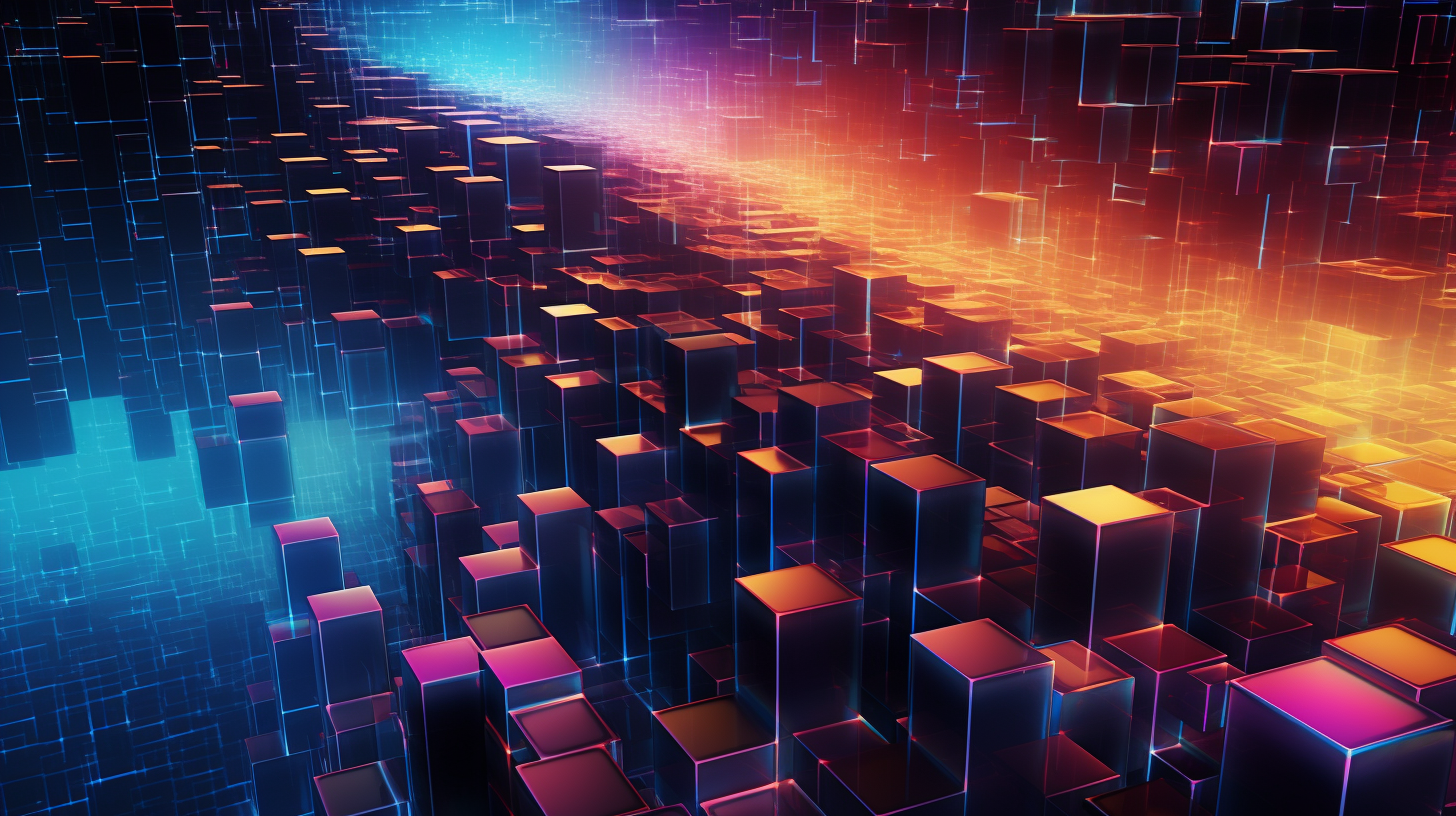
Python Syntax and Indentation Rules
Python syntax is designed to be clear and simpler, which makes the language highly accessible to developers, including those new to programming. In Python, code readability is emphasized, and this is achieved through a set of rules that dictate how code should be structured. This structure enables developers to express concepts in fewer lines of code compared to other programming languages, which can often lead to increased productivity.
At its core, Python syntax consists of various elements such as keywords, operators, and punctuation, which are combined to create statements and expressions. For instance, the use of def
to define functions or if
for conditional statements are quintessential aspects of Python’s syntax. Such keywords serve as commands that dictate the flow of the program.
Here is a simple function definition in Python:
def greet(name): return f"Hello, {name}!"
In the above example, def
indicates that we are defining a function named greet
, which takes a parameter name
. The string returned by the function utilizes an f-string for formatting, showcasing Python’s intuitive approach to integrating variables into strings.
Moreover, Python syntax is consistent in its use of whitespace. Unlike many programming languages that use braces or keywords to define blocks of code, Python uses indentation to establish scope. This contributes to the language’s clean aesthetic and helps in reducing syntactic clutter.
For example, the following code snippet illustrates the significance of indentation:
if True: print("This will always print.") else: print("This will never print.")
Here, the indented line following the if
statement belongs to the block controlled by that statement, while the else
block is at the same indentation level as its corresponding if
. This explicit structure especially important in Python and underscores the idea that indentation is not merely a stylistic choice; it’s a fundamental part of the syntax.
As you delve deeper into Python, understanding its syntax will enable you to write code that’s not only functional but also clear and maintainable. The combination of readability and simplicity is what makes Python a favorite among developers, whether they are building simple scripts or complex applications.
Importance of Indentation in Python
Indentation in Python is not just a matter of style; it’s a critical component of the language’s syntax that defines the structure and flow of a program. Unlike many other programming languages that rely on curly braces or other delimiters to indicate code blocks, Python uses indentation levels to signify grouping of statements. This unique approach has profound implications for how developers write and understand code.
Firstly, the significance of indentation can be observed in defining control structures, such as loops and conditionals. For instance, in an if-else statement, the lines of code that belong to each block must be properly indented. If the indentation is inconsistent, Python raises an IndentationError, making it clear that the programmer’s intent was not properly communicated. Here’s an illustrative example:
if condition: execute_action() additional_action() else: alternative_action()
In the above snippet, both execute_action()
and additional_action()
are executed if condition
is true, while alternative_action()
is executed otherwise. The clarity provided by indentation allows anyone who reads the code to immediately understand the logical flow without needing to sift through extraneous syntax.
Moreover, consistent and coherent indentation enhances the readability of the code, making it easier to maintain and debug. A well-indented block of code visually conveys its structure and intent, allowing developers to quickly ascertain the program’s logic. For instance:
for i in range(5): if i % 2 == 0: print(f"{i} is even") else: print(f"{i} is odd")
In this example, the indentation clearly demarcates the actions that occur within the loop and the conditional. Such clear delineation reduces cognitive load and minimizes the likelihood of errors during code modification.
It is also essential to note that mixing tabs and spaces for indentation can lead to unexpected behavior. Python 3 disallows mixing of the two, enforcing a uniform approach to whitespace. This strict adherence to indentation rules makes Python a language that values both simplicity and precision. Take a look at the following incorrect example:
def example_function(): print("Hello, World!") print("This will raise an error.")
Running the above code will raise an IndentationError due to the inconsistency between the two lines. Such errors can be easily avoided by adhering to a single method of indentation—either using spaces or tabs consistently throughout your codebase.
Ultimately, the importance of indentation in Python cannot be overstated. It’s a fundamental aspect that not only dictates how code executes but also how it is perceived by others. By adhering to proper indentation practices, developers can write Python code that is not just functional, but also readable, maintainable, and less prone to errors.
Basic Syntax Elements
Python syntax is built on a foundation of basic elements that together form the structure of the language. These fundamental elements include keywords, operators, literals, and punctuation, which work in concert to allow developers to express ideas clearly and concisely.
Keywords are reserved words in Python that have special meanings. They serve as the building blocks of Python programming and dictate the flow and structure of the code. For example, the keyword if
is used to introduce conditional statements:
if condition: # execute this block if the condition is true
Another essential keyword is def
, which is used to define functions. Combining these keywords with operators and other elements allows developers to create complex logic. For example:
def calculate_square(number): return number * number
Here, def
initiates the function definition, while the multiplication operator *
computes the square of the provided number.
Operators play a critical role in Python’s syntax, enabling developers to perform various operations on data. Python supports a range of operators, including arithmetic, logical, comparison, and bitwise operators. For instance, arithmetic operators allow for mathematical computations:
a = 10 b = 5 sum_result = a + b # Addition difference = a - b # Subtraction product = a * b # Multiplication quotient = a / b # Division
In this example, each operation uses an operator to manipulate the variables a
and b
. Understanding how to use these operators effectively is key to performing calculations within your Python programs.
Literals are constant values that are represented directly in the code. They can be numbers, strings, lists, or any other type of data that’s used in your program. For example:
name = "Alice" # string literal age = 30 # integer literal height = 5.5 # float literal is_student = True # boolean literal
In the code snippet above, the variables are assigned literal values that can be used throughout the program. This allows developers to refer to data easily without needing to redefine it.
Punctuation marks, such as commas, colons, and parentheses, are also integral to Python syntax. They help in delineating statements, defining function parameters, and organizing code structure. For instance, the use of a comma in a list:
fruits = ["apple", "banana", "cherry"] # list with punctuations
Here, commas separate the elements of the list, while the square brackets denote the list itself. Similarly, colons are used to indicate the beginning of a block, as seen in function definitions and control flow statements.
The interaction of these basic syntax elements creates a language that’s not only powerful but also uncomplicated to manage. Each element is designed to contribute to the overall clarity and efficiency of Python code, empowering developers to create solutions that are both effective and easy to understand.
Common Syntax Errors
Common syntax errors in Python can be particularly vexing, especially for newcomers who are still acclimating to the language’s unique structure. These errors often stem from minor oversights, yet they can halt program execution and lead to confusion. Understanding the most prevalent syntax errors can empower developers to write cleaner, more reliable code.
One of the most frequently encountered syntax errors is the IndentationError. This error arises when the indentation levels of code blocks are inconsistent. Python relies on indentation to define the scope of loops, conditionals, and functions. For example, consider the following code snippet:
def example_function(): print("This is correctly indented.") print("This will raise an IndentationError.")
In this case, the second print statement has inconsistent indentation compared to the first statement, leading to an error. The interpreter will raise an IndentationError, indicating that the expected indentation is not met. Properly aligning the statements resolves the issue:
def example_function(): print("This is correctly indented.") print("This is also correctly indented.")
Another common syntax error is the SyntaxError, which occurs when the Python interpreter encounters code that does not conform to the expected syntax structure. This can happen for various reasons, such as missing colons, mismatched parentheses, or improper use of keywords. For instance:
if x > 10 print("x is greater than 10") # Missing colon
In this example, the absence of a colon at the end of the if statement causes a syntax error. To fix it, simply add the colon:
if x > 10: print("x is greater than 10")
Syntax errors can also arise from improperly terminated strings. For example:
message = "Hello, World # Missing closing quote
In this case, the string is not properly closed with a quotation mark, resulting in a syntax error. Adding the closing quote will correct this:
message = "Hello, World"
Another point of contention is the use of unexpected EOF while parsing error, which indicates that the interpreter reached the end of the file without finding the expected closing statement, such as for a loop or function. Think the following snippet:
for i in range(5): print(i)
If this loop were accidentally left incomplete, such as omitting a closing parenthesis in a nested function call, it would lead to this type of syntax error. Properly closing all statements is essential to prevent such issues.
Lastly, using the wrong type of quotes can lead to syntax errors as well. If single quotes are opened, they must be closed with a single quote, and similarly for double quotes. Mixing them can produce unexpected results:
greeting = "Hello, World' # Mismatched quotes
Correcting this requires ensuring that the opening and closing quotes match:
greeting = "Hello, World"
By familiarizing oneself with these common syntax errors, developers can enhance their Python programming skills and minimize interruptions in their coding flow. Adhering to Python’s structural rules and maintaining consistent formatting will lead to fewer syntax-related issues and allow for more efficient coding practices.
Best Practices for Writing Python Code
When it comes to writing Python code, adhering to best practices can significantly enhance the quality, maintainability, and readability of your codebase. These practices are not just about personal preference; they also establish a common ground among developers, making collaboration smoother and reducing the likelihood of errors.
One fundamental best practice is to follow the PEP 8 style guide, which outlines a set of conventions for writing Python code. This includes guidelines on naming conventions, line lengths, and spacing. For instance, function names should be in snake_case, while class names should follow PascalCase. Here’s how you might implement these conventions:
def calculate_area(radius): return 3.14 * radius * radius class Circle: def __init__(self, radius): self.radius = radius
In this example, the function calculate_area
uses snake_case, while the class Circle
follows PascalCase rules. Consistency in naming helps other developers quickly understand the purpose of the code.
Another important aspect of writing good Python code is keeping functions and classes small and focused. Each function should perform a single task, making it easier to test, understand, and reuse. For example:
def get_circle_area(radius): return calculate_area(radius) def print_circle_area(radius): area = get_circle_area(radius) print(f"Area of the circle: {area}")
By separating concerns into different functions, you enhance modularity and clarity. It becomes easier to test get_circle_area
independently from print_circle_area
, promoting better code design.
Moreover, documentation especially important for maintaining clarity, especially in larger codebases. Using docstrings to describe the purpose of functions and classes is a best practice that can save time for both the original developer and future contributors. Here’s how you can document a function:
def calculate_square(number): """Calculate the square of a number. Args: number (int or float): The number to be squared. Returns: int or float: The square of the input number. """ return number * number
The use of docstrings not only clarifies the function’s intent but also allows tools like Sphinx to automatically generate documentation for your code. This contributes to a more professional and usable codebase.
When it comes to error handling, using exceptions is a vital best practice. Wrapping code that may cause errors in try-except
blocks helps manage unexpected situations gracefully. For instance:
def safe_division(a, b): try: return a / b except ZeroDivisionError: return "Cannot divide by zero!"
This approach not only prevents your program from crashing but also provides meaningful feedback to the user, thereby improving the overall user experience.
Lastly, make use of version control systems like Git to track changes and collaborate more effectively. Committing changes with clear messages allows other developers to understand the evolution of the codebase. Here’s a simple Git command to commit changes:
git commit -m "Refactor area calculation function for clarity"
Implementing these best practices—following PEP 8, keeping functions focused, documenting your code, handling errors gracefully, and using version control—will greatly improve the quality of your Python code. Emphasizing readability and maintainability leads to a more enjoyable programming experience and facilitates teamwork in any development project.