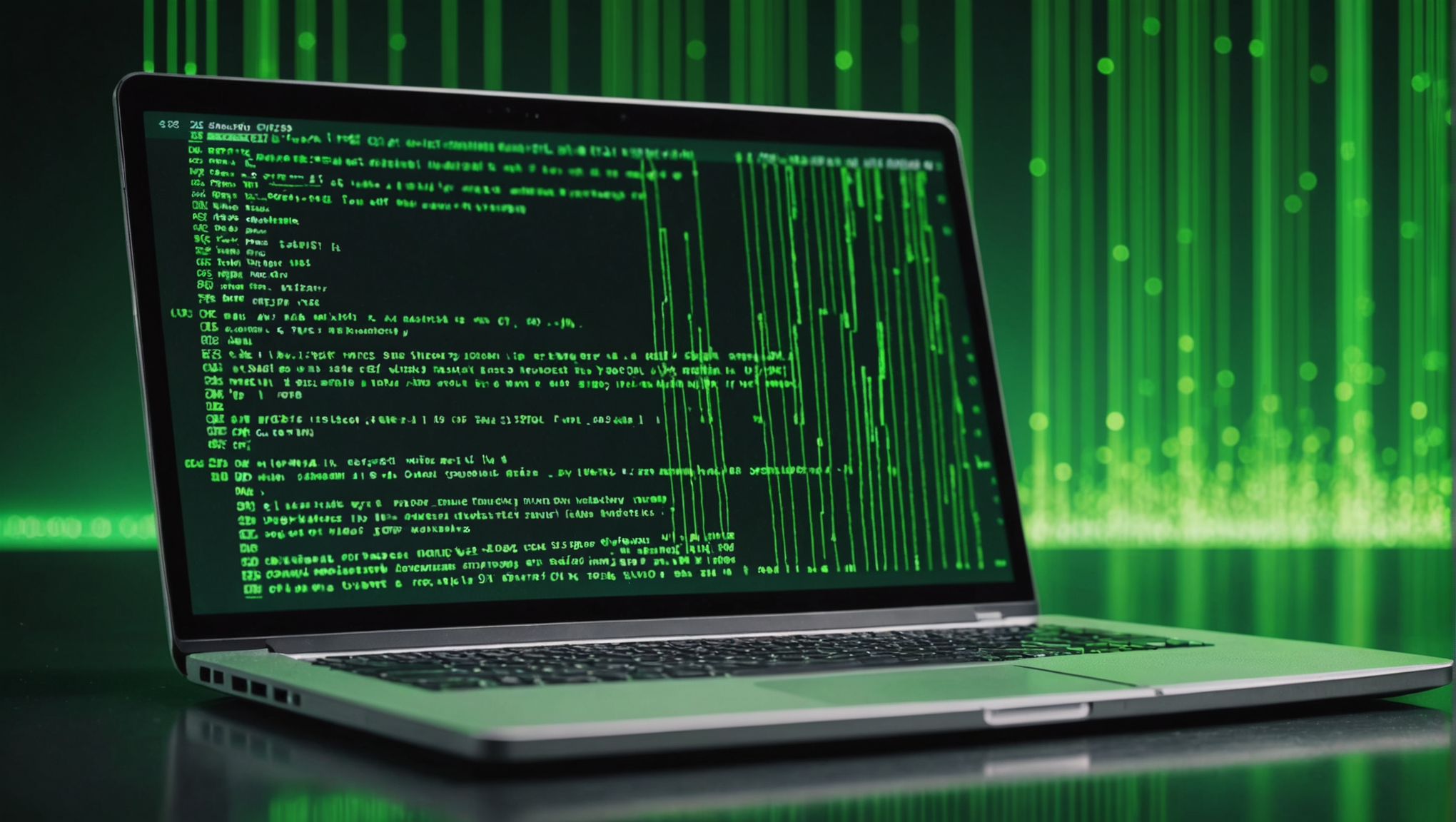
SQL Best Practices for Data Export
When it comes to data export, understanding the different formats available especially important for ensuring that the data is usable and meets the requirements of its end users. Various formats cater to different needs, and selecting the right one can significantly affect data interoperability and usability. Here are some common data export formats:
- That is one of the most widely used formats for data export. CSV files are plain text files that contain data separated by commas. They are simple, lightweight, and can be easily opened in spreadsheet applications like Microsoft Excel or Google Sheets.
SELECT * FROM your_table INTO OUTFILE 'your_data.csv' FIELDS TERMINATED BY ',' ENCLOSED BY '"' LINES TERMINATED BY 'n';
SELECT json_agg(row_to_json(your_table)) FROM your_table;
SELECT xmlelement(name "your_table", xmlagg(xmlelement(name "row", your_column1 || ', ' || your_column2 )) ) FROM your_table;
COPY (SELECT * FROM your_table) TO 'your_data.parquet' WITH (FORMAT 'PARQUET');
COPY (SELECT * FROM your_table) TO PROGRAM 'csv2excel your_data.csv' WITH (FORMAT 'CSV');
Choosing the Right Export Method
Choosing the right export method is important for ensuring that data is exported efficiently and effectively. Different methods come with varied capabilities, and the choice hinges on factors like the data volume, destination systems, and the complexity of the data structure. Below are some commonly used methods for exporting data, along with considerations for each.
- This method involves executing SQL commands directly to create export files. It’s simpler for smaller datasets and allows for quick exports. However, as data volumes increase, this method may lead to performance issues.
COPY (SELECT * FROM your_table) TO 'your_data.csv' WITH (FORMAT 'CSV');
CREATE EVENT daily_export ON SCHEDULE EVERY 1 DAY DO BEGIN COPY (SELECT * FROM your_table) TO 'daily_export.csv' WITH (FORMAT 'CSV'); END;
-- Pseudo code for API data export POST /api/data/export { "data": (SELECT * FROM your_table) }
Optimizing Query Performance for Exports
Optimizing query performance for exports is a critical factor that can significantly enhance the speed and efficiency of your data export processes. When dealing with large datasets, slow queries can lead to bottlenecks, extended wait times, and might even affect the overall database performance. Here are some strategies to optimize query performance during data exports:
- Instead of using SELECT *, specify only the columns you need. This reduces the amount of data transferred and processed.
SELECT column1, column2 FROM your_table;
SELECT column1, column2 FROM your_table WHERE condition;
CREATE INDEX idx_column ON your_table(column1);
DO $$ DECLARE r RECORD; BEGIN FOR r IN SELECT DISTINCT batch_key FROM your_table LOOP COPY (SELECT * FROM your_table WHERE batch_key = r.batch_key) TO 'export_batch_' || r.batch_key || '.csv' WITH (FORMAT 'CSV'); END LOOP; END $$;
COPY (SELECT * FROM your_table) TO 'your_data.csv' WITH (FORMAT 'CSV', COMPRESSION 'gzip');
EXPLAIN SELECT column1, column2 FROM your_table WHERE condition;
Ensuring Data Integrity During Export
Ensuring data integrity during export is critical to maintain the accuracy and reliability of the information being shared. Data integrity involves safeguarding the consistency and accuracy of data over its entire lifecycle, and this is especially important when transferring data between systems or formats. Here are some best practices to ensure data integrity throughout the export process:
- Always perform checks on the data to ensure it meets the required standards and formats. This step can help catch discrepancies before data is exported.
SELECT * FROM your_table WHERE column1 IS NULL OR column2 < 0;
BEGIN; COPY (SELECT * FROM your_table WHERE condition) TO 'your_data.csv' WITH (FORMAT 'CSV'); COMMIT;
SELECT md5(array_agg(column1::text)) FROM your_table;
INSERT INTO export_log (export_time, record_count, status) VALUES (NOW(), (SELECT COUNT(*) FROM your_table), 'success');
SELECT COUNT(*) AS source_count FROM your_table; SELECT COUNT(*) AS export_count FROM 'your_data.csv';
SELECT column1::varchar FROM your_table;
Automating Data Export Processes
Automating data export processes is an essential aspect of state-of-the-art data management, enabling organizations to streamline their workflows and ensure timely availability of data. By automating exports, you not only reduce the chances of human error but also free up valuable resources for other tasks. Below are several approaches to effectively automate data export processes:
- Many database systems offer built-in features to schedule tasks, so that you can specify when exports should occur. For instance, you can utilize cron jobs in PostgreSQL or SQL Server Agent in SQL Server to automate routine exports. That’s particularly useful for regular data backups or generating reports.
CREATE EVENT daily_export ON SCHEDULE EVERY 1 DAY DO BEGIN COPY (SELECT * FROM your_table) TO 'daily_export.csv' WITH (FORMAT 'CSV'); END;
CREATE PROCEDURE export_data() BEGIN DECLARE exit handler for SQLEXCEPTION BEGIN ROLLBACK; END; START TRANSACTION; COPY (SELECT * FROM your_table) TO 'export_data.csv' WITH (FORMAT 'CSV'); COMMIT; END;
CREATE TRIGGER after_insert AFTER INSERT ON your_table FOR EACH ROW BEGIN COPY (SELECT * FROM your_table) TO 'incremental_export.csv' WITH (FORMAT 'CSV'); END;
Documentation and Maintenance of Export Procedures
Documentation and maintenance of export procedures are essential components of a robust data management strategy. Properly documenting the steps involved in data exports ensures that team members can replicate and understand the processes, leading to consistent results and reduced errors. Additionally, regular maintenance of these procedures helps to keep them relevant and effective as data systems evolve. Here are several best practices for documenting and maintaining your data export procedures:
- Document every aspect of the export process, including SQL queries used, data sources, export formats, and any transformations applied. This provides a clear reference for team members and aids in troubleshooting.
-- Example of documentation for an export procedure -- Export Data Procedure -- Description: Exports data from your_table to a CSV file -- SQL Query: COPY (SELECT * FROM your_table) TO 'your_data.csv' WITH (FORMAT 'CSV'); -- Frequency: Daily at 2 AM -- Responsible Person: Data Manager
-- Common export errors and resolutions -- Error: "Permission denied" when writing file -- Resolution: Check file permissions and ensure the export location is writable.