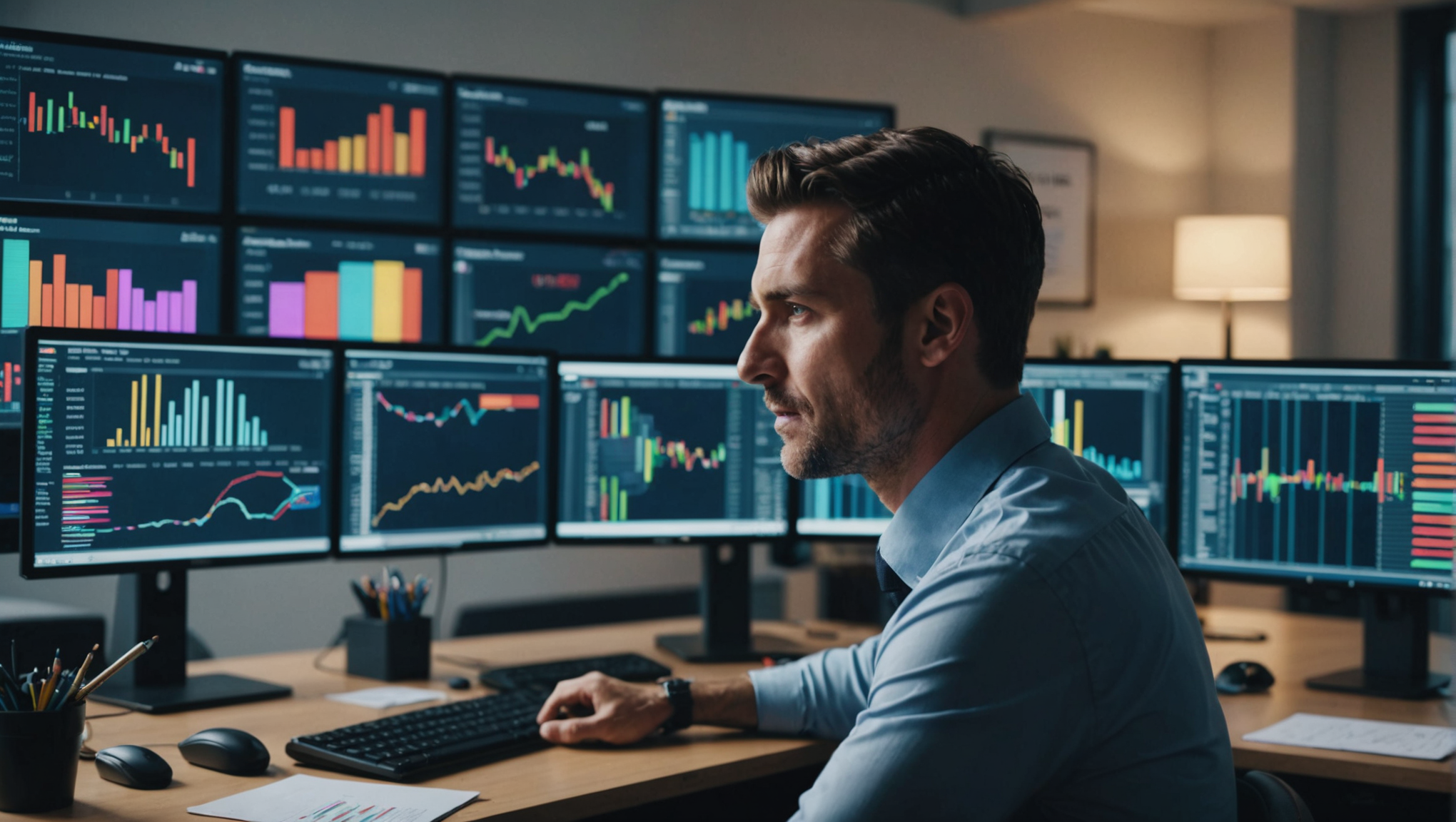
Python for Human Resources: Automating Tasks
Recruitment, often viewed as a tedious and time-consuming process, can be revolutionized with the power of automation. By using Python’s capabilities, HR professionals can streamline various aspects of recruitment, from resume parsing to candidate tracking. The crux of the solution lies in using libraries and APIs designed to automate repetitive tasks, allowing HR teams to focus on what really matters—the candidates themselves.
One of the first steps in automating the recruitment process is to parse resumes. The Python library PyPDF2 can be utilized to extract text from PDF resumes. Here’s a simple example of how to read a resume file:
import PyPDF2 def read_resume(file_path): with open(file_path, 'rb') as file: reader = PyPDF2.PdfFileReader(file) text = '' for page in range(reader.numPages): text += reader.getPage(page).extractText() return text resume_text = read_resume('resume.pdf') print(resume_text)
Once extracted, this text can be analyzed and filtered using Python’s natural language processing capabilities. Libraries such as spaCy or NLTK can help identify relevant keywords and phrases that match job descriptions.
Moreover, automating the tracking of candidates through a recruitment pipeline can be achieved with a simple database using SQLite. The following code snippet demonstrates how to create a basic database to store candidate information:
import sqlite3 def create_database(): conn = sqlite3.connect('recruitment.db') cursor = conn.cursor() cursor.execute(''' CREATE TABLE IF NOT EXISTS candidates ( id INTEGER PRIMARY KEY, name TEXT, email TEXT, resume TEXT, status TEXT ) ''') conn.commit() conn.close() create_database()
With the database in place, you can easily insert new candidates as they apply:
def add_candidate(name, email, resume, status='Applied'): conn = sqlite3.connect('recruitment.db') cursor = conn.cursor() cursor.execute(''' INSERT INTO candidates (name, email, resume, status) VALUES (?, ?, ?, ?) ''', (name, email, resume, status)) conn.commit() conn.close() add_candidate('Mitch Carter', '[email protected]', resume_text)
Finally, automating email notifications to candidates can enhance the recruitment experience. Using the smtplib library, you can send personalized emails to candidates:
import smtplib from email.mime.text import MIMEText def send_email(to_email, subject, body): msg = MIMEText(body) msg['Subject'] = subject msg['From'] = '[email protected]' msg['To'] = to_email with smtplib.SMTP('smtp.example.com', 587) as server: server.starttls() server.login('[email protected]', 'password') server.send_message(msg) send_email('[email protected]', 'Application Received', 'Thank you for applying!')
By integrating these automation techniques, HR professionals can ensure that the recruitment process is not only faster but also more efficient, allowing for a greater focus on engaging potential employees in meaningful ways.
Automating Employee Onboarding Tasks
Employee onboarding is another critical area where Python can streamline and automate processes, ultimately leading to a more organized and efficient experience for new hires. The onboarding process often involves multiple tasks, from document collection to training schedules, and automating these tasks can significantly reduce the administrative burden on HR teams.
One effective way to manage the onboarding process is through the creation of onboarding checklists, which can be automated using Python. By using a simple script, HR professionals can generate personalized checklists for each new employee, ensuring that no important steps are overlooked. Here’s an example of how to create a basic onboarding checklist:
def create_onboarding_checklist(employee_name, start_date): checklist = [ "Complete HR paperwork", "Set up employee benefits", "Schedule introduction meetings with the team", "Assign a mentor", "Provide necessary training materials" ] print(f"Onboarding Checklist for {employee_name} (Start Date: {start_date}):") for item in checklist: print(f"- {item}") create_onboarding_checklist('Jane Smith', '2023-10-01')
In addition to checklists, automating document collection very important for a smooth onboarding experience. This can be accomplished by creating a simple form using the Flask web framework that collects necessary documents from new hires. Here’s a basic example of how to set up a form for document uploads:
from flask import Flask, request, redirect, url_for import os app = Flask(__name__) app.config['UPLOAD_FOLDER'] = 'uploads/' @app.route('/upload', methods=['GET', 'POST']) def upload_file(): if request.method == 'POST': if 'file' not in request.files: return 'No file part' file = request.files['file'] if file.filename == '': return 'No selected file' file.save(os.path.join(app.config['UPLOAD_FOLDER'], file.filename)) return redirect(url_for('upload_file')) return ''' ''' if __name__ == '__main__': app.run(debug=True)
This Flask application allows new employees to upload their documents securely. Once the documents are collected, HR can move on to the next steps without delay.
Another automation opportunity lies in sending welcome emails and training materials. By using the smtplib library, HR can personalize welcome messages and include links to necessary training materials. Here’s how to automate sending a welcome email:
def send_welcome_email(to_email, employee_name): subject = "Welcome to the Team!" body = f"Dear {employee_name},nnWelcome aboard! We are excited to have you with us. Please find your training materials attached.nnBest,nThe Team" send_email(to_email, subject, body) send_welcome_email('[email protected]', 'Jane Smith')
A comprehensive onboarding experience can have a lasting impact on employee satisfaction and retention. By automating these tasks with Python, HR departments can ensure that new hires feel welcomed, informed, and supported from day one, thus fostering a positive work environment right from the start.
Enhancing Payroll Management through Automation
Payroll management is a vital function within any HR department, often characterized by its complexity and the potential for human error. Automating payroll processes with Python can not only alleviate these issues but also enhance accuracy and efficiency. By using Python’s capabilities, HR professionals can ensure timely salary disbursements, compliance with regulations, and accurate record-keeping—all while reducing the time spent on manual tasks.
One of the first steps in automating payroll management is to consolidate employee data, including hours worked, salary rates, and deductions. Using a CSV file to store this information is a simpler approach. The pandas
library can be extremely useful for reading and manipulating this data. Here’s a basic example of how to read employee payroll data from a CSV file:
import pandas as pd def load_payroll_data(file_path): return pd.read_csv(file_path) payroll_data = load_payroll_data('payroll.csv') print(payroll_data.head())
With the payroll data loaded, you can easily compute salaries for the pay period. This can include calculations for overtime, bonuses, and deductions such as taxes and insurance. Here’s an example of how to compute the total salary for each employee:
def calculate_salary(row): base_salary = row['salary'] overtime_hours = row['overtime_hours'] overtime_rate = base_salary * 1.5 # Assume 1.5 times the base rate for overtime total_salary = base_salary + (overtime_hours * overtime_rate) return total_salary payroll_data['total_salary'] = payroll_data.apply(calculate_salary, axis=1) print(payroll_data[['name', 'total_salary']])
Next, automating the generation of payslips for each employee can further streamline payroll management. A simple Python script can create personalized payslips in PDF format using the FPDF
library. Here’s how you can generate a payslip:
from fpdf import FPDF def generate_payslip(employee): pdf = FPDF() pdf.add_page() pdf.set_font("Arial", size=12) pdf.cell(200, 10, txt=f"Payslip for {employee['name']}", ln=True) pdf.cell(200, 10, txt=f"Total Salary: ${employee['total_salary']:.2f}", ln=True) pdf.output(f"{employee['name']}_payslip.pdf") for index, employee in payroll_data.iterrows(): generate_payslip(employee)
In addition to generating payslips, automating the distribution of these documents can save valuable time. Using the smtplib
library, you can send emails containing the payslips as attachments. Here’s an example of how to send a payslip via email:
import smtplib from email.mime.multipart import MIMEMultipart from email.mime.base import MIMEBase from email import encoders def send_payslip(to_email, file_path): msg = MIMEMultipart() msg['From'] = '[email protected]' msg['To'] = to_email msg['Subject'] = "Your Payslip" with open(file_path, 'rb') as file: part = MIMEBase('application', 'octet-stream') part.set_payload(file.read()) encoders.encode_base64(part) part.add_header('Content-Disposition', f'attachment; filename={file_path}') msg.attach(part) with smtplib.SMTP('smtp.example.com', 587) as server: server.starttls() server.login('[email protected]', 'password') server.send_message(msg) for index, employee in payroll_data.iterrows(): send_payslip(employee['email'], f"{employee['name']}_payslip.pdf")
Finally, maintaining compliance with tax regulations and deadlines is essential in payroll management. Python can be employed to automate the calculation of tax liabilities and submission of necessary filings. By integrating Python with APIs from tax authorities, HR professionals can ensure that all payroll activities comply with regulations, further minimizing the risk of penalties.
By employing these automation techniques, HR departments can transform payroll management from a burdensome task into a streamlined process, freeing up time to focus on strategic initiatives that enhance employee satisfaction and organizational success.
Using Data Analysis for Employee Engagement
In the realm of employee engagement, using data analysis through Python can provide profound insights that drive employee satisfaction and retention. Understanding how employees feel about their workplace and identifying areas for improvement are critical factors in maintaining a motivated workforce. Python, with its rich ecosystem of data analysis libraries, offers the tools necessary to delve into employee feedback, performance metrics, and engagement surveys effectively.
One of the first steps in analyzing employee engagement data is to gather feedback through surveys. Organizations often employ platforms like SurveyMonkey or Google Forms to collect data. Once the data is collected, it can be exported into a CSV format for analysis. The pandas library in Python is particularly useful for handling such datasets. Here’s a snippet showing how to load and examine survey responses:
import pandas as pd def load_survey_data(file_path): return pd.read_csv(file_path) survey_data = load_survey_data('employee_engagement_survey.csv') print(survey_data.head())
After loading the data, you can perform descriptive analysis to get a sense of overall employee sentiment. This can be achieved by calculating metrics such as average satisfaction scores, response rates, and identifying common themes in open-ended responses. The next step might involve visualizing this data to communicate insights effectively. Libraries like Matplotlib or Seaborn can be invaluable for creating visual representations. Here’s an example of generating a simple bar chart for satisfaction scores:
import matplotlib.pyplot as plt def plot_satisfaction_scores(data): scores = data['satisfaction_score'].value_counts().sort_index() plt.bar(scores.index, scores.values) plt.xlabel('Satisfaction Score') plt.ylabel('Number of Responses') plt.title('Employee Satisfaction Scores') plt.show() plot_satisfaction_scores(survey_data)
Furthermore, sentiment analysis can be employed on textual feedback to gauge overall sentiment. The Natural Language Toolkit (nltk) library can aid in processing text data. By analyzing the sentiment of open-ended responses, HR professionals can pinpoint specific issues or areas of concern. Here’s an example of how to perform basic sentiment analysis:
from nltk.sentiment import SentimentIntensityAnalyzer def analyze_sentiment(text): sia = SentimentIntensityAnalyzer() return sia.polarity_scores(text) survey_data['sentiment'] = survey_data['open_ended_feedback'].apply(analyze_sentiment) print(survey_data[['open_ended_feedback', 'sentiment']])
Once insights are obtained, it’s essential to communicate findings to the relevant stakeholders effectively. This can be done through automated reporting. By generating periodic reports summarizing employee engagement metrics, HR can foster a culture of transparency and continuous improvement. Here’s how to automate the generation of a summary report:
def generate_engagement_report(data): average_score = data['satisfaction_score'].mean() total_responses = data.shape[0] report = f"Employee Engagement Report:nAverage Satisfaction Score: {average_score:.2f}nTotal Responses: {total_responses}n" with open('engagement_report.txt', 'w') as file: file.write(report) generate_engagement_report(survey_data)
By employing these data analysis techniques, HR professionals can harness the power of Python to gain actionable insights into employee engagement. This not only helps in addressing current concerns but also informs strategies for improving the overall workplace environment, ultimately leading to a more engaged and productive workforce.
Implementing Python Scripts for Performance Tracking
Performance tracking is an essential function of any HR department, providing valuable insights into employee productivity and effectiveness. Implementing Python scripts for performance tracking can automate the collection and analysis of performance data, making it easier to identify trends, recognize high achievers, and support those who may need improvement. By using the power of Python, HR professionals can transform performance tracking from a cumbersome manual process into a streamlined and data-driven operation.
One of the first steps in automating performance tracking is to consolidate data from various sources, such as employee evaluations, project outcomes, and productivity metrics. A common approach is to use CSV files or databases to store this information. The pandas library in Python makes it simpler to load and manipulate performance data. Here’s an example of how to load employee performance data from a CSV file:
import pandas as pd def load_performance_data(file_path): return pd.read_csv(file_path) performance_data = load_performance_data('performance_data.csv') print(performance_data.head())
Once the performance data is loaded, you can analyze it to calculate key performance indicators (KPIs) such as average performance scores, completion rates for goals, and improvements over time. This analysis can be performed using simple aggregation functions in pandas. Here’s an example of calculating the average performance score for each employee:
def calculate_average_scores(data): return data.groupby('employee_id')['performance_score'].mean().reset_index() average_scores = calculate_average_scores(performance_data) print(average_scores)
In addition to calculating averages, it’s beneficial to visualize performance trends over time. Libraries like Matplotlib or Seaborn can help create visual representations of performance data. For instance, you can generate a line chart showing the progression of an employee’s performance score across several evaluation periods:
import matplotlib.pyplot as plt def plot_performance_trends(data, employee_id): employee_data = data[data['employee_id'] == employee_id] plt.plot(employee_data['evaluation_period'], employee_data['performance_score']) plt.title(f'Performance Trend for Employee {employee_id}') plt.xlabel('Evaluation Period') plt.ylabel('Performance Score') plt.xticks(rotation=45) plt.grid(True) plt.show() plot_performance_trends(performance_data, 101) # Example for employee with ID 101
Automating the performance review process can also enhance efficiency. Instead of relying on manual evaluations, you can create a Python script that automatically compiles performance data and generates reports. For example, you can generate a PDF report for each employee summarizing their performance over a given period:
from fpdf import FPDF def generate_performance_report(employee_id, name, performance_scores): pdf = FPDF() pdf.add_page() pdf.set_font("Arial", size=12) pdf.cell(200, 10, txt=f"Performance Report for {name} (ID: {employee_id})", ln=True) for period, score in performance_scores.items(): pdf.cell(200, 10, txt=f"{period}: {score}", ln=True) pdf.output(f"{name}_performance_report.pdf") # Example usage employee_scores = performance_data[performance_data['employee_id'] == 101].set_index('evaluation_period')['performance_score'].to_dict() generate_performance_report(101, 'Mitch Carter', employee_scores)
Lastly, integrating feedback mechanisms into performance tracking can greatly enhance the process. By sending automated reminders for feedback submissions or evaluations using the smtplib library, HR can ensure that all necessary information is collected promptly. Here’s how to automate the sending of feedback request emails:
import smtplib from email.mime.text import MIMEText def send_feedback_request(to_email, employee_name): subject = "Performance Feedback Request" body = f"Dear {employee_name},nnPlease provide your feedback for the recent performance evaluation.nnThank you!" msg = MIMEText(body) msg['Subject'] = subject msg['From'] = '[email protected]' msg['To'] = to_email with smtplib.SMTP('smtp.example.com', 587) as server: server.starttls() server.login('[email protected]', 'password') server.send_message(msg) send_feedback_request('[email protected]', 'Mitch Carter')
By adopting these automation techniques, HR departments can ensure that performance tracking is not only comprehensive and accurate but also conducive to continuous employee development. Automation in performance tracking enables a proactive approach to employee management, fostering a culture of accountability and growth within the organization.