instead of raw SQL queries. This abstraction simplifies the process of data management significantly.
from sqlalchemy import create_engine import pandas as pd # Creating a SQLite database connection engine = create_engine('sqlite:///event_planning.db') # Example DataFrame to be stored data = {'Name': ['Alice', 'Bob'], 'RSVP': ['Yes', 'No']} df = pd.DataFrame(data) # Storing the DataFrame in a SQLite table df.to_sql('attendees', con=engine, if_exists='replace', index=False) # Querying the database to confirm storage stored_data = pd.read_sql('SELECT * FROM attendees', con=engine) print(stored_data)
Moreover, managing event data isn’t just about collection and storage—it’s also about ensuring data quality. This involves cleaning the data, which can be accomplished using pandas’ built-in functionalities. Handling missing values, removing duplicates, and standardizing formats plays a vital role in maintaining the integrity of the data being analyzed.
# Example of data cleaning data_with_duplicates = { 'Name': ['Alice', 'Bob', 'Alice', 'Charlie'], 'Email': ['[email protected]', '[email protected]', '[email protected]', '[email protected]'] } df_duplicates = pd.DataFrame(data_with_duplicates) # Removing duplicates based on 'Name' column cleaned_data = df_duplicates.drop_duplicates(subset=['Name']) print(cleaned_data)
Finally, with all data collected, managed, and cleaned, the next step is to analyze it effectively. Using libraries like matplotlib or seaborn, planners can create visual representations of the data to identify trends and patterns. This analysis can provide insights into attendee demographics, satisfaction levels, and even areas for improvement in future events.
import matplotlib.pyplot as plt # Sample data for analysis attendance_counts = {'Yes': 60, 'No': 20} plt.bar(attendance_counts.keys(), attendance_counts.values()) plt.title('Event Attendance Overview') plt.xlabel('RSVP Status') plt.ylabel('Number of Attendees') plt.show()
The integration of Python into data collection and management processes not only streamlines workflows but also empowers event planners to base their decisions on solid data analysis. By using the power of Python’s libraries and tools, planners can elevate their event management practices, ensuring a more organized and successful outcome.
Automating Tasks: From Invitations to Follow-ups
In the fast-paced world of event planning, automating tasks can significantly enhance efficiency and reduce the risk of human error. Python’s robust capabilities allow planners to streamline processes from the initial invitation to the final follow-up, ensuring that every detail is attended to without excessive manual intervention.
Starting with invitations, the automation process can be implemented using Python’s smtplib library, as previously mentioned. However, we can expand this functionality by integrating a list of recipients and customizing each message based on attendee preferences. This level of personalization can significantly improve engagement and attendance rates.
import smtplib from email.mime.text import MIMEText import pandas as pd def send_invitations(attendee_list, event_details): for index, attendee in attendee_list.iterrows(): msg = MIMEText(event_details + f"nnBest,nYour Event Team") msg['Subject'] = 'You're Invited!' msg['From'] = '[email protected]' msg['To'] = attendee['Email'] with smtplib.SMTP('smtp.example.com') as server: server.login('[email protected]', 'your_password') server.send_message(msg) # Example usage attendees_data = { 'Name': ['Alice', 'Bob', 'Charlie'], 'Email': ['[email protected]', '[email protected]', '[email protected]'] } attendee_list = pd.DataFrame(attendees_data) event_details = 'Join us for an evening of fun and networking!' send_invitations(attendee_list, event_details)
After invitations are dispatched, tracking RSVPs can also be automated. Using a simple web application built with Flask, planners can create a registration page where attendees can respond. The collected data can then be stored in a database for easy access and analysis.
from flask import Flask, request, render_template from sqlalchemy import create_engine app = Flask(__name__) engine = create_engine('sqlite:///event_planning.db') @app.route('/register', methods=['GET', 'POST']) def register(): if request.method == 'POST': name = request.form['name'] email = request.form['email'] rsvp = request.form['rsvp'] # Store RSVP in the database df = pd.DataFrame({'Name': [name], 'Email': [email], 'RSVP': [rsvp]}) df.to_sql('attendees', con=engine, if_exists='append', index=False) return "Registration Successful" return render_template('register.html') if __name__ == '__main__': app.run(debug=True)
Once the RSVPs have been captured, follow-ups can be automated based on the attendee’s response. For instance, those who have confirmed their attendance can receive reminders as the event date approaches. This can be achieved using the schedule library, which enables planners to set recurring tasks.
import schedule import time def send_reminder(name, email): msg = f"Hello {name}, that is a reminder for the upcoming event!" # Code to send email (similar to previous example) print(f"Sending reminder email to {email}") # Schedule reminders for confirmed attendees def schedule_reminders(attendees): for index, attendee in attendees.iterrows(): if attendee['RSVP'] == 'Yes': schedule.every().day.at("10:00").do(send_reminder, attendee['Name'], attendee['Email']) # Mock attendee DataFrame for scheduling attendees_data = { 'Name': ['Alice', 'Bob', 'Charlie'], 'RSVP': ['Yes', 'No', 'Yes'], 'Email': ['[email protected]', '[email protected]', '[email protected]'] } attendees = pd.DataFrame(attendees_data) schedule_reminders(attendees) while True: schedule.run_pending() time.sleep(1)
Finally, after the event, follow-up tasks like sending thank-you emails can be automated similarly. Maintaining engagement post-event is important for building lasting relationships with attendees.
def send_thank_you(attendee): msg = f"Thank you for attending the event, {attendee['Name']}!" # Code to send email (similar to previous example) print(f"Sending thank you email to {attendee['Email']}") # Sending thank-you emails to all attendees for index, attendee in attendees.iterrows(): send_thank_you(attendee)
By embracing these automation techniques, event planners can not only save time but also ensure that every aspect of the event is executed flawlessly. With Python handling the repetitive tasks, planners can focus on the creative elements that make an event truly memorable.
Analyzing Event Data for Future Improvements
In the landscape of event planning, analyzing data is not merely a beneficial practice; it’s a necessity for continuous improvement. Python offers a suite of tools that empower planners to delve into their event data, extracting insights that inform future strategies and enhance attendee experiences. Whether it’s feedback from participants, attendance rates, or engagement metrics, the ability to analyze this data can significantly influence the success of subsequent events.
At the core of data analysis in Python lies the pandas library, which provides robust data manipulation capabilities. Event planners can use pandas to read datasets, perform statistical analysis, and visualize trends over time. For example, they can analyze feedback scores collected from attendees to understand satisfaction levels and identify areas for improvement.
import pandas as pd # Example dataset of feedback scores feedback_data = { 'Attendee': ['Alice', 'Bob', 'Charlie'], 'Feedback Score': [4, 5, 3], 'Comments': ['Great event!', 'Loved the networking opportunities.', 'Need more breakout sessions.'] } df_feedback = pd.DataFrame(feedback_data) # Calculating average feedback score average_score = df_feedback['Feedback Score'].mean() print(f'Average Feedback Score: {average_score}') # Displaying comments for further qualitative analysis print('Comments:') print(df_feedback['Comments'])
Visualization is another crucial aspect of data analysis. By employing libraries such as Matplotlib and Seaborn, planners can create compelling charts and graphs that represent attendance trends, survey results, and overall event engagement. Visualizations can help stakeholders quickly grasp complex data, making it easier to convey insights during planning meetings.
import matplotlib.pyplot as plt # Sample attendance data attendance_data = { 'Event': ['Event A', 'Event B', 'Event C'], 'Attendance': [100, 150, 120] } df_attendance = pd.DataFrame(attendance_data) plt.bar(df_attendance['Event'], df_attendance['Attendance'], color='skyblue') plt.title('Attendance Overview for Recent Events') plt.xlabel('Events') plt.ylabel('Number of Attendees') plt.show()
In addition to visualizing attendance, planners can analyze engagement metrics through follow-up surveys. By comparing responses from different events, planners can identify patterns that may indicate what aspects of their events resonate most with attendees. For instance, they may discover that networking opportunities consistently receive high ratings, guiding them to allocate more resources to this element in the future.
# Example of comparing feedback across multiple events event_feedback_data = { 'Event': ['Event A', 'Event A', 'Event B', 'Event B', 'Event C', 'Event C'], 'Feedback Score': [4, 5, 3, 4, 5, 4] } df_event_feedback = pd.DataFrame(event_feedback_data) # Grouping by event to calculate average feedback score average_event_feedback = df_event_feedback.groupby('Event')['Feedback Score'].mean() print('Average Feedback Scores by Event:') print(average_event_feedback)
Moreover, predictive analytics can be employed to forecast attendance and engagement for future events based on historical data. By analyzing trends and patterns, planners can make informed decisions regarding venue selection, marketing strategies, and program offerings. Python’s scikit-learn library offers tools for building predictive models, which can further enhance planning accuracy.
from sklearn.linear_model import LinearRegression import numpy as np # Historical attendance data X = np.array([[1], [2], [3]]) # Event numbers (1, 2, 3) y = np.array([100, 150, 120]) # Corresponding attendance numbers # Creating and fitting the model model = LinearRegression() model.fit(X, y) # Predict attendance for the next event next_event_number = np.array([[4]]) predicted_attendance = model.predict(next_event_number) print(f'Predicted attendance for Event 4: {predicted_attendance[0]}')
Finally, the synthesis of qualitative and quantitative data will produce a comprehensive overview of the event’s effectiveness. By integrating feedback, attendance statistics, and predictive analytics, planners can craft a strategic roadmap for future events, ensuring they evolve and improve with each iteration. Python’s capabilities in data analysis empower event planners to make decisions grounded in insights, fostering a cycle of continuous improvement that drives success.
Case Studies: Successful Python Implementations in Event Planning
In the real world, Python has found a high number of applications in event planning that underscore its versatility and power. Case studies from various organizations demonstrate how they have successfully implemented Python to streamline operations, enhance attendee engagement, and drive better outcomes.
One notable example comes from a tech conference organizer that leveraged Python for automating its registration process. Before adopting Python, the organization faced challenges with manual registration, leading to errors and inefficiencies. By implementing a Python-based web application using Flask, the organizer created a seamless registration interface. Attendees could register online, with their data being automatically saved to a PostgreSQL database via SQLAlchemy. This integration not only reduced errors but also allowed for real-time updates on attendee numbers.
from flask import Flask, request, render_template from sqlalchemy import create_engine app = Flask(__name__) engine = create_engine('postgresql://user:password@localhost/event_planning') @app.route('/register', methods=['GET', 'POST']) def register(): if request.method == 'POST': name = request.form['name'] email = request.form['email'] # Store registration in the database df = pd.DataFrame({'Name': [name], 'Email': [email]}) df.to_sql('registrations', con=engine, if_exists='append', index=False) return "Registration Successful" return render_template('register.html') if __name__ == '__main__': app.run(debug=True)
Additionally, the organizer utilized Python’s pandas library to analyze registration data post-event. By examining patterns in attendee demographics and registration times, they were able to better target their marketing efforts for future conferences. This not only improved registration rates but also enhanced the overall attendee experience.
Another case study involves a corporate event planner who sought to integrate feedback collection into their post-event routine. They employed Python scripts to automatically send out surveys via email using the smtplib library, gathering valuable insights from participants. By analyzing the feedback scores with pandas, the planner could visualize trends over time, identifying which aspects of the event were well-received and which required improvement.
import smtplib from email.mime.text import MIMEText import pandas as pd def send_feedback_request(attendees): for attendee in attendees: msg = MIMEText("We’d love your feedback on our recent event! Please fill out our survey.") msg['Subject'] = 'Feedback Request' msg['From'] = '[email protected]' msg['To'] = attendee['Email'] with smtplib.SMTP('smtp.example.com') as server: server.login('[email protected]', 'your_password') server.send_message(msg) attendees_data = [{'Name': 'Alice', 'Email': '[email protected]'}, {'Name': 'Bob', 'Email': '[email protected]'}] send_feedback_request(attendees_data)
Post-event analysis revealed that the event’s networking opportunities received exceptionally high marks, prompting the planner to focus on expanding these elements in future events. Not only did this feedback loop improve the event experience, but it also fostered stronger relationships with attendees, as they felt their opinions were valued.
One more compelling example is from a non-profit organization that used Python to manage fundraising events. The organization employed web scraping techniques with Beautiful Soup to gather information on potential sponsors and venues, which streamlined their planning process significantly. Coupled with data visualization tools like Matplotlib, they could present compelling data to potential sponsors, showcasing attendee demographics and past event successes.
import requests from bs4 import BeautifulSoup def scrape_venue_data(url): response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') # Extract relevant venue information venues = soup.find_all('div', class_='venue-details') return [venue.text for venue in venues] venue_url = 'https://example.com/venues' venue_data = scrape_venue_data(venue_url) print(venue_data)
This strategic use of Python not only saved the organization time and resources but also enhanced its credibility when approaching potential sponsors. By presenting data-driven insights, they were able to secure more funding for their events, ultimately benefiting their cause.
These case studies illustrate the transformative impact that Python can have in the context of event planning. By automating processes, analyzing data, and using insights, organizations can enhance their operational efficiency, improve attendee experiences, and drive better overall outcomes. As Python continues to evolve, its role in event planning will undoubtedly expand, offering even more innovative solutions to meet the demands of the industry.