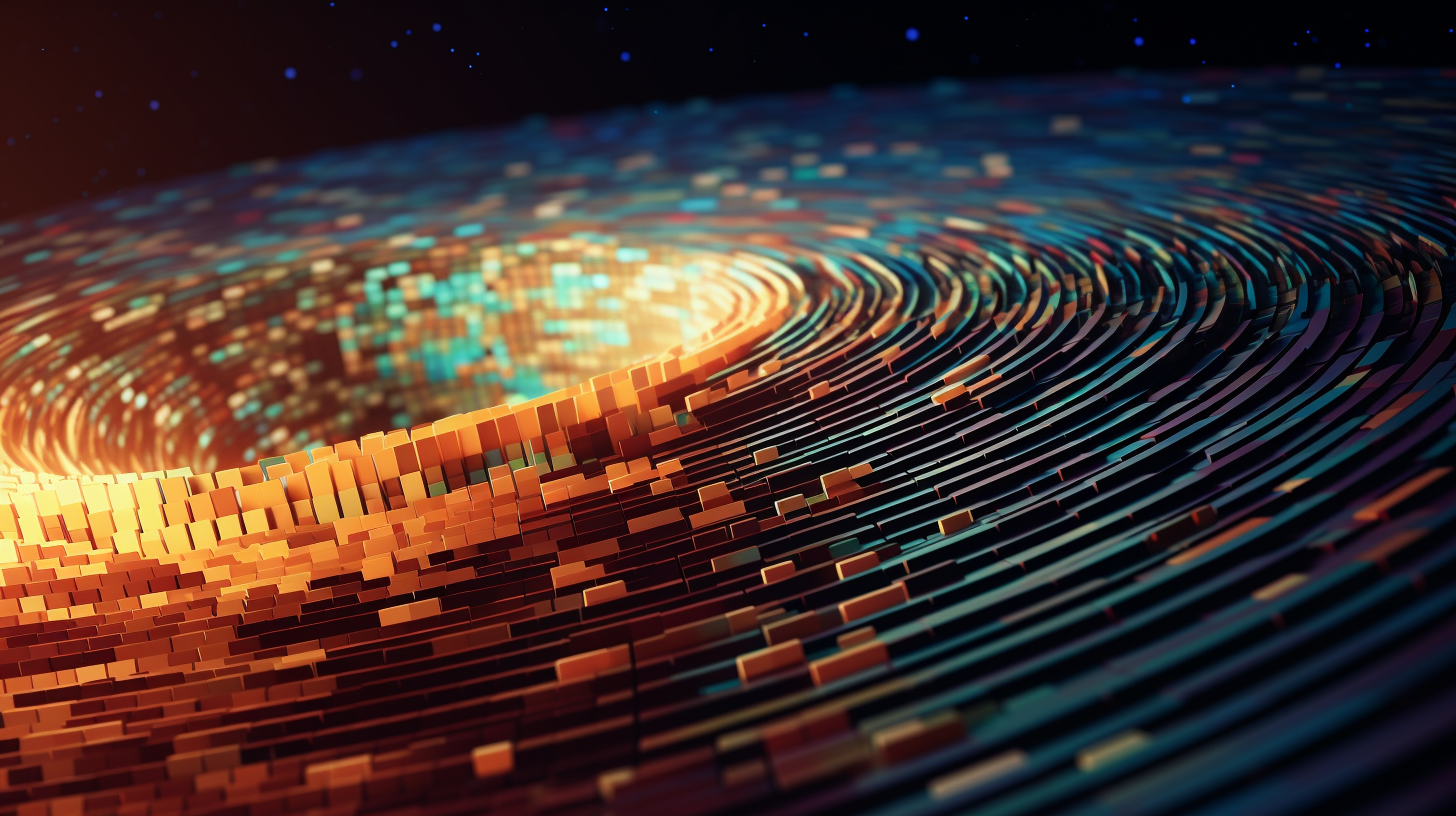
SQL and Functions for Data Transformation
Data transformation in SQL is the process of converting data from one format or structure into another, facilitating analysis and decision-making. It is an essential aspect of data handling that helps ensure data quality, integrity, and usability. The transformation process often involves cleaning, aggregating, and reshaping data to suit analytical needs.
Understanding how to manipulate data effectively within SQL is foundational for any data professional. SQL provides various built-in functions and techniques that streamline the transformation process. Here, we delve into the significance of data transformation and its practical application in SQL.
Think a scenario where you need to analyze sales data from multiple regions. The raw data may come in different formats or contain inconsistencies that can obscure insights. Using SQL functions, you can standardize this data, making it more coherent and ready for analysis.
Key components of data transformation include:
- Removing duplicates, correcting errors, and ensuring data integrity.
- Converting data types or structures, such as changing date formats or string casing.
- Summarizing data to obtain meaningful insights, such as total sales per region.
- Selecting only relevant records based on specific criteria.
For example, to clean and format a dataset containing sales records, you might use the following SQL code:
SELECT DISTINCT UPPER(customer_name) AS customer_name, TRIM(order_date) AS order_date, ROUND(total_amount, 2) AS total_amount FROM sales_data WHERE total_amount > 0;
This example demonstrates how SQL functions like UPPER()
, TRIM()
, and ROUND()
are used for data transformation. The UPPER()
function standardizes customer names to uppercase, TRIM()
removes any extraneous spaces from order dates, and ROUND()
formats the total amounts to two decimal places.
Moreover, transforming data isn’t just about cleaning; it’s also about reshaping it for further analysis. For instance, if you wanted to aggregate sales data by month, you could use the following SQL code:
SELECT DATE_TRUNC('month', order_date) AS month, SUM(total_amount) AS total_sales FROM sales_data GROUP BY month ORDER BY month;
In this example, the DATE_TRUNC()
function is utilized to group sales by month, while SUM()
aggregates the total sales for each month. This illustrates how transformation can provide deeper insights into sales trends over time.
Common SQL Functions for Data Manipulation
When it comes to manipulating data within SQL, a variety of functions are available that allow users to perform essential tasks efficiently. These functions help standardize, clean, and transform data into a more usable format, enhancing its quality and making it suitable for analysis. Below, we explore some common SQL functions that play an important role in data manipulation.
One of the most frequently used functions is the CASE statement, which allows for conditional logic in SQL queries. This function can simplify complex data transformation by allowing you to create new columns based on specific conditions. For example, if you want to categorize sales based on their amounts, you could use:
SELECT order_id, total_amount, CASE WHEN total_amount < 100 THEN 'Low' WHEN total_amount BETWEEN 100 AND 500 THEN 'Medium' ELSE 'High' END AS sales_category FROM sales_data;
The CASE statement categorizes each order based on the total amount, providing a clearer insight into sales distribution.
String manipulation functions are also vital. The CONCAT function, for example, can be used to combine multiple strings into one. That is particularly useful when you want to present data in a more readable format. Consider an example where you want to create a full name column from separate first and last name columns:
SELECT CONCAT(first_name, ' ', last_name) AS full_name FROM customers;
In this case, CONCAT merges the first and last names with a space in between, making it easier to read and analyze customer data.
Another commonly used function is COALESCE, which returns the first non-null value in the list of provided arguments. This function proves to be immensely helpful in dealing with missing data. For example:
SELECT order_id, COALESCE(discount, 0) AS discount_amount FROM orders;
Here, if the discount value is null, it will return 0 instead, ensuring that calculations involving discounts do not break due to null values.
Moreover, SQL provides functions for modifying date and time data, such as EXTRACT and NOW(). These functions facilitate the analysis of time-based data. For instance, you can extract the year from a date to analyze yearly trends:
SELECT EXTRACT(YEAR FROM order_date) AS order_year, COUNT(order_id) AS total_orders FROM orders GROUP BY order_year;
This query allows you to count the total orders per year, aiding in trend analysis and forecasting.
Using Aggregate Functions for Summarization
Aggregate functions in SQL are pivotal for summarizing large datasets, enabling analysts to extract significant insights quickly. These functions operate on multiple rows of data and produce a single result, making them indispensable for data transformation tasks such as generating reports or analyzing trends. The most common aggregate functions include COUNT(), SUM(), AVG(), MIN(), and MAX().
For instance, if you are tasked with determining the total sales from a dataset containing numerous transactions, the SUM() function serves this purpose adeptly. Consider the following SQL query:
SELECT SUM(total_amount) AS total_sales FROM sales_data;
This query will return the total sales amount by summing up the “total_amount” column from the sales_data table. The simplicity of this function belies its power; it allows analysts to obtain crucial summaries from large datasets with ease.
Another essential aggregate function is COUNT(), which counts the number of rows that meet a specified condition. This can be particularly useful for understanding how many transactions occurred within a specific timeframe or category. An example query using COUNT() might look like this:
SELECT COUNT(order_id) AS total_orders FROM sales_data WHERE order_date >= '2023-01-01';
In this case, the COUNT() function counts all orders placed since the beginning of 2023, providing a quick overview of sales activity in that period.
Average calculations are often critical for analyzing performance metrics, and the AVG() function allows analysts to compute the mean of a set of values efficiently. To find the average order value, you could use the following SQL statement:
SELECT AVG(total_amount) AS average_order_value FROM sales_data;
This query computes the average total amount of all transactions, offering insights into typical customer spending.
Furthermore, the MIN() and MAX() functions are invaluable for identifying extremes in your data. For instance, if you want to find the smallest and largest order amounts, the queries would look like this:
SELECT MIN(total_amount) AS lowest_order, MAX(total_amount) AS highest_order FROM sales_data;
These functions help in understanding the range of customer transactions and can inform business strategies related to pricing and inventory management.
Aggregate functions can also be combined with the GROUP BY clause to segment summaries into meaningful categories. For example, if you wish to analyze total sales by product category, you can do so with a query like this:
SELECT product_category, SUM(total_amount) AS total_sales FROM sales_data GROUP BY product_category ORDER BY total_sales DESC;
This query groups sales data by product categories and sums the total sales for each category, presenting a clear view of which categories are performing best.
String Functions for Formatting and Cleaning Data
String functions in SQL are essential for formatting and cleaning data, providing the tools necessary to manipulate text and ensure consistency across datasets. These functions can transform raw data into a more simple to operate format, which is important for effective analysis and reporting. By using string functions, data professionals can clean up inconsistencies, remove unwanted characters, and format strings to meet specific requirements.
One of the most commonly used string functions is SUBSTRING(), which allows users to extract a portion of a string based on specified starting positions and lengths. This function is particularly useful when dealing with concatenated data or when specific segments of a string need to be analyzed. For example, if you have a column containing full addresses and you want to extract just the zip code, you could implement:
SELECT SUBSTRING(address, LENGTH(address) - 4, 5) AS zip_code FROM customer_addresses;
In this example, LENGTH() is used to determine where the zip code starts, and SUBSTRING() extracts the last five characters, assuming the zip code is always at the end of the address string.
Another vital function is REPLACE(), which allows for the substitution of specific characters or substrings within a string. This function is particularly helpful for cleaning data by removing unwanted characters or correcting common entry errors. For instance, if you need to replace all instances of “N/A” with NULL in a dataset of customer feedback, you could write:
SELECT REPLACE(feedback, 'N/A', NULL) AS cleaned_feedback FROM customer_feedback;
This query searches the feedback column for “N/A” and replaces it with NULL, resulting in cleaner dataset entries ready for analysis.
String manipulation functions also include LOWER() and UPPER(), which are invaluable when standardizing text data. Consistency in casing can significantly affect data integrity. For instance, if you want to ensure all email addresses are in lowercase for accurate comparisons, you could use:
SELECT LOWER(email) AS standardized_email FROM users;
This ensures that all email addresses are stored in a consistent format, eliminating issues when performing queries based on email addresses.
Additionally, the TRIM() function is essential for removing leading and trailing spaces from strings, which can often cause problems during data comparison or reporting. To clean up user input data such as usernames, you could execute:
SELECT TRIM(username) AS cleaned_username FROM user_accounts;
This ensures that any extra spaces added during data entry do not interfere with user authentication processes or reports. String functions can also be combined for complex transformations. For example, if you wanted to concatenate first and last names into a full name while ensuring proper casing, your query might look like this:
SELECT CONCAT(UPPER(LEFT(first_name, 1)), LOWER(SUBSTRING(first_name, 2)), ' ', UPPER(LEFT(last_name, 1)), LOWER(SUBSTRING(last_name, 2))) AS full_name FROM customers;
This query utilizes multiple string functions to format first and last names correctly, ensuring that they’re displayed with the first letter capitalized and the remainder in lowercase.
Date and Time Functions for Temporal Analysis
Date and time functions are critical components of SQL that enable the analysis of temporal data, allowing users to manipulate and extract meaningful insights from date and time stamps. These functions are designed to perform operations such as extracting parts of dates, calculating differences, and formatting date values, which are essential for time-based analysis. Understanding how to leverage these functions can significantly enhance your ability to work with time series data, historical trends, and temporal patterns.
A fundamental function in SQL for date manipulation is NOW(), which retrieves the current date and time. This can be particularly useful when you want to compare or calculate time intervals against the current moment. For instance, to insert a new record with the current timestamp, you might use:
INSERT INTO orders (customer_id, total_amount, order_date) VALUES (1, 150.00, NOW());
Additionally, SQL provides the DATE_TRUNC() function, which is invaluable for aggregating data by specific time intervals, such as days, months, or years. This function allows you to truncate a date to a given precision, making time-based grouping simpler. For example, if you want to analyze sales data aggregated by month, you could execute:
SELECT DATE_TRUNC('month', order_date) AS month, SUM(total_amount) AS total_sales FROM sales_data GROUP BY month ORDER BY month;
This query groups the sales data by month, summing the total sales for each period, thus revealing trends over time.
Another useful function is EXTRACT(), which allows you to retrieve specific parts from a date or timestamp. For instance, if you’re interested in analyzing the distribution of orders by year, you can use:
SELECT EXTRACT(YEAR FROM order_date) AS order_year, COUNT(order_id) AS total_orders FROM sales_data GROUP BY order_year ORDER BY order_year;
Here, EXTRACT(YEAR FROM order_date) isolates the year from each order date, enabling an efficient count of orders per year.
SQL also offers the DATEDIFF() function, which computes the difference between two dates, returning the result in days. This can be particularly useful for calculating the duration between events. For example, to find out how many days it took for orders to be delivered after the order date, you might write:
SELECT order_id, DATEDIFF(delivery_date, order_date) AS days_to_delivery FROM orders;
This query provides insights into delivery efficiency by calculating the number of days between the order and delivery dates.
Formatting dates is also a common requirement, and SQL provides the TO_CHAR() function for this purpose. TO_CHAR() allows you to convert date values into a more readable string format. For instance, if you wish to display order dates in a ‘MM-DD-YYYY’ format, you could use:
SELECT order_id, TO_CHAR(order_date, 'MM-DD-YYYY') AS formatted_date FROM orders;
This converts the order dates into a standard format, enhancing readability in reports and output.
Lastly, SQL’s INTERVAL functionality is instrumental for performing arithmetic operations on dates. If you need to add or subtract a specific time period from a date, you can achieve this easily. For example, to find out what the date will be 30 days after an order was placed, you might write:
SELECT order_id, order_date + INTERVAL '30 days' AS due_date FROM orders;
Best Practices for Efficient Data Transformation in SQL
When it comes to executing efficient data transformations, adhering to best practices in SQL can significantly enhance both performance and maintainability. The goal is to ensure that your SQL queries are not only correct but also optimized for speed and resource utilization. Here are several key strategies to consider when performing data transformations.
1. Optimize Your Queries
One of the most effective ways to enhance performance is to avoid unnecessary computations. When writing SQL queries, it is crucial to select only the columns you need rather than using SELECT * to fetch all available data. For instance:
SELECT customer_id, total_amount FROM sales_data;
This approach minimizes data retrieval time and reduces memory usage, which is especially important with large datasets.
2. Use Proper Indexing
Indexing is a powerful technique to enhance the speed of data retrieval operations. By creating indexes on columns frequently used in WHERE clauses or JOIN conditions, you can significantly boost query performance. For instance:
CREATE INDEX idx_customer_id ON sales_data(customer_id);
Having an index on customer_id can accelerate searches involving this field, leading to faster data transformations when filtering or joining tables.
3. Leverage Temporary Tables
In complex transformations involving multiple steps, ponder using temporary tables. Temporary tables can store intermediate results, which can be useful for breaking down large queries into smaller, more manageable units. For example:
CREATE TEMPORARY TABLE temp_sales AS SELECT customer_id, SUM(total_amount) AS total_spent FROM sales_data GROUP BY customer_id;
This allows subsequent transformations to be simpler and can improve overall performance by reducing the complexity of the final query.
4. Avoid Cursors When Possible
Cursors can be slow and resource-intensive because they process each row one at a time. Instead, strive to use set-based operations wherever possible. For example, instead of using a cursor to update records individually, you can perform batch updates, as shown here:
UPDATE sales_data SET total_amount = total_amount * 1.1 WHERE order_date < '2023-01-01';
This single operation is more efficient than looping through each row with a cursor.
5. Use Aggregate Functions Wisely
When performing aggregations, always ensure you use the appropriate GROUP BY clauses to minimize the amount of data processed. For instance, when calculating average sales by month, use:
SELECT DATE_TRUNC('month', order_date) AS month, AVG(total_amount) AS average_sales FROM sales_data GROUP BY month;
This reduces the data volume and optimizes processing time.
6. Monitor Query Performance
Regularly check the performance of your SQL queries using tools like execution plans or query analyzers. These tools can reveal inefficiencies in your queries, enabling you to make informed adjustments. For instance, if a particular join operation is taking longer than expected, you may need to revise the indexing strategy or rewrite the query.
7. Document Your Queries
Documentation is a critical aspect of best practices. Clearly comment on your SQL code to explain the rationale behind complex transformations. This not only aids in your understanding but is also invaluable for future maintenance, especially when working in teams or revisiting code after some time.