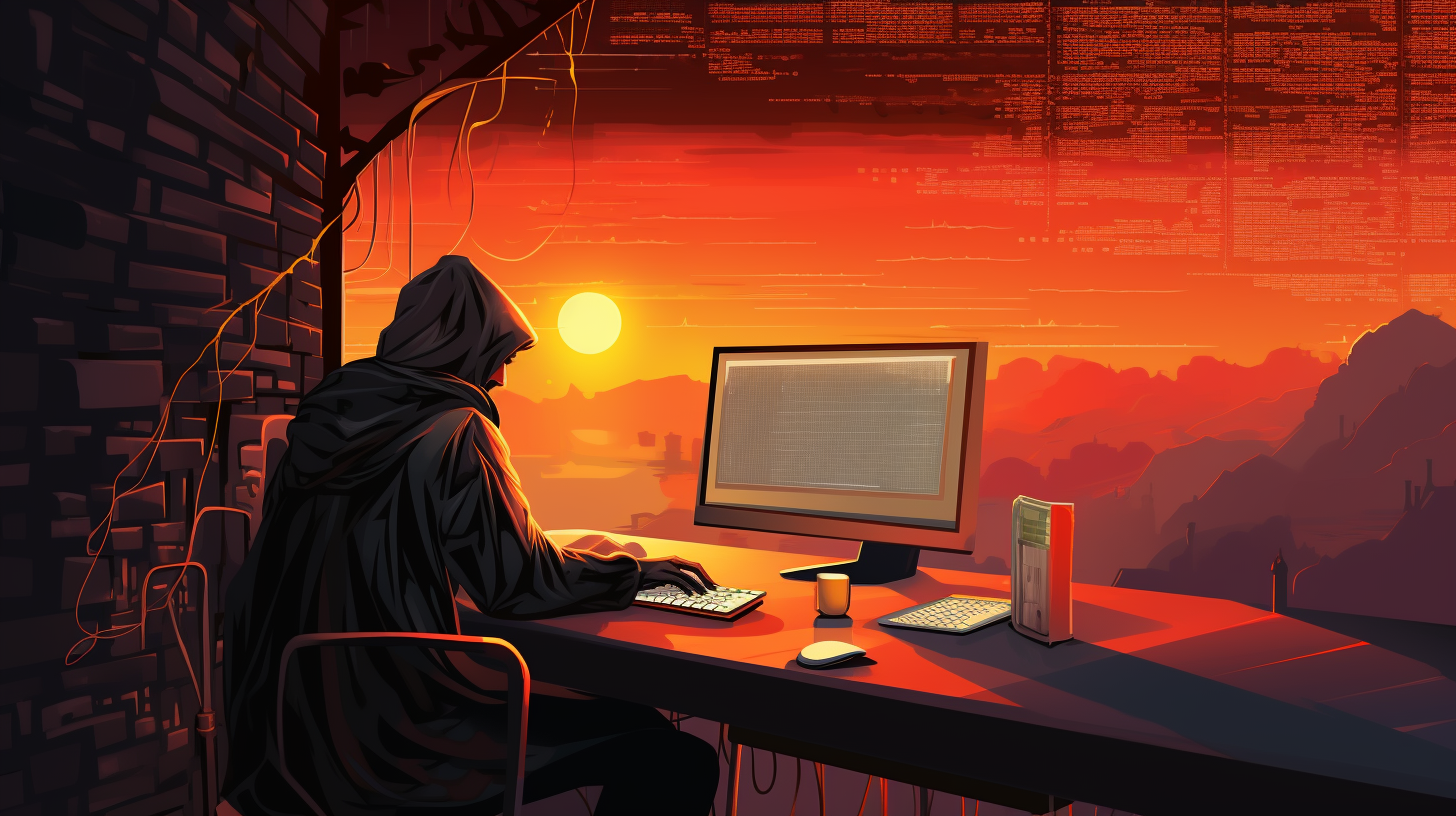
Python in Fashion Technology: Trend Analysis
Within the scope of fashion technology, data analysis plays a pivotal role in shaping design decisions and understanding market dynamics. Python, with its rich ecosystem of libraries and frameworks, serves as a powerful tool for fashion data analysts. It enables the extraction, manipulation, and analysis of vast amounts of data, ranging from consumer preferences to sales trends, providing insights that drive strategic choices.
Data Collection and Preprocessing
Collecting data is the first step in the analysis process. Python offers libraries such as requests
for web scraping and pandas
for data manipulation, allowing fashion analysts to gather data from various sources like e-commerce platforms, social media, and fashion blogs.
import requests import pandas as pd # Example of data collection via web scraping url = 'https://example-fashion-site.com/trends' response = requests.get(url) data = response.json() # Assuming the site returns JSON data # Convert to DataFrame for analysis df = pd.DataFrame(data)
Once the data is collected, preprocessing becomes essential. This involves cleaning the data, handling missing values, and transforming variables to suit analysis requirements. Python’s pandas
library simplifies these tasks significantly.
# Handling missing values and data cleaning df.dropna(inplace=True) # Remove rows with missing values df['price'] = df['price'].str.replace('$', '').astype(float) # Convert price to float
Data Analysis Techniques
With clean data in hand, analysts can employ various statistical techniques and visualizations to uncover trends. The matplotlib
and seaborn
libraries offer rich visualization capabilities, allowing analysts to depict trends and relationships effectively.
import matplotlib.pyplot as plt import seaborn as sns # Visualizing price trends over time sns.lineplot(data=df, x='date', y='price') plt.title('Price Trends Over Time') plt.xlabel('Date') plt.ylabel('Price ($)') plt.show()
Moreover, Python’s statsmodels
library provides functionalities to conduct more complex analyses, such as regression analysis, which can help in understanding the impact of different variables on fashion sales.
import statsmodels.api as sm # Preparing data for regression analysis X = df[['advertising_budget', 'season']] y = df['sales'] X = sm.add_constant(X) # Adding a constant term for the intercept # Fitting the regression model model = sm.OLS(y, X).fit() print(model.summary()) # Displaying the regression results
Ultimately, the integration of Python into fashion data analysis not only enhances the efficiency of data handling but also empowers analysts to derive actionable insights that can significantly influence branding and marketing strategies. This foundation sets the stage for more advanced techniques such as trend forecasting and machine learning applications.
Techniques for Trend Forecasting in Fashion
Trend forecasting in fashion is a dynamic and challenging endeavor, where the intersection of consumer behavior and market signals creates a complex landscape. Using Python to facilitate these forecasts introduces a level of sophistication and efficiency that is essential in a rapidly evolving industry. Various techniques can be employed to anticipate trends by using historical data and sophisticated algorithms.
One primary method for trend forecasting is time series analysis, which allows analysts to identify patterns and trends over time. Python’s statsmodels library is particularly useful for this purpose, offering tools for Seasonal Decomposition of Time Series (STL) and Autoregressive Integrated Moving Average (ARIMA) models. These models help in understanding the underlying patterns in the data, such as seasonality and trends.
import pandas as pd import statsmodels.api as sm # Preparing time series data df['date'] = pd.to_datetime(df['date']) df.set_index('date', inplace=True) # Decomposing the time series decomposition = sm.tsa.seasonal_decompose(df['sales'], model='additive') decomposition.plot() plt.show() # Fitting ARIMA model from statsmodels.tsa.arima.model import ARIMA model = ARIMA(df['sales'], order=(1, 1, 1)) model_fit = model.fit() print(model_fit.summary())
Another technique frequently employed in trend forecasting is the application of machine learning models. Algorithms such as Random Forests and Gradient Boosting Machines are used to predict future trends based on a multitude of features derived from historical data. These models can capture complex interactions between variables that traditional statistical methods might miss.
from sklearn.ensemble import RandomForestRegressor from sklearn.model_selection import train_test_split # Defining features and target variable X = df[['advertising_budget', 'season', 'previous_sales']] y = df['sales'] # Splitting the dataset X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Fitting the Random Forest model model = RandomForestRegressor(n_estimators=100) model.fit(X_train, y_train) # Making predictions predictions = model.predict(X_test) print(predictions)
Additionally, Natural Language Processing (NLP) techniques can be effectively utilized to analyze social media posts, fashion blogs, and customer reviews to gauge public sentiment and emerging trends. Libraries like NLTK and SpaCy can be employed for text processing and sentiment analysis, helping to enrich the dataset with qualitative insights.
import nltk from nltk.sentiment import SentimentIntensityAnalyzer # Sample text data from social media texts = ["I love this new fashion trend!", "This style is so outdated.", "Can't wait to wear this!"] # Sentiment analysis nltk.download('vader_lexicon') sia = SentimentIntensityAnalyzer() sentiments = [sia.polarity_scores(text) for text in texts] # Converting to DataFrame sentiment_df = pd.DataFrame(sentiments) print(sentiment_df)
By synthesizing insights from these various techniques, fashion analysts can develop robust trend forecasts that guide design, marketing, and inventory decisions. The power of Python allows for the integration of diverse data sources and methodologies, thereby enhancing the accuracy and reliability of predictions in the ever-changing fashion landscape.
Machine Learning Applications in Fashion Technology
In the sphere of fashion technology, machine learning has emerged as a transformative force, reshaping how data is analyzed and utilized to drive innovation and efficiency. Python, with its extensive array of libraries dedicated to machine learning, provides a robust platform for fashion technologists to implement predictive models and automate processes. This capability is not merely an enhancement; it is fundamentally changing the landscape of fashion analytics.
One prominent application of machine learning in fashion is in the area of customer segmentation. By using algorithms such as K-Means clustering, analysts can categorize customers based on purchasing behavior, preferences, and demographics. This segmentation enables targeted marketing strategies and personalized shopping experiences.
from sklearn.cluster import KMeans import pandas as pd # Sample customer data data = { 'age': [25, 34, 28, 40, 22, 36, 30], 'annual_spending': [500, 600, 700, 800, 300, 900, 750] } df = pd.DataFrame(data) # Applying K-Means clustering kmeans = KMeans(n_clusters=3) df['cluster'] = kmeans.fit_predict(df[['age', 'annual_spending']]) print(df)
Additionally, recommendation systems powered by collaborative filtering and content-based filtering have become ubiquitous in online retail, enhancing customer engagement by suggesting products tailored to individual tastes. Python’s Surprise library is quite valuable in building these recommendation engines, allowing for the incorporation of user preferences and item characteristics.
from surprise import Dataset, Reader, SVD from surprise.model_selection import train_test_split # Load sample dataset data = Dataset.load_builtin('ml-100k') reader = Reader(rating_scale=(1, 5)) # Prepare data for training trainset, testset = train_test_split(data.build_full_trainset(), test_size=0.2) # Train a collaborative filtering model algo = SVD() algo.fit(trainset) # Make predictions predictions = algo.test(testset) print(predictions)
Another impactful application of machine learning in fashion is the use of image recognition technologies to analyze fashion trends visually. Convolutional Neural Networks (CNNs) can be trained to identify patterns in clothing, colors, and styles from images, providing insights that can inform design decisions. Python’s TensorFlow and Keras libraries make the development of these deep learning models accessible and efficient.
from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense # Defining a simple CNN model model = Sequential() model.add(Conv2D(32, (3, 3), activation='relu', input_shape=(64, 64, 3))) model.add(MaxPooling2D(pool_size=(2, 2))) model.add(Flatten()) model.add(Dense(128, activation='relu')) model.add(Dense(1, activation='sigmoid')) # Binary classification model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
Furthermore, machine learning assists in supply chain optimization through predictive analytics. By forecasting demand based on historical sales data, fashion businesses can better manage inventory, minimizing excess stock and stockouts. Python’s Scikit-learn library is well-equipped for building models that can predict future sales trends based on various input features.
from sklearn.linear_model import LinearRegression # Sample sales data X = df[['advertising_budget', 'season']] y = df['sales'] # Fitting a linear regression model model = LinearRegression() model.fit(X, y) # Making predictions predictions = model.predict(X) print(predictions)
The integration of machine learning within fashion technology not only streamlines operational processes but also enhances the strategic decision-making framework, leading to more informed and agile responses to market changes. As Python continues to evolve, its role in fashion technology will undoubtedly expand, fostering further innovation and efficiency in this dynamic industry.
Visualizing Fashion Trends with Python
In the vibrant world of fashion, visualizations play an important role in translating complex data into understandable insights. Python’s powerful visualization libraries enable fashion analysts and designers to present data in ways that can reveal trends, highlight correlations, and forecast future shifts. Using tools such as matplotlib, seaborn, and Plotly, professionals can create stunning visual narratives that resonate with stakeholders and audiences alike.
One of the fundamental libraries for data visualization in Python is matplotlib. It provides a flexible platform to generate a wide range of static, animated, and interactive plots. For example, when analyzing sales trends over time, a line plot can be created to visualize how sales fluctuate throughout the seasons:
import matplotlib.pyplot as plt import pandas as pd # Sample sales data data = { 'date': pd.date_range(start='2023-01-01', periods=12, freq='M'), 'sales': [200, 220, 250, 280, 300, 260, 300, 320, 340, 360, 380, 400] } df = pd.DataFrame(data) # Creating a line plot for sales trends plt.figure(figsize=(10, 5)) plt.plot(df['date'], df['sales'], marker='o') plt.title('Monthly Sales Trends') plt.xlabel('Date') plt.ylabel('Sales ($)') plt.grid() plt.xticks(rotation=45) plt.tight_layout() plt.show()
Another library, seaborn, builds on matplotlib and provides a higher-level interface for creating visually appealing graphics. It offers built-in themes and color palettes to help analysts create aesthetic visualizations with minimal effort. For instance, a bar plot can be used to compare sales by product category:
import seaborn as sns # Sample product category sales data category_data = { 'category': ['Dresses', 'Tops', 'Bottoms', 'Accessories'], 'sales': [1500, 1200, 800, 500] } category_df = pd.DataFrame(category_data) # Creating a bar plot plt.figure(figsize=(8, 4)) sns.barplot(x='category', y='sales', data=category_df, palette='viridis') plt.title('Sales by Product Category') plt.xlabel('Category') plt.ylabel('Sales ($)') plt.tight_layout() plt.show()
For more interactive visualizations, Plotly is an excellent choice. It allows users to create interactive graphs that can be embedded into web applications or dashboards. By using Plotly, fashion analysts can provide stakeholders with interactive capabilities to explore data dynamically. For instance, ponder creating an interactive scatter plot to explore the relationship between advertising spend and sales:
import plotly.express as px # Sample advertising spend and sales data scatter_data = { 'advertising_budget': [1000, 1500, 2000, 2500, 3000], 'sales': [200, 250, 300, 350, 400] } scatter_df = pd.DataFrame(scatter_data) # Creating an interactive scatter plot fig = px.scatter(scatter_df, x='advertising_budget', y='sales', title='Sales vs. Advertising Budget', labels={'advertising_budget': 'Advertising Budget ($)', 'sales': 'Sales ($)'}) fig.show()
Using these visualization techniques, fashion analysts can effectively communicate their findings and insights. Well-designed visualizations not only make the data more accessible but also facilitate better decision-making within the industry. By using Python’s visualization capabilities, stakeholders are equipped to recognize patterns, understand dynamics, and ultimately, make more informed choices in the fast-paced world of fashion.
Case Studies: Successful Implementations of Python in Fashion
Within the scope of fashion technology, a high number of case studies demonstrate Python’s transformative influence on various aspects of the industry. One prominent example can be seen in a leading fashion retail brand that utilized Python for demand forecasting. By aggregating historical sales data, promotional activities, and external factors such as weather patterns, they developed a predictive model using the Scikit-learn library. This model enabled them to adjust inventory levels dynamically, minimizing stockouts and excess inventory, ultimately leading to a significant increase in sales efficiency.
from sklearn.ensemble import RandomForestRegressor import pandas as pd # Sample dataset creation data = { 'previous_sales': [100, 120, 130, 170, 150], 'advertising_budget': [1000, 1500, 2000, 2500, 3000], 'weather_impact': [0.2, 0.1, 0.3, 0.4, 0.3], 'sales': [110, 135, 145, 180, 160] } df = pd.DataFrame(data) # Splitting data into features and target variable X = df[['previous_sales', 'advertising_budget', 'weather_impact']] y = df['sales'] # Fitting the Random Forest model model = RandomForestRegressor(n_estimators=100) model.fit(X, y) # Making predictions predictions = model.predict(X) print(predictions)
Another compelling case study is focused on a fashion e-commerce platform that harnessed Natural Language Processing (NLP) to analyze customer reviews. By employing Python’s NLTK library, they processed and classified sentiments from thousands of reviews, gaining insights into customer preferences and emerging trends. This data not only informed their product development but also enhanced marketing strategies, ensuring they aligned with the evolving tastes of their consumer base.
import nltk from nltk.sentiment import SentimentIntensityAnalyzer # Sample customer review data reviews = [ "Absolutely love this new collection!", "The quality is not as expected.", "Great style, but the sizing is off.", "I am thrilled with my purchase!", "Not worth the price." ] # Sentiment analysis nltk.download('vader_lexicon') sia = SentimentIntensityAnalyzer() sentiments = [sia.polarity_scores(review) for review in reviews] # Converting to DataFrame for easier analysis sentiment_df = pd.DataFrame(sentiments) print(sentiment_df)
A further illustration of Python’s application is found in a fashion startup that relied on image recognition technology to analyze social media trends. By training a Convolutional Neural Network (CNN) using TensorFlow, they processed images from platforms like Instagram to identify trending colors, styles, and patterns. This capability allowed them to stay ahead of fashion trends and curate collections that resonated with their target audience.
from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense # Defining a simple CNN model model = Sequential() model.add(Conv2D(32, (3, 3), activation='relu', input_shape=(64, 64, 3))) model.add(MaxPooling2D(pool_size=(2, 2))) model.add(Flatten()) model.add(Dense(128, activation='relu')) model.add(Dense(1, activation='sigmoid')) # Binary classification for trending or not model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
These case studies collectively show how Python is not merely a programming language within fashion technology; it’s a catalyst for innovation, enabling companies to leverage data-driven strategies effectively. From predictive modeling and sentiment analysis to image recognition, Python’s versatility is paving the way for informed decision-making and strategic advancement in an industry that thrives on trends and consumer engagement.
Future Perspectives: Python’s Impact on Fashion Innovation
As the fashion industry continues to evolve, the integration of Python is anticipated to have a profound impact on innovation and creativity. The future of fashion technology is being shaped by the capabilities Python offers, particularly in data analysis, machine learning, and automation. The trend towards data-driven decision-making is not only enhancing operational efficiency but also enabling designers and marketers to develop collections that resonate with consumer desires.
One significant area where Python is expected to drive innovation is in the creation of hyper-personalized shopping experiences. As consumers increasingly seek individualized products, fashion brands can leverage Python’s machine learning libraries, such as TensorFlow and Scikit-learn, to analyze customer data and develop tailored recommendations. By implementing advanced algorithms that predict individual preferences based on browsing history, purchase patterns, and social media interactions, brands can curate a shopping experience that feels uniquely crafted for each customer.
from sklearn.ensemble import RandomForestClassifier import pandas as pd # Sample customer data data = { 'age': [25, 34, 28, 40, 22, 36, 30], 'previous_purchases': [5, 2, 3, 7, 1, 4, 6], 'clicks': [10, 15, 5, 20, 1, 12, 8], 'target_category': ['Dresses', 'Tops', 'Dresses', 'Bottoms', 'Accessories', 'Tops', 'Dresses'] } df = pd.DataFrame(data) # Preparing data for classification X = df[['age', 'previous_purchases', 'clicks']] y = df['target_category'] # Fitting the Random Forest model model = RandomForestClassifier(n_estimators=100) model.fit(X, y) # Making predictions for a new customer new_customer = pd.DataFrame({'age': [29], 'previous_purchases': [3], 'clicks': [15]}) predicted_category = model.predict(new_customer) print(predicted_category)
Moreover, Python’s capabilities in Natural Language Processing (NLP) are poised to revolutionize how fashion brands engage with their audience. By analyzing social media conversations, customer feedback, and market trends, brands can gain valuable insights into consumer sentiment and emerging trends. With tools like SpaCy and NLTK, brands can not only monitor sentiment but also identify key themes and topics that resonate with their audience, allowing for more agile marketing strategies and product launches.
import spacy # Load the English NLP model nlp = spacy.load("en_core_web_sm") # Sample social media comments comments = [ "I can't get enough of this new color trend!", "This style is so last year.", "Absolutely love the new collection!" ] # Analyzing sentiment and extracting key themes for comment in comments: doc = nlp(comment) for ent in doc.ents: print(f"Entity: {ent.text}, Label: {ent.label_}")
In addition to personalization and sentiment analysis, Python’s role in facilitating the circular economy in fashion is becoming more pronounced. Sustainability is increasingly becoming a priority for brands and consumers alike. By deploying Python for predictive analytics, brands can optimize their supply chains, reduce waste, and forecast demand more accurately. This not only fosters environmental responsibility but also enhances profitability, as brands can align their production more closely with consumer demand.
from sklearn.linear_model import LinearRegression # Sample data for inventory optimization data = { 'previous_sales': [100, 150, 120, 130, 140], 'advertising_budget': [1000, 1200, 1100, 1300, 1400], 'seasonality_factor': [1.2, 1.1, 1.3, 1.0, 1.2], 'forecasted_demand': [110, 160, 125, 135, 145] } df = pd.DataFrame(data) # Fitting a linear regression model for demand forecasting X = df[['previous_sales', 'advertising_budget', 'seasonality_factor']] y = df['forecasted_demand'] model = LinearRegression() model.fit(X, y) # Making future demand predictions future_data = pd.DataFrame({'previous_sales': [150], 'advertising_budget': [1500], 'seasonality_factor': [1.1]}) predicted_demand = model.predict(future_data) print(predicted_demand)
As we look ahead, the synergy between Python and fashion technology is likely to foster unprecedented creativity and innovation. The ability to harness big data, machine learning, and automation will empower fashion professionals to respond more swiftly to market changes, create innovative designs, and ultimately provide consumers with the products they desire. Therefore, the future of fashion is not only about style but also about the intelligent use of technology, and Python is at the forefront of this evolution.
The article offers a comprehensive look at how Python is revolutionizing fashion technology, but it could benefit from discussing the ethical implications of data collection and analysis. As consumer data becomes increasingly integral to targeted marketing and trend forecasting, considerations around privacy, consent, and the potential for algorithmic bias are crucial. Addressing these ethical dimensions would provide a more balanced view of the technologies being employed in the fashion industry.