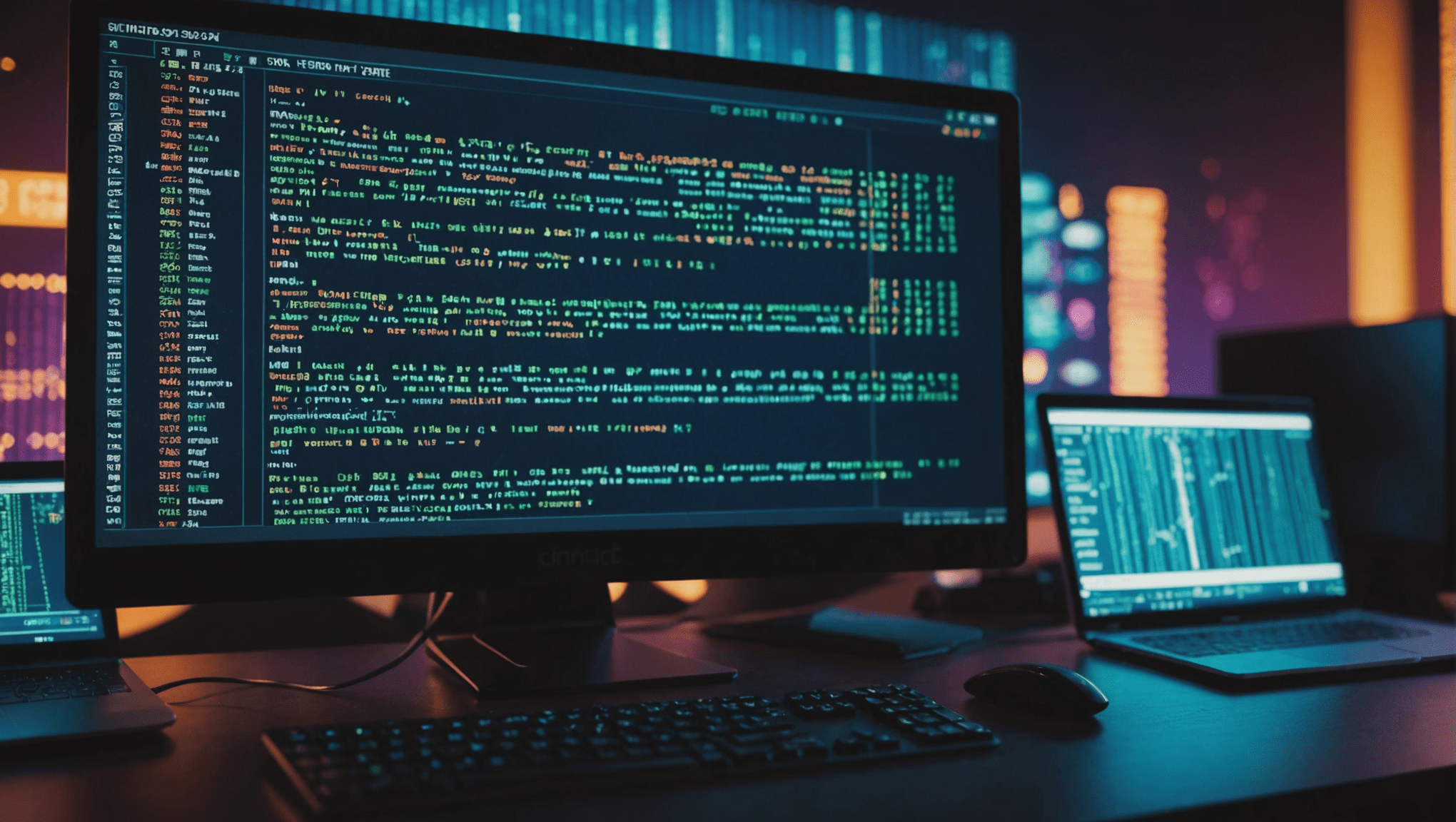
Python Testing: Unit Tests and Test Cases
Unit testing is a fundamental aspect of software development, especially in Python. It focuses on verifying that individual components of a program, known as units, work as intended. A unit can be a single function, method, or class. By testing these small parts of an application, developers can identify issues early and ensure that their code behaves as expected.
In Python, the primary objective of unit testing is to facilitate defect detection. It allows developers to isolate pieces of code, making it easier to track down bugs. This method also contributes to better design, as writing unit tests often leads to cleaner and more modular code.
Unit tests typically follow a simple structure that includes:
- Preparing the necessary context or state before executing the unit to be tested.
- Calling the unit under test with specific inputs.
- Checking that the output matches the expected result.
- Cleaning up any resources or state after the test has executed.
Python’s unittest framework provides tools and conventions to write and manage unit tests effectively. A well-designed unit test not only checks for correct outputs but also examines edge cases and handles exceptions gracefully.
For example, ponder a simple function that calculates the square of a number:
def square(n): return n * n
To thoroughly test this function, one would write a set of unit tests like this:
import unittest class TestMathFunctions(unittest.TestCase): def test_square_positive(self): self.assertEqual(square(3), 9) def test_square_zero(self): self.assertEqual(square(0), 0) def test_square_negative(self): self.assertEqual(square(-3), 9) if __name__ == '__main__': unittest.main()
In this example, we have defined three tests that cover positive, zero, and negative inputs. By running these tests, we ensure that the square
function behaves correctly across a range of scenarios.
Understanding unit testing very important in Python as it lays the groundwork for creating robust and maintainable code. It helps in identifying issues early in the development cycle and encourages developers to write well-structured programs.
Writing Your First Unit Test
To begin writing your first unit test, you will first need to create a function that you want to test. Let’s expand on the previous example and write a function that checks whether a number is even or odd:
def is_even(n): return n % 2 == 0
Now that we have our function, we can create tests to verify its correctness. Here’s how you can write your first unit test using Python’s unittest framework:
import unittest class TestEvenOddFunctions(unittest.TestCase): def test_is_even_with_even_number(self): self.assertTrue(is_even(4), "Should be True for even numbers") def test_is_even_with_odd_number(self): self.assertFalse(is_even(5), "Should be False for odd numbers") def test_is_even_with_zero(self): self.assertTrue(is_even(0), "Should be True for zero") if __name__ == '__main__': unittest.main()
In the above code, we define a class TestEvenOddFunctions that inherits from unittest.TestCase. Each method within this class represents a specific test. We use self.assertTrue() and self.assertFalse() methods to perform our assertions. This structure allows us to verify that:
- Number 4 is identified as even.
- Number 5 is identified as odd.
- Zero is identified as even.
When you run the unit tests, the unittest framework will automatically call each test method and report back on their success or failure. That is a powerful way to validate that your code is functioning correctly.
By following this method, you can begin to cover more complex scenarios and additional functions within your codebase. It’s essential to continuously add unit tests as you write more feature-rich applications to maintain code reliability and integrity.
Organizing Test Cases in Python
Organizing your test cases effectively especially important for maintaining clear and manageable unit tests in Python. A well-structured test suite not only helps in the understanding of the tests themselves but also facilitates easier modifications and troubleshooting as your codebase grows. Below are some key strategies for organizing test cases in Python.
- Group related tests into classes, where each class typically covers a specific module or class in your codebase. This encapsulation simplifies managing tests for a given functionality.
- Create a dedicated directory for your tests, often named
tests
. Within that directory, you might have subdirectories that correspond to different application modules. This structure keeps your codebase organized. - Use descriptive names for your test methods that indicate what functionality is being tested, following a consistent naming pattern. For example, use the format
test__
, which enhances readability. - Use setup methods to prepare the state before running tests. This can minimize code duplication across tests that share common setup requirements.
- Document your test cases. Although self-explanatory names help, adding docstrings to your test classes and methods can clarify the purpose of complex tests.
Here’s an example that illustrates these practices:
import unittest def add(a, b): return a + b def subtract(a, b): return a - b class TestMathOperations(unittest.TestCase): def setUp(self): self.num1 = 10 self.num2 = 5 def test_add(self): """Test addition of two numbers.""" self.assertEqual(add(self.num1, self.num2), 15) def test_subtract(self): """Test subtraction of two numbers.""" self.assertEqual(subtract(self.num1, self.num2), 5) if __name__ == '__main__': unittest.main()
In this example, we have a TestMathOperations
class that groups tests related to basic mathematical operations. The setUp
method initializes common inputs, reducing redundancy in the individual test methods. Each test method also includes a docstring to clarify its purpose, following the established naming conventions.
By employing these strategies, you can ensure that your unit tests remain organized, easy to read, and maintainable as your project evolves, ultimately contributing to more stable and reliable software development.
Using the unittest Module
The unittest module in Python provides a robust framework for testing your code. It allows developers to create test cases, test suites, and test runners, facilitating an organized approach to validating code correctness. With unittest, you can define your tests in a way that they can be bundled together, making it easier to run a group of tests collectively.
To leverage the unittest module, you typically start by importing it, as shown in the previous examples. The unittest framework includes a variety of assert methods that simplify the process of verifying conditions in your test cases. Here’s a brief overview of some commonly used assert methods:
- Checks if
a
equalsb
. - Checks if
a
does not equalb
. - Checks if
x
is True. - Checks if
x
is False. - Checks if
x
is None. - Checks if
x
is not None. - Checks if an exception is raised when calling a function with specified arguments.
To illustrate the use of unittest more concretely, let’s ponder a new function that raises an exception when dividing by zero. We can implement both the function and its corresponding tests as follows:
def divide(a, b): if b == 0: raise ValueError("Cannot divide by zero") return a / b import unittest class TestDivideFunction(unittest.TestCase): def test_divide_positive(self): self.assertEqual(divide(10, 2), 5) def test_divide_negative(self): self.assertEqual(divide(-10, 2), -5) def test_divide_zero_divisor(self): with self.assertRaises(ValueError): divide(10, 0) if __name__ == '__main__': unittest.main()
In the above example, we defined a divide
function that raises a ValueError
if an attempt to divide by zero is made. The TestDivideFunction
class contains three tests: one for regular positive division, one for negative division, and one that specifically checks for the division by zero exception using the assertRaises
method.
When you run this test suite, unittest executes each method prefixed with test_
and reports successes and failures. This encapsulation of tests makes it simpler to validate that the function behaves correctly in different scenarios.
Moreover, the unittest module supports fixtures, which are setup methods that allow for common initialization logic shared across tests. Using the setUp
and tearDown
methods, you can manage the test environment in a consistent way. For instance:
class TestSetUpAndTearDown(unittest.TestCase): def setUp(self): self.value = 10 def tearDown(self): print("Cleaning up after test.") def test_double_value(self): self.assertEqual(self.value * 2, 20) if __name__ == '__main__': unittest.main()
In this example, the setUp
method initializes a variable before each test runs. The tearDown
method prints a message after each test, indicating cleanup has occurred. This modularization not only keeps your tests organized but also ensures that each test operates under well-defined conditions.
Using the unittest module allows you to formally document your tests, which can be beneficial for both current and future developers working on the codebase. The combination of a structured testing approach and powerful assertions makes unittest an essential tool for Python developers looking to deliver high-quality, reliable software.
Best Practices for Writing Effective Tests
When writing effective tests in Python, adhering to certain best practices can make a significant difference in the maintainability and usefulness of your unit tests. Here are some strategies to ensure that your tests are not only effective but also easy to understand and manage:
- Each test should be simpler and focused on a single functionality. Avoid testing too many features in a single test case. If your test is too complex or covers multiple scenarios, consider breaking it down into smaller, more targeted tests.
- Name your test methods to reflect the behavior being tested. This clarity will make it easier for both you and others to understand what each test is meant to validate. For example, instead of naming a test method
test1
, usetest_is_even_with_even_number
. - When possible, use constants or variables for the values you’re testing. This approach enhances the readability of your tests and makes it easier to update them if the requirements change. For example, consider defining constants for expected results instead of hardcoding numbers directly in assertions.
Here’s an example of these best practices in action:
import unittest def is_even(n): return n % 2 == 0 class TestEvenFunction(unittest.TestCase): def test_is_even_with_positive_even_number(self): """Test if the function correctly identifies even numbers.""" self.assertTrue(is_even(4), "Expected True for even number.") def test_is_even_with_positive_odd_number(self): """Test if the function correctly identifies odd numbers.""" self.assertFalse(is_even(5), "Expected False for odd number.") def test_is_even_with_negative_even_number(self): """Test if the function correctly identifies negative even numbers.""" self.assertTrue(is_even(-2), "Expected True for negative even number.") def test_is_even_with_negative_odd_number(self): """Test if the function correctly identifies negative odd numbers.""" self.assertFalse(is_even(-3), "Expected False for negative odd number.") def test_is_even_with_zero(self): """Test if the function correctly identifies zero as even.""" self.assertTrue(is_even(0), "Expected True for zero.") if __name__ == '__main__': unittest.main()
- Strive to isolate units of code being tested. Tests should not rely on external systems, databases, or services. This isolation allows tests to run faster and with more reliability. Mocking external dependencies can help achieve this goal.
- Aim for comprehensive test coverage, but avoid inconsequential tests. Focus on writing tests for critical functionalities and edge cases to ensure that potential issues are addressed. Use coverage tools to identify untested parts of your code.
- Integrate tests into your workflow. Running tests after every change (e.g., using continuous integration practices) helps catch bugs early and improves overall software quality.
- Just as you refactor your code, regularly review and refactor your tests. Remove redundant tests, simplify complex ones, and ensure they remain relevant as the codebase evolves.
By implementing these best practices, you can enhance the effectiveness of your unit tests, leading to a more robust and maintainable codebase. The clarity and reliability of your tests will ultimately enable faster development cycles and higher confidence in the quality of your software.
Test-Driven Development (TDD) in Python
Test-Driven Development (TDD) is a software development methodology that emphasizes writing tests before writing the corresponding code. TDD can significantly enhance the quality of the software you develop while also improving your productivity. The core principles of TDD revolve around the simple mantra: “Red, Green, Refactor.”
- Start by writing a failing test case for a new feature or functionality. This step ensures that you have a clear understanding of the requirements and the desired behavior of the code.
- Write just enough code to make the test pass. Focus on fulfilling the requirements of the test, avoiding any unnecessary complexity.
- Once the test is passing, clean up the code while ensuring that all tests still pass. Refactoring helps improve code quality and maintainability while keeping the functionality intact.
The TDD cycle can be summarized in these steps:
1. Identify a new feature you want to implement.
2. Write a test case for that feature.
3. Run the test to confirm it fails (expecting a failure signifies that the test is valid).
4. Implement the minimum code necessary to pass the test.
5. Run the tests again to confirm they pass.
6. Refactor the code as needed to improve structure, efficiency, or readability.
7. Repeat the cycle as new features are added.
Let’s illustrate TDD with a simple example by developing a function that checks whether a string is a palindrome.
First, we would write a test case for this function:
import unittest def is_palindrome(s): """Function to check if the given string is a palindrome.""" pass # The implementation will be provided after the test class TestPalindromeFunction(unittest.TestCase): def test_palindrome_with_even_length(self): self.assertTrue(is_palindrome("abba")) def test_palindrome_with_odd_length(self): self.assertTrue(is_palindrome("racecar")) def test_non_palindrome(self): self.assertFalse(is_palindrome("hello")) if __name__ == '__main__': unittest.main()
Running this code will produce failures, as the `is_palindrome` function has not yet been implemented.
Next, we write the minimal code to satisfy the tests:
def is_palindrome(s): return s == s[::-1]
Now, if we run the tests again, they should pass, demonstrating that the function behaves as expected. After confirming that the tests are successful, you may want to improve the implementation or add more tests to cover edge cases.
TDD encourages you to ponder critically about your code and its structure before diving into implementation, leading to better-designed solutions. Further, by developing a comprehensive suite of tests in tandem with your application, you create a safety net that enables the refactoring process and guards against unforeseen bugs.
In practice, teams who adopt TDD often find their codebases more robust due to the clear specifications provided by tests and the disciplined methodology inherent in TDD practices. With continued iterations of the “Red, Green, Refactor” cycle, developers can confidently expand upon existing functionalities while ensuring quality and correctness throughout the development lifecycle.
Common Mistakes and Troubleshooting Tips
When working with unit tests in Python, developers can encounter common mistakes that might lead to ineffective testing or misleading results. Here are several pitfalls to avoid, along with troubleshooting tips to ensure your tests are more reliable and meaningful.
-
- Tests should be simple and focused on one specific aspect of the functionality. If a test tries to cover too much, it can become confusing and difficult to maintain. Aim for tests that validate a single condition or behavior.
- Focusing only on standard use cases can lead to oversights. It’s important to think edge cases, such as empty inputs or extreme values. A robust test suite should account for both normal and exceptional scenarios.
- Using hard-coded values in assertions can make tests brittle and difficult to maintain. Instead, use variables or constants for expected outcomes, enhancing the clarity and flexibility of your tests.
- If your code could raise exceptions, it’s important to include tests that assert this behavior. Use `assertRaises()` to check for specific exceptions and ensure that your functions handle errors as expected.
- Tests should be independent of one another. If the outcome of one test depends on the results of another, it can lead to false positives or negatives. Use setup methods to create isolated environments for each test.
- As your application grows, it’s easy to lose track of which parts are covered by tests. Regularly review test coverage and use tools like `coverage.py` to identify untested areas. This can help ensure thorough testing across your codebase.
- Tests should be run frequently throughout the development cycle. Integrate running tests into your workflow, such as during code reviews or before merging changes, to catch issues early.
Here’s a small example highlighting how to test for exceptions properly:
def divide(a, b): if b == 0: raise ValueError("Cannot divide by zero") return a / b import unittest class TestDivideFunction(unittest.TestCase): def test_divide_by_zero(self): with self.assertRaises(ValueError): divide(10, 0) if __name__ == '__main__': unittest.main()
In this example, the test method test_divide_by_zero
ensures that a ValueError
is raised when attempting to divide by zero. Correctly testing exceptional behavior is important for the robustness of your application.
By being mindful of these common mistakes and implementing the related troubleshooting tips, you can create a more effective testing environment that not only catches bugs but also aids in the long-term maintainability of your code.