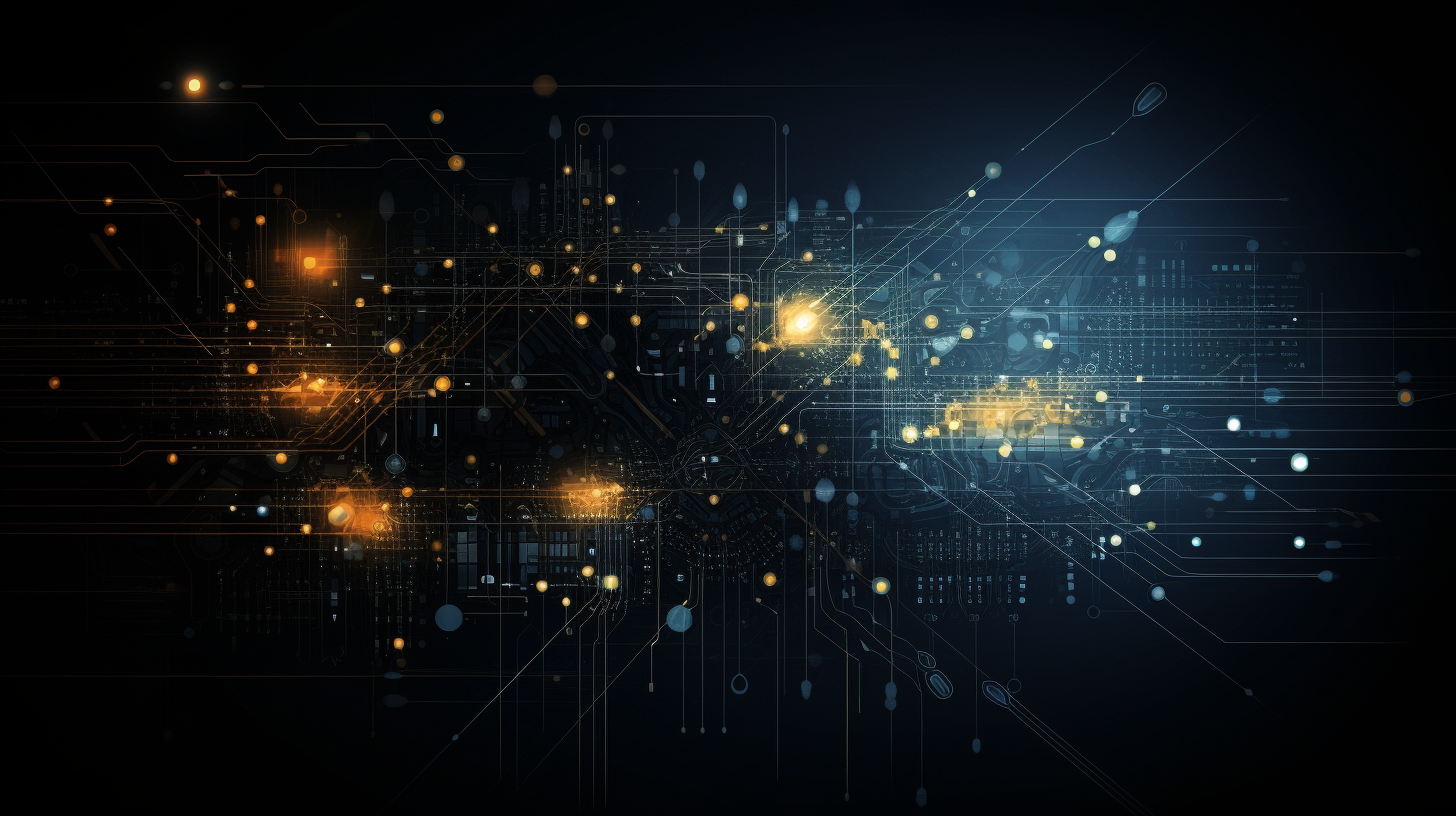
SQL Scripts for Database Backup
When it comes to safeguarding your data, understanding the different types of database backups is important. Backups can generally be categorized into a few primary types: full backups, incremental backups, and differential backups. Each type serves a unique purpose and has its own set of advantages and disadvantages.
- This type of backup captures the entire database at a specific point in time. It includes all data, schema, and stored procedures. Although full backups provide a complete snapshot, they can be time-consuming and require significant storage space. The basic SQL command for creating a full backup in SQL Server can look like this:
BACKUP DATABASE YourDatabaseName TO DISK = 'C:BackupsYourDatabaseName_Full.bak';
- Unlike full backups, incremental backups only capture changes made since the last backup, whether it was a full or incremental backup. This method is more efficient in terms of time and storage, but restoring data can take longer since multiple backup files may need to be applied. An example SQL command for creating an incremental backup can be shown as follows:
-- SQL Server does not support incremental backups directly; -- instead, you might achieve this through differential backups or transaction log backups.
- This type of backup captures all changes made since the last full backup. It is a middle ground between full and incremental backups, offering quicker restore times than incremental backups while requiring less storage than full backups. The SQL command for a differential backup is:
BACKUP DATABASE YourDatabaseName TO DISK = 'C:BackupsYourDatabaseName_Diff.bak' WITH DIFFERENTIAL;
- These backups capture the transaction log, which records all changes to the database. This allows for point-in-time recovery, meaning you can restore the database to any point before a failure occurred. The SQL command for a transaction log backup is:
BACKUP LOG YourDatabaseName TO DISK = 'C:BackupsYourDatabaseName_Log.trn';
Creating Full Database Backups
Creating a full database backup is a fundamental part of any data management strategy. It ensures that you have a complete snapshot of your database at a specific moment, enabling you to restore your entire database in the event of data loss or corruption. The process is simpler, but it is important to execute it properly to avoid pitfalls.
In SQL Server, the command to create a full backup is relatively simple. You can specify the database you want to back up, the destination where the backup will be stored, and additional options as needed. Below is an example of the SQL command used to perform a full database backup:
BACKUP DATABASE YourDatabaseName TO DISK = 'C:BackupsYourDatabaseName_Full.bak' WITH INIT, SKIP, NOREWIND, NOUNLOAD, STATS = 10;
In this command:
- YOURDatabaseName is the name of the database you wish to back up.
- TO DISK specifies the file path where the backup will be stored.
- WITH INIT indicates that the backup file should be overwritten if it already exists.
- SKIP allows you to bypass any existing backup set that may have the same name and sequence number.
- NOREWIND and NOUNLOAD are options that pertain to tape drives; they can be ignored for disk backups.
- STATS = 10 provides feedback every 10 percent of the backup process.
It’s also worth considering the timing of your full backups. Depending on the size of your database and the amount of traffic it experiences, you may want to schedule your full backups during off-peak hours. This helps minimize the impact on performance and ensures that the backup process completes in a timely manner.
Additionally, full backups can be part of a larger backup strategy that includes differential or incremental backups. By combining these methods, you can balance the need for complete data recovery with the efficiency of smaller, faster backups.
Once the full backup is created, you should regularly verify the integrity of the backup file. This can be done using the RESTORE VERIFYONLY command:
RESTORE VERIFYONLY FROM DISK = 'C:BackupsYourDatabaseName_Full.bak';
This command checks the backup file’s readability and confirms that it can be restored successfully. Regularly verifying your backups ensures that your data remains safe and recoverable in the event of a failure.
Implementing Incremental Backups
Implementing incremental backups ultimately comes down to understanding how to balance efficiency with safety. In SQL Server, while direct incremental backups aren’t supported in the same way as full backups, you can achieve similar results through transaction log backups, which only capture changes made since the last backup.
Incremental backups allow you to save time and storage space by only recording the modifications made after your last backup, whether it was a full or another incremental backup. This means that if you’ve just performed a full backup, your next step would be to set up transaction log backups. You can schedule these backups at frequent intervals, ensuring that your data remains secure with minimal storage overhead.
The SQL command to create a transaction log backup is as follows:
BACKUP LOG YourDatabaseName TO DISK = 'C:BackupsYourDatabaseName_Log.trn';
In this command:
- Replace this with the name of your database.
- Specifies where the backup file will be saved.
When implementing incremental backups using transaction logs, it’s essential to understand that these logs need to be backed up regularly to prevent unwanted growth, which can lead to performance issues or potential data loss if the log file fills up.
To automate the process, you might create a SQL Server Agent job that runs the backup command at specified intervals. This could be every hour, every few hours, or whatever suits your operational needs. Here’s an example of how you could set up the job in T-SQL:
EXEC msdb.dbo.sp_add_job @job_name = N'Incremental Backup Job'; EXEC msdb.dbo.sp_add_jobstep @job_name = N'Incremental Backup Job', @step_name = N'Backup Transaction Log', @subsystem = N'TSQL', @command = N'BACKUP LOG YourDatabaseName TO DISK = ''C:BackupsYourDatabaseName_Log.trn'';', @retry_attempts = 5, @retry_interval = 5; EXEC msdb.dbo.sp_add_schedule @schedule_name = N'Every Hour', @freq_type = 4, @freq_interval = 1, @freq_subday_type = 4, @freq_subday_interval = 1; EXEC msdb.dbo.sp_attach_schedule @job_name = N'Incremental Backup Job', @schedule_name = N'Every Hour'; EXEC msdb.dbo.sp_add_jobserver @job_name = N'Incremental Backup Job';
This script creates a job that performs your transaction log backup every hour, thus automating your incremental backup process. Remember that after restoring from a transaction log backup, you will need to restore the most recent full backup first, followed by any subsequent transaction log backups to bring your database to the desired point in time.
Automating Backup Processes with SQL Scripts
Automating the backup process is an essential step in maintaining database integrity and availability. SQL Server provides various tools and methods that allow database administrators to set up automated backup routines, ensuring that backups are performed consistently and without manual intervention. This not only reduces the risk of human error but also allows for compliance with organizational policies regarding data retention and recovery.
One of the most effective ways to automate your backup process is to use SQL Server Agent, a component of SQL Server that allows you to automate administrative tasks, including backups. By creating jobs that execute backup commands at predefined intervals, you can ensure that your backup strategy is both efficient and reliable.
Below is an example of how to create a SQL Server Agent job that automates the full backup of a database:
EXEC msdb.dbo.sp_add_job @job_name = N'Full Database Backup Job'; EXEC msdb.dbo.sp_add_jobstep @job_name = N'Full Database Backup Job', @step_name = N'Backup Full Database', @subsystem = N'TSQL', @command = N'BACKUP DATABASE YourDatabaseName TO DISK = ''C:BackupsYourDatabaseName_Full.bak'' WITH INIT, SKIP, NOREWIND, NOUNLOAD, STATS = 10;', @retry_attempts = 5, @retry_interval = 5; EXEC msdb.dbo.sp_add_schedule @schedule_name = N'Daily Backup Schedule', @freq_type = 1, -- Daily @freq_interval = 1, @active_start_time = 090000; -- 9 AM EXEC msdb.dbo.sp_attach_schedule @job_name = N'Full Database Backup Job', @schedule_name = N'Daily Backup Schedule'; EXEC msdb.dbo.sp_add_jobserver @job_name = N'Full Database Backup Job';
In this script:
- Specifies the name of the job. In this case, it is named ‘Full Database Backup Job’.
- Defines the name of the step that will execute the backup command.
- Contains the actual SQL command to perform the backup. The parameters used (INIT, SKIP, NOREWIND, NOUNLOAD, STATS) enhance the backup process as described earlier.
- Set to 1, meaning a daily schedule.
- Indicates that the job will start at 9 AM.
After executing this script, the SQL Server Agent will automatically run the backup job every day at the specified time, ensuring that your database is regularly backed up without requiring manual intervention.
For incremental backups, you can create a similar job that regularly backs up the transaction log, as shown in a previous example. This way, you can maintain a robust backup strategy that combines both full and incremental backups, allowing for comprehensive data recovery options.
It’s vital to monitor the success of your automated backups. SQL Server Agent provides logging features that can help you keep track of job execution results. You can configure alerts to notify you of any failures, ensuring that you can respond quickly to issues that may arise.
Additionally, think implementing a strategy to manage the backup files themselves. Regularly check the storage space available on your backup disk and set up a cleanup job that removes old backups, thus preventing the disk from filling up and potentially causing new backups to fail.
Restoring Databases from Backups
Restoring a database from backups is a critical operation that every database administrator must be prepared to execute. In scenarios where data loss occurs—be it due to hardware failure, accidental deletions, or malicious actions—the ability to restore your database is paramount. Understanding how to correctly execute restoration commands ensures that you can bring your database back to a usable state swiftly and efficiently.
There are several scenarios to consider when restoring a database. You might need to restore a full backup, a differential backup, or apply transaction logs to bring the database to a specific point in time. Each of these operations involves specific SQL commands that dictate how the restoration takes place.
To start with a full database restoration, the basic command in SQL Server is as follows:
RESTORE DATABASE YourDatabaseName FROM DISK = 'C:BackupsYourDatabaseName_Full.bak' WITH RECOVERY;
In this command:
- The name of the database you wish to restore.
- Specifies the backup file to be used for the restoration.
- Indicates that the database should be left in a functional state after the restoration is complete, allowing for immediate access.
In cases where differential backups have been performed, you will first restore the full backup and then the most recent differential backup, like so:
RESTORE DATABASE YourDatabaseName FROM DISK = 'C:BackupsYourDatabaseName_Full.bak' WITH NORECOVERY; RESTORE DATABASE YourDatabaseName FROM DISK = 'C:BackupsYourDatabaseName_Diff.bak' WITH RECOVERY;
Here, the first command restores the full backup and leaves the database in a state that can accept additional backups (using WITH NORECOVERY). The second command then restores the differential backup and completes the restoration process by using WITH RECOVERY.
If you’re dealing with transaction log backups, restoring requires a slightly different approach. After restoring the full backup, you need to apply each subsequent transaction log backup. The commands would look like this:
RESTORE DATABASE YourDatabaseName FROM DISK = 'C:BackupsYourDatabaseName_Full.bak' WITH NORECOVERY; RESTORE LOG YourDatabaseName FROM DISK = 'C:BackupsYourDatabaseName_Log1.trn' WITH NORECOVERY; RESTORE LOG YourDatabaseName FROM DISK = 'C:BackupsYourDatabaseName_Log2.trn' WITH RECOVERY;
In these commands:
- The first command restores the full backup, similar to before.
- The following commands restore transaction logs in the order they were created. Each log restoration maintains the NORECOVERY option until the last transaction log, which then uses WITH RECOVERY to bring the database online.
It’s essential to note that during the restoration process, the database will be offline and inaccessible. Therefore, it is prudent to carry out restoration during maintenance windows or when user impact can be minimized.
Furthermore, post-restoration verification is an important step. You should ensure that the database is operational and that data integrity is maintained. You can run checks against the database to confirm that all objects are in place and functioning correctly.