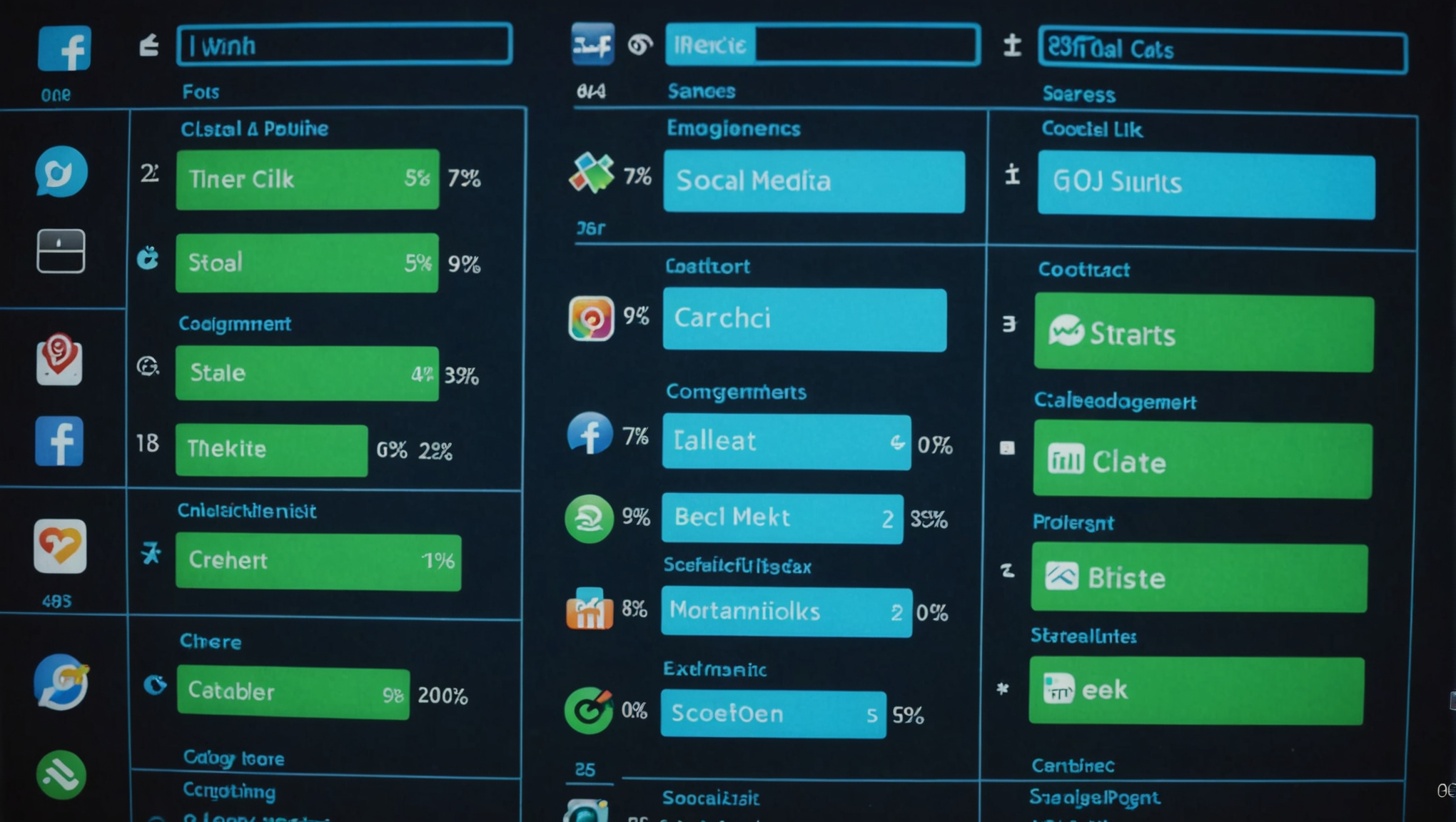
Python for Social Media Analysis: Techniques and Tools
When diving into the vast sea of social media data analysis, it’s imperative to equip yourself with the right tools. Python, being a versatile language, boasts an extensive ecosystem of libraries tailored for data manipulation, analysis, and visualization. Here are some essential libraries that will serve as your arsenal in decoding the intricate patterns hidden within social media data.
Pandas is a cornerstone library that provides data structures and functions needed to manipulate structured data effectively. It allows you to read, analyze, and visualize datasets in a way that’s both intuitive and efficient. The DataFrame structure in Pandas is particularly useful for handling tabular data, which is common in social media analysis.
import pandas as pd # Load a CSV file of social media data data = pd.read_csv('social_media_data.csv') # Display the first few rows of the dataset print(data.head())
NumPy is another indispensable library that adds support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. Its efficiency and speed make it ideal for handling numerical data, which is often the backbone of analysis.
import numpy as np # Create a NumPy array from a list of social media metrics metrics = np.array([100, 150, 200, 250]) # Calculate the mean engagement mean_engagement = np.mean(metrics) print("Mean Engagement:", mean_engagement)
Matplotlib and Seaborn are essential for visualization. While Matplotlib offers flexibility in creating a wide range of static, animated, and interactive plots, Seaborn builds on it by providing a high-level interface for drawing attractive statistical graphics. These libraries allow analysts to visualize data trends, sentiment distributions, and other insights effectively.
import matplotlib.pyplot as plt import seaborn as sns # Example dataset for visualizing social media engagement over time times = ['Jan', 'Feb', 'Mar', 'Apr', 'May'] engagements = [100, 150, 200, 250, 300] plt.figure(figsize=(10, 5)) sns.lineplot(x=times, y=engagements, marker='o') plt.title('Social Media Engagement Over Time') plt.xlabel('Month') plt.ylabel('Engagement') plt.show()
NLTK (Natural Language Toolkit) and TextBlob are powerful libraries for handling text data. NLTK offers functionalities for classification, tokenization, stemming, tagging, parsing, and semantic reasoning, making it a comprehensive library for natural language processing tasks. On the other hand, TextBlob simplifies these tasks, providing a more simple to operate interface for common operations like sentiment analysis.
from textblob import TextBlob # Analyzing sentiment of a social media post post = "I love using Python for social media analysis!" blob = TextBlob(post) # Print the polarity print("Sentiment Polarity:", blob.sentiment.polarity)
Tweepy is indispensable for accessing the Twitter API, which will allow you to gather tweets, user data, and more. This library simplifies API interactions, making it easier for you to collect relevant data for analysis.
import tweepy # Authenticate to Twitter auth = tweepy.OAuthHandler('consumer_key', 'consumer_secret') auth.set_access_token('access_token', 'access_token_secret') # Create API object api = tweepy.API(auth) # Fetch recent tweets with a specific hashtag tweets = api.search(q='#Python', count=10) for tweet in tweets: print(tweet.text)
These libraries, combined with your analytical mindset, can unlock profound insights from social media data, allowing you to uncover trends, gauge public sentiment, and make data-driven decisions. Each of these tools serves a distinct purpose, yet they harmoniously work together to provide a robust framework for social media analysis in Python.
Data Collection Methods from Social Media Platforms
In the realm of social media analysis, data collection is the foundational step that sets the stage for insightful analysis. Various methods exist for gathering data from social media platforms, and understanding these methods is important for any analyst aiming to extract meaningful insights.
APIs (Application Programming Interfaces) are the primary gateways to accessing social media data. Most major platforms like Twitter, Facebook, Instagram, and LinkedIn provide APIs that allow developers to programmatically interact with their services. The APIs generally allow you to retrieve posts, comments, user profiles, and various metrics related to interactions.
To illustrate this, let’s look at how to collect data from Twitter using the Tweepy library, which simplifies the process of working with the Twitter API. The following code snippet demonstrates how to authenticate and fetch recent tweets from a specific user’s timeline:
import tweepy # Replace these values with your own Twitter API keys consumer_key = 'your_consumer_key' consumer_secret = 'your_consumer_secret' access_token = 'your_access_token' access_token_secret = 'your_access_token_secret' # Authenticate to Twitter auth = tweepy.OAuthHandler(consumer_key, consumer_secret) auth.set_access_token(access_token, access_token_secret) # Create API object api = tweepy.API(auth) # Fetch recent tweets from your timeline public_tweets = api.home_timeline(count=5) for tweet in public_tweets: print(tweet.text)
Another method for data collection involves web scraping, which can be particularly useful when APIs do not provide sufficient access to the data you need. Libraries like Beautiful Soup and Scrapy are popular choices for scraping web pages. However, it’s essential to respect the terms of service of the platform you’re scraping from, as unauthorized scraping can lead to IP bans.
For example, here’s how you can use Beautiful Soup to scrape tweets from a public Twitter profile page:
import requests from bs4 import BeautifulSoup # URL of the Twitter profile to scrape url = 'https://twitter.com/username' response = requests.get(url) # Parse the page content soup = BeautifulSoup(response.content, 'html.parser') # Find and print tweets tweets = soup.find_all('div', class_='tweet') for tweet in tweets: content = tweet.find('p', class_='tweet-text').text print(content)
It’s worth noting that scraping comes with its challenges, including handling dynamic content loaded by JavaScript, which may require the use of additional tools like Selenium to interact with the webpage more effectively.
Furthermore, social media platforms often provide data export features that allow users to download their data in CSV or JSON formats. This method can be simpler for analysts who seek personal account data rather than large-scale data collection.
Lastly, consider using data aggregation platforms or services. These platforms often provide ready-made datasets or APIs that aggregate data from multiple social media channels, making it easier to access and analyze vast amounts of data without having to navigate the intricacies of each platform’s API.
Whether you’re fetching data via APIs, scraping web pages, using built-in export features, or using third-party services, understanding the available methods for data collection is vital for robust social media analysis. With these tools at your disposal, you can gather a wealth of data that serves as the foundation for deeper insights and analyses.
Text Mining and Sentiment Analysis Techniques
Text mining and sentiment analysis are pivotal components in extracting meaningful insights from the vast troves of social media data. As social media platforms are predominantly text-based, techniques in natural language processing (NLP) have become essential for deciphering the sentiments and opinions expressed by users. By employing robust text mining methods alongside sentiment analysis techniques, analysts can grasp public sentiment, identify trends, and even predict future behaviors based on the data collected.
Text mining begins with the preprocessing of the raw text data. This step especially important, as it helps eliminate noise and standardizes the text for further analysis. The tasks involved often include:
- Breaking the text into individual words or tokens.
- Filtering out common words that don’t contribute to the meaning, such as “and,” “the,” and “is.”
- Reducing words to their base or root form to consolidate variations of a word.
In Python, the NLTK library offers comprehensive tools for these preprocessing tasks. Here’s how you can perform tokenization and remove stop words:
import nltk from nltk.corpus import stopwords from nltk.tokenize import word_tokenize # Sample text from social media text = "Python is great for social media analysis!" # Tokenization tokens = word_tokenize(text) # Remove stop words nltk.download('stopwords') stop_words = set(stopwords.words('english')) filtered_tokens = [word for word in tokens if word.lower() not in stop_words] print("Filtered Tokens:", filtered_tokens)
Once the text is preprocessed, sentiment analysis can be performed to gauge the emotional tone of the data. Sentiment analysis typically categorizes text into positive, negative, or neutral sentiments. The TextBlob library simplifies the implementation of sentiment analysis in Python. Here’s an example of how to analyze the sentiment of a group of social media posts:
from textblob import TextBlob # Sample list of social media posts posts = [ "I love Python programming!", "This is the worst day ever.", "Python makes data analysis easy and fun.", "I am not sure how I feel about this." ] # Analyze sentiment for each post for post in posts: blob = TextBlob(post) print(f"Post: {post}nSentiment Polarity: {blob.sentiment.polarity}n")
In this example, each post is evaluated for its polarity score, which ranges from -1 (negative) to 1 (positive). This allows data analysts to quantify sentiments and derive actionable insights from the aggregated sentiment scores.
Furthermore, more sophisticated techniques in sentiment analysis leverage machine learning models. Libraries like Scikit-learn can be employed to train classifiers on labeled datasets, using features extracted from the text. For instance, the following snippet illustrates the training of a simple sentiment classifier:
from sklearn.feature_extraction.text import CountVectorizer from sklearn.naive_bayes import MultinomialNB from sklearn.pipeline import make_pipeline # Sample training data train_data = [ "I love the product.", "This is a terrible experience.", "Absolutely fantastic!", "I hate waiting in line." ] train_labels = [1, 0, 1, 0] # 1: positive, 0: negative # Create a model model = make_pipeline(CountVectorizer(), MultinomialNB()) # Train the model model.fit(train_data, train_labels) # Test with new data test_data = ["I enjoy this!", "I dislike the service."] predictions = model.predict(test_data) for review, sentiment in zip(test_data, predictions): print(f"Review: {review} | Sentiment: {'Positive' if sentiment == 1 else 'Negative'}")
This approach enables analysts to classify sentiments with greater accuracy and adapt to the evolving language used in social media. By using the power of Python’s libraries, analysts can efficiently perform text mining and sentiment analysis, turning unstructured social media data into structured insights, ready for further exploration and visualization.
Visualizing Social Media Insights with Python
Visualizing social media insights is a critical aspect of transforming raw data into comprehensible information. Effective visualization not only aids in better understanding of the data but also enhances communication of insights to stakeholders. Python provides a robust set of libraries that make it easy to create a variety of visualizations, catering to different needs and preferences.
To begin with, Matplotlib serves as the foundational library for creating static, animated, and interactive plots in Python. It provides unparalleled control over every aspect of a figure, allowing users to customize visualizations to their needs. Here’s a simple example illustrating how to visualize engagement metrics from social media data:
import matplotlib.pyplot as plt # Data for the visualization labels = ['Likes', 'Shares', 'Comments', 'Mentions'] values = [300, 150, 75, 90] # Creating a bar chart plt.figure(figsize=(8, 4)) plt.bar(labels, values, color=['blue', 'green', 'orange', 'red']) plt.title('Social Media Engagement Metrics') plt.xlabel('Engagement Type') plt.ylabel('Count') plt.show()
Seaborn enhances Matplotlib by offering a high-level interface for drawing attractive statistical graphics. It automatically manages complex visualizations and provides themes to improve the aesthetic allure of your plots. For instance, visualizing the distribution of sentiments derived from social media posts can be done as follows:
import seaborn as sns import pandas as pd # Sample data for sentiment analysis data = {'Sentiment': ['Positive', 'Negative', 'Neutral', 'Positive', 'Negative', 'Neutral', 'Positive']} df = pd.DataFrame(data) # Create a count plot plt.figure(figsize=(8, 4)) sns.countplot(x='Sentiment', data=df, palette='Set2') plt.title('Sentiment Distribution of Social Media Posts') plt.xlabel('Sentiment') plt.ylabel('Frequency') plt.show()
Another powerful tool for visualization is Plotly, which allows for the creation of interactive plots. This is particularly useful when presenting data insights in a web-based format, where users can hover, zoom, and explore different data aspects dynamically. Here’s an example of how to create an interactive scatter plot with Plotly:
import plotly.express as px # Sample data for an interactive plot df = pd.DataFrame({ 'Engagement': [100, 150, 200, 250, 300], 'Month': ['Jan', 'Feb', 'Mar', 'Apr', 'May'] }) # Create an interactive scatter plot fig = px.scatter(df, x='Month', y='Engagement', size='Engagement', title='Social Media Engagement Over Time', labels={'Engagement': 'Engagement Count', 'Month': 'Month'}) fig.show()
Using these visualization libraries enables analysts to uncover patterns and trends in social media data easily. Whether you are exploring engagement metrics, sentiment distributions, or user interactions, visualizations serve as a powerful means to communicate insights effectively. By integrating these visual tools into your analysis workflow, you can enhance your storytelling, making your data not just informative but also engaging for your audience.