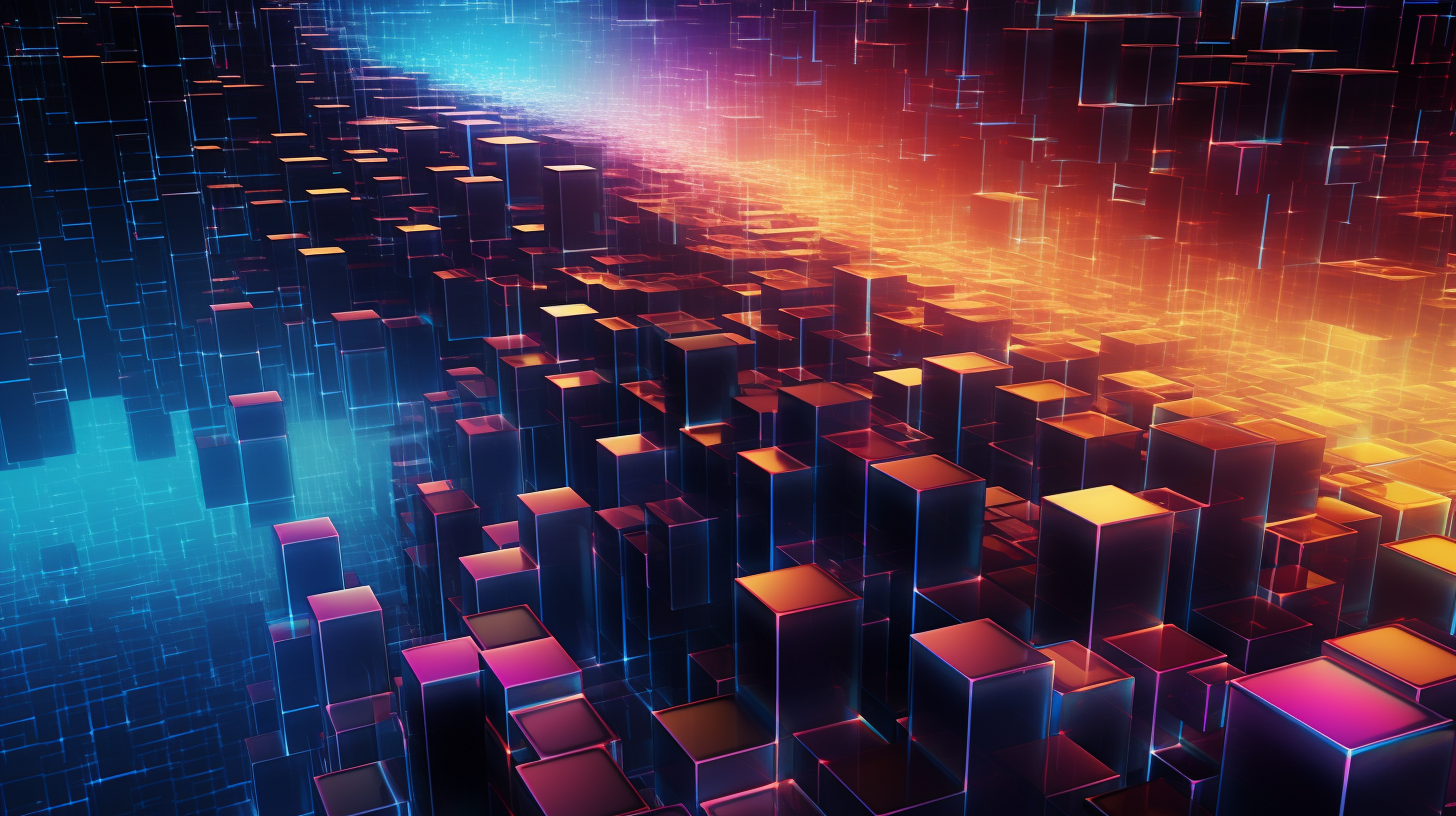
Python for Journalism: Data Analysis and Visualization
Data journalism stands at the intersection of journalism and data science, transforming numbers and statistics into compelling narratives that engage and inform the public. In this realm, journalists utilize data as a primary source, much like they would quotes from interviews or documents. This approach allows for uncovering trends, patterns, and insights that might otherwise go unnoticed.
At its core, data journalism is about asking questions and seeking answers through data. It involves not just the collection of data but also its analysis and presentation in a way that makes sense to the audience. Journalists must possess a critical eye to discern what data is relevant and how it can be leveraged to tell a story.
Ponder a scenario where a journalist investigates the impact of a new city policy on homelessness. The reporter might gather data from various sources, such as government reports, nonprofit organizations, and academic studies. By cleaning, analyzing, and visualizing this data, they can illustrate the policy’s effects over time, revealing trends that inform the public and policymakers alike.
Understanding data journalism also means recognizing its ethical implications. Journalists must navigate the complexities of data privacy, the accuracy of data sources, and the potential for misinterpretation. The trustworthiness of a story often hinges on the integrity of the data behind it. Thus, data journalists must ensure that their findings are transparent and reproducible.
As the field of journalism evolves, so does the need for proficiency in data handling. Modern journalists must not only possess traditional reporting skills but also be equipped with quantitative abilities and a working knowledge of data analysis tools. Python, with its rich ecosystem of libraries for data manipulation and visualization, becomes an indispensable ally for data journalists.
The ability to quickly analyze datasets, create visual representations, and generate insights can significantly enhance the storytelling process. In the following sections, we will explore essential Python libraries that facilitate these tasks, equipping journalists with the tools necessary to thrive in the data-driven world.
Essential Python Libraries for Data Analysis
To effectively dive into data analysis, Python offers a plethora of libraries tailored to various aspects of data manipulation and visualization. These libraries not only simplify complex tasks but also enhance the overall efficiency of data journalism. Here are some of the essential Python libraries that every data journalist should ponder integrating into their toolkit:
Pandas is perhaps the most crucial library for data analysis in Python. It provides powerful data structures such as DataFrames, which allow for easy manipulation, cleaning, and analysis of structured data. The intuitive syntax of Pandas makes it simpler to filter, aggregate, and transform data. Below is an example of how to use Pandas to load a dataset and perform some basic operations:
import pandas as pd # Load a CSV file into a DataFrame df = pd.read_csv('data/homelessness_data.csv') # Display the first five rows of the DataFrame print(df.head()) # Group by a relevant column and calculate the mean average_homelessness = df.groupby('year')['number_of_homeless'].mean() print(average_homelessness)
NumPy complements Pandas by providing support for numerical operations and array manipulations. It’s particularly beneficial when dealing with large datasets requiring mathematical computations. NumPy arrays are much faster than Python lists, making them perfect for performance-critical applications. Here’s an example of using NumPy to perform basic statistical analysis:
import numpy as np # Example data: number of homeless individuals over several years data = np.array([200, 250, 300, 400, 350]) # Calculate mean and standard deviation mean_homeless = np.mean(data) std_homeless = np.std(data) print(f'Mean: {mean_homeless}, Standard Deviation: {std_homeless}
Matplotlib and Seaborn are both excellent libraries for data visualization. While Matplotlib is the foundational plotting library that allows for extensive customization of plots, Seaborn builds on Matplotlib to provide a high-level interface for drawing attractive statistical graphics. Here’s how you can use these libraries to visualize trends in homelessness data:
import matplotlib.pyplot as plt import seaborn as sns # Example DataFrame with years and number of homeless individuals years = df['year'] homeless_count = df['number_of_homeless'] # Create a line plot with Matplotlib plt.figure(figsize=(10, 6)) plt.plot(years, homeless_count, marker='o') plt.title('Trends in Homelessness Over Time') plt.xlabel('Year') plt.ylabel('Number of Homeless Individuals') plt.grid() plt.show() # Create a more advanced visualization with Seaborn sns.lineplot(x=years, y=homeless_count, marker='o') plt.title('Trends in Homelessness Over Time') plt.xlabel('Year') plt.ylabel('Number of Homeless Individuals') plt.show()
Scikit-learn is essential for data journalists interested in predictive modeling and machine learning. This library provides simple and efficient tools for data mining and analysis, allowing journalists to build models that can make predictions based on historical data. Below is an example of how to use Scikit-learn to perform a simple linear regression:
from sklearn.linear_model import LinearRegression # Preparing data for linear regression X = df[['year']].values.reshape(-1, 1) # Features y = df['number_of_homeless'].values # Target variable # Creating a linear regression model model = LinearRegression() model.fit(X, y) # Making predictions predictions = model.predict(X) # Plotting the results plt.scatter(X, y, color='blue') plt.plot(X, predictions, color='red') plt.title('Linear Regression on Homelessness Data') plt.xlabel('Year') plt.ylabel('Number of Homeless Individuals') plt.show()
Each of these libraries plays a pivotal role in the data journalism process, empowering journalists to uncover insights, visualize data effectively, and ultimately tell compelling stories backed by evidence. By using these tools, journalists can elevate their reporting and provide a clear, data-driven narrative that resonates with their audience.
Techniques for Data Cleaning and Preparation
Data cleaning and preparation serve as the foundation for effective data analysis in journalism. This critical phase involves transforming raw data into a structured format suitable for analysis. Often, real-world data comes with imperfections: missing values, inconsistent formatting, and erroneous entries. Properly addressing these issues not only enhances the quality of the analysis but also ensures the integrity of the resulting narratives.
The first step in cleaning data typically involves identifying and handling missing values. In journalism, missing data can skew findings and misrepresent the story. Pandas provides various methods to manage these gaps. For example, one can choose to fill these gaps with an average value or drop the affected rows altogether. Here’s how you can do this:
import pandas as pd # Load the dataset df = pd.read_csv('data/homelessness_data.csv') # Check for missing values print(df.isnull().sum()) # Fill missing values in the 'number_of_homeless' column with the mean df['number_of_homeless'].fillna(df['number_of_homeless'].mean(), inplace=True) # Alternatively, drop rows with missing values df.dropna(inplace=True)
Next, data preparation often includes data type conversion. For instance, dates might be read as strings but should be converted to datetime objects for accurate analysis. This conversion enables more effective operations such as sorting and filtering by date. Here’s how to convert a column:
# Convert 'date' column to datetime format df['date'] = pd.to_datetime(df['date'])
Normalization is another essential technique, especially when dealing with numerical data across different scales. This process transforms features to a common scale, which can improve the performance of machine learning models. You can normalize data using the MinMaxScaler from Scikit-learn:
from sklearn.preprocessing import MinMaxScaler # Initialize the scaler scaler = MinMaxScaler() # Normalize the 'number_of_homeless' column df['normalized_homeless'] = scaler.fit_transform(df[['number_of_homeless']])
Another aspect of data preparation is categorization. Categorical data needs to be encoded into numerical formats for analysis. Techniques such as one-hot encoding are frequently used to convert categorical variables into a format that can be provided to machine learning algorithms. Here’s how you can implement one-hot encoding with Pandas:
# One-hot encode a categorical column 'city' df = pd.get_dummies(df, columns=['city'], drop_first=True)
Cleaning and preparing data is not just a technical necessity but an ethical responsibility for journalists. Every decision made during this phase can impact the story’s narrative. Ensuring data accuracy can prevent misleading conclusions and foster trust with the audience. As journalists work with data, they must remain vigilant about the methods and choices they employ in this preparatory stage.
Effective data cleaning and preparation are integral for any data journalism project. With the right techniques and tools, journalists can transform raw data into a well-structured dataset, paving the way for insightful analysis and compelling stories. The next step will delve into visualizing data with Python, where we will explore various tools and techniques that bring data-driven narratives to life.
Visualizing Data with Python: Tools and Techniques
In the context of data journalism, visualization serves as the bridge between raw numbers and compelling storytelling. The ability to present data in an easily digestible format allows journalists to uncover patterns and insights that resonate with their audience. Python, with its rich array of libraries, provides an arsenal of tools for crafting visuals that can highlight trends, reveal correlations, and emphasize key findings.
Matplotlib is the cornerstone of data visualization in Python. Its flexibility and extensive capabilities allow for the creation of a wide variety of plots and charts. The library empowers journalists to customize every aspect of their visualizations, from colors and fonts to labels and legends. Below is a simple example of generating a bar chart to compare the number of homeless individuals across different cities:
import matplotlib.pyplot as plt # Example data cities = ['City A', 'City B', 'City C'] homeless_count = [150, 200, 300] # Create a bar chart plt.figure(figsize=(8, 5)) plt.bar(cities, homeless_count, color='skyblue') plt.title('Homeless Count by City') plt.xlabel('City') plt.ylabel('Number of Homeless Individuals') plt.show()
For more statistically-inclined visualizations, Seaborn simplifies the process while enhancing aesthetics. It integrates seamlessly with Pandas DataFrames, making it a favorite among data journalists. Here’s an example of using Seaborn to create a boxplot, which can illustrate the distribution of homeless numbers across cities, showcasing any outliers and variability:
import seaborn as sns # Assuming df contains 'city' and 'number_of_homeless' columns plt.figure(figsize=(10, 6)) sns.boxplot(x='city', y='number_of_homeless', data=df) plt.title('Distribution of Homeless Numbers by City') plt.xlabel('City') plt.ylabel('Number of Homeless Individuals') plt.show()
Another widely used library is Plotly, which enables the creation of interactive visualizations. This interactivity allows users to engage with the data, facilitating a deeper understanding. Plotly can be an effective tool for presentations or web stories where audience engagement is key. Here’s how to create an interactive scatter plot:
import plotly.express as px # Scatter plot with Plotly fig = px.scatter(df, x='year', y='number_of_homeless', color='city', title='Homelessness Over Time by City') fig.show()
Furthermore, when it comes to geographical data, libraries like Folium can visualize data on maps, adding another layer of depth to the storytelling. For instance, one could visualize homelessness data across different neighborhoods within a city, providing a geographical context to the statistics. Below is an example of how to create a simple map with Folium:
import folium # Create a base map m = folium.Map(location=[latitude, longitude], zoom_start=12) # Add markers for each neighborhood with homelessness data for _, row in df.iterrows(): folium.Marker([row['latitude'], row['longitude']], popup=f"{row['neighborhood']}: {row['number_of_homeless']}").add_to(m) # Display the map m.save('homelessness_map.html')
By employing these visualization techniques, data journalists can transform complex datasets into accessible and engaging visual narratives. The right visualization not only enhances comprehension but also drives home the emotional impact of the data, making the story more relatable and urgent to the audience.
In a rapidly evolving media landscape, where visual content reigns supreme, the ability to visualize data effectively becomes an important skill for journalists. Armed with Python’s powerful visualization libraries, journalists can present data-driven stories that not only inform but also inspire action and awareness among their readers.
Case Studies: Successful Data-Driven Journalism Projects
Data-driven journalism has seen a high number of successful projects that have not only informed the public but also prompted action and policy change. These case studies serve as powerful examples of how data, when analyzed and visualized effectively, can create compelling narratives that resonate with audiences. One hallmark case is the “Pulitzer Prize-winning reporting by The New York Times on the COVID-19 pandemic.” Their team utilized a combination of data from various public health sources to track and visualize the spread of the virus across the United States. By employing sophisticated data analysis techniques and clear visualizations, they were able to convey the urgency and gravity of the situation, ultimately influencing public behavior and policy.
Another exemplary project is the “Guardian’s ‘The Counted’ initiative,” which aimed to track the number of people killed by police in the United States. In this project, reporters collected data from multiple sources, including social media and public records, to compile a comprehensive database. They utilized Python libraries like Pandas for data manipulation and Matplotlib for visualizations, creating an interactive map that highlighted the tragic impact of police violence. This initiative not only raised awareness but also fueled discussions about police reform and accountability.
Similarly, “ProPublica’s ‘Machine Bias’ investigation” demonstrated how algorithms used in the criminal justice system may show bias against certain racial groups. By analyzing data collected from multiple jurisdictions, the journalists uncovered discrepancies in how risk assessments were applied to different demographics. The findings were visualized using Seaborn and Plotly, allowing readers to interact with the data and understand the implications of algorithmic bias. This investigation sparked national conversations about the fairness of algorithmic decision-making in law enforcement.
Lastly, the “Chicago Tribune’s data-driven investigation into the city’s tax increment financing (TIF) program” showcased how data journalism can hold power to account. By analyzing public records and financial data, the journalists exposed how TIFs, intended to promote urban development, were often misallocated, resulting in significant losses for the city. Using Python’s visualization libraries, they created detailed graphs and maps that illustrated the disparity in funding and the consequences for communities. The impact of this investigation led to increased scrutiny of the TIF program and calls for reform.
These case studies illustrate that data journalism is not merely about the numbers; it is about the stories those numbers reveal. The ability to harness data effectively can empower journalists to uncover truths that might otherwise remain hidden. Through meticulous analysis and thoughtful visualization, data journalists can engage their audiences, shed light on pressing issues, and inspire meaningful change.