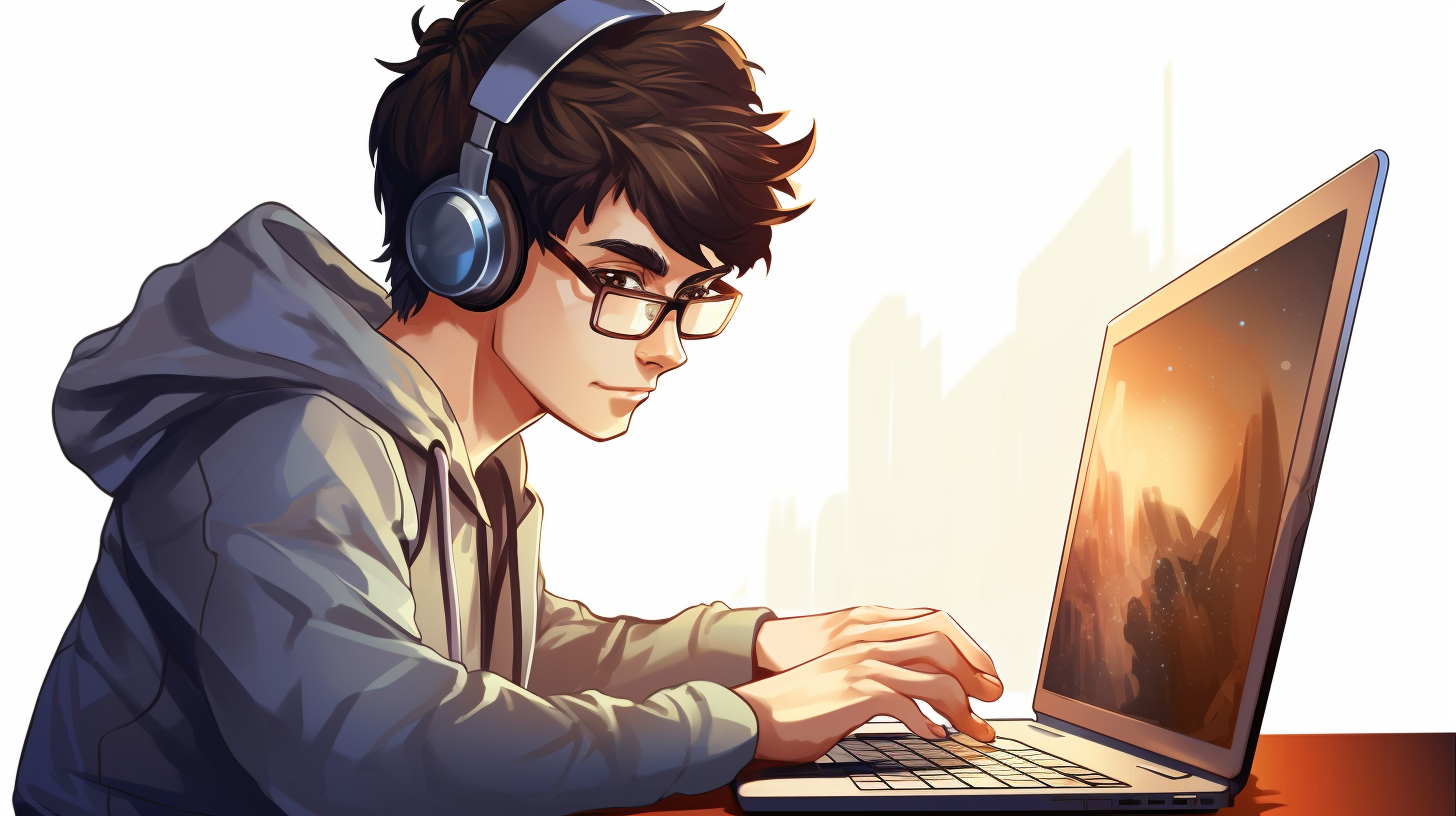
Python in Mining Industry: Data Analysis
Data analysis in the mining industry is a multifaceted discipline that leverages statistical techniques, advanced computational methods, and domain-specific knowledge to glean insights from vast amounts of data generated throughout the mining process. As mining operations become increasingly complex, the importance of data analysis has never been more pronounced. The industry is characterized by its reliance on heavy machinery, geological surveys, environmental impact assessments, and operational efficiency metrics—all of which produce substantial datasets that, when analyzed effectively, can lead to enhanced decision-making.
At its core, data analysis in mining seeks to optimize resource extraction while minimizing costs and environmental impacts. This involves exploring data from a variety of sources, including geological models, equipment performance metrics, and even social factors like community impact. The analysis can inform everything from operational strategies to predictive maintenance schedules, ultimately leading to more sustainable mining practices.
Traditionally, data analysis in the mining sector has operated on a conservative model—often relying on conventional statistical tools. However, with the advent of modern technology and the explosion of data availability, mining companies are increasingly turning to advanced techniques, such as machine learning and artificial intelligence. These methods can uncover hidden patterns and correlations that were previously obscured, enabling companies to anticipate issues before they arise and make data-driven decisions that enhance their operations.
As mining operations generate daily reams of data from various sensors and monitoring systems, the challenge lies in effectively integrating and analyzing this data. Python, with its diverse ecosystem of libraries and tools, has emerged as a preferred programming language for data analysis in mining. It provides data scientists and engineers with powerful capabilities to manipulate and visualize data, making it an essential resource in the industry’s data analysis arsenal.
Moreover, the application of data analysis in mining extends to risk management and compliance. Regulatory frameworks necessitate rigorous environmental monitoring and reporting, making data analysis essential not only for operational efficiency but also for adhering to legal standards. Companies that invest in robust data analysis capabilities are better positioned to navigate these complexities while maintaining competitiveness in a sector this is becoming more data-driven.
Key Python Libraries for Mining Data Analysis
Within the scope of data analysis within the mining industry, using the right tools can significantly enhance the ability to process and interpret complex datasets. Python stands out due to its simplicity and the extensive library ecosystem that it offers. Several key libraries have risen to prominence, each contributing unique capabilities that cater specifically to the demands of mining data analysis.
Pandas is arguably at the heart of data manipulation and analysis in Python. Its DataFrame structure allows users to handle large datasets efficiently, making it an ideal choice for mining operations that often deal with extensive geospatial and operational data. With Pandas, data can be cleaned, transformed, and aggregated with ease. For example, one can quickly calculate summary statistics or group data by geological features:
import pandas as pd # Load a dataset containing mining operational data data = pd.read_csv('mining_data.csv') # Group by geological feature and calculate mean production mean_production = data.groupby('geological_feature')['production'].mean() print(mean_production)
NumPy complements Pandas by providing support for numerical operations and efficient array processing. This library very important when performing mathematical computations that require speed and performance, such as matrix operations in geospatial analysis or simulations for resource estimation. NumPy’s array objects allow for operations that are both concise and computationally efficient.
import numpy as np # Create an array representing mineral concentrations concentrations = np.array([0.5, 1.2, 0.8, 1.5, 0.9]) # Calculate the mean and standard deviation of concentrations mean_concentration = np.mean(concentrations) std_dev = np.std(concentrations) print(f'Mean: {mean_concentration}, Std Dev: {std_dev}')
Matplotlib and Seaborn are essential for data visualization, enabling mining professionals to create impactful visual representations of their data. Understanding trends and anomalies through graphs is vital for decision-making processes. These libraries allow for the creation of detailed plots that can illustrate production trends over time, geological formations, and much more.
import matplotlib.pyplot as plt import seaborn as sns # Visualize production trends over several years years = [2018, 2019, 2020, 2021, 2022] production = [200, 250, 300, 280, 320] plt.figure(figsize=(10, 6)) sns.lineplot(x=years, y=production, marker='o') plt.title('Mining Production Trends') plt.xlabel('Year') plt.ylabel('Production (tons)') plt.grid(True) plt.show()
Scikit-learn brings machine learning to the forefront of data analysis in mining. This library provides tools for regression, classification, and clustering, enabling the application of predictive models to optimize operations. For instance, predictive maintenance models can be built to foresee equipment failures and reduce downtime.
from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestRegressor # Sample feature set and target variable X = data[['feature1', 'feature2', 'feature3']] y = data['target'] # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2) # Train a random forest regressor model = RandomForestRegressor() model.fit(X_train, y_train) # Predict on the test set predictions = model.predict(X_test) print(predictions)
Applications of Data Analytics in Mining Operations
In mining operations, data analytics finds applications across multiple domains, enhancing efficiency, safety, and profitability. One significant area is operational optimization, where analytical techniques are employed to streamline processes and reduce waste. For example, by analyzing equipment performance data, mining companies can identify inefficiencies or faults in machinery, leading to timely maintenance and reduced downtime. This predictive maintenance approach can be implemented using Python’s advanced machine learning libraries.
Think the following example where we utilize the Scikit-learn library to build a predictive maintenance model that forecasts potential failures based on historical data:
from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier import pandas as pd # Load historical maintenance and failure data data = pd.read_csv('equipment_data.csv') # Define features and target variable X = data[['operating_hours', 'temperature', 'vibration']] y = data['failure'] # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Train a random forest classifier model = RandomForestClassifier() model.fit(X_train, y_train) # Predict on the test set predictions = model.predict(X_test) print(predictions)
This approach empowers companies to proactively address maintenance issues, thus minimizing operational interruptions and enhancing productivity. Furthermore, the model’s insights can inform resource allocation, ensuring that maintenance crews are deployed efficiently.
Another key application of data analytics in mining is in geological exploration and resource estimation. By analyzing geological data collected from various sources—such as drill logs, geological surveys, and geophysical data—Python can be employed to build sophisticated models that estimate the quantity and quality of minerals in a given area. This process often involves spatial data analysis, which can be effectively managed using libraries like GeoPandas and Pyproj.
Here’s an example of how we can visualize geological data using GeoPandas:
import geopandas as gpd import matplotlib.pyplot as plt # Load geological data geo_data = gpd.read_file('geological_map.geojson') # Plot the geological features fig, ax = plt.subplots(figsize=(10, 10)) geo_data.plot(ax=ax, color='lightblue', edgecolor='black') plt.title('Geological Map of Mining Area') plt.xlabel('Longitude') plt.ylabel('Latitude') plt.show()
This visualization can help geologists identify key areas for further exploration and optimize drilling strategies based on predictive models that evaluate potential mineral deposits.
Data analytics also plays an important role in enhancing safety protocols within mining operations. By analyzing incident reports and safety data, companies can identify trends and hotspots for accidents or near misses. Machine learning algorithms can be trained to predict potential safety hazards based on environmental data, equipment usage, and employee behavior. This can lead to the implementation of targeted safety measures that mitigate risks.
For instance, clustering algorithms can help categorize incidents based on various factors, allowing safety managers to focus their training and resources where they are needed most. Here’s how clustering can be implemented using the K-Means algorithm from Scikit-learn:
from sklearn.cluster import KMeans # Load safety incident data incident_data = pd.read_csv('safety_incidents.csv') # Select relevant features for clustering X = incident_data[['incident_severity', 'environmental_conditions', 'equipment_type']] # Apply K-Means clustering kmeans = KMeans(n_clusters=3, random_state=42) incident_data['cluster'] = kmeans.fit_predict(X) # Visualize the clusters plt.scatter(incident_data['incident_severity'], incident_data['environmental_conditions'], c=incident_data['cluster']) plt.title('Clustering of Safety Incidents') plt.xlabel('Incident Severity') plt.ylabel('Environmental Conditions') plt.show()
By employing these analytical techniques, mining operators can derive actionable insights that bolster safety measures, thereby protecting their workforce and reducing liability.
Furthermore, data analysis supports environmental monitoring and compliance, which have become vital in today’s mining industry. Regulatory bodies require stringent reporting on environmental impacts, and data analytics can facilitate accurate monitoring of parameters such as emissions, water quality, and habitat disruption. Python libraries like Matplotlib and seaborn can be instrumental in visualizing these data points, helping stakeholders understand the environmental footprint of mining operations.
Case Studies: Successful Implementation of Python in Mining
In the mining industry, numerous case studies highlight the successful implementation of Python for data analysis, showcasing how companies have leveraged its capabilities to drive efficiency, reduce costs, and enhance decision-making processes. One notable example involves a large mining corporation that faced challenges in equipment maintenance and operational downtime. By integrating Python’s data analysis libraries, the company developed a predictive maintenance system to monitor the health of its machinery.
The team utilized historical equipment performance data, which included variables such as operating hours, temperature, and vibration levels. They applied machine learning algorithms provided by Scikit-learn to build a model that could predict potential equipment failures before they occurred. The process involved several steps, beginning with data preparation and cleaning, followed by feature selection and model training. Here’s a concise implementation demonstrating the predictive maintenance approach:
from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier import pandas as pd # Load historical maintenance and failure data data = pd.read_csv('equipment_data.csv') # Define features and target variable X = data[['operating_hours', 'temperature', 'vibration']] y = data['failure'] # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Train a random forest classifier model = RandomForestClassifier() model.fit(X_train, y_train) # Predict on the test set predictions = model.predict(X_test) print(predictions)
As a result of this initiative, the mining corporation reported a significant reduction in unplanned maintenance events, leading to improved operational efficiency and cost savings. The predictive maintenance system not only minimized downtime but also allowed for better planning of maintenance activities, ensuring that resources were allocated effectively.
Another compelling case study involves a mining company that sought to enhance its resource estimation processes. By employing Python libraries like Pandas and GeoPandas, the organization was able to analyze geological data derived from various sources, including drill logs and geophysical surveys. This analysis facilitated the creation of detailed 3D models of mineral deposits, improving the accuracy of resource estimates and enabling more informed investment decisions.
The integration of geospatial analysis alongside data visualization techniques elevated the company’s exploration strategy. For instance, the use of GeoPandas to plot geological features helped the geologists visualize potential mineral-rich areas for further investigation:
import geopandas as gpd import matplotlib.pyplot as plt # Load geological data geo_data = gpd.read_file('geological_map.geojson') # Plot the geological features fig, ax = plt.subplots(figsize=(10, 10)) geo_data.plot(ax=ax, color='lightblue', edgecolor='black') plt.title('Geological Map of Mining Area') plt.xlabel('Longitude') plt.ylabel('Latitude') plt.show()
This visualization enabled the team to identify trends and anomalies that might not have been apparent through traditional analysis methods. The enhanced resource estimation capabilities ultimately allowed the company to optimize its drilling programs, resulting in more successful exploration outcomes.
In a further example, a mining operation focused on improving workplace safety through the analysis of incident reports. By using clustering algorithms, the safety team sought to identify patterns in accidents and near misses. Implementing K-Means clustering using Scikit-learn, they were able to categorize incidents based on severity and contributing factors:
from sklearn.cluster import KMeans # Load safety incident data incident_data = pd.read_csv('safety_incidents.csv') # Select relevant features for clustering X = incident_data[['incident_severity', 'environmental_conditions', 'equipment_type']] # Apply K-Means clustering kmeans = KMeans(n_clusters=3, random_state=42) incident_data['cluster'] = kmeans.fit_predict(X) # Visualize the clusters plt.scatter(incident_data['incident_severity'], incident_data['environmental_conditions'], c=incident_data['cluster']) plt.title('Clustering of Safety Incidents') plt.xlabel('Incident Severity') plt.ylabel('Environmental Conditions') plt.show()
This analytical approach allowed the safety managers to pinpoint the most hazardous conditions and allocate training resources where they were needed most. Consequently, the mining operation reported a decrease in incidents, fostering a safer work environment for employees.
Challenges and Limitations of Data Analysis in Mining
The landscape of data analysis in the mining industry is not without its challenges and limitations. Despite its potential, mining companies face several hurdles that can impede the successful adoption and implementation of data analytics. Understanding these challenges very important for stakeholders seeking to leverage data-driven insights for operational improvement.
One significant challenge is the quality and consistency of data collected from various sources. Mining operations generate vast amounts of data, often from disparate systems and sensors. Inconsistent formats, missing values, and inaccurate readings can complicate data analysis efforts. For example, if equipment sensors report different metrics due to calibration issues, the resulting analysis could lead to misguided decisions. This necessitates robust data cleaning and preprocessing steps, often requiring extensive effort to ensure reliability and validity.
Moreover, integrating data from multiple sources poses another obstacle. Mining companies typically utilize an array of technologies—geological surveys, equipment monitoring systems, and environmental assessments—that operate in silos. Combining this multifaceted data into a cohesive dataset for analysis can be technically challenging, requiring specialized skills and tools. Python, with libraries like Pandas and NumPy, can help manage this complexity, but the initial setup can be resource-intensive.
Furthermore, the mining industry often grapples with legacy systems that may not be compatible with modern data analytics platforms. These outdated systems can hinder the flow of information and make it difficult to implement advanced analytics. Transitioning from legacy systems to more agile, data-friendly platforms requires significant investment in both technology and training, which can deter companies from pursuing these upgrades.
Another limitation is related to the skill gap within the workforce. While Python has become a leading language for data analysis, the mining sector still faces a shortage of skilled data scientists and analysts who are familiar with both the domain and the technology. Bridging this gap requires ongoing training and education initiatives, as well as collaboration with academic institutions to develop curricula that meet industry needs.
Additionally, regulatory compliance presents a unique challenge for mining operations. As data analytics become integral to decision-making, companies must navigate a complex web of regulations concerning data privacy, environmental reporting, and safety standards. Ensuring that data analysis practices adhere to these regulations can be daunting, particularly in regions with stringent environmental laws. The need for transparency and accountability can also complicate data sharing and external collaborations, limiting opportunities for comprehensive analysis.
Finally, there is the challenge of adopting a data-driven culture within organizations. Many mining companies have historically relied on traditional methods and experience-based decision-making. Shifting to a data-driven approach requires not only investments in technology but also a fundamental change in mindset. Leadership must champion this transition, fostering an environment where data insights are valued and integrated into daily operations. This cultural shift can be one of the most significant barriers to effective data analytics implementation.
Future Trends: The Role of Python in Mining Industry Evolution
The evolution of the mining industry is increasingly intertwined with technological advancements, particularly in data analysis. As companies strive to improve efficiency, sustainability, and safety, Python is positioned at the forefront of this transformation. The future trends in mining operations, driven by data analytics, promise to reshape operational practices and strategic decision-making profoundly.
One notable trend is the integration of real-time data analytics into mining operations. With the proliferation of IoT devices and sensors, mining companies can collect vast amounts of data in real-time, facilitating immediate insights and responses. Python, with its powerful libraries such as Pandas and Dask, enables the processing and analysis of streaming data. This can allow for immediate adjustments in operations, whether in production optimization or environmental monitoring.
import pandas as pd import dask.dataframe as dd # Load real-time data real_time_data = dd.read_csv('real_time_mining_data.csv') # Perform calculations on large datasets results = real_time_data.groupby('sensor_type')['value'].mean().compute() print(results)
Additionally, machine learning and artificial intelligence (AI) will continue to permeate mining operations. By using predictive analytics, companies can anticipate equipment failures, optimize resource allocation, and refine exploration strategies. For instance, using Python’s Scikit-learn, mining companies can build sophisticated models that learn from historical data, allowing them to make proactive decisions that save time and reduce costs.
from sklearn.ensemble import GradientBoostingRegressor from sklearn.model_selection import train_test_split # Load historical data data = pd.read_csv('historical_mining_data.csv') # Define features and target variable X = data[['feature1', 'feature2', 'feature3']] y = data['target'] # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Train a gradient boosting regressor model = GradientBoostingRegressor() model.fit(X_train, y_train) # Make predictions predictions = model.predict(X_test) print(predictions)
The rise of data visualization tools is another trend that will significantly enhance decision-making within mining companies. The ability to visualize complex datasets allows stakeholders to grasp insights quickly and intuitively. Libraries such as Matplotlib and Plotly can create interactive dashboards that display critical metrics, helping teams monitor operations and performance in real-time.
import matplotlib.pyplot as plt import plotly.express as px # Sample data for visualization data = {'Month': ['January', 'February', 'March'], 'Production': [100, 150, 130]} df = pd.DataFrame(data) # Create a bar plot using Matplotlib plt.bar(df['Month'], df['Production']) plt.title('Monthly Production') plt.xlabel('Month') plt.ylabel('Production (tons)') plt.show() # Create an interactive line plot using Plotly fig = px.line(df, x='Month', y='Production', title='Monthly Production Over Time') fig.show()
Moreover, as sustainability becomes a critical focus in the mining industry, data analytics will play a pivotal role in environmental management. Python can facilitate the analysis of environmental impact data, ensuring compliance with regulations while identifying opportunities for reducing the carbon footprint. Advanced analytics can help predict environmental risks, allowing companies to implement preventive measures rather than reactive ones.
# Load environmental data env_data = pd.read_csv('environmental_impact_data.csv') # Analyze emissions over time emissions_by_year = env_data.groupby('year')['emissions'].sum() print(emissions_by_year)
Finally, collaboration and data sharing among mining companies, governments, and academia are likely to increase. By adopting open data practices and sharing insights, stakeholders can collectively address industry challenges, from safety to sustainability. Python’s versatility will facilitate this collaboration, as it can be used to build data-sharing platforms that accommodate various data formats and standards.