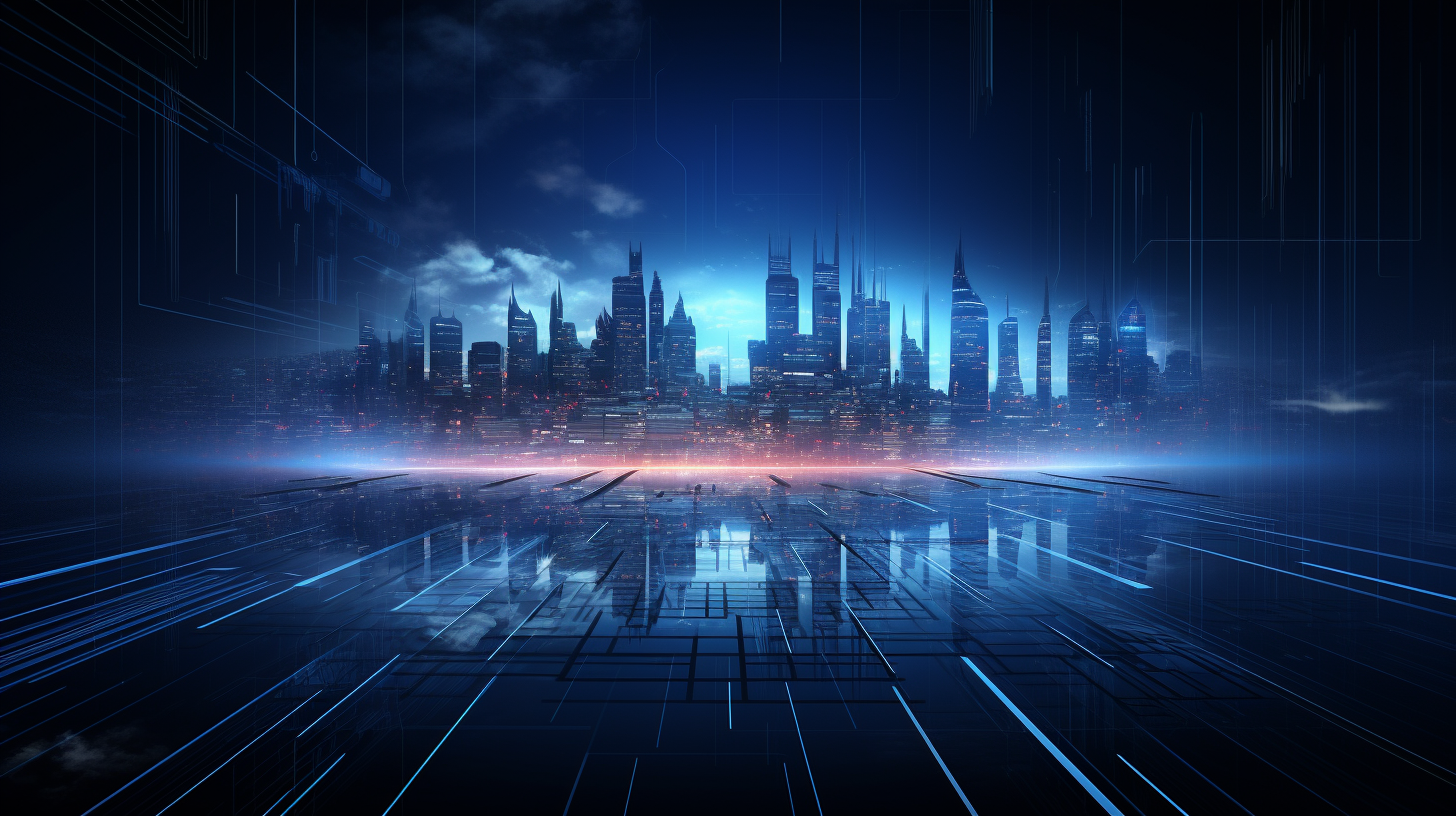
SQL Scripting for Database Automation
SQL scripting is a powerful method for automating database tasks that can significantly improve efficiency and accuracy in managing data. By using SQL scripts, database administrators and developers can execute a series of SQL commands in one go, rather than running them individually. This not only saves time but also ensures consistency in operations.
At its core, SQL scripting involves writing a sequence of SQL statements, which can include commands for creating, updating, or deleting database objects, as well as for manipulating data. Understanding the foundational elements of SQL scripting is important for using its full potential.
Here are the key components to grasp when diving into SQL scripting:
- The building blocks of any SQL script, these include
SELECT
,INSERT
,UPDATE
,DELETE
, and Data Definition Language (DDL) commands likeCREATE
andALTER
. - SQL scripts can include multiple SQL statements that are executed as a batch. This allows for the execution of complex operations in one go, improving performance.
- These statements such as
IF...ELSE
,WHILE
, andBEGIN...END
allow for conditional execution of SQL statements, which is vital for creating dynamic scripts. - You can declare and use variables in your scripts to store temporary data. That’s useful for managing state and passing values within your SQL operations.
Here’s an example of a simple SQL script that demonstrates some of these concepts:
DECLARE @CustomerID INT SET @CustomerID = 1 IF EXISTS (SELECT * FROM Customers WHERE CustomerID = @CustomerID) BEGIN PRINT 'Customer exists.' SELECT * FROM Customers WHERE CustomerID = @CustomerID END ELSE BEGIN PRINT 'Customer does not exist.' END
This script declares a variable @CustomerID
, checks for its existence in the Customers
table, and executes different SQL commands based on the result.
In addition to the basic components, understanding how to manage errors and handle transactions is essential for robust SQL scripting. Using transactions ensures that a series of operations can be committed as a single unit of work, or rolled back if any part of the operation fails, which maintains data integrity.
Here’s an example of a transaction in SQL:
BEGIN TRANSACTION BEGIN TRY INSERT INTO Orders (OrderDate, CustomerID) VALUES (GETDATE(), @CustomerID) COMMIT TRANSACTION END TRY BEGIN CATCH ROLLBACK TRANSACTION PRINT 'Error occurred while inserting order.' END CATCH
In this code, if the insertion into the Orders
table fails, the transaction is rolled back, and an error message is displayed.
By mastering these foundational concepts of SQL scripting, you lay the groundwork to develop sophisticated automated solutions that enhance database management and streamline operations.
Key Techniques for Database Automation
The next step in mastering SQL scripting for database automation is understanding the key techniques that can be employed to make your scripts more effective and reliable. These techniques not only improve the performance of your SQL scripts but also enhance their maintainability and scalability.
Dynamic SQL is one such technique that allows you to build SQL statements dynamically at runtime. This is particularly useful when you need to execute SQL commands based on user input or other variable data. Dynamic SQL can be implemented using the EXEC
or sp_executesql
commands. Here’s an example:
DECLARE @SQL NVARCHAR(MAX) SET @SQL = 'SELECT * FROM Customers WHERE Country = @Country' EXEC sp_executesql @SQL, N'@Country NVARCHAR(50)', @Country = 'USA'
In this script, we construct a SQL statement that selects customers based on their country. The use of a parameterized query with sp_executesql
not only protects against SQL injection but also improves performance by allowing the SQL Server to reuse the execution plan.
Using Stored Procedures is another effective technique for automation. Stored procedures encapsulate SQL scripts, allowing for encapsulation of logic, reusability, and improved performance. They can accept parameters, manage error handling, and can be executed with a single call, thus simplifying the execution of complex operations. Here’s how you can create a stored procedure:
CREATE PROCEDURE GetCustomerOrders @CustomerID INT AS BEGIN SELECT * FROM Orders WHERE CustomerID = @CustomerID END
Once the stored procedure is created, you can execute it using a simple command:
EXEC GetCustomerOrders @CustomerID = 1
Batch Processing is another cornerstone technique. Using transactions, as discussed previously, allows you to process batches of SQL commands together. This is beneficial when you want to ensure that a series of operations succeed or fail as a unit. Here’s an example of batch processing with error handling:
BEGIN TRANSACTION BEGIN TRY INSERT INTO Customers (CustomerName, ContactName) VALUES ('Cardinal', 'Tom B.') INSERT INTO Orders (OrderDate, CustomerID) VALUES (GETDATE(), SCOPE_IDENTITY()) COMMIT TRANSACTION END TRY BEGIN CATCH ROLLBACK TRANSACTION PRINT 'An error occurred: ' + ERROR_MESSAGE() END CATCH
In this case, we insert a new customer and then create an order for that customer within a single transaction. If an error occurs during either insertion, the entire transaction is rolled back, ensuring data integrity.
Automation with Scheduling can also enhance your SQL scripts. SQL Server Agent can be used to schedule jobs that run your scripts at defined intervals. That is particularly useful for routine maintenance tasks, such as backing up databases or cleaning up old data. Here’s a simple example of a SQL Server Agent job step:
EXEC sp_add_job @job_name = 'Backup Job' EXEC sp_add_jobstep @job_name = 'Backup Job', @step_name = 'Backup Database', @subsystem = 'TSQL', @command = 'BACKUP DATABASE MyDatabase TO DISK = ''C:BackupsMyDatabase.bak''' EXEC sp_add_jobserver @job_name = 'Backup Job'
This job backs up the database `MyDatabase` to a specified location on a schedule, automating an otherwise manual process. With the right scheduling setup, you can ensure that critical tasks are performed without the need for constant human intervention.
By adopting these key techniques—Dynamic SQL, Stored Procedures, Batch Processing, and Automation with Scheduling—you can significantly enhance the effectiveness of your SQL automation scripts. Each technique allows you to write cleaner, more efficient code that not only accomplishes tasks more quickly but also maintains the integrity and reliability of your database operations.
Best Practices for Writing SQL Scripts
Writing effective SQL scripts requires adherence to best practices that enhance readability, maintainability, and performance. When creating scripts, clarity and organization are paramount. You want your scripts to be easily understandable for anyone who might work with them in the future, including your future self. Here are some best practices to keep in mind:
1. Use Descriptive Naming Conventions:
Names for tables, columns, variables, and procedures should be intuitive and descriptive. This allows anyone reading the script to quickly grasp its purpose. For example, instead of naming a variable @x
, you might use @TotalSalesAmount
to convey its meaning directly.
DECLARE @TotalSalesAmount DECIMAL(10, 2)
2. Comment Your Code:
Adding comments throughout your script helps clarify the intent of complex operations. Use single-line comments (--
) for brief explanations, or multi-line comments (/.../
) for more detailed descriptions. This practice is invaluable for future maintenance and debugging.
-- Calculate total sales amount for a specific customer SELECT @TotalSalesAmount = SUM(Amount) FROM Sales WHERE CustomerID = @CustomerID
3. Structure Your Code for Readability:
Formatting your SQL code with consistent indentation and line breaks enhances readability. Break long SQL statements into multiple lines to avoid horizontal scrolling. Group related commands to visually separate sections of your script, making it easier to follow the logic.
SELECT CustomerName, ContactName FROM Customers WHERE Country = 'USA' ORDER BY CustomerName
4. Avoid Hardcoding Values:
Use parameters for values that may change, instead of hardcoding them into your scripts. This practice not only increases flexibility but also reduces the risk of errors when values need to be updated in multiple places.
CREATE PROCEDURE GetCustomerDetails @CustomerID INT AS BEGIN SELECT * FROM Customers WHERE CustomerID = @CustomerID END
5. Optimize Performance:
Pay attention to performance by avoiding unnecessary complexity. Use indexes wisely to enhance query performance, especially for large datasets. Analyze query execution plans to identify bottlenecks and optimize your queries accordingly.
CREATE INDEX IX_CustomerName ON Customers(CustomerName)
6. Handle Errors Gracefully:
Implement error handling to ensure that your scripts can cope with unexpected situations without causing data corruption or loss. Using TRY...CATCH
blocks in your scripts can help manage errors effectively.
BEGIN TRY -- Your SQL statement here END TRY BEGIN CATCH PRINT 'An error occurred: ' + ERROR_MESSAGE() END CATCH
7. Test Your Scripts Thoroughly:
Before deploying scripts in a production environment, conduct rigorous testing. Execute your scripts in a test database to ensure they function as expected and handle edge cases. This practice minimizes the risk of introducing errors into critical systems.
By adhering to these best practices, you can craft SQL scripts that not only fulfill their intended purpose but are also maintainable and efficient. This investment in quality will pay dividends in the long run, facilitating smoother operations and quicker adaptations in an ever-evolving data landscape.
Troubleshooting and Optimizing SQL Automation Scripts
Troubleshooting and optimizing SQL automation scripts is a critical skill for anyone involved in database management. No matter how well-written your scripts are, issues will arise. These may range from syntax errors to performance bottlenecks that can affect the overall efficiency of your database operations. Understanding how to diagnose and resolve issues is essential for maintaining smooth automation processes.
Identifying Errors
When a script fails to execute, the first step is to identify the error. SQL Server’s built-in error handling mechanisms, including the TRY…CATCH block, can be invaluable in capturing and reporting errors. This allows you to understand what went wrong without causing significant disruptions. Here’s a simple example:
BEGIN TRY -- Attempt to insert data into a table INSERT INTO Orders (OrderDate, CustomerID) VALUES (GETDATE(), @CustomerID) END TRY BEGIN CATCH PRINT 'Error occurred: ' + ERROR_MESSAGE() END CATCH
In this code, if an error occurs during the insertion, the CATCH block captures it and provides a meaningful error message. This feedback is essential for debugging.
Examining Execution Plans
After addressing immediate errors, the next focus should be on performance optimization. SQL Server provides execution plans, which offer insights into how your queries are executed. Analyzing these plans can help identify inefficient operations, such as table scans that can be replaced with index seeks, significantly improving performance.
SET STATISTICS IO ON; SET STATISTICS TIME ON; SELECT * FROM Orders WHERE CustomerID = @CustomerID
By enabling statistics, you can review the I/O and CPU time used by the query, allowing you to pinpoint inefficiencies.
Indexing Strategies
One common performance bottleneck can be traced back to the absence of proper indexes. When your queries are running slowly, think whether indexing can help. Indexes allow SQL Server to find data without scanning the entire table, which can drastically reduce query execution time. Here’s an example of creating an index:
CREATE INDEX IX_Orders_CustomerID ON Orders(CustomerID)
This index on the `CustomerID` column of the `Orders` table can significantly enhance lookup times for queries filtering on that column.
Batch Size Management
Another area to think is batch size management when performing bulk operations. If you attempt to process too many rows at the same time, you may encounter timeout errors or excessive resource consumption. Adjusting the batch size can lead to better performance and lower resource usage. Here’s an example of how to implement batch processing:
DECLARE @BatchSize INT = 1000 WHILE (1=1) BEGIN DELETE TOP (@BatchSize) FROM OldRecords WHERE RecordDate < DATEADD(YEAR, -1, GETDATE()) IF @@ROWCOUNT = 0 BREAK END
In this loop, records older than one year are deleted in manageable batches of 1000, ensuring that the operation doesn’t overwhelm the server.
Logging and Monitoring
Effective logging and monitoring are essential for proactive troubleshooting. Implement logging within your scripts to capture execution details and error messages. This information can be invaluable for understanding patterns over time and pinpointing recurring issues. You can create a log table and insert log entries like this:
INSERT INTO ExecutionLog (ExecutionTime, Message) VALUES (GETDATE(), 'Started processing orders')
By maintaining a detailed log, you can later analyze the performance and effectiveness of your automation scripts, leading to continuous improvement.
Regular Review and Refactoring
Finally, regular review and refactoring of your SQL scripts ensure they remain efficient as database structures and business needs evolve. Periodically revisit your scripts to eliminate redundancy, simplify complex logic, and update any deprecated functions. This practice not only keeps your automation running smoothly but also prepares your scripts for future enhancements.
By actively engaging in troubleshooting and optimization, you can ensure that your SQL automation scripts remain reliable and efficient, reducing downtime and improving the overall performance of your database systems.