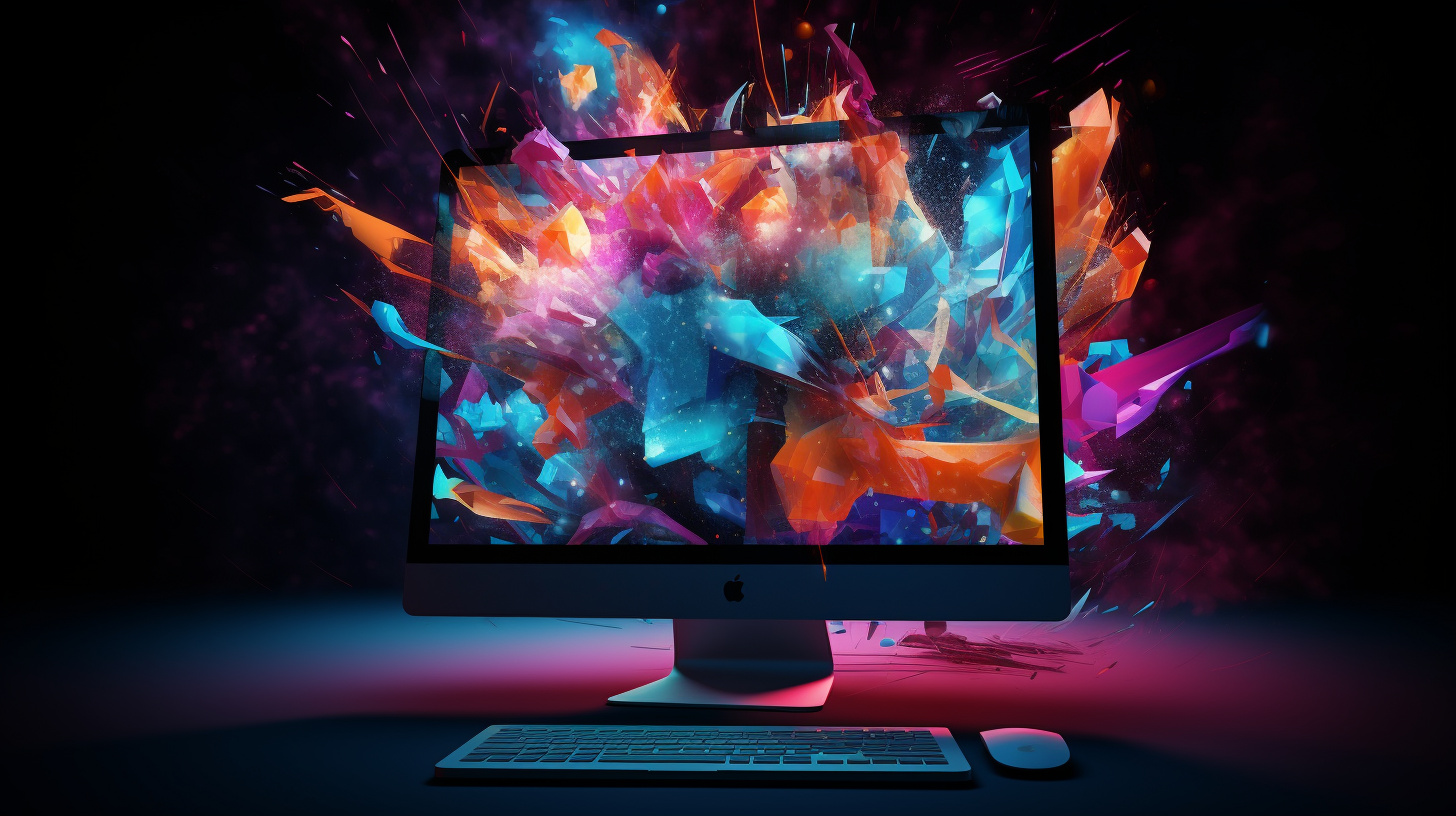
SQL for Data Integrity and Consistency
In SQL, data integrity refers to the accuracy and consistency of data stored in a database. Maintaining data integrity especially important for ensuring that the data remains reliable and trustworthy over time. Data integrity is primarily achieved through various constraints that enforce rules at the database level, preventing invalid data entry and preserving the overall quality of the dataset.
There are several types of data integrity, which can be categorized into different dimensions:
- This ensures that each table has a unique identifier, usually implemented through a primary key. As a rule, no two rows in a table should have the same primary key value.
- This maintains the consistency of the relationships between tables. Foreign keys are used to enforce referential integrity, ensuring that relationships between tables remain valid.
- This ensures that all entries in a column are valid according to the defined data type and constraints. For example, a date field should only contain date values.
- These are specific rules defined by users to meet particular business requirements. They can be implemented through check constraints or triggers.
By enforcing these integrity constraints, SQL databases can prevent the entry of invalid data, thus ensuring that the information remains accurate and consistent. That’s particularly important in applications where data accuracy is critical, such as financial systems or customer data management.
For example, let’s think a scenario where we want to ensure that the “users” table maintains entity integrity by implementing a primary key:
CREATE TABLE users ( user_id INT PRIMARY KEY, username VARCHAR(50) NOT NULL, email VARCHAR(100) NOT NULL );
Here, the user_id
serves as the primary key, ensuring that each user has a unique identifier.
To further enforce referential integrity, we can create a second table that references the user_id
from the “users” table as a foreign key:
CREATE TABLE orders ( order_id INT PRIMARY KEY, user_id INT, order_date DATE NOT NULL, FOREIGN KEY (user_id) REFERENCES users(user_id) );
In this example, the user_id
in the “orders” table must match a valid entry in the “users” table, thus ensuring that orders cannot exist without a corresponding user.
Types of Data Integrity Constraints
Data integrity constraints serve as foundational rules within a relational database, ensuring that the data adheres to specific standards and remains valid throughout its lifecycle. Each type of constraint has its role in maintaining the integrity of the data, and understanding these constraints is essential for any SQL practitioner.
Entity Integrity is established through the use of primary keys, which guarantee that each row in a table is unique and identifiable. The enforcement of this constraint prevents duplicate entries and ensures that no primary key value can be NULL.
CREATE TABLE products ( product_id INT PRIMARY KEY, product_name VARCHAR(100) NOT NULL, price DECIMAL(10, 2) NOT NULL );
In the example above, the product_id
column acts as the primary key, enforcing entity integrity by ensuring that each product has a distinct identifier.
Referential Integrity ensures that relationships between tables remain consistent. This is accomplished through foreign keys, which link a column in one table to the primary key in another table. This constraint ensures that a foreign key value must either match an existing primary key or be NULL, thus preventing orphaned records.
CREATE TABLE reviews ( review_id INT PRIMARY KEY, product_id INT, review_text TEXT NOT NULL, FOREIGN KEY (product_id) REFERENCES products(product_id) );
In this case, the product_id
in the reviews
table refers to the primary key in the products
table, enforcing referential integrity and ensuring that reviews cannot exist for non-existent products.
Domain Integrity refers to the validity of the data in each column based on the defined constraints. This includes restrictions on data types, allowable values, and formats. For instance, if a column is defined to hold integer values, any attempt to insert a non-integer will be rejected.
CREATE TABLE employees ( employee_id INT PRIMARY KEY, name VARCHAR(100) NOT NULL, hire_date DATE CHECK (hire_date > '2000-01-01') );
The CHECK
constraint on the hire_date
column ensures that only valid dates are entered, specifically those after January 1, 2000, thus preserving domain integrity.
User-Defined Integrity allows developers to implement specific business rules not covered by standard constraints. These rules are typically enforced through check constraints or triggers, which can provide additional validation based on complex logic.
CREATE TABLE accounts ( account_id INT PRIMARY KEY, balance DECIMAL(10, 2) NOT NULL CHECK (balance >= 0) );
In the accounts
table example, the check constraint ensures that no account can have a negative balance, aligning the data with business logic.
Implementing Primary and Foreign Keys
Implementing primary and foreign keys is a fundamental aspect of designing relational databases that uphold data integrity. These keys play an important role in establishing relationships between tables, ensuring that data remains consistent and accurate across the database system. Understanding how to properly define and use these keys is essential for any serious SQL practitioner.
A primary key serves as a unique identifier for each record in a table. It guarantees that no two rows can have the same key value, effectively preventing duplicate entries. To define a primary key, you can use the SQL PRIMARY KEY
constraint when creating a table. Here’s an example of creating a “customers” table with a primary key:
CREATE TABLE customers ( customer_id INT PRIMARY KEY, first_name VARCHAR(50) NOT NULL, last_name VARCHAR(50) NOT NULL, email VARCHAR(100) NOT NULL UNIQUE );
In this example, customer_id
is defined as the primary key, ensuring that each customer can be uniquely identified by their ID. Additionally, the email
column is marked as UNIQUE
to prevent any two customers from sharing the same email address.
On the other hand, a foreign key enforces referential integrity between two tables by linking a column in one table to the primary key of another table. This relationship ensures that the foreign key value must match an existing primary key value or be NULL
. For instance, consider an orders
table that references the customers
table:
CREATE TABLE orders ( order_id INT PRIMARY KEY, order_date DATE NOT NULL, customer_id INT, FOREIGN KEY (customer_id) REFERENCES customers(customer_id) );
Here, the customer_id
in the orders
table is defined as a foreign key, linking it to the customer_id
in the customers
table. This relationship ensures that each order is associated with a valid customer, thereby upholding referential integrity.
When inserting data into related tables, it’s crucial to follow the rules established by primary and foreign keys. For example, if we attempt to insert an order for a customer that does not exist in the customers
table, the database will reject the action:
INSERT INTO orders (order_id, order_date, customer_id) VALUES (1, '2023-10-01', 99); -- Assuming customer_id 99 does not exist
Attempting to execute this statement would result in a foreign key violation error, as 99
does not correspond to any customer_id
in the customers
table.
Additionally, it’s essential to ponder what happens to related records when changes occur. For example, if a customer is deleted from the customers
table, we need to decide how to handle their associated orders. SQL provides options such as ON DELETE CASCADE
, which automatically removes any related rows from the orders
table:
CREATE TABLE orders ( order_id INT PRIMARY KEY, order_date DATE NOT NULL, customer_id INT, FOREIGN KEY (customer_id) REFERENCES customers(customer_id) ON DELETE CASCADE );
With this setup, deleting a customer will also delete all their corresponding orders, maintaining the integrity of the data and preventing orphaned records.
Using Check Constraints for Validation
Check constraints in SQL are powerful tools for ensuring that the data entered into a table meets specific criteria. They allow developers to define rules for allowable values in a column, thereby enforcing data integrity at the database level. This type of validation can help prevent the entry of erroneous or nonsensical data, which could lead to inconsistencies and degradation of data quality.
A check constraint is defined at the column level or table level and can reference one or more columns within the same table. The validation rule can involve simple conditions such as ranges or lists of acceptable values, or more complex expressions involving multiple columns. Here’s an example of how to implement a check constraint to ensure that employees must be at least 18 years old:
CREATE TABLE employees ( employee_id INT PRIMARY KEY, name VARCHAR(100) NOT NULL, birth_date DATE NOT NULL, CHECK (DATEDIFF(CURDATE(), birth_date) / 365 >= 18) );
In this case, the CHECK constraint uses the DATEDIFF function to calculate the age of the employee based on their birth date, ensuring that only employees who are 18 or older can be inserted into the table.
Furthermore, check constraints can also be used to enforce business rules that are specific to an organization’s operational needs. For instance, in a product inventory table, we might want to ensure that the stock quantity cannot be negative:
CREATE TABLE inventory ( product_id INT PRIMARY KEY, product_name VARCHAR(100) NOT NULL, quantity INT NOT NULL CHECK (quantity >= 0) );
Here, the check constraint on the quantity column prevents any entry of negative values, thus maintaining the integrity of inventory data.
Check constraints are particularly useful in situations where data entry occurs from various sources, such as user inputs through forms or automated data ingestion processes. By embedding these rules directly into the database schema, developers can ensure that even if the application layer fails to validate the input properly, the database itself will not accept invalid data.
However, it’s important to note that check constraints can introduce performance overhead when inserting or updating records, especially if the conditions become complex. Therefore, it’s advisable to strike a balance between data validation and performance considerations, using check constraints judiciously to enforce only the most critical rules.
Maintaining Consistency with Transactions
In the sphere of SQL, maintaining consistency during transactions is an essential practice, especially when dealing with multiple operations that need to succeed or fail as a unit. Transactions are sequences of operations performed as a single logical unit of work, and they play an important role in ensuring that the database remains in a valid state. The primary goal of using transactions is to uphold the ACID properties: Atomicity, Consistency, Isolation, and Durability.
Atomicity guarantees that either all operations within a transaction are completed successfully, or none at all. If any part of the transaction fails, the entire transaction is rolled back, leaving the database unchanged. This very important in scenarios like transferring funds from one account to another, where both the debit and credit operations must succeed together.
Consistency ensures that a transaction takes the database from one valid state to another, maintaining all defined rules, such as integrity constraints. For example, if a check constraint restricts negative balances, a transaction that attempts to violate this rule would be rejected.
Isolation prevents transactions from interfering with each other. This means that the operations of a transaction are invisible to others until the transaction is committed. That is vital in multi-user environments, where concurrent transactions can lead to unpredictable results.
Durability guarantees that once a transaction is committed, its changes will persist even in the event of a system failure. That’s typically ensured through logging mechanisms that record transactions before they are finalized.
To illustrate the idea of transactions in SQL, ponder a scenario involving a bank transfer between two accounts:
START TRANSACTION; UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; -- Debiting account 1 UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; -- Crediting account 2 COMMIT; -- Finalize the transaction, making changes permanent
If any of the updates were to fail (for instance, if account 1 has insufficient funds), the transaction can be rolled back to ensure that neither account is affected:
ROLLBACK; -- Reverses the changes made in the transaction
This mechanism not only preserves the integrity of the accounts involved, but also reinforces the overall consistency of the database. Furthermore, SQL databases provide options for handling isolation levels, allowing developers to choose the balance between performance and the strictness of isolation according to their application needs. Common isolation levels include READ COMMITTED, REPEATABLE READ, and SERIALIZABLE, each providing different guarantees about the visibility of uncommitted changes made by other transactions.
In practice, thorough error handling within transactions is paramount. This ensures that applications can respond appropriately to any issues that arise during transactional operations. For example, encompassing transaction logic within a try-catch block can help manage exceptions gracefully:
BEGIN TRY START TRANSACTION; -- Perform multiple updates UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; COMMIT; -- Commit if no errors END TRY BEGIN CATCH ROLLBACK; -- Rollback if any error occurs -- Handle the error (logging, messaging, etc.) END CATCH;
Best Practices for Ensuring Data Integrity
Ensuring data integrity in SQL is not just about implementing constraints; it also involves adhering to best practices that enhance the reliability and maintainability of the database. Here are several crucial best practices to think when working with data integrity:
1. Define Clear Data Models: A well-structured data model is the foundation of data integrity. Before creating tables, ensure that the relationships between entities are clearly defined. This involves identifying the necessary primary and foreign keys, as well as understanding the data types required for each column. Spend time in the design phase to avoid costly modifications later.
2. Use Appropriate Data Types: Choosing the correct data type for each column is essential for maintaining data integrity. Using overly broad data types can lead to invalid data entries. For instance, using VARCHAR for storing dates is not advisable, as it allows for incorrect formats. Always opt for the most restrictive type that meets your needs.
CREATE TABLE products ( product_id INT PRIMARY KEY, product_name VARCHAR(100) NOT NULL, price DECIMAL(10, 2) CHECK (price > 0) -- Ensure price is positive );
3. Implement Constraints Strategically: Don’t just add constraints for the sake of it; strategically implement them where they add value. Primary keys, foreign keys, check constraints, and unique constraints should be thoughtfully considered to enforce rules that reflect business logic without overly complicating data entry.
4. Regularly Review and Update Constraints: As business requirements evolve, so too should your data integrity constraints. Regularly auditing and updating these rules helps ensure that they remain relevant and effective in maintaining data integrity. This could involve adding new constraints or modifying existing ones to reflect changes in business logic.
5. Use Transactions Wisely: Transactions are critical for maintaining consistency, especially in multi-step operations. Always group related operations within a transaction to ensure that either all changes are applied or none at all. This practice prevents partial updates, which can lead to data corruption.
BEGIN TRANSACTION; UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; -- Debit UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; -- Credit COMMIT;
6. Validate Data at the Application Level: While SQL constraints provide a robust layer of data validation, it is also important to validate data on the application side before it reaches the database. Implementing application-level validation can capture errors early and provide easy to use feedback, reducing the likelihood of database contention and errors caused by invalid inputs.
7. Document All Constraints and Rules: Clear documentation is key to maintaining data integrity. Make a habit of documenting all constraints, triggers, and validation rules applied to the database. This not only aids current developers but also serves as a valuable resource for onboarding new team members and maintaining institutional knowledge.
8. Backup and Recovery Plans: Regular backups are vital for protecting data integrity. Develop a comprehensive backup and recovery plan to safeguard against data corruption or loss. Ensure that backups are tested frequently to verify the integrity of the data being restored.
9. Monitor Database Performance: Keep an eye on database performance, as poorly performing queries can lead to timeouts and potential data integrity issues. Regularly analyze and optimize your database queries to ensure that all operations remain efficient, especially those that involve complex joins and data manipulations.
10. Educate Your Team: Lastly, ensure that all team members understand the importance of data integrity and are trained in best practices. Establishing a culture of data integrity within your organization can significantly reduce errors and enhance the overall quality of the data.