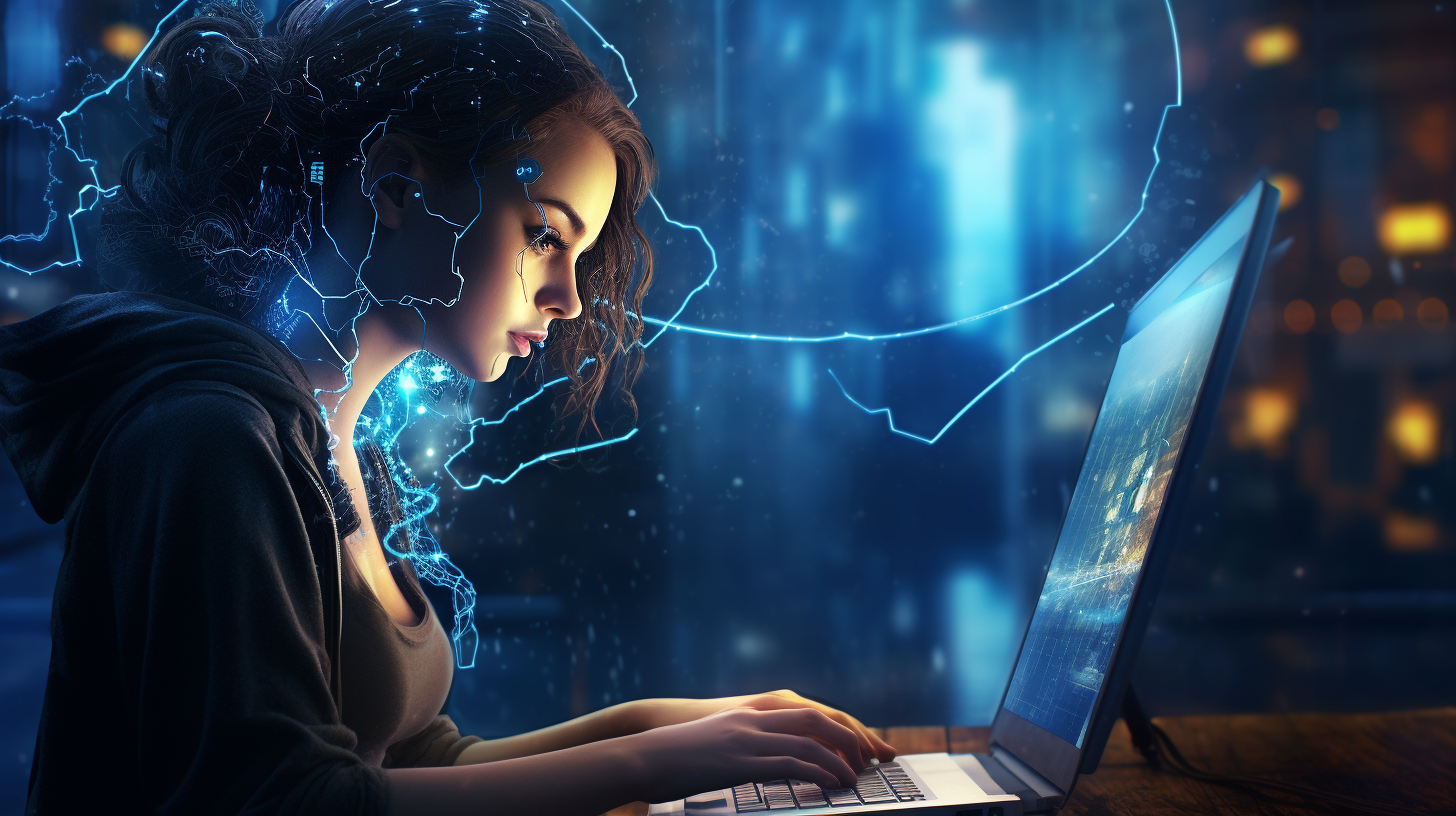
SQL for IoT Data Management
In the context of the Internet of Things (IoT), data is generated at an unprecedented scale and speed. Understanding the characteristics of this data especially important for anyone looking to manage, analyze, or leverage it effectively. IoT data can be classified into several key characteristics:
- IoT devices produce vast amounts of data continuously. This high volume requires robust storage solutions and efficient data handling techniques.
- The data generated by IoT devices comes in various formats, including structured, semi-structured, and unstructured. Examples include sensor readings, logs, images, and video streams.
- IoT data is often generated in real-time or near-real-time, necessitating swift processing and analysis to derive actionable insights. Understanding how to manage and query this data quickly is essential.
- The accuracy and reliability of IoT data can vary significantly. It’s important to implement mechanisms to ensure data integrity and to filter out noise from sensor data.
- IoT data can change over time, both in terms of its structure and its semantics. This variability requires flexible data models that can adapt to changes without significant overhead.
To manage IoT data effectively, one must think how these characteristics influence database design and data querying strategies. For instance, a schema that accommodates the high volume and variety of data might utilize NoSQL databases, but relational databases can still play a vital role in structured data handling.
When designing SQL queries for IoT data, it’s important to understand the typical patterns of data access. An example of a simple SQL query to retrieve the latest sensor readings might look like this:
SELECT device_id, sensor_type, reading, timestamp FROM sensor_data WHERE timestamp >= NOW() - INTERVAL '1 hour' ORDER BY timestamp DESC;
This query retrieves all sensor readings from the last hour, which illustrates both the velocity of data access and the need to filter based on time. Understanding these characteristics allows developers to optimize their queries and database structures for better performance.
Ultimately, grasping the nuances of IoT data characteristics shapes how one approaches data management strategies. It informs decisions about schema design, query optimization, and data processing, ultimately leading to more efficient and effective IoT solutions.
Designing an Efficient Database Schema for IoT
Designing an efficient database schema for IoT data involves a careful balance of performance, scalability, and adaptability to the unique data characteristics outlined earlier. Given the high volume and variety of IoT data, the schema must be structured in a way that allows for quick access, efficient storage, and ease of management.
One of the key considerations in schema design is normalization versus denormalization. In many traditional databases, normalization is employed to reduce redundancy and ensure data integrity. However, in IoT applications where read performance is critical and data is often accessed in real-time, denormalization can be advantageous. By duplicating certain data across tables, you can reduce the complexity of queries and speed up data retrieval.
Example of a Denormalized Schema:
CREATE TABLE device_data ( device_id SERIAL PRIMARY KEY, device_name VARCHAR(255), sensor_type VARCHAR(100), reading FLOAT, timestamp TIMESTAMP DEFAULT CURRENT_TIMESTAMP, location VARCHAR(255), unit_of_measurement VARCHAR(50) );
In this schema, the `device_data` table consolidates various attributes of the device and its readings into a single entry, which simplifies querying. For instance, if you need to retrieve the most recent readings for a specific device, a simpler query can be executed:
SELECT device_name, sensor_type, reading, timestamp FROM device_data WHERE device_id = 5 ORDER BY timestamp DESC LIMIT 1;
Moreover, accommodating the variety of data types generated by IoT devices is essential. This may lead to the inclusion of JSON or XML fields within the schema, especially when dealing with unstructured data. PostgreSQL, for instance, offers excellent support for JSON data types, which allows for greater flexibility in storing varied data formats:
CREATE TABLE sensor_logs ( log_id SERIAL PRIMARY KEY, device_id INT, log_data JSONB, timestamp TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
In this example, the `log_data` field can store a variety of sensor outputs in a flexible JSON format, allowing for structured querying without the need for a rigid schema. To retrieve specific elements from this JSON data, you can use the following SQL query:
SELECT log_data->>'temperature' AS temperature, log_data->>'humidity' AS humidity FROM sensor_logs WHERE device_id = 3 AND timestamp >= NOW() - INTERVAL '24 hours';
The choice of primary keys is another critical aspect of schema design in IoT. Using a composite key that includes both the device ID and timestamp can be beneficial in ensuring uniqueness and quick access to the most recent data:
CREATE TABLE readings ( device_id INT, reading_time TIMESTAMP, reading FLOAT, PRIMARY KEY (device_id, reading_time) );
This approach not only prevents duplicate entries but also allows for efficient querying of time series data, which is common in IoT applications.
Overall, designing an efficient database schema for IoT requires a keen understanding of the inherent data characteristics and access patterns. By strategically choosing between normalization and denormalization, accommodating various data types, and ensuring effective key usage, you can create a robust framework that facilitates rapid data access and analysis.
Querying and Analyzing IoT Data with SQL
Querying and analyzing IoT data with SQL requires a strategic approach that considers the unique characteristics of the data being handled. The sheer volume and velocity of IoT data necessitate efficient querying methods to extract meaningful insights quickly. SQL remains a powerful tool for this purpose, allowing for complex queries that can aggregate, filter, and join data from multiple sources.
One common challenge in IoT data querying is the need to handle time series data effectively. When working with data that is constantly being generated, you often need to perform operations like aggregating readings over time intervals. For instance, to calculate the average temperature readings from sensors over the last 24 hours, you might use the following SQL query:
SELECT AVG(reading) AS avg_temperature FROM readings WHERE sensor_type = 'temperature' AND reading_time >= NOW() - INTERVAL '24 hours';
This query utilizes the `AVG()` function to compute the average of temperature readings, showcasing how SQL can efficiently handle aggregations over time. Such queries are crucial for deriving trends and patterns from the data, enabling better decision-making.
Another important aspect of querying IoT data is filtering based on specific device attributes. By using indexes on frequently queried columns, you can significantly enhance performance. For example, if you frequently look for readings from a specific device, creating an index on the `device_id` column can drastically speed up your queries:
CREATE INDEX idx_device_id ON readings(device_id);
With this index in place, a query to retrieve the last five readings for a specific device becomes much faster:
SELECT reading_time, reading FROM readings WHERE device_id = 10 ORDER BY reading_time DESC LIMIT 5;
In addition to filtering and aggregating, analyzing IoT data often involves joining multiple tables to gain a comprehensive view of the data. Ponder a scenario where you need to analyze sensor readings alongside device metadata. This could be done with a query that combines the `readings` table and a `devices` table to get a full picture of each reading:
SELECT d.device_name, r.reading, r.reading_time FROM readings r JOIN devices d ON r.device_id = d.device_id WHERE r.reading_time >= NOW() - INTERVAL '1 week';
This query shows how joins can be utilized to enrich the data being queried, allowing users to see not just the readings but also contextual information about the devices that generated them.
Moreover, grouping data to analyze trends over time is another powerful feature of SQL. For example, if you want to see the average readings for different sensor types grouped by day, you can use:
SELECT DATE(reading_time) AS reading_date, sensor_type, AVG(reading) AS avg_reading FROM readings GROUP BY reading_date, sensor_type ORDER BY reading_date, sensor_type;
This query provides a day-by-day breakdown of average readings for each sensor type, allowing analysts to identify trends and anomalies in their IoT data easily.
As IoT data continues to grow in complexity and size, understanding how to effectively query and analyze this data using SQL is essential for using its full potential. By using aggregation, filtering, indexing, and joining techniques, one can create efficient query strategies that yield actionable insights from the vast streams of IoT information.
Implementing Real-Time Data Processing
Implementing real-time data processing in IoT systems is critical for enabling immediate insights and actions based on the continuous and rapid influx of data generated by devices. This requires not only efficient data ingestion but also the ability to process and analyze this data on-the-fly. Traditional batch processing methods fall short here, as they cannot keep pace with the velocity of IoT data. Instead, we must embrace streaming data architectures and explore SQL extensions that facilitate real-time processing.
One of the key technologies for real-time data processing in the IoT landscape is Apache Kafka, which serves as a distributed streaming platform. It allows for the ingestion of data streams from multiple IoT devices in real-time. By integrating Kafka with SQL databases, such as PostgreSQL or MySQL, you can create a seamless pipeline that ensures data is captured and made accessible for immediate querying.
To show how real-time data ingestion can work, consider the following SQL example that utilizes a trigger to automatically insert new data into a table as it arrives from a stream:
CREATE TABLE real_time_readings ( device_id INT, reading FLOAT, timestamp TIMESTAMP DEFAULT CURRENT_TIMESTAMP ); CREATE OR REPLACE FUNCTION insert_reading() RETURNS TRIGGER AS $$ BEGIN INSERT INTO real_time_readings (device_id, reading, timestamp) VALUES (NEW.device_id, NEW.reading, NOW()); RETURN NEW; END; $$ LANGUAGE plpgsql; CREATE TRIGGER new_reading_trigger AFTER INSERT ON kafka_reading_stream FOR EACH ROW EXECUTE FUNCTION insert_reading();
This approach ensures that as new data is produced in the Kafka stream, it’s automatically captured and stored in the `real_time_readings` table. The `AFTER INSERT` trigger effectively bridges the gap between the streaming data source and the SQL database, allowing for immediate access to the latest readings.
Once data is in the database, the next challenge is to query it efficiently in real-time. SQL databases often offer window functions, which are particularly useful for analyzing data trends over specific intervals. For instance, if you want to calculate the average reading over the last five minutes from the `real_time_readings` table, you might execute the following query:
SELECT AVG(reading) AS avg_reading, device_id, DATE_TRUNC('minute', timestamp) AS reading_minute FROM real_time_readings WHERE timestamp >= NOW() - INTERVAL '5 minutes' GROUP BY device_id, reading_minute ORDER BY reading_minute DESC;
This query efficiently aggregates real-time data by using the `AVG()` function and grouping results by minute, providing insights into current trends and fluctuations in readings.
Furthermore, to create a robust real-time processing architecture, it’s essential to implement alerting mechanisms that trigger notifications or actions based on certain conditions. For instance, if the temperature reading exceeds a predefined threshold, an alert can be generated:
CREATE OR REPLACE FUNCTION alert_high_temperature() RETURNS TRIGGER AS $$ BEGIN IF NEW.reading > 75 THEN PERFORM pg_notify('high_temperature_alert', 'Device ' || NEW.device_id || ' has exceeded the temperature threshold!'); END IF; RETURN NEW; END; $$ LANGUAGE plpgsql; CREATE TRIGGER check_temperature AFTER INSERT ON real_time_readings FOR EACH ROW EXECUTE FUNCTION alert_high_temperature();
In this example, a trigger is created to monitor incoming readings. If the reading exceeds 75 degrees, a notification is sent, allowing the system to react immediately—be it sending alerts to operators or activating cooling systems in response to high temperatures.
Employing efficient real-time data processing strategies is paramount in IoT applications. By using streaming platforms like Kafka, using SQL triggers, and implementing window functions, you create a powerful framework that allows for immediate insights and actions based on real-time data. This dynamic approach not only enhances operational efficiency but also empowers organizations to respond swiftly to emerging patterns and anomalies in their IoT data.
Best Practices for Securing IoT Data in SQL Databases
As IoT continues to proliferate, securing the vast amounts of data flowing from devices becomes paramount. The sensitive nature of this data, often involving personal information, operational details, and critical infrastructure metrics, necessitates robust security practices within SQL databases. Below are several best practices for ensuring the integrity, confidentiality, and availability of IoT data stored in SQL databases.
1. Implement Strong Authentication and Authorization: One of the first lines of defense is ensuring that only authorized personnel and applications can access the database. Utilize strong password policies, multi-factor authentication, and role-based access control (RBAC) to limit access based on the principle of least privilege. For example, you can create user roles with specific permissions in PostgreSQL:
CREATE ROLE analyst WITH LOGIN PASSWORD 'secure_password'; GRANT SELECT ON sensor_data TO analyst;
This approach ensures that users only have access to the data they require for their tasks, minimizing potential security breaches.
2. Encrypt Data at Rest and in Transit: Data encryption should be a fundamental part of your security strategy. Encrypting data at rest protects it from unauthorized access, while encryption in transit safeguards against interception during data transmission. For example, you can enable SSL for PostgreSQL connections to encrypt data during transit:
# In postgresql.conf ssl = on ssl_cert_file = 'server.crt' ssl_key_file = 'server.key'
Additionally, use built-in encryption functions to encrypt sensitive data stored in the database:
CREATE TABLE secure_sensor_data ( device_id INT, encrypted_reading BYTEA, timestamp TIMESTAMP DEFAULT CURRENT_TIMESTAMP ); INSERT INTO secure_sensor_data (device_id, encrypted_reading) VALUES (1, pgp_sym_encrypt('23.5', 'encryption_key'));
3. Regularly Update and Patch Database Systems: Keeping your database software updated is important for protecting against vulnerabilities. Security patches released by database vendors often address known exploits that could be leveraged by malicious actors. Establish a routine for monitoring and applying updates as they become available.
4. Utilize Network Security Measures: Implement firewalls and network segmentation to protect your database from unauthorized access. By isolating your SQL database from the public internet and ensuring that only trusted network devices can communicate with it, you reduce the attack surface significantly. Ensure that only specific IP addresses or subnets are allowed access to the database:
# Example for PostgreSQL host all all 192.168.1.0/24 md5
5. Monitor and Audit Database Activity: Continuous monitoring of database activity can help you detect anomalies or unauthorized access attempts. Implement logging mechanisms to track all database transactions and changes. SQL databases often provide built-in logging features that can be configured to capture detailed information:
# Enable logging in postgresql.conf logging_collector = on log_directory = 'pg_log' log_filename = 'postgresql-%Y-%m-%d_%H%M%S.log'
Additionally, think setting up alerts for suspicious activities, such as failed login attempts or unexpected changes to critical tables.
6. Regular Backups and Disaster Recovery Plans: Implement a robust backup strategy that includes regular backups of your SQL database. This ensures that you can restore data in the event of corruption, loss, or a cyber-attack. Test your backup and recovery process periodically to ensure its effectiveness. A simple SQL command to create a backup could look like:
pg_dump -U username -F c -b -v -f "backup_file.backup" dbname
By following these best practices, you can significantly enhance the security posture of your SQL databases in IoT environments. A proactive approach to data security not only protects sensitive information but also builds trust among users and stakeholders, ensuring that IoT applications can operate effectively and securely in an ever-evolving digital landscape.