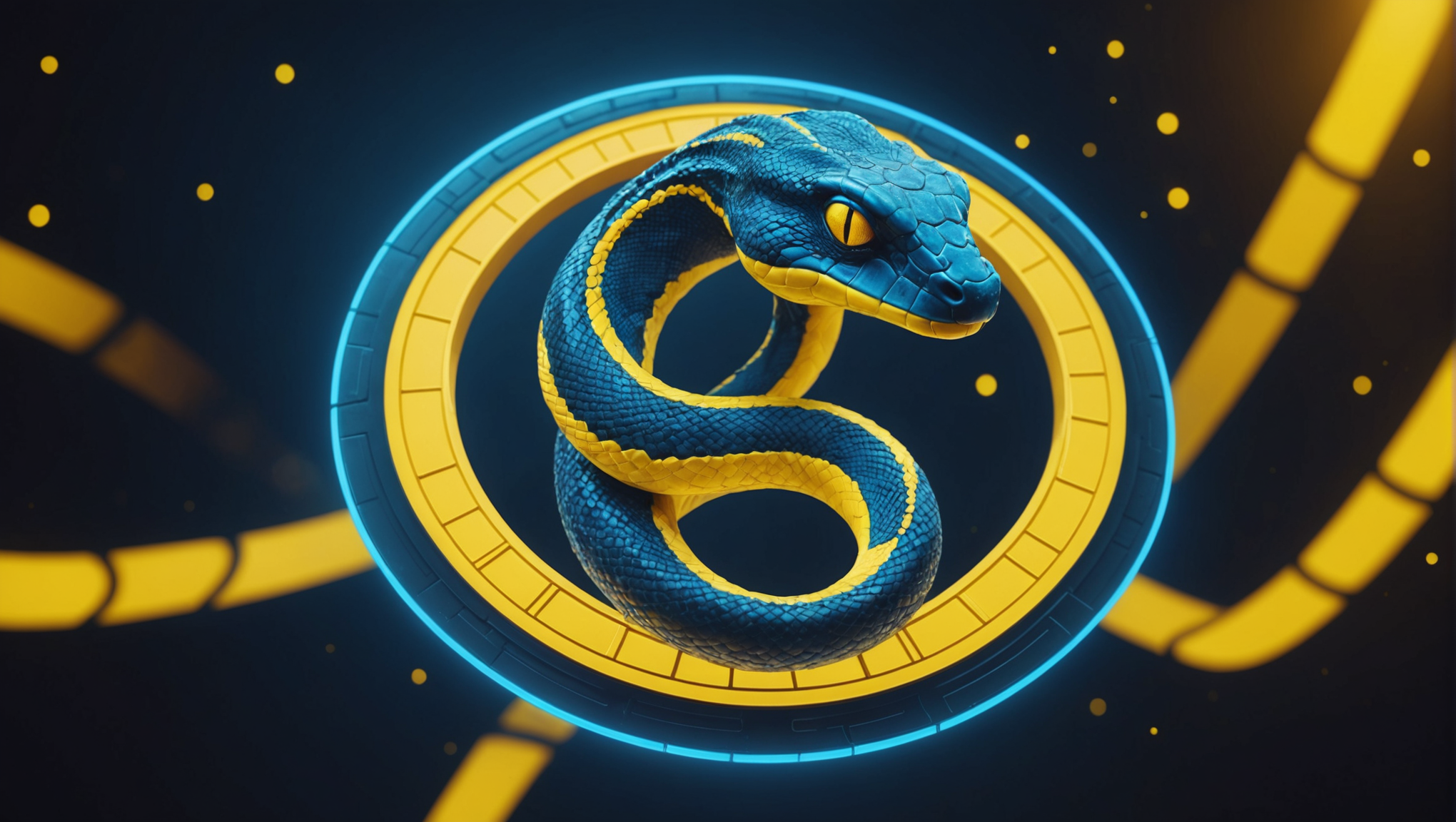
Python Functions: Definition and Usage
In Python, functions serve as fundamental building blocks that enable modular programming. They encapsulate reusable code, enhancing both readability and maintainability. A function can be thought of as a discrete unit of work that performs a specific task. This encapsulation is not just a matter of convenience; it also promotes code organization, allowing developers to break down complex problems into smaller, more manageable pieces.
At the core of a function’s utility is its ability to take inputs, process them, and produce outputs. This input-output mechanism can be understood in terms of parameters and return values. When you define a function, you specify the parameters that will accept the input values. Once the function executes its task, it can return a value back to the caller, completing the cycle of interaction.
The beauty of Python functions lies in their flexibility. Not only can they accept different types of arguments, but they can also be nested within other functions, creating a powerful structure for abstraction and code reuse. This allows programmers to build libraries of functions that can be shared and utilized across various projects.
Additionally, functions can help in reducing redundancy. Instead of rewriting the same block of code multiple times, you can define a function once and call it whenever needed. This not only saves time but also reduces the risk of errors, as changes only need to be made in one place.
def greet(name): return f"Hello, {name}!" print(greet("Alice")) # Outputs: Hello, Alice!
In the example above, the function greet
takes a single parameter, name
, and returns a greeting string. This demonstrates the simplicity and power of functions in executing tasks and managing complexity.
Defining a Function in Python
Defining a function in Python is simpler and intuitive, reflecting the language’s philosophy of simplicity and readability. To create a function, you begin with the def
keyword, followed by the function’s name and a set of parentheses that may include parameters. The definition ends with a colon, indicating the start of the function’s body, which is indented to indicate the scope of the function.
A basic function definition can look like this:
def function_name(parameters): # function body statement(s)
For instance, consider a function that adds two numbers:
def add_numbers(a, b): return a + b
In this example, add_numbers
is the name of the function, and it takes two parameters, a
and b
. When you call this function and provide it with two numeric values, it will return their sum:
result = add_numbers(5, 3) print(result) # Outputs: 8
This simplicity allows you to focus on what the function does without getting bogged down in the details of how it works. You can define functions that perform computations, manipulate data, or even control program flow.
Function names in Python should be descriptive and ideally use lowercase letters, with words separated by underscores. This makes the code self-documenting, revealing the purpose of the function at a glance. In addition, Python follows the convention of using a single leading underscore for functions intended for internal use within a module, while double leading underscores can be used to invoke name mangling.
When defining a function, you may also choose to specify default values for parameters. This allows the function to be called with fewer arguments than the total number of parameters defined. For example:
def greet(name="World"): return f"Hello, {name}!"
In this case, if you call greet()
without any arguments, it defaults to greeting “World”:
print(greet()) # Outputs: Hello, World! print(greet("Alice")) # Outputs: Hello, Alice!
Function Arguments and Parameters
When discussing function arguments and parameters, it’s essential to recognize their role in the interaction between the function and the external environment. Parameters act as placeholders within the function definition, while arguments are the actual values supplied to these parameters during a function call. This distinction is important for understanding how data is passed and manipulated within functions.
Python provides several ways to define and handle function arguments, offering a high degree of flexibility. The most common are positional arguments, keyword arguments, default arguments, and variable-length arguments.
Positional arguments are the simplest form. When a function is called, the arguments are assigned to the parameters in the order they are defined. For example:
def multiply(x, y): return x * y result = multiply(4, 5) print(result) # Outputs: 20
Here, the values 4 and 5 are passed to the parameters x and y, respectively, demonstrating how positional arguments work.
Keyword arguments allow you to specify the arguments by their parameter names, which can make function calls more understandable. Consider the following example:
def power(base, exponent): return base ** exponent result = power(exponent=3, base=2) print(result) # Outputs: 8
In this case, the order of the arguments does not matter, as they are matched with the parameters based on the provided names.
Default arguments enhance the flexibility of functions by allowing certain parameters to have pre-defined values. This means that if an argument is not provided during the function call, the default value is used instead:
def describe_pet(pet_name, pet_type='dog'): return f"I have a {pet_type} named {pet_name}." print(describe_pet('Buddy')) # Outputs: I have a dog named Buddy. print(describe_pet('Whiskers', 'cat')) # Outputs: I have a cat named Whiskers.
In the example above, the pet_type parameter defaults to ‘dog’ if no other value is provided.
For functions that require a variable number of arguments, Python offers *args and **kwargs. The *args syntax allows you to pass a variable number of non-keyword arguments to a function, while **kwargs allows for passing keyword arguments. That’s particularly useful in cases where the number of inputs may vary:
def collect_items(*args): return f"Collected items: {', '.join(args)}" print(collect_items('apple', 'banana', 'cherry')) # Outputs: Collected items: apple, banana, cherry def print_scores(**kwargs): for student, score in kwargs.items(): print(f"{student}: {score}") print_scores(Alice=90, Bob=85, Charlie=92) # Outputs: # Alice: 90 # Bob: 85 # Charlie: 92
Return Statement in Functions
In Python, the return statement is a pivotal element within a function that determines what value is sent back to the caller once the function has completed its execution. This mechanism very important for producing output from a function, allowing it to pass data on to the next stage in the program’s workflow. The absence of a return statement results in a function that returns None by default, which is a key concept to grasp when working with Python functions.
To illustrate the use of the return statement, think a simple function that computes the square of a number:
def square(x): return x * x
When the square function is called with an argument, it computes the square of that number and returns it:
result = square(4) print(result) # Outputs: 16
Here, the return statement allows the function to send the computed value back to the caller, which can then utilize it as needed. This showcases how a return statement effectively conveys the result of a function’s operation.
Functions can also return multiple values, which Python conveniently packs into a tuple. This is particularly useful when a function needs to produce more than one output. For example:
def arithmetic_operations(a, b): return a + b, a - b, a * b, a / b
In this case, the arithmetic_operations function calculates the sum, difference, product, and quotient of two numbers and returns them as a tuple. When calling this function, you can unpack the returned values as follows:
sum_result, diff_result, product_result, quotient_result = arithmetic_operations(10, 2) print(sum_result) # Outputs: 12 print(diff_result) # Outputs: 8 print(product_result) # Outputs: 20 print(quotient_result) # Outputs: 5.0
This example highlights the versatility of the return statement in Python, allowing functions to convey complex results in a clear and organized manner.
Another important aspect of the return statement is that it concludes the execution of the function. Once a return statement is executed, no subsequent code within the function will run. For instance:
def test_return(): return "This is before the return" return "This will never be executed"
In the test_return function, the second return statement is unreachable and will never execute, demonstrating that the function returns control to the caller immediately after the first return statement.
Additionally, it is worth mentioning that functions can return no value explicitly using the return statement without any arguments. This effectively returns None:
def do_nothing(): return
Calling do_nothing will result in None being returned:
result = do_nothing() print(result) # Outputs: None
Scope and Lifetime of Variables
The scope and lifetime of variables in Python functions are fundamental concepts that determine how variables behave within the context of function execution. Scope refers to the region of a program where a variable is defined and accessible, while lifetime pertains to the duration for which a variable exists in memory during program execution. Understanding these concepts very important for writing clean and efficient code.
In Python, variables defined within a function have a local scope. This means they can only be accessed and modified within that function. Once the function execution is complete, any local variables are destroyed, and their memory is released. For instance, think the following example:
def local_scope_example(): x = 10 # x is a local variable print(f"Inside the function, x: {x}") local_scope_example() # Outputs: Inside the function, x: 10 print(x) # Raises NameError: name 'x' is not defined
Here, the variable x
is defined within the local_scope_example
function. It is accessible as long as the function is running, but attempting to access x
outside the function results in a NameError
because it isn’t defined in the global scope.
Conversely, variables defined outside of any function are considered global variables. They can be accessed from within functions, provided that they’re not overshadowed by local variables with the same name. Here’s an example to illustrate this:
y = 5 # Global variable def global_scope_example(): print(f"Inside the function, y: {y}") # Accessing global variable global_scope_example() # Outputs: Inside the function, y: 5
In this case, the global variable y
is accessible within the global_scope_example
function, demonstrating the interaction between local and global scopes. However, if you try to assign a value to a global variable inside a function without declaring it as global, Python treats it as a new local variable:
z = 20 # Global variable def modify_global(): z = 30 # Local variable, shadows the global variable print(f"Inside the function, z: {z}") modify_global() # Outputs: Inside the function, z: 30 print(z) # Outputs: 20
To modify a global variable within a function, you need to declare it using the global
keyword:
a = 50 # Global variable def modify_global_correctly(): global a # Declare a as global a = 70 # Modify the global variable print(f"Inside the function, a: {a}") modify_global_correctly() # Outputs: Inside the function, a: 70 print(a) # Outputs: 70
In this example, the global
keyword allows the function modify_global_correctly
to change the value of the global variable a
.
Best Practices for Writing Functions
When it comes to writing functions in Python, adhering to best practices can significantly enhance the clarity, maintainability, and efficiency of your code. These practices are not merely suggestions; they are essential habits that can help you avoid common pitfalls and make your code easier to understand for yourself and others. Here are some key best practices to think when defining and using functions in Python.
1. Keep Functions Small and Focused
A well-structured function should perform a single task or a closely related set of tasks. This approach not only makes the function easier to understand but also facilitates testing and reuse. If a function tries to accomplish too much, it becomes difficult to follow, debug, and maintain. For example:
def process_data(data): cleaned_data = clean_data(data) analyzed_data = analyze_data(cleaned_data) return analyzed_data
In this example, process_data is focused on a specific workflow: cleaning and analyzing data. Each of its components (clean_data and analyze_data) can be tested independently, making the codebase more robust.
2. Use Descriptive Naming Conventions
Function names should clearly convey their purpose. This not only aids in self-documentation but also helps other developers (or your future self) understand the intent behind the function. Use verbs in your function names to indicate actions, and avoid vague names. For instance:
def calculate_area(radius): return 3.14 * radius * radius
This name is descriptive and indicates that the function calculates the area of a circle, based on the provided radius.
3. Limit Side Effects
Functions should ideally not have side effects, such as modifying global variables or altering the state of arguments that are mutable. Side effects can lead to unpredictable behavior, especially in larger codebases. Instead, return new values or data structures, allowing the caller to decide how to use them. For example:
def append_to_list(item, lst): new_list = lst.copy() # Create a copy new_list.append(item) # Append item to the copy return new_list
In this case, the original list remains unchanged, and the function returns a new list with the appended item.
4. Document Your Functions
Writing docstrings for your functions is an excellent practice. A docstring provides a convenient way of associating documentation with functions. It describes what the function does, its parameters, and its return value. Ponder the following example:
def factorial(n):
"""Calculate the factorial of a number.Args:
n (int): A non-negative integer.