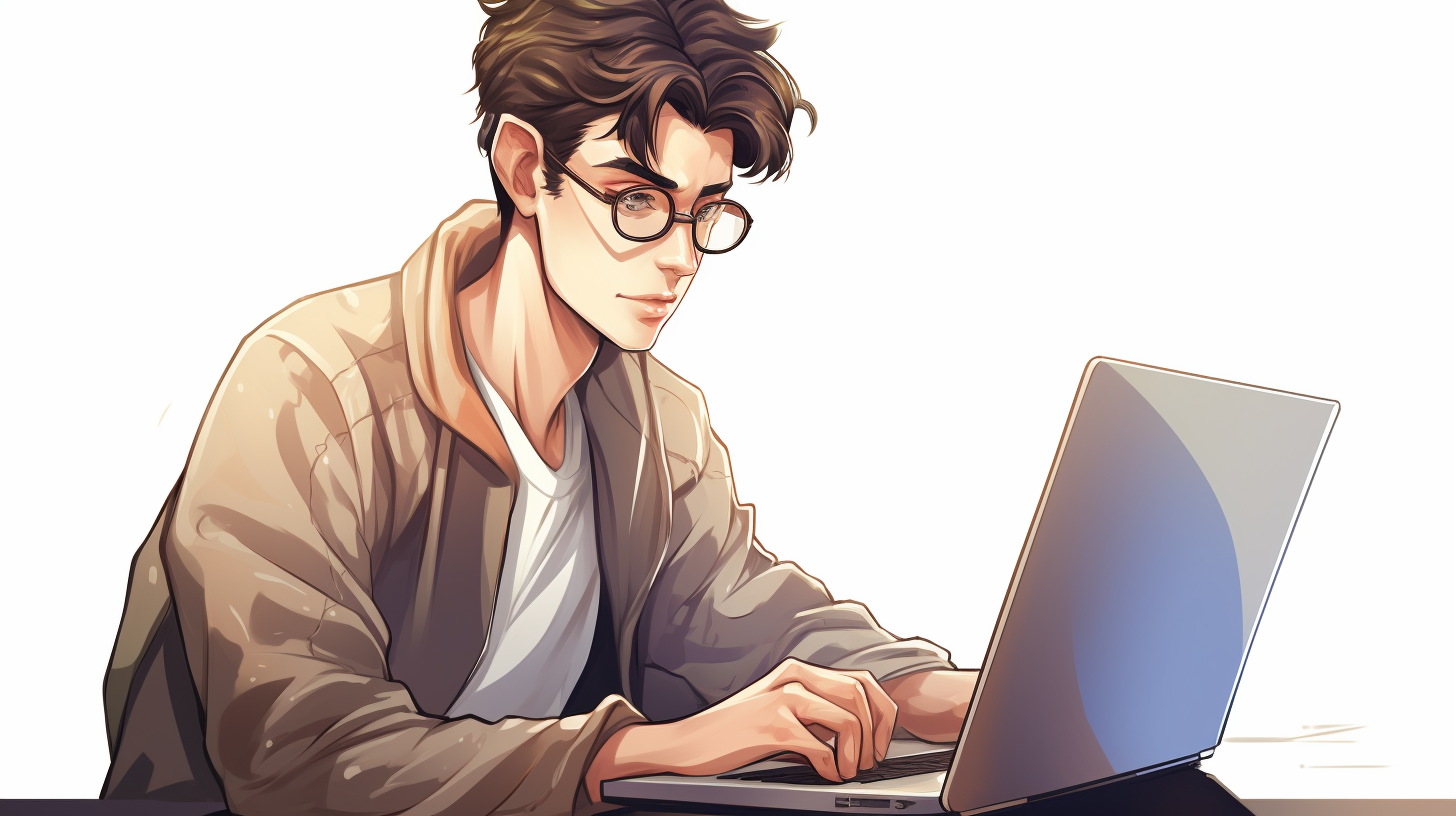
Python in Manufacturing: Process Optimization
Process optimization in manufacturing is a critical aspect that directly impacts productivity, efficiency, and cost-effectiveness. At its core, process optimization involves analyzing various facets of the production workflow to identify inefficiencies and opportunities for improvement. With the increasing complexity of manufacturing processes, using data-driven insights has become paramount.
In traditional manufacturing environments, decision-making often relied on experience and intuition. However, as industries evolve, the integration of data analytics has transformed this landscape. Manufacturers now have access to vast amounts of data generated from equipment, production lines, and supply chains. The challenge lies in extracting actionable insights from this data to refine processes.
To understand process optimization, one must think several key elements:
- Gathering data from various sources such as sensors, machines, and production logs is the first step. This data serves as the foundation for analysis.
- Using statistical methods and machine learning algorithms, manufacturers can analyze historical data to identify patterns and trends.
- Creating simulations of manufacturing processes can help visualize potential improvements without disrupting actual production.
- Once optimization opportunities are identified, implementing changes in a controlled manner is important to ensure that the production process does not suffer.
- Optimization is not a one-time effort; continuous monitoring and adjustment are necessary to adapt to ever-changing conditions.
Python emerges as a powerful tool in this optimization journey. Its rich ecosystem of libraries allows for efficient data manipulation, analysis, and visualization. For instance, libraries like Pandas and Numpy facilitate data handling, while Matplotlib and Seaborn make it easier to visualize the results of analysis, helping stakeholders make informed decisions.
Here’s an example of how to use Python to analyze production data:
import pandas as pd # Load production data data = pd.read_csv('production_data.csv') # Calculate the average production output average_output = data['output'].mean() # Identify days with below-average performance below_average_days = data[data['output'] < average_output] print("Average Production Output:", average_output) print("Days with Below Average Output:") print(below_average_days)
This simple script reads production data, computes the average output, and identifies days when production fell below this average. Such insights can help managers pinpoint issues in the production line and take proactive measures.
In sum, understanding process optimization in manufacturing is a multifaceted endeavor that benefits immensely from data analysis and Python’s capabilities. With the right approach, manufacturers can not only enhance their operational efficiency but also gain a competitive edge in the market.
Key Python Libraries for Data Analysis
The landscape of data analysis in manufacturing is significantly enhanced by the integration of key Python libraries that specialize in handling, processing, and visualizing data. These libraries are crucial for extracting meaningful insights that can drive process optimization. Here are some of the most prominent Python libraries that every manufacturing data analyst should be familiar with:
Pandas: That’s perhaps the most essential library for data manipulation and analysis. Pandas provides powerful data structures, such as DataFrames, which allow for easy storage and manipulation of structured data. It simplifies tasks such as data cleaning, merging datasets, and filtering data based on conditions.
import pandas as pd # Example of data cleaning with Pandas data = pd.read_csv('production_data.csv') data.dropna(inplace=True) # Remove rows with missing values data['date'] = pd.to_datetime(data['date']) # Convert date strings to datetime objects print(data.head()) # Display the first few rows of the cleaned data
Numpy: This library is indispensable for numerical computations. It provides support for arrays and matrices, along with a wide array of mathematical functions to operate on these data structures. When dealing with large datasets, Numpy’s efficiency becomes a major asset, allowing for fast calculations.
import numpy as np # Example of using Numpy for calculations production_output = np.array(data['output']) average_output = np.mean(production_output) # Calculate average using Numpy print("Average Production Output using Numpy:", average_output)
Matplotlib and Seaborn: Visualization is a key component of data analysis that aids in understanding trends and patterns. Matplotlib is a plotting library that allows for the creation of static, animated, and interactive visualizations in Python. Seaborn, built on top of Matplotlib, offers a high-level interface for drawing attractive statistical graphics.
import matplotlib.pyplot as plt import seaborn as sns # Example of visualizing production output plt.figure(figsize=(10, 6)) sns.lineplot(data=data, x='date', y='output', marker='o') plt.title('Production Output Over Time') plt.xlabel('Date') plt.ylabel('Output') plt.xticks(rotation=45) plt.tight_layout() plt.show()
Scikit-learn: As the manufacturing industry increasingly incorporates machine learning into its processes, Scikit-learn has become a vital library. It provides simple and efficient tools for data mining and data analysis, including a wide variety of algorithms for classification, regression, clustering, and dimensionality reduction.
from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression # Example of using Scikit-learn for predictive analysis X = data[['input_variable1', 'input_variable2']] # Features y = data['output'] # Target variable X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) model = LinearRegression() model.fit(X_train, y_train) predictions = model.predict(X_test) print("Predictions on Test Data:", predictions)
By mastering these libraries, data analysts in the manufacturing sector can unlock the potential of their datasets, leading to significant improvements in effectiveness and productivity. The synergy between these libraries enables a comprehensive approach to data analysis, making it possible to derive actionable insights that drive process optimization.
Implementing Machine Learning for Predictive Maintenance
In the sphere of predictive maintenance, machine learning plays a transformative role by anticipating equipment failures before they occur. By using historical data from machinery and operational parameters, manufacturers can create state-of-the-art models that predict when maintenance should be performed, thus avoiding unexpected downtime. This predictive capability is vital, as it not only reduces costs associated with unplanned maintenance but also enhances the overall efficiency of the manufacturing process.
To implement machine learning for predictive maintenance, we start by gathering relevant data, which often includes a combination of machine operational data, maintenance logs, and environmental conditions. This data is then preprocessed to improve its quality and relevance for analysis. A common approach is to label the data based on failure occurrences, enabling supervised learning techniques to recognize patterns associated with equipment failures.
Here’s a concise example of how to prepare data and implement a machine learning model using Python for predictive maintenance:
import pandas as pd from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier from sklearn.metrics import classification_report, confusion_matrix # Load the maintenance dataset data = pd.read_csv('maintenance_data.csv') # Preprocessing: Drop rows with missing values and encode categorical variables data.dropna(inplace=True) data = pd.get_dummies(data, drop_first=True) # Define features and target variable X = data.drop('failure', axis=1) # Features y = data['failure'] # Target variable # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Initialize and train the Random Forest model model = RandomForestClassifier(n_estimators=100, random_state=42) model.fit(X_train, y_train) # Make predictions y_pred = model.predict(X_test) # Evaluate the model print(confusion_matrix(y_test, y_pred)) print(classification_report(y_test, y_pred))
In this example, we use the Random Forest algorithm, a robust choice for classification tasks, due to its ability to handle large datasets and its effectiveness in dealing with diverse feature types. The model is trained on historical maintenance data, where the target variable indicates whether a failure occurred. After training, the model’s performance is evaluated using confusion matrices and classification reports, providing insights into its accuracy and reliability.
Furthermore, once an effective model is developed, it can be deployed into the manufacturing environment. By continuously feeding it with real-time data, the model can refine its predictions, adapting to changes in machinery behavior and operational conditions. This adaptability especially important for maintaining high levels of accuracy over time.
Moreover, integrating predictive maintenance solutions with existing manufacturing systems can be achieved through automation scripts that trigger alerts or schedule maintenance when the model predicts an imminent failure. This proactive approach not only minimizes operational disruptions but also facilitates a more efficient allocation of maintenance resources.
By using the power of machine learning in predictive maintenance, manufacturers can significantly enhance their process optimization efforts. The shift from reactive to proactive maintenance strategies leads to a more streamlined operation, reduced costs, and ultimately, a more competitive position within the industry.
Streamlining Production with Automation Scripts
Automation scripts are at the forefront of streamlining production in manufacturing environments. By automating repetitive tasks and processes, manufacturers can significantly reduce the likelihood of human error, enhance efficiency, and free up valuable time for employees to focus on higher-level decision-making. Python, with its simple syntax and rich ecosystem of libraries, serves as an ideal language for creating these automation scripts.
One of the common applications of automation scripts in manufacturing includes automating data collection and reporting. For example, a script can be written to automatically pull data from production databases, process it, and generate performance reports in a standardized format. This not only saves time but also ensures that the data is consistently reported.
Here’s an illustrative Python script that demonstrates how to automate the generation of daily production reports using the Pandas library:
import pandas as pd from datetime import datetime, timedelta # Function to generate daily production report def generate_daily_report(): # Load production data data = pd.read_csv('production_data.csv') # Filter data for the last day yesterday = datetime.now() - timedelta(days=1) yesterday_str = yesterday.strftime('%Y-%m-%d') daily_data = data[data['date'] == yesterday_str] # Calculate total output and number of units produced total_output = daily_data['output'].sum() total_units = daily_data['units'].count() # Create a summary report report = f"Daily Production Report for {yesterday_str}n" report += f"Total Output: {total_output}n" report += f"Total Units Produced: {total_units}n" # Save report to a text file with open('daily_production_report.txt', 'w') as file: file.write(report) print("Daily report generated successfully.") # Call the function to generate the report generate_daily_report()
This script defines a function that loads production data, filters it for the previous day, computes total output and unit counts, and then generates a concise report saved to a text file. By scheduling this script to run at the end of each production day using a task scheduler, manufacturers can ensure that production data is automatically summarized and made readily available for review.
Another significant application of automation scripting is in inventory management. Python scripts can be employed to monitor stock levels in real-time and trigger alerts when items fall below predefined thresholds. This proactive approach helps in maintaining optimal inventory levels and avoiding production delays due to stock shortages.
Here’s an example of a simple automation script that checks inventory levels and sends an alert if any items are running low:
import pandas as pd import smtplib from email.mime.text import MIMEText # Load inventory data inventory = pd.read_csv('inventory_data.csv') # Function to check inventory levels def check_inventory(): low_stock_items = inventory[inventory['quantity'] < inventory['reorder_level']] if not low_stock_items.empty: send_alert(low_stock_items) # Function to send email alert def send_alert(low_stock_items): # Prepare email content items_list = low_stock_items.to_string(index=False) email_content = f"The following items are low in stock:n{items_list}" # Send email msg = MIMEText(email_content) msg['Subject'] = 'Low Inventory Alert' msg['From'] = '[email protected]' msg['To'] = '[email protected]' with smtplib.SMTP('smtp.example.com') as server: server.login('[email protected]', 'password') server.send_message(msg) print("Low inventory alert sent successfully.") # Call the inventory check function check_inventory()
This script checks the inventory data for any items whose quantity is below the reorder level. If any such items are found, it sends an email alert to the designated recipient. Automating this inventory monitoring process ensures that stock levels are managed efficiently without requiring constant manual checks.
By using the power of automation scripts in Python, manufacturers can streamline their production processes significantly. These scripts not only reduce the workload on employees but also ensure that critical tasks are completed consistently and accurately. As manufacturers continue to embrace automation, the integration of Python scripting into their workflows will play an essential role in driving operational efficiencies and enhancing overall productivity.
Case Studies: Successful Python Implementations in Industry
Driven by renewed attention to manufacturing, the implementation of Python has led to remarkable success stories across various sectors. These case studies exemplify how companies have harnessed the power of Python to address unique challenges, drive efficiencies, and enhance productivity.
One notable example comes from a leading automotive manufacturer that sought to optimize its supply chain operations. By using Python’s Pandas library, the company was able to integrate data from multiple sources, including suppliers, logistics, and production schedules. They developed a comprehensive dashboard that provided real-time visibility into the supply chain, enabling decision-makers to identify bottlenecks quickly and make informed adjustments. The result was a significant reduction in lead times and inventory costs.
import pandas as pd # Load supply chain data supply_chain_data = pd.read_csv('supply_chain_data.csv') # Group data by supplier and calculate average lead time average_lead_time = supply_chain_data.groupby('supplier')['lead_time'].mean() # Identify suppliers with above-average lead times slow_suppliers = average_lead_time[average_lead_time > average_lead_time.mean()] print("Suppliers with Above-Average Lead Times:") print(slow_suppliers)
Through this analysis, the manufacturer not only improved efficiency but also fostered better relationships with their suppliers by addressing issues promptly.
Another compelling case is that of a consumer electronics company that implemented a predictive maintenance solution using machine learning algorithms in Python. They collected operational data from their production equipment and used Scikit-learn to build a model that predicted potential failures. By transitioning from a reactive to a proactive maintenance approach, the company was able to reduce downtime significantly, leading to higher overall equipment effectiveness (OEE).
from sklearn.ensemble import RandomForestClassifier from sklearn.model_selection import train_test_split # Load the equipment data equipment_data = pd.read_csv('equipment_data.csv') # Preprocessing X = equipment_data.drop('failure', axis=1) # Features y = equipment_data['failure'] # Target variable # Split data into training and test sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Train the model model = RandomForestClassifier(n_estimators=100, random_state=42) model.fit(X_train, y_train) # Make predictions predictions = model.predict(X_test) print("Predictions on Test Data:", predictions)
This proactive maintenance strategy not only minimized unplanned downtime but also optimized maintenance schedules, thus reducing costs associated with emergency repairs and lost production.
Additionally, a food and beverage manufacturer utilized Python’s automation capabilities to improve their inventory management processes. They created a script that monitored stock levels and automatically generated reorder requests when supplies dipped below a certain threshold. This automation not only saved time but also minimized the risk of stockouts, ensuring that production could proceed smoothly without interruptions.
import smtplib from email.mime.text import MIMEText # Load inventory data inventory_data = pd.read_csv('inventory_data.csv') # Define the reorder threshold reorder_threshold = 100 # Check inventory levels low_stock_items = inventory_data[inventory_data['quantity'] < reorder_threshold] # Function to send alert for low stock def send_inventory_alert(low_stock_items): items_list = low_stock_items.to_string(index=False) email_content = f"The following items are low in stock:n{items_list}" msg = MIMEText(email_content) msg['Subject'] = 'Low Inventory Alert' msg['From'] = '[email protected]' msg['To'] = '[email protected]' with smtplib.SMTP('smtp.example.com') as server: server.login('[email protected]', 'password') server.send_message(msg) if not low_stock_items.empty: send_inventory_alert(low_stock_items) print("Low inventory alert sent successfully.")
This case study illustrates how automation can effectively mitigate risks associated with inventory management, driving operational efficiency and elevating service levels to customers.
These case studies collectively illustrate the transformative impact of Python in manufacturing. By using data analysis, machine learning, and automation capabilities, companies can tailor solutions to their specific challenges, paving the way for enhanced productivity and sustained competitive advantage in the market. As Python continues to gain traction within the industry, the potential for further innovations and success stories appears boundless.
Future Trends: The Role of Python in Smart Manufacturing
The future of manufacturing is moving towards a more interconnected and intelligent infrastructure, often referred to as smart manufacturing. At the heart of this evolution is the role of Python, which provides the flexibility and power needed to harness the vast amounts of data generated by modern manufacturing processes. As industries look to optimize their operations, Python emerges as a pivotal tool in integrating advanced technologies such as IoT, AI, and big data analytics.
Smart manufacturing leverages real-time data to make informed decisions, optimize production processes, and enhance product quality. Python plays a central role in this ecosystem, enabling manufacturers to develop sophisticated applications that process data from various sources, including sensors embedded in machinery, supply chain networks, and customer feedback.
One significant trend is the integration of Python with IoT devices. Manufacturers are increasingly using sensors to collect data on equipment performance, environmental conditions, and production metrics. Python’s ability to interface with these devices allows for seamless data collection and analysis. For instance, using libraries like MQTT and requests, manufacturers can easily communicate with IoT devices, retrieving data for further processing.
import paho.mqtt.client as mqtt # Define the MQTT callback function def on_message(client, userdata, message): print("Received message:", message.payload.decode()) # Initialize the MQTT client client = mqtt.Client() client.on_message = on_message # Connect to the MQTT broker client.connect("mqtt_broker_address") # Subscribe to a topic client.subscribe("manufacturing/sensor_data") # Start the loop to process messages client.loop_start()
In this example, a simple MQTT client subscribes to a topic that receives sensor data. This real-time data can be analyzed to detect anomalies or inefficiencies in production, leading to proactive adjustments and maintenance.
Another crucial aspect of smart manufacturing is predictive analytics, which employs machine learning models to forecast future production scenarios based on historical data. Python’s extensive libraries, such as TensorFlow and PyTorch, empower manufacturers to develop deep learning models that predict demand, optimize inventory levels, and enhance supply chain efficiency.
import pandas as pd from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestRegressor # Load historical production data data = pd.read_csv('historical_production_data.csv') # Prepare features and target variable X = data.drop('future_demand', axis=1) # Features y = data['future_demand'] # Target variable # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Initialize and train the Random Forest model model = RandomForestRegressor(n_estimators=100, random_state=42) model.fit(X_train, y_train) # Make predictions predictions = model.predict(X_test) print("Predictions on Future Demand:", predictions)
This code demonstrates how to train a Random Forest model to predict future demand based on historical production data. Such predictive capabilities allow manufacturers to align their production schedules with market demand, minimizing waste and maximizing resource use.
Furthermore, the integration of Python into manufacturing processes fosters collaboration and enhances transparency across the supply chain. With the help of web frameworks like Flask and Django, manufacturers can create dashboards that visualize key performance indicators (KPIs) in real-time, providing stakeholders with insights into production status, quality metrics, and operational bottlenecks.
from flask import Flask, jsonify import pandas as pd app = Flask(__name__) @app.route('/api/production_stats', methods=['GET']) def get_production_stats(): data = pd.read_csv('production_data.csv') # Calculate metrics total_output = data['output'].sum() return jsonify({"total_output": total_output}) if __name__ == '__main__': app.run(debug=True)
This simplistic Flask application exposes an API endpoint that returns total production output, enabling easy access to critical metrics by different stakeholders, thereby enhancing decision-making processes.
As we look forward, the role of Python in smart manufacturing will only expand. With the continued advancement of AI, machine learning, and IoT technologies, manufacturers are positioned to harness Python’s capabilities for deeper insights, more efficient processes, and ultimately, greater competitive advantages. The convergence of these technologies promises to reshape the manufacturing landscape, paving the way for innovations that were once unimaginable.