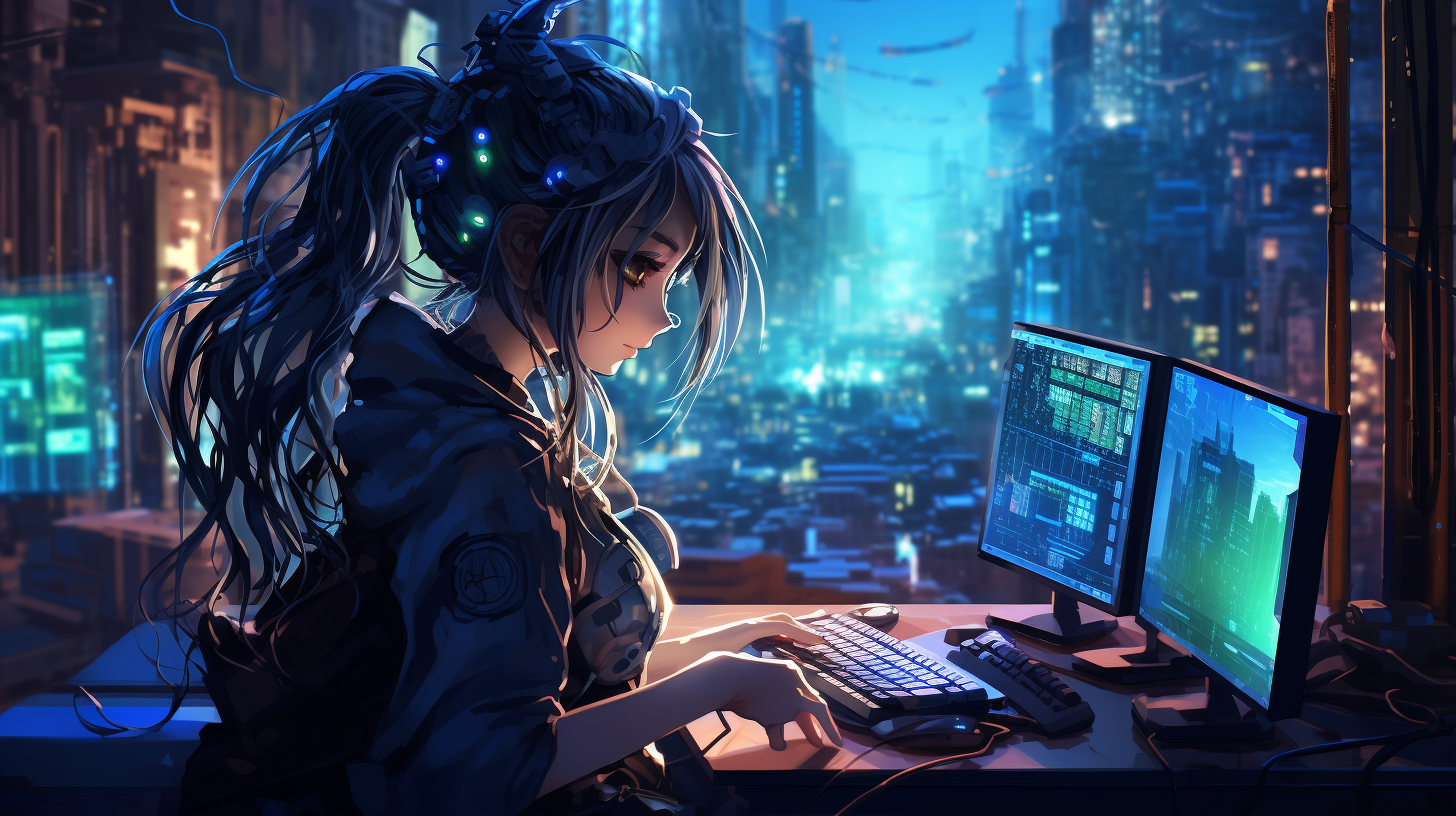
Python Memory Management and Optimization
Python’s memory model is a vital aspect of its design, influencing how objects are created, stored, and managed. At its core, Python uses a dynamic memory allocation strategy, meaning that memory is allocated as needed at runtime. This allows developers to create objects without worrying about the underlying memory management details, but it does come with its own set of complexities.
In Python, every object and data structure is represented as an object in memory. This includes everything from integers to lists to user-defined classes. When an object is created, Python allocates memory for it from a private heap, which is not directly accessible to the programmer. The size and layout of this memory can vary depending on the implementation of Python you’re using, but the core principles remain the same.
One of the key aspects of Python’s memory model is the idea of reference counting. Each object maintains a count of the number of references pointing to it. When a new reference to an object is created, the reference count increases; conversely, when a reference is deleted or goes out of scope, the count decreases. When the reference count drops to zero, it means that no references to the object exist, and the memory can be reclaimed.
class MyClass: def __init__(self, value): self.value = value obj1 = MyClass(10) obj2 = obj1 # Reference count for obj1 increases del obj1 # Reference count for obj1 decreases del obj2 # Reference count for obj1 becomes 0; memory can be reclaimed
However, reference counting alone cannot address all memory management issues. Circular references—where two or more objects reference each other—can lead to memory leaks since their reference counts never reach zero. To combat this, Python employs a garbage collection mechanism that periodically scans for and collects objects that are no longer accessible.
Another important concept within Python’s memory model is the distinction between mutable and immutable objects. Mutable objects, such as lists and dictionaries, can be changed after their creation, while immutable objects, like tuples and strings, cannot. This distinction affects how Python handles memory allocation and deallocation, ultimately influencing performance.
Understanding Python’s memory model especially important for writing efficient and effective code. By being aware of how memory is allocated and managed, developers can make informed decisions about object creation, reference management, and performance optimization.
Garbage Collection Mechanism
The garbage collection mechanism in Python serves as a powerful complement to the reference counting system. While reference counting efficiently manages most memory by immediately reclaiming memory once an object’s reference count drops to zero, it struggles with circular references. Circular references occur when two or more objects reference each other, preventing their reference counts from ever reaching zero. That’s where garbage collection steps in.
Python employs a cyclic garbage collector, which is part of the `gc` module. This collector identifies and removes groups of interconnected objects that are no longer accessible from the root of the program. The primary goal is to free memory this is no longer needed, thus preventing memory leaks that could degrade performance over time.
The garbage collector operates in a two-phase approach: marking and sweeping. During the marking phase, the collector identifies all objects that are directly or indirectly reachable from the root objects, such as global variables and active function calls. Any object not marked in this phase is considered unreachable and is eligible for collection during the sweeping phase, where the memory occupied by these objects is reclaimed.
Developers can interact with the garbage collector using the `gc` module. For example, you can manually trigger collection or adjust its behavior to suit specific application needs. Below is an example of how to interact with the garbage collector:
import gc # Enable automatic garbage collection gc.enable() # Check the number of unreachable objects unreachable_objects = gc.collect() print(f"Unreachable objects collected: {unreachable_objects}") # Manually disable garbage collection gc.disable() # Perform some operations... # Re-enable garbage collection gc.enable()
It is also possible to use the `gc` module to inspect objects that are currently tracked by the garbage collector, which can be helpful for debugging memory leaks:
# List all objects tracked by the garbage collector tracked_objects = gc.get_objects() print(f"Number of tracked objects: {len(tracked_objects)}")
Understanding and using the garbage collection mechanism effectively allows developers to write more memory-efficient Python programs. While Python’s automatic memory management simplifies the coding process, awareness of how garbage collection works enables programmers to optimize their applications further and avoid common pitfalls associated with memory leaks.
Memory Allocation Strategies
Memory allocation strategies in Python are essential for optimizing performance and ensuring efficient use of resources. Python utilizes a variety of allocation techniques, with the most notable being the private heap space and the use of specialized memory pools for different data types. The implementation of these strategies varies across Python’s implementations, such as CPython, PyPy, and others, but the fundamental principles remain similar.
When an object is created in Python, it’s allocated from a private heap that the memory manager oversees. The memory manager’s role is to handle memory allocation and deallocation to ensure that Python’s memory usage is efficient. Python’s memory manager employs different strategies based on the size and type of the object being created. For small objects, Python uses a technique called “pymalloc,” which is optimized for speed and efficiency.
pymalloc is a specialized allocator implemented in CPython that manages memory for small objects, typically less than 512 bytes. This allocator divides memory into blocks, each capable of holding a fixed number of objects, reducing fragmentation and speeding up allocation and deallocation. Here’s a brief view of how pymalloc works:
class SmallObject: pass # Creating multiple small objects small_objects = [SmallObject() for _ in range(100)]
For larger objects, Python uses the system’s built-in memory allocation functions, such as malloc
and free
. This allows for efficient allocation of larger chunks of memory when needed. It’s worth noting that memory allocation for large objects may cause fragmentation, which can lead to inefficient memory use over time.
Python also employs a technique known as “arena allocation” for managing memory in a more efficient way. This involves grouping similar-sized objects together in a single memory block or “arena.” By doing so, Python minimizes fragmentation and maximizes memory utilization, particularly for intermediate objects created during computation.
Another key aspect of Python’s memory allocation strategy is how it handles mutable versus immutable objects. Immutable objects, such as strings and tuples, can be shared among different parts of a program, reducing the need for duplicate allocations. This sharing means that when multiple references to an immutable object exist, they point to the same memory address:
immutable_string = "hello" another_reference = immutable_string # Both refer to the same memory location
On the other hand, mutable objects, like lists and dictionaries, require individual copies, especially when changes are made:
mutable_list = [1, 2, 3] another_list = mutable_list.copy() # Creates a new copy of the list mutable_list.append(4) # Modifying the original list does not affect the copy
Understanding these allocation strategies can significantly impact performance. Developers can optimize memory usage by being mindful of the types of objects being created and how they’re managed. For instance, using immutable objects where possible can lead to reduced memory overhead and improved performance due to Python’s ability to share these instances.
Additionally, Python’s memory allocation strategies are complemented by tools that allow developers to monitor and analyze memory usage. Using modules like sys
and tracemalloc
, developers can track memory allocations and identify areas for optimization:
import tracemalloc # Start tracking memory allocations tracemalloc.start() # Some operations data = [i for i in range(1000)] # Get memory statistics snapshot = tracemalloc.take_snapshot() top_stats = snapshot.statistics('lineno') print("[ Top 10 ]") for stat in top_stats[:10]: print(stat)
By using Python’s memory allocation strategies effectively, developers can create applications that are not only efficient in terms of performance but also maintainable and scalable over time. Understanding the intricacies of memory allocation and using the tools available makes it possible to write Python code that truly harnesses the power of the language.
Optimizing Memory Usage in Python
Optimizing memory usage in Python requires a multifaceted approach, focusing on how objects are created, manipulated, and discarded. By being cognizant of Python’s memory model and using efficient coding practices, developers can greatly reduce memory overhead and enhance the performance of their applications.
One of the primary strategies for optimizing memory usage is to minimize the creation of unnecessary objects. For instance, using generators instead of lists can help save memory by yielding items one at a time rather than holding an entire list in memory. Here’s an example of using a generator for a simple calculation:
def generate_numbers(n): for i in range(n): yield i * 2 # Using the generator for number in generate_numbers(1000000): if number > 10: break
In this example, generate_numbers
yields numbers on the fly, allowing the code to handle large ranges without consuming memory for the entire list.
Another optimization technique is to prefer built-in data types over custom classes wherever possible. Built-in types are implemented in C and are often more memory-efficient than their class counterparts. For instance, using tuples instead of lists can lead to less memory usage since tuples are immutable and Python can optimize their storage:
# Using a tuple coordinates = (10.0, 20.0)
When it comes to mutable data types, careful management of list sizes and avoiding excessive growth can also aid in memory optimization. For example, if you know the maximum size a list will reach, pre-allocating space using the list
constructor can help:
# Pre-allocating list size max_size = 1000 data_list = [None] * max_size for i in range(max_size): data_list[i] = i * 2
This approach reduces the overhead associated with dynamic resizing as items are appended to the list.
Additionally, reusing objects can be a powerful optimization strategy. Instead of creating new instances of an object, developers can reuse existing ones, especially in cases where object instantiation is costly:
class Point: def __init__(self, x, y): self.x = x self.y = y # Reusing objects point = Point(1, 2) for _ in range(100): point.x += 1 point.y += 1
Using techniques like object pooling—where a fixed number of objects are created and reused—can also be beneficial for applications that frequently create and destroy objects. This technique is particularly useful in scenarios like game development or handling graphical elements.
Furthermore, being mindful of the overall data structure choice is vital. For instance, using a set
for membership checks is often more efficient than using a list due to the underlying hash table implementation. Here’s a comparison:
# Using a list my_list = [1, 2, 3, 4, 5] if 3 in my_list: print("Found in list") # Using a set my_set = {1, 2, 3, 4, 5} if 3 in my_set: print("Found in set")
Using a set drastically reduces the time complexity of membership checks from O(n) for lists to O(1) for sets, thus improving performance when dealing with large data collections.
Finally, employing memory profiling tools such as memory_profiler
or objgraph
can provide insight into memory usage patterns within your application. These tools allow you to visually inspect memory consumption and identify potential leaks or inefficient memory usage:
from memory_profiler import profile @profile def my_function(): a = [i for i in range(10000)] return a my_function()
By applying these optimization techniques judiciously, developers can craft Python applications that not only perform efficiently but also maintain a manageable memory footprint, allowing for scalability and robustness in a variety of environments.
Common Memory Management Pitfalls
Despite Python’s robust memory management capabilities, developers often encounter common pitfalls that can lead to inefficient memory usage and potential performance degradation. Recognizing and avoiding these pitfalls is essential for writing high-performance Python applications.
One major pitfall is over-reliance on mutable objects. While mutable types like lists and dictionaries provide flexibility, they can lead to unintended memory usage patterns. For example, if a large list is being modified frequently, it may lead to frequent reallocations. This not only consumes memory but can also slow down program execution due to the overhead associated with resizing. Here’s an illustration:
my_list = [] for i in range(1000000): my_list.append(i) # Frequent resizing may occur if capacity is exceeded
In cases where the final size of a list is known in advance, it’s beneficial to pre-allocate the list to avoid these frequent reallocations:
max_size = 1000000 my_list = [None] * max_size for i in range(max_size): my_list[i] = i # Now we avoid frequent resizing
Another common pitfall arises from circular references, particularly when dealing with complex data structures or classes that reference each other. Although Python’s garbage collector can handle these cases, excessive circular references can lead to performance issues during garbage collection cycles. Developers should aim to minimize circular references, or if necessary, use weak references from the `weakref` module to prevent memory leaks:
import weakref class Node: def __init__(self, value): self.value = value self.next = None # Using weak references to break circular references a = Node(1) b = Node(2) a.next = weakref.ref(b) # a holds a weak reference to b b.next = weakref.ref(a) # b holds a weak reference to a
Another pitfall is the tendency to create unnecessary object copies. This often happens with mutable objects where a developer mistakenly believes that sharing an object is unsafe. Instead, using methods like slicing and the `copy` module judiciously can help manage memory more efficiently. For instance, instead of copying a large list, consider using views or references when applicable:
import copy original_list = [1, 2, 3, 4, 5] # Creating a shallow copy copied_list = copy.copy(original_list) # Here, modify the copied_list without affecting the original copied_list.append(6)
Moreover, developers often overlook the implications of global variables or long-lived objects. Objects that are unnecessarily kept in memory can lead to increased memory consumption and slower performance. To combat this, it’s advisable to limit the scope of variables as much as possible and to clean up objects that are no longer needed:
def process_data(): data = [i for i in range(100000)] # Local scope # Process data... return data result = process_data() # Once the function returns, the data can be garbage collected
Lastly, using excessive logging and debugging statements can lead to bloated memory usage, especially in production environments. Each log statement consumes memory, and if log levels are not managed properly, it can result in high memory overhead. It’s advisable to use logging levels effectively and to disable extensive logging in production code:
import logging # Set logging level logging.basicConfig(level=logging.INFO) # In production, consider using a higher level like WARNING or ERROR logging.info("This is an informational message.") # Avoid excessive info logging in production
By being mindful of these common memory management pitfalls, developers can improve the efficiency and performance of their Python applications. Recognizing when to use mutable versus immutable types, managing object lifetimes, and carefully structuring data can lead to more robust and performant code.
Tools and Techniques for Memory Profiling
In the sphere of Python development, memory profiling serves as an indispensable tool for identifying bottlenecks and optimizing resource utilization. By gathering data on memory usage, developers can make informed decisions that enhance performance and mitigate potential leaks. Fortunately, Python provides several libraries and techniques to facilitate effective memory profiling.
One of the most commonly used tools for memory profiling in Python is the memory_profiler library. This tool allows developers to monitor memory consumption line by line, making it easier to pinpoint areas of high memory usage. To use memory_profiler, you first need to install it via pip:
pip install memory-profiler
Once installed, you can decorate functions with the @profile
decorator to collect memory usage statistics. Here’s an example illustrating this:
from memory_profiler import profile @profile def compute_numbers(): total = [] for i in range(100000): total.append(i ** 2) return total compute_numbers()
This will produce a detailed report showing how much memory each line of code uses, allowing you to identify which operations are the most memory-intensive.
Another powerful tool for memory profiling is tracemalloc, which is included in the standard library starting from Python 3.4. The tracemalloc module tracks memory allocations and helps you identify where memory is being consumed in your code. To use it, you can start tracking memory at the beginning of your program and take snapshots at various points:
import tracemalloc tracemalloc.start() # Start tracking memory allocations # Code that consumes memory data = [i for i in range(100000)] # Take a snapshot of the current memory usage snapshot = tracemalloc.take_snapshot() top_stats = snapshot.statistics('lineno') print("[ Top 10 memory usage ]") for stat in top_stats[:10]: print(stat)
This approach provides insights into your program’s memory allocation over time, highlighting the lines that consume the most memory.
For a more visual representation, the objgraph library can be invaluable. It helps visualize the object graphs and can highlight memory leaks by displaying the references between objects. To install objgraph, you can use pip:
pip install objgraph
Here’s a basic example of using objgraph to display the most common types of objects in memory:
import objgraph # Generate some objects my_list = [str(i) for i in range(10000)] # Show the most common types of objects objgraph.show_most_common_types(limit=10)
This command prints out the most common object types in memory, helping you understand what types of objects are consuming memory in your application.
Lastly, Python’s built-in gc (garbage collection) module can also assist in memory profiling. By using it, you can analyze how many objects are currently being tracked by the garbage collector, which can help identify potential memory leaks:
import gc # Collect and print garbage collection statistics gc.collect() # Force garbage collection print(f"Unreachable objects collected: {gc.collect()}")
Using these tools effectively allows developers to gain deep insights into memory usage patterns, identify inefficiencies, and optimize their applications accordingly. Profiling should be an integral part of the development process, especially for memory-intensive applications. By proactively managing memory, developers can ensure their Python programs run smoothly and efficiently.