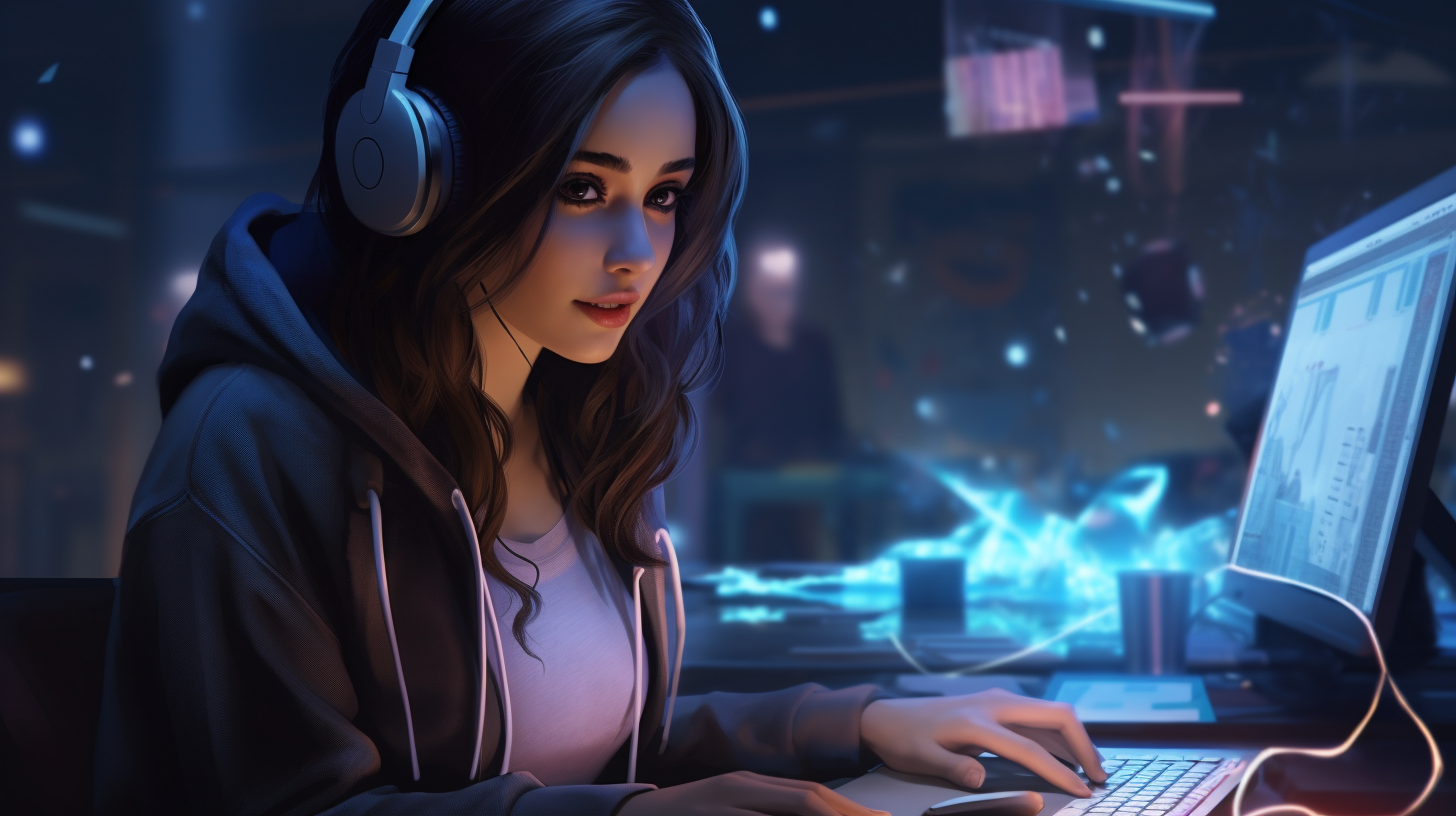
Python in Astronomy: Data Analysis and Simulation
The landscape of astronomy has been irrevocably transformed with the advent of Python, a language that has become a cornerstone for researchers and astronomers alike. Its usability, coupled with a rich ecosystem of libraries and frameworks tailored for scientific computing, has positioned Python as an essential tool in the astronomical toolkit.
Python’s interpretative nature and dynamic typing facilitate rapid prototyping and experimentation. This flexibility allows astronomers to quickly test hypotheses and develop complex models without the overhead of more rigid programming languages. As a result, Python accelerates the pace of discovery in a field that relies heavily on data analysis and simulation.
Furthermore, the integration of Python with powerful libraries, such as NumPy and SciPy, provides the numerical capabilities required for handling large datasets. These libraries enable efficient manipulation of arrays, mathematical modeling, and numerical simulations, which are pivotal for analyzing astronomical data.
In addition to numerical analysis, Python’s robust data visualization capabilities are crucial in astronomy. Libraries like Matplotlib and Seaborn allow researchers to create compelling visualizations that can reveal patterns and insights hidden within vast amounts of data. For instance, a simple scatter plot of star magnitudes can be generated as follows:
import matplotlib.pyplot as plt # Sample data: star magnitudes and distances magnitudes = [5.0, 4.5, 6.2, 3.8, 5.5] distances = [10, 20, 15, 25, 30] plt.scatter(distances, magnitudes) plt.xlabel('Distance (light years)') plt.ylabel('Magnitude') plt.title('Star Magnitudes vs. Distance') plt.gca().invert_yaxis() # Invert y-axis for magnitude plt.show()
Python’s role in astronomy is not limited to data analysis; it extends to simulation as well. Libraries such as AstroPy and Astropy’s affiliated packages enable astronomers to model celestial phenomena with accuracy. These tools make it possible to simulate the behavior of stars, galaxies, and other celestial objects under various physical conditions.
As we delve deeper into the specifics of Python’s applications in astronomy, we uncover not just its utility, but also the ongoing collaborative efforts within the community. Open-source contributions have led to a continually evolving suite of libraries and tools that enhance Python’s capabilities and accessibility for astronomers worldwide.
Key Libraries for Astronomical Data Analysis
When it comes to astronomical data analysis, Python’s rich collection of libraries stands out as a fundamental asset for researchers. Each library serves a specific purpose, and together they form a powerful toolkit that enables astronomers to explore, analyze, and interpret vast amounts of data with unprecedented ease.
NumPy is often the starting point for any scientific computing task. It provides support for large, multi-dimensional arrays and matrices, along with a collection of high-level mathematical functions to operate on these arrays. This library is essential for numerical computations that require high performance, such as data transformations and statistical analyses. Consider the following example, which demonstrates how to compute the mean and standard deviation of a dataset containing measurements of celestial bodies:
import numpy as np # Sample data: brightness measurements of celestial objects data = np.array([10.5, 11.0, 9.8, 12.2, 11.5]) mean_brightness = np.mean(data) std_dev_brightness = np.std(data) print(f'Mean Brightness: {mean_brightness:.2f}') print(f'Standard Deviation of Brightness: {std_dev_brightness:.2f}')
Next in line is Pandas, which excels in data manipulation and analysis. It introduces data structures like Series and DataFrames that allow for robust data handling—ideal for managing tabular data often encountered in astronomical datasets. The following example illustrates how one can read a CSV file containing star data, filter it based on a specific criterion, and compute the average magnitude:
import pandas as pd # Load star data from a CSV file df = pd.read_csv('stars.csv') # Filter stars with magnitude less than 5.0 bright_stars = df[df['magnitude'] < 5.0] # Calculate the average magnitude of bright stars average_magnitude = bright_stars['magnitude'].mean() print(f'Average Magnitude of Bright Stars: {average_magnitude:.2f}')
Another indispensable library is AstroPy, specifically designed for astronomy-related tasks. It provides functionalities for reading and writing astronomical data formats, unit conversions, and access to various astronomical databases. The simplicity with which AstroPy allows users to work with celestial coordinates makes it a favorite among astronomers. Below is a short example showing how to convert celestial coordinates from equatorial to galactic:
from astropy.coordinates import SkyCoord import astropy.units as u # Define celestial coordinates in equatorial system ra = 10.684*u.deg # Right Ascension dec = 41.269*u.deg # Declination # Create SkyCoord object coord = SkyCoord(ra, dec, frame='icrs') # Convert to galactic coordinates galactic_coord = coord.galactic print(f'Galactic Coordinates: l={galactic_coord.l:.2f}, b={galactic_coord.b:.2f}')
For visualizing data, Matplotlib is the go-to library. It enables the creation of static, interactive, and animated visualizations in Python. Coupled with Seaborn, which builds on Matplotlib’s capabilities, researchers can create beautiful and informative graphics with minimal code. Here’s how one might visualize the distribution of star magnitudes using a histogram:
import matplotlib.pyplot as plt import seaborn as sns # Sample magnitude data magnitudes = [5.0, 4.5, 6.2, 3.8, 5.5, 4.0, 6.0, 5.9] # Create a histogram of magnitudes sns.histplot(magnitudes, bins=5, kde=True) plt.xlabel('Magnitude') plt.title('Distribution of Star Magnitudes') plt.show()
Each of these libraries contributes uniquely to the astronomer’s toolkit, making Python a versatile and powerful language for astronomical data analysis. By using these libraries, researchers can efficiently process and analyze data, leading to more insightful discoveries in the ever-expanding universe.
Techniques for Data Visualization in Astronomy
Data visualization is a fundamental aspect of astronomical research, as it allows scientists to interpret complex datasets in a more intuitive manner. In astronomy, visualizations can reveal patterns, trends, and anomalies that might otherwise go unnoticed in raw numerical data. Using Python, astronomers can harness various libraries to create a wide range of visualizations, adapting them to their specific needs and the characteristics of the data at hand.
One of the most powerful features of Python’s visualization libraries is their flexibility. For instance, Matplotlib, a widely used plotting library, provides extensive customization options, enabling researchers to tailor their plots to convey information effectively. Matplotlib can generate a variety of plot types, including line plots, scatter plots, bar charts, and more. Take, for example, the following code snippet, which demonstrates how to create a simple line plot to represent the light curve of a variable star:
import matplotlib.pyplot as plt import numpy as np # Sample time and brightness data for a variable star time = np.array([0, 1, 2, 3, 4, 5, 6]) brightness = np.array([10.5, 10.2, 10.8, 10.1, 10.3, 10.0, 10.4]) # Create a line plot plt.plot(time, brightness, marker='o') plt.xlabel('Time (days)') plt.ylabel('Brightness') plt.title('Light Curve of a Variable Star') plt.grid(True) plt.show()
This code snippet illustrates how to depict the variation in brightness over time, which very important for understanding the behavior of variable stars. The use of grid lines enhances readability, allowing the viewer to discern trends more easily.
Beyond Matplotlib, Seaborn elevates statistical data visualization, making it easier to create attractive and informative graphics. By simplifying the syntax and enhancing the appearance of the plots, Seaborn allows astronomers to create visualizations that are not only functional but also publication-ready. For instance, a heatmap can be useful for visualizing correlations between different astronomical measurements. The following example demonstrates how to create a heatmap for a mock dataset:
import seaborn as sns import pandas as pd # Sample data: correlation matrix of different measurements data = pd.DataFrame({ 'Magnitude': [5.0, 4.5, 6.2, 3.8, 5.5], 'Temperature': [5800, 5900, 5500, 6000, 5800], 'Luminosity': [1.0, 1.2, 0.8, 1.5, 1.1] }) # Calculate the correlation matrix correlation = data.corr() # Create a heatmap sns.heatmap(correlation, annot=True, cmap='coolwarm') plt.title('Correlation Matrix of Astronomical Measurements') plt.show()
In this example, the correlation matrix highlights relationships between different attributes of celestial objects, such as magnitude, temperature, and luminosity, providing insights into their interdependencies.
The combination of Matplotlib and Seaborn, along with other libraries like Plotly for interactive visualizations, equips astronomers with powerful tools to visualize their findings. The ability to create dynamic plots that respond to user input can be particularly useful when exploring large datasets, enabling real-time data exploration and analysis.
Moreover, the integration of visualizations in Jupyter Notebooks enhances the exploratory data analysis process, allowing researchers to document their findings alongside code and visual outputs. This interactive environment fosters a more robust understanding of the data, facilitating collaboration and communication of results within the scientific community.
The techniques for data visualization in astronomy provided by Python libraries empower researchers to effectively analyze and present their findings, facilitating the discovery of new insights about the cosmos. By using these tools, astronomers can continue to push the boundaries of our understanding of the universe, one visualization at a time.
Simulating Astronomical Phenomena with Python
Simulating astronomical phenomena is a sophisticated endeavor that requires not only mathematical rigor but also the ability to translate complex theoretical models into computational frameworks. Python’s versatility and the strength of its libraries empower astronomers to conduct simulations of celestial events ranging from planetary movements to the dynamics of galaxies. With the right tools, researchers can create models that replicate the conditions of the universe and predict the behavior of astronomical objects under various scenarios.
One of the foundational libraries for such simulations is NumPy, which provides the necessary numerical capabilities to perform complex calculations efficiently. By using NumPy’s array functionalities, scientists can handle large datasets and perform mathematical operations swiftly. The following example demonstrates how to simulate the orbits of two bodies under the influence of gravity, using Newton’s law of universal gravitation:
import numpy as np import matplotlib.pyplot as plt # Define constants G = 6.67430e-11 # gravitational constant m1 = 5.972e24 # mass of the first body (Earth) m2 = 7.348e22 # mass of the second body (Moon) r = 3.844e8 # distance between the two bodies # Initialize positions and velocities pos1 = np.array([0, 0]) # position of Earth pos2 = np.array([r, 0]) # position of Moon vel1 = np.array([0, 0]) # initial velocity of Earth vel2 = np.array([0, 1022]) # initial velocity of Moon # Time parameters dt = 60 * 60 # time step (1 hour) num_steps = 24 # simulate for 24 hours # Record positions for visualization positions_earth = [] positions_moon = [] for _ in range(num_steps): # Calculate gravitational force r_vec = pos2 - pos1 distance = np.linalg.norm(r_vec) force = G * m1 * m2 / distance**2 force_vec = force * (r_vec / distance) # unit vector # Update velocities vel1 += force_vec / m1 * dt vel2 -= force_vec / m2 * dt # Update positions pos1 += vel1 * dt pos2 += vel2 * dt # Store positions positions_earth.append(pos1.copy()) positions_moon.append(pos2.copy()) # Plot the results positions_earth = np.array(positions_earth) positions_moon = np.array(positions_moon) plt.plot(positions_earth[:, 0], positions_earth[:, 1], label='Earth', color='blue') plt.plot(positions_moon[:, 0], positions_moon[:, 1], label='Moon', color='gray') plt.xlabel('X Position (m)') plt.ylabel('Y Position (m)') plt.title('Orbital Simulation of Earth and Moon') plt.legend() plt.axis('equal') plt.show()
This simulation illustrates how the Earth and Moon interact under gravitational forces, showcasing their respective orbits over a 24-hour period. Such modeling not only enhances our understanding of celestial mechanics but also serves as a foundational tool for more complex simulations involving multiple bodies, such as star clusters or galaxy formations.
For more advanced simulations, particularly those involving hydrodynamics or magnetohydrodynamics, libraries such as SciPy and specialized packages like PySPH come into play. These libraries are designed to handle the intricacies of fluid dynamics, making them suitable for simulating phenomena like star formation in molecular clouds or the behavior of accretion disks around black holes. Here’s a brief example of how one might set up a basic simulation using a particle-based approach:
from pysph.base.utils import get_particle_array from pysph.solver.application import Application class StarFormationSimulation(Application): def create_particles(self): # Create an array of particles representing gas in a molecular cloud particles = get_particle_array(name='gas', x=np.random.uniform(0, 1, 100), y=np.random.uniform(0, 1, 100), h=0.1) return [particles] def configure_solver(self): self.solver = Solver(kernel='gauss', dim=2, integrator='symplectic') # Run the simulation sim = StarFormationSimulation() sim.run()
In this code snippet, we define a simple particle simulation for gas in a molecular cloud, which could eventually lead to star formation. The PySPH library allows for the easy manipulation of particles, which very important for capturing the dynamics of gaseous systems.
Moreover, Python’s ecosystem also includes tools for visualizing simulation results. Libraries such as Matplotlib and Mayavi can be utilized to create insightful visualizations of the phenomena being simulated, providing a clearer picture of the underlying physics at play. The ability to visualize simulation data effectively enhances both analysis and presentation, making findings more accessible to the scientific community.
Ultimately, Python’s capabilities for simulating astronomical phenomena not only bolster our understanding of the universe but also foster collaboration among researchers. By sharing code and methodologies, astronomers can reproduce and build upon each other’s work, amplifying the collective knowledge in the field. As computational methods continue to advance, Python is poised to remain at the forefront of astronomical research, enabling even more complex simulations that delve deeper into the mysteries of the cosmos.
Case Studies: Python Applications in Recent Astronomical Research
import numpy as np import matplotlib.pyplot as plt # Example data: light curves of variable stars time = np.linspace(0, 10, 100) # Time from 0 to 10 days light_curves = [ 10 + 0.5 * np.sin(2 * np.pi * time / 2), # Star A 11 + 0.3 * np.sin(2 * np.pi * time / 3), # Star B 9 + 0.4 * np.sin(2 * np.pi * time / 1.5) # Star C ] # Plotting the light curves for light_curve in light_curves: plt.plot(time, light_curve) plt.xlabel('Time (days)') plt.ylabel('Brightness (arbitrary units)') plt.title('Light Curves of Variable Stars') plt.legend(['Star A', 'Star B', 'Star C']) plt.grid(True) plt.show()
The application of Python in astronomical research is vividly illustrated through various case studies that showcase its power and flexibility. For instance, ponder the analysis of light curves from variable stars, which are essential for understanding their behavior and classification. By employing Python, astronomers can easily manipulate and visualize time-series data, revealing periodicities and anomalies that may indicate significant astrophysical phenomena.
In one compelling case, researchers utilized Python to analyze light curves from a sample of variable stars observed by space telescopes. The data comprised sequences of brightness measurements taken over time, and the scientists needed to discern the underlying patterns. By using libraries like NumPy and Matplotlib, they could efficiently process the data and produce informative visualizations. The above code snippet exemplifies how light curves can be generated and plotted, allowing researchers to investigate the fluctuations in brightness across different stars.
Another noteworthy example comes from the analysis of gravitational wave signals detected by observatories like LIGO. Researchers harnessed Python to process and analyze the data streams, employing the SciPy library to apply Fourier transforms and extract meaningful features from the raw data. This analytical approach enabled them to identify potential astrophysical events, such as the merger of black holes, by comparing the observed signals against theoretical templates.
from scipy.signal import find_peaks # Simulated gravitational wave signal (mock data) np.random.seed(0) time = np.linspace(0, 1, 1000) signal = np.sin(2 * np.pi * 60 * time) + np.random.normal(0, 0.5, signal.shape) peaks, _ = find_peaks(signal, height=0) # Plot the signal and detected peaks plt.plot(time, signal) plt.plot(time[peaks], signal[peaks], "x") plt.title("Simulated Gravitational Wave Signal with Detected Peaks") plt.xlabel("Time (s)") plt.ylabel("Signal Amplitude") plt.grid(True) plt.show()
In this example, the `find_peaks` function from SciPy is utilized to detect peaks in a simulated gravitational wave signal. This method effectively highlights potential events of interest, aiding astronomers in their quest to understand the universe’s most cataclysmic occurrences.
Another case study involves the use of Python to analyze large datasets from sky surveys, such as those conducted by the Sloan Digital Sky Survey (SDSS). By employing Pandas and AstroPy, researchers systematically filtered and categorized millions of celestial objects, investigating their properties and distributions. This analysis could yield insights into the formation and evolution of galaxies, as well as the behavior of dark matter.
import pandas as pd # Load SDSS data # Assuming data.csv contains relevant astronomical data from SDSS df = pd.read_csv('sdss_data.csv') # Filter for galaxies with a specific redshift range filtered_galaxies = df[(df['redshift'] > 0.01) & (df['redshift'] < 0.1)] # Analyze the distribution of galaxy magnitudes magnitude_counts = filtered_galaxies['magnitude'].value_counts() # Plot the distribution of magnitudes magnitude_counts.sort_index().plot(kind='bar') plt.title('Distribution of Galaxy Magnitudes') plt.xlabel('Magnitude') plt.ylabel('Count') plt.show()
In this code snippet, scientists load and filter astronomical data using Pandas, allowing for the investigation of galaxy properties based on redshift, an important aspect for understanding cosmic expansion.
The versatility of Python extends to the realm of cosmological simulations as well. In advanced studies, researchers have utilized Python-based frameworks like GADGET or ENZO to model the formation of large-scale structures in the universe, using vast computational resources to simulate billions of particles. Python’s ability to interface with these high-performance computing tools allows for the analysis and visualization of simulation results, leading to deeper insights into cosmic evolution.
Through these diverse case studies, Python demonstrates its pivotal role in modern astronomical research. By enabling the exploration, analysis, and simulation of astronomical phenomena, it fosters a collaborative and innovative environment that propels the field forward, shedding light on the mysteries of the universe.
Future Trends: Python and the Evolution of Astronomy Data Science
The future of Python’s role in astronomy looks remarkably promising, driven by ongoing advancements in both technology and data science. As the volume of astronomical data continues to surge—thanks in part to new telescopes and space missions—the demand for robust, scalable, and efficient tools for analysis and simulation becomes ever more pressing. Python’s adaptability, combined with the community’s commitment to open-source development, positions it uniquely to meet these challenges head-on.
One significant trend is the increasing adoption of machine learning and artificial intelligence in astronomical research. Python’s rich ecosystem for data science, comprising libraries like TensorFlow, Keras, and Scikit-learn, enables astronomers to apply sophisticated algorithms to tackle complex problems. For example, researchers are now using machine learning techniques to classify celestial objects, detect exoplanets, and even predict the behavior of transient astronomical events. The following snippet illustrates a simple use case of a neural network to classify images of galaxies:
import tensorflow as tf from tensorflow.keras import layers, models # Define a simple convolutional neural network model = models.Sequential([ layers.Conv2D(32, (3, 3), activation='relu', input_shape=(64, 64, 3)), layers.MaxPooling2D((2, 2)), layers.Conv2D(64, (3, 3), activation='relu'), layers.MaxPooling2D((2, 2)), layers.Flatten(), layers.Dense(64, activation='relu'), layers.Dense(10, activation='softmax') # Assuming 10 classes of galaxies ]) model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
In this code, a convolutional neural network (CNN) is defined to classify images of galaxies based on their features. Such advancements not only streamline the classification process but also open up new avenues for understanding the characteristics and evolution of galaxies across the universe.
Another trend is the integration of Python with cloud computing platforms and big data technologies. As astronomical datasets grow larger, the need for scalable storage and processing solutions becomes paramount. Python’s compatibility with platforms like Amazon Web Services (AWS) and Google Cloud allows astronomers to leverage cloud resources for data storage, processing, and analysis. Using libraries such as Dask or PySpark, researchers can perform parallel computing on large datasets, dramatically reducing computation times. The following example demonstrates how to use Dask to handle a large dataset:
import dask.dataframe as dd # Load large astronomical dataset into Dask DataFrame df = dd.read_csv('large_astronomy_data.csv') # Perform computations in parallel mean_value = df['brightness'].mean().compute() print(f'Mean Brightness: {mean_value:.2f}')
Here, Dask allows for the efficient computation of the mean brightness from a potentially massive dataset without the need to load the entire dataset into memory simultaneously. That is particularly beneficial in astronomy, where datasets can be enormous, often containing millions of entries.
Furthermore, the growing interest in citizen science projects is fostering a more inclusive approach to astronomical research. Python plays a vital role in empowering enthusiasts and non-experts to contribute to scientific discovery. Platforms like Galaxy Zoo and other similar initiatives leverage Python for data processing and analysis, allowing volunteers to classify galaxies or search for transient events. The incorporation of easy to use interfaces and educational resources ensures that Python remains accessible to a broader audience.
As the field of astronomy continues to evolve, so too will the tools and methodologies utilized by researchers. The community-driven nature of Python development means that it will likely adapt swiftly to emerging trends, integrating new techniques and technologies as they arise. This ongoing evolution will not only enhance the capabilities of astronomers to analyze and interpret data but also foster collaboration across disciplines, leading to more comprehensive explorations of the universe.
The future of Python in astronomy promises a dynamic landscape filled with innovation and collaboration. With advancements in machine learning, cloud computing, and citizen science, Python is well-positioned to remain a pivotal force in the ongoing quest to unveil the mysteries of the cosmos.