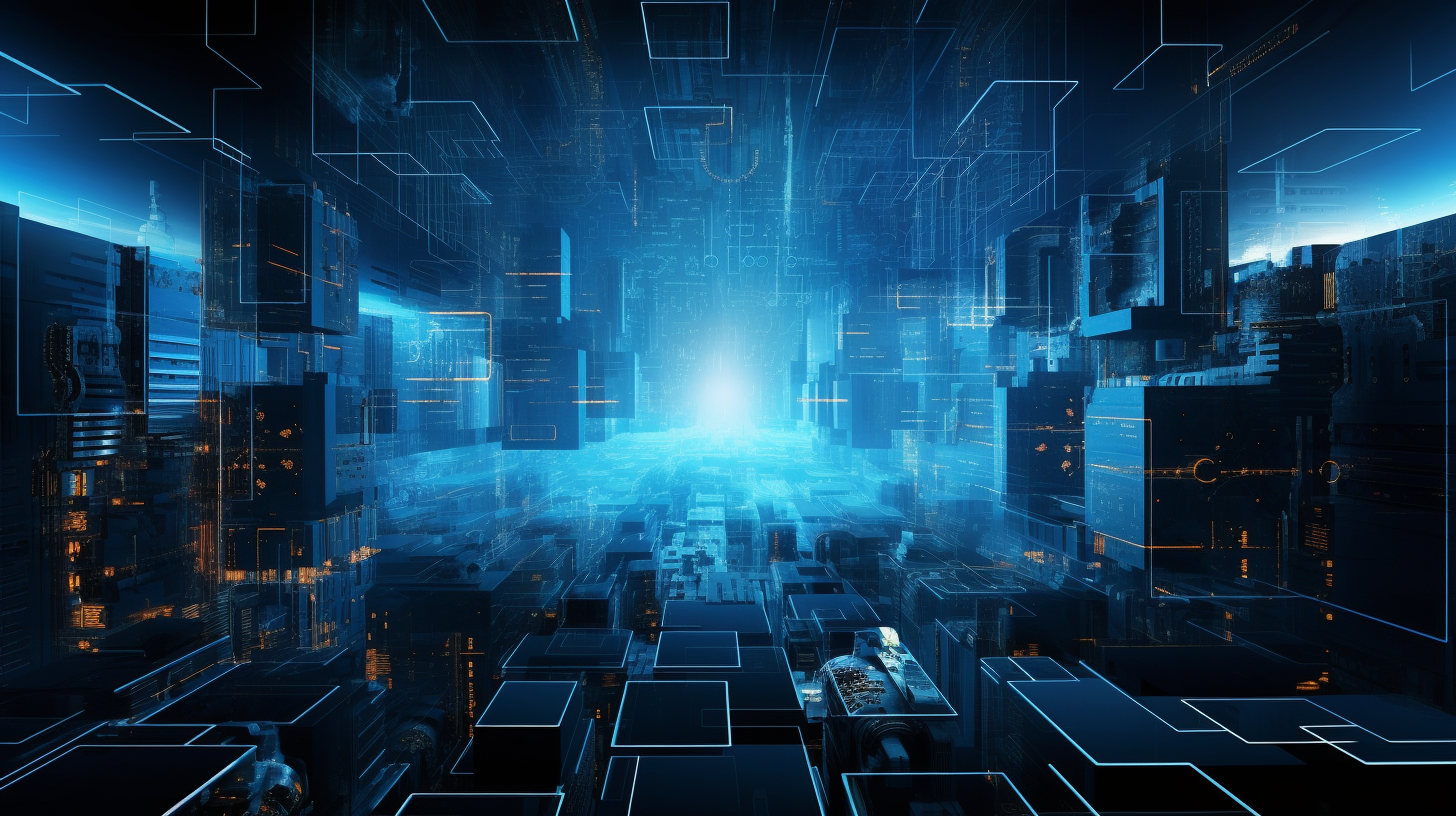
SQL Query Building for Dynamic Applications
Dynamic SQL refers to SQL statements that are constructed and executed at runtime rather than being statically defined in the code. This flexibility allows developers to create queries that can adapt to user input or various conditions, making it a powerful tool in scenarios where the exact SQL to be executed is not known until the program is running. By using dynamic SQL, developers can build more interactive and responsive applications.
One of the primary use cases for dynamic SQL is in applications that require user-driven data retrieval. For instance, consider a search feature in a web application where users can specify various criteria. A static SQL query would be cumbersome and impractical, as it would need to be designed to accommodate every possible combination of input. Instead, dynamic SQL allows for the creation of queries that can adjust based on the selection of parameters.
Here’s an example illustrating the construction of a dynamic SQL query in a scenario where a user may want to filter results based on optional criteria:
DECLARE @sql NVARCHAR(MAX) DECLARE @criteria NVARCHAR(100) = 'active' DECLARE @dateFrom DATE = NULL DECLARE @dateTo DATE = '2023-12-31' SET @sql = 'SELECT * FROM Users WHERE status = @criteria' IF @dateFrom IS NOT NULL BEGIN SET @sql = @sql + ' AND created_at >= @dateFrom' END IF @dateTo IS NOT NULL BEGIN SET @sql = @sql + ' AND created_at <= @dateTo' END EXEC sp_executesql @sql, N'@criteria NVARCHAR(100), @dateFrom DATE, @dateTo DATE', @criteria, @dateFrom, @dateTo
In this example, the SQL statement is built dynamically based on the presence of user input. The use of `sp_executesql` allows for parameterized execution, which can help in mitigating security risks while still enabling flexibility.
Another common use case is in the context of reporting applications where users can request reports based on various dimensions such as time, geography, or product categories. Dynamic SQL can help generate these reports by constructing the necessary queries based on user-selected options. This not only enhances user experience but also minimizes the need for multiple hard-coded queries.
However, with great power comes great responsibility. While dynamic SQL offers unmatched flexibility, it very important for developers to understand its implications and be aware of best practices. Learning to effectively manage these queries ensures that the application remains efficient, secure, and maintainable.
Best Practices for Building Dynamic SQL Queries
<= @dateTo' END
While dynamic SQL brings significant advantages, it is essential to adhere to best practices to ensure that the resulting queries are maintainable, secure, and performant. Here are some key practices to keep in mind:
1. Use Parameterized Queries: One of the most important rules in dynamic SQL is to always use parameters instead of concatenating user input directly into the SQL string. This mitigates the risk of SQL injection attacks, which can compromise the database’s integrity and security. Here’s how you can implement parameterization:
DECLARE @sql NVARCHAR(MAX) SET @sql = 'SELECT * FROM Users WHERE status = @criteria' EXEC sp_executesql @sql, N'@criteria NVARCHAR(100)', @criteria
2. Build Queries Incrementally: Instead of creating a single large SQL string, consider building your query incrementally, appending conditions only when necessary. This approach not only improves readability but also helps in understanding the query’s structure during debugging.
DECLARE @sql NVARCHAR(MAX) = 'SELECT * FROM Users WHERE 1=1' IF @criteria IS NOT NULL BEGIN SET @sql = @sql + ' AND status = @criteria' END
3. Limit the Use of Dynamic SQL: Dynamic SQL can introduce complexity into your code, making it harder to read and maintain. Use it judiciously; if a static query suffices, prefer it for better performance and clarity.
4. Consider Security Implications: Beyond SQL injection, be aware of other security concerns, such as exposing sensitive information through error messages. Employ proper error handling and logging to minimize risks.
5. Monitor Performance: Dynamic SQL can sometimes lead to performance issues due to the lack of proper query plans being cached. Regularly analyze the performance of your queries and ponder using stored procedures when appropriate, as they can provide better performance optimization opportunities.
6. Document Your Queries: Given that dynamic SQL can become complex, thorough documentation especially important. Comment your code extensively to explain the purpose of each section of the query, especially when building it conditionally.
By following these best practices, you can harness the power of dynamic SQL while minimizing associated risks and ensuring that your applications remain robust and efficient. The balance between flexibility and safety is delicate, but with careful consideration, you can navigate it successfully.
Handling User Input and Preventing SQL Injection
When dealing with dynamic SQL, one of the most critical considerations is how to safely handle user input. Users can inadvertently or maliciously supply input that could lead to SQL injection attacks, which occur when an attacker is able to manipulate the SQL query by injecting their own SQL code into the input fields. This can lead to unauthorized access to sensitive data or even complete database compromise.
To prevent SQL injection, one of the most effective strategies is to use parameterized queries or stored procedures. These approaches allow developers to define SQL commands with placeholders for user inputs, ensuring that the inputs are treated strictly as data and not executable code. This significantly reduces the risk of injection attacks.
Here’s an example of how to implement parameterized queries in dynamic SQL:
DECLARE @sql NVARCHAR(MAX); DECLARE @status NVARCHAR(100) = 'active'; DECLARE @dateFrom DATE = NULL; DECLARE @dateTo DATE = '2023-12-31'; SET @sql = 'SELECT * FROM Users WHERE status = @status'; IF @dateFrom IS NOT NULL BEGIN SET @sql = @sql + ' AND created_at >= @dateFrom'; END IF @dateTo IS NOT NULL BEGIN SET @sql = @sql + ' AND created_at <= @dateTo'; END EXEC sp_executesql @sql, N'@status NVARCHAR(100), @dateFrom DATE, @dateTo DATE', @status, @dateFrom, @dateTo;
In this example, the use of sp_executesql
allows us to execute a dynamically built SQL command while safely passing in parameters. The parameters are defined in the second argument, which helps ensure that any user input is treated as data rather than executable SQL.
Another technique to guard against SQL injection is to validate and sanitize user inputs rigorously. This can involve checking input types, applying length restrictions, and using allow-lists to limit permissible values. Such validation can help prevent unwanted characters from being processed in the SQL query.
Additionally, employing a web application firewall (WAF) can add another layer of protection against SQL injection attacks. WAFs are designed to detect and block malicious HTTP requests before they reach the application, thus serving as a frontline defense.
It’s also essential to monitor and log database interactions. Keeping a close watch on user inputs and executed queries can help identify unusual patterns or potential attacks, allowing for quick remediation.
Handling user input securely when building dynamic SQL queries requires a multifaceted approach, involving the use of parameterized queries, thorough input validation, and proactive monitoring. By implementing these practices, developers can protect their applications from SQL injection vulnerabilities and ensure the integrity of their database systems.
Optimizing Performance of Dynamic SQL Queries
SET @sql = @sql + ' AND created_at <= @dateTo'; END EXEC sp_executesql @sql, N'@criteria NVARCHAR(100), @dateFrom DATE, @dateTo DATE', @criteria, @dateFrom, @dateTo;
While the power of dynamic SQL is clear, so too are the potential pitfalls when it comes to performance. The inherent flexibility can lead to inefficiencies if not managed correctly. Here are several strategies for optimizing the performance of dynamic SQL queries.
1. Parameterization
One of the most effective ways to boost the performance of dynamic SQL is through parameterization. By using parameters instead of concatenating user input directly into your SQL strings, you not only improve performance but also enhance security by protecting against SQL injection. SQL Server can cache execution plans for parameterized queries, which means that repeated executions of the same query can benefit from the cached plan without the need for recompilation.
Think this revised example that uses parameters effectively:
DECLARE @sql NVARCHAR(MAX) DECLARE @criteria NVARCHAR(100) = 'active' DECLARE @dateFrom DATE = NULL DECLARE @dateTo DATE = '2023-12-31' SET @sql = 'SELECT * FROM Users WHERE status = @criteria' IF @dateFrom IS NOT NULL BEGIN SET @sql = @sql + ' AND created_at >= @dateFrom' END IF @dateTo IS NOT NULL BEGIN SET @sql = @sql + ' AND created_at <= @dateTo' END EXEC sp_executesql @sql, N'@criteria NVARCHAR(100), @dateFrom DATE, @dateTo DATE', @criteria, @dateFrom, @dateTo;
2. Avoiding Unnecessary Complexity
When crafting your dynamic SQL, it is essential to avoid unnecessary complexity that can lead to performance degradation. As a rule of thumb, keep your queries as simple as possible. Complex joins or nested queries that are dynamically constructed can result in significant overhead during execution. Strive for clarity and simplicity in your SQL logic.
3. Using Execution Plan Reuse
Execution plans can significantly impact the performance of your dynamic SQL. SQL Server leverages execution plan caching, but it can only do so effectively if the queries are structured to allow for plan reuse. Use consistent parameter types and ensure that dynamic SQL queries share similar structures to facilitate this reuse.
Additionally, think using the `OPTION (RECOMPILE)` hint judiciously, as it can lead to recompilation overhead. It may be beneficial for queries that change frequently, but should be avoided where possible to keep execution times low.
4. Monitoring Performance
Regular monitoring and profiling can provide insights into the performance of your dynamic SQL queries. Utilize SQL Server’s built-in tools, such as the SQL Server Profiler or Extended Events, to capture query performance metrics. Analyzing the execution plans of your dynamic queries can reveal slow operations and help identify areas for optimization.
For example, if a particular dynamic query shows up frequently in your monitoring tools with high resource usage, it may warrant a closer examination. Query tuning, such as adding appropriate indexes or rewriting inefficient joins, can yield significant performance improvements.
By adhering to these best practices for optimizing dynamic SQL, developers can ensure their applications are not only responsive and uncomplicated to manage but also performant and scalable.
Testing and Debugging Dynamic SQL in Applications
Within the scope of dynamic SQL, testing and debugging becomes a critical aspect of ensuring that the constructed queries function as intended and yield the desired results. Given the complexity and variability inherent in dynamic SQL, the challenges of effectively testing and debugging can be substantial, requiring a strategic approach to manage potential pitfalls.
One of the initial steps in testing dynamic SQL is to verify the correctness of the generated query. This involves isolating the SQL text being constructed and executing it independently to observe its behavior. By capturing the final SQL string before execution, developers can manually run it in a database management tool to assess its results and syntax.
PRINT @sql
Using the PRINT
statement allows developers to output the final form of the SQL command and examine it for any logical errors or issues with parameter insertion. That is especially useful when the dynamic query is long and complex, making it difficult to predict exactly how it will behave.
Debugging dynamic SQL also involves logging the results of executed queries. By capturing both the query execution and its outcomes, it becomes easier to trace the cause of errors or unexpected results. Implementing a logging mechanism can help you track which queries were run, along with their parameters and execution results.
Moreover, it’s essential to handle exceptions appropriately. Surrounding dynamic SQL executions with try-catch blocks can help in catching errors that arise during execution, allowing the inclusion of informative error messages that can lead to quicker resolutions.
BEGIN TRY EXEC sp_executesql @sql, N'@criteria NVARCHAR(100), @dateFrom DATE, @dateTo DATE', @criteria, @dateFrom, @dateTo END TRY BEGIN CATCH SELECT ERROR_MESSAGE() AS ErrorMessage; END CATCH
Another aspect of testing dynamic SQL is validating user inputs. Given that dynamic SQL often relies on user-generated data, ensuring that the input is sanitized and validated is important. This not only prevents SQL injection attacks but also helps to eliminate input-related errors that could lead to unexpected behavior during execution.
Unit testing frameworks can also be leveraged to automate the testing of dynamic SQL queries. By writing unit tests that validate the output of dynamic SQL against expected results, developers can ensure that changes in the codebase do not break existing functionality. Mocking frameworks can be particularly useful for simulating database responses and environments, enabling comprehensive testing without affecting the actual database.
Testing and debugging dynamic SQL queries require a multifaceted approach that includes validating generated SQL, logging execution results, and sanitizing user inputs. By implementing robust error handling and using automated testing tools, developers can streamline the process of ensuring the reliability and security of dynamic SQL within applications.