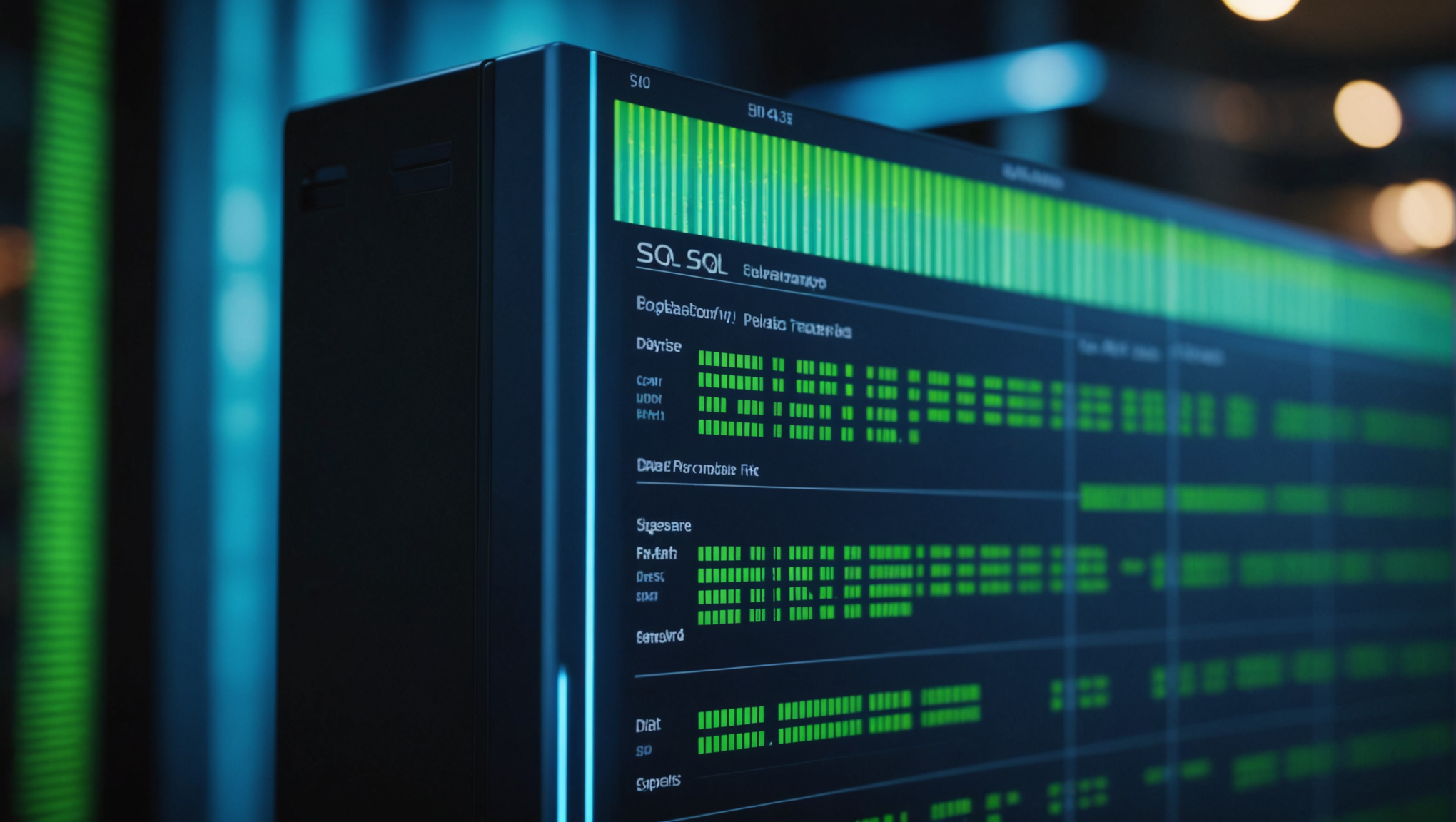
SQL for Custom Data Analysis Tools
When embarking on a journey to create custom data analysis tools, the first step is to thoroughly understand the specific requirements of the analysis. This involves defining the objectives clearly, identifying the types of data needed, and determining how that data will be processed and interpreted. Each analysis requirement is unique, influenced by the goals of the project and the specific insights sought.
To begin, ponder the following key points:
- Clearly articulate the purpose of the analysis. Are you looking to identify trends, forecast future values, or simply summarize data? The objectives will guide the entire process.
- Determine where the relevant data resides. This could involve extracting data from multiple tables, databases, or even external sources.
- Familiarize yourself with how the data is structured. Knowing the relationships between tables and the types of data available is important for crafting effective queries.
- Identify which metrics are essential for the analysis. This could include averages, counts, or other statistics that will yield meaningful insights.
- Keep in mind who will be using the analysis. What are their needs? How will they interpret the results? Their perspective can significantly impact how you structure your queries.
Once you have a foundational understanding of your analysis requirements, translating these into SQL queries becomes the next step. For instance, if your goal is to calculate the average sales per region from a sales table, you might structure your SQL query as follows:
SELECT region, AVG(sales) AS average_sales FROM sales_data GROUP BY region;
This simple query illustrates the importance of clearly defining your objectives and understanding your data structure. Here, you’re grouping sales data by region and calculating the average sales for each. Tailoring such queries to meet specific requirements can significantly enhance the effectiveness of your data analysis.
Designing SQL Queries for Specific Use Cases
Creating effective SQL queries tailored for specific use cases demands a deep understanding of both the data at your disposal and the outcomes you aim to achieve. Let’s explore several scenarios that illustrate how to design SQL queries to meet diverse analysis requirements.
Ponder a situation where you are tasked with analyzing customer behavior data to identify purchasing trends over time. Here, a time series analysis is essential. By using the DATE_TRUNC
function, you can group your results by month, allowing for a clearer visualization of trends. The query could look like this:
SELECT DATE_TRUNC('month', purchase_date) AS month, COUNT(*) AS total_purchases FROM customer_purchases GROUP BY month ORDER BY month;
This query succinctly aggregates the total number of purchases made each month. By understanding the business requirement to analyze trends, you can formulate your SQL to extract actionable insights.
Another common use case is summarizing data to derive key performance indicators (KPIs). Suppose you need to calculate the total revenue generated by each product category. Your query would employ a similar grouping method to yield the desired insights:
SELECT category, SUM(revenue) AS total_revenue FROM product_sales GROUP BY category ORDER BY total_revenue DESC;
This example emphasizes the critical role of the SUM
function in aggregating data, making it easier for stakeholders to assess which categories are driving revenue.
Additionally, when the analysis requires filtering data based on specific criteria, the WHERE
clause becomes indispensable. Let’s say you want to find all customers who made purchases over a certain threshold, such as $1000. The query would be structured as follows:
SELECT customer_id, SUM(purchase_amount) AS total_spent FROM customer_purchases GROUP BY customer_id HAVING SUM(purchase_amount) > 1000;
The HAVING
clause is essential here, which will allow you to filter the aggregated results based on the total spent. This lets you focus on a specific segment of your customer base that meets your criteria.
In scenarios involving multiple tables, using JOIN
operations very important. For example, if you need to analyze customer feedback alongside their purchase data, a query like the following can be used:
SELECT c.customer_id, AVG(f.rating) AS average_rating FROM customers c JOIN feedback f ON c.customer_id = f.customer_id GROUP BY c.customer_id;
This query combines data from the customers
and feedback
tables, providing insight into customer satisfaction relative to their purchasing behavior.
Optimizing Performance for Large Data Sets
When dealing with large data sets, optimizing performance is critical to ensure that your SQL queries run efficiently and yield results in a reasonable time frame. The sheer volume of data can lead to slow query response times and increased resource consumption. Therefore, understanding techniques for performance optimization is essential for anyone involved in custom data analysis.
One of the fundamental strategies for enhancing SQL performance is indexing. An index acts like a lookup table, allowing the SQL engine to find rows more quickly than it would by scanning the entire table. However, it’s important to use indexes judiciously, as they can slow down write operations. For example, if you frequently query a `sales` table based on the `transaction_date`, creating an index on that column can significantly speed up your query times:
CREATE INDEX idx_transaction_date ON sales(transaction_date);
Another vital consideration is the use of proper joins. When combining data from multiple tables, using the right type of join can drastically impact performance. For instance, using INNER JOINs instead of OUTER JOINs when you only need matching records can reduce the amount of data processed:
SELECT c.customer_id, SUM(s.amount) AS total_spent FROM customers c INNER JOIN sales s ON c.customer_id = s.customer_id GROUP BY c.customer_id;
Furthermore, using database partitioning can be an effective way to manage large datasets. Partitioning divides a table into smaller, more manageable pieces, which can enhance query performance by allowing SQL to scan only the relevant partitions. For example, if you partition a large `transactions` table by year:
CREATE TABLE transactions ( transaction_id SERIAL PRIMARY KEY, transaction_date DATE, amount DECIMAL ) PARTITION BY RANGE (transaction_date);
Then, you can create partitions for each year. This way, queries targeting a specific year will skip over irrelevant partitions, leading to faster execution times.
Another optimization technique is to avoid SELECT *, which retrieves all columns from a table. Instead, specify only the columns needed for your analysis. This reduces the amount of data transferred and processed, which can significantly enhance performance:
SELECT customer_id, SUM(amount) AS total_spent FROM sales GROUP BY customer_id;
Also, consider using aggregate functions wisely. When aggregating large datasets, it’s crucial to ensure that you’re filtering out unnecessary data either through the WHERE clause or by using subqueries. For instance, if you only need sales data from the last quarter, applying this filter early can lead to substantial performance improvements:
SELECT product_id, SUM(amount) AS total_sales FROM sales WHERE transaction_date >= '2023-07-01' AND transaction_date < '2023-10-01' GROUP BY product_id;
In addition to these techniques, regularly analyzing and tuning your SQL queries using execution plans can uncover inefficiencies. Tools like EXPLAIN can provide insights into how your queries are executed and highlight potential bottlenecks:
EXPLAIN SELECT product_id, SUM(amount) AS total_sales FROM sales GROUP BY product_id;
Integrating SQL with Visualization Tools
Integrating SQL with visualization tools is a critical step in the development of custom data analysis solutions. Visualization tools provide the means to translate raw data into visual formats that can be easily interpreted and understood by users. By combining SQL with these tools, you unlock the potential to convey complex insights in a more accessible and engaging manner.
The first step in this integration process is to establish a clear connection between your SQL database and the chosen visualization tool. Many contemporary visualization platforms, such as Tableau, Power BI, and Looker, offer built-in connectors that allow users to directly query databases. This allows you to execute SQL queries and pull the resulting data for visualization without needing to write additional code. For example, in Tableau, you can connect to a PostgreSQL database and input SQL queries directly in the data source tab.
Once the connection is established, it’s essential to design your SQL queries with visualization in mind. These queries should return data in a structure that’s conducive to the types of visualizations you intend to create. For instance, if you’re planning to create a line chart to show sales trends over time, your SQL query should aggregate the data by time intervals. Here’s a sample query that prepares data for such visualization:
SELECT DATE_TRUNC('month', transaction_date) AS month, SUM(amount) AS total_sales FROM sales GROUP BY month ORDER BY month;
This SQL query aggregates sales data by month, which can be easily fed into a line chart to visualize sales trends over time. By structuring your SQL outputs meaningfully, you streamline the process of creating insightful visualizations.
In addition to raw data, many visualization tools allow for calculated fields that can further enhance the analysis. For example, if you want to visualize customer segments based on their spending behavior, you might create a SQL query that categorizes customers. Here’s an example:
SELECT customer_id, CASE WHEN SUM(amount) > 1000 THEN 'High Spender' WHEN SUM(amount) BETWEEN 500 AND 1000 THEN 'Medium Spender' ELSE 'Low Spender' END AS spending_category FROM sales GROUP BY customer_id;
This query classifies customers into spending categories, allowing visualization tools to easily create segmented views, such as pie charts or bar graphs, showcasing the distribution of customer spending behaviors.
Another important aspect of integrating SQL with visualization tools is the ability to refresh and update data dynamically. Most visualization platforms allow for scheduled refreshes, ensuring that the visualizations reflect the latest data from the SQL database. This capability is important for real-time analytics, where stakeholders rely on the most current data to make decisions. Ensure that your SQL queries are optimized, as frequent execution can strain database resources, especially with large datasets.
When dealing with interactive dashboards, think how user input can influence the SQL queries executed. Some visualization tools support parameterized queries, allowing users to filter or drill down data through interactive controls. Here’s a simplified example to illustrate this:
SELECT product_id, SUM(amount) AS total_sales FROM sales WHERE transaction_date BETWEEN :start_date AND :end_date GROUP BY product_id;
In this query, `:start_date` and `:end_date` serve as placeholders that can be dynamically replaced with user-selected dates, enabling a tailored analysis based on user input. This flexibility enhances user engagement and fosters deeper exploration of the data.