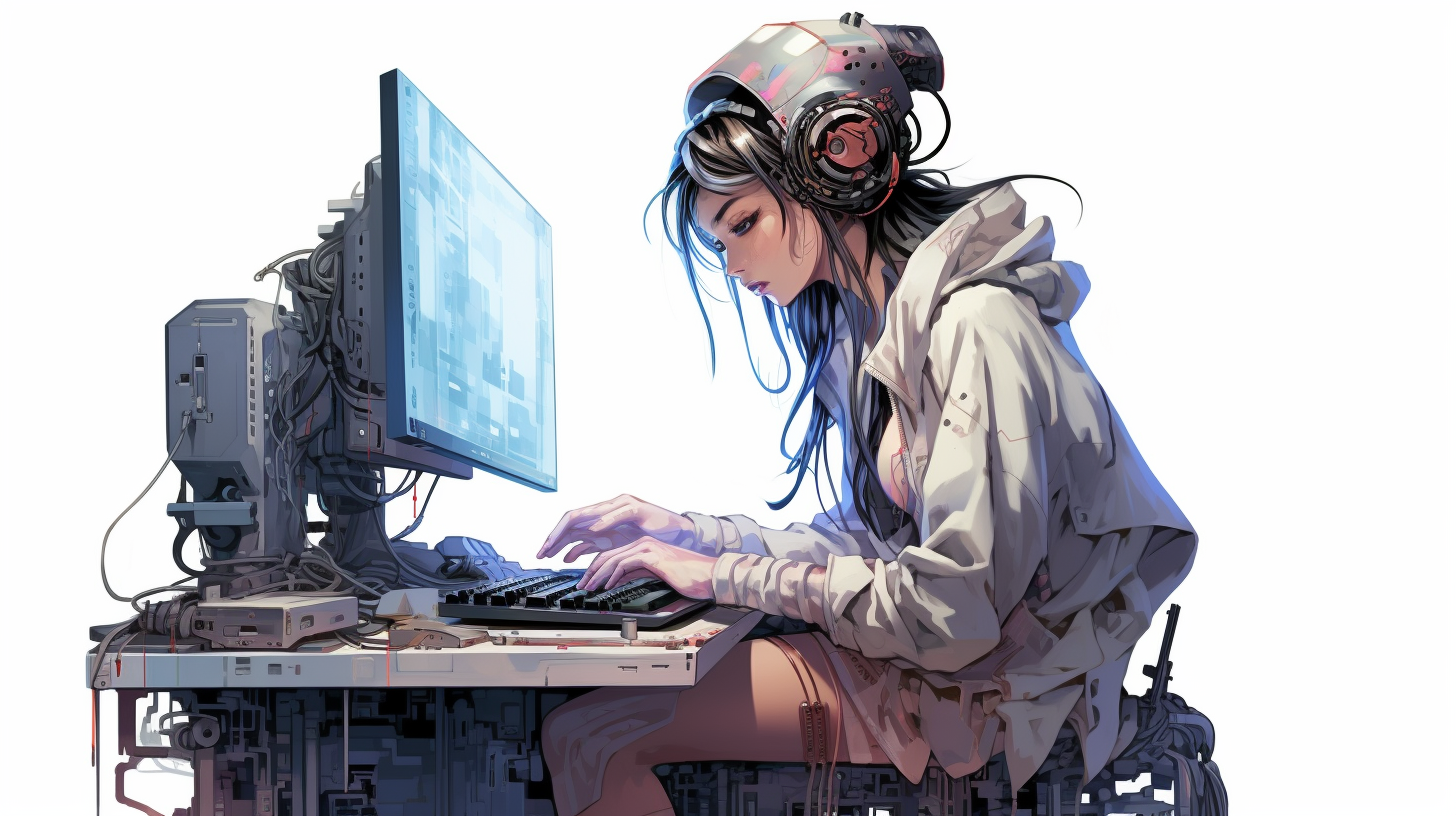
SQL for E-commerce: Best Practices
When it comes to e-commerce, an optimized database design serves as the backbone of your operations, influencing everything from product retrieval times to the overall user experience. Each table in your database should be carefully structured to not only meet current needs but also to anticipate future growth and functionality.
Normalization is an important step in database design. It involves organizing tables and relationships to reduce redundancy. Ideally, you should aim for at least the Third Normal Form (3NF). This means that:
- Each table should represent a single subject or entity.
- All attributes of a table should depend on the primary key.
- There should be no transitive dependencies.
For example, ponder the following tables in an e-commerce database:
CREATE TABLE Categories ( CategoryID INT PRIMARY KEY, CategoryName VARCHAR(255) NOT NULL ); CREATE TABLE Products ( ProductID INT PRIMARY KEY, ProductName VARCHAR(255) NOT NULL, Price DECIMAL(10, 2) NOT NULL, CategoryID INT, FOREIGN KEY (CategoryID) REFERENCES Categories(CategoryID) );
In the example above, the Products
table maintains a foreign key relationship with the Categories
table, ensuring that each product is associated with a valid category. This structure supports data integrity while enabling efficient queries.
Next, consider the indexing strategy. Proper indexing can significantly enhance data retrieval speeds. For example, if the e-commerce platform frequently searches products by name, implementing an index on the ProductName
field can optimize search performance:
CREATE INDEX idx_product_name ON Products(ProductName);
However, you must exercise caution; over-indexing can lead to performance penalties during data manipulation operations. A balance must be struck between read and write efficiency.
In addition to normalization and indexing, you should also consider the scalability of your database design. As your e-commerce platform grows, you may need to accommodate larger datasets and more complex queries. One approach is to use partitioning, which divides large tables into smaller, more manageable pieces. Here’s an example of how to partition a Sales
table by date:
CREATE TABLE Sales ( SaleID INT PRIMARY KEY, ProductID INT, SaleDate DATE, Amount DECIMAL(10, 2) ) PARTITION BY RANGE (YEAR(SaleDate)) ( PARTITION p2021 VALUES LESS THAN (2022), PARTITION p2022 VALUES LESS THAN (2023) );
The use of partitions makes it easier to manage historical data and improves query performance on recent transactions.
Finally, don’t overlook the importance of data types. Choosing the right data types can lead to better performance and more efficient storage. For example, if you know that a field will only contain a certain range of values, opting for a smaller data type can save space and improve processing speed:
CREATE TABLE Inventory ( InventoryID INT PRIMARY KEY, ProductID INT, Quantity TINYINT NOT NULL );
Essential SQL Queries for Product Management
When managing products in an e-commerce environment, SQL queries become essential tools for executing a variety of tasks, from adding new products to updating existing inventory levels. Mastering these essential SQL queries allows you to maintain product data integrity while facilitating seamless operations. Below are some fundamental SQL queries tailored for product management.
To insert a new product into the Products table, you can use the following SQL statement:
INSERT INTO Products (ProductID, ProductName, Price, CategoryID) VALUES (1, 'Wireless Mouse', 25.99, 2);
This query adds a new product, ‘Wireless Mouse,’ with a price of $25.99 and associates it with the category identified by CategoryID 2. Careful attention should be given to ensuring that the CategoryID exists in the Categories table to maintain referential integrity.
Updating product details is another common operation. For instance, to change the price of an existing product, you would use an UPDATE statement:
UPDATE Products SET Price = 22.99 WHERE ProductID = 1;
This query updates the price of this product with ProductID 1 to $22.99, so that you can adjust pricing strategies responsively.
To retrieve product information, the SELECT statement is your go-to tool. For example, if you want to view all products within a specific category, you can use:
SELECT ProductName, Price FROM Products WHERE CategoryID = 2;
This query fetches the names and prices of all products that belong to the category with CategoryID 2, enabling easy inventory browsing for that category.
When it comes to handling inventory levels, you might want to check the stock of a particular product. You can accomplish this with:
SELECT Quantity FROM Inventory WHERE ProductID = 1;
This query retrieves the quantity of this product identified by ProductID 1 from the Inventory table, helping you keep track of stock levels.
In addition to these basic operations, it is crucial to implement bulk operations when needed. For instance, if you need to update multiple product prices at once, using a CASE statement in your UPDATE query can be very effective:
UPDATE Products SET Price = CASE WHEN ProductID = 1 THEN 22.99 WHEN ProductID = 2 THEN 19.99 ELSE Price END WHERE ProductID IN (1, 2);
This allows you to adjust prices for several products in a single query, reducing the number of database calls and improving performance.
Finally, as your product catalog grows, you may find it beneficial to implement a stored procedure for common tasks, such as adding new products. Here’s an example of a simple stored procedure:
CREATE PROCEDURE AddProduct @ProductName VARCHAR(255), @Price DECIMAL(10, 2), @CategoryID INT AS BEGIN INSERT INTO Products (ProductName, Price, CategoryID) VALUES (@ProductName, @Price, @CategoryID); END;
This stored procedure streamlines the process of adding new products, accepting parameters for product name, price, and category ID. By encapsulating this logic within a procedure, you can simplify the calling code and ensure consistent data handling.
Ensuring Data Integrity and Security
Ensuring data integrity and security is paramount in the context of e-commerce, where customer trust and business reputation hinge on reliable data management. A robust SQL strategy not only protects the data but also maintains the accuracy and consistency of that data throughout its lifecycle.
One of the first lines of defense in ensuring data integrity is the use of primary keys and foreign keys. A primary key uniquely identifies each record in a table, while foreign keys create a link between tables, enforcing referential integrity. For example, in our earlier Products and Categories tables, we ensured that every product is tied to an existing category using a foreign key constraint:
CREATE TABLE Products ( ProductID INT PRIMARY KEY, ProductName VARCHAR(255) NOT NULL, Price DECIMAL(10, 2) NOT NULL, CategoryID INT, FOREIGN KEY (CategoryID) REFERENCES Categories(CategoryID) ON DELETE CASCADE );
The addition of ON DELETE CASCADE
ensures that if a category is deleted, all products associated with that category are automatically removed, preventing orphaned records and maintaining data integrity.
Beyond structural integrity, transaction management plays a critical role in maintaining data accuracy. SQL transactions allow you to group multiple operations into a single unit of work. If any operation within the transaction fails, the entire transaction can be rolled back, ensuring that your database remains in a consistent state. Here’s how you can implement a transaction in SQL:
BEGIN TRANSACTION; BEGIN TRY INSERT INTO Inventory (ProductID, Quantity) VALUES (1, 100); UPDATE Products SET Price = Price * 0.9 WHERE ProductID = 1; -- Apply discount COMMIT TRANSACTION; END TRY BEGIN CATCH ROLLBACK TRANSACTION; PRINT 'Transaction failed and has been rolled back'; END CATCH;
In this example, if either the insert into Inventory or the update to Products fails, the entire transaction is rolled back, ensuring that neither operation partially succeeds, thus maintaining data integrity.
Security is another crucial component of data integrity. Implementing user roles and permissions in your SQL database can help safeguard sensitive information. Limit access to only those who need it to perform their job functions. For example, you can create roles for various user levels:
CREATE ROLE ProductManager; GRANT SELECT, INSERT, UPDATE, DELETE ON Products TO ProductManager; CREATE ROLE InventoryManager; GRANT SELECT, INSERT, UPDATE ON Inventory TO InventoryManager;
By carefully controlling who has access to modify which tables, you reduce the risk of unauthorized changes and potential data corruption.
Additionally, consider implementing encryption for sensitive data, such as customer credit card information. SQL Server, for instance, provides features for encrypting sensitive data within your database. You can create a symmetric key and use it to encrypt data during insertion:
CREATE SYMMETRIC KEY CreditCardKey WITH ALGORITHM = AES_256 ENCRYPTION BY PASSWORD = 'StrongPassword123'; OPEN SYMMETRIC KEY CreditCardKey DECRYPTION BY PASSWORD = 'StrongPassword123'; INSERT INTO Customers (CustomerID, CreditCardNumber) VALUES (1, EncryptByKey(Key_GUID('CreditCardKey'), '1234-5678-9012-3456'));
By encrypting sensitive data, you ensure that even if someone gains unauthorized access to the database, the data remains protected.
Performance Tuning for High Traffic Scenarios
Performance tuning for high traffic scenarios is an essential practice for e-commerce platforms, especially as user demand can fluctuate dramatically. With the right strategies, you can ensure that your database remains responsive and efficient under load, providing a seamless shopping experience for customers. This involves not just optimizing queries, but also enhancing the overall architecture of your database.
One of the first steps in performance tuning is to analyze and optimize your SQL queries. Use the SQL Server Profiler or the EXPLAIN command in MySQL to identify slow-running queries. By looking into execution plans, you can pinpoint bottlenecks and areas for improvement. A common optimization technique is to rewrite inefficient queries. For example, using JOINs rather than subqueries can sometimes yield better performance. Here’s a simple example that demonstrates fetching product details along with category names:
SELECT p.ProductName, p.Price, c.CategoryName FROM Products p JOIN Categories c ON p.CategoryID = c.CategoryID WHERE p.Price < 50;
In this case, we’re joining the Products and Categories tables to fetch data in a single query, which can be more efficient than executing multiple separate queries.
Another vital aspect of performance tuning is the proper use of caching. Caching frequently accessed data can drastically reduce database load. For instance, you might cache the results of popular product queries or category listings. Think using a caching layer like Redis or Memcached to store these results temporarily. Here’s a basic example of how you might query the cache before hitting the database:
IF NOT EXISTS (SELECT * FROM Cache WHERE Key = 'popular_products') BEGIN INSERT INTO Cache (Key, Value) SELECT 'popular_products', (SELECT * FROM Products WHERE Popular = 1); END;
Additionally, the implementation of load balancing can enhance performance during peak traffic times. By distributing requests across multiple database servers, you can prevent any single server from becoming overwhelmed. This can be achieved using a primary-replica database architecture where read operations are directed to replicas while write operations go to the primary database. This ensures that read requests do not strain the primary database, which very important during busy shopping periods.
Database connection pooling is another technique worth exploring. By reusing existing connections rather than opening new connections for each request, you reduce overhead and improve response times. SQL Server, for example, offers built-in connection pooling. Ensure that your application is configured to take advantage of this feature:
-- Example: Connection string with pooling enabled SqlConnection conn = new SqlConnection("Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;Pooling=true;Max Pool Size=100;");
Indexing strategies also play a critical role in performance tuning. Beyond basic indexing, ponder implementing composite indexes for queries that filter on multiple columns. For instance, if you frequently search products by both category and price, a composite index on both columns could improve query performance:
CREATE INDEX idx_product_category_price ON Products(CategoryID, Price);
However, as with any optimization, it’s essential to monitor the performance impact of your indexes and to remove those that are underutilized or that negatively affect write performance.
Lastly, conducting regular database health checks and maintenance will keep your e-commerce platform running smoothly. This includes updating statistics, rebuilding fragmented indexes, and archiving old data. For instance, you can use the following command to rebuild an index:
ALTER INDEX ALL ON Products REBUILD;
Analyzing Customer Behavior with SQL
Analyzing customer behavior with SQL is a fundamental aspect of optimizing e-commerce strategies, enabling businesses to tailor their offerings and enhance user engagement effectively. Through careful interrogation of the data, you can identify purchasing patterns, preferences, and trends, which can inform marketing strategies and inventory management. Using SQL queries to extract and analyze this data is an efficient way to derive actionable insights.
One of the primary queries you can execute is to analyze the purchase history of customers. By joining the Sales and Customers tables, you can generate insights into which customers are the most active and the types of products they prefer. For instance, to retrieve the total sales value grouped by customer, you can use the following SQL query:
SELECT c.CustomerID, c.CustomerName, SUM(s.Amount) AS TotalSpent FROM Customers c JOIN Sales s ON c.CustomerID = s.CustomerID GROUP BY c.CustomerID, c.CustomerName ORDER BY TotalSpent DESC;
This query allows you to see which customers are contributing the most to your business, helping you target them with personalized offers or loyalty programs.
Another useful analysis involves identifying the most popular products over a specific period. By aggregating data from the Sales table, you can pinpoint which items are performing well. Here’s an example to find the top-selling products within a given timeframe:
SELECT p.ProductID, p.ProductName, COUNT(s.SaleID) AS QuantitySold FROM Products p JOIN Sales s ON p.ProductID = s.ProductID WHERE s.SaleDate BETWEEN '2023-01-01' AND '2023-12-31' GROUP BY p.ProductID, p.ProductName ORDER BY QuantitySold DESC LIMIT 10;
This query aggregates sales data for the year 2023, which will allow you to quickly identify the top ten products sold, which can inform restocking and promotional efforts.
Furthermore, customer segmentation can be achieved by analyzing purchasing frequency and average order value. This allows you to tailor marketing campaigns based on customer behavior. For instance, to categorize customers into different segments based on their purchase frequency, you can run:
SELECT c.CustomerID, COUNT(s.SaleID) AS PurchaseCount, AVG(s.Amount) AS AverageOrderValue FROM Customers c LEFT JOIN Sales s ON c.CustomerID = s.CustomerID GROUP BY c.CustomerID HAVING PurchaseCount > 5 ORDER BY AverageOrderValue DESC;
This query identifies customers who have made more than five purchases, providing insights into your most engaged clientele and their spending habits.
Additionally, understanding the timing of customer purchases can provide valuable insights into seasonal trends or peak shopping periods. For example, to analyze the number of sales per month, you can use:
SELECT DATE_FORMAT(s.SaleDate, '%Y-%m') AS Month, COUNT(s.SaleID) AS TotalSales FROM Sales s GROUP BY Month ORDER BY Month ASC;
This query aggregates sales data by month, helping you identify trends in customer purchasing behavior over time. Such insights can be critical for planning marketing campaigns around peak seasons or promotional events.
Implementing Best Practices for Transaction Management
Implementing best practices for transaction management in SQL is vital for ensuring data consistency and reliability in an e-commerce environment where financial transactions and inventory management must be executed flawlessly. Transactions allow multiple SQL operations to be executed as a single unit of work, providing a mechanism to ensure that either all operations succeed or none do, which very important for maintaining data integrity.
To begin, it is essential to understand the ACID properties of transactions: Atomicity, Consistency, Isolation, and Durability. These properties ensure that database transactions are processed reliably. Atomicity guarantees that all parts of a transaction are completed successfully; if any part fails, the entire transaction is rolled back. Consistency ensures that a transaction takes the database from one valid state to another, maintaining integrity constraints. Isolation guarantees that transactions are processed independently, and Durability ensures that once a transaction has been committed, it will survive permanently, even in the event of a system failure.
The basic structure of a transaction in SQL involves using the BEGIN TRANSACTION
, COMMIT
, and ROLLBACK
commands. Here’s a simple example:
BEGIN TRANSACTION; INSERT INTO Orders (OrderID, CustomerID, OrderDate, TotalAmount) VALUES (1, 123, '2023-10-05', 250.00); INSERT INTO Inventory (ProductID, Quantity) VALUES (456, -1); -- Subtracting one from inventory IF @@ERROR 0 BEGIN ROLLBACK TRANSACTION; PRINT 'Transaction failed and has been rolled back'; END ELSE BEGIN COMMIT TRANSACTION; PRINT 'Transaction completed successfully'; END;
In this example, the first insert adds an order, while the second modifies inventory. If the inventory update fails (for instance, if it attempts to set a negative stock level), the ROLLBACK
statement is executed, reverting the database to its previous state before the transaction began.
Isolation levels are another critical aspect of transaction management, affecting how transaction integrity is visible to other transactions. SQL offers several isolation levels, including Read Uncommitted, Read Committed, Repeatable Read, and Serializable. The choice of isolation level impacts performance and consistency. For example, using READ COMMITTED
prevents dirty reads by ensuring that only committed data is read, while SERIALIZABLE
provides the highest level of isolation but can lead to reduced concurrency.
SET TRANSACTION ISOLATION LEVEL READ COMMITTED; BEGIN TRANSACTION; SELECT * FROM Products WHERE ProductID = 1; -- Read data for processing -- Further operations can follow COMMIT TRANSACTION;
Moreover, handling concurrent transactions is vital in an e-commerce environment where multiple users or processes may attempt to access or modify data at the same time. Implementing appropriate locking mechanisms, such as optimistic concurrency control, can help manage these scenarios. For instance, you can utilize timestamps or version numbers in records to determine if a record has been changed since it was last read:
BEGIN TRANSACTION; DECLARE @CurrentVersion INT; SELECT @CurrentVersion = Version FROM Products WHERE ProductID = 1; UPDATE Products SET Price = 19.99, Version = Version + 1 WHERE ProductID = 1 AND Version = @CurrentVersion; IF @@ROWCOUNT = 0 BEGIN ROLLBACK TRANSACTION; PRINT 'Update failed due to concurrent modification'; END ELSE BEGIN COMMIT TRANSACTION; PRINT 'Update successful'; END;
This example checks the version of the product before the update, ensuring that it has not been modified by another transaction since it was last read. If the version has changed, the transaction is rolled back, maintaining data integrity.
Additionally, implementing logging for transactions can provide valuable insights into transaction performance and issues. By maintaining an audit trail, you can monitor changes and troubleshoot problems effectively. For instance, you might log transaction details, such as the operation type, affected records, and timestamps, into a dedicated logging table.
CREATE TABLE TransactionLog ( LogID INT PRIMARY KEY IDENTITY(1,1), OperationType VARCHAR(50), AffectedTable VARCHAR(50), AffectedID INT, Timestamp DATETIME DEFAULT GETDATE() ); INSERT INTO TransactionLog (OperationType, AffectedTable, AffectedID) VALUES ('INSERT', 'Orders', 1);