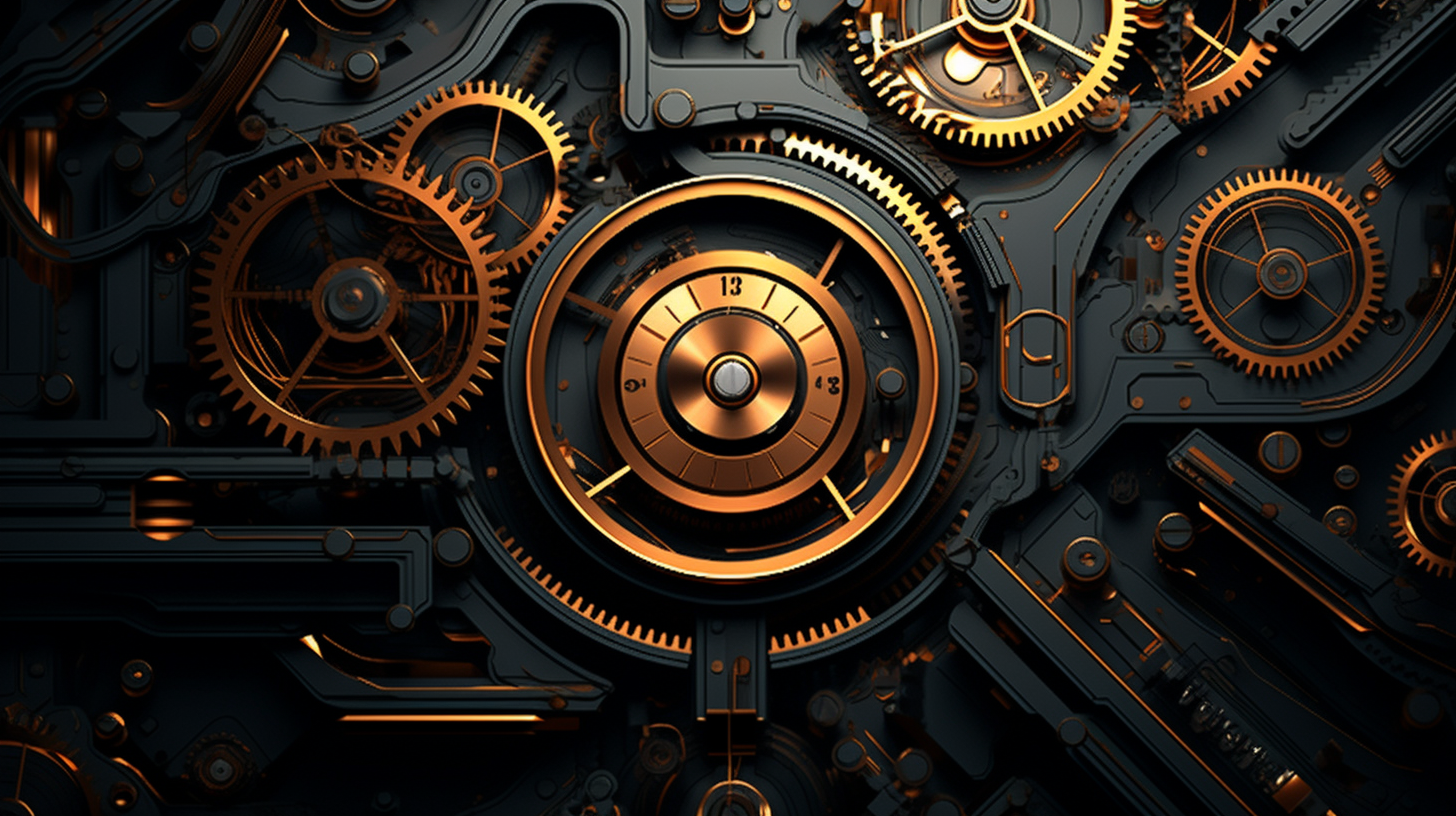
SQL and Constraints for Data Integrity
SQL constraints are rules that can be applied to a database table’s columns to ensure the integrity and accuracy of the data within that table. By imposing these constraints, the database management system can enforce certain conditions on the data, preventing invalid entries and maintaining consistency across the dataset. Understanding SQL constraints especially important for any database developer looking to safeguard data integrity.
Constraints can be defined at the column level or table level, depending on the desired scope of enforcement. When a constraint is violated, the database system will reject the operation that caused the violation, thus preserving the integrity of the data.
Here are some common types of constraints:
- Ensures that a column cannot have a NULL value.
- Ensures that all values in a column are different.
- A combination of NOT NULL and UNIQUE. It uniquely identifies each row in a table.
- Ensures referential integrity by linking a column to the primary key of another table.
- Ensures that all values in a column satisfy a specific condition.
To demonstrate how constraints work, ponder the following SQL code examples:
Creating a table with various constraints:
CREATE TABLE Employees ( EmployeeID INT PRIMARY KEY, FirstName VARCHAR(50) NOT NULL, LastName VARCHAR(50) NOT NULL, Email VARCHAR(100) UNIQUE, DepartmentID INT, FOREIGN KEY (DepartmentID) REFERENCES Departments(DepartmentID), Salary DECIMAL(10, 2) CHECK (Salary > 0) );
In the example above:
- The
EmployeeID
is defined as the primary key, which uniquely identifies each employee. - The
FirstName
andLastName
columns cannot contain NULL values. - The
Email
column must contain unique values. - The
DepartmentID
serves as a foreign key that links to aDepartments
table, enforcing referential integrity. - The
Salary
must be a positive value due to the CHECK constraint.
As you begin to explore SQL constraints, it’s important to recognize how they provide a foundational layer of protection for your data. By implementing these constraints properly, you can ensure that your database remains reliable and that the data adheres to your organization’s standards and requirements.
Types of Constraints in SQL
Within the realm of SQL, constraints are fundamental constructs that govern the behavior of data within tables, ensuring that the stored information remains accurate, consistent, and reliable. Each type of constraint serves a specific purpose and can be leveraged to maintain data integrity across various scenarios.
NOT NULL Constraint
The NOT NULL constraint is one of the simplest yet essential types of constraints in SQL. It prevents the entry of NULL values in a specified column. That is critical in cases where a piece of information is mandatory for the application to function correctly.
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, OrderDate DATE NOT NULL, CustomerID INT NOT NULL );
In the example above, both OrderDate
and CustomerID
are required fields, which means each order must have a specific date and a corresponding customer.
UNIQUE Constraint
The UNIQUE constraint ensures that all values in a column are distinct from one another. This is particularly useful for fields that must hold unique identifiers, like email addresses or social security numbers.
CREATE TABLE Users ( UserID INT PRIMARY KEY, Username VARCHAR(50) UNIQUE, Email VARCHAR(100) UNIQUE );
In the Users
table, the Username
and Email
columns must each contain unique values, preventing duplicate entries that could lead to confusion or data management issues.
PRIMARY KEY Constraint
The PRIMARY KEY constraint is a combination of both NOT NULL and UNIQUE constraints. It designates a column (or a combination of columns) as the unique identifier for a record within the table. This constraint very important for ensuring that each record can be accessed and referenced without ambiguity.
CREATE TABLE Products ( ProductID INT PRIMARY KEY, ProductName VARCHAR(100) NOT NULL );
Here, ProductID
serves as the primary key for the Products
table, ensuring that each product has a distinct identifier that cannot be duplicated.
FOREIGN KEY Constraint
The FOREIGN KEY constraint is pivotal for maintaining referential integrity between tables. It creates a link between the data in two tables, ensuring that a value in one table corresponds to a valid entry in another. This prevents orphan records and promotes consistent data across the database.
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerID INT, FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID) );
In this example, the CustomerID
in the Orders
table must refer to a valid CustomerID
in the Customers
table, thus enforcing a relational constraint between the two entities.
CHECK Constraint
The CHECK constraint allows you to enforce specific conditions that data must meet before it can be entered into a column. That is useful for ensuring that only valid data is stored, based on custom rules defined by the organization.
CREATE TABLE Employees ( EmployeeID INT PRIMARY KEY, Age INT CHECK (Age >= 18) );
In this case, the Age
column has a check constraint that ensures only employees aged 18 and older can be recorded in the Employees
table, thus upholding a standard that aligns with legal employment requirements.
Each of these constraints serves a specific purpose, and when implemented correctly, they provide a robust framework for maintaining data integrity within SQL databases. As you delve deeper into database management, understanding and using these constraints will become an indispensable part of your arsenal, ensuring that your data remains reliable and consistent throughout its lifecycle.
Implementing Constraints for Data Integrity
Implementing constraints for data integrity is a critical aspect of database design and management. The proper use of constraints can significantly enhance the quality and reliability of your data, enforcing rules that prevent erroneous data entry and maintaining consistency across related tables. To implement constraints in SQL effectively, you will often define them during table creation or modify existing tables to add new constraints as necessary.
When creating a new table, constraints can be integrated directly into the CREATE TABLE statement. This approach not only makes the structure of your database clear but also ensures that the constraints are enforced from the moment the table is created.
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, Name VARCHAR(100) NOT NULL, Email VARCHAR(100) UNIQUE, CreatedAt TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
In this example, the Customers table is established with several constraints:
- PRIMARY KEY for CustomerID ensures that each customer can be uniquely identified.
- NOT NULL on the Name column guarantees that every customer has a name, preventing the entry of incomplete records.
- UNIQUE for the Email column ensures that no two customers can have the same email address, which is important for communication and user identification.
Additionally, constraints can be added to existing tables using the ALTER TABLE statement. This flexibility allows for the gradual enhancement of data integrity as business rules evolve or as new requirements arise.
ALTER TABLE Orders ADD CONSTRAINT FK_Customer FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID);
Here, we are adding a foreign key constraint to the Orders table, linking the CustomerID in Orders to the CustomerID in the Customers table. This relationship is essential for ensuring that all orders are associated with valid customer records, thereby promoting referential integrity.
Another important aspect of implementing constraints is understanding how they interact with transactions. If a data modification operation violates a constraint, the entire transaction can be rolled back, preventing any changes that would compromise data integrity. For example, if an attempt is made to insert an order with a CustomerID that does not exist in the Customers table, the database will reject the operation:
INSERT INTO Orders (OrderID, CustomerID, OrderDate) VALUES (1, 999, '2023-10-01'); -- Assuming 999 is not a valid CustomerID
This level of enforcement especially important for maintaining the reliability of data across your database. By implementing constraints judiciously and understanding their implications within your transaction logic, you can create a robust framework that supports accurate and consistent data management.
It’s also worth noting the potential trade-offs when implementing constraints. While they provide essential data integrity checks, excessive constraints can lead to increased complexity and reduced performance, especially in high-transaction environments. As such, a careful balance must be struck between the need for data integrity and the performance characteristics of the database.
Implementing constraints is not just about enforcing rules; it is about fostering an environment where data integrity is a fundamental principle of your database design. By embedding these constraints thoughtfully into your SQL schema, you ensure that your data remains accurate, reliable, and consistent throughout its lifecycle.
Best Practices for Maintaining Data Integrity
Maintaining data integrity is not merely a matter of setting constraints; it involves a comprehensive approach to database design, implementation, and ongoing management. To achieve robust data integrity, here are several best practices to consider:
1. Establish Clear Data Models
Before creating your database, it’s essential to have a well-defined data model. This model should outline the relationships between different entities and define how data flows within your application. By understanding the relationships, you can effectively apply the right constraints to maintain data integrity.
2. Use Constraints Judiciously
While constraints are vital for ensuring data integrity, over-constraining can lead to complications. Each constraint should serve a clear purpose and align with business rules. For instance, using a CHECK constraint to enforce valid ranges on data is beneficial, but avoid excessive constraints that may hinder data entry and application performance.
3. Regularly Review and Update Constraints
As business requirements evolve, the constraints in your database should also be reviewed and potentially updated. Regular audits of your constraints can help identify areas for improvement or adjustment based on new business rules or operational needs.
4. Leverage Transactions
Using transactions effectively can enhance data integrity. Grouping multiple SQL statements into a single transaction ensures that either all changes are committed or none at all. This atomicity prevents the database from ending up in an inconsistent state. For example:
BEGIN TRANSACTION; INSERT INTO Orders (OrderID, CustomerID, OrderDate) VALUES (1, 123, '2023-10-01'); INSERT INTO OrderDetails (OrderDetailID, OrderID, ProductID) VALUES (1, 1, 456); COMMIT;
If any of the above statements fail, you can roll back the entire transaction, ensuring no partial changes are made to the database.
5. Implement Robust Error Handling
Effective error handling mechanisms allow your application to respond gracefully when a constraint violation occurs. Instead of failing silently or crashing, your application should provide meaningful feedback to the user, indicating the nature of the error. For example:
BEGIN TRY INSERT INTO Orders (OrderID, CustomerID, OrderDate) VALUES (1, 999, '2023-10-01'); END TRY BEGIN CATCH SELECT ERROR_MESSAGE() AS ErrorMessage; END CATCH;
This approach not only improves user experience but also aids in diagnosing and resolving issues promptly.
6. Document Your Constraints
Documentation is often overlooked but is important for maintaining data integrity. Clearly documenting the purpose and logic behind each constraint helps other developers understand the database structure and prevents inadvertent changes that could compromise data integrity. This practice is particularly helpful in teams or environments where multiple developers interact with the database.
7. Monitor and Audit Data Quality
Ongoing monitoring of data quality is essential for ensuring that constraints remain effective over time. Regularly auditing your data can help identify anomalies or inconsistencies that may arise from external factors or user errors. Implementing periodic checks can help catch issues before they escalate into significant problems.
8. Train Users and Developers
Training for both end-users and developers on the importance of data integrity and the specific constraints in place can go a long way in maintaining data quality. Users should understand how to input data correctly and the implications of their entries, while developers should be aware of the constraints to avoid inadvertently compromising them during coding.
By following these best practices, you can foster a culture of data integrity within your organization. This proactive approach not only protects the quality of your data but also enhances the overall performance and reliability of your applications.