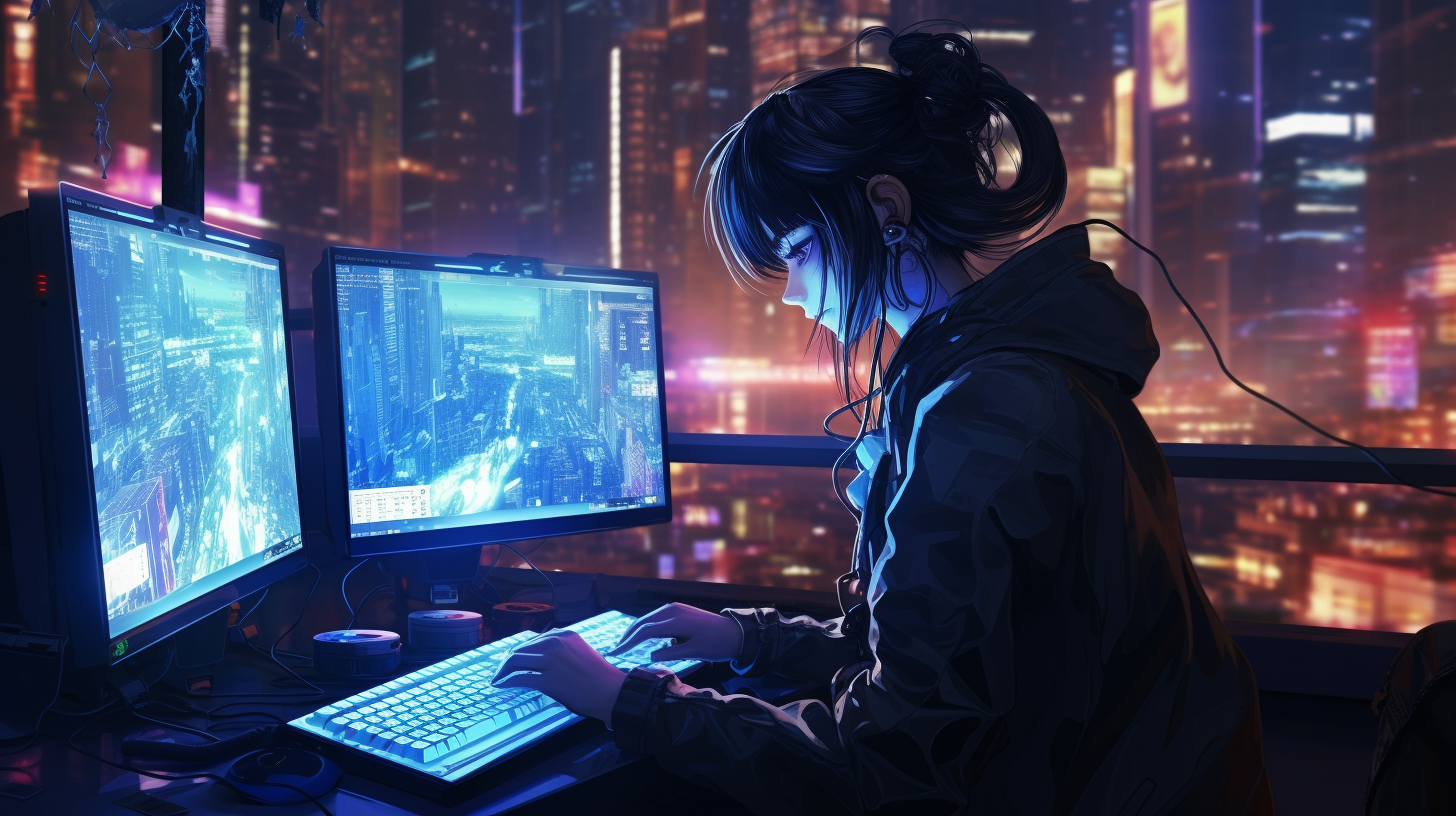
SQL and Triggers for Data Workflow Automation
SQL triggers are powerful tools that enable automatic execution of a set of actions in response to specific events that occur in a database. They’re particularly useful for enforcing business rules, maintaining data integrity, and automating workflows without the need for complex application logic.
A trigger is defined to execute either before or after an event occurs on a specific table or view. The events that can activate a trigger include INSERT, UPDATE, and DELETE operations. This mechanism allows developers to encapsulate complex logic directly within the database, ensuring that the necessary actions are consistently executed whenever the defined events take place.
Understanding how to implement triggers effectively involves grasping their structure and operational flow. A typical trigger consists of the following components:
- A unique identifier for the trigger.
- Specifies whether the trigger should execute BEFORE or AFTER the triggering event.
- Defines the specific database operation (INSERT, UPDATE, DELETE) that activates the trigger.
- The actual SQL statements that are executed when the trigger is fired.
Here’s a simple example of a trigger that logs changes to a table called employees whenever a new employee record is inserted:
CREATE TRIGGER log_employee_insert AFTER INSERT ON employees FOR EACH ROW BEGIN INSERT INTO employee_audit (employee_id, action, action_time) VALUES (NEW.id, 'INSERT', NOW()); END;
In this example:
- The trigger is named log_employee_insert.
- It’s set to execute AFTER a new record is inserted into the employees table.
- For each new row inserted, it adds a record to the employee_audit table, capturing the employee’s ID, the action taken, and the timestamp of the action.
Triggers are not without their complexities. Proper management and understanding of their behavior are crucial, especially when dealing with cascading triggers or potential performance implications. Therefore, as you implement triggers in your database workflows, it’s essential to maintain clarity and simplicity in their logic to avoid unintended consequences.
Types of Triggers in SQL
In SQL, triggers can be categorized primarily based on their timing and the events that activate them. Understanding these categories is essential for effectively employing triggers in your database management tasks. The two main types of triggers are Row-Level Triggers and Statement-Level Triggers, each serving different use cases.
Row-Level Triggers are invoked for each row affected by the triggering event. This means that if an operation (like an UPDATE or DELETE) impacts multiple rows, the trigger will be executed separately for each row. This type is best suited for situations where the logic needs to be applied to individual records, such as auditing or validation on a per-row basis. The syntax for defining a row-level trigger is as follows:
CREATE TRIGGER check_salary BEFORE UPDATE ON employees FOR EACH ROW BEGIN IF NEW.salary < 0 THEN SIGNAL SQLSTATE '45000' SET MESSAGE_TEXT = 'Salary cannot be negative'; END IF; END;
In this example, the check_salary
trigger checks the salary of an employee before any update occurs. If the new salary is negative, it raises an error, preserving data integrity.
Statement-Level Triggers, on the other hand, are executed once per triggering event, regardless of the number of rows affected. That is useful for operations where the logic pertains to the entire statement rather than individual records. For instance, you might use a statement-level trigger to log an operation when a batch of records is deleted from a table:
CREATE TRIGGER log_employee_deletion AFTER DELETE ON employees BEGIN INSERT INTO deletion_audit (deleted_at, total_deleted) VALUES (NOW(), ROW_COUNT()); END;
In this case, the log_employee_deletion
trigger logs the time of deletion and the number of rows deleted simultaneously. This is particularly advantageous for bulk operations where individual row logging might be inefficient.
Additionally, triggers can be categorized based on their specific events:
- These triggers execute prior to the triggering event. They are commonly used for validation or modifications before data changes are committed.
- Executing after the triggering event, these are suitable for actions that rely on the outcome of the data modification, such as logging or cascading changes to other tables.
Understanding the nuances of these trigger types allows for a more tailored approach in implementing database automation strategies. It is vital to assess the needs of your application and choose the appropriate trigger type to optimize performance while ensuring the desired behavior of your database workflows.
Use Cases for Workflow Automation
Workflow automation using SQL triggers opens up numerous opportunities for enhancing data management processes across various domains. The versatility of triggers can be seen in a high number of practical scenarios, from data validation to complex business rules enforcement. Here, we explore several compelling use cases that illustrate the power of triggers in streamlining workflows.
One of the most common use cases for triggers is **audit logging**. Businesses often need to maintain a historical record of changes made to critical data for compliance and accountability. Triggers can automatically insert records into an audit table whenever significant operations occur, such as inserts, updates, or deletes. For example, you can create a trigger that logs all updates made to a customer table, capturing details like the previous value and the timestamp:
CREATE TRIGGER log_customer_updates AFTER UPDATE ON customers FOR EACH ROW BEGIN INSERT INTO customer_audit (customer_id, old_value, new_value, change_time) VALUES (OLD.id, OLD.name, NEW.name, NOW()); END;
This trigger ensures that every time a customer’s name is updated, the old and new values are recorded, which is invaluable for tracking changes over time.
Another powerful use case is **data validation**. Triggers can enforce business rules at the database level, preventing invalid data from being entered. For instance, if a company has a policy that employees cannot have a salary below a certain threshold, a trigger can be employed to enforce this rule:
CREATE TRIGGER validate_salary BEFORE INSERT OR UPDATE ON employees FOR EACH ROW BEGIN IF NEW.salary < 30000 THEN SIGNAL SQLSTATE '45000' SET MESSAGE_TEXT = 'Salary must be at least $30,000'; END IF; END;
This trigger prevents any unauthorized changes to employee salaries that do not comply with the company policy, thus ensuring data integrity.
Triggers can also facilitate **automatic data transformations**. For instance, when a new order is placed, a trigger can automatically calculate the total price based on the items ordered, applying any applicable discounts before finalizing the order record:
CREATE TRIGGER calculate_order_total BEFORE INSERT ON orders FOR EACH ROW BEGIN SET NEW.total_price = NEW.item_price * NEW.quantity - NEW.discount; END;
This trigger ensures that the total price of an order is always computed correctly, eliminating the need for manual calculations or additional application logic.
Triggers can also be instrumental in **maintaining referential integrity**. For instance, if a related record is deleted from a parent table, a trigger can ensure that corresponding records in a child table are also deleted to maintain a clean data state:
CREATE TRIGGER cascade_delete_orders AFTER DELETE ON customers FOR EACH ROW BEGIN DELETE FROM orders WHERE customer_id = OLD.id; END;
This cascade delete trigger automatically removes all orders associated with a deleted customer, ensuring that no orphan records remain in the database.
In addition to the aforementioned scenarios, triggers can also be employed for **notifications and alerts**. For example, a trigger can send an alert or notification when stock levels for a product fall below a certain threshold:
CREATE TRIGGER notify_low_stock AFTER UPDATE ON inventory FOR EACH ROW BEGIN IF NEW.stock < 10 THEN CALL send_stock_alert(NEW.product_id); END IF; END;
In this example, the trigger checks the stock level after an update and calls a stored procedure to send an alert if the stock is critically low.
These use cases exemplify the myriad ways SQL triggers can be leveraged for workflow automation, enhancing both operational efficiency and data integrity. By embedding business logic directly within the database, triggers empower developers to focus on high-level application design while ensuring that fundamental rules and processes are rigorously enforced at the database level.
Best Practices for Implementing Triggers
Implementing triggers effectively requires a careful approach to design and maintenance, as poorly designed triggers can lead to performance issues, unintended consequences, and debugging challenges. Here are some best practices to follow when implementing triggers in your SQL database.
1. Keep Trigger Logic Simple
A trigger should perform a specific function without overly complex logic. The more complex the trigger, the harder it will be to maintain and debug. Aim to encapsulate a single, clear action within each trigger. If you find yourself writing extensive logic in a trigger, it may be a sign that the logic should be moved to a stored procedure or application layer.
2. Minimize Side Effects
Triggers can have side effects that are not immediately obvious when the triggering event occurs. For example, a trigger that performs an additional update on another table can introduce unexpected behavior. Always ponder the broader implications of the trigger’s actions and strive to avoid cascading triggers where possible, to prevent issues with data consistency and performance.
3. Use Transaction Control Wisely
When designing triggers, be mindful of transactions. Triggers execute within the context of the transaction that initiated them. If a trigger encounters an error, it can cause the entire transaction to roll back, potentially leading to data loss or inconsistency. Use appropriate error handling mechanisms to manage exceptions in your triggers.
4. Monitor Performance
Triggers can impact performance, especially if they are executed frequently or involve time-consuming operations. Regularly monitor the performance of your database to identify any slow-running triggers. Consider using SQL performance profiling tools to help identify and optimize bottlenecks.
5. Document Your Triggers
Documentation is key in maintaining a healthy database. Clearly document every trigger: its purpose, the events that fire it, and its expected behavior. This practice not only helps other developers understand your logic but also aids in troubleshooting and debugging when issues arise.
6. Test Thoroughly
Before deploying triggers to a production environment, ensure they are thoroughly tested. Create test cases that simulate various scenarios to validate the trigger’s behavior under different conditions. Pay special attention to edge cases and error conditions to ensure that the trigger behaves as expected.
7. Consider Alternatives
Sometimes, using triggers may not be the most efficient approach for a particular situation. Evaluate whether other database features, such as stored procedures, scheduled jobs, or application-level logic, might serve your needs better. While triggers can be powerful, they’re not always the best solution for every automation task.
By adhering to these best practices, you can harness the full potential of triggers while minimizing risks and complications in your SQL databases. Always remember that clarity, simplicity, and thoroughness are your allies within the scope of database automation.
Troubleshooting Common Trigger Issues
Even with careful implementation, triggers can sometimes present challenges that require troubleshooting. Common issues may arise from the complexity of the logic, unintended side effects, or performance degradation. Addressing these challenges effectively requires a systematic approach to identifying and resolving problems as they occur.
One frequent issue with triggers is the potential for recursive behavior. This happens when a trigger’s actions inadvertently cause it to fire again, leading to infinite loops. For example, if an AFTER INSERT trigger updates a table that has another trigger on it, this can create a recursive situation. To mitigate this, ensure that your trigger logic is designed to prevent such scenarios, possibly by using flags or dedicated logging tables to track trigger execution.
CREATE TRIGGER prevent_recursion AFTER INSERT ON example_table FOR EACH ROW BEGIN IF (SELECT COUNT(*) FROM recursion_log WHERE action_id = NEW.id) = 0 THEN INSERT INTO recursion_log (action_id, action_time) VALUES (NEW.id, NOW()); -- Trigger logic here END IF; END;
Another common pitfall is performance issues caused by triggers that execute expensive operations, particularly if they’re row-level triggers. Each invocation of such a trigger can result in a significant performance hit if the operation scales with the number of rows affected. To diagnose performance problems, utilize your SQL database’s query execution plan and profiling tools to identify which triggers are consuming resources disproportionately.
SQL server logs and monitoring tools can also provide insights into trigger performance. For instance, if you notice high response times for certain operations, check the execution frequency of any associated triggers.
SELECT COUNT(*) AS trigger_execution_count FROM information_schema.triggers WHERE trigger_name = 'your_trigger_name';
Additionally, be aware of data integrity issues that may arise from triggers that modify data without proper safeguards. It’s essential to implement validation checks within your triggers to prevent invalid data from corrupting your database. Use the SIGNAL statement to raise exceptions when encountering invalid data conditions, as demonstrated in the salary validation example mentioned earlier.
Testing is critical when it comes to triggers. Ensure that you have a comprehensive test suite that includes various scenarios, including edge cases. If a trigger fails or behaves unexpectedly, the ability to reproduce the problem is invaluable for troubleshooting. Ponder maintaining a version history of your triggers to help identify when a change may have introduced a problem.
-- Sample test case for a trigger BEGIN TRY INSERT INTO employees (name, salary) VALUES ('Mitch Carter', -5000); EXCEPTION WHEN SQLSTATE '45000' THEN -- Handle trigger exception here END TRY;
Finally, if you find yourself frequently troubleshooting the same set of issues, it may be time to reassess the structure and complexity of your triggers. Simplifying trigger logic and distributing functionality across multiple, smaller triggers or moving some logic to stored procedures can often lead to more maintainable and robust database operations.