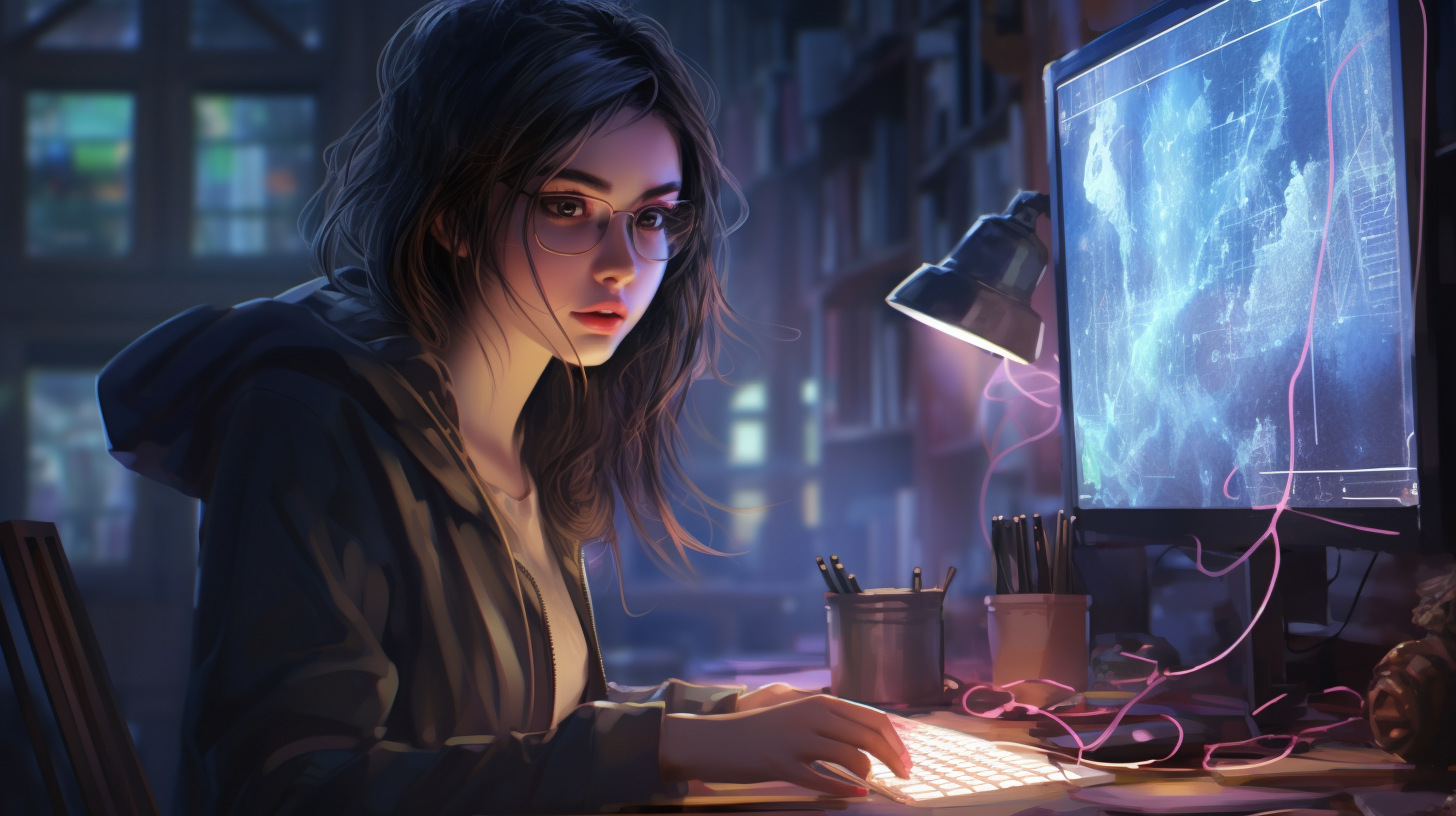
SQL and Views for Data Abstraction
Data abstraction in SQL is a powerful concept that enables developers to interact with complex data structures while limiting their exposure to the underlying intricacies of the database schema. It’s essentially a way to simplify data access and manipulation, allowing users to focus on what data they need rather than how it is organized and stored.
At its core, data abstraction involves separating the interface of the data from its implementation. That’s particularly useful in relational databases, where data is often spread across multiple tables and relationships between them can be complex. By using abstraction techniques, SQL developers can create more intuitive interfaces for data retrieval and manipulation.
In SQL, data abstraction can be achieved through:
- Virtual tables that present data from one or more tables in a simplified manner, allowing users to work with data without needing to know the underlying table structures.
- Predefined SQL code that can encapsulate complex queries and logic, enabling users to execute these routines without needing to understand the details of their implementation.
- SQL functions that allow users to perform calculations or transformations on data, abstracting the complexity of the underlying logic.
By using these features, developers can create a cleaner and more uncomplicated to manage experience. For example, a view can be created to present only the necessary columns from a table, thereby hiding the rest of the data that may not be relevant to the user:
CREATE VIEW SimpleView AS SELECT id, name, email FROM Users;
In this case, the SimpleView
provides a simpler way to access user information without exposing the entire Users
table. This not only simplifies the queries for the end-users but also enhances security by restricting access to sensitive data.
Ultimately, understanding data abstraction in SQL is essential for building scalable and maintainable applications. It allows developers to craft interfaces that can evolve independently of the underlying data structures, thus making it easier to adapt to changing requirements over time.
Defining Views in SQL
Defining a view in SQL is a fundamental operation that transforms how users interact with data stored in databases. A view acts as a virtual table, representing the result set of a stored query, which can be queried just like a regular table. This can significantly simplify data retrieval, particularly when dealing with complex queries that involve multiple tables.
To create a view, you use the CREATE VIEW statement, followed by the name of the view and the AS keyword, which is then followed by a SELECT statement that defines the data to be included in the view. For instance, consider a scenario where you have a database with a table named Orders. You might want to create a view that consolidates the orders along with their corresponding customer names from a Customers table:
CREATE VIEW OrderDetails AS SELECT Orders.order_id, Customers.customer_name, Orders.order_date, Orders.total_amount FROM Orders JOIN Customers ON Orders.customer_id = Customers.customer_id;
In this example, the OrderDetails view allows users to access order information alongside customer names without needing to understand or write the join logic themselves. This encapsulation of complexity is a key benefit of views, enhancing data access and usability.
Additionally, views can also include aggregate functions, allowing for summary data to be directly accessible. For instance, if you want to create a view that shows the total sales per customer, you could write:
CREATE VIEW TotalSalesPerCustomer AS SELECT Customers.customer_name, SUM(Orders.total_amount) AS total_sales FROM Orders JOIN Customers ON Orders.customer_id = Customers.customer_id GROUP BY Customers.customer_name;
With the TotalSalesPerCustomer view, users can quickly retrieve summarized sales data without having to write complex SQL queries repeatedly. This not only reduces the chance for errors but also improves the performance of applications by allowing the database engine to optimize the execution of the view.
It’s important to note that views can also be defined with restrictions, such as filtering rows based on certain conditions using the WHERE clause. This can help in applying security measures, ensuring users only access data they are permitted to see. For example:
CREATE VIEW ActiveCustomers AS SELECT * FROM Customers WHERE status = 'active';
The ActiveCustomers view ensures that only customers who are currently active are presented to the users, thereby limiting exposure to unnecessary or sensitive information.
Defining views in SQL is an important aspect of effective database management. It streamlines access to data, enhances security, and allows for better organization of complex queries into manageable components. By abstracting the underlying complexity, views facilitate a more intuitive interaction with the database.
Creating and Managing Views
Creating and managing views in SQL is a simpler yet powerful process that significantly enhances the way users interact with the underlying data. Once a view is defined, it becomes a persistent object in the database, providing an encapsulated representation of the data that can simplify user queries and improve maintainability.
The syntax for creating a view is simple, as illustrated previously. However, managing views involves not only creating them but also knowing how to modify or remove them as needs evolve. To update a view, one can use the CREATE OR REPLACE VIEW
statement, which allows alterations without dropping and recreating the view. For instance:
CREATE OR REPLACE VIEW OrderDetails AS SELECT Orders.order_id, Customers.customer_name, Orders.order_date, Orders.total_amount, Orders.status FROM Orders JOIN Customers ON Orders.customer_id = Customers.customer_id;
This command updates the OrderDetails
view, adding a new column, status
, to the result set while preserving the existing functionality. Such flexibility is important in dynamic environments where data structures and requirements frequently change.
To remove a view that is no longer needed, the DROP VIEW
statement is utilized. This operation is simpler but should be done with caution, as it will eliminate the view and any associated access to the defined data representation:
DROP VIEW ActiveCustomers;
When managing views, it’s also essential to keep in mind the associated permissions. Views can be granted specific user access rights, enabling you to control who can see or manipulate the data represented by the view. This is accomplished using the GRANT
statement:
GRANT SELECT ON OrderDetails TO data_analyst;
Conversely, if a view needs to be stripped of its access rights, the REVOKE
statement can be employed:
REVOKE SELECT ON OrderDetails FROM data_analyst;
Additionally, views can be indexed to improve performance, particularly for more complex queries. That is done using the CREATE INDEX
statement on the underlying base tables rather than the view itself, as views cannot be indexed directly. The performance gains are realized when operations on the view are executed, allowing the database engine to optimize query execution using the indexes defined on the base tables.
Creating and managing views is a vital aspect of SQL database interaction that empowers developers and users alike. It allows for the encapsulation of complex queries, simplification of data access, and enhancement of security measures. By mastering the techniques of view creation, modification, and management, one can significantly improve the usability and performance of SQL-based applications.
The Benefits of Using Views
Using views in SQL provides a high number of advantages that enhance the overall functionality and usability of database interactions. One of the primary benefits is the simplification of complex queries. In many cases, users need to access data from multiple tables and apply various transformations to derive meaningful insights. By encapsulating these complexities within a view, users can retrieve the desired information with a simple SELECT statement, which significantly reduces the risk of errors and simplifies query writing.
For example, consider a situation where analysts need to obtain sales data along with corresponding product details and customer information. Instead of writing intricate JOIN operations every time, they can create a view that does this work for them:
CREATE VIEW SalesReport AS SELECT Sales.sale_id, Products.product_name, Customers.customer_name, Sales.sale_date, Sales.amount FROM Sales JOIN Products ON Sales.product_id = Products.product_id JOIN Customers ON Sales.customer_id = Customers.customer_id;
Once the SalesReport view is established, users can simply query it:
SELECT * FROM SalesReport;
This not only makes retrieval simpler but also allows for greater focus on data analysis rather than the underlying schema.
Another remarkable benefit of views is the enhancement of security and access control. Views can act as a security layer by limiting user access to specific rows or columns of data. For instance, if there are sensitive fields within a table, a view can be created that excludes these fields, ensuring that users can access only the information they need. That is particularly critical in environments where data privacy is paramount.
CREATE VIEW PublicCustomerData AS SELECT customer_id, customer_name, email FROM Customers;
In this example, the PublicCustomerData view provides essential customer information without exposing sensitive data such as phone numbers or addresses, thus adhering to data protection regulations.
Views also facilitate easier maintenance of applications. When underlying database schemas change, developers can update the view without necessitating changes to the application code that depends on it. This decoupling between the database schema and application logic is invaluable for long-term project sustainability. For instance, if the data structure of the Products table changes, the view can be modified accordingly without impacting users:
CREATE OR REPLACE VIEW SalesReport AS SELECT Sales.sale_id, Products.product_name, Customers.customer_name, Sales.sale_date, Sales.amount, Products.category FROM Sales JOIN Products ON Sales.product_id = Products.product_id JOIN Customers ON Sales.customer_id = Customers.customer_id;
By updating the view definition, the same simpler query can continue to provide relevant data while reflecting the new schema.
Moreover, views can enhance query performance under certain conditions. When a view is defined based on complex calculations or aggregations, it can act as a materialized view (if supported by the database) that stores the result set, thereby speeding up access to frequently queried data. This can lead to substantial efficiency gains, especially in reporting scenarios where data is aggregated and accessed repeatedly.
Overall, the benefits of using views in SQL are manifold. They simplify complex data interactions, bolster security, support maintenance and adaptability, and can even improve performance when used appropriately. By using views effectively, both developers and users can work more efficiently and with greater confidence in their data analysis tasks.
Performance Considerations with Views
When considering performance with views in SQL, it’s important to understand how they interact with the underlying data and how they can impact query execution. Views can introduce various performance implications, both positive and negative, depending on how they are defined and utilized. Understanding these nuances is critical for optimizing database performance.
One of the primary concerns regarding views is the potential for performance degradation when executing complex queries. Since a view essentially encapsulates a SELECT statement, every time the view is queried, the underlying SQL statement is executed. This means that if the view is built on a complex query involving multiple joins, aggregations, or subqueries, it can lead to increased execution time and resource consumption. For example:
CREATE VIEW ComplexView AS SELECT Orders.order_id, Customers.customer_name, SUM(Orders.total_amount) AS total_sales FROM Orders JOIN Customers ON Orders.customer_id = Customers.customer_id GROUP BY Orders.order_id, Customers.customer_name;
When querying the ComplexView, the database engine must process the full logic of the view, which can be costly if the underlying tables are large:
SELECT * FROM ComplexView WHERE total_sales > 1000;
In such cases, the performance impact can be mitigated by ensuring that the underlying tables are properly indexed. By creating indexes on frequently queried columns in the base tables, the execution speed of queries involving views can be improved significantly. For instance:
CREATE INDEX idx_customer_id ON Customers(customer_id); CREATE INDEX idx_order_id ON Orders(order_id);
Another performance consideration is related to the use of materialized views, if supported by the database system. Unlike regular views, which are virtual tables that execute their queries on-the-fly, materialized views store the results of the query physically. This can yield substantial performance benefits for queries that are frequently executed but do not require real-time data. For example:
CREATE MATERIALIZED VIEW FastSalesReport AS SELECT Sales.sale_id, SUM(Sales.amount) AS total_amount FROM Sales GROUP BY Sales.sale_id;
Since the result set of a materialized view is precomputed, querying it is typically much faster than querying a regular view, especially for large datasets. However, it is important to note that materialized views require management regarding when to refresh the stored data to ensure it reflects the latest changes in the underlying tables.
Furthermore, views can also help optimize performance by providing a layer of abstraction that can simplify complex queries. For instance, developers can create smaller, focused views that encapsulate specific data requirements. This modular approach allows users to build their queries on top of these simplified views instead of repeatedly executing complex logic, thus reducing the likelihood of errors and improving maintainability.
CREATE VIEW SimpleSales AS SELECT product_id, SUM(amount) AS total_sales FROM Sales GROUP BY product_id;
Users can then easily query the SimpleSales view to get the relevant sales data without delving into the complexities of the original Sales table.
While views can introduce performance challenges, they also offer a high number of ways to optimize SQL interactions. By using proper indexing, using materialized views where applicable, and designing views that encapsulate complex queries effectively, developers can enhance query performance while benefiting from the abstraction that views provide. Understanding these performance considerations is essential for maintaining an efficient and responsive SQL database environment.
Common Use Cases for Views
Common use cases for views in SQL exemplify their versatility and power in streamlining complex data interactions. Views can be tailored to cater to specific reporting needs, facilitate data security, and enhance data management practices. Let’s explore some practical scenarios where views shine, demonstrating how they can significantly improve the efficiency and clarity of database operations.
One of the most common use cases for views is in the sphere of reporting. Organizations often require periodic reports that aggregate data from multiple tables. By creating a view that consolidates relevant data, users can easily access these reports without needing to understand the underlying complexities. For instance, think a business that wants to generate monthly sales reports. A view can be created to summarize sales by month:
CREATE VIEW MonthlySales AS SELECT DATE_TRUNC('month', sale_date) AS sales_month, SUM(amount) AS total_sales FROM Sales GROUP BY sales_month ORDER BY sales_month;
This MonthlySales view allows the sales team to quickly obtain the total sales for each month with a simple query:
SELECT * FROM MonthlySales;
Another prevalent use case for views is to enhance security. In scenarios where sensitive information exists within a database, views can limit user access to only the data necessary for their role. For example, if a payroll database contains employee salaries, but you want to create a view for HR personnel that excludes sensitive salary information, you could define a view as follows:
CREATE VIEW EmployeeDetails AS SELECT employee_id, first_name, last_name, department FROM Employees;
This EmployeeDetails view provides HR staff the essential information they require without exposing sensitive salary data, thus maintaining confidentiality while ensuring access to necessary information.
Views are also invaluable for simplifying complex queries across multiple tables, especially in scenarios where users may not be well-versed in SQL. By encapsulating intricate logic within a view, users can focus on retrieving data without worrying about how to construct complex joins or aggregations. For instance, if an organization needs to track product sales alongside customer details, a view can be defined as follows:
CREATE VIEW ProductSales AS SELECT Products.product_name, Customers.customer_name, Sales.sale_date, Sales.amount FROM Sales JOIN Products ON Sales.product_id = Products.product_id JOIN Customers ON Sales.customer_id = Customers.customer_id;
With the ProductSales view in place, users can retrieve comprehensive sales information without needing to compose the underlying join logic:
SELECT * FROM ProductSales;
Moreover, views find their application in data transformation tasks. When data needs to be presented in a particular format for analytics or reporting purposes, rather than altering the base tables, a view can be constructed to present the data as needed. For instance, if an organization wants to display customer information formatted in a user-friendly way, it can create a view that concatenates the customer’s first and last name:
CREATE VIEW CustomerFullName AS SELECT customer_id, CONCAT(first_name, ' ', last_name) AS full_name, email FROM Customers;
This CustomerFullName view makes it easy for users to access a formatted version of customer names while keeping the original structure intact.
Lastly, views are often employed in data warehousing scenarios where predetermined aggregations or transformations are necessary for performance optimization. Creating views that summarize large volumes of transactional data can drastically improve retrieval times for analytic queries. For instance, if a business requires a view that aggregates sales by product category for quick access, it might look like this:
CREATE VIEW SalesByCategory AS SELECT Products.category, SUM(Sales.amount) AS total_sales FROM Sales JOIN Products ON Sales.product_id = Products.product_id GROUP BY Products.category;
This SalesByCategory view allows analysts to quickly assess sales performance across different categories without executing complex queries against the raw data.
Views serve as a powerful tool in SQL for addressing various use cases, from simplifying reporting processes and enhancing security to streamlining complex queries and improving data management. By considering these scenarios, developers can leverage views to create efficient, uncomplicated to manage database environments that cater to the diverse needs of their organizations.