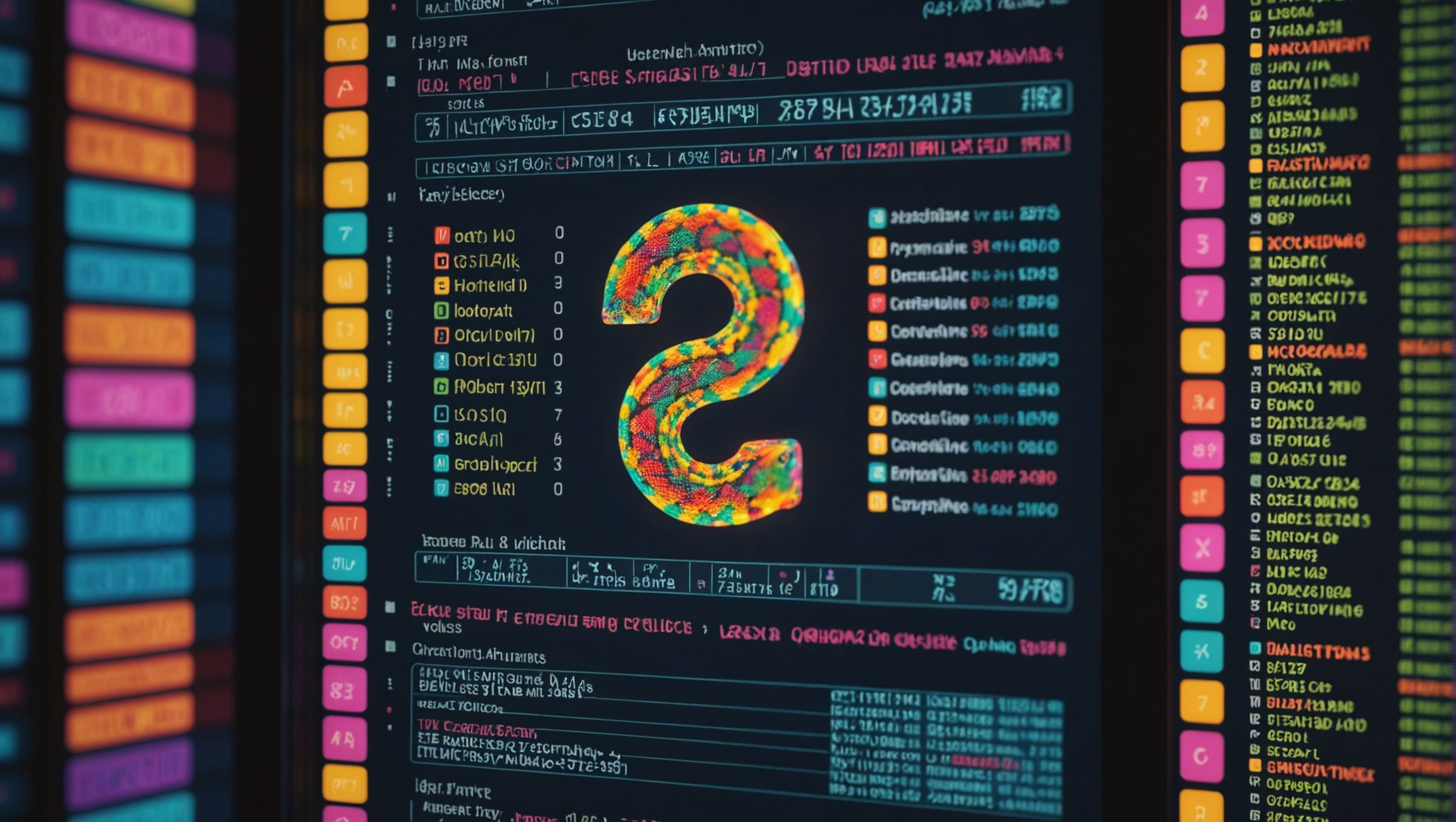
Python List Comprehensions: Simplifying Loops
List comprehensions in Python are a powerful tool that allows you to create new lists by applying an expression to each item in an existing iterable. This elegant approach not only simplifies your code but also makes it more readable and concise. If you’ve ever found yourself writing loops to construct lists, you’ll appreciate the elegance of list comprehensions.
At its core, a list comprehension consists of a single expression followed by a for
clause and optionally, one or more if
clauses. This structure allows you to iterate over an iterable, apply a transformation, and filter elements—all in a single line of code. The beauty of this syntax lies in its clarity and expressiveness, which can often replace several lines of traditional loop code.
To better understand how list comprehensions work, consider the following example: suppose you want to create a list of squares for the numbers from 0 to 9. Using a traditional loop, you might write:
squares = [] for x in range(10): squares.append(x ** 2)
In contrast, you can achieve the same result with a list comprehension:
squares = [x ** 2 for x in range(10)]
This example illustrates how a simple mathematical operation can be expressed in a more compact form, making your code cleaner and easier to understand. The list comprehension not only accomplishes the same goal but does so with an elegance that invites the reader to grasp the intent quickly.
Furthermore, list comprehensions can include conditions to filter items. For instance, if you want only the squares of even numbers, you can add an if
clause:
even_squares = [x ** 2 for x in range(10) if x % 2 == 0]
This variation shows the versatility of list comprehensions, so that you can both transform and filter data with ease. List comprehensions not only enhance the expressiveness of your code but also reduce the likelihood of errors that can occur with more verbose implementations.
Understanding list comprehensions is essential for contemporary Python programming. They provide a succinct way to generate lists, combining both readability and efficiency. As you explore Python further, you’ll find that mastering list comprehensions can significantly improve your coding style and productivity.
Syntax and Structure of List Comprehensions
To break down the syntax of list comprehensions further, we can look at the general structure, which can be summarized as follows:
[expression for item in iterable if condition]
Here, each component has its specific role:
- This is the value or calculation that will be included in the new list. It can be as simple as a variable or a more complex expression involving multiple variables.
- Represents the current value from the iterable during each iteration.
- An iterable object, such as a list, tuple, or string, from which items are drawn.
- A filter that determines whether the current item should be included in the new list. If the condition evaluates to True, the expression is evaluated and added to the new list; otherwise, it’s skipped.
This structure allows for flexibility and power within a compact syntax, letting you express complex logic succinctly. Consider the following examples to illustrate further nuances.
Suppose you want to create a list of the lengths of words in a sentence:
sentence = "List comprehensions are powerful" word_lengths = [len(word) for word in sentence.split()]
In this case, the len(word)
serves as the expression, word
is the item iterated over from the result of sentence.split()
, which is the iterable. Here, every word in the sentence is processed, and its length is added to the new list.
Moreover, you can implement more intricate conditions. For example, if you want to gather only the lengths of words longer than three characters:
long_word_lengths = [len(word) for word in sentence.split() if len(word) > 3]
Here, the condition if len(word) > 3
ensures that only words exceeding three characters contribute their lengths to the final list.
Understanding these components allows you to harness the full power of list comprehensions. They can be nested as well, enabling multi-level transformations. For instance, if you wanted to flatten a list of lists while also squaring the numbers, you could do it like this:
nested_list = [[1, 2], [3, 4], [5, 6]] flattened_squares = [x ** 2 for sublist in nested_list for x in sublist]
This example shows two for
clauses, making it clear that you’re iterating through each sublist and each number within those sublists. The nested structure showcases one of the powerful features of list comprehensions: the ability to perform complex operations in a single, readable expression.
The syntax and structure of list comprehensions in Python not only streamline the coding process but also elevate the readability of your code. By encapsulating logic into a concise format, they invite you to think differently about how you construct your lists, ultimately leading to cleaner, more maintainable programs.
Common Use Cases for List Comprehensions
When it comes to practical applications, list comprehensions excel in a variety of scenarios, allowing programmers to streamline their code while maintaining readability. Here are some common use cases that demonstrate the power and versatility of list comprehensions in Python.
One of the most simpler applications of list comprehensions is transforming data. For example, if you have a list of temperatures in Celsius and you want to convert them to Fahrenheit, a traditional loop would be verbose:
celsius = [0, 10, 20, 34.5] fahrenheit = [] for temp in celsius: fahrenheit.append((temp * 9/5) + 32)
Using a list comprehension, this transformation becomes concise:
fahrenheit = [(temp * 9/5) + 32 for temp in celsius]
This example not only reduces the lines of code but also clarifies the intent of the operation—transforming each item in the `celsius` list into its Fahrenheit equivalent.
Another common use case is filtering data. Suppose you need to extract a list of even numbers from a given range. Instead of using a loop to check each number, you can efficiently accomplish this with a list comprehension:
even_numbers = [num for num in range(20) if num % 2 == 0]
Here, the list comprehension iterates through numbers and applies the `if` condition to filter out odd numbers, resulting in a clean list of even integers.
List comprehensions also shine when dealing with strings. Imagine you want to extract the first letter of each word in a sentence. A typical loop might take several lines:
sentence = "List comprehensions are powerful" first_letters = [] for word in sentence.split(): first_letters.append(word[0])
Conversely, using a list comprehension simplifies this to a single line:
first_letters = [word[0] for word in sentence.split()]
This not only makes the code shorter but also highlights the logic clearly, demonstrating how to extract characters from a collection.
Furthermore, list comprehensions can be nested, allowing for more complex operations. For instance, if you need to create a list of all pairs (i, j) where i is from a list of numbers and j is from a list of letters, you can do this succinctly:
numbers = [1, 2, 3] letters = ['a', 'b', 'c'] pairs = [(i, j) for i in numbers for j in letters]
This example not only demonstrates the elegance of combining two lists but also emphasizes how list comprehensions can handle multiple iterables in a clean manner.
Whether you’re transforming, filtering, or nesting data, list comprehensions in Python provide a robust and efficient way to concisely express your intentions. By using this feature, you can write cleaner, more maintainable code that still conveys your logic clearly to anyone reading it.
Performance Considerations and Best Practices
When using list comprehensions, it’s essential to think their performance and adhere to best practices to maximize efficiency and maintainability. First, we must acknowledge that while list comprehensions are often faster than traditional loops, their performance can vary based on the complexity of the expression and the size of the input data.
In general, list comprehensions can be more efficient because they minimize the overhead associated with function calls and multiple append operations found in traditional for loops. For instance, creating a list with a list comprehension is often faster than appending elements to a list one at a time:
import time # Traditional loop start_time = time.time() squares = [] for x in range(10000): squares.append(x ** 2) print("Traditional loop:", time.time() - start_time) # List comprehension start_time = time.time() squares = [x ** 2 for x in range(10000)] print("List comprehension:", time.time() - start_time)
However, this speed advantage can diminish when the list comprehension becomes excessively complex. For example, if you have multiple nested loops or complicated conditions, the overhead of evaluating those conditions may outweigh the performance benefits. In such cases, it can be more readable and efficient to use traditional loops or helper functions to keep your code manageable.
Another performance consideration relates to memory usage. List comprehensions generate the entire list in memory concurrently. For very large datasets, this can lead to increased memory consumption, potentially causing your program to slow down or even crash. If you are working with large data, ponder using generator expressions instead. They yield items one at a time, allowing you to process elements without storing the entire list in memory:
# Generator expression squares_gen = (x ** 2 for x in range(10000)) for square in squares_gen: # Process each square without storing all in memory print(square)
When employing list comprehensions, clarity should be your guiding principle. If a comprehension becomes too convoluted, it can obscure the intent of your code, rendering it less readable. Strive for simplicity; if your comprehension requires extensive logic or multiple conditions, think breaking it into smaller parts or using helper functions. This not only aids readability but also makes debugging and maintenance easier.
For example, think this complex comprehension:
complex_list = [str(x) + " is even" if x % 2 == 0 else str(x) + " is odd" for x in range(100)]
While this line may be compact, it becomes challenging to parse quickly. A clearer approach is to split the logic into a function:
def describe_number(x): return f"{x} is even" if x % 2 == 0 else f"{x} is odd" descriptions = [describe_number(x) for x in range(100)]
This not only enhances clarity but also provides a single point to update the logic if needed.
Lastly, when aiming to optimize performance, profiling your code can be a valuable exercise. Using modules such as `cProfile` allows you to identify bottlenecks in your code, allowing you to make informed decisions on where to simplify or improve performance.
While list comprehensions are an invaluable feature of Python, their successful use hinges on understanding their performance implications and adhering to best practices. Striving for clarity and maintainability, while balancing performance, will lead to cleaner, more efficient code that is easier to read and maintain.