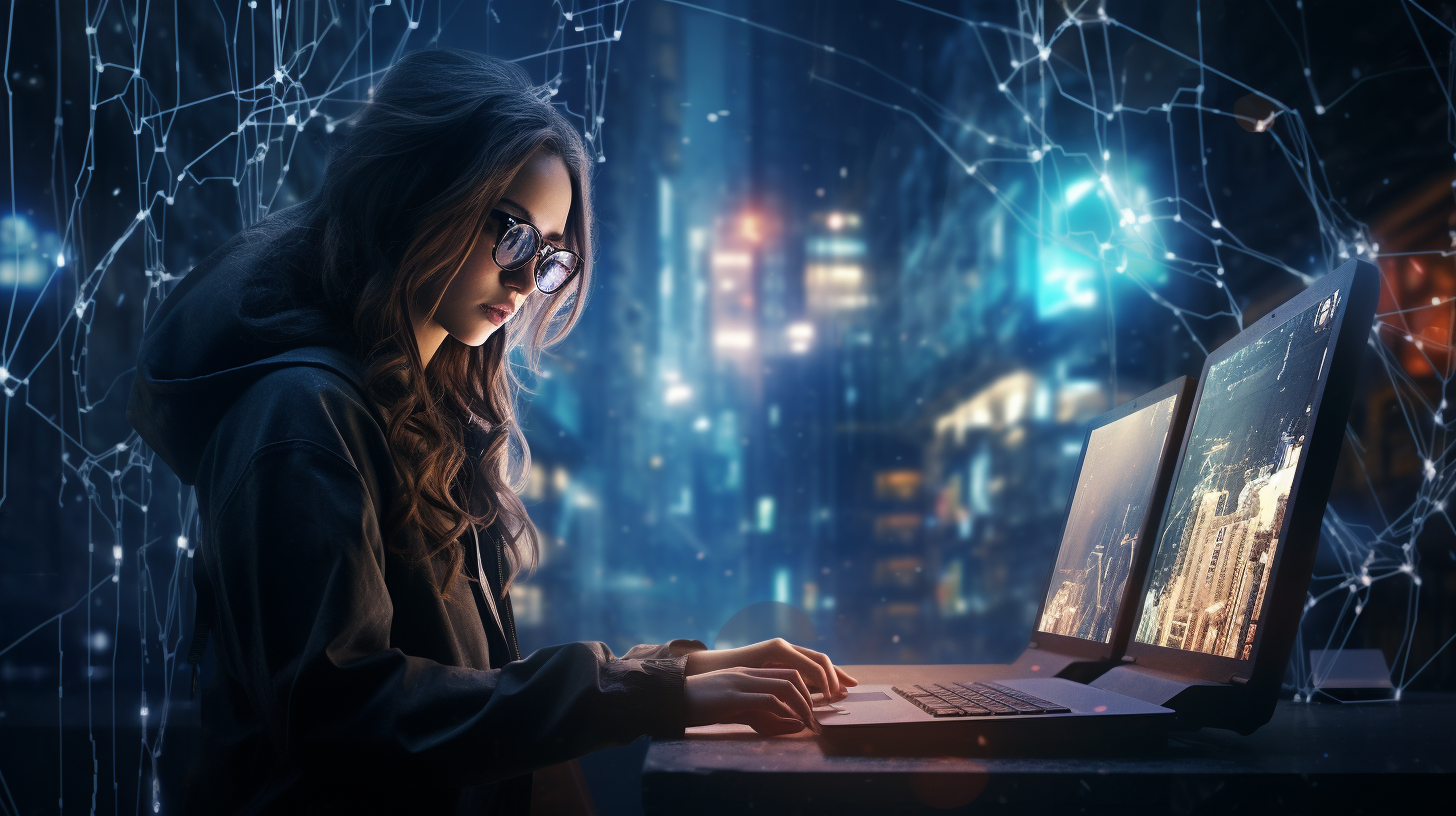
Python for Game Development: An Overview
Python has carved out a niche for itself within the scope of game development, primarily due to its simplicity and readability. This language, often lauded for its ease of learning, allows developers to focus on the creative aspects of game design rather than getting bogged down in complex syntax. While Python may not be the first choice for high-performance gaming, where speed is important, it excels in rapid prototyping and as a scripting language in larger projects.
One of the key advantages of Python is its versatility. It can be employed for building entire games or for scripting behavior within games developed with other languages. This dual capability makes it particularly appealing to indie developers and hobbyists who want to create games without the steep learning curve associated with more complex languages like C++.
In the game development ecosystem, Python shines when it comes to integrating with other tools and technologies. For instance, you can use Python in conjunction with C or C++ to handle less performance-critical components, enhancing productivity while maintaining speed where necessary. This approach leverages Python’s ease of use for scripting, while relying on more performant languages for computationally intensive tasks.
Moreover, Python’s extensive standard library and the availability of third-party libraries specifically designed for game development extend its utility. Libraries such as Pygame, Panda3D, and Godot (which offers a Python-like scripting language) provide a solid foundation for building games across various genres.
For example, using Pygame, you can quickly create a simple game loop. Here’s a minimal example:
import pygame import sys # Initialize Pygame pygame.init() # Set up display width, height = 800, 600 screen = pygame.display.set_mode((width, height)) # Main game loop while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() # Fill the screen with a color screen.fill((0, 0, 0)) # Refresh the screen pygame.display.flip()
In this example, the Pygame library is initialized, a window is created, and a basic game loop is implemented. The simplicity of the loop allows developers to focus on the game logic rather than the complexities of window management.
Additionally, Python’s strong community support cannot be overlooked. With a wealth of tutorials, forums, and documentation available, aspiring game developers can find an abundance of resources to assist them in their journey. This community aspect enhances learning and encourages collaboration, which is vital in the iterative process of game design.
Ultimately, while Python may not be the go-to language for blockbuster games, its strengths in rapid development, ease of integration, and community support make it a valuable tool in a developer’s arsenal, particularly for those exploring the vast creative possibilities of game design.
Popular Python Game Development Libraries and Frameworks
When it comes to game development with Python, several libraries and frameworks stand out due to their robustness and the features they offer. These tools not only simplify the development process but also provide a variety of functionalities tailored for different types of games.
Pygame is perhaps the most well-known library for game development in Python. It provides a set of modules designed for writing video games and includes functionalities for handling graphics, sound, and input devices. Pygame is particularly suited for 2D games, and its ease of use makes it a popular choice among beginners. Here’s how you might implement a simple sprite in Pygame:
import pygame import sys # Initialize Pygame pygame.init() # Set up display width, height = 800, 600 screen = pygame.display.set_mode((width, height)) # Load an image sprite_image = pygame.image.load('sprite.png') sprite_rect = sprite_image.get_rect() # Main game loop while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() # Move the sprite sprite_rect.x += 5 # Move right # Fill the screen with a color screen.fill((0, 0, 0)) # Draw the sprite on the screen screen.blit(sprite_image, sprite_rect) # Refresh the screen pygame.display.flip()
Another noteworthy framework is Panda3D, which is more focused on 3D game development. Developed by Disney and maintained by Carnegie Mellon University, Panda3D provides a comprehensive set of tools for rendering 3D graphics, managing assets, and even integrating physics engines. Its flexibility allows developers to create complex games while using Python for scripting. Here’s a basic example to create a simple scene in Panda3D:
from panda3d.core import Point3 from direct.showbase.ShowBase import ShowBase class MyApp(ShowBase): def __init__(self): ShowBase.__init__(self) self.load_environment() def load_environment(self): self.environ = self.loader.loadModel("models/environment") self.environ.reparentTo(self.render) self.environ.setScale(0.25, 0.25, 0.25) self.environ.setPos(-8, 42, 0) app = MyApp() app.run()
For developers seeking to build more sophisticated games, the Godot Engine has become increasingly popular. While Godot supports its own GDScript language, it also allows for integration with Python through various methods, enabling developers to write game logic in Python if they prefer. Godot is particularly praised for its scene system and node-based architecture, which can greatly enhance development efficiency.
Additionally, there are various other libraries such as PyOpenGL, which allows for OpenGL rendering in Python, and Arcade, a contemporary Python framework for 2D games that emphasizes simplicity and performance. Each of these libraries provides unique features suited for different types of games and levels of complexity.
The choice of a library or framework often depends on the specific requirements of the game being developed, the developer’s familiarity with the tools, and the desired features of the final product. Whether building simple 2D games with Pygame or complex 3D worlds with Panda3D or Godot, Python offers an array of powerful options for game developers eager to explore their creativity.
Setting Up Your Python Game Development Environment
Setting up your Python game development environment is an important first step that can significantly impact your productivity and development experience. A well-configured environment helps streamline your workflow, so that you can focus on crafting your game rather than being bogged down by technical issues.
To begin, the first thing you’ll need is to install Python. You can download the latest version from the official Python website. Make sure to select the option to add Python to your system PATH during installation. This will allow you to run Python commands from your terminal or command prompt easily.
Once Python is installed, it is a good practice to set up a virtual environment. Virtual environments allow you to manage dependencies for different projects independently, helping avoid version conflicts. You can create a virtual environment using the following command:
python -m venv mygameenv
This command creates a new directory called mygameenv
in your current working directory. To activate the virtual environment, use:
# On Windows mygameenvScriptsactivate # On macOS/Linux source mygameenv/bin/activate
Once activated, you can install the necessary game development libraries, such as Pygame or Panda3D, using pip. For example, to install Pygame, run:
pip install pygame
After installation, you can verify that Pygame is properly installed by running a simple script. Create a file named test_pygame.py
and add the following code:
import pygame # Initialize Pygame pygame.init() # Check if Pygame is installed correctly print("Pygame is successfully installed!")
Run the script using the command:
python test_pygame.py
If you see “Pygame is successfully installed!” in your terminal, you’re ready to move on!
In addition to installing game libraries, think setting up an Integrated Development Environment (IDE) or text editor that suits your workflow. Popular choices for Python development include PyCharm, Visual Studio Code, and Sublime Text. These environments support Python syntax highlighting, code completion, and debugging features that can enhance your coding efficiency.
For a more game-specific workflow, you might ponder using engines like Godot, which has its own set of tools but allows for Python-like scripting through GDScript and third-party integrations. Setting up Godot is straightforward: download the executable from the official site, and follow the installation instructions provided there.
Finally, don’t forget about version control. Learning to use git will help you keep track of your changes and collaborate with others effectively. Initialize a git repository in your game project directory with:
git init
From here, you can start tracking your game development progress and revert to previous versions if necessary.
Game Design Principles and Best Practices Using Python
When diving into game development with Python, it’s essential to adopt certain design principles and best practices to ensure that your projects are not only functional but also enjoyable and maintainable. These principles can help you streamline your workflow and enhance the overall quality of your game.
1. Maintainability and Organization
One of the foremost practices in game development is maintaining an organized codebase. As your game project grows, it becomes increasingly challenging to manage files and code. Structuring your project with a clear directory hierarchy and using meaningful naming conventions can significantly improve readability and maintainability. For instance, think organizing your assets into directories such as assets/images
, assets/sounds
, and src
for your source code.
Here’s a simple structure for a Pygame project:
my_game/ ├── assets/ │ ├── images/ │ ├── sounds/ ├── src/ │ ├── main.py │ ├── game.py │ ├── player.py │ └── enemy.py └── README.md
2. Modular Design
Adopting a modular design encourages you to break your game into discrete components. Each module can handle a specific aspect of the game, such as rendering, physics, or input handling. This separation not only clarifies responsibilities but also makes it easier to test and debug individual components.
For example, consider the following modular approach where a player class encapsulates player-related functionality:
class Player: def __init__(self, x, y): self.x = x self.y = y self.speed = 5 def move(self, dx, dy): self.x += dx * self.speed self.y += dy * self.speed def get_position(self): return (self.x, self.y)
This Player class can be imported and utilized in your main game loop, keeping the game logic clean and focused.
3. Game Loop Design
A robust game loop especially important for managing the flow of your game. It should handle updating game state, processing input, and rendering graphics efficiently. A common approach is to separate these functionalities into distinct methods within your game class. Here’s a sample game loop structure:
class Game: def __init__(self): self.player = Player(100, 100) def update(self): # Update game state pass def render(self): # Draw everything pass def run(self): while True: self.process_events() self.update() self.render() pygame.display.flip() # Update the full display Surface to the screen
4. Resource Management
Efficiently managing resources such as images and sounds is vital for performance. Loading assets at the beginning of your game instead of during gameplay can help prevent lag during critical moments. You can create a resource manager that handles loading and caching of assets:
class ResourceManager: def __init__(self): self.images = {} self.sounds = {} def load_image(self, name, path): self.images[name] = pygame.image.load(path) def get_image(self, name): return self.images.get(name) def load_sound(self, name, path): self.sounds[name] = pygame.mixer.Sound(path) def get_sound(self, name): return self.sounds.get(name)
This way, you can call load_image
or get_image
whenever you need to access graphics, keeping your main game loop free from clutter.
5. Testing and Iteration
Finally, embrace testing and iteration as fundamental parts of your development process. Regularly testing your game for bugs and gameplay balance helps ensure a polished final product. Consider using Python’s built-in unittest
framework to create automated tests for critical components. A simple test case for the Player class might look like this:
import unittest class TestPlayer(unittest.TestCase): def test_move(self): player = Player(100, 100) player.move(1, 0) # Move right self.assertEqual(player.get_position(), (105, 100)) if __name__ == '__main__': unittest.main()
By integrating these principles into your workflow, you can create games that are not only functional but also enjoyable to play and easy to maintain. Embracing the iterative nature of game development will allow you to refine your ideas and elevate your projects to new heights.
Case Studies: Successful Games Developed with Python
When examining the landscape of successful games developed with Python, it’s essential to acknowledge that while Python may not dominate the AAA game market, it has been instrumental in creating innovative and engaging titles across various genres. Many indie games have leveraged Python’s simplicity and rapid development capabilities, proving that great games can emerge from the most accessible tools.
One noteworthy example is Frets on Fire, a free, open-source music game that challenges players to perform guitar covers of their favorite songs. Developed using Pygame, the game allows users to play using a keyboard as a guitar fretboard. Its engaging gameplay and community-driven song library exemplify how Python can facilitate creativity and collaboration among developers and players alike.
# Import required libraries import pygame import sys # Define constants SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600 # Initialize Pygame pygame.init() screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT)) def main(): # Main game loop while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() # Update game state and render here pygame.display.flip() if __name__ == "__main__": main()
Another impressive example is Battle for Wesnoth, a turn-based strategy game that has garnered a devoted fan base. Originally written in C++, the game has utilized Python for scripting, allowing developers to create complex scenarios and gameplay mechanics while keeping the main engine performant. The use of Python made it easier for modders to contribute to the game, fostering a vibrant community that continually expands the game’s universe.
Similarly, Panda3D has facilitated the development of visually stunning games like Toontown Online, which was an MMORPG designed for children. This game utilized Panda3D for its 3D rendering capabilities, showcasing how Python can handle large-scale, immersive worlds. The engine’s Python scripting allowed for rapid iteration and updates, enabling the developers to refine their game mechanics and enhance player experiences.
# Example snippet for creating a character in Panda3D from panda3d.core import NodePath from direct.showbase.ShowBase import ShowBase class MyGame(ShowBase): def __init__(self): ShowBase.__init__(self) self.character = self.loader.loadModel("models/character") self.character.reparentTo(self.render) # Scale and position the character self.character.setScale(0.5) self.character.setPos(0, 10, 0) app = MyGame() app.run()
In the sphere of educational games, PyGame School demonstrates Python’s potential to engage young learners. This project showcases a series of simple educational games designed to teach fundamental concepts such as math, spelling, and problem-solving. By using Pygame, developers can create interactive learning environments that make education both fun and accessible.
Moreover, the game Cataclysm: Dark Days Ahead offers a gripping experience in post-apocalyptic survival. It is a roguelike game that utilizes Python for its complex mechanics and deep gameplay. The flexibility of Python allows developers to implement intricate systems for crafting, combat, and exploration. This highly modifiable nature has attracted a dedicated community, fostering continuous enhancements and new content.
# Example configuration of a game entity in Cataclysm: Dark Days Ahead class Character: def __init__(self, name, health): self.name = name self.health = health def take_damage(self, amount): self.health -= amount if self.health <= 0: self.die() def die(self): print(f"{self.name} has died!") # Create a character instance hero = Character("Hero", 100) hero.take_damage(50)
These examples illustrate that Python’s impact on game development is not just limited to small projects. From indie games to larger community-driven projects, Python has proven to be a powerful ally in the sphere of game design. Its ability to streamline development and foster collaboration makes it an invaluable tool for creators eager to bring their visions to life. Whether you are a seasoned developer or a novice looking to create your first game, Python opens doors to endless possibilities in the gaming industry.
Future Trends in Python Game Development
As we look ahead, the landscape of Python game development is poised for exciting changes, driven by both technological advancements and shifting developer needs. The rise of artificial intelligence (AI) and machine learning (ML) is expected to influence game design significantly, enabling developers to create more interactive and adaptive experiences. Python, with its robust libraries for AI, such as TensorFlow and scikit-learn, is well-positioned to facilitate this evolution. Developers can implement smarter non-player characters (NPCs) that adapt to player behavior, creating dynamic gameplay that evolves over time.
Moreover, the integration of virtual reality (VR) and augmented reality (AR) is becoming increasingly prevalent in the gaming industry. While traditionally dominated by languages such as C#, Python is making strides in this area as well. Libraries like OpenVR and OpenGL can be utilized within Python environments, allowing developers to experiment with VR and AR experiences. This shift opens up new avenues for creativity, enabling developers to craft immersive worlds that blend seamlessly with real life.
Another trend is the growth of cloud gaming, which allows players to access high-quality gaming experiences on various devices without requiring high-end hardware. Python can play an important role here, particularly in backend development. Frameworks like Flask and Django can be leveraged to create robust server-side applications that manage game state and player interactions in real time. This approach not only enhances accessibility but also broadens the reach of indie developers, allowing their games to be played by a larger audience.
Cross-platform development is also gaining traction, and Python’s platform-independent nature makes it an ideal candidate for this trend. Developers can create games that run seamlessly on multiple operating systems and devices, reaching players wherever they are. Tools like Kivy and BeeWare are making it easier for developers to create cross-platform applications with Python, further solidifying its place in the game development ecosystem.
Finally, the emphasis on community collaboration and open-source projects continues to grow. Developers now have access to a wealth of shared resources, libraries, and frameworks that they can build upon. This collaborative spirit encourages innovation and reduces the time required to bring game concepts to life. As more developers share their work, the Python game development community is likely to expand, fostering a vibrant ecosystem where knowledge and resources are freely exchanged.
The future of Python in game development is bright, driven by AI, VR/AR integration, cloud gaming, cross-platform capabilities, and a strong community ethos. These trends will not only enhance the quality of games produced with Python but also empower developers to explore new frontiers in creativity and interaction. By embracing these changes, developers can continue to push the boundaries of what is possible in gaming, making Python a key player in the industry’s evolving landscape.