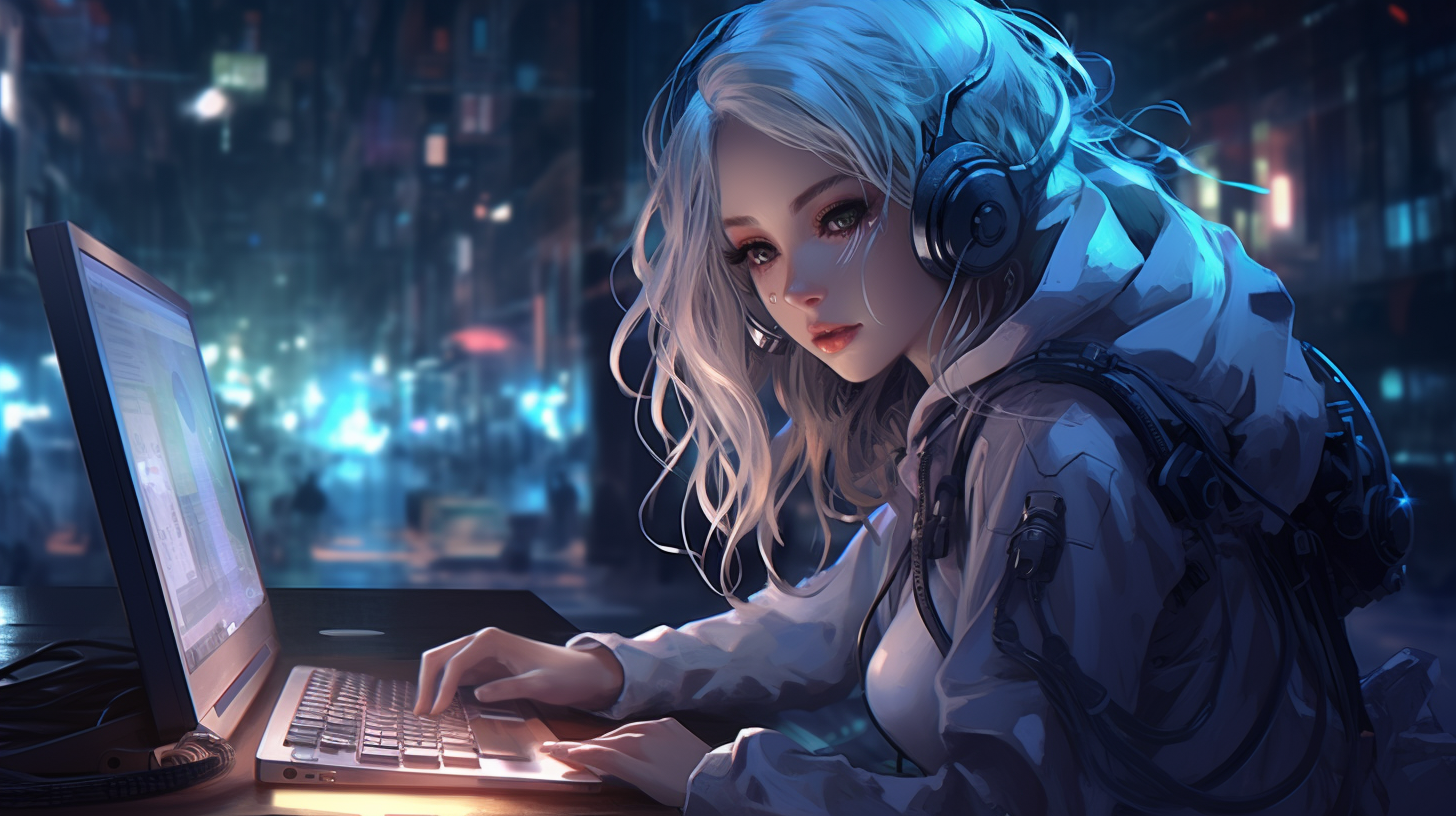
Python for Machine Learning: Getting Started
Machine learning is a fascinating subset of artificial intelligence that empowers computers to learn from data rather than relying solely on explicit programming. This process revolves around developing algorithms capable of recognizing patterns and making decisions based on input data. To grasp the fundamental concepts of machine learning, it is essential to understand a few key terms and mechanisms.
Supervised Learning is where the model learns from labeled data. Imagine having a dataset where each input is paired with the correct output, like predicting housing prices based on certain features such as size, location, and age. The algorithm makes predictions based on this input-output pairing, gradually improving its accuracy over time.
In contrast, Unsupervised Learning deals with unlabeled data. Here, the model attempts to identify patterns and groupings without predefined labels—think of clustering customers based on purchasing behavior. This can be incredibly powerful for identifying hidden structures in data.
Reinforcement Learning is another interesting paradigm. In this approach, an agent learns to make decisions by taking actions in an environment to maximize some notion of cumulative reward. That is akin to training a dog: give it treats for good behavior, and it will learn to repeat those actions.
Every machine learning model consists of two main stages: training and inference. During training, the model learns from historical data, adjusting its parameters to minimize error. Once trained, it transitions to inference, where it applies this learning to make predictions on unseen data.
To illustrate these concepts, ponder a simple example using a linear regression model. This algorithm attempts to fit a line through a set of data points, allowing predictions for new input values based on the learned relationship.
import numpy as np import matplotlib.pyplot as plt from sklearn.linear_model import LinearRegression # Generate some synthetic data x = np.array([[1], [2], [3], [4], [5]]) y = np.array([1.5, 1.7, 3.2, 3.8, 5.1]) # Create and train the model model = LinearRegression() model.fit(x, y) # Make predictions predictions = model.predict(np.array([[6], [7]])) # Visualize the results plt.scatter(x, y, color='blue') plt.plot(x, model.predict(x), color='red') plt.scatter([[6], [7]], predictions, color='green') plt.title("Linear Regression Example") plt.xlabel("Input (X)") plt.ylabel("Output (y)") plt.show()
This code snippet generates a simple linear regression model using synthetic data. The model learns the relationship between the input variable x
and the output y
, allowing it to predict future values. As you dive deeper into machine learning, you will encounter various models and techniques, each tailored to specific types of data and problem domains.
Setting Up Your Python Environment
Before delving into machine learning, it is crucial to establish a solid Python environment that supports efficient development and experimentation. Setting up your Python environment involves several key steps, from installing Python itself to configuring libraries that streamline your machine learning tasks.
First, you’ll want to download and install the latest version of Python. The official Python website offers installers for various operating systems. It’s generally recommended to install Python via the Anaconda distribution, as it comes bundled with a plethora of essential scientific libraries and tools, making it a favorite among data scientists.
Once you have Anaconda installed, you can create isolated environments for your projects, ensuring that dependencies do not clash with one another. That’s particularly useful in machine learning, as different projects may require different versions of libraries. You can create a new environment by running the following command in your terminal:
conda create -n my_ml_env python=3.8
After the environment is created, activate it using:
conda activate my_ml_env
Next, you’ll need to install the key libraries required for machine learning. Some fundamental packages include NumPy, Pandas, Matplotlib, and Scikit-learn. You can install these libraries easily using conda as follows:
conda install numpy pandas matplotlib scikit-learn
For deep learning specifically, libraries like TensorFlow or PyTorch are necessary. They can be installed similarly:
conda install tensorflow
conda install pytorch torchvision torchaudio -c pytorch
Now that your environment is set up and libraries are installed, it’s advisable to use an Integrated Development Environment (IDE) or a notebook interface for your work. Jupyter Notebook is highly popular in the data science community for its interactivity and ease of use. You can install Jupyter using the following command:
conda install jupyter
Once Jupyter is installed, you can launch it by running:
jupyter notebook
This will open a web interface where you can create new notebooks and start writing your machine learning code right away. With this setup, you will be well-equipped to explore the vast landscape of machine learning algorithms, preprocess data, and refine your models effectively.
Key Libraries for Machine Learning in Python
When it comes to machine learning in Python, several key libraries serve as the backbone for building robust models and performing data analysis. Each library provides unique functionalities that address different aspects of machine learning, from data manipulation to model training and evaluation. Understanding these libraries is essential for anyone looking to embark on a machine learning journey.
NumPy is one of the foundational libraries in Python for numerical computing. It offers support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. NumPy is important for handling the data that machine learning models will process. It optimizes performance through its array-oriented computing capabilities, making it more efficient than standard Python lists.
import numpy as np # Create a NumPy array data = np.array([1, 2, 3, 4, 5]) print("NumPy Array:", data)
Pandas builds on the capabilities of NumPy by providing data structures like DataFrames, which allow for easy data manipulation and analysis. It simplifies data loading, filtering, cleaning, and transformation. In machine learning, Pandas plays an important role in preparing datasets, so that you can handle missing values and perform exploratory data analysis (EDA).
import pandas as pd # Create a DataFrame data = {'Feature1': [1, 2, 3], 'Feature2': [4, 5, 6]} df = pd.DataFrame(data) print("Pandas DataFrame:") print(df)
Matplotlib and Seaborn are essential libraries for data visualization. Matplotlib provides low-level plotting capabilities, allowing for the creation of a wide range of static, animated, and interactive plots. Seaborn builds on Matplotlib and offers a higher-level interface, making it easier to generate attractive and informative statistical graphics. Visualizing data is a key step in understanding patterns and relationships before diving into model training.
import matplotlib.pyplot as plt import seaborn as sns # Sample data x = [1, 2, 3, 4, 5] y = [5, 4, 3, 2, 1] # Create a simple line plot plt.plot(x, y) plt.title("Simple Line Plot") plt.xlabel("X-axis") plt.ylabel("Y-axis") plt.show()
Scikit-learn is perhaps the most widely used library for machine learning in Python. It provides a simple and consistent interface for a variety of algorithms for classification, regression, clustering, and more. Additionally, Scikit-learn includes tools for model evaluation, validation, and hyperparameter tuning, making it a comprehensive framework for building and deploying machine learning models.
from sklearn.linear_model import LinearRegression # Sample data X = np.array([[1], [2], [3], [4], [5]]) y = np.array([1, 2, 3, 4, 5]) # Create and train a linear regression model model = LinearRegression() model.fit(X, y) # Make predictions predictions = model.predict(np.array([[6], [7]])) print("Predictions for 6 and 7:", predictions)
TensorFlow and PyTorch are the leading libraries for deep learning applications. TensorFlow, developed by Google, provides a rich ecosystem for building and deploying machine learning models at scale. PyTorch, developed by Facebook, is favored for its usability and dynamic computational graph, making it particularly appealing for research and experimentation. Both libraries support GPU acceleration, which especially important for training large models efficiently.
Each of these libraries plays a significant role in the machine learning workflow, from data preprocessing to model training and visualization. By mastering these tools, you will be well-equipped to tackle a wide range of machine learning problems and develop effective solutions in Python.
Data Preprocessing Techniques
Data preprocessing is a critical step in the machine learning pipeline, serving as the bridge between raw data and model training. The performance of any machine learning model heavily relies on the quality of the data it’s trained on. Without proper preprocessing, even the most sophisticated algorithms may yield poor results. This stage involves cleaning the data, transforming it, and preparing it for analysis. Let’s delve into some fundamental preprocessing techniques you should be familiar with.
First, handling missing values is paramount. Real-world datasets are often incomplete, and machine learning algorithms typically require a complete set of data to function correctly. There are several strategies to deal with missing values. One common approach is to impute them with mean, median, or mode values, depending on the data distribution and context. Alternatively, you may consider dropping rows or columns with a significant number of missing values. Here’s a quick example using Pandas:
import pandas as pd import numpy as np # Create a DataFrame with missing values data = {'Feature1': [1, 2, np.nan, 4], 'Feature2': [5, np.nan, 7, 8]} df = pd.DataFrame(data) # Impute missing values with mean df.fillna(df.mean(), inplace=True) print("DataFrame after imputation:") print(df)
Next comes data normalization or scaling. Features in a dataset can vary significantly in scale, which can adversely affect the performance of many machine learning algorithms, particularly those based on distance calculations such as K-Nearest Neighbors. Two prevalent methods for scaling are Min-Max Scaling and Standardization. Min-Max Scaling rescales the features to a fixed range, typically [0, 1], while Standardization transforms the data to have a mean of 0 and a standard deviation of 1. Here’s how to apply Standardization using Scikit-learn:
from sklearn.preprocessing import StandardScaler # Sample dataset X = np.array([[1, 2], [2, 3], [3, 4], [4, 5]]) # Create a StandardScaler instance scaler = StandardScaler() # Fit and transform the data X_scaled = scaler.fit_transform(X) print("Scaled Data:") print(X_scaled)
Furthermore, encoding categorical variables is another essential preprocessing step. Machine learning algorithms typically require numerical input; hence, categorical variables must be converted into a numerical format. One common method is One-Hot Encoding, where a new binary column is created for each category. For instance, ponder a dataset with a ‘Color’ feature that can take values ‘Red’, ‘Blue’, and ‘Green’. By applying One-Hot Encoding, we can represent these categories numerically as follows:
# Sample DataFrame with categorical variable data = {'Color': ['Red', 'Blue', 'Green', 'Blue']} df = pd.DataFrame(data) # Perform One-Hot Encoding df_encoded = pd.get_dummies(df, columns=['Color']) print("DataFrame after One-Hot Encoding:") print(df_encoded)
Lastly, feature selection plays an important role in enhancing model performance by eliminating irrelevant or redundant features. This not only reduces overfitting but also improves the model’s interpretability. Techniques like Recursive Feature Elimination (RFE) or feature importance from tree-based models can be employed to determine which features contribute most to the prediction. Here’s an example using RFE with a Logistic Regression model:
from sklearn.datasets import load_iris from sklearn.linear_model import LogisticRegression from sklearn.feature_selection import RFE # Load dataset iris = load_iris() X, y = iris.data, iris.target # Create a Logistic Regression model model = LogisticRegression() # Create RFE model and select top 2 features rfe = RFE(model, 2) fit = rfe.fit(X, y) print("Selected Features:", fit.support_) print("Feature Ranking:", fit.ranking_)
By incorporating these preprocessing techniques into your workflow, you set a solid foundation for building robust machine learning models. The objective is to ensure that the data fed into your models is clean, well-structured, and encoded appropriately. As you progress, remember that effective data preprocessing is as critical as the choice of the model itself.
Building Your First Machine Learning Model
Now that we’ve laid the groundwork, now, let’s move forward and build your first machine learning model. This section will guide you through the essential steps to construct a basic machine learning model using the Scikit-learn library, illustrated with a practical example that showcases the process from start to finish.
To begin with, let’s assume we’re working with a classic dataset known as the Iris dataset. This dataset contains measurements for various species of iris flowers, including sepal length, sepal width, petal length, and petal width. Our task will be to create a model that can predict the species of an iris flower based on these measurements.
First, we need to load the dataset and inspect it to understand its structure. Scikit-learn provides a convenient function to load this data:
from sklearn.datasets import load_iris import pandas as pd # Load the Iris dataset iris = load_iris() X = iris.data # Features y = iris.target # Target variable # Create a DataFrame for better visualization df = pd.DataFrame(data=X, columns=iris.feature_names) df['species'] = y print(df.head())
With the dataset loaded, the next step is to split it into training and testing sets. This division is critical, as it allows us to train our model on one portion of the data and evaluate its performance on unseen data. The typical split ratio is 80/20, meaning 80% of the data will be used for training, and 20% for testing:
from sklearn.model_selection import train_test_split # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) print(f'Training set size: {X_train.shape[0]}') print(f'Testing set size: {X_test.shape[0]}')
Now that we have our training and testing sets prepared, now, let’s select a model. For our example, we will use the K-Nearest Neighbors (KNN) algorithm, which is intuitive and effective for classification tasks. We will instantiate the model and fit it to our training data:
from sklearn.neighbors import KNeighborsClassifier # Create a KNN model model = KNeighborsClassifier(n_neighbors=3) # Fit the model on the training data model.fit(X_train, y_train)
After fitting the model, we can now make predictions on the test set to evaluate its performance. This step involves using the `predict` method to produce class labels for the test set:
# Make predictions on the test set predictions = model.predict(X_test) print("Predictions:", predictions)
Next, we need to assess how well our model performed. A common metric for classification tasks is accuracy, which measures the proportion of correctly classified instances out of the total instances. We can calculate accuracy using Scikit-learn’s built-in functions:
from sklearn.metrics import accuracy_score # Calculate accuracy accuracy = accuracy_score(y_test, predictions) print(f'Accuracy: {accuracy * 100:.2f}%')
This accuracy score gives us insight into how well our model is performing on unseen data. Depending on the results, you might want to refine the model further by tuning parameters, selecting different algorithms, or employing more advanced techniques.
In this example, we successfully constructed a basic machine learning model using Scikit-learn, from data loading and preprocessing to model training and evaluation. As you gain more experience, you can explore different models and techniques, allowing you to tackle increasingly complex machine learning tasks with confidence.
Evaluating and Improving Model Performance
Evaluating and improving model performance is an important phase in the machine learning pipeline. Once you’ve built a model, simply observing its predictions isn’t enough; you need to quantify how well it performs and find ways to improve its capabilities. This involves not only assessing the model’s accuracy but also identifying potential shortcomings and implementing strategies to address them.
A standard approach to evaluate model performance is to use metrics that reflect how well the model predicts outcomes on unseen data. In classification tasks, accuracy is a frequently used metric, but it can be misleading, especially in cases of imbalanced datasets. In such scenarios, it’s essential to ponder additional metrics like precision, recall, and the F1 score.
Confusion Matrix
To visualize the performance of a classification model, a confusion matrix provides an excellent overview. This table summarizes the number of correct and incorrect predictions across actual classes. Using Scikit-learn, you can easily generate a confusion matrix and visualize it with Matplotlib and Seaborn:
from sklearn.metrics import confusion_matrix import seaborn as sns # Generate the confusion matrix cm = confusion_matrix(y_test, predictions) # Visualize the confusion matrix sns.heatmap(cm, annot=True, fmt='d', cmap='Blues', xticklabels=iris.target_names, yticklabels=iris.target_names) plt.title("Confusion Matrix") plt.xlabel("Predicted Label") plt.ylabel("True Label") plt.show()
The confusion matrix not only shows the overall accuracy but also helps identify which classes are being misclassified, guiding your next steps in model enhancement.
Cross-Validation
Another effective method to evaluate model performance is cross-validation, which involves partitioning the data into multiple subsets and training the model multiple times. This technique helps ensure that the model’s performance is consistent across different subsets of data. The most common form is k-fold cross-validation:
from sklearn.model_selection import cross_val_score # Perform k-fold cross-validation cv_scores = cross_val_score(model, X, y, cv=5) # 5-fold cross-validation print("Cross-Validation Scores:", cv_scores) print("Mean CV Score:", cv_scores.mean())
This approach gives you a more reliable estimate of the model’s generalization performance, as it evaluates the model on multiple train-test splits.
Hyperparameter Tuning
Once you have a baseline model, improving its performance often entails tuning hyperparameters. Many machine learning algorithms have parameters that can be adjusted to optimize performance, such as the number of neighbors in KNN, the depth of a decision tree, or learning rates in gradient boosting models.
Grid Search is a popular method for hyperparameter tuning. It allows you to define a grid of parameters and systematically evaluate all possible combinations:
from sklearn.model_selection import GridSearchCV # Define the parameter grid param_grid = {'n_neighbors': [1, 3, 5, 7, 9]} # Instantiate the GridSearchCV object grid_search = GridSearchCV(KNeighborsClassifier(), param_grid, cv=5) # Fit the model grid_search.fit(X_train, y_train) # Best parameters and score print("Best Parameters:", grid_search.best_params_) print("Best Cross-Validation Score:", grid_search.best_score_)
By employing Grid Search, you can often achieve a significant boost in model performance, ensuring that you’re using the most effective parameter settings.
Feature Selection
Improving model performance can also be achieved through feature selection, which involves identifying and retaining only the most relevant features while discarding the less informative ones. This not only reduces overfitting but can also improve the model’s interpretability. Techniques such as Recursive Feature Elimination (RFE) or using feature importance scores from tree-based models are effective ways to conduct feature selection.
from sklearn.ensemble import RandomForestClassifier from sklearn.feature_selection import RFE # Create a Random Forest model model_rf = RandomForestClassifier() # Create RFE and fit it rfe = RFE(model_rf, n_features_to_select=2) fit = rfe.fit(X, y) print("Selected Features:", fit.support_) print("Feature Ranking:", fit.ranking_)
This process helps in refining the model by focusing on features that contribute most to the prediction task, leading to improved performance.
Ultimately, the goal of evaluating and improving model performance is to improve the model’s ability to make accurate predictions on new, unseen data. Through careful assessment and systematic optimization of model parameters and features, you can elevate your machine learning models from basic benchmarks to high-performing solutions that yield valuable insights and predictions.