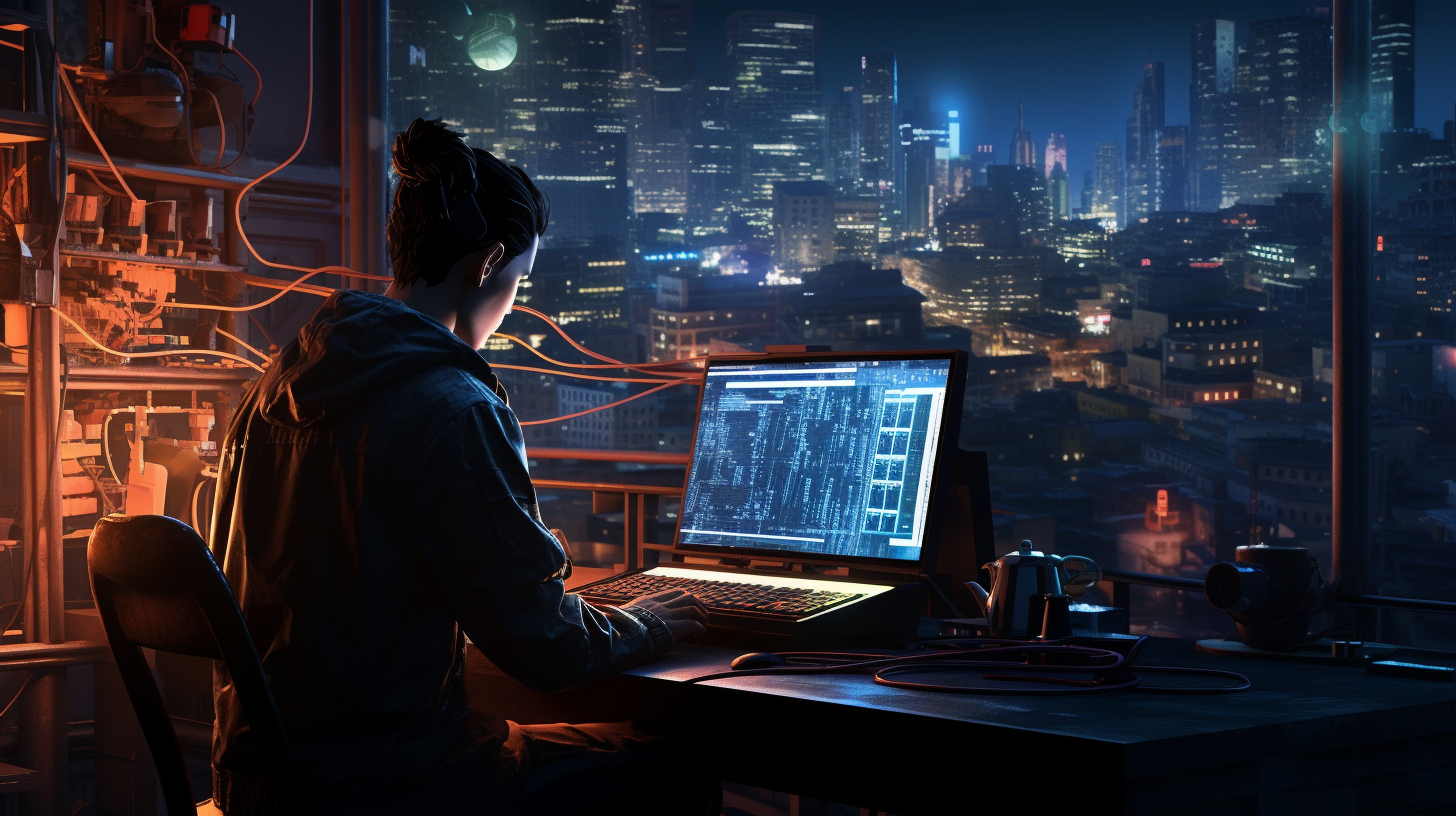
SQL Best Practices for Data Indexing
Indexing is an important aspect of database performance optimization. Effective indexing strategies can significantly reduce data retrieval times and improve query performance. Here are some essential strategies to consider:
- Before creating indexes, analyze the queries that are frequently executed. Look for patterns such as filters, sorts, and joins. Use tools like
EXPLAIN
to understand how queries are executed and which indexes are used. - When queries involve multiple columns, consider creating composite indexes. A composite index on columns
column1
andcolumn2
can improve the performance of queries that filter or sort on both columns. However, the order of columns in the index matters:
CREATE INDEX idx_column1_column2 ON table_name (column1, column2);
Choosing the Right Index Type
Choosing the right index type is a pivotal decision in optimizing database performance. Different indexing strategies cater to various data access patterns and query requirements. Understanding the distinct types of indexes available allows you to tailor your approach to your specific use case.
B-Tree Indexes: The most common type of index in relational databases, B-tree indexes are efficient for a wide range of query operations, including equality and range queries. They maintain a balanced tree structure, enabling logarithmic search times. You can create a B-tree index simply with:
CREATE INDEX idx_example ON table_name (column_name);
This index type is particularly effective for columns that are frequently searched or sorted.
Hash Indexes: If your queries primarily consist of equality comparisons, hash indexes may be more efficient. They store a hash of the indexed column’s value, allowing for constant time complexity in lookups. However, they do not support range queries. Creating a hash index can be done as follows:
CREATE INDEX idx_hash ON table_name USING HASH (column_name);
Be mindful that hash indexes are typically used in specific scenarios, such as with in-memory databases or specific database systems that support them.
Full-Text Indexes: For searching large textual fields, full-text indexes provide powerful capabilities, allowing for full-text queries that can match words and phrases. This type of index is essential for applications that require text search functionalities. You can create a full-text index in SQL Server with:
CREATE FULLTEXT INDEX ON table_name(column_name) KEY INDEX idx_primary;
Using a full-text index can greatly enhance search capabilities but requires careful planning regarding the data it will index.
Spatial Indexes: If you are dealing with geographic data, spatial indexes are crucial for optimizing queries that involve spatial data types. They enable efficient querying of complex geometric shapes and geographical data, particularly useful for applications in mapping and location-based services.
Filtered Indexes: A filtered index is a special type of index that only includes a subset of rows in the index based on a specified condition. This can drastically reduce the size of the index and improve performance for specific queries. You can create a filtered index like this:
CREATE INDEX idx_filtered ON table_name (column_name) WHERE condition_column = 'value';
Using filtered indexes allows you to optimize performance for queries that only apply to a particular subset of data.
Monitoring and Maintaining Indexes
Monitoring and maintaining indexes is a critical component of database performance management that can often be overlooked. Indexes, while powerful tools for speeding up data retrieval, require regular attention to ensure they remain efficient and effective. Failing to monitor indexes can lead to degraded performance over time as data changes and query patterns evolve.
Tracking Index Usage
The first step in effective index maintenance is to monitor how indexes are used. Database management systems provide tools to track index usage statistics, which can help identify indexes that are rarely or never used. For instance, in SQL Server, you can execute the following query to obtain index usage statistics:
SELECT OBJECT_NAME(I.object_id) AS TableName, I.name AS IndexName, u.user_seeks, u.user_scans, u.user_lookups, u.user_updates FROM sys.indexes AS I INNER JOIN sys.dm_db_index_usage_stats AS u ON I.object_id = u.object_id AND I.index_id = u.index_id WHERE OBJECTPROPERTY(I.object_id, 'IsUserTable') = 1;
This query gives a detailed view of how often each index is utilized in various operations, which will allow you to determine which indexes can be safely dropped to improve performance and reduce maintenance overhead.
Addressing Index Fragmentation
As data is inserted, updated, and deleted, indexes can become fragmented, which negatively impacts read performance. Fragmentation occurs when the logical order of the index does not match the physical order on disk, leading to inefficient I/O operations. Regularly checking for fragmentation and addressing it through rebuilding or reorganizing indexes is essential. You can assess the fragmentation of an index with the following query:
SELECT OBJECT_NAME(object_id) AS TableName, name AS IndexName, index_id, avg_fragmentation_in_percent FROM sys.dm_db_index_physical_stats(DB_ID(), NULL, NULL, NULL, NULL) WHERE avg_fragmentation_in_percent > 10;
Once you identify fragmented indexes, you can choose to either rebuild or reorganize them. Rebuilding an index is a more resource-intensive operation but provides a more significant performance boost, especially for heavily fragmented indexes:
ALTER INDEX index_name ON table_name REBUILD;
Alternatively, if fragmentation is moderate, reorganizing the index is a lighter operation that can be performed online without extensive locks:
ALTER INDEX index_name ON table_name REORGANIZE;
Regular Maintenance Tasks
In addition to monitoring usage and fragmentation, implementing a regular maintenance schedule is vital. Scheduling tasks to rebuild or reorganize indexes during off-peak hours can help maintain performance without impacting users. You can utilize SQL Server Agent to automate these tasks, ensuring that your indexes are routinely assessed and maintained.
Monitoring Performance Impact
As you implement changes to your indexing strategy, it’s essential to monitor the impact on overall database performance. Tools such as SQL Server Profiler can help analyze query performance before and after index maintenance tasks. If you notice an improvement in query response times, you can confidently continue with your maintenance strategy. Conversely, if performance degrades, you may need to revisit your indexing decisions.
Common Pitfalls to Avoid in Indexing
Indexes, while powerful, can lead to significant performance issues if not managed correctly. One common pitfall to avoid is the creation of too many indexes. While indexes are designed to speed up data retrieval, each additional index adds overhead to write operations. When you perform an INSERT, UPDATE, or DELETE, all applicable indexes must also be updated, which can slow down these operations considerably. It’s essential to strike a balance between read and write performance based on your application’s specific usage patterns.
Another pitfall is neglecting to analyze index performance over time. As your application’s data grows and query patterns evolve, the indexes that once were effective may become less so. Regularly using tools such as sys.dm_db_index_usage_stats
in SQL Server can help identify which indexes are being used and which are not. For example, the following query can be employed to find indexes that have not been used for a significant amount of time:
SELECT OBJECT_NAME(object_id) AS TableName, name AS IndexName, user_seeks, user_scans, user_lookups, user_updates FROM sys.indexes WHERE OBJECTPROPERTY(object_id, 'IsUserTable') = 1 AND index_id > 0 AND user_seeks = 0 AND user_scans = 0;
This query helps identify candidates for removal, which can lead to reduced maintenance overhead and improved overall performance.
Index fragmentation is another significant concern that can affect performance. Over time, as data is modified, indexes can become fragmented, leading to inefficient data access patterns. Regularly checking for fragmentation and performing maintenance such as rebuilding or reorganizing indexes is vital. A common mistake is to neglect this maintenance, allowing fragmentation to accumulate and degrade performance. Here’s how you can check the fragmentation level of your indexes:
SELECT OBJECT_NAME(object_id) AS TableName, name AS IndexName, index_id, avg_fragmentation_in_percent FROM sys.dm_db_index_physical_stats(DB_ID(), NULL, NULL, NULL, NULL) WHERE avg_fragmentation_in_percent > 30;
If you detect fragmentation levels above a certain threshold, you can take action to rebuild or reorganize the index:
ALTER INDEX index_name ON table_name REBUILD;
Lastly, a frequent oversight involves overlooking the impact of covering indexes. While they can dramatically reduce the number of reads required to satisfy certain queries, they can also consume substantial additional storage and increase maintenance costs. It’s crucial to evaluate whether the performance benefits outweigh these costs for your specific queries and access patterns.