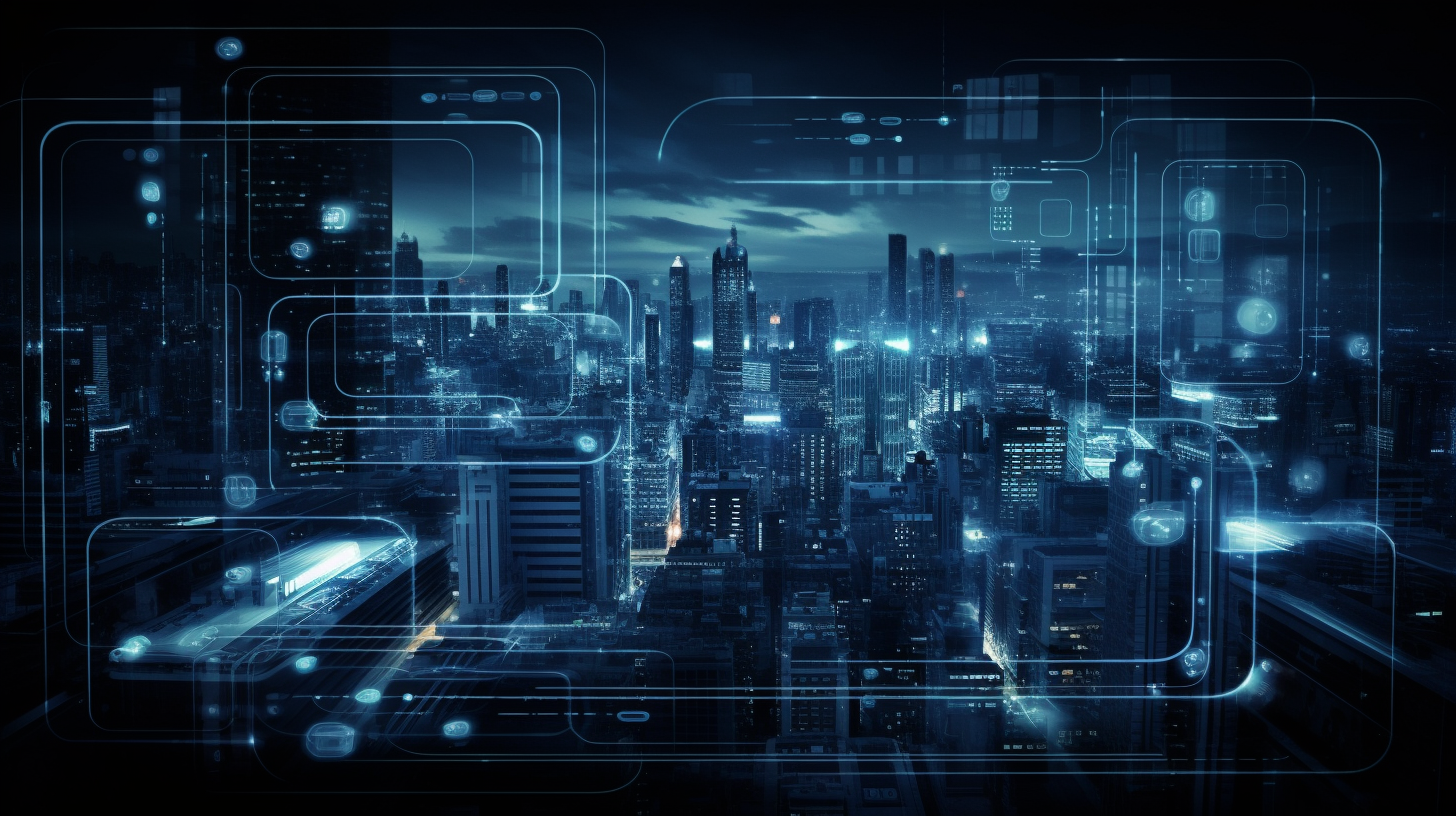
SQL for Data Cleansing and Normalization
Data cleansing is an essential part of ensuring the quality and reliability of your dataset. SQL provides various techniques that help in identifying and correcting errors, duplicates, and inconsistencies in the data. Here are some key methods for data cleansing using SQL:
- Duplicate records can skew analysis and lead to incorrect conclusions. You can identify and remove duplicates using the
ROW_NUMBER()
function in combination with a Common Table Expression (CTE).
WITH CTE AS ( SELECT *, ROW_NUMBER() OVER (PARTITION BY column1, column2 ORDER BY column3) AS RowNum FROM table_name ) DELETE FROM CTE WHERE RowNum > 1;
- Extra spaces can lead to discrepancies when comparing string values. The
TRIM()
function can be used to clean up these values.
UPDATE table_name SET column_name = TRIM(column_name);
- Consistency in data formats very important. The
CONVERT()
function can be utilized to standardize date formats or numeric values.
UPDATE table_name SET date_column = CONVERT(datetime, date_column, 101);
- It’s important to ensure that the data adheres to specified rules. For example, you can check for valid email addresses using regular expressions.
SELECT * FROM table_name WHERE column_name NOT LIKE '%_@__%.__%';
- You can employ
CASE
statements to handle specific cleansing scenarios, like converting invalid entries to a default value.
UPDATE table_name SET column_name = CASE WHEN column_name IS NULL THEN 'Default Value' ELSE column_name END;
Identifying and Handling Missing Values
Identifying missing values in a dataset is an important first step in the data cleansing process. Missing values can lead to misleading analyses, skewed results, and ultimately poor decision-making. In SQL, there are effective strategies to detect and manage these missing values, ensuring that your dataset remains robust and reliable.
Identifying Missing Values
To find missing values in your dataset, you can use the IS NULL
condition. This allows you to filter out rows where a specific column contains NULL values. For example, to identify all records in a table where the column_name
contains NULL:
SELECT * FROM table_name WHERE column_name IS NULL;
Additionally, if you want to find out how many missing values are present in a particular column, you can use the COUNT
function:
SELECT COUNT(*) FROM table_name WHERE column_name IS NULL;
Handling Missing Values
Once you have identified the missing values, you’ll need to decide how to handle them. There are several approaches, including:
1. Deletion of Rows
If a significant number of rows contain missing values in a critical column, it might be reasonable to delete them. You can execute a delete operation to remove these entries:
DELETE FROM table_name WHERE column_name IS NULL;
2. Imputation of Values
Another common approach is to impute missing values with either a default value or a calculated value, such as the average or median. This method helps maintain the dataset’s size while improving data quality. For example, to replace NULL values in a numeric column with the column’s average:
UPDATE table_name SET column_name = (SELECT AVG(column_name) FROM table_name) WHERE column_name IS NULL;
3. Using Conditional Statements
You can also use CASE
statements to handle missing values selectively. Here’s how you can replace NULL values with descriptive defaults:
UPDATE table_name SET column_name = CASE WHEN column_name IS NULL THEN 'Default Value' ELSE column_name END;
4. Flagging Missing Values
In certain scenarios, you might want to keep the original data intact and simply flag the missing values for further analysis. You can create an additional column to indicate whether the value was missing:
ALTER TABLE table_name ADD is_missing BIT; UPDATE table_name SET is_missing = CASE WHEN column_name IS NULL THEN 1 ELSE 0 END;
Normalization Methods in SQL
Normalization is a critical process in database design and data management, aimed at reducing data redundancy and ensuring data integrity. SQL provides various methods for normalization, which will allow you to structure your data efficiently. Here are some common normalization methods that can be implemented using SQL.
1. First Normal Form (1NF): To achieve 1NF, you must ensure that each column in a table contains atomic values, and each record is unique. You can use the GROUP BY
clause in conjunction with aggregation functions to identify non-atomic values.
SELECT column1, MIN(column2) AS column2 FROM table_name GROUP BY column1;
2. Second Normal Form (2NF): To move to 2NF, you need to ensure that all non-key attributes are fully functionally dependent on the primary key. If partial dependencies exist, you can create separate tables for those attributes. For instance, if you have a table that includes customer and order data, you may want to split them into two tables.
-- Create a customers table CREATE TABLE customers ( customer_id INT PRIMARY KEY, customer_name VARCHAR(100), contact_info VARCHAR(100) ); -- Create an orders table CREATE TABLE orders ( order_id INT PRIMARY KEY, customer_id INT, order_date DATE, FOREIGN KEY (customer_id) REFERENCES customers(customer_id) );
3. Third Normal Form (3NF): Achieving 3NF involves ensuring that all columns are not only dependent on the primary key but also that they do not depend on other non-key attributes. To normalize to 3NF, you might need to remove transitive dependencies. This can be accomplished by creating additional tables.
-- Create a separate table for product details CREATE TABLE products ( product_id INT PRIMARY KEY, product_name VARCHAR(100), product_price DECIMAL(10, 2) ); -- Modify orders table to reference products ALTER TABLE orders ADD product_id INT, ADD FOREIGN KEY (product_id) REFERENCES products(product_id);
4. Boyce-Codd Normal Form (BCNF): BCNF is a stricter version of 3NF and requires that every determinant in the table is a candidate key. If you have a situation where a non-prime attribute determines another non-prime attribute, you’ll need to decompose the table further. This often requires creating new tables to maintain the dependencies accurately.
-- If employee roles can determine departments, you may need to create a separate table CREATE TABLE roles ( role_id INT PRIMARY KEY, role_name VARCHAR(100) ); -- Associating employees with roles CREATE TABLE employee_roles ( employee_id INT, role_id INT, FOREIGN KEY (employee_id) REFERENCES employees(employee_id), FOREIGN KEY (role_id) REFERENCES roles(role_id) );
Best Practices for Data Integrity and Consistency
Maintaining data integrity and consistency is paramount in any database management system. As data is entered, modified, or deleted, it’s crucial to ensure that the database remains valid and reliable. Here are some best practices you can adopt using SQL to enhance data integrity and consistency.
1. Use Constraints: Constraints are a powerful feature that restrict the types of data that can be entered into a table. Common types of constraints include NOT NULL, UNIQUE, PRIMARY KEY, and FOREIGN KEY. Implementing these constraints helps prevent invalid data entries. For example, to make sure that a column for email addresses is unique, you can create a UNIQUE constraint:
ALTER TABLE users ADD CONSTRAINT unique_email UNIQUE (email);
2. Implement Foreign Key Relationships: Foreign keys ensure that relationships between tables remain valid. By referencing primary keys in other tables, you enforce referential integrity. Here’s how to set up a foreign key relationship:
CREATE TABLE orders ( order_id INT PRIMARY KEY, user_id INT, FOREIGN KEY (user_id) REFERENCES users(user_id) );
This ensures that every order is linked to a valid user, preventing orphan records in the orders table.
3. Utilize Transactions: Transactions allow you to group multiple SQL statements into a single unit of work. This ensures that either all changes are committed, or none are, preserving data integrity in case of errors. Here’s an example:
BEGIN TRANSACTION; UPDATE users SET balance = balance - 100 WHERE user_id = 1; UPDATE users SET balance = balance + 100 WHERE user_id = 2; COMMIT;
In this case, both updates must succeed; otherwise, the transaction can be rolled back to maintain consistency.
4. Regularly Back Up Your Data: Backing up your database is essential to protect against data loss. Even with constraints and transactions, unforeseen issues can arise. A regular backup strategy ensures you can recover your data in case of corruption or accidental deletion.
5. Normalize Your Database Structure: As discussed previously, normalization reduces data redundancy and improves data integrity. By organizing your tables appropriately, you minimize the risk of inconsistent data. Ensuring that your database adheres to normalization rules will help maintain a clean and efficient structure.
6. Use Triggers for Automatic Actions: Triggers can automate certain actions when specific events occur in the database, such as inserting, updating, or deleting records. For example, you can create a trigger to ensure that no user can set a balance that would cause their account to go negative:
CREATE TRIGGER prevent_negative_balance BEFORE UPDATE ON users FOR EACH ROW BEGIN IF NEW.balance < 0 THEN SIGNAL SQLSTATE '45000' SET MESSAGE_TEXT = 'Balance cannot be negative'; END IF; END;
This ensures that data integrity is preserved without requiring manual checks after each transaction.
7. Regularly Audit Data: Conducting regular audits of your data can help identify and rectify inconsistencies. Write SQL queries to check for anomalies, such as duplicate entries, invalid values, or records that don’t meet certain criteria.
SELECT email, COUNT(*) FROM users GROUP BY email HAVING COUNT(*) > 1;