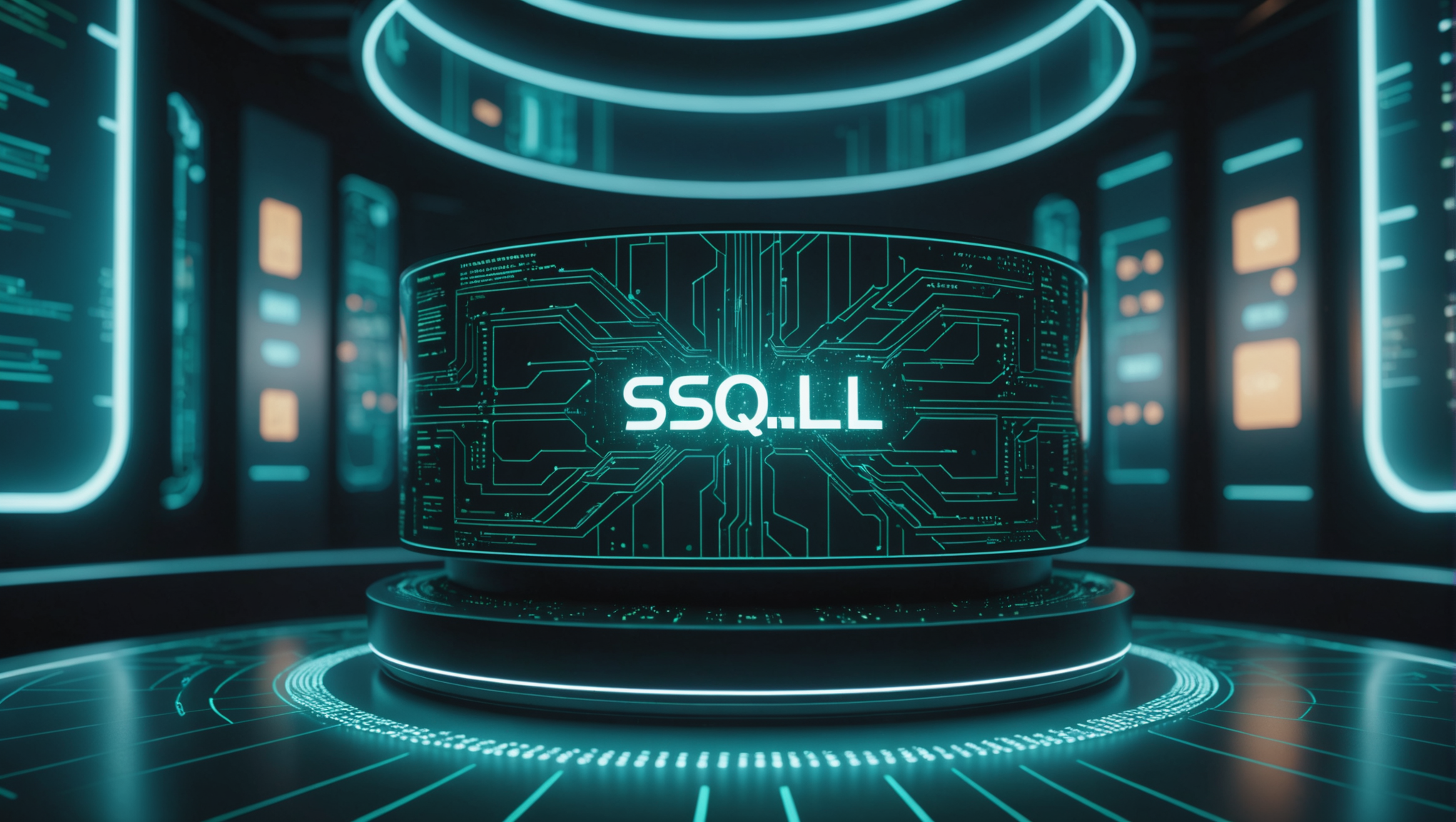
SQL and Blockchain for Data Integrity
At the intersection of contemporary technology lies a fascinating synergy between SQL and blockchain, two powerful paradigms that, when combined, unlock new potentials for data integrity and management. SQL, or Structured Query Language, serves as the backbone for relational databases, allowing for the efficient storage, retrieval, and manipulation of structured data. Its deterministic nature provides a solid foundation for ensuring data consistency, making it a go-to choice for many enterprise applications.
On the other hand, blockchain represents a decentralized ledger that records transactions across multiple computers in a manner that the registered transactions cannot be altered retroactively. This decentralized approach enhances the integrity and security of data, as every participant in the blockchain network has access to the same records, mitigating the risk of data tampering.
SQL databases are primarily centralized, relying on a single point of control to manage data, whereas blockchain operates on a distributed model where multiple parties validate transactions. This fundamental difference gives rise to unique advantages and challenges when integrating SQL with blockchain technologies.
To illustrate these concepts further, let’s ponder a practical example where SQL is used to manage user data in a relational database and blockchain is employed to ensure the integrity of transaction records.
-- Example of SQL table creation for user data CREATE TABLE Users ( UserID INT PRIMARY KEY, UserName VARCHAR(100), Email VARCHAR(100), CreatedAt DATETIME DEFAULT CURRENT_TIMESTAMP ); -- Inserting a new user into the Users table INSERT INTO Users (UserID, UserName, Email) VALUES (1, 'Alice', '[email protected]');
In this SQL example, we create a simple Users table to store user information. Each entry is timestamped upon creation, ensuring we have a record of when the data was added. Now, let’s take a look at how blockchain can be utilized alongside this SQL database.
Imagine integrating a blockchain smart contract that logs each user creation event. This would look something like this in pseudocode:
contract UserRegistry { event UserCreated(uint indexed userId, string userName, string email, uint createdAt); function registerUser(uint userId, string memory userName, string memory email) public { emit UserCreated(userId, userName, email, block.timestamp); } }
In this smart contract, we define an event that will be emitted every time a new user is created. By doing so, we enable a transparent audit trail that can be verified by any parties involved in the blockchain network. The power of this approach lies in the ability to combine the structured storage capabilities of SQL with the immutable and decentralized nature of blockchain.
Understanding the strengths and limitations of each technology very important for using their full potential. SQL excels at handling complex queries efficiently, while blockchain ensures that the underlying data remains tamper-proof. Together, they can form a robust architecture for applications requiring high levels of trust and accountability.
The Role of SQL in Data Management
SQL plays a pivotal role in data management by providing a structured approach to storing, querying, and manipulating data. Its capability to handle complex transactions and relationships makes it indispensable in environments where data integrity, consistency, and accessibility are paramount. SQL databases enable organizations to enforce data constraints, relationships, and referential integrity, thereby ensuring that the data remains accurate and aligned with business rules.
One of the defining features of SQL is its use of tables to organize data into rows and columns, allowing for easy comprehension and management. Each table can represent an entity, such as customers or products, and the relationships between these entities can be effectively modeled through foreign keys. This relational model is not only intuitive but also powerful when it comes to executing complex queries.
For instance, ponder a scenario in an e-commerce application where we need to manage products and orders. We might define two tables: Products and Orders. The Products table could look like this:
CREATE TABLE Products ( ProductID INT PRIMARY KEY, ProductName VARCHAR(255), Price DECIMAL(10, 2), StockQuantity INT );
And the Orders table could be defined as follows:
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, ProductID INT, Quantity INT, OrderDate DATETIME DEFAULT CURRENT_TIMESTAMP, FOREIGN KEY (ProductID) REFERENCES Products(ProductID) );
In this setup, the Orders table references the Products table through a foreign key, which helps maintain the integrity of the data across the two tables. When a new order is placed, you can insert a record into the Orders table while ensuring that the referenced product exists in the Products table. This relationship not only preserves data integrity but also enables complex queries to retrieve meaningful insights, such as total sales per product or inventory levels.
However, while SQL excels in managing structured data, it’s essential to recognize its limitations when it comes to decentralized applications. In scenarios where trust and immutability are critical, integrating blockchain can enhance the overall system. Imagine extending our e-commerce solution by logging each order transaction on a blockchain to ensure its integrity and transparency.
For example, a smart contract could be implemented in a blockchain environment to record each order placed. Pseudocode for such a smart contract might look like this:
contract OrderRegistry { event OrderPlaced(uint indexed orderId, uint indexed productId, uint quantity, uint orderDate); function placeOrder(uint orderId, uint productId, uint quantity) public { emit OrderPlaced(orderId, productId, quantity, block.timestamp); } }
This approach provides a verifiable record of every transaction that can be independently audited, enhancing the trustworthiness of the data managed in the SQL database. The combination of SQL for data management and blockchain for ensuring data integrity creates a robust solution that leverages the strengths of both technologies.
Moreover, SQL’s capability to perform complex analytics on the structured data complements the immutable records stored on the blockchain, allowing businesses to derive insights while maintaining a trustworthy data source. The flexibility of SQL in handling various data types and its rich query language enables applications to meet diverse business needs effectively.
As organizations continue to evolve and adapt to the digital landscape, the role of SQL in data management will remain central, especially when paired with the revolutionary attributes of blockchain technology. This collaboration fosters an environment where data integrity and management can thrive, addressing the challenges of contemporary data landscapes.
Blockchain’s Contribution to Data Integrity
Blockchain’s contribution to data integrity lies in its unique architectural design and operational principles, which prioritize transparency, security, and trust. At its core, blockchain technology utilizes a distributed ledger system where transactions are grouped into blocks and linked together in a chronological chain. Each block contains a cryptographic hash of the previous block, along with a timestamp and transaction data, ensuring that any attempt to alter a block retrospectively would require changes to all subsequent blocks. This immutability is a cornerstone of blockchain’s integrity.
By using consensus mechanisms such as Proof of Work or Proof of Stake, blockchain networks mitigate the risk of fraudulent transactions. Participants in the network must agree on the validity of transactions before they’re added to the chain, creating a robust framework for trust. This decentralized validation process contrasts sharply with traditional data management systems, which often rely on a single point of failure, making them more susceptible to data breaches and inaccuracies.
To further illustrate this, ponder a blockchain-based voting system. Each vote can be recorded as a transaction on the blockchain, ensuring that once a vote is cast, it cannot be altered or deleted. The pseudocode for recording a vote might look like this:
contract VotingRegistry { event VoteCast(uint indexed electionId, uint indexed voterId, uint candidateId, uint timestamp); function castVote(uint electionId, uint voterId, uint candidateId) public { emit VoteCast(electionId, voterId, candidateId, block.timestamp); } }
In this example, the event VoteCast
is emitted every time a vote is placed, creating a transparent and immutable record of the voting process. This application of blockchain technology enhances data integrity by ensuring each vote is independently verifiable while being securely stored within the distributed network.
Moreover, blockchain’s properties extend beyond just data integrity; it also fosters accountability. Since every transaction is time-stamped and linked to its preceding transaction, it creates a clear audit trail that can be easily traced back to its origin. This traceability is particularly valuable in industries such as finance, supply chain management, and healthcare, where regulatory compliance and accountability are paramount.
For example, consider a supply chain scenario where the movement of goods needs to be documented. Each transaction related to a product—from manufacturing to delivery—can be logged on the blockchain, ensuring that every stakeholder has access to the same accurate information about the product’s journey. A simplified version of the smart contract handling the logging of product movement might look like this:
contract SupplyChainRegistry { event ProductShipped(uint indexed productId, string location, uint timestamp); function shipProduct(uint productId, string memory location) public { emit ProductShipped(productId, location, block.timestamp); } }
This not only assures that the data remains tamper-proof but also builds trust among participants in the supply chain, as they can verify each step the product has taken. The integration of such blockchain solutions with SQL databases ensures that while the structured data is efficiently managed, the integrity of critical transactions is uncompromised.
Overall, blockchain’s contribution to data integrity transforms the way organizations approach data management. By combining the strengths of blockchain’s immutability and decentralized validation with SQL’s structured querying capabilities, businesses can create comprehensive solutions that not only secure their data but also enhance transparency and trust across their operations.
Integrating SQL with Blockchain Solutions
Integrating SQL with blockchain solutions presents an exciting frontier for enhancing data integrity and management capabilities in various applications. The challenge lies in marrying the centralized nature of traditional SQL databases with the decentralized architecture of blockchain technology. This integration can provide a seamless experience for users while maintaining the trust and immutability offered by blockchain.
One effective method of integration is to leverage the strengths of both systems: using SQL for transactions and data management while using blockchain for auditable logs and data integrity. This can be achieved by implementing a hybrid architecture where critical events in the SQL database trigger updates in the blockchain. For instance, every time a significant change occurs in the SQL database, such as an insertion, update, or deletion, a corresponding transaction can be recorded in the blockchain.
Think a scenario where we manage an inventory system for a retail application. The SQL database would handle all day-to-day transactions, like tracking product quantities or managing suppliers. When a new product is added to the inventory, a blockchain transaction could simultaneously log this event, ensuring an immutable record. Below is an example of how this might be structured:
-- SQL command to insert a new product INSERT INTO Inventory (ProductID, ProductName, Quantity, SupplierID) VALUES (1, 'Gadget', 100, 2);
At the same time, an event can be emitted in a smart contract on the blockchain to log this action:
contract InventoryRegistry { event ProductAdded(uint indexed productId, string productName, uint quantity, uint timestamp); function addProduct(uint productId, string memory productName, uint quantity) public { emit ProductAdded(productId, productName, quantity, block.timestamp); } }
By invoking the addProduct function from the smart contract right after the SQL insert operation, we effectively create a dual record of the event—one in the SQL database for operational purposes and another in the blockchain for integrity and auditing. This approach provides a solid audit trail while keeping the SQL database responsive to business operations.
Moreover, integrating SQL with blockchain can also be enhanced by using middleware solutions or APIs that facilitate communication between the two systems. This could involve custom-built services that listen for changes in the SQL database and automatically invoke blockchain transactions accordingly. This way, organizations can maintain a consistent and synchronized state between their transactional data and the immutable ledger without manual intervention.
However, while promising, this integration also presents certain challenges. The foremost is the complexity of managing two disparate systems. Organizations must establish robust mechanisms to handle discrepancies and ensure data consistency. Another challenge is ensuring that the performance of the SQL database remains optimal, as frequent interactions with the blockchain can introduce latency or bottleneck conditions.
Performance considerations must be taken into account, especially in high-transaction environments where speed is critical. It’s vital to assess the trade-offs between the immediate access provided by SQL and the secure, albeit slower, updates made to the blockchain.
Integrating SQL with blockchain solutions unlocks significant potential for enhancing data integrity while using the best of both technologies. As organizations navigate these integrations, they can build more robust systems that not only maintain operational efficiency but also uphold the integrity of their data through the unique attributes of blockchain technology.
Use Cases for SQL and Blockchain in Industry
Use cases for SQL and blockchain technology are rapidly emerging across various industries, showcasing the capability of these technologies to imropve data integrity, transparency, and trust. By taking advantage of SQL’s structured data management and the immutable nature of blockchain, organizations can solve complex problems while maintaining a high level of accountability.
One notable application of SQL and blockchain integration can be found in the finance sector. Traditional banking systems rely heavily on centralized databases to manage transactions and customer information. However, with the increasing demand for transparency and security, integrating blockchain technology can transform the way financial institutions operate. For instance, a bank can utilize SQL databases to manage customer accounts and transaction histories while logging every transaction on a blockchain for verification and audit purposes. This dual approach not only enhances data integrity but also builds customer trust.
-- SQL command to create a Transactions table CREATE TABLE Transactions ( TransactionID INT PRIMARY KEY, AccountID INT, Amount DECIMAL(10, 2), TransactionDate DATETIME DEFAULT CURRENT_TIMESTAMP ); -- Inserting a new transaction record INSERT INTO Transactions (TransactionID, AccountID, Amount) VALUES (1, 101, 250.00);
Simultaneously, a blockchain smart contract could be employed to log each transaction:
contract TransactionRegistry { event TransactionExecuted(uint indexed transactionId, uint indexed accountId, uint amount, uint transactionDate); function recordTransaction(uint transactionId, uint accountId, uint amount) public { emit TransactionExecuted(transactionId, accountId, amount, block.timestamp); } }
This integration guarantees that every transaction is recorded on both the SQL database for operational needs and on the blockchain for integrity and verification. Such a system enables banks to provide customers with a reliable record of their transactions while ensuring compliance with regulatory requirements.
Healthcare is another sector where the combination of SQL and blockchain proves advantageous. Patient records must be meticulously managed to ensure accuracy, privacy, and compliance with regulations like HIPAA. Using SQL, healthcare providers can efficiently manage patient data and treatment histories. At the same time, blockchain can secure sensitive patient information, ensuring it is tamper-proof and accessible only to authorized personnel.
-- SQL command to create a PatientRecords table CREATE TABLE PatientRecords ( RecordID INT PRIMARY KEY, PatientID INT, Diagnosis VARCHAR(255), Treatment VARCHAR(255), RecordDate DATETIME DEFAULT CURRENT_TIMESTAMP ); -- Inserting a new patient record INSERT INTO PatientRecords (RecordID, PatientID, Diagnosis, Treatment) VALUES (1, 1001, 'Flu', 'Rest and hydration');
Alongside this, a blockchain solution could track who accesses patient records and when, providing an immutable log of interactions:
contract PatientRegistry { event RecordAccessed(uint indexed recordId, uint indexed patientId, address accessor, uint accessDate); function logAccess(uint recordId, uint patientId) public { emit RecordAccessed(recordId, patientId, msg.sender, block.timestamp); } }
This dual-layered approach not only secures patient data but also fosters trust amongst patients, knowing that their records are protected and access is logged transparently.
In the supply chain industry, the integration of SQL and blockchain can significantly enhance transparency and traceability. Companies can use SQL to manage inventory and supplier relationships while logging each step in the supply chain process on a blockchain. This allows all participants to verify the authenticity of products and their journey through the supply chain.
-- SQL command to create a Shipments table CREATE TABLE Shipments ( ShipmentID INT PRIMARY KEY, ProductID INT, Quantity INT, ShipmentDate DATETIME DEFAULT CURRENT_TIMESTAMP ); -- Inserting a new shipment record INSERT INTO Shipments (ShipmentID, ProductID, Quantity) VALUES (1, 501, 200);
To log the shipment on the blockchain, a smart contract could be utilized:
contract SupplyChainTracker { event ShipmentLogged(uint indexed shipmentId, uint indexed productId, uint quantity, uint shipmentDate); function logShipment(uint shipmentId, uint productId, uint quantity) public { emit ShipmentLogged(shipmentId, productId, quantity, block.timestamp); } }
This system ensures that every shipment is documented in both the SQL database for operational use and on the blockchain for integrity, enabling stakeholders to trace products back to their origin confidently.
These examples highlight just a fraction of the potential use cases for combining SQL and blockchain technologies across various industries. As organizations continue to explore these integrations, the collaborative power of SQL and blockchain is set to redefine data integrity, transparency, and trust in the digital era.
Challenges and Future Trends in Data Integrity Solutions
The challenges and future trends in data integrity solutions reflect a rapidly evolving landscape, characterized by the increasing need for security, transparency, and efficiency. As organizations grapple with the complexities of managing data across various systems, they face several key challenges related to the integration of SQL and blockchain technologies.
One of the primary challenges is the issue of data consistency. In a traditional SQL database, data is managed in a centralized manner, allowing for simpler transactions and queries. In contrast, blockchain’s decentralized nature can complicate how data is synchronized across systems. Maintaining consistency between the transactional SQL database and the immutable records on the blockchain requires robust mechanisms. For instance, if a transaction is recorded in the SQL database but fails to be logged on the blockchain due to network issues or transaction failures, the integrity of the data can be compromised.
-- SQL to ensure consistency before committing to the blockchain BEGIN TRANSACTION; INSERT INTO Transactions (TransactionID, AccountID, Amount) VALUES (2, 102, 150.00); -- Pseudocode for blockchain transaction after SQL insert if (transaction success) { recordTransaction(2, 102, 150.00); } COMMIT;
Another challenge lies in performance. SQL databases are designed for high-speed transactions, which can be hindered by the slower pace of blockchain confirmations. When integrating both systems, organizations must evaluate the trade-offs between the responsiveness required for day-to-day operations and the security benefits offered by blockchain technology. This often necessitates a careful balancing act, where critical operations are performed in SQL, while less frequent, high-stakes transactions are recorded on the blockchain.
Scalability also emerges as a significant concern. While SQL databases can efficiently handle millions of records, blockchain technology is inherently limited by its consensus mechanisms, which can slow down transaction processing as the network grows. Organizations must anticipate future growth and design their systems to accommodate increased transaction volumes without sacrificing performance or security.
Looking toward the future, trends are emerging that may address these challenges. One such trend is the development of hybrid solutions that optimize the strengths of both SQL and blockchain. These systems may include layer-2 solutions that enable faster transaction processing on the blockchain while maintaining a decentralized approach. Innovations in consensus algorithms, such as Proof of Authority or Delegated Proof of Stake, promise to reduce the bottlenecks typically associated with blockchain transactions.
Furthermore, the rise of decentralized finance (DeFi) and blockchain-as-a-service (BaaS) platforms is opening new avenues for integrating SQL with blockchain. As more companies adopt these technologies, the demand for seamless interoperability between SQL databases and blockchain networks will grow, prompting vendors and developers to create more efficient integration tools and APIs.
Additionally, machine learning and artificial intelligence (AI) can play a pivotal role in enhancing data integrity solutions. By using AI algorithms, organizations can analyze transaction patterns, detect anomalies, and improve decision-making processes in real-time. This can lead to more proactive data integrity measures, reducing the likelihood of data breaches or inconsistencies.
While the integration of SQL and blockchain presents numerous challenges, it also paves the way for innovative solutions that can redefine data integrity in the digital age. As organizations explore these technologies and the trends surrounding them, they can create robust systems that not only address current challenges but also position themselves for future success.