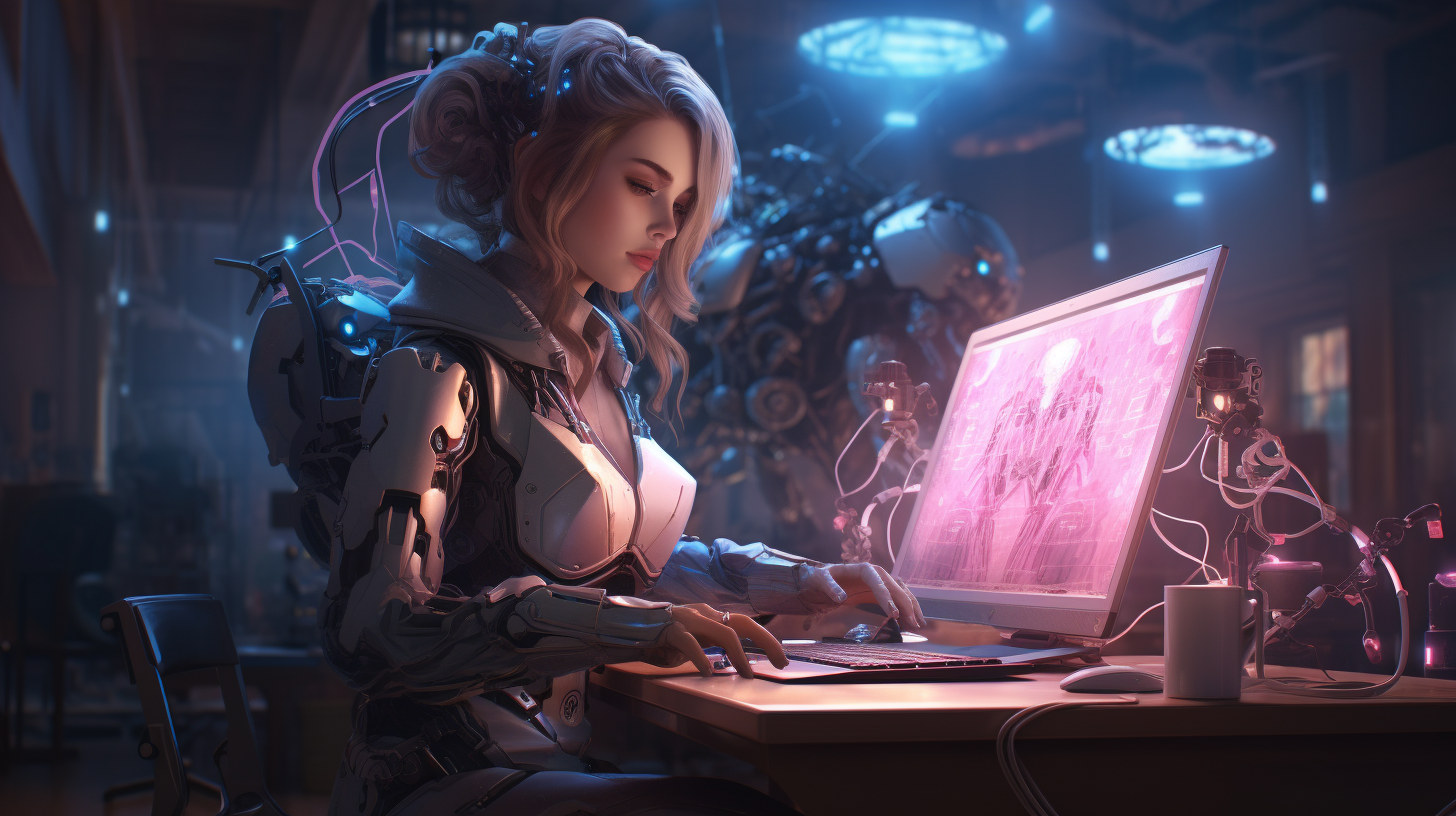
SQL for Streamlining Data Imports
When dealing with data imports, several challenges can arise that can complicate the process and lead to data inconsistencies. Understanding these challenges is fundamental for anyone looking to streamline data imports effectively.
One common challenge is dealing with inconsistent data formats. Data may come from various sources, leading to discrepancies in formats, such as date representations, number formatting, or even text capitalization. These inconsistencies can cause errors during the import process or result in corrupted data this is difficult to cleanse later.
Another hurdle is missing or incomplete data. When importing data, you may encounter rows with missing values, which can disrupt relational integrity. Handling these gaps requires careful consideration of how to either fill in these values, exclude incomplete records, or implement default values.
Data duplication is also a significant issue. In scenarios where the same records are imported multiple times, this can lead to inflated datasets and inaccurate reporting. Identifying and managing these duplicates is essential to maintain the integrity of the data.
Additionally, the volume of data being imported can pose performance challenges. Importing large datasets can lead to slow performance or even timeouts if the database is not optimized for handling bulk operations. Understanding how your database handles transactions will help prevent these bottlenecks.
Finally, data validation is important. When data is imported, it should be validated against business rules to ensure its accuracy and relevance. Without proper validation, you risk introducing erroneous data into your database, which can have cascading effects on analytics and reporting.
Addressing these challenges requires a strategic approach. Below are some recommendations for overcoming these common data import hurdles:
- Implement a data profiling process to assess incoming data for consistency and quality.
- Utilize ETL (Extract, Transform, Load) tools to automate and standardize data formatting and cleansing.
- Employ deduplication techniques like primary keys or unique indexes to avoid duplicate records.
- Optimize database performance through indexing and partitioning to facilitate smooth data imports.
- Enforce data validation rules at the point of entry to maintain data quality.
By understanding the intricacies of these challenges, you are better equipped to tackle the complexities of data imports and enhance the overall efficiency of your database operations.
Best Practices for Data Cleanup
Data cleanup is a pivotal step in preparing your database for a successful data import. Without it, you risk carrying over errors, inconsistencies, and redundancies that can undermine the integrity of your database. Below are some best practices to ensure your data is clean and ready for import.
1. Data Profiling
Before any cleaning can begin, it is essential to analyze the incoming data. Data profiling involves examining the data for accuracy, completeness, and consistency. This process will help you identify anomalies such as outliers, incorrect data types, and missing values. You can use SQL queries to facilitate this analysis.
SELECT column_name, COUNT(*) AS total_rows, COUNT(DISTINCT column_name) AS unique_entries, COUNT(CASE WHEN column_name IS NULL THEN 1 END) AS null_values FROM your_table GROUP BY column_name;
This query provides a clear overview of each column’s data quality and helps identify areas that need attention.
2. Standardizing Data Formats
Once you have profiled your data, standardizing formats is the next step. For example, dates may be stored in multiple formats across different records, which can lead to errors when querying. Use SQL functions to convert data into a uniform format. The CAST
or CONVERT
functions in SQL can be particularly handy.
UPDATE your_table SET date_column = CONVERT(DATE, date_column, 101) WHERE ISDATE(date_column) = 1;
This command converts the date_column
into a consistent date format, ensuring compatibility across your database.
3. Handling Missing Values
Missing data can derail your import process. You have several options for dealing with missing values: you can delete rows with nulls, fill them with a default value, or use statistical methods to estimate the missing data. SQL’s COALESCE
function can be useful for substituting default values.
UPDATE your_table SET column_name = COALESCE(column_name, 'default_value');
By replacing null entries with a specific default, you maintain the integrity of your dataset.
4. Removing Duplicates
Data duplication is a frequent issue that requires careful handling. To identify duplicates, you can use the ROW_NUMBER()
function along with a common identifier. Once identified, you can choose to keep the first occurrence and delete the rest.
WITH CTE AS ( SELECT *, ROW_NUMBER() OVER (PARTITION BY unique_column ORDER BY id) AS row_num FROM your_table ) DELETE FROM CTE WHERE row_num > 1;
This query creates a Common Table Expression (CTE) to isolate duplicates, which will allow you to retain only the first occurrence of each record.
5. Validating Data
Finally, data validation is a critical step to ensure the integrity of your imported data. Setting up constraints and triggers in your SQL schema helps enforce data validation rules at the point of entry. For instance, you can use CHECK
constraints to limit the range of values accepted in a table column.
ALTER TABLE your_table ADD CONSTRAINT check_column_value CHECK (column_name > 0);
This constraint ensures that only positive values can be entered into column_name
, thus maintaining data quality.
By adhering to these best practices for data cleanup, you can significantly enhance the quality of your data imports, ensuring a clean slate for analysis and reporting purposes.
Optimizing SQL Queries for Performance
Optimizing SQL queries for performance is essential for ensuring that data imports proceed smoothly, particularly when dealing with large datasets. The efficiency of your SQL queries can have a significant impact on the overall speed and reliability of data import processes. Below are several strategies to optimize your SQL queries for better performance during data imports.
1. Use Indexing Wisely
Indexes can drastically improve the performance of SELECT operations, but they can also slow down INSERT, UPDATE, and DELETE operations due to the overhead of maintaining the index. Therefore, it very important to evaluate the use of indexes based on the specific needs of your data import. A typical approach is to temporarily disable or drop non-essential indexes during the import process and recreate them afterward.
ALTER TABLE your_table DISABLE TRIGGER ALL; -- Temporarily disable triggers -- Perform your bulk insert operations here ALTER TABLE your_table ENABLE TRIGGER ALL; -- Enable triggers again
2. Batch Inserts
Instead of executing a single INSERT statement for each row of data, batching multiple rows into a single INSERT statement can significantly reduce the number of transactions, which in turn minimizes overhead and improves performance. For example:
INSERT INTO your_table (column1, column2) VALUES ('value1', 'value2'), ('value3', 'value4'), ('value5', 'value6');
By using this method, you efficiently reduce the interaction with the database, resulting in faster data imports.
3. Avoid SELECT *
Using SELECT * in your queries can lead to unnecessary overhead, particularly when dealing with large tables. Instead, specify only the columns you need. This approach not only improves performance but also enhances readability and maintainability of your SQL code.
SELECT column1, column2 FROM your_table WHERE condition;
4. Leverage Temporary Tables
When dealing with complex transformations or large datasets, using temporary tables can help break down the process into manageable steps. Load your data into a temporary table first, perform any necessary transformations, and then insert the cleaned data into your target table. This can help isolate issues and optimize the import process.
CREATE TEMPORARY TABLE temp_table AS SELECT * FROM source_table WHERE condition; -- Perform necessary transformations INSERT INTO final_table SELECT * FROM temp_table;
5. Optimize Query Execution Plans
Understanding and optimizing execution plans can lead to significant performance improvements. Use the EXPLAIN command to analyze how your SQL queries are being executed. Look for costly operations such as full table scans, and adjust your indexing or query structure accordingly to avoid these inefficiencies.
EXPLAIN SELECT column1 FROM your_table WHERE condition;
6. Maintain Statistics
Database engines use statistics to create optimized query execution plans. Regularly updating these statistics can lead to better performance. Ensure that your database is set to automatically update statistics or do so manually after significant changes to the data.
UPDATE STATISTICS your_table;
By implementing these optimization techniques, you can drastically improve the performance of your SQL queries during data imports. This not only enhances the efficiency of the import process but also contributes to maintaining overall database performance and reliability.
Using Bulk Insert Operations
Using bulk insert operations is a powerful technique that can greatly enhance the efficiency of your data import processes. When dealing with large datasets, traditional row-by-row insertion methods can be slow and can lead to significant overhead. Bulk inserts can drastically reduce the number of transactions, minimizing delays and improving performance. Here’s how to effectively utilize bulk insert operations in SQL.
One of the most common methods for performing bulk inserts is using the BULK INSERT
command, which allows you to import a data file directly into a SQL Server table. This is especially useful when you have data in CSV or text file formats. The syntax for a basic bulk insert looks like this:
BULK INSERT your_table FROM 'C:pathtoyourdatafile.csv' WITH ( FIELDTERMINATOR = ',', ROWTERMINATOR = 'n', FIRSTROW = 2 -- Skip header row if applicable );
This command reads data from a specified file and inserts it into your_table
. The FIELDTERMINATOR
and ROWTERMINATOR
options allow you to define how fields and rows in the data file are separated. By setting FIRSTROW
to 2, you can skip the header row, which is often present in CSV files.
Another effective approach for bulk inserts is using the INSERT INTO ... SELECT
statement. This method is particularly useful when you want to import data from one table into another within the same database. For instance:
INSERT INTO target_table (column1, column2) SELECT column1, column2 FROM source_table WHERE condition;
This statement selects data from source_table
and inserts it directly into target_table
. This method is efficient as it leverages SQL Server’s ability to handle set-based operations, reducing the number of transactions required for the insert process.
For scenarios where you need to insert a large amount of data in batches, think using a loop combined with a transaction. This approach allows you to manage the volume of data being processed at any time and can help avoid locking issues.
DECLARE @BatchSize INT = 1000; DECLARE @RowCount INT = 1; WHILE @RowCount > 0 BEGIN BEGIN TRANSACTION; INSERT INTO your_table (column1, column2) SELECT TOP(@BatchSize) column1, column2 FROM source_table WHERE condition; SET @RowCount = @@ROWCOUNT; COMMIT TRANSACTION; END;
This loop will continue inserting data in batches of 1000 rows (or whatever batch size you specify) until no more rows are available to insert, ensuring that your database can handle the load efficiently.
Moreover, configuring your database to support bulk operations can yield even greater performance. Ensuring that the target table has appropriate indexes and constraints in place—without being overly restrictive—can lead to faster bulk insert operations. Additionally, think disabling constraints and indexes during the bulk insert process and re-enabling them afterward to further enhance performance:
ALTER TABLE your_table NOCHECK CONSTRAINT ALL; -- Disable constraints -- Perform your bulk insert operations here ALTER TABLE your_table CHECK CONSTRAINT ALL; -- Re-enable constraints
Finally, be mindful of your transaction log when performing bulk inserts. For large imports, you might want to switch your database to BULK_LOGGED
recovery model if it is not already set, which can help reduce the size of the transaction log during large operations:
ALTER DATABASE your_database SET RECOVERY BULK_LOGGED;
After completing your bulk insert operations, remember to restore the recovery model back to its original state if necessary.
By effectively using bulk insert operations, you can significantly streamline your data import processes, leading to faster imports and more efficient database management. The key is to understand the capabilities of your SQL environment and leverage the best practices outlined to achieve optimal performance.
Automating Data Import Processes
Automating data import processes can significantly reduce manual effort and enhance the accuracy and consistency of your data management operations. By implementing automation, you can streamline the workflow, minimize human errors, and ensure that data imports occur regularly and predictably. Here are some effective strategies to automate your data import processes using SQL and related tools.
1. **Scheduled Tasks**
One of the most simpler ways to automate data imports is to use scheduled tasks. Most database management systems (DBMS) offer built-in scheduling capabilities to run SQL scripts at specified intervals. For example, in SQL Server, you can create a SQL Server Agent job to execute your import scripts automatically. Here’s a simple example of how you might set up a job to run a script for importing data from a CSV file:
EXEC msdb.dbo.sp_add_job @job_name = N'ImportDataJob'; EXEC msdb.dbo.sp_add_jobstep @job_name = N'ImportDataJob', @step_name = N'ExecuteImportScript', @subsystem = N'TSQL', @command = N'BULK INSERT your_table FROM ''C:pathtoyourdatafile.csv'' WITH (FIELDTERMINATOR = '','', ROWTERMINATOR = ''n'')'; EXEC msdb.dbo.sp_add_schedule @schedule_name = N'DailyImportSchedule', @freq_type = 4, -- Daily @freq_interval = 1, @active_start_time = 090000; -- 9 AM EXEC msdb.dbo.sp_attach_schedule @job_name = N'ImportDataJob', @schedule_name = N'DailyImportSchedule'; EXEC msdb.dbo.sp_add_jobserver @job_name = N'ImportDataJob';
This configuration sets up a job that performs the bulk insert operation daily at 9 AM, eliminating the need for manual intervention.
2. **Using ETL Tools**
ETL (Extract, Transform, Load) tools are specifically designed for automating data import processes. These tools can connect to multiple data sources, perform necessary transformations, and load data into your target database seamlessly. Solutions such as Talend, Apache Nifi, or Microsoft SQL Server Integration Services (SSIS) provide uncomplicated to manage interfaces to schedule and manage your data imports effectively. You typically set up a workflow that defines the data source, any transformation logic, and the target destination.
3. **Database Triggers**
Another method for automation is to implement database triggers. Triggers can automatically respond to certain events within the database, such as the insertion of data into a staging table. For example, you might create an `AFTER INSERT` trigger that processes data as soon as it is inserted:
CREATE TRIGGER trg_AfterInsert ON staging_table AFTER INSERT AS BEGIN INSERT INTO target_table (column1, column2) SELECT column1, column2 FROM inserted; END;
This trigger ensures that whenever new data is added to the `staging_table`, it is immediately processed and transferred to the `target_table`.
4. **Scripting Languages**
You can leverage scripting languages such as Python, PowerShell, or Bash to automate your SQL data imports. These scripts can contain logic to handle file retrieval, data processing, and executing SQL commands. For instance, a Python script using the `pandas` library can read a CSV file and execute the necessary SQL commands to import the data:
import pandas as pd import pyodbc # Load data from CSV data = pd.read_csv('C:/path/to/your/datafile.csv') # Connect to the database conn = pyodbc.connect('Driver={SQL Server};' 'Server=your_server;' 'Database=your_database;' 'UID=user;' 'PWD=password;') # Write data to SQL table data.to_sql('your_table', conn, if_exists='append', index=False)
You can schedule this script using cron jobs or Windows Task Scheduler, allowing for automated and periodic imports.
5. **APIs for Dynamic Data Importing**
If your data sources support APIs, you can automate the import process by fetching data directly from these APIs at regular intervals. This ensures that you’re always working with the most current data. You would typically write a script that makes API calls, retrieves data, and then processes and imports it into your database, similar to the earlier example with Python.
By embracing automation in data import processes, you can enhance the efficiency and reliability of your data management operations. The combination of scheduled jobs, ETL tools, triggers, scripting languages, and APIs provides a robust framework for ensuring your data imports are timely, consistent, and accurate.