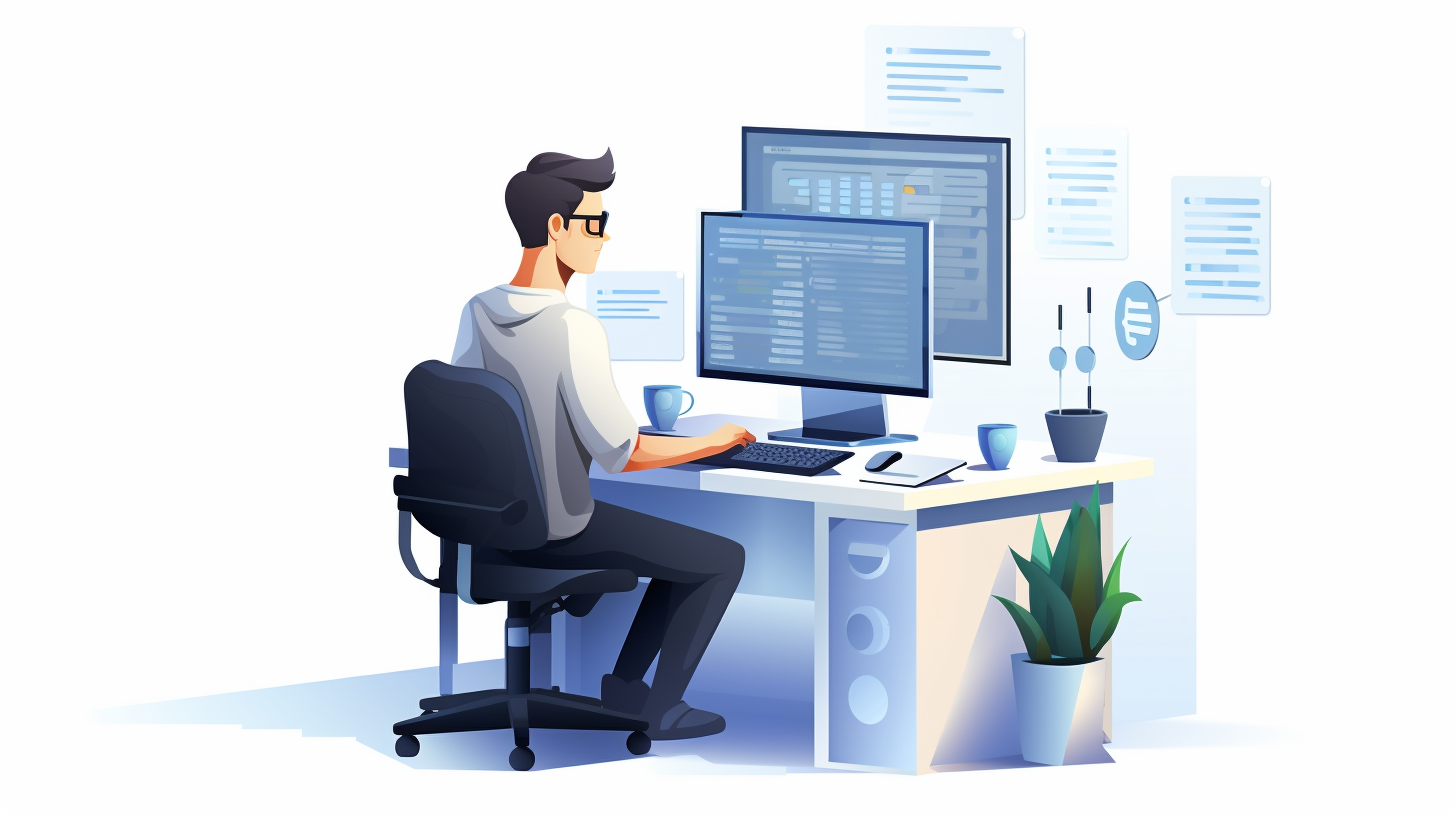
SQL and Regular Expressions for Data Search
Structured Query Language (SQL) offers powerful pattern matching capabilities that enable users to perform a wide range of search operations on textual data. At the core of these capabilities is the LIKE operator, which allows for simple wildcard matching, as well as more advanced features that come into play when using regular expressions.
The LIKE operator is accompanied by two wildcards: the percent sign (%
) represents zero or more characters, while the underscore (_
) represents a single character. This operator is particularly useful for filtering records based on specific patterns. For instance, if you want to find all entries in a customers
table where the name starts with ‘A’, you can execute the following SQL query:
SELECT * FROM customers WHERE name LIKE 'A%';
In addition to the LIKE operator, SQL’s pattern matching capabilities can be enhanced through the use of regular expressions, which are supported in several SQL database systems like PostgreSQL, MySQL, and Oracle. Regular expressions provide a more flexible and powerful way to define search patterns compared to the basic wildcard functionality of LIKE.
For example, if you want to find all email addresses in a users
table that follow a specific pattern, you can use regular expressions with the REGEXP
operator. In the example below, we fetch all users whose email addresses end with @example.com
:
SELECT * FROM users WHERE email REGEXP '@example\.com$';
Regular expressions allow for more complex searching, including the use of character classes, quantifiers, and anchors. For instance, if you want to find phone numbers in various formats, you can craft a regular expression that matches numbers formatted with or without dashes or spaces:
SELECT * FROM contacts WHERE phone_number REGEXP '^[0-9]{3}[- ]?[0-9]{3}[- ]?[0-9]{4}$';
This flexibility makes regular expressions an essential tool for SQL developers when it comes to data validation and extraction. However, it’s important to note that performance may vary depending on the complexity of the regular expression and the size of the dataset being queried.
Using Regular Expressions in SQL Queries
Using regular expressions in SQL queries allows developers to perform complex searches that are often difficult or impossible with the standard LIKE operator. Regular expressions provide a sophisticated syntax for matching patterns, making it easier to extract data that fits specific criteria. This section delves into various ways to harness the power of regular expressions within SQL queries, enhancing data manipulation capabilities.
In SQL, using regular expressions can simplify the process of filtering records based on intricate patterns. For example, ponder a scenario where you need to find all users whose usernames consist of a specific set of characters. You can employ the REGEXP operator in your SQL query as shown below:
SELECT * FROM users WHERE username REGEXP '^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$';
This query retrieves all usernames that adhere to a common email format. The regular expression breaks down as follows:
- ^ asserts the start of the string.
- [a-zA-Z0-9._%+-]+ matches one or more alphanumeric characters or specific symbols.
- @ is a literal character that separates the username from the domain.
- [a-zA-Z0-9.-]+ matches the domain name, which can include hyphens and dots.
- \.[a-zA-Z]{2,}$ matches the top-level domain, ensuring it’s at least two characters long.
Another compelling use case for regular expressions in SQL is sanitizing input data. Think a situation where you want to validate user input for a form field, ensuring it only contains alphanumeric characters. You can accomplish this with the following query:
SELECT * FROM form_submissions WHERE input_field REGEXP '^[a-zA-Z0-9]+$';
In this case, the input_field is filtered to include only those entries made up of letters and numbers, excluding any other characters.
Regular expressions also lend themselves well to complex data transformations. For instance, if you wish to extract specific components from strings, such as removing unwanted characters or formatting data consistently, you can use the REGEXP_REPLACE function available in certain SQL databases. Here is an example that removes all non-numeric characters from a phone number:
SELECT REGEXP_REPLACE(phone_number, '[^0-9]', '') AS clean_phone_number FROM contacts;
This query replaces any character this is not a digit with an empty string, effectively cleaning up the phone number format.
Comparing SQL LIKE and Regular Expressions
When comparing the SQL LIKE operator with regular expressions, it’s important to understand the strengths and limitations of each approach. The LIKE operator is often simpler and more intuitive for simpler pattern matching. It is particularly useful for matching basic patterns or wildcards in data. However, its functionality is limited when faced with more complex matching requirements. Regular expressions, on the other hand, offer a more powerful and flexible approach to pattern matching.
One of the primary differences between LIKE and regular expressions is the syntax and capabilities they offer. The LIKE operator uses percent signs (%) and underscores (_) as wildcards, allowing for basic pattern matching. For example, if you want to find all products whose names begin with the letter ‘B’, you can use the following query:
SELECT * FROM products WHERE product_name LIKE 'B%';
In this case, the query will return any product names that start with the letter ‘B’, followed by any number of characters.
In contrast, regular expressions allow for a much broader range of pattern matching. They can include character classes, quantifiers, and anchors which enable developers to construct intricate search patterns. For instance, if you want to find all email addresses in a table that start with ‘user’ and end with ‘@example.com’, you could employ a regular expression as follows:
SELECT * FROM users WHERE email REGEXP '^user.*@example\.com$';
This query showcases how regular expressions can match a wider array of patterns compared to the LIKE operator. Here, the caret (^) asserts the start of the string, while the dollar sign ($) asserts the end of the string, providing precise control over the pattern being matched.
Another noteworthy aspect is that regular expressions can handle more sophisticated criteria. For example, if you wish to find strings that contain only alphanumeric characters with optional dashes or underscores, a regular expression would be highly effective:
SELECT * FROM identifiers WHERE value REGEXP '^[a-zA-Z0-9_-]+$';
In this query, the regular expression allows for both letters and numbers, while also permitting the presence of dashes (-) and underscores (_).
Performance is also a key consideration in the comparison between LIKE and regular expressions. Generally, the LIKE operator is faster on large datasets when searching for simple patterns due to its simpler nature. Regular expressions, although more powerful, can become computationally expensive, particularly with complex expressions and large datasets. Proper indexing and query optimization are essential when working with regular expressions to mitigate potential performance issues.
Best Practices for Using Regular Expressions in SQL
When it comes to using regular expressions in SQL, best practices can significantly enhance both the performance and maintainability of your queries. Regular expressions are powerful but can also be complex and resource-intensive. Here are several considerations and strategies to optimize their use.
1. Understand Your Database’s Regular Expression Implementation:
Different SQL databases have varying syntax and capabilities when it comes to regular expressions. Familiarize yourself with your specific database’s implementation details, such as supported functions and regex syntax. For instance, MySQL uses the REGEXP operator, while PostgreSQL employs the ~ operator for case-sensitive matches. Knowing the nuances can prevent unexpected behaviors in your queries.
2. Keep Regex Patterns Simple:
Simplicity is key. Complex regular expressions can slow down query performance and make them harder to read and maintain. Break down complicated patterns into simpler components when possible. For example, rather than using a long regex to validate an email format, ponder validating parts of the email using simpler patterns:
SELECT * FROM users WHERE email REGEXP '^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$';
3. Use Anchors Wisely:
Utilize anchors such as ^ (start of string) and $ (end of string) to ensure that your regex matches the intended string positions. This not only improves performance by eliminating unnecessary checks but also increases the accuracy of your matches. For instance:
SELECT * FROM products WHERE product_code REGEXP '^[A-z]{3}-[0-9]{3}$';
4. Limit Dataset Size with WHERE Clauses:
Applying regular expressions on large datasets can be expensive. Always try to filter the dataset first using more efficient WHERE clauses to reduce the number of rows that the regex has to process. For example, if you’re looking for specific patterns in a smaller subset, do this before applying regex:
SELECT * FROM orders WHERE order_date > '2023-01-01' AND customer_id IS NOT NULL AND order_code REGEXP '^[A-Z]{2}[0-9]{4}$';
5. Profile and Optimize Queries:
Regularly profile the performance of your queries, especially when using regex. Tools available in most SQL databases, like EXPLAIN in MySQL and PostgreSQL, can provide insights into how queries are executed and help identify bottlenecks. If a regex is causing slowdowns, ponder optimizing it or revising your approach to data validation and extraction.
6. Document Your Regex Patterns:
Because regular expressions can be cryptic, providing comments or documentation for your patterns is essential. Describe what each component of the regex does to make it easier for others (or yourself in the future) to understand the purpose and functionality. For instance:
-- Matching emails with specific patterns SELECT * FROM users WHERE email REGEXP '^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$';
7. Test Regular Expressions Thoroughly:
Before deploying regex patterns into production queries, test them with a variety of input data to ensure they work correctly. Edge cases can often produce unexpected results, so comprehensive testing is vital to avoid data retrieval issues.