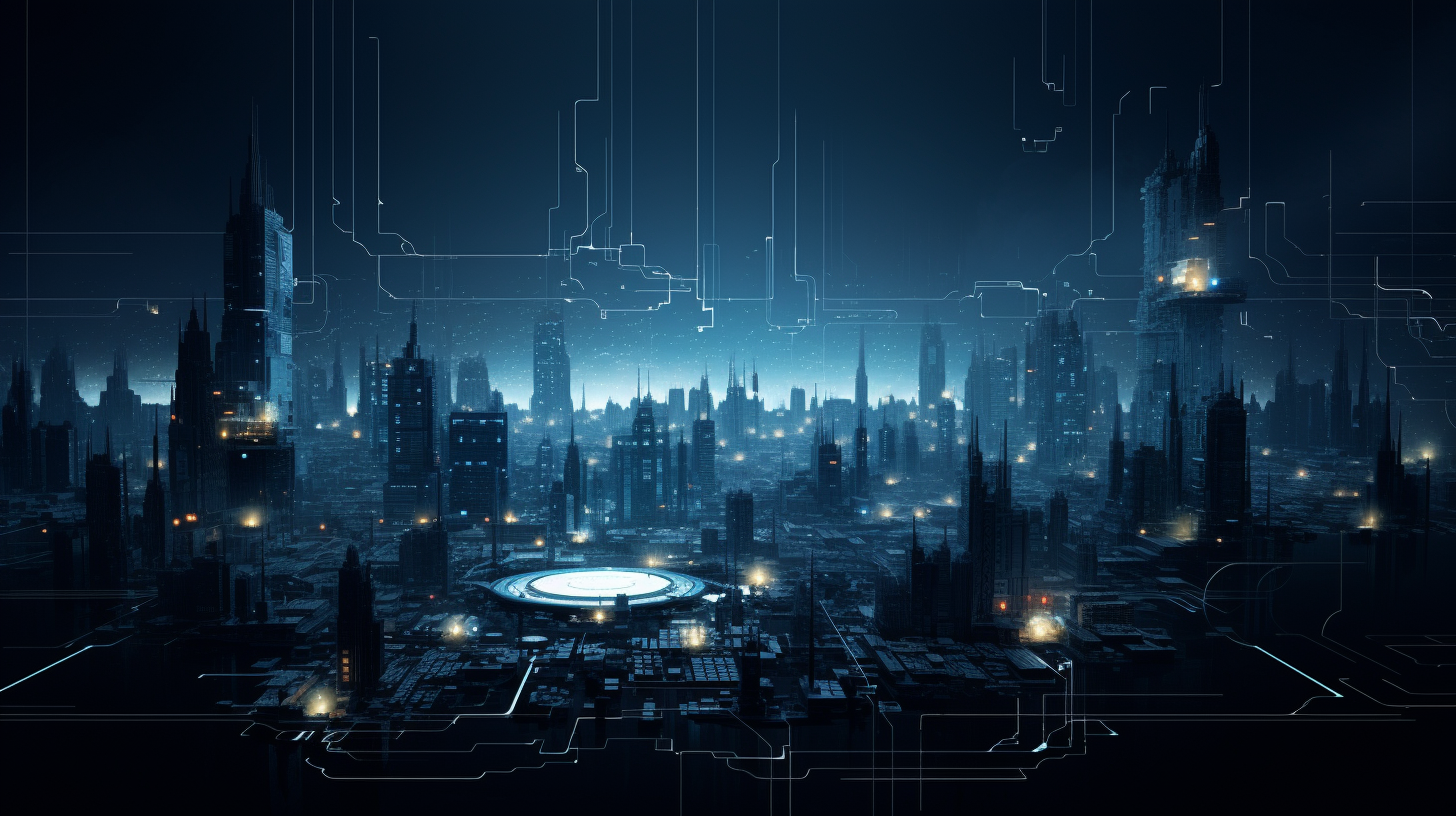
Python Regular Expressions for Pattern Matching
Regular expressions, or regex, serve as a powerful tool for string manipulation, allowing you to define search patterns that can match sequences of characters within text. In Python, regular expressions are implemented through the `re` module, providing a wide array of functionalities that can efficiently handle complex string operations.
At its core, a regular expression is a special sequence of characters that forms a search pattern. This pattern can be as simple as matching a single character or as complex as a multi-line string search. The beauty of regex lies in its ability to condense intricate search criteria into concise expressions, making it indispensable for tasks such as data validation, searching, and text extraction.
When using regex in Python, you’ll often encounter several common elements:
- These are the simplest forms of regex. For instance, the pattern
hello
will match the substring ‘hello’ in your text. - Characters like
.
,*
,?
, and+
have special meanings. For example, the dot.
matches any single character, while the asterisk*
matches zero or more occurrences of the preceding element. - Enclosed in square brackets
[ ]
, character classes allow you to specify a set of characters any of which can match at that position. For instance,[abc]
will match any single ‘a’, ‘b’, or ‘c’. - These help to specify the position of a match. The caret
^
indicates the start of a string, while the dollar sign$
indicates the end. Thus,^hello$
matches the string ‘hello’ only if it appears in its entirety.
To illustrate, ponder the following Python code snippet that demonstrates how to compile and use a basic regular expression:
import re # Define a simple regex pattern to match email addresses pattern = r'[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,}' # Sample text text = "Please contact us at [email protected] for assistance." # Perform the search matches = re.findall(pattern, text) # Output the matches print(matches) # Output: ['[email protected]']
In this example, the regular expression is designed to match basic email structures. The re.findall()
function is employed to search for all occurrences of the pattern within the given text. The result is a list of all email addresses that fit the specified pattern.
Understanding the fundamentals of regular expressions in Python opens up a world of possibilities for efficient text processing. Whether you are parsing logs, validating user inputs, or searching through large datasets, regex can simplify these tasks, enabling you to focus on the logic and functionality of your application.
Common Patterns and Syntax
Regular expressions offer a rich syntax that allows for the creation of patterns capable of matching a variety of text structures. To harness the power of regex effectively, one must become familiar with several common patterns and syntax rules. Below are key components of regex syntax that are essential for constructing effective patterns.
Anchors: Anchors are critical for defining the position of a match within a string. The caret (^) signifies the start of a string, while the dollar sign ($) indicates the end. For example, if you want to match a string that starts with “Hello” and ends with “World”, you could use the pattern:
pattern = r'^Hello.*World$'
This will only match strings that begin with “Hello” and conclude with “World”, regardless of what content is found in between.
Quantifiers: Quantifiers specify how many instances of a character or group must be present for a match to occur. Here are some common quantifiers:
- Matches 0 or more occurrences of the preceding expression.
- Matches 1 or more occurrences.
- Matches 0 or 1 occurrence.
- Matches exactly n occurrences.
- Matches n or more occurrences.
- Matches between n and m occurrences.
For example, the regex pattern r'd{3,5}'
will match any sequence of digits consisting of 3 to 5 characters. If you want to extract numbers from a text, you could use:
import re # Define a pattern to match numbers with 3 to 5 digits pattern = r'd{3,5}' # Sample text text = "The lottery numbers are 123, 45678, and 90." # Perform the search matches = re.findall(pattern, text) # Output the matches print(matches) # Output: ['123', '45678']
Groups and capturing: Parentheses are used to create groups in regex, which will allow you to apply quantifiers to entire blocks of expressions or to capture portions of the matched text for further processing. For instance, the pattern r'(d{3})-(d{2})-(d{4})'
captures Social Security numbers in the format XXX-XX-XXXX.
import re # Define a pattern to capture parts of a Social Security number pattern = r'(d{3})-(d{2})-(d{4})' # Sample text text = "My SSN is 123-45-6789." # Perform the search match = re.search(pattern, text) # Output captured groups if match: print(match.groups()) # Output: ('123', '45', '6789')
Character Classes: Character classes allow for the specification of a set of characters any of which can match at that position. For example, the regex pattern r'[aeiou]
will match any single vowel. You can also combine ranges within character classes, such as r'[a-zA-Z]
to match any letter.
Escape Sequences: Since certain characters (like . ^ $ * + ? { } [ ] | ( )) are reserved and have special meanings in regex, you must escape these characters with a backslash () when you want to match them literally. For instance, to match a period, you would use r'.'
.
By understanding these common patterns and syntax elements, you can craft powerful regular expressions tailored to your specific needs. Mastering these building blocks of regex will enable you to take on even the most complex text-processing challenges with confidence.
Using the `re` Module for Pattern Matching
To effectively utilize regular expressions in Python, you must become intimately familiar with the `re` module, which serves as the principal interface for regex operations. This module provides a suite of functions that enable you to search for, match, and manipulate strings through the power of regex. The primary functions you will interact with include re.search()
, re.match()
, re.findall()
, re.sub()
, and re.split()
.
The re.search()
function scans through a string, looking for any location where the regex pattern produces a match. It returns a match object if a match is found; otherwise, it returns None
. For example:
import re # Define a regex pattern to find the word 'Python' pattern = r'Python' # Sample text text = "I love programming in Python and Java." # Perform the search match = re.search(pattern, text) # Check if a match was found if match: print("Match found at position:", match.start()) # Output: Match found at position: 20
The re.match()
function, in contrast, only checks for a match at the start of the string. It returns a match object if the pattern matches the beginning; otherwise, it returns None
. Here’s an example:
import re # Define a regex pattern to match 'Hello' at the start of the string pattern = r'Hello' # Sample text text = "Hello, world!" # Perform the match match = re.match(pattern, text) # Check if a match was found if match: print("Match found at the beginning of the string.") # This will print
For finding all occurrences of a pattern in a string, re.findall()
is your go-to function. It returns all non-overlapping matches of the pattern as a list. Ponder the following example that extracts all words from a given text:
import re # Define a pattern to match all words pattern = r'bw+b' # Sample text text = "This is a test sentence." # Perform the search matches = re.findall(pattern, text) # Output the matches print(matches) # Output: ['This', 'is', 'a', 'test', 'sentence']
When you need to perform string substitutions based on a regex pattern, re.sub()
is the function to use. It allows you to replace occurrences of the matched pattern with a specified string. For example:
import re # Define a pattern to match digits pattern = r'd+' # Sample text text = "My phone number is 123-456-7890." # Replace digits with X's new_text = re.sub(pattern, 'X', text) # Output the result print(new_text) # Output: My phone number is X-XXX-XXXX.
Lastly, re.split()
is useful for splitting a string by the occurrences of a regex pattern. This can be particularly handy when dealing with delimiters. Here’s how you might split a string based on whitespace:
import re # Define a pattern to split by whitespace pattern = r's+' # Sample text text = "Split this sentence into words." # Perform the split words = re.split(pattern, text) # Output the result print(words) # Output: ['Split', 'this', 'sentence', 'into', 'words.']
With these functions at your disposal, the `re` module equips you with powerful tools for pattern matching and text manipulation. Mastery of these capabilities not only enhances your ability to process strings effectively but also allows you to build robust applications that can handle complex text operations with ease.
Practical Examples and Use Cases
When it comes to practical applications of regular expressions in Python, the possibilities are endless. Whether you are parsing logs, validating forms, extracting data from documents, or cleaning up textual data, knowing how to leverage the power of regex is key. Here are several scenarios that illustrate the utility of regex alongside relevant code examples.
1. Validating User Inputs
Validating user inputs, such as emails, phone numbers, or passwords, is a common use case for regular expressions. For example, think a scenario where you want to ensure that a user enters a valid phone number in the format (XXX) XXX-XXXX.
import re # Define a pattern for phone numbers pattern = r'^(d{3}) d{3}-d{4}$' # Sample text text = "(123) 456-7890" # Perform the validation if re.match(pattern, text): print("Valid phone number.") else: print("Invalid phone number.") # This will not print in this case
This regex pattern checks for a specific format, ensuring the input adheres to the desired structure before processing it further.
2. Extracting Dates from Text
Extracting structured data from unstructured text can be efficiently handled with regex. For example, suppose you need to extract dates in the format DD/MM/YYYY from a block of text.
import re # Define a pattern for dates pattern = r'b(d{2})/(d{2})/(d{4})b' # Sample text text = "The deadlines are 15/05/2023 and 30/06/2024." # Find all dates matches = re.findall(pattern, text) # Output the matches print(matches) # Output: [('15', '05', '2023'), ('30', '06', '2024')]
In this example, the `re.findall()` function extracts all occurrences of the date pattern, returning them as tuples for further manipulation or storage.
3. Replacing Text Patterns
Regular expressions are also incredibly useful for replacing specific text patterns. For instance, if you want to anonymize certain sensitive information, such as email addresses, in a document, you can use regex to substitute them with a placeholder.
import re # Define a pattern to match email addresses pattern = r'[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,}' # Sample text text = "Please contact us at [email protected] or [email protected]." # Replace email addresses with '[REDACTED]' new_text = re.sub(pattern, '[REDACTED]', text) # Output the result print(new_text) # Output: Please contact us at [REDACTED] or [REDACTED].
This example demonstrates how you can leverage regex to find and replace patterns, making it an invaluable tool for maintaining privacy in applications.
4. Splitting Strings on Complex Delimiters
Sometimes, you may need to split a string using complex delimiters, such as multiple whitespace characters, commas, or semicolons. The `re.split()` function allows for just that.
import re # Define a pattern to split by comma or whitespace pattern = r'[,s]+' # Sample text text = "apple, orange; banana,grape melon" # Perform the split fruits = re.split(pattern, text) # Output the result print(fruits) # Output: ['apple', 'orange', 'banana', 'grape', 'melon']
Here, the regex pattern accounts for both commas and whitespace, allowing for flexible parsing of the input string. This can be especially useful when dealing with user-generated content, where formatting inconsistencies can occur.
As demonstrated, regular expressions provide a powerful means of handling various text-processing tasks in Python. The combination of `re` module functions with well-crafted regex patterns allows you to tackle a wide array of challenges in data manipulation, validation, and extraction with grace and efficiency.