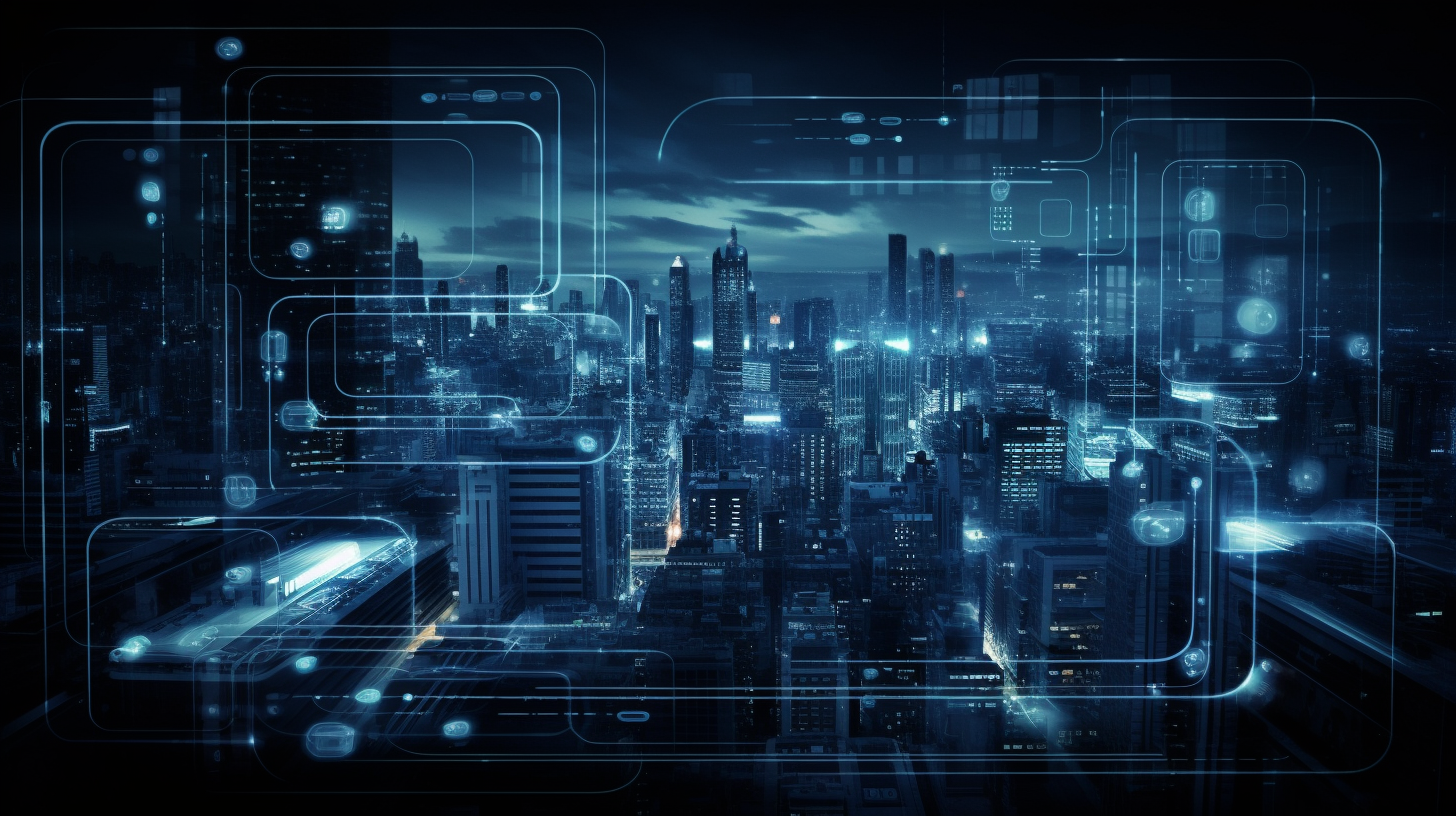
SQL for Data Distribution Analysis
Data distribution in SQL refers to how data values are spread across a range of categories or numerical intervals within a dataset. Understanding this distribution is critical for data analysis, as it can reveal insights into the underlying trends, patterns, and anomalies present in the dataset. In SQL, this analysis can be achieved using various functions and techniques that allow for the examination of both categorical and continuous data.
One of the foundational concepts in data distribution analysis is the frequency distribution, which illustrates how often each value or range of values occurs in a dataset. Frequency distributions can be visualized through histograms or other graphical representations, but in SQL, you can also query this distribution directly.
To get started with understanding data distribution, think the following SQL query that calculates the frequency of occurrences for each category in a sample dataset:
SELECT category, COUNT(*) AS frequency FROM sales_data GROUP BY category ORDER BY frequency DESC;
This query groups the data by the category field and counts the number of occurrences of each category, allowing you to see which categories are most common within the dataset.
When dealing with continuous data, it’s essential to create bins or intervals to analyze the distribution effectively. Binning allows the conversion of continuous data into categorical data based on specified ranges. Here’s how you can create a histogram in SQL:
SELECT CASE WHEN sales_amount < 100 THEN '0-99' WHEN sales_amount BETWEEN 100 AND 199 THEN '100-199' WHEN sales_amount BETWEEN 200 AND 299 THEN '200-299' ELSE '300+' END AS sales_range, COUNT(*) AS frequency FROM sales_data GROUP BY sales_range ORDER BY sales_range;
In this example, the sales_amount values are grouped into bins, and the frequency count of each bin is calculated. This distribution will help you understand how sales are spread across different ranges.
Another crucial aspect of data distribution analysis is the identification of measures of central tendency, such as the mean, median, and mode, as well as measures of variability like standard deviation and variance. These statistics provide additional context to the distribution of data and can be computed easily in SQL:
SELECT AVG(sales_amount) AS mean, MEDIAN(sales_amount) AS median, MODE() WITHIN GROUP (ORDER BY sales_amount) AS mode, STDDEV(sales_amount) AS standard_deviation, VARIANCE(sales_amount) AS variance FROM sales_data;
Understanding these statistical measures will help you interpret the distribution of your data more effectively, making it easier to draw meaningful conclusions and make informed decisions based on your findings.
Ultimately, grasping data distribution in SQL is about more than just calculating frequencies or averages; it’s about developing an intuition for how data behaves, preparing you to uncover deeper insights through more advanced analysis techniques.
Key SQL Functions for Distribution Analysis
Within the scope of SQL, several key functions stand out as essential tools for conducting distribution analysis. These functions not only facilitate the computation of various statistical measures but also aid in the visualization and interpretation of data distributions. Mastery of these functions very important for analysts who seek to derive meaningful insights from their datasets.
One of the most fundamental functions for distribution analysis is the COUNT() function. This function, as demonstrated earlier, provides the basis for frequency distributions. By counting occurrences of distinct values, you can quickly ascertain how data points are dispersed across categories. The GROUP BY clause is often used in conjunction with COUNT() to aggregate data effectively.
Another pivotal function is SUM(), which can be used alongside GROUP BY to analyze total values per category. For instance, you might want to know the total sales per product category:
SELECT category, SUM(sales_amount) AS total_sales FROM sales_data GROUP BY category ORDER BY total_sales DESC;
This query highlights how effectively you can derive meaningful insights by combining functions like SUM() with a grouping mechanism, allowing for a deeper understanding of the distribution of total sales across different product lines.
When working with continuous data, the NTILE() function becomes invaluable. It helps in creating quantiles, which can be particularly useful for dividing data into groups of equal size. For example, suppose you want to analyze how sales data is distributed across quartiles:
SELECT sales_amount, NTILE(4) OVER (ORDER BY sales_amount) AS quartile FROM sales_data;
This query assigns each row a quartile based on the sales amount, allowing for a nuanced understanding of how values are distributed across different segments.
Additionally, SQL provides analytical functions such as RANK() and DENSE_RANK(), which can help in categorizing data based on its rank within a dataset. For instance, to rank sales amounts while accounting for ties, you might use:
SELECT sales_amount, RANK() OVER (ORDER BY sales_amount DESC) AS sales_rank FROM sales_data;
This query provides a ranked list of sales amounts, making it easier to identify top performers and outliers within the dataset.
For a more comprehensive analysis, the PERCENTILE_CONT() function can be employed to compute specific percentiles, which can shed light on the distribution’s shape and spread. For example, to find the median sales amount, you could execute:
SELECT PERCENTILE_CONT(0.5) WITHIN GROUP (ORDER BY sales_amount) AS median_sales FROM sales_data;
This function provides a robust way to extract deeper insights into your data, particularly when considering variations in distributions and understanding central tendencies.
Incorporating these functions into your SQL arsenal will greatly enhance your ability to analyze data distributions. Whether it’s through counting occurrences, summing totals, creating quantiles, or calculating ranks and percentiles, each function plays an important role in unveiling the story behind the data. Armed with this knowledge, analysts can conduct more sophisticated investigations into distribution patterns, leading to more informed decisions and strategies.
Visualizing Data Distribution with SQL Queries
Visualizing data distribution with SQL queries is a powerful way to understand the underlying patterns and trends present in your datasets. While SQL is primarily a query language, it can still facilitate the creation of visual representations of data distributions that can be invaluable for analysis. One of the most common visualization techniques is to generate summary tables that can be further exported to visualization tools or used in reporting.
To start visualizing data distributions within SQL, you can create aggregate tables that summarize key metrics. For instance, if you want to visualize the distribution of sales across different regions, you might use the following query:
SELECT region, SUM(sales_amount) AS total_sales FROM sales_data GROUP BY region ORDER BY total_sales DESC;
This query aggregates sales amounts by region, providing a clear view of which regions contribute most to total sales. You can then take these results and feed them into a dashboard tool such as Tableau or Power BI to create bar charts or pie charts representing the sales distribution across regions.
Another effective way to visualize data distribution is through the use of percentiles and quantiles. By calculating these metrics, you can understand how your data is spread out, particularly in terms of outliers and where most of your data points lie. For example, to create a table that shows the sales data spread over a series of percentiles, you can run the following SQL query:
SELECT PERCENTILE_CONT(0.25) WITHIN GROUP (ORDER BY sales_amount) AS first_quartile, PERCENTILE_CONT(0.5) WITHIN GROUP (ORDER BY sales_amount) AS median, PERCENTILE_CONT(0.75) WITHIN GROUP (ORDER BY sales_amount) AS third_quartile FROM sales_data;
This will yield the first quartile, median, and third quartile of sales amounts, offering insight into the data’s distribution across its range. These statistics can guide further visualizations by helping to identify where the bulk of your sales data falls and where potential outliers may exist.
For visualizing frequency distributions, you might ponder using the idea of binning, as previously discussed. Once you’ve created bins, you can summarize the counts for each bin with a query like this:
SELECT CASE WHEN sales_amount < 100 THEN '0-99' WHEN sales_amount BETWEEN 100 AND 199 THEN '100-199' WHEN sales_amount BETWEEN 200 AND 299 THEN '200-299' ELSE '300+' END AS sales_range, COUNT(*) AS frequency FROM sales_data GROUP BY sales_range ORDER BY sales_range;
After executing this query, you’ll have a frequency distribution table that can easily be transformed into a histogram visualization in a reporting tool. This representation aids in quickly grasping how sales amounts are distributed across various ranges, highlighting trends and potential areas for performance improvement.
Furthermore, when comparing distributions between two or more datasets, you might want to visualize the results side by side. Ponder the following example that compares sales distributions across two different years:
SELECT YEAR(sale_date) AS sale_year, CASE WHEN sales_amount < 100 THEN '0-99' WHEN sales_amount BETWEEN 100 AND 199 THEN '100-199' WHEN sales_amount BETWEEN 200 AND 299 THEN '200-299' ELSE '300+' END AS sales_range, COUNT(*) AS frequency FROM sales_data GROUP BY sale_year, sales_range ORDER BY sale_year, sales_range;
This query allows you to analyze how the frequency of sales amounts changes over the years. By exporting the results, you can create a stacked bar chart or a line graph to visualize trends across multiple years, helping to pinpoint shifts in customer behavior or market conditions.
When performing data distribution analysis in SQL, the key to effective visualization lies in aggregating your data thoughtfully, using SQL’s capabilities for grouping and summarizing. By transforming raw data into summarized forms, you can leverage external tools to create visually compelling and interpretable graphics that tell the story behind your data distributions.
Comparing Distribution Across Different Datasets
When comparing distribution across different datasets, it is essential to employ SQL techniques that allow for a comprehensive analysis of how different segments of data relate to one another. This can involve comparing distributions based on categorical variables or assessing shifts in continuous variables across separate datasets. The comparison can reveal meaningful insights about trends, anomalies, and variations that exists between datasets.
To begin with, consider you have multiple sales datasets from different time periods. A simpler way to compare their distributions is by aggregating data using a common structure, such as sales ranges. For example, if you want to compare how sales amounts are distributed across two separate years, you could utilize the following query:
SELECT YEAR(sale_date) AS sale_year, CASE WHEN sales_amount < 100 THEN '0-99' WHEN sales_amount BETWEEN 100 AND 199 THEN '100-199' WHEN sales_amount BETWEEN 200 AND 299 THEN '200-299' ELSE '300+' END AS sales_range, COUNT(*) AS frequency FROM sales_data WHERE YEAR(sale_date) IN (2022, 2023) GROUP BY sale_year, sales_range ORDER BY sale_year, sales_range;
This query groups data by year and defined sales ranges, which will allow you to see the frequency of sales amounts for each category in both years. The output can then be visualized, for instance, through side-by-side bar charts that illustrate how sales behaviors differ from one year to the next.
Another method for comparison is to calculate statistics such as averages, medians, and standard deviations for each dataset. This provides a quantitative basis for comparing distributions beyond mere frequencies. Here’s how you can achieve this:
SELECT YEAR(sale_date) AS sale_year, AVG(sales_amount) AS average_sales, MEDIAN(sales_amount) AS median_sales, STDDEV(sales_amount) AS sales_standard_deviation FROM sales_data WHERE YEAR(sale_date) IN (2022, 2023) GROUP BY sale_year;
This query calculates average sales, median sales, and standard deviation for two years, reflecting central tendency and dispersion within the datasets. Comparing these statistics can help you ascertain not only differences in overall performance but also the variability of sales within each year.
Additionally, when comparing categorical distributions, you may want to analyze how different categories contribute to the overall distribution across datasets. For example, if you have product categories and you want to see how their sales distributions vary year over year, consider the following SQL query:
SELECT category, YEAR(sale_date) AS sale_year, SUM(sales_amount) AS total_sales FROM sales_data WHERE YEAR(sale_date) IN (2022, 2023) GROUP BY category, sale_year ORDER BY category, sale_year;
This will yield total sales for each product category by year, so that you can analyze shifts in category performance over time. By exporting this result and creating a grouped bar chart, you can visualize which categories gained traction and which faced declines.
Moreover, testing hypotheses can also be an integral part of comparing distributions. For instance, you may want to perform a statistical test to determine if the distributions of two datasets are significantly different. In SQL, you might prepare the data for such a test by calculating necessary statistics:
SELECT sales_amount, ROW_NUMBER() OVER (PARTITION BY YEAR(sale_date) ORDER BY sales_amount) AS row_num, COUNT(*) OVER (PARTITION BY YEAR(sale_date)) AS total_count FROM sales_data WHERE YEAR(sale_date) IN (2022, 2023);
This query prepares the data by assigning row numbers and counting total entries for each year. These metrics can then be utilized in further statistical analysis outside of SQL to determine whether the distributions differ significantly.
Ultimately, comparing distributions across different datasets in SQL is not just about executing queries, but weaving a narrative through data. By aggregating results, calculating descriptive statistics, and employing visualizations, analysts can uncover insights that drive better decision-making and strategy formulation.
Advanced Techniques for Analyzing Distribution
Advanced techniques for analyzing data distribution in SQL involve using a variety of methods and functions that provide deeper insights into the patterns within your data. While basic aggregation and frequency distribution are important, more sophisticated statistical analyses allow for a nuanced understanding of how data behaves across different conditions and dimensions. This section delves into a series of advanced techniques that can be applied to improve distribution analysis.
One of the foremost methods is using window functions, which allow you to perform calculations across a set of rows related to the current row without collapsing the result set. This capability is particularly useful when you want to compute running totals, moving averages, or cumulative distribution functions. For example, to calculate the cumulative sales amount over a specified period, you could use the following query:
SELECT sale_date, SUM(sales_amount) OVER (ORDER BY sale_date) AS cumulative_sales FROM sales_data ORDER BY sale_date;
This query produces a cumulative sum of sales amounts, showing how sales accumulate over time. Such insights are vital for understanding trends and the impact of time on distribution.
Another advanced technique is the use of statistical tests to compare distributions. For example, if you want to determine whether the median sales amounts between two groups are significantly different, you could compute the median for each group and then apply a statistical test such as the Mann-Whitney U test. Preparing the data for this analysis in SQL might look like:
SELECT CASE WHEN region = 'North' THEN 'Group 1' ELSE 'Group 2' END AS group_label, MEDIAN(sales_amount) AS median_sales FROM sales_data GROUP BY group_label;
This will enable you to evaluate the central tendencies of different groups before moving into a more detailed analysis, such as performing the Mann-Whitney U test in an appropriate statistical software package.
Additionally, implementing bootstrapping techniques can be an innovative way to analyze the variability of your estimates. This resampling method allows you to understand the distribution of a statistic (like the mean or median) by repeatedly sampling from your dataset. While SQL is not inherently designed for such complex statistical methods, you can prepare the data set for bootstrapping with a query like this:
SELECT sales_amount FROM sales_data ORDER BY RANDOM() LIMIT 1000;
This query randomly samples 1,000 sales amounts from the dataset, which can then be used in further analysis to estimate the distribution of a statistic. By repeating this process, you can build a distribution of your statistic of interest.
Furthermore, anomaly detection techniques can be implemented directly within SQL to identify outliers in your data distribution. For instance, using Z-scores to find values that deviate significantly from the mean can help in flagging potential anomalies:
WITH stats AS ( SELECT AVG(sales_amount) AS mean, STDDEV(sales_amount) AS stddev FROM sales_data ) SELECT sales_amount, (sales_amount - mean) / stddev AS z_score FROM sales_data, stats WHERE ABS(z_score) > 3;
This query computes the Z-score for each sales amount and filters for those that are greater than three standard deviations from the mean, effectively identifying outliers. Recognizing these extreme values can provide insight into unusual events or errors in data entry.
Lastly, exploring multivariate distributions can yield deeper insights, especially when examining relationships between multiple variables. By employing techniques such as clustering or regression analysis, you can uncover patterns that might not be evident when analyzing a single variable. For example, you might want to cluster customers based on their purchase behaviors to understand different distribution patterns across demographic segments:
SELECT customer_id, AVG(sales_amount) AS avg_purchase, COUNT(*) AS purchase_count FROM sales_data GROUP BY customer_id HAVING purchase_count > 5 ORDER BY avg_purchase DESC;
This results in a summary of customer purchasing behavior, enabling segmentation for further analysis or targeted marketing efforts. Such advanced techniques allow for a more comprehensive understanding of distributions, leading to better strategic decisions based on thorough data exploration.
Case Studies: Real-World Applications of Data Distribution Analysis in SQL
Real-world applications of data distribution analysis in SQL span various industries and use cases, showcasing the versatility and power of SQL in deriving actionable insights from data. By understanding how data behaves, organizations can make informed decisions that drive business strategies. Let’s delve into some case studies that highlight the practical utility of these techniques.
In the retail industry, a major company sought to optimize its inventory management by analyzing sales distributions across various product categories. Using SQL, analysts aggregated sales data to identify which products had the highest turnover rates. The following query was used to ascertain the frequency distribution of sales amounts across different product categories:
SELECT category, SUM(sales_amount) AS total_sales FROM sales_data GROUP BY category ORDER BY total_sales DESC;
This analysis allowed the company to identify underperforming product categories, leading to strategic decisions regarding inventory restocking and promotional efforts. By understanding the distribution of sales amounts, the retailer was able to optimize stock levels, reduce waste, and increase overall profitability.
Another case study involved a financial institution focused on credit risk assessment. The organization utilized SQL to analyze the distribution of credit scores among its customers to identify potential risks. By segmenting customers into different credit score ranges, the institution could better understand its risk exposure. The following SQL query illustrated how the distribution of credit scores was categorized:
SELECT CASE WHEN credit_score < 600 THEN 'Poor' WHEN credit_score BETWEEN 600 AND 699 THEN 'Fair' WHEN credit_score BETWEEN 700 AND 799 THEN 'Good' ELSE 'Excellent' END AS credit_range, COUNT(*) AS frequency FROM customer_data GROUP BY credit_range ORDER BY frequency DESC;
This categorization enabled the financial institution to tailor its lending policies, targeting specific customer segments with appropriate risk assessments and interest rates. The institution also leveraged this data to develop risk mitigation strategies that minimized potential defaults.
In the healthcare sector, a hospital utilized SQL to analyze patient wait times across different departments. Understanding the distribution of wait times helped the administration identify bottlenecks in service delivery. The following query was employed to calculate the frequency distribution of wait times:
SELECT CASE WHEN wait_time < 15 THEN '0-14 minutes' WHEN wait_time BETWEEN 15 AND 29 THEN '15-29 minutes' WHEN wait_time BETWEEN 30 AND 59 THEN '30-59 minutes' ELSE '60+ minutes' END AS wait_time_range, COUNT(*) AS frequency FROM patient_data GROUP BY wait_time_range ORDER BY frequency DESC;
By visualizing this data, hospital administrators could prioritize staffing and streamline processes to reduce unnecessary wait times, ultimately enhancing patient satisfaction and care quality.
In the tech industry, a software company analyzed user engagement metrics to improve its product offerings. By examining the distribution of user activity across its platform, the company could identify feature usage patterns. The following SQL query was utilized to analyze user activity distribution:
SELECT user_id, COUNT(activity_id) AS activity_count FROM user_activity GROUP BY user_id HAVING activity_count > 10 ORDER BY activity_count DESC;
This analysis provided insights into which features were most popular among users, informing product development and marketing strategies. The data enabled the company to improve user experience by focusing on high-engagement features while considering potential improvements for less-utilized options.
These case studies illustrate the robust applications of SQL in analyzing data distributions across various industries. By using SQL’s capabilities, organizations can uncover significant patterns and trends, thereby making data-driven decisions that align with their strategic goals. Whether in retail, finance, healthcare, or technology, understanding data distribution is pivotal for optimizing operations and enhancing overall performance.