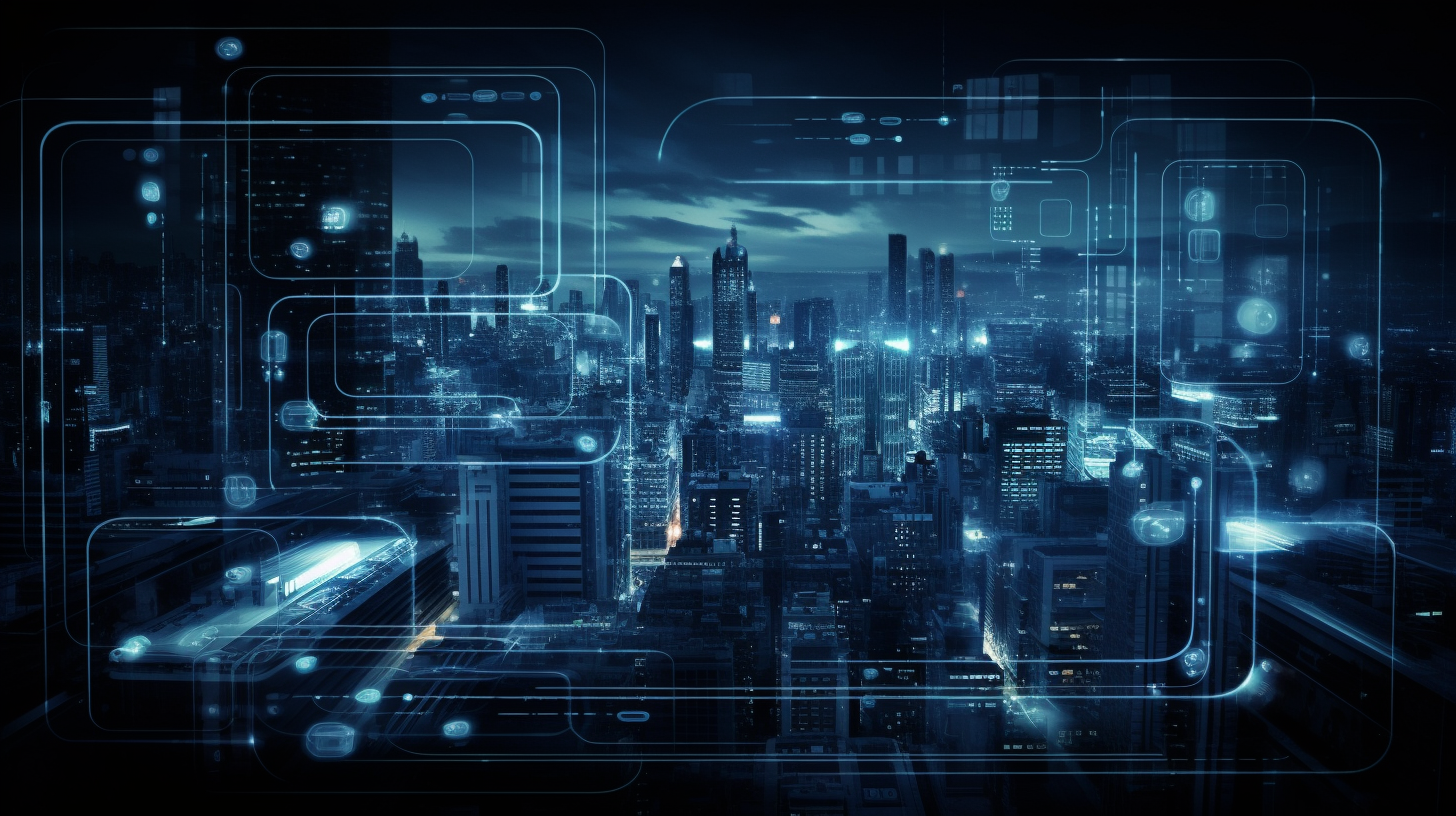
SQL and Date Functions for Time Analysis
In SQL, handling date and time values effectively requires a solid understanding of the various date data types available. Different database systems may implement these data types with slight variations, but the fundamental concepts remain consistent across platforms.
Most relational databases provide a few core date and time types. Here’s a rundown of the commonly used types:
- This type stores the date value without any time component. It typically includes the year, month, and day. For example, a DATE value can be ‘2023-10-01’.
- This type captures the time of day, with precision down to microseconds. It usually omits the date component, storing values like ’14:30:00′ for 2:30 PM.
- This type combines both DATE and TIME, enabling you to store a specific point in time. For instance, ‘2023-10-01 14:30:00’ represents October 1, 2023, at 2:30 PM.
- Similar to DATETIME, but often includes time zone information. It can be used to track changes to records, as it typically updates automatically when this record is created or modified.
- This type represents a duration of time. It can be utilized for performing arithmetic on date and time values, such as adding days or subtracting hours.
Each of these types serves different purposes and very important for various operations. Understanding when to use each type is fundamental for ensuring data integrity and accuracy in your applications.
Here are some SQL code examples that demonstrate the creation of tables with different date types:
CREATE TABLE events ( event_id INT PRIMARY KEY, event_name VARCHAR(100), event_date DATE, event_time TIME, event_datetime DATETIME, event_timestamp TIMESTAMP DEFAULT CURRENT_TIMESTAMP, duration INTERVAL );
When inserting data into a table with date and time values, it’s important to format the input correctly. Here’s an example of how to insert data into the table we just created:
INSERT INTO events (event_id, event_name, event_date, event_time, event_datetime, duration) VALUES (1, 'SQL Workshop', '2023-10-01', '14:00:00', '2023-10-01 14:00:00', INTERVAL '2 hours');
Understanding these data types and their appropriate uses will allow you to handle date and time information more effectively in your SQL queries and operations.
Common SQL Date Functions and Their Usage
SQL provides a suite of built-in date functions that simplify complex date manipulations and calculations. These functions can help developers extract, format, and manipulate date and time values simply. Familiarity with these functions enhances your ability to analyze and interpret time-related data effectively.
Some of the commonly used date functions include:
1. NOW(): This function returns the current date and time based on the server’s time zone settings. It is particularly useful for recording timestamps or for setting default values in tables.
SELECT NOW();
2. CURDATE(): This function retrieves the current date without the time component, making it ideal for queries that focus solely on date values.
SELECT CURDATE();
3. DATE_FORMAT(date, format): This function allows you to format a date value according to a specified format string. That’s useful for presenting dates in a simple to operate manner or for exporting data to external systems.
SELECT DATE_FORMAT(event_date, '%Y-%m-%d') AS formatted_date FROM events;
4. DATEDIFF(date1, date2): This function calculates the difference between two date values and returns the result in days. It is particularly handy for calculating durations or intervals between events.
SELECT DATEDIFF('2023-10-10', '2023-10-01') AS days_difference;
5. ADDDATE(date, INTERVAL expr unit) and SUBDATE(date, INTERVAL expr unit): These functions allow you to add or subtract a specified time interval from a date value. They’re beneficial for calculating future or past dates relative to a given point.
SELECT ADDDATE(event_date, INTERVAL 7 DAY) AS next_week FROM events;
SELECT SUBDATE(event_date, INTERVAL 1 MONTH) AS last_month FROM events;
6. EXTRACT(unit FROM date): This function extracts a specific part of a date, such as the year, month, or day. It is useful for breaking down date components for analysis or reporting purposes.
SELECT EXTRACT(YEAR FROM event_date) AS event_year FROM events;
By using these functions, you can efficiently handle a variety of date-related tasks in your SQL queries. Whether you are analyzing historical data or generating reports for future events, mastering these functions will enhance your capabilities as a SQL developer.
Performing Date Arithmetic for Time Calculations
Once you have a firm grasp on date data types and common functions, the next logical step is to delve into date arithmetic. Date arithmetic encompasses the operations you can perform on date and time values to calculate intervals, determine future or past dates, and analyze durations. SQL’s robust capabilities allow you to manipulate dates effectively, which is important for time-based analyses and reporting.
To start, one of the most simpler operations is adding or subtracting time intervals from a date. You can use the ADDDATE
and SUBDATE
functions, which allow you to add or subtract a specified interval to a date. That’s particularly handy when scheduling events or determining deadlines. For instance, if you want to find out the date one week from a given event date, you could execute the following:
SELECT ADDDATE(event_date, INTERVAL 7 DAY) AS next_week FROM events;
Conversely, if you need to trace back to a previous date, you could use:
SELECT SUBDATE(event_date, INTERVAL 10 DAY) AS last_week FROM events;
In addition to simple additions and subtractions, you can also perform more complex arithmetic using the DATEDIFF
function. This function computes the difference between two dates in days, which can be incredibly useful for reporting durations or time intervals between events. For example, if you need to calculate how many days are left until an event:
SELECT DATEDIFF(event_date, CURDATE()) AS days_until_event FROM events;
Another useful function is TIMESTAMPDIFF
, which works similarly to DATEDIFF
but allows you to specify the unit of time you wish to measure (such as seconds, minutes, hours, etc.). Here’s how you can measure the duration between two timestamps in hours:
SELECT TIMESTAMPDIFF(HOUR, event_datetime, NOW()) AS hours_since_event FROM events;
This ability to perform arithmetic on dates can be expanded with the use of the INTERVAL
keyword, allowing for a variety of units such as YEAR, MONTH, DAY, HOUR, MINUTE, and SECOND, allowing you to tailor your calculations to the specific requirements of your analysis.
For instance, if you wanted to calculate a future date that is three months from the current date:
SELECT ADDDATE(CURDATE(), INTERVAL 3 MONTH) AS future_date;
By mastering these arithmetic operations, you can enhance the temporal analysis capabilities of your SQL queries, ensuring you can derive meaningful insights from time-based data. With date arithmetic at your disposal, you can effectively address business needs related to scheduling, compliance, and reporting, making you a more proficient SQL developer within the scope of time analysis.
Filtering and Sorting Data Using Date Criteria
Filtering and sorting data based on date criteria is an important aspect of SQL querying, especially when dealing with datasets that include time-sensitive information. By applying the right filters and sort orders, you can quickly retrieve the relevant records needed for analysis, reporting, or decision-making. This section will explore various techniques you can employ to effectively filter and sort date data in SQL.
One of the most fundamental methods of filtering data by date is using the WHERE clause to specify conditions that date values must meet. For instance, if you want to find all events that occur after a certain date, you can execute a query like the following:
SELECT * FROM events WHERE event_date > '2023-10-01';
This query retrieves all events scheduled for dates after October 1, 2023. You can also employ logical operators to combine multiple conditions. For example, to filter events happening within a specific date range, you can use the BETWEEN operator:
SELECT * FROM events WHERE event_date BETWEEN '2023-10-01' AND '2023-10-31';
In this case, the query will return events that occur throughout October 2023. Additionally, using the AND operator allows for more complex filtering. For instance, if you want to find events that are not only scheduled for October but also start after 2 PM, you could write:
SELECT * FROM events WHERE event_date BETWEEN '2023-10-01' AND '2023-10-31' AND event_time > '14:00:00';
Sorting your results by date can enhance readability and aid in analysis. The ORDER BY clause allows you to arrange your result set in ascending or descending order based on one or more columns. For example, to list all events sorted by their dates in ascending order, you can run the following:
SELECT * FROM events ORDER BY event_date ASC;
If you wish to sort the events in descending order, simply replace ASC with DESC:
SELECT * FROM events ORDER BY event_date DESC;
Combining filtering and sorting in a single query can provide you with a compact and relevant result set. For instance, if you want to find events occurring in the past month and sort them by their event date in descending order, your query would look like this:
SELECT * FROM events WHERE event_date > SUBDATE(CURDATE(), INTERVAL 1 MONTH) ORDER BY event_date DESC;
This query retrieves all events that have occurred in the last month, sorting them from the most recent to the oldest. By mastering these filtering and sorting techniques, you can effectively manipulate date data to suit your analysis needs, providing insights that drive informed decisions.
Advanced Techniques for Time Zone Management in SQL
When it comes to managing time zones in SQL, the challenge often lies in reconciling the differences in how various systems store and interpret time values. As businesses increasingly operate across multiple time zones, having a robust strategy for time zone management becomes essential. SQL offers several advanced techniques to work with time zones, enabling developers to ensure accuracy and consistency in date and time data.
Many relational databases, such as PostgreSQL and MySQL, allow you to store timestamp values with time zone information. This capability especially important for applications that need to display time-related data accurately based on the user’s location or a specific time zone. To create a table that includes a timestamp with time zone, you might use:
CREATE TABLE user_sessions ( session_id INT PRIMARY KEY, user_id INT, session_start TIMESTAMP WITH TIME ZONE, session_end TIMESTAMP WITH TIME ZONE );
Inserting data into this table also requires understanding how to specify time zone information. For example:
INSERT INTO user_sessions (session_id, user_id, session_start, session_end) VALUES (1, 101, '2023-10-01 14:00:00-05', '2023-10-01 16:00:00-05');
Here, the ‘-05’ indicates that the time is in the UTC-5 time zone. This capability ensures that all timestamps are stored consistently, maintaining their context for later retrieval and analysis.
To convert time zone values, SQL provides functions to alter timestamps according to specific time zones. For instance, in PostgreSQL, you can use the AT TIME ZONE clause to convert a timestamp from one time zone to another:
SELECT session_start AT TIME ZONE 'UTC' AS utc_time FROM user_sessions;
This query will convert the session_start timestamp to UTC, enabling comparison across different time zones. Similarly, you can adjust the output for a different time zone:
SELECT session_start AT TIME ZONE 'America/New_York' AS ny_time FROM user_sessions;
Understanding the context of time zones is essential for accurate reporting. The CONVERT_TZ function in MySQL serves a similar purpose, which will allow you to convert a datetime value from one time zone to another. For example:
SELECT CONVERT_TZ(session_start, 'UTC', 'America/New_York') AS session_ny_time FROM user_sessions;
Moreover, if you need to find records that fall within a specific time range adjusted for a certain time zone, you can combine the filtering with time zone conversion. For instance:
SELECT * FROM user_sessions WHERE session_start AT TIME ZONE 'UTC' BETWEEN '2023-10-01 00:00:00' AND '2023-10-31 23:59:59';
In addition to conversions, handling daylight saving time (DST) changes is another crucial aspect of time zone management. Many databases automatically handle DST adjustments when using time zone-aware types, but it’s always prudent to test and verify this behavior. For example, PostgreSQL provides the functionality to automatically adjust for DST when you specify a time zone that observes these changes.
Effective time zone management in SQL encompasses the proper use of timestamp data types, conversion functions, and careful handling of DST. By incorporating these techniques, you can ensure that your applications handle date and time data accurately, regardless of the geographical context in which they operate.