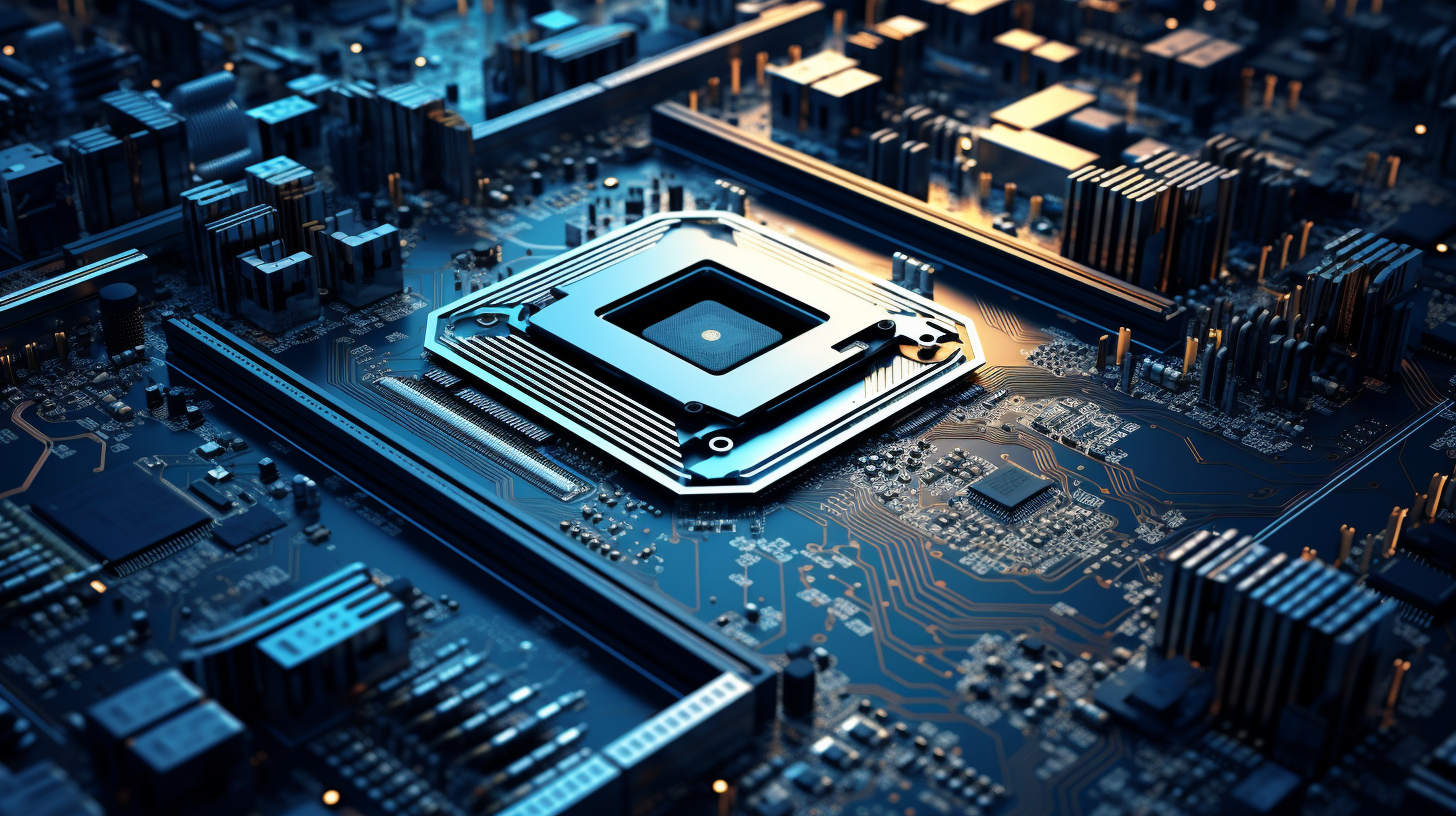
Recursive Queries Using Common Table Expressions
Common Table Expressions (CTEs) are a powerful feature in SQL that enable the definition of temporary result sets that can be referenced within a SELECT, INSERT, UPDATE, or DELETE statement. They contribute to improving the readability and maintainability of complex queries by allowing developers to break down intricate logic into manageable parts.
CTEs can be particularly useful for performing recursive queries, which involve a table that references itself. This can be beneficial in scenarios such as traversing hierarchical data structures, like organizational charts or product categories.
To define a CTE, you use the WITH
clause, followed by a name for the CTE and a query that generates the result set. Here’s the basic syntax:
WITH CTE_Name AS ( SELECT column1, column2 FROM table_name WHERE condition ) SELECT * FROM CTE_Name;
In this syntax:
- A temporary name for the result set generated by the CTE.
- The query that defines the content of the CTE.
- The source from which data is retrieved.
- An optional filtering criterion applied to the data.
CTEs can also be self-referential, allowing recursive behavior. That’s essential for queries that need to process hierarchical data. Each recursive CTE consists of two parts: an anchor member, which is the initial query that terminates the recursion, and a recursive member, which references the CTE itself to build upon the previous results.
Thus, CTEs serve as a versatile tool in SQL, granting developers the ability to simplify complex queries and handle recursive data structures seamlessly.
Types of Recursive Queries
When delving into recursive queries using Common Table Expressions (CTEs), it’s important to recognize the two primary types of recursive queries: the hierarchical query and the iterative query. Each type serves distinct purposes and is best suited for different data retrieval scenarios.
Hierarchical Queries are designed to navigate and retrieve data from tree-like structures, where each record has a parent-child relationship. For instance, ponder an organizational chart where employees report to managers. Hierarchical queries allow you to traverse this structure efficiently, starting from a specific node (like a particular manager) and moving down through their direct reports, all the way to the lowest level of the hierarchy.
Here’s an example of a hierarchical query using a CTE:
WITH EmployeeHierarchy AS ( SELECT EmployeeID, EmployeeName, ManagerID FROM Employees WHERE ManagerID IS NULL -- Anchor member: top-level employees (no managers) UNION ALL SELECT e.EmployeeID, e.EmployeeName, e.ManagerID FROM Employees e INNER JOIN EmployeeHierarchy eh ON e.ManagerID = eh.EmployeeID -- Recursive member ) SELECT * FROM EmployeeHierarchy;
This query starts with the top-level employees (those without managers) and recursively joins the Employees table to find all employees reporting to those identified in the previous step. The result is a complete hierarchy of employees under a specified manager.
Iterative Queries, on the other hand, are typically used for scenarios where you need to perform repetitive calculations or aggregations that depend on previous iterations. This type of recursion works well for cases such as calculating Fibonacci numbers or generating sequences.
Here’s how an iterative recursive query might look:
WITH RECURSIVE Fibonacci AS ( SELECT 0 AS n, 0 AS Value -- Anchor member: Fibonacci(0) UNION ALL SELECT 1 AS n, 1 AS Value -- Anchor member: Fibonacci(1) UNION ALL SELECT n + 1, (SELECT Value FROM Fibonacci WHERE n = f.n - 1) + (SELECT Value FROM Fibonacci WHERE n = f.n - 2) FROM Fibonacci f WHERE n < 10 -- Limiting the number of iterations ) SELECT * FROM Fibonacci;
In this example, the CTE calculates Fibonacci numbers iteratively. The first two Fibonacci numbers are defined as the anchor members, and the recursive member builds upon these to generate the next numbers in the sequence until the desired count is reached.
Understanding the distinction between hierarchical and iterative recursive queries is important for using the full power of CTEs in SQL. Each type allows for structured data retrieval in different contexts, catering to the requirements of your specific application.
Syntax and Structure of Recursive CTEs
To implement recursive Common Table Expressions (CTEs) in SQL, it’s essential to grasp their syntax and structure. A recursive CTE, by definition, comprises two principal components: the anchor member and the recursive member. The anchor member initializes the recursion, establishing the base case that serves as the starting point. The recursive member builds upon the results of the anchor, allowing for repeated execution until a termination condition is met.
The syntax for defining a recursive CTE begins similarly to a standard CTE, with the WITH clause. However, when defining a recursive CTE, the keyword RECURSIVE is often included (though it may not be required in some databases). The anchor member is followed by the UNION ALL operator, which connects to the recursive member of the CTE. This recursive member references the CTE itself, allowing the query to process data iteratively.
WITH RECURSIVE CTE_Name AS ( -- Anchor member SELECT column1, column2 FROM table_name WHERE condition UNION ALL -- Recursive member SELECT column1, column2 FROM table_name t INNER JOIN CTE_Name c ON t.foreign_key = c.primary_key ) SELECT * FROM CTE_Name;
In this structure:
- The designated name for the CTE instance.
- This initial query produces a result set that does not reference the CTE itself. It should ideally return the foundational records that will seed the recursion.
- This operator combines the results of the anchor member with those of the recursive member, allowing the query to grow dynamically.
- This part references the CTE (using CTE_Name), enabling it to build upon the results generated in previous iterations.
The termination of the recursion is controlled by the logic defined in the recursive member’s query, often involving a filtering condition that ensures the recursion halts when no further qualifying records exist. If the termination criteria are not adequately defined, it can lead to infinite recursion, which will ultimately result in an error or performance issues.
Additionally, it is crucial to note that while the structure of recursive CTEs is similar across different database systems, there may be subtle differences in syntax and behavior. Therefore, referring to the specific documentation of the SQL dialect in use is beneficial to understand any nuances that may apply. As you construct recursive queries, keep in mind the importance of maintaining clarity in your logic, as well as performance implications, particularly when dealing with large datasets.
Practical Examples of Recursive Queries
Practical applications of recursive queries using Common Table Expressions (CTEs) can be found in various scenarios, ranging from data hierarchy traversal to complex aggregations. By using the recursive capabilities of CTEs, developers can simplify seemingly convoluted queries, making the SQL code more readable and maintainable.
One common use case is to query hierarchical data structures, such as organization charts or product categories. In this context, you might want to retrieve all employees under a specific manager, regardless of the level in the hierarchy. Below is an example that demonstrates how to achieve this:
WITH RECURSIVE EmployeeTree AS ( SELECT EmployeeID, EmployeeName, ManagerID FROM Employees WHERE EmployeeID = 1 -- Start with a specific manager (e.g., EmployeeID = 1) UNION ALL SELECT e.EmployeeID, e.EmployeeName, e.ManagerID FROM Employees e INNER JOIN EmployeeTree et ON e.ManagerID = et.EmployeeID ) SELECT * FROM EmployeeTree;
In this example, the CTE EmployeeTree starts with a specific employee and recursively retrieves all employees reporting to that individual. This approach can be easily adapted to find employees under different managers by changing the initial condition.
Recursive CTEs can also be beneficial in generating sequences or calculating items like factorials. For instance, if you want to calculate the factorial of a number, you can set up a recursive CTE as follows:
WITH RECURSIVE Factorial(n, result) AS ( SELECT 1 AS n, 1 AS result -- Base case: Factorial(1) = 1 UNION ALL SELECT n + 1, (n + 1) * result FROM Factorial WHERE n < 5 -- Calculate factorial up to 5 ) SELECT * FROM Factorial;
In this example, the CTE generates the factorial for numbers from 1 to 5. The result column accumulates this product as the recursion unfolds, demonstrating how recursive queries can encapsulate complex calculations elegantly.
Moreover, when dealing with XML or JSON data, recursive CTEs can be used to parse and manipulate nested structures. For example, if you have a table storing hierarchical categories, you could extract the full path of each category as follows:
WITH RECURSIVE CategoryPaths AS ( SELECT CategoryID, CategoryName, ParentID, CAST(CategoryName AS VARCHAR(MAX)) AS FullPath FROM Categories WHERE ParentID IS NULL -- Anchor member: top-level categories UNION ALL SELECT c.CategoryID, c.CategoryName, c.ParentID, CAST(cp.FullPath + ' > ' + c.CategoryName AS VARCHAR(MAX)) FROM Categories c INNER JOIN CategoryPaths cp ON c.ParentID = cp.CategoryID ) SELECT * FROM CategoryPaths;
This recursive query retrieves each category along with its full path in the hierarchy, providing valuable insights into the structure of categories. The use of the CAST function ensures that the FullPath is built correctly as the recursion progresses.
These examples illustrate the versatility of recursive CTEs in various contexts, enabling developers to tackle complex data retrieval tasks with ease. By mastering recursive queries, SQL practitioners can enhance their ability to work with hierarchical data, perform intricate calculations, and manipulate nested structures effectively.
Performance Considerations for Recursive CTEs
When working with recursive Common Table Expressions (CTEs), performance considerations are paramount. Recursive queries can quickly become resource-intensive, particularly if the data set is large or the recursion depth is significant. Understanding how to design these queries for optimal performance is essential for maintaining application efficiency and ensuring a smooth user experience.
One of the first factors to consider is the termination of recursion. A well-defined termination condition is important to prevent infinite loops that can lead to excessive resource consumption and ultimately crash the server. The recursive member of the CTE should include conditions that limit the depth of recursion, ensuring that it stops when no further qualifying records exist. For example:
WITH RECURSIVE EmployeeHierarchy AS ( SELECT EmployeeID, EmployeeName, ManagerID FROM Employees WHERE ManagerID IS NULL -- Start with top-level employees UNION ALL SELECT e.EmployeeID, e.EmployeeName, e.ManagerID FROM Employees e INNER JOIN EmployeeHierarchy eh ON e.ManagerID = eh.EmployeeID WHERE e.IsActive = 1 -- Limit to active employees ) SELECT * FROM EmployeeHierarchy;
In this example, adding a condition to filter only active employees not only refines the results but also reduces the workload on the database during recursion. It’s a simple but effective way to enhance performance.
Another key consideration is the size of the result set generated by the recursive query. While CTEs can handle considerable amounts of data, retrieving very large result sets can lead to performance bottlenecks. To mitigate this, it’s advisable to paginate results or limit the number of records returned. Implementing a row count limit can help manage load and improve response times:
WITH RECURSIVE CategoryPaths AS ( SELECT CategoryID, CategoryName, ParentID, CAST(CategoryName AS VARCHAR(MAX)) AS FullPath FROM Categories WHERE ParentID IS NULL UNION ALL SELECT c.CategoryID, c.CategoryName, c.ParentID, CAST(cp.FullPath + ' > ' + c.CategoryName AS VARCHAR(MAX)) FROM Categories c INNER JOIN CategoryPaths cp ON c.ParentID = cp.CategoryID ) SELECT * FROM CategoryPaths LIMIT 100; -- Limit the number of records returned
Additionally, one must consider indexing strategies. Proper indexing on the columns used in the recursive joins can significantly improve performance. For instance, if the recursive CTE frequently accesses a foreign key, ensuring that this column is indexed can reduce lookup times and overall query execution times.
Another performance consideration is the database engine itself. Different SQL databases may have varying implementations of CTEs and recursion handling. Understanding the specific optimizations and limitations of the database in use can provide insights into how to structure recursive queries more effectively. Always consult the database documentation for best practices related to recursive queries.
Lastly, profiling and monitoring the performance of recursive CTEs in a production environment can highlight potential issues before they affect users. Utilize database performance tools to analyze execution plans and identify any bottlenecks in recursive query execution. By continuously monitoring and optimizing these queries, developers can ensure that their applications remain responsive and performant.
Common Pitfalls and Best Practices
When working with recursive Common Table Expressions (CTEs), developers must be aware of common pitfalls that can arise during their implementation, as well as best practices that can help mitigate potential issues. One prevalent challenge is the risk of infinite recursion, which occurs when the termination condition within the recursive member is not appropriately defined. This can lead to excessive resource consumption and potentially crash the database server.
To avoid infinite recursion, it’s critical to establish a robust termination condition. The recursive member should always be designed to stop when no further records match the criteria specified. For instance, if the recursion is intended to traverse a hierarchy, ensure that there is logic in place to prevent the recursive member from repeatedly pulling the same rows. This is often done by incorporating conditions that filter out already processed records:
WITH RECURSIVE OrgChart AS ( SELECT EmployeeID, EmployeeName, ManagerID FROM Employees WHERE EmployeeID = 1 -- Start with a specific employee UNION ALL SELECT e.EmployeeID, e.EmployeeName, e.ManagerID FROM Employees e INNER JOIN OrgChart o ON e.ManagerID = o.EmployeeID WHERE e.EmployeeID NOT IN (SELECT EmployeeID FROM OrgChart) -- Preventing cycles ) SELECT * FROM OrgChart;
Another common pitfall arises from the complexity of the logic contained within recursive queries. As the logic becomes more complicated, it can become difficult to maintain clarity and ensure that the recursion behaves as expected. It is advisable to break down complex recursive queries into simpler, smaller components, or to document the logic thoroughly. This not only enhances readability but also allows for easier debugging when issues arise.
Performance is another area where best practices come into play. Recursive queries can be resource-intensive, especially when processing large datasets. Limiting the number of iterations through appropriate conditions can help optimize performance. Additionally, when dealing with large result sets, ponder using pagination techniques to manage data retrieval effectively:
WITH RECURSIVE ProductCategories AS ( SELECT CategoryID, CategoryName, ParentID FROM Categories WHERE ParentID IS NULL -- Start with top-level categories UNION ALL SELECT c.CategoryID, c.CategoryName, c.ParentID FROM Categories c INNER JOIN ProductCategories pc ON c.ParentID = pc.CategoryID ) SELECT * FROM ProductCategories LIMIT 100; -- Limiting the results to enhance performance
When implementing recursive CTEs, be mindful of indexing. Properly indexing the columns that are frequently accessed in the recursive joins can substantially improve query performance. For instance, if the recursive query involves frequent lookups based on a parent-child relationship, ensure that the foreign keys involved are indexed:
CREATE INDEX idx_ManagerID ON Employees(ManagerID);
It is essential to test recursive queries thoroughly in a variety of scenarios to ensure they handle edge cases effectively. Pay attention to scenarios where the data structure may change, as this can introduce unexpected behavior in recursive logic. Regularly profiling the queries in a production environment can also uncover performance bottlenecks and help maintain an optimal user experience.
By adhering to these best practices, developers can harness the full potential of recursive CTEs while minimizing common pitfalls that often lead to performance issues and logical errors in SQL. The end result is cleaner, more maintainable code that effectively handles complex data structures with ease.