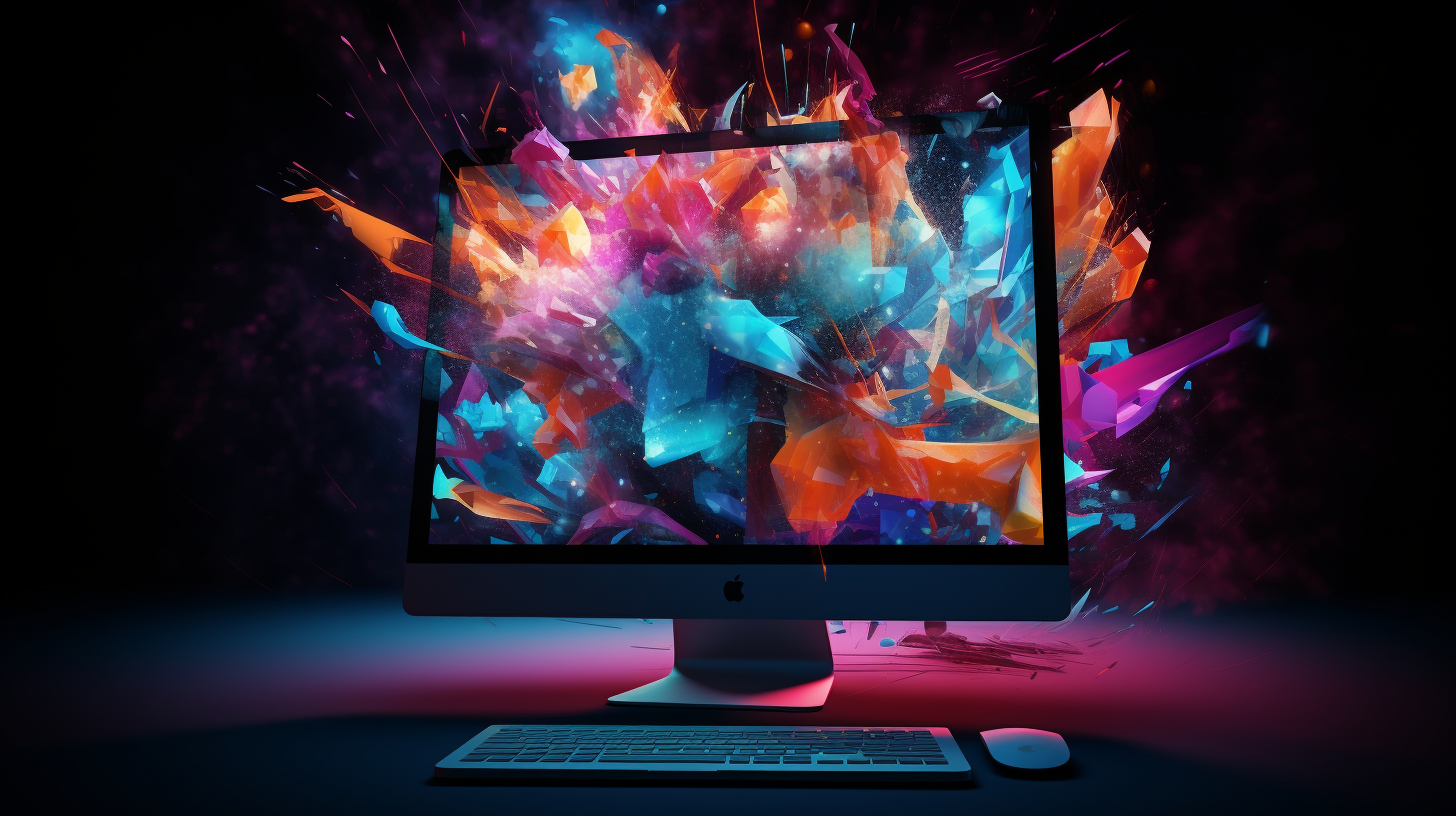
SQL for Data Compression and Storage
Data compression in SQL is an essential technique that optimizes storage by reducing the size of data stored within a database. Understanding the fundamentals of data compression techniques very important for any database administrator or developer looking to improve performance and save space.
At its core, data compression transforms data into a format that occupies less physical space on disk. SQL databases leverage various algorithms and methods to achieve this goal. The most common techniques involve lossless compression, which ensures that the original data can be perfectly reconstructed from the compressed data.
There are several approaches to data compression in SQL databases, including:
- This method compresses data at the row level. Each row is stored in a compact format, often by eliminating redundancies within the row itself.
- This approach compresses data at the page level, which is a collection of rows. It uses techniques like dictionary encoding and prefix sum to reduce size more effectively across multiple rows.
- Particularly useful in analytical databases, column-level compression compresses data within each column separately. This is beneficial for columns with similar data types, allowing for more efficient storage.
- Some databases allow for a combination of algorithms that can apply different methods depending on the data type and access patterns.
In SQL Server, for example, you can utilize built-in commands to implement compression. Here’s a basic SQL command to enable row-level compression:
ALTER TABLE YourTableName REBUILD WITH (DATA_COMPRESSION = ROW);
In addition to SQL Server, other databases like MySQL and PostgreSQL also provide various options for data compression, though the specific implementations may vary.
Understanding these techniques is just the first step. Each method has its own strengths and weaknesses, which can significantly influence performance based on the specific use case. Choosing the right data compression technique often involves balancing storage savings against the potential impact on query performance.
As databases continue to grow in size and complexity, the role of data compression becomes increasingly important, making it a critical skill for developers and DBAs alike.
Types of Data Compression in SQL Databases
Row-Level Compression is one of the most simpler techniques employed in SQL databases. By compressing data at the row level, it focuses on optimizing the storage of individual rows. This method works effectively by recognizing and removing redundancies within a single row, allowing for more compact storage without sacrificing data integrity. For instance, if multiple rows contain similar values, row-level compression can significantly reduce the amount of space those rows occupy.
On the other hand, Page-Level Compression takes a broader approach by compressing data at the page level, which is essentially a collection of multiple rows. This technique not only eliminates redundancy within individual rows but also across multiple rows within the same page. It employs algorithms such as dictionary encoding, which replaces frequently occurring values with shorter representations, and prefix sum encoding, which reduces the storage size of numeric columns by storing only the differences between consecutive values. This method can lead to substantial savings in storage space, especially in large tables.
Column-Level Compression is particularly advantageous in analytical environments where data is organized by columns rather than rows. By compressing data within each column separately, this method capitalizes on the homogeneity of data types, which often leads to more effective compression. For example, if a column contains a large number of repeated values or similar types, column-level compression can efficiently encode this data, leading to reduced disk space usage and potentially improved query performance due to reduced I/O operations.
For those databases that support it, Hybrid Compression combines various compression techniques, applying the most suitable method based on specific data types and access patterns. This flexibility allows database administrators to tailor compression strategies for different scenarios, maximizing both storage efficiency and performance.
Implementing these compression techniques in SQL Server is simpler. Here’s how to enable page-level compression for a given table:
ALTER TABLE YourTableName REBUILD WITH (DATA_COMPRESSION = PAGE);
In MySQL, you can leverage the InnoDB storage engine to apply compression at the table level. For instance, you can create a compressed table with the following SQL command:
CREATE TABLE YourTableName ( ID INT, Data VARCHAR(255) ) ENGINE=InnoDB ROW_FORMAT=COMPRESSED;
PostgreSQL also offers compression through its TOAST (The Oversized-Attribute Storage Technique). This mechanism automatically compresses large field values to optimize storage without requiring explicit commands from the user. However, users can influence this behavior by altering the storage parameters for specific columns.
Understanding the various types of data compression available in SQL databases is fundamental for optimizing storage and enhancing performance. Each method brings its unique advantages, and the choice of which to use should be guided by the specific needs of the application and data characteristics.
Benefits of Data Compression for Storage Efficiency
Data compression offers a multitude of benefits for storage efficiency that can significantly impact the performance and cost-effectiveness of SQL database management. By reducing the physical footprint of data, organizations can save on storage costs, improve processing speeds, and enhance overall system performance.
First and foremost, one of the most apparent advantages of data compression is the reduction in storage space usage. That’s particularly crucial in environments where data volumes are constantly increasing. For instance, when using row-level or page-level compression techniques, databases can effectively shrink the size of large tables, leading to lower storage costs. The following example demonstrates how to apply row-level compression to a table, enabling more efficient use of disk space:
ALTER TABLE YourTableName REBUILD WITH (DATA_COMPRESSION = ROW);
Moreover, a smaller data footprint means that backups and data transfers are faster and require less bandwidth. This can lead to significant time savings during routine maintenance operations, such as backup and restore processes, especially for large datasets. In addition, as compressed data takes up less room, it can also lead to lower costs associated with cloud storage solutions, where payment is often based on the amount of data stored.
Another significant benefit of data compression is the potential for improved performance in data retrieval and query execution. Compressed data reduces the amount of I/O required to read data from disk, as fewer bytes need to be loaded into memory. This can result in faster query response times, particularly for read-heavy workloads. For example, with page-level compression, multiple rows within a page can be compressed together, allowing the database management system (DBMS) to retrieve and process data more efficiently:
ALTER TABLE YourTableName REBUILD WITH (DATA_COMPRESSION = PAGE);
This enhanced performance can be critical in environments that require real-time analytics or support high transaction volumes. Additionally, the reduced amount of data being moved in memory can also lead to decreased memory usage, allowing the server to allocate resources more effectively across various processes.
Furthermore, data compression can contribute to better cache use. Since compressed data occupies less space, the database engine can store more data in the cache. This means that frequently accessed data is more likely to be found in memory, which dramatically speeds up access times and reduces the need for disk reads. Better cache use can lead to notable performance improvements, especially in high-throughput applications.
However, it’s essential to think the trade-offs involved. While compressed data reduces storage usage and can enhance performance, it can also introduce a CPU overhead for decompressing data during retrieval operations. Therefore, understanding the workload characteristics very important to finding the right balance between compression and performance. For instance, in analytical databases where read operations are predominant, the benefits of compression often outweigh the costs associated with decompression.
The benefits of data compression for storage efficiency in SQL databases are manifold. By effectively reducing storage space, speeding up data retrieval, and maximizing cache use, organizations can achieve significant enhancements in performance while managing costs. As database environments continue to evolve, using these compression techniques becomes essential for optimizing data management strategies.
Implementing SQL Data Compression: Step-by-Step Guide
Implementing SQL data compression is a systematic process that begins with selecting the appropriate compression technique based on your data characteristics and usage patterns. This section provides a step-by-step guide to effectively applying data compression in SQL databases, focusing primarily on SQL Server, MySQL, and PostgreSQL as examples.
Firstly, assess your database and identify tables that would benefit the most from compression. Look specifically for large tables with lots of repeated values or where I/O performance can be improved. Once you have identified these tables, you can begin the implementation process.
For SQL Server, the implementation process typically starts with enabling data compression at the table or index level. Here is how you can enable row-level compression:
ALTER TABLE YourTableName REBUILD WITH (DATA_COMPRESSION = ROW);
This command reconstructs the table with the specified compression type. It is essential to note that this operation can lead to increased resource use, so it’s advisable to perform it during off-peak hours to minimize the impact on your system’s performance.
For page-level compression, you can use a similar command:
ALTER TABLE YourTableName REBUILD WITH (DATA_COMPRESSION = PAGE);
This command enables page compression, which is particularly useful for tables with many rows. To verify the compression settings post-implementation, you can run the following command:
SELECT OBJECT_NAME(object_id) AS TableName, name AS IndexName, type_desc AS IndexType, data_compression AS CompressionType FROM sys.indexes WHERE OBJECT_NAME(object_id) = 'YourTableName';
Moving on to MySQL, if you are using the InnoDB storage engine, you can create a compressed table with the following command:
CREATE TABLE YourTableName ( ID INT, Data VARCHAR(255) ) ENGINE=InnoDB ROW_FORMAT=COMPRESSED;
This command sets the row format to compressed, reducing the storage footprint right from the moment of table creation. If you need to convert an existing table to use compressed storage, you can alter the table as follows:
ALTER TABLE YourTableName ROW_FORMAT=COMPRESSED;
For PostgreSQL, the TOAST mechanism automatically compresses large field values. However, for explicit control over compression, you can define specific column storage options. For instance:
CREATE TABLE YourTableName ( ID SERIAL PRIMARY KEY, Data TEXT ) WITH (autovacuum_enabled = true, toast_tuple_target = 2048);
In this command, you can adjust the TOAST settings to influence the compression behavior based on your performance and storage needs. Additionally, for existing tables, you can use the ALTER TABLE command to modify storage parameters, thereby enabling compression for specific columns.
Once you have implemented compression, it very important to perform regular maintenance. Monitor the performance of your compressed tables and assess their impact on query response times. Utilize SQL performance monitoring tools to analyze I/O load and cache hit ratios. This will help you identify whether the benefits of compression outweigh any potential CPU overhead incurred during data retrieval.
The implementation of SQL data compression involves careful planning and execution. By understanding the specific requirements of your database environment and following these systematic steps, you can effectively optimize storage and enhance performance through data compression.
Performance Considerations When Using Data Compression
When implementing data compression in SQL databases, it very important to keep in mind the potential performance implications that may arise from compressed data usage. While compression techniques can significantly enhance storage efficiency and reduce I/O operations, they may also introduce overhead that impacts the overall system performance. Understanding these trade-offs is essential for making informed decisions about when and how to apply data compression.
One of the primary considerations is the CPU overhead associated with compressing and decompressing data. Compression algorithms, while effective in reducing storage size, require additional processing power during data retrieval operations. This can lead to slower response times if the overhead outweighs the benefits gained from reduced I/O. For example, when retrieving compressed data, the database management system must first decompress the data before it can be processed, which adds latency to query execution.
To illustrate this, think the following scenario: if you have a table that is frequently accessed for read operations, the performance impact of decompression might be negligible compared to the savings realized from reduced disk access time. However, in a write-heavy environment, where data is frequently inserted or updated, the cost of compression can become more pronounced, potentially leading to increased transaction times and reduced throughput. In such cases, evaluating whether the storage savings justify the performance hit is critical.
SELECT COUNT(*) AS TotalRows, AVG(LEN(Data)) AS AvgRowLength, SUM(LEN(Data)) AS TotalDataSize FROM YourTableName;
This SQL command can help you analyze the storage characteristics of your table, providing insights into average row lengths and total data size. Such metrics can guide decisions on whether to compress tables, especially when considering the performance overhead of compression algorithms.
Another essential factor to weigh is the impact on query performance. While compressed data can reduce the amount of data that needs to be read from disk, it can also lead to increased computational requirements for extracting and processing that data. It is advisable to conduct performance testing on a subset of your data to evaluate how compression affects specific queries. This can help pinpoint any operations that may experience significant slowdowns due to decompression overhead.
EXEC sp_spaceused 'YourTableName';
This command can be used to check the space usage of the specified table before and after implementing compression. It provides a clear indication of the storage savings achieved, allowing you to assess whether the benefits of compression are worth the potential performance trade-offs.
Furthermore, ponder how your database’s workload will influence performance outcomes. In environments where analytical queries dominate, the advantages of compression often outweigh the costs, as the reduced I/O can lead to faster query execution times. Conversely, in transactional systems where real-time data access is critical, careful consideration of compression settings is necessary to avoid negatively impacting user experience.
Lastly, it’s important to maintain an ongoing evaluation of your compression strategies. Regularly monitoring performance metrics and conducting tests as changes occur in data volume and access patterns will help ensure that the benefits of compression remain aligned with your operational goals. This dynamic approach allows for adjustments to be made in response to evolving workloads, ultimately optimizing both storage efficiency and performance.
Best Practices for Maintaining Compressed Data
Maintaining compressed data in SQL databases is critical for ensuring that the advantages of compression persist over time. As data environments evolve, so too do the challenges associated with managing compressed data effectively. Here are some best practices to follow to ensure optimal performance and storage efficiency when working with compressed data.
Regular Monitoring and Analysis
Establish a routine for monitoring the performance of your compressed tables. Utilize SQL performance monitoring tools to examine query response times, CPU usage, and I/O statistics. By analyzing these metrics, you can determine the impact of compression on your database’s performance and make informed decisions on whether adjustments are necessary.
SELECT OBJECT_NAME(object_id) AS TableName, data_compression AS CompressionType, SUM(reserved_page_count) * 8 AS ReservedSpaceKB, SUM(used_page_count) * 8 AS UsedSpaceKB FROM sys.dm_db_partition_stats GROUP BY object_id, data_compression;
This query provides insights into how much space is being reserved and used by compressed tables, serving as a foundation for further performance tuning.
Evaluate Compression Settings Periodically
As data patterns and access behaviors change, the effectiveness of your chosen compression method may fluctuate. Regularly reevaluate your compression settings, especially after significant data growth or changes in usage patterns. This can be achieved by testing various compression options, such as switching from row-level to page-level compression or adjusting the compression algorithm if supported by your SQL database.
ALTER TABLE YourTableName REBUILD WITH (DATA_COMPRESSION = PAGE);
Implementing this command allows you to switch to a more efficient compression method based on your analysis.
Backup and Recovery Considerations
Compressed data can complicate backup and recovery processes, particularly in environments that rely heavily on incremental or differential backups. Ensure that your backup strategies take into account the specifics of compressed data, as it may require additional planning to optimize backup performance and recovery times. Regularly test your backup and recovery process to confirm that compressed tables can be restored efficiently.
BACKUP DATABASE YourDatabaseName TO DISK = 'C:BackupYourDatabaseName.bak' WITH COMPRESSION;
This command enables compressed backups, so that you can save storage space while ensuring that recovery processes remain efficient.
Data Integrity and Decompression
While SQL compression techniques are designed to maintain data integrity, it is vital to perform routine checks to ensure that data remains intact and accessible. Implement automated processes for data validation and consistency checks to catch any issues that may arise due to decompression errors or data corruption. Regularly running integrity checks can prevent long-term problems that could arise from corrupted compressed data.
DBCC CHECKTABLE('YourTableName');
This command checks the integrity of the specified table, providing peace of mind regarding the state of your compressed data.
Optimize Query Performance
As you maintain compressed data, also keep a close eye on how queries are executed against that data. In some cases, the overhead of decompressing data during reads can lead to suboptimal performance. Analyze your most common queries and adjust indexing strategies to complement compression techniques. Ensure that appropriate indexes are in place, as they can significantly enhance query performance even when accessing compressed data.
CREATE INDEX IX_YourIndexName ON YourTableName (YourColumnName);
By implementing the right indexes, you can help mitigate the performance hit from decompression, thus improving overall query response times.
Documentation and Change Management
Finally, maintain thorough documentation of your compression strategies, settings, and performance evaluations. This documentation can serve as a valuable resource when troubleshooting issues or making future adjustments. Establish a change management process to track modifications to compression settings, ensuring that all changes are logged and communicated among team members.
By adhering to these best practices, database administrators can effectively maintain compressed data, using its benefits while minimizing potential pitfalls. Regular assessment, optimization, and rigorous management of compressed data will ultimately lead to enhanced database performance and more efficient storage management.