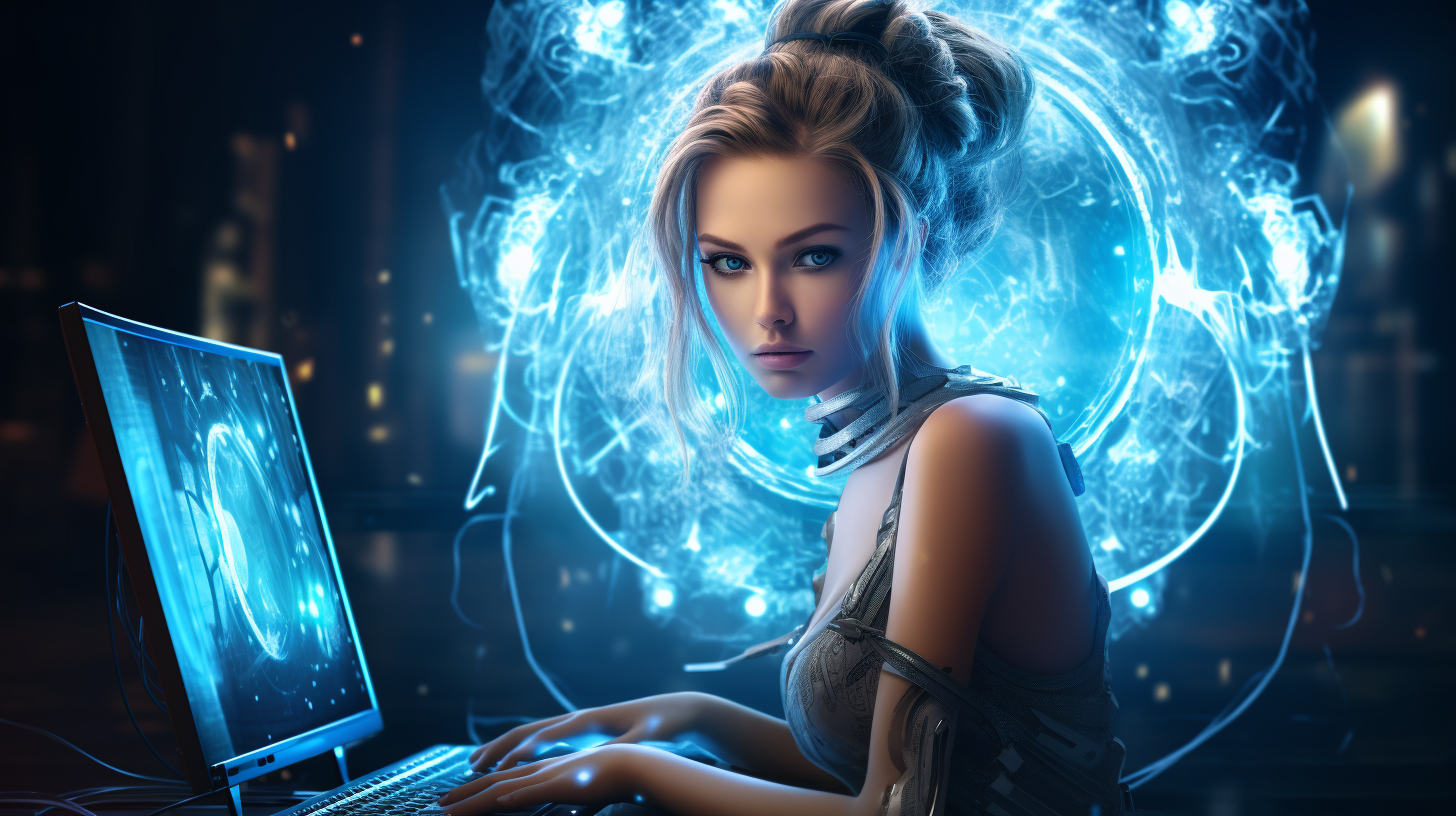
SQL for Application Logging and Monitoring
When it comes to structuring log data effectively, there are several best practices that can significantly enhance both the performance of your logging system and the clarity of the information it provides. The nuances of data organization directly impact how efficiently you can retrieve, analyze, and visualize logs.
1. Use a Consistent Schema
Establishing a uniform schema for log entries is vital. A consistent structure allows for easier querying and analysis. Ponder including fields such as:
- timestamp – When the event occurred.
- level – The severity of the log (INFO, WARN, ERROR).
- message – A description of the logged event.
- source – The application or service that generated the log.
- context – Additional data that can provide insights about the event.
The following SQL snippet demonstrates the creation of a logging table with a structured schema:
CREATE TABLE application_logs ( id SERIAL PRIMARY KEY, timestamp TIMESTAMP DEFAULT CURRENT_TIMESTAMP, level VARCHAR(10), message TEXT, source VARCHAR(50), context JSONB );
2. Normalize Log Data
Normalization can reduce redundancy and improve data integrity. When logs contain repetitive data, think creating separate tables to store common information. For example, if multiple logs share the same source, you might create a sources
table.
CREATE TABLE sources ( id SERIAL PRIMARY KEY, name VARCHAR(50) UNIQUE ); CREATE TABLE application_logs ( id SERIAL PRIMARY KEY, timestamp TIMESTAMP DEFAULT CURRENT_TIMESTAMP, level VARCHAR(10), message TEXT, source_id INT REFERENCES sources(id), context JSONB );
3. Indexing for Performance
Optimizing your log data retrieval requires careful indexing. By indexing frequently queried fields, such as timestamp
and level
, you can drastically improve query performance. Here’s how to create an index on the timestamp
column:
CREATE INDEX idx_timestamp ON application_logs (timestamp);
4. Archiving Old Logs
Over time, log tables can grow significantly. Implementing an archiving strategy can help manage this growth. Consider moving older logs to a separate archive table or database. This makes the main table more performant while ensuring that historical data is still accessible when needed.
CREATE TABLE archived_logs AS SELECT * FROM application_logs WHERE timestamp < NOW() - INTERVAL '6 months'; DELETE FROM application_logs WHERE timestamp < NOW() - INTERVAL '6 months';
5. Enriching Logs with Metadata
Adding contextual metadata can provide deeper insights during log analysis. Fields like user ID, session ID, or transaction ID can help trace the flow of operations across different components of your application. This metadata can be stored in the context
field as a JSON object, allowing for flexible structures.
Implementing SQL Queries for Log Analysis
Once you’ve established a solid foundation for your log data structure, the next crucial step is implementing SQL queries that facilitate effective log analysis. These queries will enable you to extract meaningful insights from your logs, identify patterns, and troubleshoot issues within your application. Below are some essential SQL queries tailored for log analysis that can help you gain valuable insights.
1. Retrieving Recent Logs
To start with, you might want to query the most recent log entries to get an overview of what’s happening in your application. This simple SQL statement retrieves the last 50 logs, sorted by the timestamp:
SELECT * FROM application_logs ORDER BY timestamp DESC LIMIT 50;
2. Filtering by Log Level
When you are interested in specific issues, filtering logs by severity level especially important. For example, if you want to analyze only ERROR logs, you can use the following query:
SELECT * FROM application_logs WHERE level = 'ERROR' ORDER BY timestamp DESC;
3. Aggregating Log Counts
Understanding the frequency of log entries can provide insights into application behavior. You can count the number of logs by their level with the following query:
SELECT level, COUNT(*) AS log_count FROM application_logs GROUP BY level ORDER BY log_count DESC;
4. Analyzing Logs Over Time
To visualize trends, you may want to analyze log entries over time. The following SQL query aggregates log counts by day:
SELECT DATE(timestamp) AS log_date, COUNT(*) AS log_count FROM application_logs GROUP BY log_date ORDER BY log_date DESC;
5. Searching for Specific Messages
If you’re troubleshooting a specific issue, searching for keywords in log messages can be incredibly useful. The following query demonstrates how to find logs containing the word ‘timeout’:
SELECT * FROM application_logs WHERE message ILIKE '%timeout%' ORDER BY timestamp DESC;
6. Joining Logs with Metadata
To enrich your analysis, you can join your log data with other tables that contain relevant metadata. For instance, if you want to include source names alongside log entries, you might execute:
SELECT al.timestamp, al.level, al.message, s.name AS source_name FROM application_logs al JOIN sources s ON al.source_id = s.id ORDER BY al.timestamp DESC;
7. Identifying High-Volume Sources
Lastly, to pinpoint which application sources are generating the most logs, you can use this query:
SELECT s.name, COUNT(*) AS log_count FROM application_logs al JOIN sources s ON al.source_id = s.id GROUP BY s.name ORDER BY log_count DESC;
Monitoring Application Performance with SQL
Monitoring application performance through SQL goes beyond merely collecting log data; it involves actively analyzing and interpreting that data to derive actionable insights. By using SQL’s powerful querying capabilities, you can identify bottlenecks, track performance trends, and make informed decisions to optimize your application. Below are several strategies to utilize SQL for monitoring application performance.
1. Tracking Response Times
One of the critical aspects of application performance is monitoring response times. If your application logs contain duration metrics, you can easily compute average and maximum response times. For instance, if you have a field called response_time
in your logs, you could use the following query to get a sense of performance:
SELECT AVG(response_time) AS avg_response_time, MAX(response_time) AS max_response_time FROM application_logs WHERE timestamp > NOW() - INTERVAL '1 day';
This query provides a quick snapshot of how your application has performed over the last 24 hours.
2. Identifying Slow Queries
Another way to monitor application performance is to analyze which operations are taking longer than expected. If you log queries along with their execution times, you could write a query like this to find the slowest queries:
SELECT message, response_time FROM application_logs WHERE level = 'QUERY' ORDER BY response_time DESC LIMIT 10;
This will return the top 10 slowest queries, so that you can focus on optimizing them.
3. Monitoring Error Rates Over Time
Keeping tabs on the error rates is important for maintaining application health. By analyzing how error logs trend over time, you can identify unusual spikes that may indicate underlying issues. The following query summarizes the error logs by day:
SELECT DATE(timestamp) AS log_date, COUNT(*) AS error_count FROM application_logs WHERE level = 'ERROR' GROUP BY log_date ORDER BY log_date DESC;
This query helps you visualize error trends and take corrective actions if necessary.
4. Logging User Interactions
Understanding how users interact with your application can also shed light on performance. If you track user actions, you can use SQL to analyze the frequency of specific actions. For example:
SELECT message, COUNT(*) AS action_count FROM application_logs WHERE source = 'UserAction' GROUP BY message ORDER BY action_count DESC;
This will give you insights into which features are used most frequently, potentially guiding optimization efforts.
5. Alerting for Anomalies
Setting up alerts for performance anomalies can be a proactive way to maintain application health. For example, if your application generates logs for specific performance metrics, you can create a query that identifies when average response times exceed a certain threshold:
SELECT AVG(response_time) AS avg_response_time FROM application_logs WHERE timestamp > NOW() - INTERVAL '5 minutes' HAVING avg_response_time > 2000;
This query checks if the average response time in the last five minutes exceeds 2000 milliseconds, which will allow you to set up an alert mechanism based on the results.
6. Resource Use Monitoring
Additionally, if your application logs include resource usage metrics, you can analyze CPU and memory usage trends. The following example assumes you have logged this data:
SELECT DATE(timestamp) AS log_date, AVG(cpu_usage) AS avg_cpu_usage, AVG(memory_usage) AS avg_memory_usage FROM application_logs GROUP BY log_date ORDER BY log_date DESC;
This provides a daily overview of resource use, helping you identify patterns that may necessitate scaling operations.
Visualizing Log Data for Insights and Reporting
Visualizing log data is a powerful way to derive insights and make informed decisions about your application’s performance and behavior. SQL provides a robust foundation for querying log data, but the true value comes when you translate this data into visual representations. By using tools such as dashboards, graphs, and charts, you can create a clearer picture of application activities, trends, and anomalies. Here are some effective approaches to visualizing log data:
1. Time-Series Graphs
One of the most effective ways to visualize log data is through time-series graphs. By aggregating log entries over time, you can identify trends and patterns. For example, you can visualize the number of error logs generated each day using the following SQL query:
SELECT DATE(timestamp) AS log_date, COUNT(*) AS error_count FROM application_logs WHERE level = 'ERROR' GROUP BY log_date ORDER BY log_date ASC;
This query can be plotted on a time-series graph, so that you can observe how error occurrences fluctuate over time.
2. Pie Charts for Log Distribution
Using pie charts can effectively display the distribution of log levels within your application. This can be done by executing a query that aggregates log counts by level:
SELECT level, COUNT(*) AS log_count FROM application_logs GROUP BY level;
This result set can be visualized as a pie chart, enabling stakeholders to quickly grasp the severity of logged events and prioritize their responses accordingly.
3. Bar Graphs for Source Contributions
To understand which components or sources of your application generate the most logs, you can use bar graphs. The following SQL query gathers log counts by source:
SELECT s.name AS source_name, COUNT(*) AS log_count FROM application_logs al JOIN sources s ON al.source_id = s.id GROUP BY s.name ORDER BY log_count DESC;
Visualizing this data in a bar graph will help you identify heavy hitters in terms of log generation, allowing you to focus your optimization efforts effectively.
4. Heatmaps for Error Frequency
For more complex visualizations, heatmaps can provide a robust way to visualize the frequency of errors over time and across different application components. A query like this can help you structure the data:
SELECT DATE(timestamp) AS log_date, s.name AS source_name, COUNT(*) AS error_count FROM application_logs al JOIN sources s ON al.source_id = s.id WHERE level = 'ERROR' GROUP BY log_date, source_name ORDER BY log_date, source_name;
By mapping this data onto a heatmap, you can easily identify peak error times and specific sources that may require immediate attention.
5. Dashboards for Real-time Monitoring
Creating a centralized dashboard that aggregates various visualizations can provide a real-time overview of application health. Utilize tools like Grafana or Tableau, which can connect directly to your SQL database, to pull in data dynamically. You can create widgets that display metrics such as:
- Total number of logs
- Error rates over time
- Most active application sources
- Response time trends
By continuously updating this dashboard, teams can respond swiftly to emerging issues and track application performance in real-time.