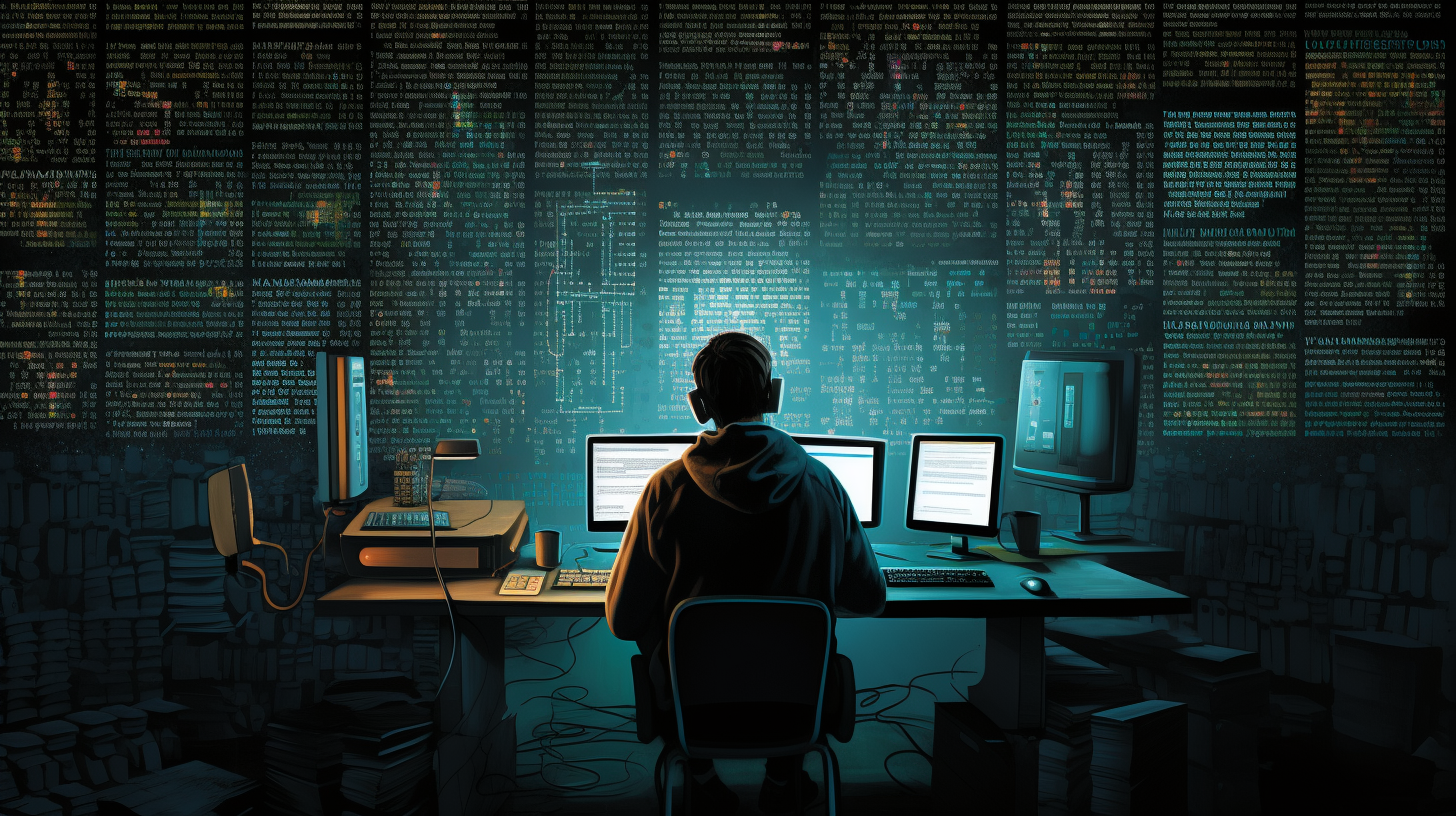
Python for Urban Planning: Data Analysis and Modeling
Python has emerged as a pivotal tool in the sphere of urban planning, offering a rich ecosystem of libraries and frameworks that facilitate various analytical and modeling tasks. Its versatility allows planners to handle large datasets, perform complex analyses, and visualize results effectively. Here, we explore several applications of Python that are reshaping urban planning.
One of the primary applications of Python in urban planning is in the area of data analysis. With libraries like pandas and NumPy, planners can manipulate and analyze vast amounts of data efficiently. For example, urban planners can use Python to assess demographic trends by analyzing census data:
import pandas as pd # Load census data data = pd.read_csv('census_data.csv') # Calculate population density data['population_density'] = data['population'] / data['land_area'] # Display the top 5 areas by population density print(data[['area_name', 'population_density']].sort_values(by='population_density', ascending=False).head())
Beyond analysis, Python also excels in geographic data processing. The GeoPandas library extends the capabilities of pandas to enable spatial operations. Urban planners can leverage GeoPandas to visualize spatial data, such as zoning maps or transportation networks:
import geopandas as gpd import matplotlib.pyplot as plt # Load the zoning map zoning_map = gpd.read_file('zoning_map.geojson') # Plot the zoning map zoning_map.plot(column='zone_type', legend=True) plt.title('Zoning Map') plt.show()
Another significant application is in simulation and modeling. Urban planners use Python libraries like SimPy for simulating various urban processes, such as traffic flow or public transportation systems. This allows planners to test scenarios and optimize systems before implementation:
import simpy def traffic_flow(env): while True: print(f'Time {env.now}: Car enters the intersection') yield env.timeout(2) # Time taken to pass the intersection env = simpy.Environment() env.process(traffic_flow(env)) env.run(until=10)
Machine learning is another area where Python shines in urban planning. With frameworks like scikit-learn, planners can build predictive models to forecast land use, property values, or traffic patterns. For instance, a simple linear regression model can predict housing prices based on various features:
from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression # Sample housing data housing_data = pd.read_csv('housing_data.csv') X = housing_data[['square_feet', 'num_bedrooms', 'num_bathrooms']] y = housing_data['price'] # Split the data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Train the model model = LinearRegression() model.fit(X_train, y_train) # Predict prices predictions = model.predict(X_test) print(predictions)
Lastly, Python’s role in visualizing urban data cannot be overstated. Using libraries such as Matplotlib and Folium, planners can create interactive maps and compelling data visualizations that communicate insights to stakeholders effectively:
import folium # Create a map centered around a specific location urban_map = folium.Map(location=[latitude, longitude], zoom_start=12) # Add a marker folium.Marker([latitude, longitude], tooltip='Important Location').add_to(urban_map) # Save the map urban_map.save('urban_map.html')
In sum, Python’s diverse applications in urban planning enable professionals to engage deeply with data, enhancing decision-making processes and fostering more sustainable urban environments.
Data Collection Techniques for Urban Analysis
Data collection is a critical step in urban analysis, as the quality and specificity of data directly influence the effectiveness of any subsequent analysis or modeling. Python provides a plethora of techniques and libraries that facilitate efficient data collection from various sources, enabling urban planners to gather the necessary information for informed decision-making.
One of the most common methods for data collection is the use of APIs to access real-time data from different platforms. For instance, urban planners can collect transportation data using APIs offered by services like Google Maps or OpenStreetMap. The requests library in Python makes it easy to retrieve this data. Below is an example of how to fetch traffic data using an API:
import requests # Define the API endpoint and parameters url = 'https://api.example.com/traffic' params = { 'location': 'Downtown', 'key': 'YOUR_API_KEY' } # Make the GET request response = requests.get(url, params=params) # Check the status code and process the data if response.status_code == 200: traffic_data = response.json() print(traffic_data) else: print('Failed to retrieve data:', response.status_code)
In addition to API calls, web scraping is a valuable technique for gathering data from websites that do not offer APIs. Python libraries such as BeautifulSoup and Scrapy can be utilized to extract data from HTML pages. This can be particularly useful for collecting data on local businesses, demographics, or historical land use changes. Here’s a brief example using BeautifulSoup to scrape data:
from bs4 import BeautifulSoup import requests # Fetch the webpage url = 'https://www.example.com/data' response = requests.get(url) # Parse the HTML content soup = BeautifulSoup(response.content, 'html.parser') # Extract specific data elements data_items = soup.find_all('div', class_='data-item') for item in data_items: print(item.text)
Another important source of data is open data portals, which provide a wealth of datasets related to urban environments. Many cities publish their data through open data initiatives, offering access to datasets on topics such as public transportation, crime statistics, and property assessments. Python’s pandas library can easily load and filter these datasets for analysis. For example:
import pandas as pd # Load data from a CSV file available on an open data portal data = pd.read_csv('https://www.examplecity.gov/opendata/public_transport.csv') # Filter data for a specific route route_data = data[data['route'] == 'Route 1'] print(route_data.head())
The integration of satellite imagery and remote sensing data is another vital aspect of urban data collection. Python libraries such as Rasterio and Geopandas allow urban planners to process and analyze spatial data derived from satellite imagery. This can aid in land use classification, environmental monitoring, and urban growth analysis. Here is a simple example using Rasterio to read a satellite image:
import rasterio # Open the satellite image with rasterio.open('satellite_image.tif') as src: band1 = src.read(1) # Read the first band print(band1.shape)
Furthermore, surveys and public engagement tools can be utilized to gather qualitative data directly from community members. Python can assist in managing and analyzing survey data, allowing planners to incorporate public opinion into their analyses. Tools like Google Forms or SurveyMonkey can be integrated with Python scripts to automate data retrieval and processing.
The diverse array of data collection techniques available through Python empowers urban planners to source, integrate, and analyze data more effectively. This ability to collect robust datasets is foundational for conducting thorough urban analyses and developing informed strategies for sustainable urban development.
Exploratory Data Analysis: Tools and Techniques
Exploratory Data Analysis (EDA) is an important stage in the urban planning process, allowing planners to understand the patterns, trends, and anomalies within their datasets before diving deeper into modeling and analysis. Python offers a variety of powerful libraries and tools that can significantly enhance the EDA process, making it easier to visualize and interpret data in meaningful ways.
One of the first steps in EDA is to load and inspect the data. The pandas library provides an intuitive interface for data manipulation, enabling planners to easily analyze the structure of their datasets. Below is an example of how to load a dataset and perform some initial checks:
import pandas as pd # Load the dataset data = pd.read_csv('urban_data.csv') # Display the first few rows of the dataset print(data.head()) # Get summary statistics print(data.describe()) # Check for missing values print(data.isnull().sum())
Once the data is loaded, visual exploration becomes essential. Matplotlib and Seaborn are two libraries that simplify the process of creating various types of visualizations. Planners can use these tools to create histograms, scatter plots, and box plots to observe distributions and relationships between variables. For example, to visualize the relationship between population density and housing prices:
import seaborn as sns import matplotlib.pyplot as plt # Scatter plot of population density vs housing prices plt.figure(figsize=(10, 6)) sns.scatterplot(data=data, x='population_density', y='housing_price', alpha=0.6) plt.title('Population Density vs Housing Prices') plt.xlabel('Population Density') plt.ylabel('Housing Price') plt.grid(True) plt.show()
Furthermore, heatmaps can be utilized to display correlations between multiple variables, providing insight into which factors might influence urban dynamics. This visual representation is invaluable for identifying multicollinearity and understanding the relationships in the urban environment:
# Correlation heatmap plt.figure(figsize=(12, 8)) correlation_matrix = data.corr() sns.heatmap(correlation_matrix, annot=True, fmt='.2f', cmap='coolwarm', square=True) plt.title('Correlation Heatmap') plt.show()
Understanding the distribution of individual features is also vital. For this purpose, plotting histograms and box plots can help reveal outliers and the general properties of the data. Here’s how to generate a histogram for housing prices:
# Histogram of housing prices plt.figure(figsize=(10, 6)) sns.histplot(data['housing_price'], bins=30, kde=True) plt.title('Distribution of Housing Prices') plt.xlabel('Housing Price') plt.ylabel('Frequency') plt.grid(True) plt.show()
In addition to visualizations, EDA often involves transforming data to enhance its usability. The pandas library includes various functions to normalize, scale, or log-transform data, which can be beneficial for modeling. For instance, log-transforming housing prices can help address skewness:
# Log transformation of housing prices data['log_housing_price'] = np.log(data['housing_price']) # Verify the transformation plt.figure(figsize=(10, 6)) sns.histplot(data['log_housing_price'], bins=30, kde=True) plt.title('Log-Transformed Housing Prices Distribution') plt.xlabel('Log Housing Price') plt.ylabel('Frequency') plt.grid(True) plt.show()
By employing these tools and techniques, urban planners can effectively conduct exploratory data analysis, uncovering insights that inform their decision-making processes. This preliminary phase is critical for ensuring that subsequent modeling and analyses are grounded in a solid understanding of the data’s underlying structure and characteristics.
Modeling Urban Systems with Python
Modeling urban systems involves creating representations of real-world processes and structures to predict outcomes and assess the impacts of various scenarios. Python’s capabilities in modeling urban systems are vast, drawing on its rich libraries tailored for scientific computation, data manipulation, and visualization. These tools enable urban planners to simulate complex interactions within urban environments, ranging from transportation networks to demographic changes.
One of the most powerful frameworks for modeling in Python is Pyomo, which is used for formulating optimization problems. Urban planners can utilize Pyomo to model transportation systems, land use, or resource allocation problems. Here’s a simple example demonstrating how to set up a linear programming model to optimize the allocation of resources across different urban projects:
import pyomo.environ as pyo # Create a model model = pyo.ConcreteModel() # Define sets for projects model.projects = pyo.Set(initialize=['Park', 'School', 'Hospital']) # Define parameters for costs and resource limits model.costs = pyo.Param(model.projects, initialize={'Park': 5000, 'School': 7000, 'Hospital': 10000}) model.budget = pyo.Param(initialize=15000) # Define decision variables model.x = pyo.Var(model.projects, within=pyo.NonNegativeIntegers) # Define the objective function (maximize number of projects) model.objective = pyo.Objective(expr=sum(model.x[j] for j in model.projects), sense=pyo.maximize) # Define constraints (total cost must not exceed the budget) model.constraints = pyo.Constraint(expr=sum(model.costs[j] * model.x[j] for j in model.projects) <= model.budget) # Solve the model solver = pyo.SolverFactory('glpk') solver.solve(model) # Display results for j in model.projects: print(f'{j}: {model.x[j].value}')
Another essential library for modeling urban systems is SimPy, which allows for discrete-event simulation. This can be used to model systems like public transportation or traffic dynamics. Below is an example of how to simulate a simple queueing system at a bus stop:
import simpy def bus_stop(env): while True: # Bus arrives print(f'Time {env.now}: Bus arrives at the stop.') yield env.timeout(5) # Time the bus stays at the stop print(f'Time {env.now}: Bus departs from the stop.') yield env.timeout(15) # Interval before the next bus arrives # Set up the simulation environment env = simpy.Environment() env.process(bus_stop(env)) # Run the simulation for a certain period env.run(until=60)
When it comes to spatial modeling, GeoPandas is invaluable for manipulating geospatial data. By combining spatial data with attribute data, planners can create rich models that reflect urban realities. The following code snippet shows how to perform a spatial join to enrich property data with zoning information:
import geopandas as gpd # Load property data and zoning data properties = gpd.read_file('properties.geojson') zoning = gpd.read_file('zoning.geojson') # Perform a spatial join properties_zoned = gpd.sjoin(properties, zoning, op='intersects') # Display the enriched dataset print(properties_zoned.head())
Machine learning techniques can also be employed for predictive modeling in urban planning. Using libraries such as scikit-learn, planners can develop models to forecast urban growth or traffic patterns based on historical data. For example, a decision tree model can be created to predict housing demand based on various features:
from sklearn.tree import DecisionTreeRegressor from sklearn.model_selection import train_test_split # Load housing demand data housing_data = pd.read_csv('housing_demand.csv') X = housing_data[['location_score', 'average_income', 'population_growth']] y = housing_data['demand'] # Split the data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create and train the model model = DecisionTreeRegressor() model.fit(X_train, y_train) # Predictions predictions = model.predict(X_test) print(predictions)
By using the diverse modeling capabilities of Python, urban planners can create comprehensive simulations and analyses that support more effective decision-making. The interplay of data-driven models enhances the planning process, allowing for insights that promote sustainable urban development and address the challenges of urbanization.
Case Studies: Successful Urban Planning Projects
<= model.budget)
# Solve the model
solver = pyo.SolverFactory('glpk')
solver.solve(model)
# Display results
for j in model.projects:
print(f'{j}: {model.x[j].value}')
In addition to optimization modeling, agent-based modeling is another powerful approach that Python facilitates through libraries like Mesa. Agent-based models enable planners to simulate the actions and interactions of autonomous agents within the urban environment, providing insights into how individual behaviors can influence overall system dynamics. Here’s a basic example of setting up an agent-based model to simulate pedestrian movement in an urban setting:
import mesa
class Pedestrian(mesa.Agent):
""" An agent representing a pedestrian. """
def __init__(self, unique_id, model):
super().__init__(unique_id, model)
def step(self):
# Define pedestrian actions (e.g., move randomly)
self.move_randomly()
class UrbanModel(mesa.Model):
""" A model with some number of agents. """
def __init__(self, N):
self.num_agents = N
self.schedule = mesa.time.RandomActivation(self)
for i in range(self.num_agents):
a = Pedestrian(i, self)
self.schedule.add(a)
def step(self):
self.schedule.step()
# Create and run the model
model = UrbanModel(100)
for i in range(10):
model.step()
Furthermore, Python supports network modeling through libraries like NetworkX, which is ideal for analyzing urban networks such as transportation and utility lines. This capability allows planners to evaluate the connectivity and resilience of urban systems. Below is an example of how to create a simple transportation network and analyze its properties:
import networkx as nx
# Create a graph
G = nx.Graph()
# Add nodes (representing intersections)
G.add_nodes_from(['A', 'B', 'C', 'D'])
# Add edges (representing roads with weights as distances)
G.add_weighted_edges_from([('A', 'B', 5), ('A', 'C', 10), ('B', 'D', 2), ('C', 'D', 1)])
# Calculate shortest paths
shortest_path = nx.shortest_path(G, source='A', target='D', weight='weight')
print(f'Shortest path from A to D: {shortest_path}')
These modeling techniques enable urban planners to explore complex scenarios, evaluate potential impacts of policies, and create data-driven strategies for urban development. The flexibility of Python ensures that planners can adapt their models to specific urban challenges, fostering smarter, more sustainable cities through informed decision-making. Future Trends in Urban Planning and Data Science
“`
Future Trends in Urban Planning and Data Science
<= model.budget)
# Solve the optimization problem
solver = pyo.SolverFactory('glpk')
results = solver.solve(model)
# Display results
print('Optimal allocation of projects:')
for project in model.projects:
print(f'{project}: {model.x[project].value}')
With the ability to simulate and optimize, another robust approach is agent-based modeling (ABM). The Mesa library offers a framework where urban planners can simulate the interactions of autonomous agents within an environment. This can be particularly useful for modeling pedestrian behavior, traffic dynamics, or the spread of urban phenomena such as gentrification. Here’s how one might set up a simple agent-based model with Mesa:
import mesa class Person(mesa.Agent): """A person who moves to a new location.""" def __init__(self, unique_id, model): super().__init__(unique_id, model) def step(self): # Logic for moving or interacting new_pos = (self.pos[0] + 1, self.pos[1]) # Example movement self.model.grid.move_agent(self, new_pos) class CityModel(mesa.Model): """A model with some number of agents.""" def __init__(self, N): self.num_agents = N self.grid = mesa.space.MultiGrid(10, 10, True) self.schedule = mesa.time.RandomActivation(self) for i in range(self.num_agents): a = Person(i, self) self.schedule.add(a) def step(self): self.schedule.step() # Create the model and run it model = CityModel(5) for i in range(10): model.step()
The visualization of these models is equally important. While libraries such as Matplotlib can plot the results of simulations, libraries like Folium can help visualize spatial data. This capability allows planners to present their findings in a geographically meaningful way, fostering better understanding and engagement among stakeholders. For instance, think a scenario where you want to visualize the results of a traffic simulation:
import folium # Create a base map traffic_map = folium.Map(location=[latitude, longitude], zoom_start=13) # Add traffic data points for point in traffic_data: folium.CircleMarker(location=[point['lat'], point['lon']], radius=5, color='blue').add_to(traffic_map) # Save the traffic map traffic_map.save('traffic_simulation_map.html')
As urban environments continue to evolve and face new challenges, the integration of predictive modeling and simulation becomes indispensable. Python’s diverse capabilities in processing and visualizing urban data empower planners to make informed decisions, ultimately leading to more resilient urban systems. The future of urban planning will increasingly rely on these sophisticated modeling techniques, underscoring the need for professionals to harness the power of Python and its extensive libraries for effective urban analysis and design.