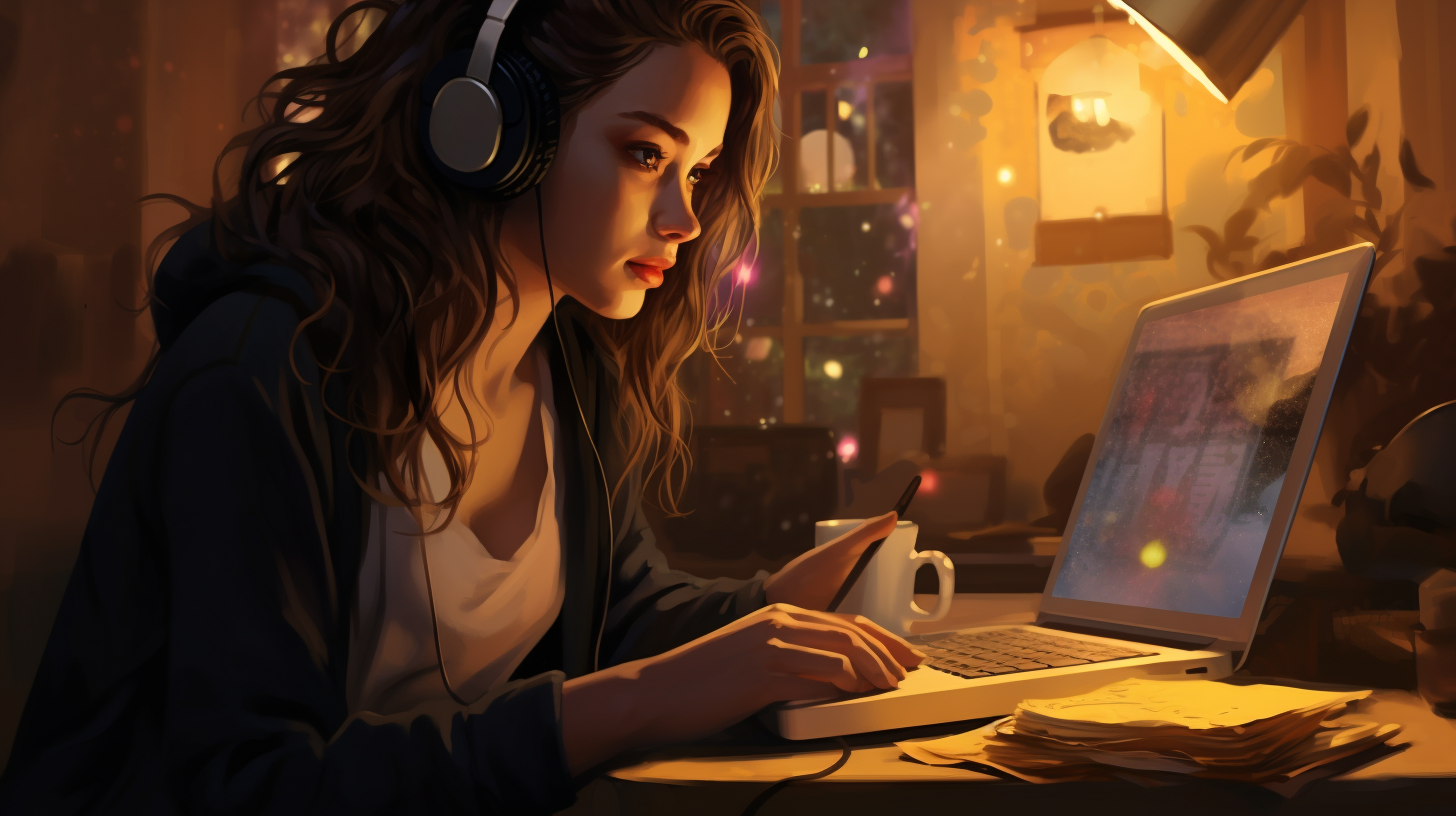
Python in Real Estate: Market Analysis
To delve into the real estate market, one must appreciate the intricate dynamics that drive property values and market fluctuations. Real estate is influenced by a multitude of factors, including economic conditions, demographics, interest rates, and local developments. Understanding these components is important for effective market analysis.
Market dynamics are often characterized by supply and demand. When demand outstrips supply, property prices tend to rise, creating a sellers’ market. Conversely, when the market is flooded with properties and demand wanes, buyers can benefit from lower prices, leading to a buyers’ market. This interplay can be modeled using various statistical techniques.
In addition to basic supply and demand principles, macroeconomic indicators such as GDP growth, employment rates, and inflation can heavily influence real estate trends. For instance, a robust job market may attract new residents to a region, increasing demand for housing.
Furthermore, microeconomic factors play a significant role, including local amenities like schools, parks, and public transport. These attributes enhance a property’s desirability and can lead to price premiums. Understanding these local dynamics requires solid data collection and analysis skills, which can be efficiently accomplished using Python.
To show how we can start analyzing these dynamics programmatically, consider the following Python code that outlines the essential steps in data analysis:
import pandas as pd import matplotlib.pyplot as plt # Load a hypothetical real estate dataset data = pd.read_csv('real_estate_data.csv') # Display the first few rows of the dataset print(data.head()) # Analyze the relationship between property prices and square footage plt.scatter(data['square_footage'], data['price']) plt.title('Price vs. Square Footage') plt.xlabel('Square Footage') plt.ylabel('Price') plt.show()
This snippet loads a dataset and visualizes the correlation between property prices and their size, a fundamental aspect of market dynamics. By using Python’s powerful data manipulation and visualization libraries, analysts can uncover insights that inform investment strategies and market predictions.
Moreover, understanding the cyclical nature of real estate markets is paramount. Markets go through phases of expansion, peak, contraction, and recovery, each presenting unique opportunities and risks. Thus, to make informed decisions, it’s essential to analyze historical data trends and project future movements.
Grasping the dynamics of the real estate market is foundational to effective analysis and decision-making. This understanding not only enhances one’s analytical skills but also opens pathways to using Python for more sophisticated data exploration and predictive modeling.
Data Collection and Preparation Techniques
Data collection is the first critical step in any analysis of the real estate market. Accurate and comprehensive data is essential for understanding market trends and making informed decisions. In this section, we’ll explore various techniques to gather and prepare data for analysis using Python.
Data can be sourced from a variety of platforms, including government databases, real estate websites, and proprietary data providers. Websites like Zillow, Redfin, and Realtor.com offer APIs that can be utilized to scrape real-time data regarding property listings, sales prices, and other relevant factors. Another possibility is using web scraping techniques to extract data when APIs are not available.
For instance, using the requests
library in Python, we can pull data from a real estate website:
import requests from bs4 import BeautifulSoup # Define the URL of the real estate website url = 'https://example-realestate-site.com/properties' # Perform a GET request to fetch the HTML content response = requests.get(url) # Parse the HTML content using BeautifulSoup soup = BeautifulSoup(response.content, 'html.parser') # Find property listings in the HTML listings = soup.find_all('div', class_='property-listing') # Extract relevant details properties = [] for listing in listings: price = listing.find('span', class_='property-price').text location = listing.find('span', class_='property-location').text properties.append({'price': price, 'location': location}) # Display the extracted properties print(properties)
Once the data is collected, it’s often in a raw format that requires cleaning and preparation. This step may involve removing duplicates, handling missing values, and converting data types to ensure that our analysis is accurate. The pandas
library is invaluable in this aspect, providing powerful tools to manipulate and prepare datasets efficiently.
Here’s an example of how to clean a real estate dataset:
import pandas as pd # Load the dataset data = pd.read_csv('real_estate_data.csv') # Display basic information about the data print(data.info()) # Remove duplicates data = data.drop_duplicates() # Handle missing values by filling with the median data['price'].fillna(data['price'].median(), inplace=True) data['location'].fillna('Unknown', inplace=True) # Convert data types if necessary data['price'] = data['price'].astype(float) # Display cleaned data print(data.head())
Data preparation might also include feature engineering, where new variables are created from existing ones to enhance the analytical model. For example, creating a variable for price per square foot can provide a more nuanced view of property values relative to their size.
# Create a new feature for price per square foot data['price_per_sqft'] = data['price'] / data['square_footage'] # Display updated dataset print(data[['price', 'square_footage', 'price_per_sqft']].head())
In essence, proper data collection and preparation set the foundation for any successful analysis in the real estate market. By using the capabilities of Python, analysts can efficiently gather, clean, and refine their datasets, ensuring a robust basis for further exploration and modeling. This meticulous groundwork ultimately enhances the reliability of the insights derived from subsequent analyses, allowing for more informed decision-making in the dynamic world of real estate.
Analyzing Market Trends with Python Libraries
With a clean dataset in hand, we can turn our attention to analyzing market trends using Python libraries that are powerful in handling statistical analysis and data visualization. Two of the most widely used libraries in this domain are pandas for data manipulation and matplotlib and seaborn for visualization. These tools allow us to explore patterns and relationships within the data, leading to insights that can inform investment strategies.
One critical aspect of analyzing market trends is the ability to identify how various factors correlate with property prices. For instance, we can examine correlations between prices and features such as location, square footage, and the number of bedrooms. Using the pandas library, we can compute the correlation matrix and visualize it using a heatmap, which provides a clear representation of these relationships.
import pandas as pd import seaborn as sns import matplotlib.pyplot as plt # Load the cleaned dataset data = pd.read_csv('cleaned_real_estate_data.csv') # Compute the correlation matrix correlation_matrix = data.corr() # Set up the matplotlib figure plt.figure(figsize=(10, 8)) # Create a heatmap of the correlation matrix sns.heatmap(correlation_matrix, annot=True, fmt='.2f', cmap='coolwarm', square=True, cbar_kws={"shrink": .8}) plt.title('Correlation Matrix of Real Estate Features') plt.show()
This heatmap offers a visual insight into how strongly different variables are related to one another. For instance, a high positive correlation between square footage and price would indicate that larger properties typically sell for higher prices. Conversely, a negative correlation might suggest that as one variable increases, the other decreases.
Another key component of market trend analysis is the ability to track price changes over time. Time series analysis is particularly useful in this regard, allowing analysts to identify seasonal trends and long-term movements in property values. The statsmodels library can be employed to perform time series analysis, enabling us to apply seasonal decomposition techniques.
import statsmodels.api as sm # Assume 'date' is a column in the dataset containing date information data['date'] = pd.to_datetime(data['date']) data.set_index('date', inplace=True) # Resample the data to a monthly frequency and calculate the mean price monthly_prices = data['price'].resample('M').mean() # Decompose the time series decomposition = sm.tsa.seasonal_decompose(monthly_prices, model='additive') decomposition.plot() plt.show()
By decomposing the time series, we can extract the underlying trend, seasonal components, and residuals, offering a clear view of how property prices evolve throughout the year. Understanding these temporal patterns enables investors to better time their purchases or sales according to expected market conditions.
Finally, we can enhance our analysis by employing machine learning techniques to uncover more complex patterns in the data. Libraries such as scikit-learn provide a range of algorithms for regression analysis, which can predict property prices based on various features. For example, we can use linear regression to model the relationship between property features and their prices.
from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression # Define the features and target variable X = data[['square_footage', 'bedrooms', 'bathrooms']] y = data['price'] # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create a linear regression model and fit it to the training data model = LinearRegression() model.fit(X_train, y_train) # Evaluate the model's performance score = model.score(X_test, y_test) print(f'Model R^2 Score: {score:.2f}')
This code outlines the process of training a linear regression model on the dataset and evaluating its performance using the R² score, which indicates how well the model explains the variability of the target variable. The insights garnered from such analyses can provide crucial guidance to investors and real estate professionals, allowing them to navigate the complexities of market dynamics with greater confidence.
Predictive Modeling for Property Valuation
When venturing into the realm of property valuation using Python, one approaches a nuanced and intricate landscape where predictive modeling becomes essential. Predictive modeling is the cornerstone of forecasting property values based on historical data and identified trends. In real estate, understanding the factors influencing property prices and how they interrelate allows us to build reliable models that can guide investment decisions and business strategies.
One of the most commonly used techniques in predictive modeling for property valuation is linear regression. Linear regression enables us to establish relationships between multiple independent variables (features) and a dependent variable (the target), which in this case is the property price. To illustrate this, let’s delve into a simple example using Python’s scikit-learn library.
import pandas as pd from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression from sklearn.metrics import mean_squared_error # Load the cleaned dataset data = pd.read_csv('cleaned_real_estate_data.csv') # Define features and target variable X = data[['square_footage', 'bedrooms', 'bathrooms', 'location_score']] # Including a feature for location y = data['price'] # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create a linear regression model model = LinearRegression() model.fit(X_train, y_train) # Make predictions on the test set y_pred = model.predict(X_test) # Evaluate the model's performance mse = mean_squared_error(y_test, y_pred) print(f'Mean Squared Error: {mse:.2f}')
In this snippet, we first define our features, which include square footage, number of bedrooms, bathrooms, and an additional hypothetical feature, location_score, which could represent the desirability of a property’s location on a numerical scale. After splitting the data into training and testing sets, we create and fit our linear regression model. Finally, we evaluate the model’s performance using the Mean Squared Error (MSE), a common metric for regression tasks that indicates how close the predictions are to the actual values.
Beyond linear regression, various advanced techniques can enhance our predictive modeling capabilities. For example, decision trees, random forests, and gradient boosting machines can capture non-linear relationships within the data, which may be overlooked by simpler models. Here’s how we can implement a random forest regressor:
from sklearn.ensemble import RandomForestRegressor # Instantiate the model rf_model = RandomForestRegressor(n_estimators=100, random_state=42) # Fit the model on the training data rf_model.fit(X_train, y_train) # Make predictions y_rf_pred = rf_model.predict(X_test) # Evaluate the model's performance rf_mse = mean_squared_error(y_test, y_rf_pred) print(f'Random Forest Mean Squared Error: {rf_mse:.2f}')
The random forest regressor aggregates the predictions from multiple decision trees, significantly improving prediction accuracy and robustness against overfitting. Such ensemble methods often outperform linear models, especially in complex datasets with many features. Additionally, they provide insights into feature importance, enabling analysts to understand which variables are most impactful in determining property values.
Moreover, to further refine our predictive capabilities, we can employ techniques like cross-validation. Cross-validation ensures that our model is not only accurate on the training data but also generalizes well to unseen data. This approach is especially crucial in real estate, where the market can exhibit seasonal patterns and sudden shifts due to external factors.
from sklearn.model_selection import cross_val_score # Apply cross-validation scores = cross_val_score(rf_model, X, y, cv=5, scoring='neg_mean_squared_error') mean_mse = -scores.mean() print(f'Cross-Validated Mean Squared Error: {mean_mse:.2f}')
By implementing cross-validation, we obtain a more reliable estimate of the model’s performance across different subsets of our data. This practice minimizes the risk of overfitting, ensuring that our predictive model remains robust and practical in real-world applications.
As we build and refine our predictive models, it’s critical to stay attuned to the external factors that may influence property values. Economic indicators, interest rates, and local market conditions must be continually monitored and incorporated into our models, thereby ensuring that our predictions reflect the latest trends and shifts in the real estate landscape.
Predictive modeling for property valuation using Python is a multifaceted approach that combines statistical techniques, machine learning algorithms, and a keen understanding of market dynamics. By using these tools, real estate professionals can make informed decisions backed by data-driven insights, ultimately leading to more successful investment strategies.
Visualization Tools for Real Estate Data Insights
When it comes to conveying insights derived from real estate data, visualization tools play a pivotal role. The ability to present complex data in a clear and impactful manner can significantly enhance understanding and facilitate informed decision-making. Python, with its rich ecosystem of libraries tailored for data visualization, offers a compelling means to bring real estate market dynamics to life.
The most widely used libraries for visualization in Python are Matplotlib and Seaborn, both of which provide the functionality to create a diverse array of plots and charts. These visual representations allow analysts to discern patterns, trends, and outliers within the data that might otherwise go unnoticed in raw numerical formats.
Let’s think a scenario where we want to visualize the distribution of property prices in a given area. A histogram is an effective way to represent this information, enabling us to observe how prices are spread across different ranges. Below is an example of how to create a histogram using Matplotlib:
import pandas as pd import matplotlib.pyplot as plt # Load the cleaned dataset data = pd.read_csv('cleaned_real_estate_data.csv') # Create a histogram of property prices plt.figure(figsize=(10, 6)) plt.hist(data['price'], bins=30, color='blue', alpha=0.7) plt.title('Distribution of Property Prices') plt.xlabel('Price') plt.ylabel('Frequency') plt.grid(axis='y', alpha=0.75) plt.show()
This histogram not only shows the frequency of property prices but also helps identify the most common price ranges. Such insights are crucial for buyers and sellers alike, helping them understand the prevailing market conditions.
Furthermore, scatter plots can be instrumental in exploring the relationship between two numerical variables, such as square footage and property prices. This visual representation can highlight trends and potential correlations. Here’s how you can create a scatter plot:
plt.figure(figsize=(10, 6)) plt.scatter(data['square_footage'], data['price'], alpha=0.5) plt.title('Price vs. Square Footage') plt.xlabel('Square Footage') plt.ylabel('Price') plt.grid(True) plt.show()
In this scatter plot, each point represents a property, allowing us to see how property size correlates with its market price. Analysts can quickly assess whether larger properties tend to command higher prices and identify any clustering or outlier properties that may merit further investigation.
When it comes to analyzing temporal data, such as tracking property prices over time, line charts are an excellent choice. They allow users to visualize trends and changes more intuitively. Below is an example of how to create a line chart to illustrate price trends:
# Assume 'date' is a column in the dataset containing date information data['date'] = pd.to_datetime(data['date']) data.set_index('date', inplace=True) # Resample the data to a monthly frequency and calculate the mean price monthly_prices = data['price'].resample('M').mean() # Create a line chart plt.figure(figsize=(12, 6)) plt.plot(monthly_prices, color='orange') plt.title('Average Monthly Property Prices Over Time') plt.xlabel('Date') plt.ylabel('Average Price') plt.xticks(rotation=45) plt.grid(True) plt.show()
This line chart enables us to track how average property prices have evolved over time, which is invaluable for detecting seasonal trends or identifying periods of price volatility.
Beyond these basic visualizations, more sophisticated tools like Plotly or Bokeh allow for interactive visualization, providing users the ability to zoom in on specific data points or filter information dynamically. Such interactivity can enhance presentations for stakeholders or clients who are engaged in real estate transactions, making it easier to convey complex analytical findings.
The power of visualization tools in Python cannot be overstated. They transform raw data into understandable and actionable insights, which are vital for anyone involved in the real estate industry. By employing these techniques, analysts and real estate professionals are better equipped to interpret market conditions, predict trends, and make data-driven decisions that lead to successful outcomes.
Case Studies: Successful Applications of Python in Real Estate Analysis
As we explore case studies highlighting the successful applications of Python in real estate analysis, it becomes evident that real-world implementations can provide a wealth of knowledge and insights. These examples serve to illustrate not only the versatility of Python in handling complex datasets but also the tangible benefits it can bring to stakeholders in the real estate market.
One prominent case study involves a real estate investment firm that sought to optimize its property acquisition strategy by using data analytics. The firm faced challenges in identifying undervalued properties and predicting future price increases based on various factors. By using Python’s data analysis capabilities, the firm implemented a machine learning model that integrated historical sales data, property features, and economic indicators to forecast property values accurately.
Using scikit-learn, the firm deployed a random forest regression model that allowed it to evaluate multiple properties at the same time. This model not only enhanced accuracy in price predictions but also provided insights into the importance of different features affecting property values. Below is a simplified example of how the firm structured its analysis:
import pandas as pd from sklearn.ensemble import RandomForestRegressor from sklearn.model_selection import train_test_split from sklearn.metrics import mean_squared_error # Load the dataset containing property and economic features data = pd.read_csv('investment_properties.csv') # Define features and target variable X = data[['square_footage', 'bedrooms', 'bathrooms', 'location_score', 'economic_indicator']] y = data['price'] # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create a random forest regression model and fit it to the training data rf_model = RandomForestRegressor(n_estimators=100, random_state=42) rf_model.fit(X_train, y_train) # Make predictions on the test set y_pred = rf_model.predict(X_test) # Evaluate the model's performance mse = mean_squared_error(y_test, y_pred) print(f'Mean Squared Error: {mse:.2f}')
This strategic approach allowed the firm to allocate its resources more effectively, leading to a substantial increase in overall portfolio returns. The ability to predict price movements with high accuracy not only streamlined its acquisition process but also minimized the risks associated with property investments.
Another notable application of Python in real estate analysis came from a city planning department that aimed to assess the impact of new housing developments on local markets. By combining spatial analysis with demographic data, the department utilized Python’s geospatial libraries, such as Geopandas, to visualize the relationships between new constructions and changes in property values.
Through the following code, the department was able to create visualizations that illustrated these relationships and provided data-driven recommendations for future developments:
import geopandas as gpd import matplotlib.pyplot as plt # Load the geospatial dataset containing property values and new developments geo_data = gpd.read_file('property_values_and_developments.geojson') # Create a plot to visualize the impact of new developments on property values fig, ax = plt.subplots(figsize=(12, 8)) geo_data.plot(column='property_value', ax=ax, legend=True, legend_kwds={'label': "Property Values", 'orientation': "horizontal"}) plt.title('Impact of New Developments on Property Values') plt.xlabel('Longitude') plt.ylabel('Latitude') plt.show()
By visualizing property values in conjunction with new housing developments, the city planning department could make informed decisions about future zoning laws and development approvals, ensuring a balanced approach to urban growth.
In another instance, a real estate analytics startup utilized Python to automate the data scraping process from various real estate listing websites. This allowed the startup to compile an extensive database of property listings, including features, pricing, and geographic information. By employing web scraping techniques with BeautifulSoup and Requests, the startup efficiently gathered valuable datasets that could then be analyzed to generate market insights.
import requests from bs4 import BeautifulSoup # Define the URL of the real estate website url = 'https://example-realestate-site.com/properties' # Perform a GET request to fetch the HTML content response = requests.get(url) # Parse the HTML content using BeautifulSoup soup = BeautifulSoup(response.content, 'html.parser') # Find property listings in the HTML listings = soup.find_all('div', class_='property-listing') # Extract relevant details properties = [] for listing in listings: price = listing.find('span', class_='property-price').text location = listing.find('span', class_='property-location').text properties.append({'price': price, 'location': location}) # Display the extracted properties print(properties)
This automated data collection process drastically reduced the time and effort required to gather information, enabling the startup to focus on analytics and insight generation, ultimately leading to a competitive edge in the marketplace.
Through these case studies, the effectiveness of Python in real estate analysis is not only demonstrated but highlighted as an invaluable tool for decision-making, strategic planning, and risk management. As Python continues to evolve and its community grows, the possibilities for its application in real estate will undoubtedly expand, paving the way for more innovative solutions in the industry.