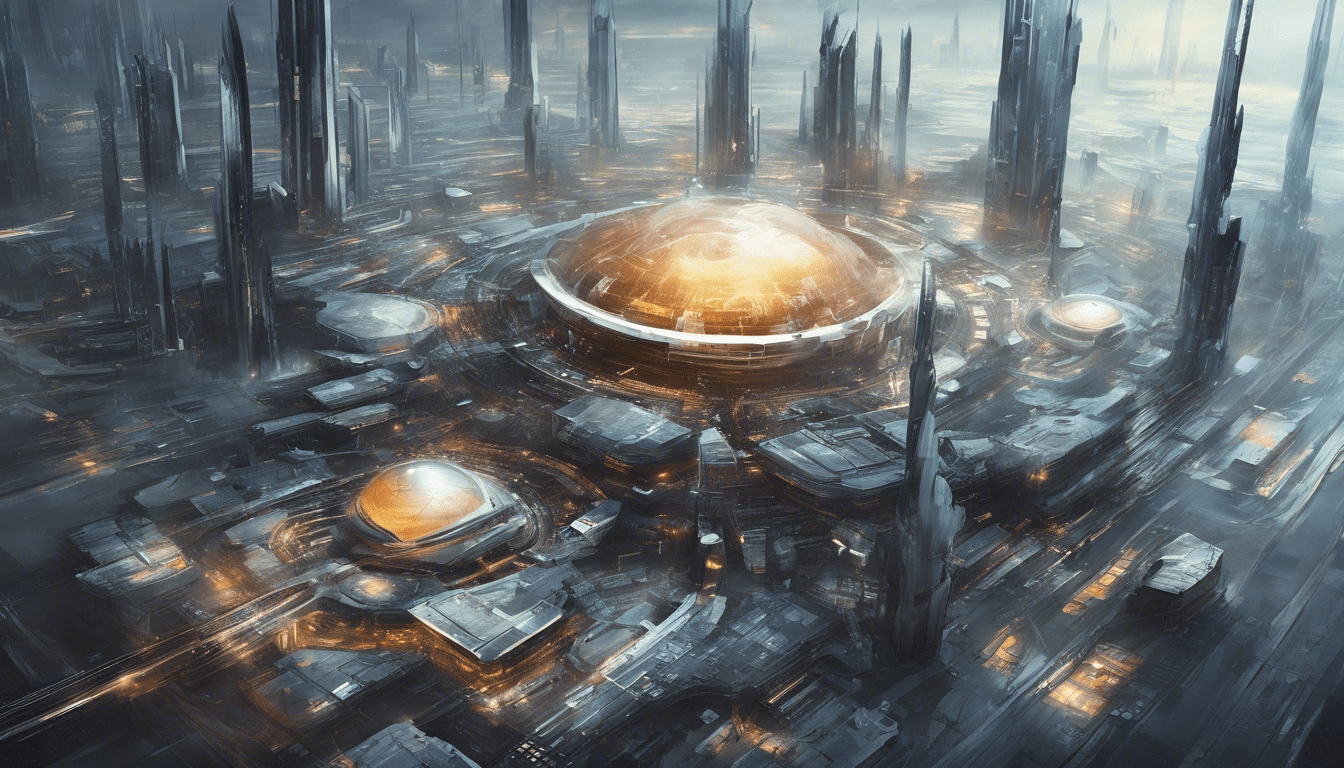
SQL for Recursive Data Organization
When it comes to organizing data in a database, one useful feature of SQL is its ability to handle recursive data. Recursive data refers to data that is structured in a tree-like fashion, where each record can be linked to a parent record. This can be incredibly useful when dealing with data that naturally forms a hierarchy, such as organizational charts, file systems, or product categories.
SQL provides a recursive query feature called the Common Table Expressions (CTEs) that allows developers to write queries that can refer to themselves, effectively creating a loop that can traverse through a data hierarchy. CTEs are usually prefixed with the WITH keyword and are followed by a SELECT statement that contains the recursion.
Here is a simple example to show how a recursive CTE works:
WITH RECURSIVE CategoryPath AS ( SELECT CategoryID, CategoryName, ParentCategoryID FROM Categories WHERE ParentCategoryID IS NULL UNION ALL SELECT c.CategoryID, c.CategoryName, c.ParentCategoryID FROM Categories c JOIN CategoryPath cp ON c.ParentCategoryID = cp.CategoryID ) SELECT * FROM CategoryPath;
In this example, we have a table called Categories with columns for CategoryID, CategoryName, and ParentCategoryID. The CTE begins by selecting all records where the ParentCategoryID is NULL, meaning these are the top-level categories. Then, the recursive part of the CTE selects all records from Categories that have a ParentCategoryID matching the CategoryID of the records selected in the previous step. The UNION ALL operator combines the results of both queries and the recursion continues until there are no more records to select.
It is important to note that when working with recursive queries, it is important to ensure that there is a base case that will stop the recursion. Otherwise, you may end up with an infinite loop which could cause your query to never complete and consume excessive resources.
The recursive CTEs in SQL can be incredibly powerful for organizing hierarchical data and can make queries much more efficient than trying to emulate recursion with multiple self-joins or server-side code. With careful design and an understanding of how recursion works in SQL, you can unlock the full potential of your database’s hierarchical data.
Here’s another example where we want to find all the subcategories under a specific parent category:
WITH RECURSIVE Subcategories AS ( SELECT CategoryID, CategoryName FROM Categories WHERE ParentCategoryID = 1 -- Assuming 1 is the ID of the parent category we're starting from UNION ALL SELECT c.CategoryID, c.CategoryName FROM Categories c INNER JOIN Subcategories s ON c.ParentCategoryID = s.CategoryID ) SELECT * FROM Subcategories;
This query will return all the categories that are children of the parent category with ID 1, plus all of their subcategories, and so on. That is an excellent way to explore all the levels of a hierarchy starting from a specific point.
Great article on using recursive queries in SQL to handle hierarchical data! One important point that I’d like to add is the concept of “anchor member” in recursive CTEs. The anchor member is the starting point of the recursion and is typically identified by a specific condition in the WHERE clause. In both examples given, the anchor member is the record with a NULL ParentCategoryID.
By explicitly specifying the anchor member, it provides a clear base case for the recursion to stop and prevents an infinite loop. It’s important to define this anchor member in order to ensure that the query terminates successfully and avoids any potential issues with resource consumption.
Overall, recursive queries in SQL are a powerful tool for managing hierarchical data, and understanding the concept of anchor members can further enhance their efficiency and effectiveness.