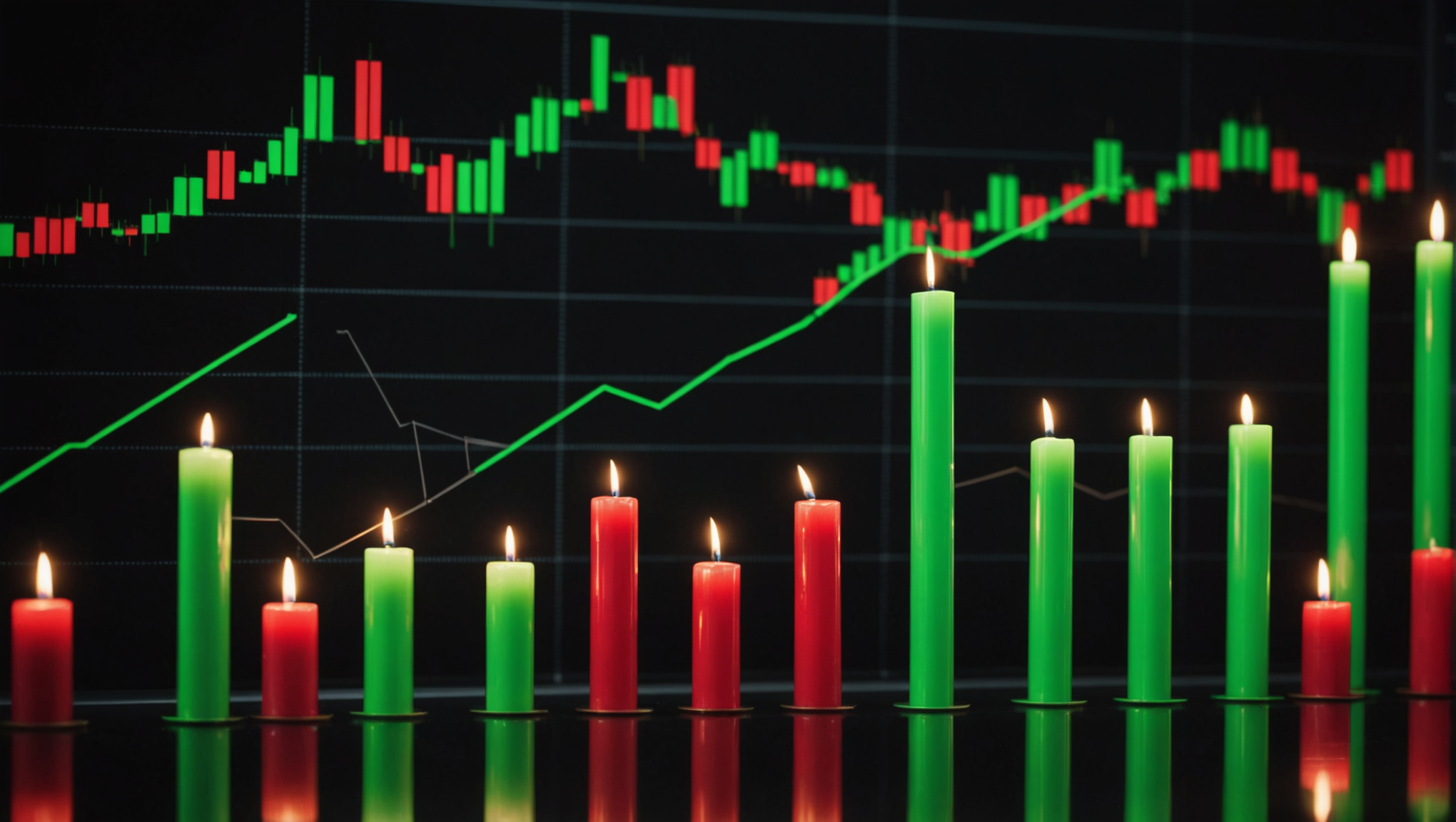
Python in Finance: Analyzing Financial Data
Financial data analysis is a discipline that has transformed with the advent of advanced programming languages like Python. The flexibility and power of Python make it a preferred choice among analysts and data scientists for handling complex financial data. With Python, one can efficiently gather, clean, analyze, and visualize financial data, facilitating insightful decision-making.
In the realm of finance, data analysis encompasses various tasks, including performance analysis of assets, risk management, portfolio optimization, and algorithmic trading strategies. Python’s extensive libraries and frameworks streamline these processes and allow for rapid prototyping and implementation of complex models.
One of the standout features of Python for financial data analysis is its ability to handle large datasets with ease. Whether you’re working with historical price data, economic indicators, or alternative data sources, Python can efficiently process and analyze this information. Additionally, Python’s readability promotes collaboration across teams, enabling financial professionals to share insights and methodologies without getting bogged down by convoluted syntax.
Furthermore, Python supports a variety of data sources, including APIs for financial data providers, databases, and CSV files. The ability to seamlessly integrate with these data sources ensures that analysts can access the most relevant and up-to-date information. Here’s an example of how to fetch historical stock data using the pandas_datareader library:
import pandas_datareader.data as web import datetime start = datetime.datetime(2020, 1, 1) end = datetime.datetime(2023, 1, 1) stock_data = web.DataReader('AAPL', 'yahoo', start, end) print(stock_data.head())
This snippet illustrates the simplicity with which you can retrieve financial data. The DataReader
function pulls data directly from Yahoo Finance, demonstrating Python’s convenience and utility in swiftly mobilizing data for analysis.
Python also excels in data cleaning and manipulation, often a prerequisite for meaningful analysis. With the powerful pandas library, analysts can manipulate DataFrames, handle missing data, and perform time-series operations, making it an indispensable tool in any financial analyst’s toolkit.
Moreover, the landscape of financial data analysis is continuously evolving, with machine learning and big data analytics becoming integral to contemporary finance. Python’s rich ecosystem of libraries for machine learning, such as scikit-learn and TensorFlow, allows analysts to build predictive models that can forecast market trends and inform investment strategies.
Python has become a cornerstone in the field of financial data analysis, enabling professionals to efficiently analyze and visualize data, develop robust models, and derive actionable insights from intricate datasets. As financial markets grow more complex, the importance of proficient data analysis cannot be overstated, and Python stands as a powerful ally in this pursuit.
Key Libraries for Financial Data Manipulation
In the realm of financial data manipulation, several key libraries stand out due to their robust functionality and ease of use. These libraries provide a wide range of capabilities that empower analysts to perform various operations on financial data, from basic data manipulation to complex financial modeling. Understanding these libraries is essential for anyone looking to leverage Python in financial analysis.
One of the most widely used libraries in the financial data analysis space is pandas. This library offers powerful data structures like Series and DataFrames, which facilitate efficient data manipulation and analysis. With pandas, you can easily read data from different sources, clean it, and perform exploratory data analysis. The `read_csv()` function, for instance, allows you to import datasets effortlessly:
import pandas as pd # Load a CSV file containing financial data df = pd.read_csv('financial_data.csv') print(df.head())
Once your data is loaded into a DataFrame, you can utilize a myriad of functions to manipulate it. For example, filtering data based on specific conditions is straightforward:
# Filter data for stocks with a closing price greater than $100 filtered_data = df[df['Close'] > 100] print(filtered_data)
Additionally, pandas provides powerful time-series functionality, allowing analysts to work with date and time data seamlessly. You can easily convert a column to datetime format, set it as the index, and perform operations like resampling:
# Convert a date column to datetime format df['Date'] = pd.to_datetime(df['Date']) df.set_index('Date', inplace=True) # Resample data to get monthly averages monthly_data = df.resample('M').mean() print(monthly_data)
Another important library in financial data manipulation is NumPy. Often used in conjunction with pandas, NumPy provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. Analysts can leverage NumPy for numerical calculations, performance improvements, and data handling. Here’s an example of using NumPy to compute the daily returns of a stock:
import numpy as np # Calculate daily returns df['Daily Return'] = df['Close'].pct_change() df['Daily Return'] = df['Daily Return'].fillna(0) # Calculate mean and standard deviation of daily returns mean_return = np.mean(df['Daily Return']) std_dev_return = np.std(df['Daily Return']) print(f'Mean Daily Return: {mean_return}, Standard Deviation: {std_dev_return}')
For more specialized financial analysis, the statsmodels library is invaluable. It provides classes and functions for estimating and testing statistical models, which is particularly useful for regression analysis in finance. Analysts can use statsmodels to perform time series analysis, estimating parameters, and conducting hypothesis tests:
import statsmodels.api as sm # Define the dependent and independent variables X = df['Market Return'] y = df['Stock Return'] # Add a constant to the independent variables X = sm.add_constant(X) # Fit the regression model model = sm.OLS(y, X).fit() print(model.summary())
Lastly, the scikit-learn library comes into play when it comes to machine learning. As predictive analytics becomes integral to financial decision-making, scikit-learn offers tools for classification, regression, clustering, and dimensionality reduction. Here’s how you can leverage scikit-learn to build a simple linear regression model:
from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression # Prepare data for modeling X = df[['Feature1', 'Feature2']] y = df['Target'] # Split the data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create and fit the model model = LinearRegression() model.fit(X_train, y_train) # Make predictions predictions = model.predict(X_test) print(predictions)
With these libraries—pandas, NumPy, statsmodels, and scikit-learn—analysts can manipulate financial data effectively, conduct sophisticated analyses, and develop predictive models that can drive investment strategies and business decisions. Their combined power makes Python a formidable tool in the arsenal of financial data analysts.
Data Visualization Techniques for Financial Insights
Data visualization plays an important role in financial analysis by transforming complex data sets into visual insights that can be easily interpreted. With the vast amount of financial data available, it’s imperative for analysts to utilize effective visualization techniques to identify trends, patterns, and anomalies that may not be immediately apparent in raw data. Python, with its rich ecosystem of visualization libraries, is particularly well-equipped to handle this task. Among the most popular libraries for data visualization in Python are Matplotlib, Seaborn, and Plotly.
Matplotlib is the foundational plotting library in Python and serves as the backbone for many other visualization libraries. It allows for the creation of static, animated, and interactive visualizations in Python. A simple example of using Matplotlib to plot stock price trends is shown below:
import matplotlib.pyplot as plt # Plot the closing price of a stock plt.figure(figsize=(14, 7)) plt.plot(df.index, df['Close'], label='Closing Price', color='blue') plt.title('Stock Closing Price Over Time') plt.xlabel('Date') plt.ylabel('Closing Price') plt.legend() plt.grid() plt.show()
This snippet generates a line chart that illustrates the closing price of a stock over a defined time period, providing a clear visual representation of price movements.
Seaborn builds on Matplotlib and provides a high-level interface for drawing attractive statistical graphics. It’s particularly useful for visualizing complex relationships and distributions in data. For example, if you want to visualize the distribution of daily returns, Seaborn can create a more aesthetically pleasing plot with minimal code:
import seaborn as sns # Create a distribution plot for daily returns plt.figure(figsize=(10, 6)) sns.histplot(df['Daily Return'], bins=30, kde=True, color='orange') plt.title('Distribution of Daily Returns') plt.xlabel('Daily Return') plt.ylabel('Frequency') plt.grid() plt.show()
In this example, the `histplot` function generates a histogram of daily returns, combined with a Kernel Density Estimate (KDE) to visualize the underlying distribution more smoothly.
For interactive visualizations, Plotly stands out as an excellent library. It allows users to create web-based plots that are responsive and can be embedded in web applications. Here’s how you can create an interactive candlestick chart to analyze stock price movements:
import plotly.graph_objects as go # Create a Candlestick chart fig = go.Figure(data=[go.Candlestick(x=df.index, open=df['Open'], high=df['High'], low=df['Low'], close=df['Close'])]) fig.update_layout(title='Candlestick Chart for Stock Prices', xaxis_title='Date', yaxis_title='Price', xaxis_rangeslider_visible=False) fig.show()
This Plotly example generates a candlestick chart, a popular visualization among traders that conveys price movements over time, allowing analysts to assess price trends and volatility.
Another essential visualization technique is the use of heatmaps to display correlations between multiple financial assets. This can be done conveniently with Seaborn:
# Calculate correlation matrix correlation_matrix = df.corr() # Create a heatmap plt.figure(figsize=(12, 8)) sns.heatmap(correlation_matrix, annot=True, cmap='coolwarm', fmt=".2f") plt.title('Correlation Heatmap of Financial Assets') plt.show()
The heatmap provides an immediate visual cue to understand the relationships between different assets, enabling analysts to make informed decisions based on correlations.
Effective data visualization in finance not only enhances comprehension but also supports storytelling with data. By using these libraries and techniques, financial analysts can convey complex insights succinctly and powerfully, contributing to more informed decision-making processes.
Time Series Analysis in Finance
import pandas as pd import numpy as np import matplotlib.pyplot as plt import statsmodels.api as sm from datetime import datetime, timedelta # Simulate some financial data for demonstration dates = pd.date_range(start='2020-01-01', end='2023-01-01', freq='D') np.random.seed(0) data = np.random.randn(len(dates)).cumsum() + 100 # Cumulative sum to simulate price data # Create a DataFrame df = pd.DataFrame(data, columns=['Price'], index=dates) # Calculate daily returns df['Daily Return'] = df['Price'].pct_change().fillna(0) # Visualize the price data plt.figure(figsize=(14, 7)) plt.plot(df.index, df['Price'], label='Price', color='blue') plt.title('Simulated Stock Price Over Time') plt.xlabel('Date') plt.ylabel('Price') plt.legend() plt.grid() plt.show() # Perform a time series decomposition from statsmodels.tsa.seasonal import seasonal_decompose # Decompose the time series decomposition = seasonal_decompose(df['Price'], model='additive', period=365) trend = decomposition.trend seasonal = decomposition.seasonal residual = decomposition.resid # Plot the decomposition plt.figure(figsize=(14, 10)) plt.subplot(411) plt.plot(df['Price'], label='Original', color='blue') plt.legend(loc='upper left') plt.subplot(412) plt.plot(trend, label='Trend', color='orange') plt.legend(loc='upper left') plt.subplot(413) plt.plot(seasonal, label='Seasonal', color='green') plt.legend(loc='upper left') plt.subplot(414) plt.plot(residual, label='Residual', color='red') plt.legend(loc='upper left') plt.tight_layout() plt.show() # Fit an ARIMA model from statsmodels.tsa.arima.model import ARIMA # Fit ARIMA model model = ARIMA(df['Price'], order=(5, 1, 0)) # Example parameters for ARIMA model_fit = model.fit() # Summary of the model print(model_fit.summary()) # Forecasting forecast = model_fit.forecast(steps=30) # Forecasting the next 30 days plt.figure(figsize=(14, 7)) plt.plot(df.index, df['Price'], label='Historical Price', color='blue') plt.plot(pd.date_range(start=df.index[-1] + timedelta(days=1), periods=30, freq='D'), forecast, label='Forecasted Price', color='red') plt.title('Price Forecasting with ARIMA') plt.xlabel('Date') plt.ylabel('Price') plt.legend() plt.grid() plt.show()
Time series analysis is a cornerstone of financial data analysis, allowing analysts to extract meaningful insights from temporal data. In finance, this often involves examining historical price movements, identifying trends, and making forecasts. This analysis is critical for portfolio management, risk assessment, and strategic decision-making.
Python’s capabilities for time series analysis are enhanced by libraries such as pandas, statsmodels, and NumPy. A typical workflow includes loading data, cleaning it, conducting exploratory analysis, and fitting statistical models to derive insights.
An essential part of analyzing financial time series is understanding the underlying components: trend, seasonality, and noise. Time series decomposition techniques, such as those provided by statsmodels, allow analysts to separate these components, making it easier to interpret the underlying data. The demonstration above illustrates how to simulate price data, calculate daily returns, and visualize the original series alongside its decomposed components.
Modeling time series data often employs autoregressive integrated moving average (ARIMA) models, which are popular for their ability to model various types of time series data. The capacity to forecast future values based on past observations is especially valuable in finance, where accurate predictions can drive significant strategic advantages.
In the example, we fit an ARIMA model to the simulated price data and generate forecasts for future price movements. This kind of analysis is indispensable for traders and investment managers seeking to optimize their strategies based on historical performance.
Ultimately, the ability to conduct comprehensive time series analysis in Python equips financial analysts with the tools to make informed decisions, derive actionable insights, and better understand the dynamics of financial markets.
Building Predictive Models for Financial Forecasting
# Import necessary libraries import pandas as pd import numpy as np import matplotlib.pyplot as plt import statsmodels.api as sm from datetime import datetime, timedelta from statsmodels.tsa.arima.model import ARIMA # Simulate some financial data for demonstration dates = pd.date_range(start='2020-01-01', end='2023-01-01', freq='D') np.random.seed(0) data = np.random.randn(len(dates)).cumsum() + 100 # Cumulative sum to simulate price data # Create a DataFrame df = pd.DataFrame(data, columns=['Price'], index=dates) # Calculate daily returns df['Daily Return'] = df['Price'].pct_change().fillna(0) # Visualize the price data plt.figure(figsize=(14, 7)) plt.plot(df.index, df['Price'], label='Price', color='blue') plt.title('Simulated Stock Price Over Time') plt.xlabel('Date') plt.ylabel('Price') plt.legend() plt.grid() plt.show() # Perform a time series decomposition from statsmodels.tsa.seasonal import seasonal_decompose # Decompose the time series decomposition = seasonal_decompose(df['Price'], model='additive', period=365) trend = decomposition.trend seasonal = decomposition.seasonal residual = decomposition.resid # Plot the decomposition plt.figure(figsize=(14, 10)) plt.subplot(411) plt.plot(df['Price'], label='Original', color='blue') plt.legend(loc='upper left') plt.subplot(412) plt.plot(trend, label='Trend', color='orange') plt.legend(loc='upper left') plt.subplot(413) plt.plot(seasonal, label='Seasonal', color='green') plt.legend(loc='upper left') plt.subplot(414) plt.plot(residual, label='Residual', color='red') plt.legend(loc='upper left') plt.tight_layout() plt.show() # Fit an ARIMA model model = ARIMA(df['Price'], order=(5, 1, 0)) # Example parameters for ARIMA model_fit = model.fit() # Summary of the model print(model_fit.summary()) # Forecasting forecast = model_fit.forecast(steps=30) # Forecasting the next 30 days plt.figure(figsize=(14, 7)) plt.plot(df.index, df['Price'], label='Historical Price', color='blue') plt.plot(pd.date_range(start=df.index[-1] + timedelta(days=1), periods=30, freq='D'), forecast, label='Forecasted Price', color='red') plt.title('Price Forecasting with ARIMA') plt.xlabel('Date') plt.ylabel('Price') plt.legend() plt.grid() plt.show()
The analysis of financial data often involves the construction of predictive models that can forecast future price movements based on historical trends. In this context, ARIMA (AutoRegressive Integrated Moving Average) models are a cornerstone technique, thanks to their ability to effectively capture the underlying patterns in time series data.
To initiate the process, we start by simulating some financial data to work with. This allows us to explore how ARIMA models function without relying on real-world data. The simulated data, representing daily stock prices, is generated using a random walk approach, cumulatively summing normally distributed random numbers to mimic price behavior.
The first step in our analysis is to visualize this simulated price data. By plotting the price over time, we can get an initial sense of the trends and fluctuations present in the dataset. After plotting the prices, we calculate daily returns, which represent the percentage change in price from one day to the next. This information is important for understanding volatility and potential risk associated with the asset.
Next, we perform a time series decomposition, which breaks down the price data into its constituent components: trend, seasonality, and residuals. This decomposition clarifies the underlying dynamics that may affect price movements, allowing us to analyze how these components interact over time. Visualizing the results reveals insights into whether there is a clear upward or downward trend and how seasonal effects may influence price behavior.
Once we have a solid understanding of the data through visualization and decomposition, we can proceed to fit an ARIMA model. The choice of model parameters (p, d, q) is critical and typically requires some experimentation or analysis of autocorrelation and partial autocorrelation plots. In our example, we specify (5, 1, 0) as the ARIMA parameters, which means we are using a lag of 5 for the autoregressive component, one differencing to stabilize the series, and no moving average component.
After fitting the model, we can summarize its results, providing insights into the coefficients and their significance. This summary helps us understand how well the model fits the historical data and the relationships captured within the dataset.
Finally, the real power of ARIMA comes into play when we use it to forecast future values. By generating predictions for the next 30 days, we can visualize both the historical prices and the forecasted values on the same plot. This not only allows us to assess the model’s performance visually but also assists in making informed decisions based on projected trends.
The capability to build predictive models using ARIMA in Python underscores the language’s robustness as a tool for finance professionals. By using the power of time series analysis, analysts can sharpen their forecasting abilities, providing a strategic edge in financial decision-making.
Case Studies: Real-World Applications of Python in Finance
# Import necessary libraries import pandas as pd import numpy as np import matplotlib.pyplot as plt from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression from sklearn.metrics import mean_squared_error # Simulate some financial data for demonstration dates = pd.date_range(start='2020-01-01', end='2023-01-01', freq='D') np.random.seed(0) data = np.random.randn(len(dates)).cumsum() + 100 # Cumulative sum to simulate price data # Create a DataFrame df = pd.DataFrame(data, columns=['Price'], index=dates) # Calculate features for modeling df['Daily Return'] = df['Price'].pct_change().fillna(0) # Prepare data for modeling # Creating lagged features for lag in range(1, 6): # Creating 5 lagged features df[f'Lag_{lag}'] = df['Daily Return'].shift(lag) # Drop missing values due to lagging df.dropna(inplace=True) # Define the features and target variable X = df[[f'Lag_{lag}' for lag in range(1, 6)]] y = df['Daily Return'] # Split the data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create and fit the model model = LinearRegression() model.fit(X_train, y_train) # Make predictions predictions = model.predict(X_test) # Evaluate the model mse = mean_squared_error(y_test, predictions) print(f'Mean Squared Error: {mse}') # Visualize predictions vs actual returns plt.figure(figsize=(10, 6)) plt.plot(y_test.index, y_test, label='Actual Returns', color='blue') plt.plot(y_test.index, predictions, label='Predicted Returns', color='red', linestyle='--') plt.title('Predicted vs Actual Returns') plt.xlabel('Date') plt.ylabel('Daily Return') plt.legend() plt.grid() plt.show()
Building predictive models for financial forecasting is a critical component in the contemporary finance landscape. These models help analysts understand potential future movements in asset prices, enabling informed investment decisions. In the realm of finance, we often leverage historical price data to create features that can predict future returns.
To illustrate the process, we begin by simulating financial data, allowing us to explore the predictive modeling without the constraints of real-world data. The simulated data, representing daily stock prices, is generated using a cumulative sum of random numbers, simulating the behavior of price movements over time.
Once we have our dataset, we calculate daily returns, which serve as the target variable for our predictive model. Next, we create lagged features, representing past values of daily returns. That is important, as past returns often correlate with future movements, a phenomenon commonly observed in financial markets.
After laying out our features, we split the dataset into training and testing subsets. This step ensures that we can evaluate the model’s performance on unseen data, which is vital for assessing its predictive capabilities.
We then fit a simple linear regression model to our training data. The model learns the relationship between the lagged features and the current return, allowing it to make predictions based on the patterns it identifies. Once trained, the model is applied to the test data, generating predictions for daily returns.
Evaluating our model is essential. We calculate the mean squared error (MSE) between the predicted and actual returns, providing a quantitative measure of the model’s performance. A lower MSE indicates better predictive accuracy, crucial for financial decision-making.
Finally, we visualize the predictions against the actual returns. This visual representation allows analysts to assess the model’s performance intuitively, understanding where it succeeds and where it falls short. Such insights are invaluable for refining the model and improving its predictive power.
The ability to build effective predictive models in Python represents a powerful tool in the arsenal of financial analysts, enabling them to navigate the complexities of financial markets with greater confidence and precision.