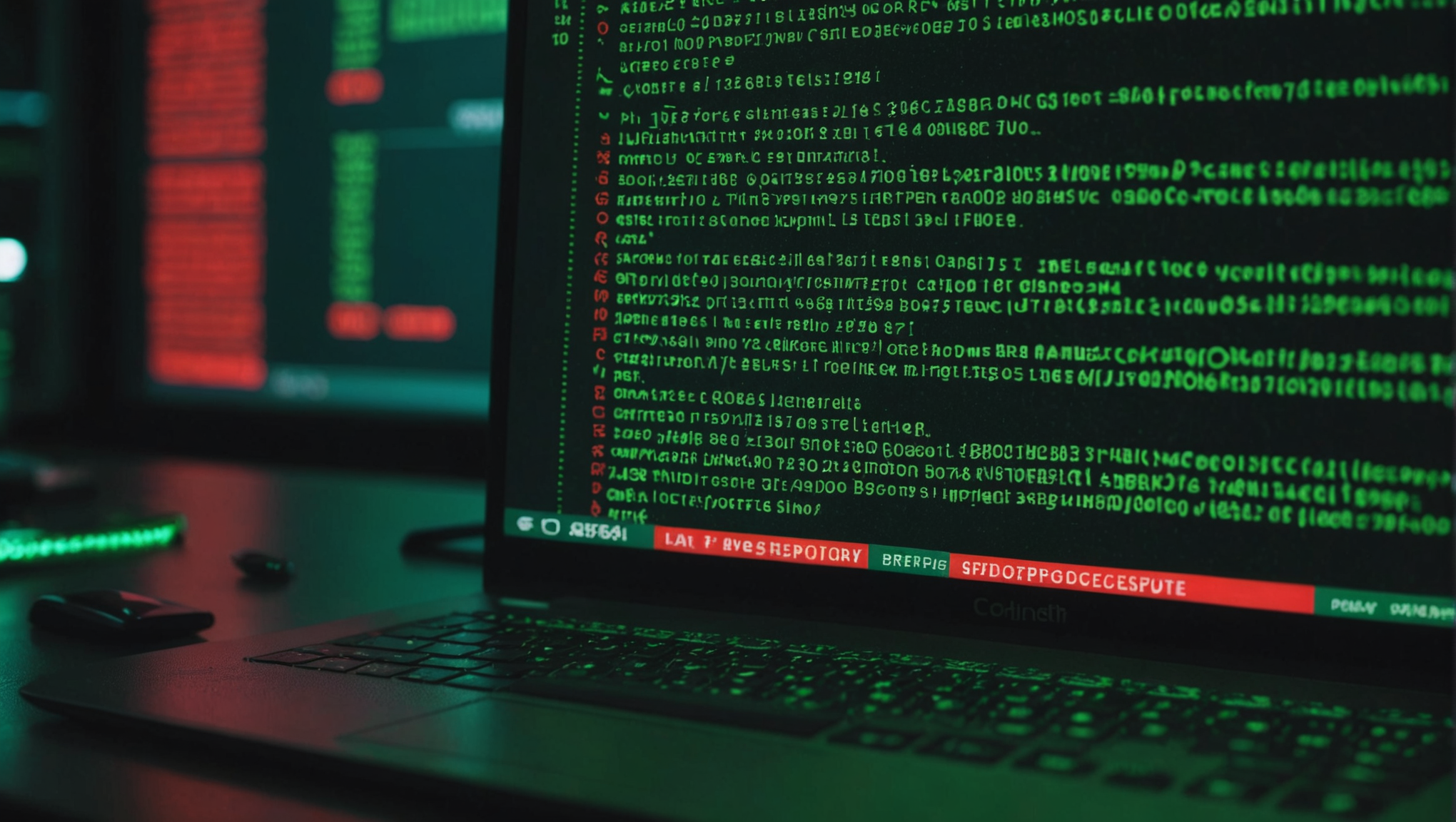
Secure Bash Scripting Practices
When delving into the world of secure Bash scripting, it’s essential to understand the foundational principles that govern safe practices. At its core, secure scripting is about anticipating potential vulnerabilities and employing strategies to mitigate them. The first step in this journey is to grasp the inherent risks associated with script execution.
A common pitfall arises from assuming that scripts will only be executed in controlled environments. However, scripts can be influenced by unintended inputs, which could lead to security breaches. Therefore, it is paramount to practice defensive programming: always assume that inputs might be hostile.
Another critical aspect is maintaining clarity and simplicity in your code. Complex scripts can obscure logic and create pathways for errors or security flaws. Strive to write clear, concise, and well-documented scripts. Employing comments judiciously can greatly enhance the maintainability of your scripts.
Think the following Bash script snippet, which demonstrates how to implement basic security checks by verifying that a required file exists before proceeding:
#!/bin/bash # Check if the required configuration file exists CONFIG_FILE="/etc/myapp/config.conf" if [[ ! -f "$CONFIG_FILE" ]]; then echo "Error: Configuration file not found!" exit 1 fi echo "Configuration file found. Proceeding..."
In this example, the script checks for the existence of a configuration file before executing further commands, thereby preventing potential errors from propagating down the line.
Additionally, it’s vital to set strict execution policies. Ensure that scripts are only run with the necessary permissions and that they operate with the least privilege principle in mind. This limits the impact of any potential vulnerabilities that could be exploited.
By implementing these foundational practices in your Bash scripts, you lay the groundwork for a secure scripting environment. The next logical step is to explore input validation and sanitization techniques to further bolster your script’s resilience against attacks.
Input Validation and Sanitization Techniques
Input validation and sanitization are fundamental practices in the development of secure Bash scripts. They serve as the first line of defense against potential exploits that can arise from malicious or unexpected input. Each piece of data that enters your script should be treated with suspicion; after all, it is not uncommon for scripts to be fed information that can disrupt their intended flow or compromise system security.
In Bash scripting, the importance of validating input cannot be overstated. Unchecked input can lead to command injection vulnerabilities, where an attacker might inject arbitrary commands into a script, leading to unauthorized access or data breaches. To protect against this, you should implement robust validation checks that confirm the input meets your expectations before it’s processed.
One common method of validating input is using conditional statements to check the format and type of the input. For example, when accepting user input for a file path, you might want to ensure that the path provided is not only valid but also points to a file that exists. Here is a simple way to achieve this:
#!/bin/bash # Prompt user for a file path read -p "Enter a file path: " FILE_PATH # Validate the file path if [[ ! -f "$FILE_PATH" ]]; then echo "Error: Invalid file path or file does not exist." exit 1 fi echo "File path is valid. Proceeding..."
In the above example, the script checks if the provided file path corresponds to an actual file. The use of the `-f` flag is important here, as it ensures that the input is not only a valid path but also points to a regular file.
Beyond basic validation, sanitization is equally important. This refers to the process of cleaning input data to eliminate any harmful elements. A common practice in sanitization is to remove unexpected characters from input strings that could be used for malicious purposes. For instance, if your script accepts a username, you might decide to allow only alphanumeric characters:
#!/bin/bash # Prompt user for a username read -p "Enter your username: " USERNAME # Sanitize username by stripping out unwanted characters SANITIZED_USERNAME=$(echo "$USERNAME" | tr -cd '[:alnum:]') if [[ "$USERNAME" != "$SANITIZED_USERNAME" ]]; then echo "Error: Username contains invalid characters." exit 1 fi echo "Username is valid and sanitized."
Here, the `tr` command is employed to strip away any characters that are not alphanumeric, thereby reducing the risk of injection attacks. By comparing the original input with the sanitized version, the script can detect and handle any discrepancies.
To further enhance security, ponder implementing whitelisting instead of blacklisting in your validation strategies. Whitelisting involves defining a set of acceptable inputs, while blacklisting entails identifying disallowed inputs. Whitelisting is typically more effective in ensuring safety, as it clearly delineates what is considered valid.
Furthermore, make extensive use of functions to encapsulate your validation and sanitization logic. This not only improves code readability but also allows you to reuse these checks throughout your script or across multiple scripts. For example:
#!/bin/bash # Function to validate a file path validate_file_path() { if [[ ! -f "$1" ]]; then echo "Error: Invalid file path or file does not exist." return 1 fi return 0 } # Function to sanitize a username sanitize_username() { echo "$1" | tr -cd '[:alnum:]' } # Prompt user for a file path and username read -p "Enter a file path: " FILE_PATH read -p "Enter your username: " USERNAME # Validate and sanitize inputs if ! validate_file_path "$FILE_PATH"; then exit 1 fi SANITIZED_USERNAME=$(sanitize_username "$USERNAME") if [[ "$USERNAME" != "$SANITIZED_USERNAME" ]]; then echo "Error: Username contains invalid characters." exit 1 fi echo "File path is valid and username is sanitized."
By integrating these techniques into your scripts, you can significantly reduce the risk of vulnerabilities caused by unvalidated and unsanitized input. Each layer of validation and sanitization contributes to a more robust defense, making your scripts not only functional but also secure against potential threats.
Proper Use of Quoting and Escaping
Proper use of quoting and escaping in Bash scripting very important for enhancing security and preventing unintended behavior. When variables contain user input or data that can change dynamically, improper handling can lead to command injection, where an attacker manipulates the input to execute arbitrary commands. Therefore, mastering quoting and escaping practices is essential for any secure Bash script.
In Bash, there are three primary types of quoting: single quotes, double quotes, and backticks (or backquotes). Each has its own behavior with respect to variable expansion and special characters.
Single Quotes (”):
When you enclose text in single quotes, the text is treated literally. No variable substitution occurs, and special characters lose their meaning. That is the safest option when you want to ensure that the contents are used exactly as they are.
echo 'This is a literal string: $USER, $(date)'
In the example above, the output will be:
This is a literal string: $USER, $(date)
Double Quotes (“”):
When you use double quotes, variable expansion occurs. That is useful when you want to include variable values in a string, but it also opens the door to injection vulnerabilities if user input is involved. Therefore, always be cautious about what data you allow to be expanded within double quotes.
echo "The current user is: $USER"
Here, the output will be the actual username of the current user, demonstrating how variables are evaluated within double quotes.
Backticks (“):
While backticks are used for command substitution, they can lead to confusion if not handled properly. It is advisable to use the more contemporary $()
syntax, which is clearer and allows for nesting.
echo "The date is: $(date)"
It’s time to discuss the importance of escaping special characters. When dealing with characters that have special meanings (like spaces, dollar signs, and backslashes), you may need to escape them to prevent Bash from interpreting them incorrectly. The backslash () is the escape character in Bash.
For example, if you want to include a dollar sign in a string without triggering variable expansion, you use:
echo "This is a dollar sign: $"
Additionally, when working with user input, it’s essential to ensure proper quoting and escaping to eliminate the risk of command injection. Think a scenario where you are taking a file name as input:
read -p "Enter a file name: " FILE_NAME echo "You entered: $FILE_NAME"
Without proper handling, if a user inputs something like ; rm -rf /
, it could lead to disastrous consequences. Instead, you can use quoting and escaping as follows:
read -p "Enter a file name: " FILE_NAME echo "You entered: "$FILE_NAME""
This ensures that even if the input includes special characters, they will be treated as part of the string rather than as commands. Always prefer using double quotes when expanding variables to safeguard against injection vulnerabilities.
Another best practice is to use printf
instead of echo
for outputting formatted strings. printf
is more predictable since it does not interpret escape sequences unless explicitly told to do so.
printf "You entered: %sn" "$FILE_NAME"
The proper use of quoting and escaping in Bash scripting goes a long way in preserving the integrity of your scripts and protecting them from malicious inputs. By adhering to these practices, you can significantly reduce the risk of command injection and other vulnerabilities that arise from improper handling of strings and user input.
Managing Permissions and Environment Variables
In secure Bash scripting, managing permissions and environment variables is a critical component that significantly impacts the safety and integrity of your scripts. By understanding and implementing the right practices in these areas, you can minimize the risk of unauthorized access and unintentional data exposure.
First and foremost, it’s vital to adhere to the principle of least privilege when executing scripts. This principle dictates that scripts should only have the permissions necessary to perform their designated tasks, and no more. For instance, if a script only needs to read certain files, it should not be given write permissions. Use the chmod
command to set appropriate permissions. Here’s a command to set a script to be readable and executable by the owner only:
chmod 700 /path/to/your/script.sh
With the above command, only the file’s owner can execute and read the script, effectively shielding it from other users.
In addition to setting proper permissions, managing environment variables is equally essential. Environment variables can influence the behavior of a script and should be handled judiciously. It’s common for scripts to use variables like PATH
, HOME
, or custom variables to dictate how commands are executed. Unchecked modifications or exposure of these variables can lead to security vulnerabilities.
To protect your environment variables from being altered or exposed, consider using declare
to restrict their scope. For instance, when defining a variable within a function, you can use:
function my_function { local MY_VAR="sensitive_value" echo "Inside function: $MY_VAR" }
By using local
, MY_VAR
is confined to the function’s scope and cannot interfere with or be accessed outside of it, which enhances the security of your script.
Furthermore, be mindful of how you export variables. When using export
, ensure you’re not unintentionally exposing sensitive information that could be accessed by other processes or users. Here’s how you might export a variable:
export MY_SECRET="12345"
In this case, the variable MY_SECRET
is now accessible to any subprocess spawned from the shell, which could pose a security risk if not handled appropriately. Only export variables that are necessary for the operation of your script and avoid sensitive data where possible.
Another security measure is to unsetting environment variables that are no longer needed. After their purpose is fulfilled, you can use:
unset MY_SECRET
This practice helps to minimize the risk of leaking sensitive data if other scripts or applications inadvertently access the variable.
Additionally, ponder using a secure way to handle sensitive information by reading from secure storage instead of hardcoding credentials into your scripts. For example, you can read passwords from a secure file with restricted permissions:
PASSWORD=$(< /path/to/secure/password.txt)
This approach keeps sensitive information out of your script code, reducing exposure to potential attackers.
Finally, regularly audit your scripts and their associated permissions. Monitor the use of environment variables to ensure that no unexpected changes have occurred. Tools and utilities such as auditd
can help track modifications and access attempts, providing an additional layer of security.
By integrating these practices into your Bash scripting routine, you significantly enhance the overall security of your scripts, ensuring that they operate in a safe, controlled environment while minimizing the risk of unauthorized access and data leaks.
Logging and Monitoring Script Activity
#!/bin/bash # Enable logging for the script exec > >(tee -i /var/log/myscript.log) 2>&1 # Log the start time echo "Script started at: $(date)" # Example command echo "Running some important command..." # Simulating command execution sleep 2 # Log completion echo "Script completed at: $(date)"
Logging and monitoring script activity is a cornerstone of secure Bash scripting practices. By diligently tracking the operations performed by your scripts, you can detect anomalies, identify potential security breaches, and maintain an audit trail for compliance purposes. Implementing effective logging mechanisms not only helps you understand the flow of your script but also aids in troubleshooting and debugging.
To start logging, you can redirect the output of your script to a log file while also displaying it on the console. This dual output can be achieved using the `tee` command, which allows you to send output to both a file and the standard output. Here’s a simple example:
exec > >(tee -i /var/log/myscript.log) 2>&1
In this command, standard output and standard error are both redirected to the log file located at `/var/log/myscript.log`. The `-i` flag ensures that the output is unbuffered, so that you can see the log entries in real-time.
Next, it is essential to log meaningful events throughout your script. This includes logging the start and end times, as well as any significant actions taken. Think the following snippet:
# Log the start time echo "Script started at: $(date)" # Example command echo "Running some important command..." # Simulating command execution sleep 2 # Log completion echo "Script completed at: $(date)"
This example logs the start and end times of the script execution, providing a clear timeline of activity. You should also log any error messages or warnings to make troubleshooting easier. For instance, if a command fails, you can capture its exit status and log an error message:
if ! some_command; then echo "Error: some_command failed with exit status $?" fi
In addition to logging actions, ponder implementing a verbosity level for your logs. This allows you to control the amount of detail in your log output based on your needs. For example, you could define a variable to set the log level:
LOG_LEVEL="INFO" log() { local level="$1" shift if [[ "$level" == "$LOG_LEVEL" || "$LOG_LEVEL" == "DEBUG" ]]; then echo "$(date) [$level] $@" fi } log "INFO" "Script started" log "DEBUG" "Debugging information here"
In this implementation, the `log` function checks the log level before printing messages. You can easily adjust the `LOG_LEVEL` variable to filter the output, allowing for more granular control over what gets logged during execution.
Monitoring script activity is equally important. Keep an eye on your log files and adjust your logging practices as needed. Tools like `logwatch` or custom scripts can help analyze log files and send notifications if certain conditions are met (e.g., repeated errors or suspicious activity).
Incorporating logging and monitoring into your scripts is not just about tracking performance; it is about enhancing security through visibility. By maintaining a clear record of what your scripts do, you can quickly respond to issues as they arise and protect against potential vulnerabilities and unauthorized access.
Common Vulnerabilities and How to Avoid Them
Common vulnerabilities in Bash scripting can leave systems exposed to exploitation, making it vital to identify and mitigate these risks effectively. Understanding how these vulnerabilities manifest in scripts is the first step toward securing your code.
One of the most prevalent vulnerabilities is command injection. This occurs when user input is not properly sanitized or validated, allowing malicious actors to execute arbitrary commands. For example, if a script accepts user input without stringent checks, a user could provide a value that leads to the execution of unintended commands. Ponder a scenario where a script appends user input to a command:
#!/bin/bash # Dangerous command execution read -p "Enter a filename to delete: " FILE_NAME rm $FILE_NAME
In this example, if a user inputs `myfile.txt; rm -rf /`, the script would execute the command to delete the specified file, along with all files in the root directory, due to the lack of input validation. To avoid such vulnerabilities, always validate and sanitize user input before using it within commands.
Another common vulnerability arises from improper handling of environment variables. Environment variables can be manipulated by users or processes, potentially leading to unexpected behavior in a script. For example, if a script uses a variable like `PATH` without checking its contents, an attacker could inject malicious directories, leading to the execution of rogue binaries. Here’s an example of how not to handle it:
#!/bin/bash # Unsafely modifying the PATH export PATH="$PATH:/some/malicious/path"
Instead, ensure that environment variables are defined and used carefully. Ponder explicitly setting a secure `PATH` or using local variables where possible.
In addition to these risks, race conditions can also pose significant threats. A race condition occurs when two processes access shared resources simultaneously, and the outcome depends on the timing of their execution. This can lead to data corruption or unauthorized access. For example:
#!/bin/bash # Potential race condition touch /tmp/myfile chmod 600 /tmp/myfile
If an attacker can access `/tmp/myfile` before the `chmod` command executes, they may exploit this vulnerability. To mitigate race conditions, utilize locking mechanisms or carefully control the sequence of commands.
File permissions represent another crucial aspect of script security. Scripts that run with excessive permissions can be exploited to gain unauthorized access or privileges. For instance, a script with world-writable permissions could allow any user to modify it:
chmod 777 /path/to/script.sh
Instead, apply the principle of least privilege, ensuring that scripts have only the permissions necessary for their operation. Set appropriate permissions:
chmod 700 /path/to/script.sh
Additionally, be vigilant about using external libraries or commands. A common vulnerability arises if scripts depend on outdated or insecure commands. Regularly update your tools and libraries, and verify the integrity of the commands you use. Avoid using commands without validating their sources.
Lastly, always keep error handling in mind. Scripts that fail silently can lead to unnoticed vulnerabilities. Implement proper error handling to ensure that failures are logged and addressed. For example:
#!/bin/bash # Error handling some_command || { echo "Error: some_command failed"; exit 1; }
By recognizing and addressing these common vulnerabilities, you can significantly enhance the security posture of your Bash scripts. Engaging in proactive measures, such as code reviews and security audits, will also help in identifying weaknesses before they can be exploited. By embedding these practices into your scripting routine, you create a more secure environment for your applications and systems.
An important piece to add to this discussion on secure Bash scripting is the importance of using version control systems, like Git, for managing your scripts. Using version control not only aids in tracking changes and rolling back faulty code, but it also enables teams to collaborate safely and maintain an audit trail of modifications over time. Combined with proper logging mechanisms, a version control system can greatly enhance accountability and traceability in script management, ensuring that any introduced vulnerabilities can be quickly identified and addressed.