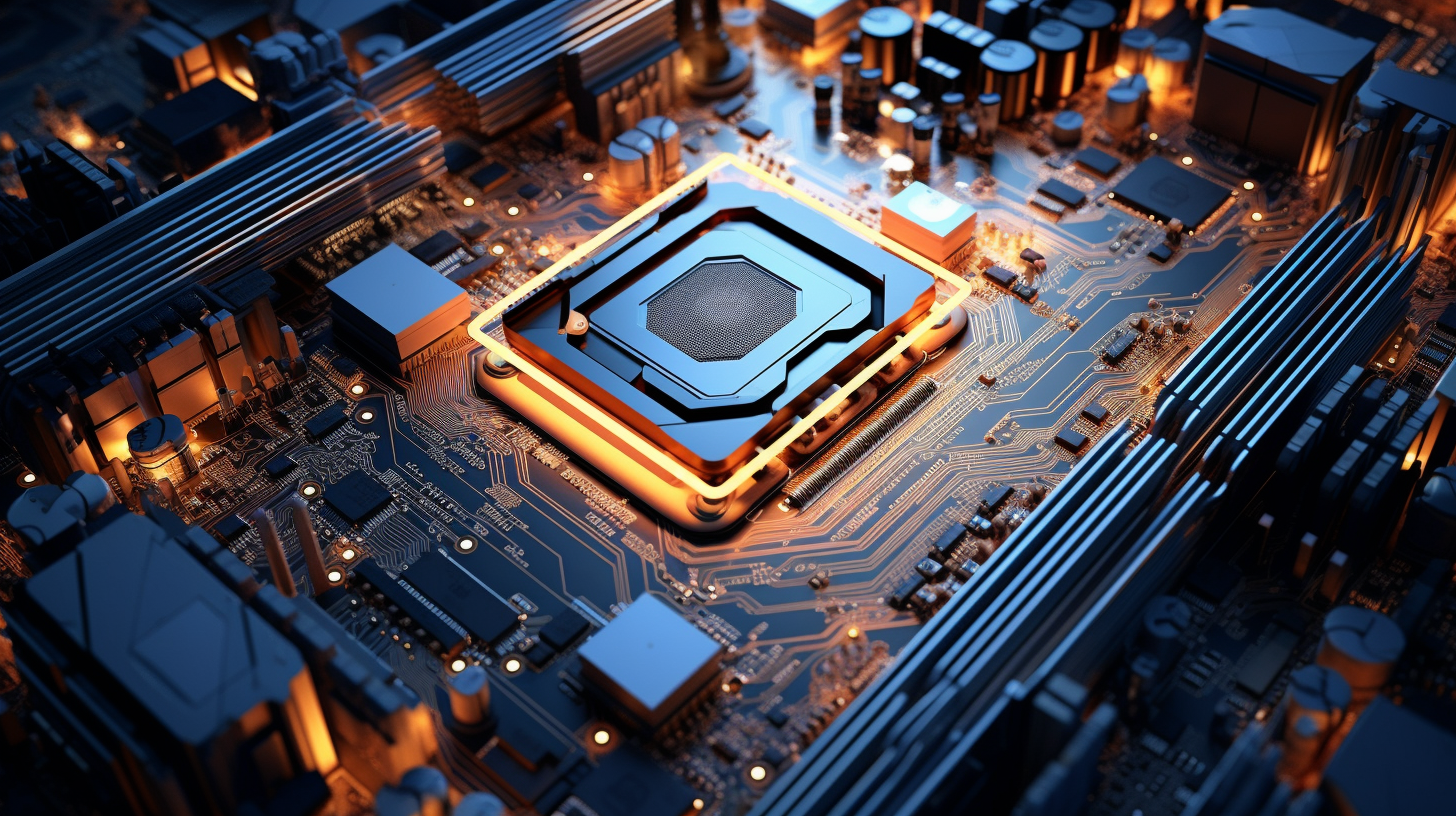
SQL for Handling Large Text Data
When working with large text data in SQL, it’s crucial to understand the various data types available and how they can impact performance and storage. SQL provides several data types specifically designed to handle large text, each with its own characteristics.
- This fixed-length data type is suitable for strings of a known length. However, it can lead to wasted space if the actual data is shorter than the defined length.
- Unlike CHAR, VARCHAR allows for variable-length strings, making it more efficient for text that can vary significantly in size. The maximum length must be specified upon creation.
- This type can store up to 255 bytes of text. It’s useful for small pieces of text but has limited size.
- Capable of storing up to 65,535 bytes, TEXT is perfect for larger text entries, such as descriptions or comments.
- This type can handle up to 16,777,215 bytes and is suited for large bodies of text, such as articles or user-generated content.
- The largest text type, LONGTEXT, can store up to 4 GB of text. It’s used for massive pieces of text data, such as books or large documents.
Choosing the appropriate data type is paramount for optimizing both storage space and query performance. For instance, using TEXT for short comments can lead to unnecessary overhead, while using VARCHAR for long articles can limit flexibility. Below is an example of how to define these types within a table:
CREATE TABLE articles ( id INT PRIMARY KEY AUTO_INCREMENT, title VARCHAR(255) NOT NULL, content LONGTEXT NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
When defining your schema, think the nature of the text data you expect to handle. For example, if you know that content will usually exceed 65,535 bytes, opting for LONGTEXT from the outset can prevent future headaches associated with schema changes.
Additionally, SQL’s handling of these data types can vary between database systems, so it is essential to consult the documentation specific to your SQL variant (such as MySQL, PostgreSQL, or SQL Server) to understand nuances and limitations.
Best Practices for Storing Large Text Data
When storing large text data in SQL, several best practices can enhance performance and ensure efficient management of that data. These practices revolve around not just choosing the right data type, but also considering other aspects such as indexing, normalization, and the handling of large text blobs.
1. Choose the Right Data Type
As previously discussed, selecting the appropriate data type especially important. Use VARCHAR
for shorter text when flexibility is needed, while TEXT
and its variants are preferable for larger content. For example, if you’re dealing with user comments that may vary in length, a VARCHAR(500)
might suffice, whereas articles would benefit from LONGTEXT
.
CREATE TABLE user_comments ( id INT PRIMARY KEY AUTO_INCREMENT, username VARCHAR(255) NOT NULL, comment VARCHAR(500), created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
2. Implement Indexing Wisely
Indexing can significantly improve read performance, especially when querying large text fields. However, it’s essential to apply indexing judiciously since indexing large text fields like TEXT
or LONGTEXT
can lead to increased storage requirements and slower insert operations. For instance, think indexing the title
of articles while leaving the content
unindexed due to its size.
CREATE INDEX idx_article_title ON articles (title);
3. Normalize Your Data
Normalization is a key practice in database design. If applicable, storing large text data in separate tables can help maintain a manageable schema. For instance, consider having a separate table for article content that connects to a main articles table via a foreign key. This separation can simplify retrieval and management of large texts.
CREATE TABLE article_content ( article_id INT, content LONGTEXT, PRIMARY KEY (article_id), FOREIGN KEY (article_id) REFERENCES articles(id) );
4. Limit the Size of Text Data
It is a good practice to enforce size limits where possible, particularly for user-generated content. Consider using triggers or application logic to validate text length before insertion. This helps prevent overly large entries that can degrade performance and complicate management.
CREATE TRIGGER limit_comment_length BEFORE INSERT ON user_comments FOR EACH ROW BEGIN IF LENGTH(NEW.comment) > 500 THEN SIGNAL SQLSTATE '45000' SET MESSAGE_TEXT = 'Comment exceeds maximum length'; END IF; END;
5. Ponder Storage Engines
Different database storage engines may have different capabilities and optimizations for handling large text types. For example, in MySQL, the InnoDB engine supports row-level locking and transactions, which can be beneficial for applications that deal with frequent updates to large text fields.
Efficient Querying Techniques for Large Text Fields
When dealing with large text fields in SQL, efficient querying techniques become essential for maintaining performance and responsiveness. As text data increases in size, the potential for slow query performance also grows, particularly if the database is not optimized for such operations. Below are several strategies to improve query efficiency when working with large text data.
1. Use WHERE Clauses to Filter Early
Applying a WHERE clause to filter results early in your query is a simple yet effective way to enhance performance. Instead of selecting all rows and then processing them, filtering helps reduce the dataset size right from the start. For instance, if you want to retrieve articles that were created after a specific date, you can use the following query:
SELECT id, title, content FROM articles WHERE created_at > '2023-01-01';
This approach minimizes the number of rows that need to be processed, making the query faster.
2. Limit the Data Retrieved
When querying large text fields, ponder using the SELECT statement to limit the columns retrieved. Often, you might not need the entire content field, especially when displaying summaries or lists. For example:
SELECT id, title FROM articles LIMIT 10;
This query retrieves just the article IDs and titles, making it more efficient, particularly when dealing with large datasets.
3. Implement Pagination
For applications displaying lists of items, pagination is an important technique. It allows users to view a subset of records, reducing the amount of data processed per query. Here’s how you can implement a simple pagination mechanism:
SELECT id, title FROM articles ORDER BY created_at DESC LIMIT 10 OFFSET 20;
This query fetches the next set of 10 articles, starting from the 21st article, allowing more manageable data presentation.
4. Use Full-Text Search for Complex Queries
When searching through large text fields, a full-text search can be significantly more efficient than using LIKE or other traditional search methods. Most SQL databases offer full-text indexing, which can enhance search capabilities. For example, in MySQL, you can create a full-text index on your content:
ALTER TABLE articles ADD FULLTEXT(content);
After indexing, you can perform searches like this:
SELECT id, title FROM articles WHERE MATCH(content) AGAINST('search term' IN NATURAL LANGUAGE MODE);
This method greatly speeds up searches compared to traditional methods by using the indexed data.
5. Utilize Subqueries and Common Table Expressions (CTEs)
Subqueries and CTEs can make complex queries more readable and potentially more efficient by breaking them down into manageable parts. For instance, if you want to retrieve articles with a specific keyword in their content and sort them by date, you might structure your query like this:
WITH relevant_articles AS ( SELECT id, title, content FROM articles WHERE MATCH(content) AGAINST('keyword') ) SELECT * FROM relevant_articles ORDER BY created_at DESC;
Using CTEs can help in organizing query logic and potentially optimizing execution paths.
6. Analyze Query Performance
Finally, regularly analyze your query performance using the EXPLAIN statement. This command provides insight into how the SQL engine processes a query, helping identify bottlenecks or areas for optimization:
EXPLAIN SELECT id, title FROM articles WHERE created_at > '2023-01-01';
By understanding the execution plan, you can make informed decisions about indexing, query restructuring, or even schema adjustments to enhance performance.
Handling Text Data with Full-Text Search and Indexing
Handling large text data in SQL can be a daunting task, especially when it comes to searching and indexing. Full-text search capabilities are essential for managing and extracting meaningful information from massive text datasets efficiently. Most SQL databases equip you with the tools necessary to perform sophisticated text searches, which will allow you to leverage the inherent structure of your large text fields, such as TEXT or LONGTEXT, to facilitate rapid data retrieval.
Full-Text Indexing
To begin using full-text search, you must first create a full-text index on the columns that contain the textual data you intend to search. This index allows the database engine to process queries more efficiently by precomputing the terms contained within the text fields. For example, if you’re dealing with an articles table, you can create a full-text index on the content column like this:
ALTER TABLE articles ADD FULLTEXT(content);
Once the index is in place, you can execute full-text searches using the MATCH()
function in conjunction with AGAINST()
. This combination allows you to search for keywords within your large text fields quickly:
SELECT id, title FROM articles WHERE MATCH(content) AGAINST('search term' IN NATURAL LANGUAGE MODE);
This query will return article IDs and titles that contain the specified search term, making it significantly faster than traditional LIKE queries, particularly as the dataset grows.
Boolean Mode Searches
In addition to natural language mode, SQL databases like MySQL support boolean mode, which allows for more complex search queries using operators such as +
(must include) and -
(must not include). For instance:
SELECT id, title FROM articles WHERE MATCH(content) AGAINST('+search -term' IN BOOLEAN MODE);
This query returns articles containing the word “search” but explicitly excludes those with the word “term.” This level of control over search behavior is invaluable when dealing with large text datasets where precision is paramount.
Handling Stopwords and Minimum Word Length
It’s important to note that many SQL implementations have a list of stopwords (common words) that are ignored in full-text searches, which can impact your queries. Additionally, there may be a minimum word length requirement, meaning that very short words might not be indexed. Understanding the behavior of your chosen SQL database regarding stopwords and minimum word length can help you craft more effective queries. You can modify these settings to suit your application needs, but be aware that doing so can impact performance.
Performance Considerations
While full-text search capabilities are powerful, they do come with specific performance considerations. A full-text index requires additional disk space and can slow down write operations (INSERT, UPDATE, DELETE) due to the overhead associated with maintaining the index. Therefore, it’s crucial to weigh the benefits of agile search capabilities against potential impacts on database performance.
Before implementing full-text search, it’s wise to analyze your use case: if your application requires frequent searching through vast amounts of text, the trade-off is often worth it. Conduct performance testing to understand how the introduction of full-text indexing affects your data manipulation operations.