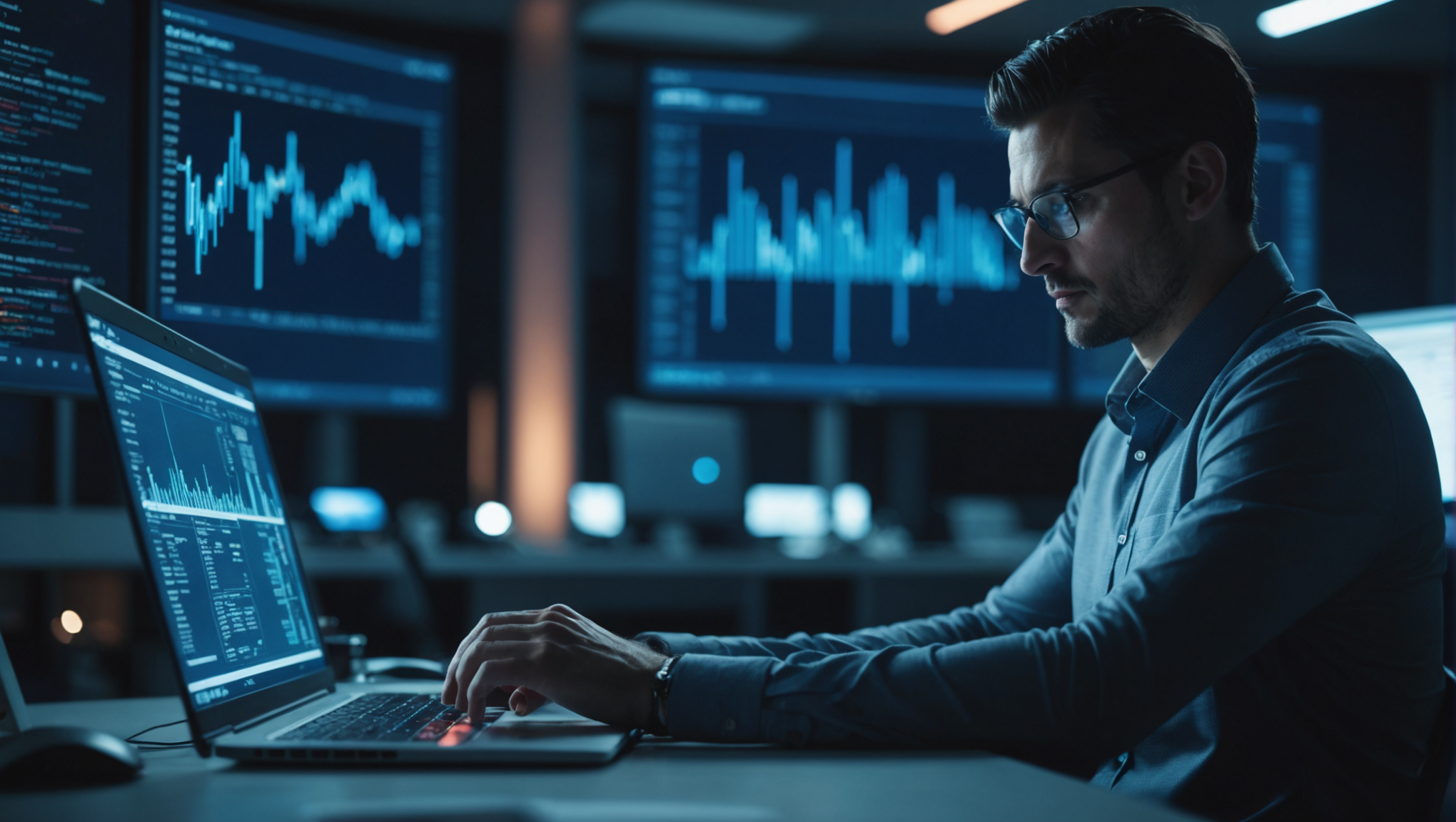
SQL Scripts for Data Maintenance
Data cleanup is an important aspect of maintaining the integrity and performance of your database system. Regularly cleaning up your database ensures that performance remains optimal, unnecessary data does not clutter your tables, and compliance with data governance policies is maintained. Below are some essential SQL scripts that can be employed for effective database cleanup.
1. Deleting Duplicate Rows
Duplicate rows can lead to inaccuracies in data querying and reporting. The following script identifies and removes duplicate entries from a table, keeping only one instance of each.
WITH CTE AS ( SELECT *, ROW_NUMBER() OVER (PARTITION BY column_name ORDER BY (SELECT NULL)) AS row_num FROM your_table_name ) DELETE FROM CTE WHERE row_num > 1;
2. Removing Unused or Old Data
Over time, databases can accumulate a lot of old or unused data that may no longer serve a purpose. This script removes records older than a specified date from a table.
DELETE FROM your_table_name WHERE date_column < DATEADD(year, -1, GETDATE());
3. Trimming Whitespace from Strings
Leading and trailing whitespace in character fields can lead to inconsistencies during data retrieval and matching. This script updates all affected rows to remove extra spaces.
UPDATE your_table_name SET column_name = LTRIM(RTRIM(column_name)) WHERE column_name IS NOT NULL;
4. Cleaning Up Null or Empty Values
Data integrity requires that unnecessary null or empty values be cleaned up. This script removes rows with null values in specified columns.
DELETE FROM your_table_name WHERE column_name IS NULL OR column_name = '';
5. Optimizing Table Size
After deletion operations, it’s beneficial to reclaim space. The following script shrinks the database file to optimize its size and improve performance.
DBCC SHRINKFILE (your_database_file, target_size_in_MB);
6. Archiving Data
For compliance and performance, periodically archiving historical data can significantly reduce the load on your active database. This script moves older data to an archive table.
INSERT INTO archive_table SELECT * FROM your_table_name WHERE date_column < DATEADD(year, -5, GETDATE()); DELETE FROM your_table_name WHERE date_column < DATEADD(year, -5, GETDATE());
The effective application of these SQL scripts can streamline your database cleanup processes, enhance performance, and maintain data integrity over time. Regular maintenance through these scripts not only boosts the efficiency of the database but also ensures that the data landscape remains organized and relevant.
Automating Data Backup and Recovery
Automating the processes of data backup and recovery is essential for safeguarding your database against data loss due to unexpected failures, human errors, or catastrophic events. SQL provides various methodologies to implement automated backup strategies that ensure your data remains intact and recoverable. Below are some examples of SQL scripts that facilitate the automation of data backup and recovery.
1. Full Database Backup
A full backup of your database especially important for a complete restoration in case of failure. The script below demonstrates how to schedule a full database backup using SQL Server Agent. This backup will be stored in a specified directory.
BACKUP DATABASE your_database_name TO DISK = 'C:Backupyour_database_name.bak' WITH FORMAT, INIT, SKIP, NOREWIND, NOUNLOAD, STATS = 10;
2. Transaction Log Backup
Backing up the transaction log is essential for databases using the full recovery model. It allows for granular recovery points. The following script schedules a transaction log backup.
BACKUP LOG your_database_name TO DISK = 'C:Backupyour_database_name_log.trn' WITH NOFORMAT, NOINIT, SKIP, NOREWIND, NOUNLOAD, STATS = 10;
3. Automating Backups with SQL Server Agent
To automate the backup process, you can create a SQL Server Agent job. Below is an example of how to create a job for full backups that will execute daily at midnight.
USE msdb; GO EXEC dbo.sp_add_job @job_name = N'Daily Full Backup'; EXEC dbo.sp_add_jobstep @job_name = N'Daily Full Backup', @step_name = N'Backup Database', @subsystem = N'TSQL', @command = N'BACKUP DATABASE your_database_name TO DISK = ''C:Backupyour_database_name.bak'' WITH INIT;', @retry_attempts = 5, @retry_interval = 5; EXEC dbo.sp_add_jobschedule @job_name = N'Daily Full Backup', @name = N'Daily Backup Schedule', @freq_type = 4, @freq_interval = 1, @freq_subday_type = 1, @freq_subday_interval = 0, @active_start_time = 000000; EXEC dbo.sp_add_jobserver @job_name = N'Daily Full Backup';
4. Restoring from Backup
In case of data loss, you can restore your database from the backup created. The following command restores the database from the full backup:
RESTORE DATABASE your_database_name FROM DISK = 'C:Backupyour_database_name.bak' WITH REPLACE;
5. Point-in-Time Restore
To perform a point-in-time restore, you must first restore the full backup and then the transaction log backups up to the specific point in time. Here’s how it can be achieved:
RESTORE DATABASE your_database_name FROM DISK = 'C:Backupyour_database_name.bak' WITH NORECOVERY; RESTORE LOG your_database_name FROM DISK = 'C:Backupyour_database_name_log.trn' WITH STOPAT = 'YYYY-MM-DD HH:MM:SS', RECOVERY;
Automating these backup and recovery processes through SQL scripts not only enhances data safety but also minimizes the risk of human error and downtime. By implementing a robust backup strategy, you can ensure that your data remains secure and recoverable, providing peace of mind in an increasingly data-driven world.
Managing Indexes and Performance Tuning
Managing indexes effectively is one of the most crucial aspects of performance tuning in SQL databases. Indexes can significantly enhance query performance by allowing the database engine to quickly locate and retrieve data without scanning entire tables. However, improper indexing can lead to performance degradation, unnecessary overhead, and increased maintenance costs. Below are some SQL scripts and strategies that can help manage indexes and optimize database performance.
1. Identifying Missing Indexes
The first step in optimizing indexing is identifying which indexes may be missing. SQL Server provides a dynamic management view (DMV) that can help identify these missing indexes. The following script retrieves a list of suggested indexes along with their potential impact on performance:
SELECT migs.avg_total_user_cost * migs.avg_user_impact * (migs.user_seeks + migs.user_scans) AS improvement_measure, mid.* FROM sys.dm_db_missing_index_group_stats migs INNER JOIN sys.dm_db_missing_index_groups mig ON migs.index_group_id = mig.index_group_id INNER JOIN sys.dm_db_missing_index_details mid ON mig.index_id = mid.index_id ORDER BY improvement_measure DESC;
2. Creating Indexes
Once you’ve identified the missing indexes, you can create them to improve query performance. The following script demonstrates how to create a simple non-clustered index:
CREATE NONCLUSTERED INDEX IX_ColumnName ON your_table_name (column_name);
For composite indexes, you can include multiple columns as follows:
CREATE NONCLUSTERED INDEX IX_Composite ON your_table_name (column1, column2);
3. Updating Statistics
Keeping statistics up-to-date is essential for query optimization. SQL Server uses statistics to make informed decisions about query plans. The following command updates statistics for a specific table:
UPDATE STATISTICS your_table_name;
To update statistics for all tables in the database, you can use:
EXEC sp_updatestats;
4. Dropping Unused Indexes
Just as adding indexes can improve performance, unnecessary indexes can hinder it. Using the following script, you can identify and drop indexes that are not being utilized:
SELECT OBJECT_NAME(i.object_id) AS TableName, i.name AS IndexName FROM sys.indexes AS i LEFT JOIN sys.dm_db_index_usage_stats AS s ON i.object_id = s.object_id AND i.index_id = s.index_id WHERE s.index_id IS NULL AND i.is_primary_key = 0 AND i.is_unique = 0;
To drop an unused index, use:
DROP INDEX IndexName ON your_table_name;
5. Monitoring Index Fragmentation
Regularly monitoring the fragmentation of indexes is vital for maintaining performance. You can check the fragmentation level using the following script:
SELECT OBJECT_NAME(object_id) AS TableName, index_id, name AS IndexName, avg_fragmentation_in_percent FROM sys.dm_db_index_physical_stats(DB_ID(), NULL, NULL, NULL, NULL) WHERE avg_fragmentation_in_percent > 10;
To rebuild a fragmented index, use:
ALTER INDEX IndexName ON your_table_name REBUILD;
For indexes with lower fragmentation, you can reorganize them instead:
ALTER INDEX IndexName ON your_table_name REORGANIZE;
By actively managing indexes and regularly employing performance tuning scripts, you can ensure your SQL database operates at peak efficiency. This not only enhances query performance but also optimizes the overall user experience, making it a vital part of your data maintenance strategy.
Data Validation and Integrity Checks
Data validation is an essential process that guarantees the accuracy and consistency of your data throughout its lifecycle. Ensuring integrity checks are in place helps in preventing bad data from affecting your operations, which is important for maintaining trust in your data-driven decisions. Below are some SQL scripts that demonstrate how to perform data validation and integrity checks effectively.
1. Checking for Data Type Violations
It is important to ensure that the data in your columns adheres to defined data types. The following script helps identify rows where a specific column violates its data type, such as attempting to store a string in a numeric column:
SELECT * FROM your_table_name WHERE TRY_CAST(numeric_column AS FLOAT) IS NULL AND numeric_column IS NOT NULL;
2. Ensuring Referential Integrity
Referential integrity ensures that relationships between tables remain consistent. The following script identifies orphaned rows in a child table that do not have corresponding entries in the parent table:
SELECT * FROM child_table c WHERE NOT EXISTS ( SELECT 1 FROM parent_table p WHERE p.id = c.parent_id );
3. Validating Unique Constraints
Uniqueness constraints are vital for ensuring that certain columns do not have duplicate values. This script finds duplicates in a specified column that should maintain uniqueness:
SELECT column_name, COUNT(*) FROM your_table_name GROUP BY column_name HAVING COUNT(*) > 1;
4. Verifying Data Completeness
Completeness checks verify that critical columns contain values. The following script identifies rows where required columns are null:
SELECT * FROM your_table_name WHERE required_column IS NULL;
5. Checking for Logical Errors
Logical validations ensure the data makes sense in context. The script below checks for logical inconsistencies, such as ensuring that end dates are after start dates:
SELECT * FROM your_table_name WHERE end_date < start_date;
6. Running Consistency Checks Across Tables
To ensure that the data across multiple tables remains consistent, you can perform consistency checks. The following script ensures that every product ID in an order table has a corresponding entry in the products table:
SELECT DISTINCT o.product_id FROM orders o LEFT JOIN products p ON o.product_id = p.id WHERE p.id IS NULL;
Implementing these SQL scripts for data validation and integrity checks can significantly contribute to maintaining a clean and reliable database. By regularly running these checks, you can catch data issues early, which not only improves the quality of your data but also enhances decision-making and operational efficiency. Regular integrity checks become a cornerstone of robust data governance.
Archiving Historical Data Efficiently
Archiving historical data efficiently is vital for maintaining an organized database while ensuring that older data does not impede the performance of active queries. As data accumulates over time, the active database can become bloated, leading to slower response times and increased maintenance overhead. Implementing a robust archiving strategy allows you to retain necessary historical data while keeping the active dataset lean and performant. Below are some SQL scripts and strategies for effective data archiving.
1. Creating an Archive Table
Before archiving data, you need to create an archive table that mirrors the structure of your active table. This ensures that you can easily move data without structural issues. Below is an example of how to create an archive table:
CREATE TABLE archive_table ( id INT PRIMARY KEY, column1 VARCHAR(255), column2 DATE, created_at DATETIME DEFAULT GETDATE() );
2. Moving Historical Data to the Archive Table
Once your archive table is set up, you can move historical data based on certain criteria, such as a date threshold. The following script demonstrates how to transfer data older than five years from the active table to the archive table:
INSERT INTO archive_table (id, column1, column2, created_at) SELECT id, column1, column2, created_at FROM your_table_name WHERE created_at < DATEADD(year, -5, GETDATE());
3. Deleting Archived Data from the Active Table
After successfully archiving the data, it is essential to remove the archived records from the active table to prevent data duplication and to free up space. The following script deletes the transferred records:
DELETE FROM your_table_name WHERE created_at < DATEADD(year, -5, GETDATE());
4. Automating the Archiving Process
To ensure that archiving occurs regularly, set up a scheduled task using SQL Server Agent. This task can automate the archiving process, which will allow you to define how frequently archiving should be performed. Below is an example of how to create a SQL Server Agent job for periodic archiving:
USE msdb; GO EXEC dbo.sp_add_job @job_name = N'Archive Old Data'; EXEC dbo.sp_add_jobstep @job_name = N'Archive Old Data', @step_name = N'Move Data to Archive', @subsystem = N'TSQL', @command = N' INSERT INTO archive_table (id, column1, column2, created_at) SELECT id, column1, column2, created_at FROM your_table_name WHERE created_at < DATEADD(year, -5, GETDATE()); DELETE FROM your_table_name WHERE created_at < DATEADD(year, -5, GETDATE());', @retry_attempts = 5, @retry_interval = 5; EXEC dbo.sp_add_jobschedule @job_name = N'Archive Old Data', @name = N'Weekly Archive Schedule', @freq_type = 4, @freq_interval = 1, @active_start_time = 010000; -- 1:00 AM
5. Indexing the Archive Table
To maintain query performance within the archive table, it’s essential to create indexes on frequently queried columns. The following script shows how to create an index on a common search column:
CREATE NONCLUSTERED INDEX IX_Archive_Column1 ON archive_table (column1);
6. Querying Archived Data
When you need to access archived data, you can do so with simpler SELECT queries against the archive table. This allows you to analyze historical trends without impacting the performance of your primary tables:
SELECT * FROM archive_table WHERE column1 = 'SomeValue';
Implementing these strategies for efficient data archiving not only improves performance but also ensures that you maintain easy access to historical data for reporting and compliance purposes. By establishing a systematic archiving process, you can enhance the overall health of your database while preserving the information that matters most.
Scheduled Tasks for Routine Maintenance
Scheduled tasks for routine maintenance are a vital component of database management, ensuring that your SQL environment remains healthy, performant, and secure. Automating these tasks minimizes the risk of human error and allows you to enforce consistent practices across your database systems. Below are several SQL scripts and strategies to implement scheduled tasks effectively.
1. Creating Maintenance Plans
SQL Server Management Studio (SSMS) allows you to create maintenance plans that group several maintenance tasks together. You can schedule these plans to run at specific intervals, simplifying management. Here’s an example of how to create a maintenance plan that includes backup and integrity checks:
EXEC msdb.dbo.sp_add_maintenance_plan @plan_name = N'Maintenance Plan', @description = N'Full backup and integrity checks', @schedule_id = '1', -- Daily @type = 1; -- Plan type: 1 = Standard
2. Automating Index Maintenance
To maintain performance, it’s essential to regularly rebuild or reorganize indexes. Below is a script that can be scheduled to rebuild fragmented indexes based on certain thresholds:
DECLARE @IndexID INT, @TableName NVARCHAR(255), @SQL NVARCHAR(MAX); DECLARE index_cursor CURSOR FOR SELECT i.index_id, OBJECT_NAME(i.object_id) AS TableName FROM sys.dm_db_index_physical_stats(DB_ID(), NULL, NULL, NULL, NULL) AS p JOIN sys.indexes AS i ON p.object_id = i.object_id AND p.index_id = i.index_id WHERE p.avg_fragmentation_in_percent > 30; -- Threshold for rebuilding OPEN index_cursor; FETCH NEXT FROM index_cursor INTO @IndexID, @TableName; WHILE @@FETCH_STATUS = 0 BEGIN SET @SQL = 'ALTER INDEX ' + QUOTENAME(OBJECT_NAME(@IndexID)) + ' ON ' + @TableName + ' REBUILD;'; EXEC sp_executesql @SQL; FETCH NEXT FROM index_cursor INTO @IndexID, @TableName; END CLOSE index_cursor; DEALLOCATE index_cursor;
3. Scheduling Database Integrity Checks
Routine integrity checks are crucial for identifying issues before they escalate. You can use the following script to create a job that runs DBCC CHECKDB on your database:
USE msdb; GO EXEC sp_add_job @job_name = N'Database Integrity Check'; EXEC sp_add_jobstep @job_name = N'Database Integrity Check', @step_name = N'Check Database Integrity', @subsystem = N'TSQL', @command = N'DBCC CHECKDB (''your_database_name'');', @retry_attempts = 5, @retry_interval = 5; EXEC sp_add_jobschedule @job_name = N'Database Integrity Check', @name = N'Daily Schedule', @freq_type = 4, @freq_interval = 1, @active_start_time = 020000; -- 2:00 AM EXEC sp_add_jobserver @job_name = N'Database Integrity Check';
4. Cleaning Up Old Backups
Over time, backup files can consume significant disk space. You can schedule a task to delete old backup files. The following script identifies and deletes backup files older than 30 days:
DECLARE @FileName NVARCHAR(255); DECLARE @Path NVARCHAR(255) = 'C:Backup'; -- Path to your backup files DECLARE file_cursor CURSOR FOR SELECT name FROM sys.master_files WHERE type_desc = 'FILE' AND name LIKE N'%.bak%'; OPEN file_cursor; FETCH NEXT FROM file_cursor INTO @FileName; WHILE @@FETCH_STATUS = 0 BEGIN EXEC xp_cmdshell 'DEL ' + @Path + @FileName + ' WHERE created_date < DATEADD(day, -30, GETDATE());'; FETCH NEXT FROM file_cursor INTO @FileName; END CLOSE file_cursor; DEALLOCATE file_cursor;
5. Monitoring SQL Server Performance
Regularly monitoring the performance of your SQL Server instance is important for identifying bottlenecks. You can schedule a job that runs performance metrics collection queries, as shown in the following example:
INSERT INTO performance_metrics (timestamp, cpu_usage, memory_usage) SELECT GETDATE(), (SELECT TOP 1 cpu_usage FROM sys.dm_os_performance_counters WHERE counter_name = 'Processor Time'), (SELECT TOP 1 total_physical_memory_kb FROM sys.dm_os_sys_memory);
By scheduling these tasks, you can ensure your database operates smoothly and efficiently. Regular maintenance through automated SQL scripts reduces the workload on database administrators while enhancing the overall health of your SQL environment, allowing for more time to focus on strategic initiatives rather than routine upkeep.